
Secure Your Spot in Our PCA Online Course Starting on April 02 (Click for More Info)

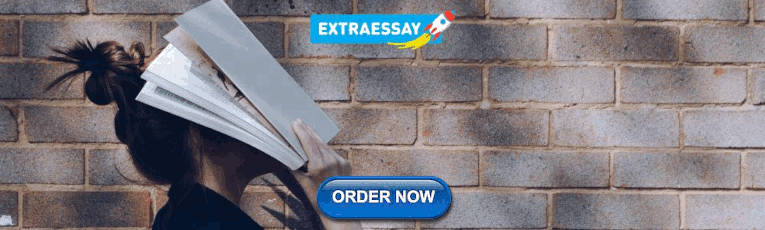
Assignment Operators in R (3 Examples) | Comparing = vs. <- vs. <<-
On this page you’ll learn how to apply the different assignment operators in the R programming language .
The content of the article is structured as follows:
Let’s dive right into the exemplifying R syntax!
Example 1: Why You Should Use <- Instead of = in R
Generally speaking, there is a preference in the R programming community to use an arrow (i.e. <-) instead of an equal sign (i.e. =) for assignment.
In my opinion, it makes a lot of sense to stick to this convention to produce scripts that are easy to read for other R programmers.
However, you should also take care about the spacing when assigning in R. False spacing can even lead to error messages .
For instance, the following R code checks whether x is smaller than minus five due to the false blank between < and -:
A properly working assignment could look as follows:
However, this code is hard to read, since the missing space makes it difficult to differentiate between the different symbols and numbers.
In my opinion, the best way to assign in R is to put a blank before and after the assignment arrow:
As mentioned before, the difference between <- and = is mainly due to programming style . However, the following R code using an equal sign would also work:
In the following example, I’ll show a situation where <- and = do not lead to the same result. So keep on reading!
Example 2: When <- is Really Different Compared to =
In this Example, I’ll illustrate some substantial differences between assignment arrows and equal signs.
Let’s assume that we want to compute the mean of a vector ranging from 1 to 5. Then, we could use the following R code:
However, if we want to have a look at the vector x that we have used within the mean function, we get an error message:
Let’s compare this to exactly the same R code but with assignment arrow instead of an equal sign:
The output of the mean function is the same. However, the assignment arrow also stored the values in a new data object x:
This example shows a meaningful difference between = and <-. While the equal sign doesn’t store the used values outside of a function, the assignment arrow saves them in a new data object that can be used outside the function.
Example 3: The Difference Between <- and <<-
So far, we have only compared <- and =. However, there is another assignment method we have to discuss: The double assignment arrow <<- (also called scoping assignment).
The following code illustrates the difference between <- and <<- in R. This difference mainly gets visible when applying user-defined functions .
Let’s manually create a function that contains a single assignment arrow:
Now, let’s apply this function in R:
The data object x_fun1, to which we have assigned the value 5 within the function, does not exist:
Let’s do the same with a double assignment arrow:
Let’s apply the function:
And now let’s return the data object x_fun2:
As you can see based on the previous output of the RStudio console, the assignment via <<- saved the data object in the global environment outside of the user-defined function.
Video & Further Resources
I have recently released a video on my YouTube channel , which explains the R syntax of this tutorial. You can find the video below:
The YouTube video will be added soon.
In addition to the video, I can recommend to have a look at the other articles on this website.
- R Programming Examples
In summary: You learned on this page how to use assignment operators in the R programming language. If you have further questions, please let me know in the comments.
assignment-operators-in-r How to use different assignment operators in R – 3 R programming examples – R programming language tutorial – Actionable R programming syntax in RStudio
Subscribe to the Statistics Globe Newsletter
Get regular updates on the latest tutorials, offers & news at Statistics Globe. I hate spam & you may opt out anytime: Privacy Policy .
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Post Comment

I’m Joachim Schork. On this website, I provide statistics tutorials as well as code in Python and R programming.
Statistics Globe Newsletter
Get regular updates on the latest tutorials, offers & news at Statistics Globe. I hate spam & you may opt out anytime: Privacy Policy .
Related Tutorials
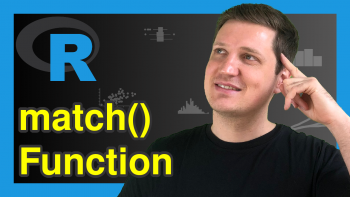
match Function in R (4 Example Codes)
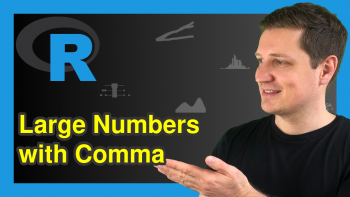
Display Large Numbers Separated with Comma in R (2 Examples)
assignOps: Assignment Operators
Assignment operators, description.
Assign a value to a name.
There are three different assignment operators: two of them have leftwards and rightwards forms.
The operators <- and = assign into the environment in which they are evaluated. The operator <- can be used anywhere, whereas the operator = is only allowed at the top level (e.g., in the complete expression typed at the command prompt) or as one of the subexpressions in a braced list of expressions.
The operators <<- and ->> are normally only used in functions, and cause a search to be made through parent environments for an existing definition of the variable being assigned. If such a variable is found (and its binding is not locked) then its value is redefined, otherwise assignment takes place in the global environment. Note that their semantics differ from that in the S language, but are useful in conjunction with the scoping rules of R . See ‘The R Language Definition’ manual for further details and examples.
In all the assignment operator expressions, x can be a name or an expression defining a part of an object to be replaced (e.g., z[[1]] ). A syntactic name does not need to be quoted, though it can be (preferably by backticks).
The leftwards forms of assignment <- = <<- group right to left, the other from left to right.
value . Thus one can use a <- b <- c <- 6 .
Becker, R. A., Chambers, J. M. and Wilks, A. R. (1988) The New S Language . Wadsworth & Brooks/Cole.
Chambers, J. M. (1998) Programming with Data. A Guide to the S Language . Springer (for = ).
assign (and its inverse get ), for “subassignment” such as x[i] <- v , see [<- ; further, environment .
R Package Documentation
Browse r packages, we want your feedback.

Add the following code to your website.
REMOVE THIS Copy to clipboard
For more information on customizing the embed code, read Embedding Snippets .
Assignment Operators in R
R provides two operators for assignment: <- and = .
Understanding their proper use is crucial for writing clear and readable R code.
Using the <- Operator
For assignments.
The <- operator is the preferred choice for assigning values to variables in R.
It clearly distinguishes assignment from argument specification in function calls.
Readability and Tradition
- This usage aligns with R’s tradition and enhances code readability.
Using the = Operator
The = operator is commonly used to explicitly specify named arguments in function calls.
It helps in distinguishing argument assignment from variable assignment.
Assignment Capability
- While = can also be used for assignment, this practice is less common and not recommended for clarity.
Mixing Up Operators
Potential confusion.
Using = for general assignments can lead to confusion, especially when reading or debugging code.
Mixing operators inconsistently can obscure the distinction between assignment and function argument specification.
- In the example above, x = 10 might be mistaken for a function argument rather than an assignment.
Best Practices Recap
Consistency and clarity.
Use <- for variable assignments to maintain consistency and clarity.
Reserve = for specifying named arguments in function calls.
Avoiding Common Mistakes
Be mindful of the context in which you use each operator to prevent misunderstandings.
Consistently using the operators as recommended helps make your code more readable and maintainable.
Quiz: Assignment Operator Best Practices
Which of the following examples demonstrates the recommended use of assignment operators in R?
- my_var = 5; mean(x = my_var)
- my_var <- 5; mean(x <- my_var)
- my_var <- 5; mean(x = my_var)
- my_var = 5; mean(x <- my_var)
- The correct answer is 3 . my_var <- 5; mean(x = my_var) correctly uses <- for variable assignment and = for specifying a named argument in a function call.
Popular Tutorials
Popular examples, learn python interactively, r introduction.
- R Reserved Words
- R Variables and Constants
R Operators
- R Operator Precedence and Associativitys
R Flow Control
- R if…else Statement
R ifelse() Function
- R while Loop
- R break and next Statement
- R repeat loop
- R Functions
- R Return Value from Function
- R Environment and Scope
- R Recursive Function
R Infix Operator
- R switch() Function
R Data Structures
- R Data Frame
R Object & Class
- R Classes and Objects
- R Reference Class
R Graphs & Charts
- R Histograms
- R Pie Chart
- R Strip Chart
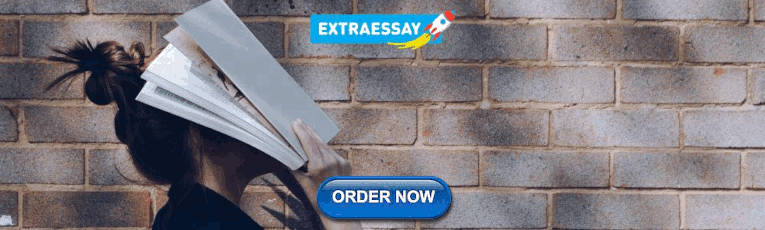
R Advanced Topics
- R Plot Function
- R Multiple Plots
- Saving a Plot in R
- R Plot Color
Related Topics
R Operator Precedence and Associativity
R Program to Add Two Vectors
In this article, you will learn about different R operators with the help of examples.
R has many operators to carry out different mathematical and logical operations. Operators perform tasks including arithmetic, logical and bitwise operations.
- Type of operators in R
Operators in R can mainly be classified into the following categories:
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- R Arithmetic Operators
These operators are used to carry out mathematical operations like addition and multiplication. Here is a list of arithmetic operators available in R.
Let's look at an example illustrating the use of the above operators:
- R Relational Operators
Relational operators are used to compare between values. Here is a list of relational operators available in R.
Let's see an example for this:
- Operation on Vectors
The above mentioned operators work on vectors . The variables used above were in fact single element vectors.
We can use the function c() (as in concatenate) to make vectors in R.
All operations are carried out in element-wise fashion. Here is an example.
When there is a mismatch in length (number of elements) of operand vectors, the elements in the shorter one are recycled in a cyclic manner to match the length of the longer one.
R will issue a warning if the length of the longer vector is not an integral multiple of the shorter vector.
- R Logical Operators
Logical operators are used to carry out Boolean operations like AND , OR etc.
Operators & and | perform element-wise operation producing result having length of the longer operand.
But && and || examines only the first element of the operands resulting in a single length logical vector.
Zero is considered FALSE and non-zero numbers are taken as TRUE . Let's see an example for this:
- R Assignment Operators
These operators are used to assign values to variables.
The operators <- and = can be used, almost interchangeably, to assign to variables in the same environment.
The <<- operator is used for assigning to variables in the parent environments (more like global assignments). The rightward assignments, although available, are rarely used.
Check out these examples to learn more:
- Add Two Vectors
- Take Input From User
- R Multiplication Table
Table of Contents
- Introduction
Sorry about that.
R Tutorials
Programming
Multiple assignment operators
Description.
Assign values to name(s).
%<-% and %->% invisibly return value .
These operators are used primarily for their assignment side-effect. %<-% and %->% assign into the environment in which they are evaluated.
Name Structure
At its simplest, the name structure may be a single variable name, in which case %<-% and %->% perform regular assignment, x %<-% list(1, 2, 3) or list(1, 2, 3) %->% x .
To specify multiple variable names use a call to c() , for example c(x, y, z) %<-% c(1, 2, 3) .
When value is neither an atomic vector nor a list, %<-% and %->% will try to destructure value into a list before assigning variables, see destructure() .
object parts
Like assigning a variable, one may also assign part of an object, c(x, x[[1]]) %<-% list(list(), 1) .
nested names
One can also nest calls to c() when needed, c(x, c(y, z)) . This nested structure is used to unpack nested values, c(x, c(y, z)) %<-% list(1, list(2, 3)) .
collector variables
To gather extra values from the beginning, middle, or end of value use a collector variable. Collector variables are indicated with a ... prefix, c(...start, z) %<-% list(1, 2, 3, 4) .
skipping values
Use . in place of a variable name to skip a value without raising an error or assigning the value, c(x, ., z) %<-% list(1, 2, 3) .
Use ... to skip multiple values without raising an error or assigning the values, c(w, ..., z) %<-% list(1, NA, NA, 4) .
default values
Use = to specify a default value for a variable, c(x, y = NULL) %<-% tail(1, 2) .
When assigning part of an object a default value may not be specified because of the syntax enforced by R . The following would raise an "unexpected '=' ..." error, c(x, x[[1]] = 1) %<-% list(list()) .
For more on unpacking custom objects please refer to destructure() .
double: Double-Precision Vectors
Description.
Create, coerce to or test for a double-precision vector.
single(length = 0) as.single(x, …)
A non-negative integer specifying the desired length. Double values will be coerced to integer: supplying an argument of length other than one is an error.
object to be coerced or tested.
further arguments passed to or from other methods.
double creates a double-precision vector of the specified length. The elements of the vector are all equal to 0 .
as.double attempts to coerce its argument to be of double type: like as.vector it strips attributes including names. (To ensure that an object is of double type without stripping attributes, use storage.mode .) Character strings containing optional whitespace followed by either a decimal representation or a hexadecimal representation (starting with 0x or 0X ) can be converted, as can special values such as "NA" , "NaN" , "Inf" and "infinity" , irrespective of case.
as.double for factors yields the codes underlying the factor levels, not the numeric representation of the labels, see also factor .
is.double returns TRUE or FALSE depending on whether its argument is of double type or not.
Double-precision values
All R platforms are required to work with values conforming to the IEC 60559 (also known as IEEE 754) standard. This basically works with a precision of 53 bits, and represents to that precision a range of absolute values from about \(2 \times 10^{-308}\) to \(2 \times 10^{308}\). It also has special values NaN (many of them), plus and minus infinity and plus and minus zero (although R acts as if these are the same). There are also denormal(ized) (or subnormal ) numbers with absolute values above or below the range given above but represented to less precision.
See .Machine for precise information on these limits. Note that ultimately how double precision numbers are handled is down to the CPU/FPU and compiler.
In IEEE 754-2008/IEC60559:2011 this is called ‘binary64’ format.
Note on names
It is a historical anomaly that R has two names for its floating-point vectors, double and numeric (and formerly had real ).
double is the name of the type . numeric is the name of the mode and also of the implicit class . As an S4 formal class, use "numeric" .
The potential confusion is that R has used mode "numeric" to mean ‘double or integer’, which conflicts with the S4 usage. Thus is.numeric tests the mode, not the class, but as.numeric (which is identical to as.double ) coerces to the class.
double creates a double-precision vector of the specified length. The elements of the vector are all equal to 0 . It is identical to numeric .
as.double is a generic function. It is identical to as.numeric . Methods should return an object of base type "double" .
is.double is a test of double type .
R has no single precision data type. All real numbers are stored in double precision format . The functions as.single and single are identical to as.double and double except they set the attribute Csingle that is used in the .C and .Fortran interface, and they are intended only to be used in that context.
Becker, R. A., Chambers, J. M. and Wilks, A. R. (1988) The New S Language . Wadsworth & Brooks/Cole.
https://en.wikipedia.org/wiki/IEEE_754-1985 , https://en.wikipedia.org/wiki/IEEE_754-2008 , https://en.wikipedia.org/wiki/Double_precision , https://en.wikipedia.org/wiki/Denormal_number .
http://754r.ucbtest.org/ for links to information on the standards.
integer , numeric , storage.mode .
Run the code above in your browser using DataLab
- book review
The difference between <- and = assignment in R
- May 5, 2020 April 5, 2021
When I started coding in R, a couple of years ago, I was stunned by the assignment operator. In most — if not all — coding languages I know, assignment is done through the equal sign ‘=’, while in R, I was taught to do it through the backarrow ‘<-‘. It didn’t take long until I realized the equal symbol also works. So… what is correct?
Let’s say we would like to assign the value 3 to the variable x. There’s multiple ways to do that.
The first five lines of code do exactly the same. The last one is slightly different. The assign function is the OG here: it assigns a value to a variable, and it even comes with more parameters that allow you to control which environment to save them in. By default, the variable gets stored in the environment it is being run in.
The arrows are respectively the leftwards assignment and the rightwards assignment. They simply are shortcuts for assign() . The double arrow in the last line is somewhat special. First, it checks if the variable already exists in the local environment, and if it doesn’t, it will store the variable in the global environment (.GlobalEnv). If you are unfamiliar with this, try reading up on scope in programming.
So why the arrow? Apparently, this is legacy from APL , a really old programming language. R (created in the early nineties) is actually a modern implementation of S (created in the mid-70’s), and S is heavily influenced by APL. APL was created on an Execuport, a now antique machine with a keyboard that had an “arrow” symbol, and it could be generated with one keystroke.
Historically, the = symbol was used to pass arguments to an expression. For example
In 2001, to bring assignment in R more in line with other programming languages, assignment using the = symbol was implemented. There are restrictions, however. According to the documentation , it can only be used at the top-level environment, or when isolated from the surrounding logical structure (e.g. in a for loop).
But this is not necessarily true. By putting brackets around an assignment, in places where intuitively it shouldn’t work, one can get around these restrictions. There’s probably not many use cases where you would want it, but it does work. In the following example, I calculate the mean of 3, 20 and 30 and at the same time assign 3 to x.
My personal advice is to use <- for assignment and = for passing arguments. When you mix things up, weird things can happen. This example from R Inferno produces two different results.
By the way, if two keystrokes really bug you. There’s a shortcut in RStudio to generate the arrow: Alt + -. Well yes, shortcut? You still need to press two keys ;-).
By the way, if you’re having trouble understanding some of the code and concepts, I can highly recommend “An Introduction to Statistical Learning: with Applications in R”, which is the must-have data science bible . If you simply need an introduction into R, and less into the Data Science part, I can absolutely recommend this book by Richard Cotton . Hope it helps!
Great success!
Say thanks, ask questions or give feedback
Technologies get updated, syntax changes and honestly… I make mistakes too. If something is incorrect, incomplete or doesn’t work, let me know in the comments below and help thousands of visitors.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Related Posts
Starting a remote selenium server in r.
- June 9, 2023 June 9, 2023
In this brief article, I explain how you can run a Selenium server, right from within your R code. This allows you to not manually…
How to set the package directory in R
- April 10, 2021 April 10, 2021
When I got my new company computer, out service desk installed R in a network folder, which made installing and loading R libraries extremely slow.…
Counting, adding or subtracting business days in R
- March 22, 2021 March 22, 2021
Calculating the number of days between two dates in R is as simple as using + or -. However, if you only want to count…
Dave Tang's blog
Computational biology and genomics
Double square brackets in R
This deserved its own post because I had some difficulty understanding the double square brackets in R. If we search for "double square brackets in R" we come across this tutorial , which shows us that the double square brackets, i.e. [[]], can be used to directly access columns:
More technically, the R manual states that one generally uses [[ to select any single element for lists and the [[ form allows only a single element to be selected using integer or character indices.
Take for example this list created following this tutorial :
I can see how the [[]]'s are quite useful when we need to reference elements within a list that have a list.
I would also like to point out that [[ can be used as a function. This is handy when you have a list within a list.
Sometimes the results returned from an analysis package is a list, which I want to convert to a data frame (say for merging reasons). Below is some code to obtain gene symbols for probe ids (as per my post on Using the Bioconductor annotation packages ) and how to convert this list into a data frame:
Conclusions
The double square brackets in R can be used to reference data frame columns, as shown with the iris dataset. An additional set of square brackets can be used in conjunction with the [[]] to reference a specific element in that vector of elements.
I can see that the [[]]'s are much more useful in lists that are structured as my_list in the example above. To flatten the list structure use the unlist() function, which can be useful when you want to convert a list into a data frame.
So how did a post on double square brackets in R turn into a post about lists? Initially, I thought I could use the double square brackets to refer to the elements in a list given a list structure (such as the results from using the annotation package). However I was unable to and the best solution seems to be flattening the list using unlist() and the use.names=F parameter.
The R Language Definition manual .
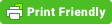
7 years later, this blog post continues to help anxious learners.
Also, I liked the way you began the blog. That it’s okay to face troubles with concepts that may seem so ordinary.
Really appreciate it!
Thanks for the comment! I’m glad the post is still useful 7 years down the road. And yes, it’s definitely OK to not understand something that may seem trivial!
Is there any way to get double square bracket semantics when creating a list? This: 1) L <- list("a"=1) Is the same as these two statements: 2) L<-list() L["a"] <- 1 Is there an assignment operator for form 1) that gives the equivalent behavior for the double bracket as-is assignment: L[["df"]] <- I(some_complex_obj_like_a_dataframe) ? Using "=" does not work and results in an embedding instead of the desired simple reference.
If you want to create a list of data frames, you can do something like this:
I'm guessing that's not what you want but I don't quite understand what you meant by results in an embedding rather than a simple reference. Did you want to store a specific column of the data frame?
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Currently you have JavaScript disabled. In order to post comments, please make sure JavaScript and Cookies are enabled, and reload the page. Click here for instructions on how to enable JavaScript in your browser.
This site uses Akismet to reduce spam. Learn how your comment data is processed .

R news and tutorials contributed by hundreds of R bloggers
Assignment in r: slings and arrows.
Posted on July 30, 2018 by Chris in R bloggers | 0 Comments
[social4i size="small" align="align-left"] --> [This article was first published on R – Doodling in Data , and kindly contributed to R-bloggers ]. (You can report issue about the content on this page here ) Want to share your content on R-bloggers? click here if you have a blog, or here if you don't.
Having recently shared my post about defensive programming in R on the r/rstats subreddit , I was blown away by the sheer number of comments as much as I was blown away by the insight many displayed. One particular comment by u/guepier struck my attention. In my previous post , I came out quite vehemently against using the = operator to effect assignment in R. u/guepier ‘s made a great point, however:
But point 9 is where you’re quite simply wrong, sorry: never, ever, ever use = to assign. Every time you do it, a kitten dies of sadness.
This is FUD, please don’t spread it. There’s nothing wrong with =. It’s purely a question of personal preference. In fact, if anything <- is more error-prone (granted, this is a very slight chance but it’s still higher than the chance of making an error when using =).
Now, assignment is no doubt a hot topic – a related issue, assignment expressions, has recently led to Python’s BDFL to be forced to resign –, so I’ll have to tread carefully. A surprising number of people have surprisingly strong feelings about assignment and assignment expressions. In R, this is complicated by its unusual assignment structure, involving two assignment operators that are just different enough to be trouble.
A brief history of <-
There are many ways in which <- in R is anomalous. For starters, it is rare to find a binary operator that consists of two characters – which is an interesting window on the R <- operator’s history.
The <- operator, apparently, stems from a day long gone by, when keyboards existed for the programming language eldritch horror that is APL . When R’s precursor, S, was conceived, APL keyboards and printing heads existed, and these could print a single ← character. It was only after most standard keyboard assignments ended up eschewing this all-important symbol that R and S accepted the digraphic <- as a substitute.
OK, but what does it do ?

Because quite peculiarly, there is another way to accomplish a simple assignment in R: the equality sign ( = ). And because on the top level, a <- b and a = b are equivalent, people have sometimes treated the two as being quintessentially identical. Which is not the case . Or maybe it is. It’s all very confusing. Let’s see if we can unconfuse it.
The Holy Writ
The Holy Writ, known to uninitiated as the R Manual , has this to say about assignment operators and their differences:
The operators – R Documentation: {base} assignOps
If this sounds like absolute gibberish, or you cannot think of what would qualify as not being on the top level or a subexpression in a braced list of expressions, welcome to the squad – I’ve had R experts scratch their head about this for an embarrassingly long time until they realised what the R documentation, in its neutron starlike denseness, actually meant.
If it’s in (parentheses) rather than {braces} , = and <- are going to behave weird
To translate the scriptural words above quoted to human speak, this means = cannot be used in the conditional part (the part enclosed by (parentheses) as opposed to {curly braces} ) of control structures, among others. This is less an issue between <- and = , and rather an issue between = and == . Consider the following example:
So far so good: you should not use a single equality sign as an equality test operator. The right way to do it is:
But what about arrow assignment?
Oh, look, it works! Or does it?
The problem is that an assignment will always yield true if successful. So instead of comparing x to 4, it assigned 4 to x , then happily informed us that it is indeed true.
The bottom line is not to use = as comparison operator, and <- as anything at all in a control flow expression’s conditional part. Or as John Chambers notes,
Disallowing the new assignment form in control expressions avoids programming errors (such as the example above) that are more likely with the equal operator than with other S assignments. – John Chambers, Assignments with the = Operator
Chain assignments
One example of where <- and = behave differently (or rather, one behaves and the other throws an error) is a chain assignment. In a chain assignment, we exploit the fact that R assigns from right to left. The sole criterion is that all except the rightmost members of the chain must be capable of being assigned to.
So we’ve seen that as long as the chain assignment is logically valid, it’ll work fine, whether it’s using <- or = . But what if we mix them up?
The bottom line from the example above is that where <- and = are mixed, the leftmost assignment has to be carried out using = , and cannot be by <- . In that one particular context, = and <- are not interchangeable.
A small note on chain assignments: many people dislike chain assignments because they’re ‘invisible’ – they literally return nothing at all. If that is an issue, you can surround your chain assignment with parentheses – regardless of whether it uses <- , = or a (valid) mixture thereof:
Assignment and initialisation in functions
This is the big whammy – one of the most important differences between <- and = , and a great way to break your code. If you have paid attention until now, you’ll be rewarded by, hopefully, some interesting knowledge.
= is a pure assignment operator. It does not necessary initialise a variable in the global namespace. <- , on the other hand, always creates a variable, with the lhs value as its name, in the global namespace. This becomes quite prominent when using it in functions.
Traditionally, when invoking a function, we are supposed to bind its arguments in the format parameter = argument. 1 And as we know from what we know about functions, the keyword’s scope is restricted to the function block. To demonstrate this:
This is expected: a (as well as b , but that didn’t even make it far enough to get checked!) doesn’t exist in the global scope, it exists only in the local scope of the function add_up_numbers . But what happens if we use <- assignment?
Now, a and b still only exist in the local scope of the function add_up_numbers . However, using the assignment operator, we have also created new variables called a and b in the global scope. It’s important not to confuse it with accessing the local scope, as the following example demonstrates:
In other words, a + b gave us the sum of the values a and b had in the global scope. When we invoked add_up_numbers(c <- 5, d <- 6) , the following happened, in order:
- A variable called c was initialised in the global scope. The value 5 was assigned to it.
- A variable called d was initialised in the global scope. The value 6 was assigned to it.
- The function add_up_numbers() was called on positional arguments c and d.
- c was assigned to the variable a in the function’s local scope.
- d was assigned to the variable b in the function’s local scope.
- The function returned the sum of the variables a and b in the local scope.
It may sound more than a little tedious to think about this function in this way, but it highlights three important things about <- assignment:
- In a function call, <- assignment to a keyword name is not the same as using = , which simply binds a value to the keyword.
- <- assignment in a function call affects the global scope, using = to provide an argument does not.
- Outside this context, <- and = have the same effect, i.e. they assign, or initialise and assign, in the current scope.
Phew. If that sounds like absolute confusing gibberish, give it another read and try playing around with it a little. I promise, it makes some sense. Eventually.
So… should you or shouldn’t you?
Which raises the question that launched this whole post: should you use = for assignment at all? Quite a few style guides, such as Google’s R style guide, have outright banned the use of = as assignment operator , while others have encouraged the use of -> . Personally, I’m inclined to agree with them , for three reasons.
- Because of the existence of -> , assignment by definition is best when it’s structured in a way that shows what is assigned to which side. a -> b and b <- a have a formal clarity that a = b does not have.
- Good code is unambiguous even if the language isn’t. This way, -> and <- always mean assignment, = always means argument binding and == always means comparison.
- Many argue that <- is ambiguous, as x<-3 may be mistyped as x<3 or x-3 , or alternatively may be (visually) parsed as x < -3 , i.e. compare x to -3. In reality, this is a non-issue. RStudio has a built-in shortcut ( Alt/⎇ + – ) for
Like with all coding standards, consistency is key. Consistently used suboptimal solutions are superior, from a coding perspective, to an inconsistent mixture of right and wrong solutions.
References [ + ]
The post Assignment in R: slings and arrows appeared first on Doodling in Data .
To leave a comment for the author, please follow the link and comment on their blog: R – Doodling in Data . R-bloggers.com offers daily e-mail updates about R news and tutorials about learning R and many other topics. Click here if you're looking to post or find an R/data-science job . Want to share your content on R-bloggers? click here if you have a blog, or here if you don't.
Copyright © 2022 | MH Corporate basic by MH Themes
Never miss an update! Subscribe to R-bloggers to receive e-mails with the latest R posts. (You will not see this message again.)
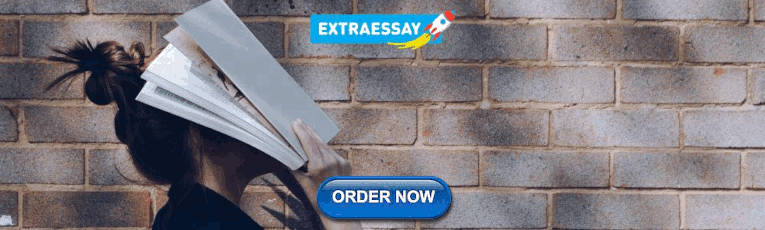
IMAGES
VIDEO
COMMENTS
It helps to think of <<-as equivalent to assign (if you set the inherits parameter in that function to TRUE).The benefit of assign is that it allows you to specify more parameters (e.g. the environment), so I prefer to use assign over <<-in most cases.. Using <<-and assign(x, value, inherits=TRUE) means that "enclosing environments of the supplied environment are searched until the variable 'x ...
On this page you'll learn how to apply the different assignment operators in the R programming language. The content of the article is structured as follows: 1) Example 1: Why You Should Use <- Instead of = in R. 2) Example 2: When <- is Really Different Compared to =. 3) Example 3: The Difference Between <- and <<-. 4) Video ...
Using double assignment in R closures. The differences between the functions's environment and the global environment will be clearer
Details. There are three different assignment operators: two of them have leftwards and rightwards forms. The operators <- and = assign into the environment in which they are evaluated. The operator <- can be used anywhere, whereas the operator = is only allowed at the top level (e.g., in the complete expression typed at the command prompt) or ...
4. It almost means global assignment (see whuber's comment, and the linked docs on scoping). So if you assign A the value of 2 using A <<- 2 within, for example, a function, and then call that function, other functions and the command line can then use the value of A. This has to do with the concept of scoping, and there are particulars of R's ...
There are three different assignment operators: two of them have leftwards and rightwards forms. The operators <- and = assign into the environment in which they are evaluated. The operator <- can be used anywhere, whereas the operator = is only allowed at the top level (e.g., in the complete expression typed at the command prompt) or as one of ...
For Assignments. The <- operator is the preferred choice for assigning values to variables in R. It clearly distinguishes assignment from argument specification in function calls. # Correct usage of <- for assignment x <- 10 # Correct usage of <- for assignment in a list and the = # operator for specifying named arguments my_list <- list (a = 1 ...
Double Check == and =: In R, == is a logical operator for comparison, while = can be used for assignment (though <- is more conventional). Ensure you're using the right one for the right task. Avoid Common Pitfalls with Logical Operators: Remember that & and | are element-wise logical operators, while && and || evaluate the first element of a ...
The <<-operator is used for assigning to variables in the parent environments (more like global assignments). The rightward assignments, although available, are rarely used. x <- 5 x x <- 9 x 10 -> x x. Output [1] 5 [1] 9 [1] 10. Check out these examples to learn more: Add Two Vectors; Take Input From User; R Multiplication Table
@ClarkThomborson The semantics are fundamentally different because in R assignment is a regular operation which is performed via a function call to an assignment function. However, this is not the case for = in an argument list. In an argument list, = is an arbitrary separator token which is no longer present after parsing. After parsing f(x = 1), R sees (essentially) call("f", 1).
It's the 'superassignment' operator. It does the assignment in the. enclosing environment. That is, starting with the enclosing frame, it. variable called ecov_xy, and then assigns to it. If it never finds. an existing ecov_xy it creates one in the global environment. that modify the state by using superassignment. a<-0.
At its simplest, the name structure may be a single variable name, in which case %<-% and %->% perform regular assignment, x. %<-% list(1, 2, 3) or list(1, 2, 3) %->% x . To specify multiple variable names use a call to c(), for example c(x, y, z) %<-% c(1, 2, 3) . When value is neither an atomic vector nor a list, %<-% and %->% will try to ...
double creates a double-precision vector of the specified length. The elements of the vector are all equal to 0 . It is identical to numeric. as.double is a generic function. It is identical to as.numeric. Methods should return an object of base type "double". is.double is a test of double type. R has no single precision data type.
The double arrow in the last line is somewhat special. First, it checks if the variable already exists in the local environment, and if it doesn't, it will store the variable in the global environment (.GlobalEnv). ... In 2001, to bring assignment in R more in line with other programming languages, assignment using the = symbol was ...
The Google R style guide prohibits the use of "=" for assignment. Hadley Wickham's style guide recommends "<-". If you want your code to be compatible with S-plus you should use "<-". I believe that the General R community recommend using "<-", but I can't find anything on the mailing list. However, I tend always use the ...
When logical OR is used in assignment, it typically means "if this first this is truthy, assign it to the variable, else assign the second thing. So it probably means" if hi is truthy, assign it, else assign abs (x [1L])". Disclaimer: I don't know r. If this is correct, Python can do this like: x = falsyVal or truthyVal.
Until 2001, in R, = could only be used for assigning function arguments, like fun(foo = "bar") (remember that R was born in 1993). So before 2001, the <-was the standard (and only way) to assign value into a variable. Before that, _ was also a valid assignment operator. It was removed in R 1.8:
The double square brackets in R can be used to reference data frame columns, as shown with the iris dataset. An additional set of square brackets can be used in conjunction with the [ []] to reference a specific element in that vector of elements. I can see that the [ []]'s are much more useful in lists that are structured as my_list in the ...
2. Another point is that <- makes it easier keep track of object names. If you're writing expressions that end in -> for assignment, your object names will be horizontally scattered, where as consistently using <- means each object name can be predictably located. - Mako212. Jul 26, 2018 at 22:53.
Having recently shared my post about defensive programming in R on the r/rstats subreddit, I was blown away by the sheer number of comments as much as I was blown away by the insight many displayed. One particular comment by u/guepier struck my attention. In my previous post, I came out quite vehemently against using theRead more The post Assignment in R: slings and arrows appeared first on ...
R: assignment using values from several rows. 0. Reassign variables to elements of list. 2. assign the same value to multiple variables. 2. How can I simultaneously assign value to multiple new columns with R and dplyr? 2. Applying the assign function over a list/vector of desired variable names. 1.