"Hello World!" in C Easy C (Basic) Max Score: 5 Success Rate: 85.68%
Playing with characters easy c (basic) max score: 5 success rate: 84.24%, sum and difference of two numbers easy c (basic) max score: 5 success rate: 94.58%, functions in c easy c (basic) max score: 10 success rate: 95.96%, pointers in c easy c (basic) max score: 10 success rate: 96.54%, conditional statements in c easy c (basic) max score: 10 success rate: 96.94%, for loop in c easy c (basic) max score: 10 success rate: 93.66%, sum of digits of a five digit number easy c (basic) max score: 15 success rate: 98.65%, bitwise operators easy c (basic) max score: 15 success rate: 94.87%, printing pattern using loops medium c (basic) max score: 30 success rate: 95.90%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- C Hello World Program
- C Program For Bubble Sort
- Structure of the C Program
- Output of C Program | Set 29
- C++ Programming Examples
- Output of C Program | Set 21
- Output of C Program | Set 19
- Output of C Programs | Set 2
- Output of C Programs | Set 3
- Output of C Programs | Set 1
- Output of C Program | Set 22
- Output of C Programs | Set 4
- Output of C Program | Set 18
- Output of C Program | Set 28
- Output of C Programs | Set 5
- Output of C Programs | Set 6
- Output of C Program | Set 20
- Output of C Programs | Set 9
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Bitwise Operators in C
- std::sort() in C++ STL
- What is Memory Leak? How can we avoid?
- Substring in C++
- Segmentation Fault in C/C++
- Socket Programming in C
- For Versus While
- Data Types in C
C Programs : Practicing and solving problems is the best way to learn anything. Here, we have provided 100+ C programming examples in different categories like basic C Programs, Fibonacci series in C, String, Array, Base Conversion, Pattern Printing, Pointers, etc. These C programs are the most asked interview questions from basic to advanced level.
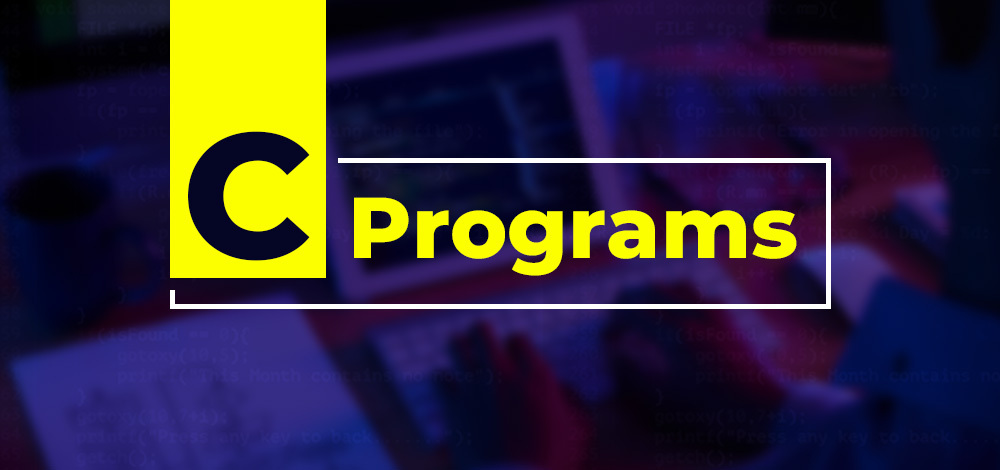
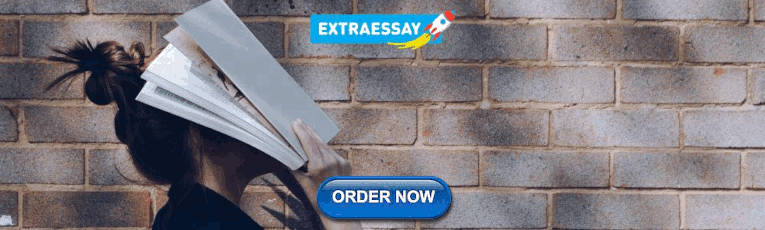
C Program Topics :
- Basic C Programs
- Control Flow Programs
- Pattern Printing Programs
- Functions Programs
- Arrays Programs
- Strings Programs
- Conversions Programs
- Pointers Programs
- Structures and Unions Programs
- File I/O Programs
- Date and Time Programs
- More C Programs
C Program – Basic
- C Program to Print Your Own Name
- C Program to Print an Integer Entered By the User
- C Program to Add Two Numbers
- C Program to Check Whether a Number is Prime or Not
- C Program to Multiply two Floating-Point Numbers
- C Program to Print the ASCII Value of a Character
- C Program to Swap Two Numbers
- C Program to Calculate Fahrenheit to Celsius
- C Program to Find the Size of int, float, double, and char
- C Program to Add Two Complex Numbers
- C Program to Print Prime Numbers From 1 to N
- C Program to Find Simple Interest
- C Program to Find Compound Interest
- C Program for Area And Perimeter Of Rectangle
C Program – Control Flow
- C Program to Check Whether a Number is Positive, Negative, or Zero
- C Program to Check Whether Number is Even or Odd
- C Program to Check Whether a Character is Vowel or Consonant
- C Program to Find Largest Number Among Three Numbers
- C Program to Calculate Sum of Natural Numbers
- C Program to Print Alphabets From A to Z Using Loop
- C Program to Check Leap Year
- C Program to Find Factorial of a Number
- C Program to Make a Simple Calculator
- C Program to Generate Multiplication Table
- C Program to Print Fibonacci Series
- C Program to Find LCM of Two Numbers
- C Program to Check Armstrong Number
- C Program to Display Armstrong Numbers Between 1 to 1000
- C Program to Display Armstrong Number Between Two Intervals
- C Program to Reverse a Number
- C Program to Check Whether a Number is a Palindrome or Not
- C Program to Display Prime Numbers Between Intervals
- C Program to Check whether the input number is a Neon Number
- C Program to Find All Factors of a Natural Number
- C program to Sum of Fibonacci Numbers at Even Indexes up to N Terms
C Program – Pattern Printing
- C Program to Print Simple Pyramid Pattern
- C Program to Print Given Triangle
- C Program to Print 180 0 Rotation of Simple Pyramid
- C Program to Print Inverted Pyramid
- C Program to Print Number Pattern
- C Program to Print Character Pattern
- C Program to Print Continuous Character Pattern
- C Program to Print Hollow Star Pyramid
- C Program to Print Inverted Hollow Star pyramid
- C Program to Print Hollow Star Pyramid in a Diamond Shape
- C Program to Print Full Diamond Shape Pyramid
- C Program to Print Pascal’s Pattern Triangle Pyramid
- C Program to Print Floyd’s Pattern Triangle Pyramid
- C Program to Print Reverse Floyd pattern Triangle Pyramid
C Program – Functions
- C Program to Check Prime Number By Creating a Function
- C Program to Display Prime Numbers Between Two Intervals Using Functions
- C Program to Find All Roots of a Quadratic Equation
- C Program to Check Whether a Number can be Express as Sum of Two Prime Numbers
- C Program to Find the Sum of Natural Numbers using Recursion
- C Program to Calculate the Factorial of a Number Using Recursion
- C Program to Find G.C.D Using Recursion
- C Program to Reverse a Stack using Recursion
- C Program to Calculate Power Using Recursion
C Program – Arrays
- C Program to Print a 2D Array
- C Program to Find the Largest Element in an Array
- C Program to Find the Maximum and Minimum in an Array
- C Program to Search an Element in an Array (Binary search)
- C Program to Calculate the Average of All the Elements Present in an Array
- C Program to Sort an Array using Bubble Sort
- C Program to Sort an Array using Merge Sort
- C Program to Sort an Array Using Selection Sort
- C Program to Sort an Array Using Insertion Sort
- C Program to Sort the Elements of an Array in Descending Order
- C Program to Sort the Elements of an Array in Ascending Order
- C Program to Remove Duplicate Elements From a Sorted Array
- C Program to Merge Two Arrays
- C Program to Remove All Occurrences of an Element in an Array
- C Program to Find Common Array Elements
- C Program to Copy All the Elements of One Array to Another Array
- C Program For Array Rotation
- C Program to Sort the 2D Array Across Rows
- C Program to Check Whether Two Matrices Are Equal or Not
- C Program to Find the Transpose
- C Program to Find the Determinant of a Matrix
- C Program to Find the Normal and Trace
- C Program to Add Two Matrices
- C Program to Multiply Two Matrices
- C Program to Print Boundary Elements of a Matrix
- C Program to Rotate Matrix Elements
- C Program to Compute the Sum of Diagonals of a Matrix
- C Program to Interchange Elements of First and Last in a Matrix Across Rows
- C Program to Interchange Elements of First and Last in a Matrix Across Columns
C Program – Strings
- C Program to Add or Concatenate Two Strings
- C Program to Add 2 Binary Strings
- C Program to Get a Non-Repeating Character From the Given String
- C Program to check if the string is palindrome or not
- C Program to Reverse an Array or String
- C program to Reverse a String Using Recursion
- C Program to Find the Length of a String
- C Program to Sort a String
- C Program to Check For Pangram String
- C Program to Print the First Letter of Each Word
- C Program to Determine the Unicode Code Point at a Given Index
- C Program to Remove Leading Zeros
- C Program to Compare Two Strings
- C Program to Compare Two Strings Lexicographically
- C Program to Insert a String into Another String
- C Program to Split a String into a Number of Sub-Strings
C Program – Conversions
- C Program For Boolean to String Conversion
- C Program For Float to String Conversion
- C Program For Double to String Conversion
- C Program For String to Long Conversion
- C Program For Long to String Conversion
- C Program For Int to Char Conversion
- C Program For Char to Int Conversion
- C Program For Octal to Decimal Conversion
- C Program For Decimal to Octal Conversion
- C Program For Hexadecimal to Decimal Conversion
- C Program For Decimal to Hexadecimal Conversion
- C Program For Decimal to Binary Conversion
- C Program For Binary to Decimal Conversion
C Program – Pointers
- How to Return a Pointer from a Function in C
- How to Declare a Two-Dimensional Array of Pointers in C?
- C Program to Find the Largest Element in an Array using Pointers
- C Program to Sort an Array using Pointers
- C Program to Sort a 2D Array of Strings
- C Program to Check if a String is a Palindrome using Pointers
- C Program to Create a Copy of a Singly Linked List using Recursion
C Program – Structures and Unions
- C Program to Store Information of Students Using Structure
- C Program to Store Student Records as Structures and Sort them by Name
- C Program to Add N Distances Given in inch-feet System using Structures
- C Program to Add Two Complex Numbers by Passing Structure to a Function
- C Program to Store Student Records as Structures and Sort them by Age or ID
- Read/Write Structure to a File in C
- Flexible Array Members in a Structure in C
C Program – File IO
- C Program to Create a Temporary File
- C Program to Read/Write Structure to a File
- C Program to Rename a file
- C Program to Make a File Read-Only
- C program to Compare Two Files and Report Mismatches
- C Program to Copy One File into Another File
- C Program to Print all the Patterns that Match Given Pattern From a File
- C Program to Append the Content of One Text File to Another
- C Program to Read Content From One File and Write it Into Another File
- C Program to Read and Print all Files From a Zip File
C Program – Date and Time
- C Program to Format time in AM-PM format
- C program to Print Digital Clock with the Current Time
- C Program to Display Dates of Calendar Year in Different Formats
- C Program to Display Current Date and Time
- C Program to Maximize Time by Replacing ‘_’ in a Given 24-Hour Format Time
- C Program to Convert the Local Time to GMT
- C Program to Convert Hours into Minutes and Seconds
C Program – More C Programs
- C Program to Show Runtime exceptions
- C Program to Show Types of errors
- C Program to Show Unreachable Code Error
- C Program to Find Quotient and Remainder
- C Program to Find the Initials of a Name
- C Program to Draw a Circle in Graphics
- Printing Source Code of a C Program Itself
FAQs on C Program
Q1: what is c programming.
C is a structured, high-level, and general-purpose programming language, developed in the early 1970s by Dennis Ritchie at Bell Labs. C language is considered as the mother language of all modern programming languages, widely used for developing system software, embedded software, and application software.
Q2: How do I write a “Hello, World!” program in C?
To write a “Hello, World!” program in C, you can use the following code: #include <stdio.h> int main() { printf(“Hello, World!\n”); return 0; } This code uses the printf function to display the “Hello, World!” message on the screen.
Q3: Why should you learn C Programming?
There are many reasons why you should learn C programming: Versatility Efficiency Portability Widely used Foundation for other languages Employment opportunities and more.
Please Login to comment...
Similar reads.
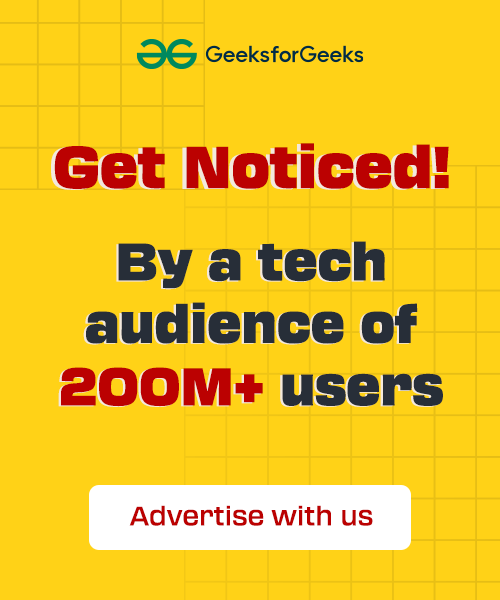
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
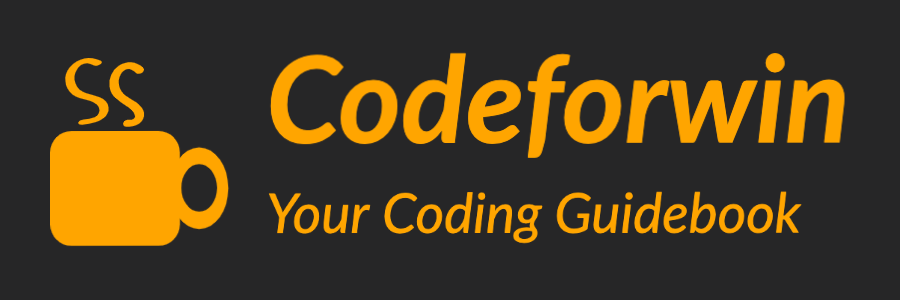
C programming examples, exercises and solutions for beginners
Fundamentals.
- Hello world program in C
- Basic input/output
- Basic IO on all data types
- Perform arithmetic operations
- Find area and perimeter of rectangle
- Find diameter and area of circle
- Find area of triangle
- Find angles of triangle
- Temperature conversion
- Length conversion
- Days conversion
- Find power of a number
- Find square root
- Calculate simple interest
- Calculate compound interest
- Find range of data types
Bitwise Operator
- Check Least Significant Bit (LSB)
- Check Most Significant Bit (MSB)
- Get nth bit of a number
- Set nth bit of a number
- Clear nth bit of a number
- Toggle nth bit of a number
- Highest set bit of a number
- Lowest set bit of a number
- Count trailing zeros of binary number
- Count leading zeros of binary number
- Flip bits of a number
- Rotate bits of a number
- Decimal to binary
- Swap two numbers
- Check even or odd
- View all Bitwise operator examples →
Ternary Operator
- Maximum of two numbers
- Maximum of three numbers
- Check leap year
- Check alphabet
- View all ternary operator examples →
- Find maximum of two numbers
- Find maximum of three numbers
- Check negative, positive or zero
- Check divisibility
- Check alphabet character
- Check vowel or consonant
- Check alphabet, digit or special character
- Check uppercase or lowercase
- Print day of week
- Find number of days in month
- Find roots of quadratic equation
- Calculate profile/loss
- View all if else examples →
Switch case
- Find total days in month
- Check positive, negative or zero
- Simple calculator application
- View all switch case examples →
Loop/Iteration
- Print natural numbers from 1 to n
- Print alphabets from a to z
- Print even numbers from 1 to n
- Print sum of all numbers from 1 to n
- Print sum of all odd numbers from 1 to n
- Print multiplication table of n
- Find number of digits in a number
- Find first and last digit
- Find sum of first and last digit
- Swap first and last digit
- Find sum of digits of a number
- Find reverse of a number
- Find frequency of digits in a number
- Find power of a number using loop
- Find factorial of a number
- Find HCF of two numbers
- Find LCM of two numbers
- View all loop examples →
Number system and conversion
- Find one's complement
- Find two's complement
- Binary to octal conversion
- Binary to decimal conversion
- Binary to hexadecimal conversion
- Octal to binary conversion
- Octal to decimal conversion
- Octal to hexadecimal conversion
- Decimal to binary conversion
- Decimal to octal conversion
- Decimal to hexadecimal conversion
- Hexadecimal to binary conversion
- Hexadecimal to octal conversion
- Hexadecimal to decimal conversion
- View all conversion examples →
Star patterns
- Pascal triangle generation
- Square star pattern
- Hollow square star pattern
- Hollow square star pattern with diagonal
- Rhombus star pattern
- Hollow rhombus star pattern
- Right triangle star pattern
- Hollow right triangle star pattern
- Inverted right triangle star pattern
- Mirrored inverted right triangle star pattern
- Pyramid star pattern
- Inverted pyramid star pattern
- Diamond star pattern
- Plus starpattern
- X star pattern
- 8 star pattern
- Heart star with name pattern
- View all star pattern examples →
Number patterns
- Square number pattern
- Right triangle number pattern
- Inverted right triangle
- Triangle with zero filled
- Half diamond pattern
- Half diamond star bordered pattern
- X number pattern
- View all number pattern examples →
- Find cube using functions
- Diameter & area of circle using functions
- Maximum and minimum using functions
- Check even odd using functions
- Check Prime number using functions
- Find all prime numbers using functions
- Find all strong numbers using functions
- Find all armstrong numbers using functions
- Find all perfect numbers using functions
- View all function examples →
- Find power using recursion
- Print natural numbers using recursion
- Print even numbers using recursion
- Sum of natural numbers using recursion
- Sum of even/odd numbers using recursion
- Find reverse using recursion
- Check palindrome using recursion
- Find sum of digits using recursion
- Find factorial using recursion
- Generate nth Fibonacci term using recursion
- Find HCF (GCD) using recursion
- Find LCM using recursion
- Sum of array elements using recursion
- View all recursion examples →
- Input and display array elements
- Sum of all array elements
- Find second largest element in array
- Copy one array to another
- Insert new element in array
- Delete an element from array
- Find frequency of array elements
- Merge two array to third array
- Delete duplicate elements from array
- Reverse an array
- Search an element in array
- Sort an array
- Left rotate an array
- Right rotate an array
- View all array examples →
Matrix (2D array)
- Add two matrices
- Scalar matrix multiplication
- Multiply two matrices
- Check if two matrices are equal
- Sum of diagonal elements of matrix
- Interchange diagonal of matrix
- Find upper triangular matrix
- Find sum of lower triangular matrix
- Find transpose of triangular matrix
- Find determinant of a matrix
- Check identity matrix
- Check sparse matrix
- Check symmetric matrix
- View all matrix examples →
- Find length of string
- Copy string to another string
- Concatenate two strings
- Compare two strings
- Convert lowercase to uppercase
- Find reverse of a string
- Check palindrome
- Reverse order of words
- Search a character in string
- Search a word in string
- Find highest occurring character
- Remove all duplicate characters
- Replace a character in string
- Trim whitespace from string
- View all string examples →
- Create, initialize and use pointer
- Add two numbers using pointers
- Swap two numbers using pointers
- Access array using pointers
- Copy array using pointers
- Reverse array using pointers
- Access 2D array using pointers
- Multiply matrices using pointers
- Copy strings using pointers
- Concatenate strings using pointers
- Compare strings using pointers
- Reverse strings using pointers
- Sort array strings using pointers
- Return multiple values from function using pointers
- View all pointers examples →
- Create file and write contents
- Read file contents and display
- Append contents to file
- Compare two files
- Copy one file to another
- Merge two files
- Remove a word from file
- Remove a line from file
- Replace a word in file
- Replace a line in file
- Print source code
- Convert uppercase to lowercase in file
- Find properties of file
- Check if file or directory exists
- Rename a file or directory
- List of files recursively
- View all files handling examples →
Macros/Pre-processor directives
- Create custom header file
- Define, undefine and redefine a macro
- Find sum using macro
- Find square and cube using macro
- Check even/odd using macro
- Find maximum or minimum using macro
- Check lowercase/uppercase using macro
- Swap two numbers using macro
- Multiline macros
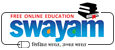
Problem Solving Through Programming In C
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
Note: This exam date is subjected to change based on seat availability. You can check final exam date on your hall ticket.
Page Visits
Course layout, books and references, instructor bio.
Prof. Anupam Basu
Course certificate.
- Assignment score = 25% of average of best 8 assignments out of the total 12 assignments given in the course.
- ( All assignments in a particular week will be counted towards final scoring - quizzes and programming assignments).
- Unproctored programming exam score = 25% of the average scores obtained as part of Unproctored programming exam - out of 100
- Proctored Exam score =50% of the proctored certification exam score out of 100

DOWNLOAD APP

SWAYAM SUPPORT
Please choose the SWAYAM National Coordinator for support. * :

- Data Structure
- Coding Problems
- C Interview Programs
- C++ Aptitude
- Java Aptitude
- C# Aptitude
- PHP Aptitude
- Linux Aptitude
- DBMS Aptitude
- Networking Aptitude
- AI Aptitude
- MIS Executive
- Web Technologie MCQs
- CS Subjects MCQs
- Databases MCQs
- Programming MCQs
- Testing Software MCQs
- Digital Mktg Subjects MCQs
- Cloud Computing S/W MCQs
- Engineering Subjects MCQs
- Commerce MCQs
- More MCQs...
- Machine Learning/AI
- Operating System
- Computer Network
- Software Engineering
- Discrete Mathematics
- Digital Electronics
- Data Mining
- Embedded Systems
- Cryptography
- CS Fundamental
- More Tutorials...
- Tech Articles
- Code Examples
- Programmer's Calculator
- XML Sitemap Generator
- Tools & Generators
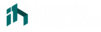
Home » C programming language
C Programs with Solutions
This section contains popular C programs with solution. Learn and practice these programs to test and enhance your C skills. Last updated : April 01, 2023
The best way to learn C programming is by practicing and solving the C programs (C problems). We have 1000+ C programs with solutions which are categorized below. Practice these C programs to learn and enhance your C problem-solving skills.
List of C programs
Practice the C programs based on the categories, library functions, advanced, top searched, and latest.
C programs by categories
- C Basic and Conditional Programs 90
- C switch case programs 06
- C 'goto' programs 10
- Bitwise related Programs 32
- Looping (for, while, do while) Programs 18
- C String Manipulation programs 10
- C String programs 50
- String User Define Functions Programs 11
- Recursion Programs 13
- Number (Digits Manipulation) Programs 10
- Number System Conversion Programs 15
- Star/Pyramid Programs 17
- Sum of Series Programs (set 1) 05
- Sum of Series Programs (set 2) 13
- Pattern printing programs 01
- User Define Function Programs (1) 05
- User Define Function Programs (2) 13
- One Dimensional Array Programs 58
- Two Dimensional Array (Matrix) Programs 21
- File Handling Programs 32
- Structure & Union Programs 12
- Pointer Programs 13
- Dynamic Memory Allocation Programs 05
- Command Line Arguments Programs 06
- Common C program Errors 22
- C scanf() programs 11
- C preprocessor programs 24
- C typedef programs 03
- C SQLite programs 11
- C MySQL programs 09
- C Tricky Programs 07
- Misc Problems & Solutions 05
C programs on standard library functions
- ctype.h Library Functions (Set 1)
- ctype.h Library Functions (Set 2)
- string.h Library Functions
- conio.h Library Functions
- dos.h Library Functions
- math.h Library Functions
- graphics.h Library Functions
- assert.h Library Functions
- stdio.h Library Functions
Advance C programs
- C program to create your own header file/ Create your your own header file in C
- gotoxy(),clrscr(),getch(),getche() for GCC, Linux.
- fork() function explanation and examples in Linux C
- C program to print character without using format specifiers.
- C program to find Binary Addition and Binary Subtraction.
- C program to print weekday of given date.
- C program to format/extract ip address octets
- C program to check given string is a valid IPv4 address or not.
- C program to extract bytes from an integer (Hexadecimal) value
- C program to store date in an integer variable
Top searched C programs
Here is the list of most important/useful programs searched on the web .
Top visited programs on IncludeHelp
- Pattern Programs in C
- C program to design calculator with basic operations using switch
- C program to find factorial of a number
- C program to check whether number is Perfect Square or not
- C program to find SUM and AVERAGE of two numbers
- C program to convert temperature from Fahrenheit to Celsius and Celsius to Fahrenheit
- C program to read and print an employee's detail using structure
- Dynamic Memory Allocation programs
- C program to convert number from Decimal to Binary
- C program to check whether number is Palindrome or not
Top searched programs on the web
- First C program to print "Hello World".
- C program to find factorial of a number.
- C program to swap two numbers without using third variable.
- C program to check whether a number if Armstrong or not.
- C program to check whether a number if Even or Odd.
- C program to print all leap years from 1 to N.
- C program to calculate employee gross salary.
- C Program to print tables of numbers from 1 to 20.
- C program to print star/pyramid series.
- C program to convert temperature from Celsius to Fahrenheit and vice versa.
- C program to convert number from Decimal to Binary.
- C program to convert number from Binary to Decimal.
- C program to print ASCII Table.
- C program to get and set current system date and time.
- C program to run dos command.
Latest C programs
- C program to generate random numbers within a range
- C program to compare strings using strcmp() function
- Interchange the two adjacent nodes in a given circular linked list | C program
- Find the largest element in a doubly linked list | C program
- Convert a given singly linked list to a circular list | C program
- Implement Circular Doubly Linked List | C program
- Print the Alternate Nodes in a Linked List without using Recursion
- Print the Alternate Nodes in a Linked List using Recursion
- Find the length of a linked list without using recursion
- Find the length of a linked list using recursion
- Count the number of occurrences of an element in a linked list without using recursion
- Count the number of occurrences of an element in a linked list using recursion
- C program to convert a Binary Tree into a Singly Linked List by Traversing Level by Level
- C program to Check if nth Bit in a 32-bit Integer is set or not
- C program to swap two Integers using Bitwise Operators
- C program to replace bit in an integer at a specified position from another integer
- C program to find odd or even number using bitmasking
- C program to check whether a given number is palindrome or not using Bitwise Operator
- C program to count number of bits set to 1 in an Integer
- C program to check if all the bits of a given integer is one (1)
- C program to find the Highest Bit Set for any given Integer
- C program to Count the Number of Trailing Zeroes in an Integer
- C Program to find the Biggest Number in an Array of Numbers using Recursion
- C program to accept Sorted Array and do Search using Binary Search
- C Program to Cyclically Permute the Elements of an Array
- C program to find two smallest elements in a one dimensional array
- Write your own memset() function in C
- memset() function in C with Example
- Write your own memcpy() function in C
- memcpy() function in C with Example
Comments and Discussions!
Load comments ↻
- Marketing MCQs
- Blockchain MCQs
- Artificial Intelligence MCQs
- Data Analytics & Visualization MCQs
- Python MCQs
- C++ Programs
- Python Programs
- Java Programs
- D.S. Programs
- Golang Programs
- C# Programs
- JavaScript Examples
- jQuery Examples
- CSS Examples
- C++ Tutorial
- Python Tutorial
- ML/AI Tutorial
- MIS Tutorial
- Software Engineering Tutorial
- Scala Tutorial
- Privacy policy
- Certificates
- Content Writers of the Month
Copyright © 2024 www.includehelp.com. All rights reserved.

Problem Solving Through Programming in C
In this lesson, we are going to learn Problem Solving Through Programming in C. This is the first lesson while we start learning the C language.
So let’s start learning the C language.
Table of Contents
Introduction to Problem Solving Through Programming in C
Regardless of the area of the study, computer science is all about solving problems with computers. The problem that we want to solve can come from any real-world problem or perhaps even from the abstract world. We need to have a standard systematic approach to problem solving through programming in c.
computer programmers are problem solvers. In order to solve a problem on a computer, we must know how to represent the information describing the problem and determine the steps to transform the information from one representation into another.
In this chapter, we will learn problem-solving and steps in problem-solving, basic tools for designing solution as an algorithm, flowchart , pseudo code etc.
A computer is a very powerful and versatile machine capable of performing a multitude of different tasks, yet it has no intelligence or thinking power.
The Computer performs many tasks exactly in the same manner as it is told to do. This places responsibility on the user to instruct the computer in a correct and precise manner so that the machine is able to perform the required job in a proper way. A wrong or ambiguous instruction may sometimes prove dangerous.
The computer cannot solve the problem on its own, one has to provide step by step solutions of the problem to the computer. In fact, the task of problem-solving is not that of the computer.
It is the programmer who has to write down the solution to the problem in terms of simple operations which the computer can understand and execute.
Problem-solving is a sequential process of analyzing information related to a given situation and generating appropriate response options.
In order to solve a problem with the computer, one has to pass through certain stages or steps. They are as follows:
Steps to Solve a Problem With the Computer
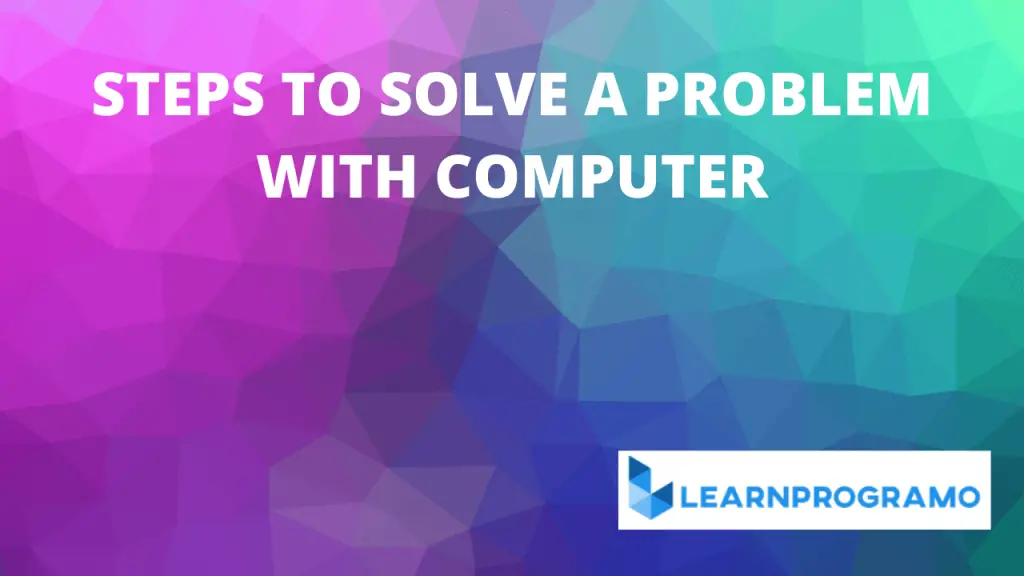
Step 1: Understanding the Problem:
Here we try to understand the problem to be solved in totally. Before with the next stage or step, we should be absolutely sure about the objectives of the given problem.
Step 2: Analyzing the Problem:
After understanding thoroughly the problem to be solved, we look at different ways of solving the problem and evaluate each of these methods.
The idea here is to search for an appropriate solution to the problem under consideration. The end result of this stage is a broad overview of the sequence of operations that are to be carried out to solve the given problem.
Step 3: Developing the solution:
Here, the overview of the sequence of operations that was the result of the analysis stage is expanded to form a detailed step by step solution to the problem under consideration.
Step 4: Coding and Implementation:
The last stage of problem-solving is the conversion of the detailed sequence of operations into a language that the computer can understand. Here, each step is converted to its equivalent instruction or instructions in the computer language that has been chosen for the implantation.
The vehicle for the computer solution to a problem is a set of explicit and unambiguous instructions expressed in a programming language. This set of instruction is called a program with problem solving through programming in C .
A program may also be thought of as an algorithm expressed in a programming language. an algorithm, therefore, corresponds to a solution to a problem that is independent of any programming language .
To obtain the computer solution to a problem once we have the program we usually have to supply the program with input or data. The program then takes this input and manipulates it according to its instructions. Eventually produces an output which represents the computer solution to the problem.
The problem solving is a skill and there are no universal approaches one can take to solving problems. Basically one must explore possible avenues to a solution one by one until she/he comes across the right path to a solution.
In general, as one gains experience in solving problems, one develops one’s own techniques and strategies, though they are often intangible. Problem-solving skills are recognized as an integral component of computer programming.
Note: Practice C Programs for problem solving through programming in C.
Problem Solving Steps
Problem-solving is a creative process which defines systematization and mechanization. There are a number of steps that can be taken to raise the level of one’s performance in problem-solving.
A problem-solving technique follows certain steps in finding the solution to a problem. Let us look into the steps one by one:
1. Problem Definition Phase:
The success in solving any problem is possible only after the problem has been fully understood. That is, we cannot hope to solve a problem, which we do not understand. So, the problem understanding is the first step towards the solution of the problem.
In the problem definition phase, we must emphasize what must be done rather than how is it to be done. That is, we try to extract the precisely defined set of tasks from the problem statement.
Inexperienced problem solvers too often gallop ahead with the task of the problem – solving only to find that they are either solving the wrong problem or solving the wrong problem or solving just one particular problem.
2. Getting Started on a Problem:
There are many ways of solving a problem and there may be several solutions. So, it is difficult to recognize immediately which path could be more productive. Problem solving through programming in C.
Sometimes you do not have any idea where to begin solving a problem, even if the problem has been defined. Such block sometimes occurs because you are overly concerned with the details of the implementation even before you have completely understood or worked out a solution.
The best advice is not to get concerned with the details. Those can come later when the intricacies of the problem have been understood.
3. Use of Specific Examples:
To get started on a problem, we can make use of heuristics i.e the rule of thumb. This approach will allow us to start on the problem by picking a specific problem we wish to solve and try to work out the mechanism that will allow solving this particular problem.
It is usually much easier to work out the details of a solution to a specific problem because the relationship between the mechanism and the problem is more clearly defined.
This approach of focusing on a particular problem can give us the foothold we need for making a start on the solution to the general problem.
4. Similarities Among Problems:
One way to make a start is by considering a specific example. Another approach is to bring the experience to bear on the current problems. So, it is important to see if there are any similarities between the current problem and the past problems which we have solved.
The more experience one has the more tools and techniques one can bring to bear in tackling the given problem. But sometimes, it blocks us from discovering a desirable or better solution to the problem.
A skill that is important to try to develop in problem-solving is the ability to view a problem from a variety of angles.
One must be able to metaphorically turn a problem upside down, inside out, sideways, backwards, forwards and so on. Once one has developed this skill it should be possible to get started on any problem.
5. Working Backwards from the Solution:
In some cases, we can assume that we already have the solution to the problem and then try to work backwards to the starting point. Even a guess at the solution to the problem may be enough to give us a foothold to start on the problem.
We can systematize the investigations and avoid duplicate efforts by writing down the various steps taken and explorations made.
Another practice that helps to develop the problem-solving skills, once we have solved a problem, to consciously reflect back on the way we went about discovering the solution.
General Problem Solving Strategies:
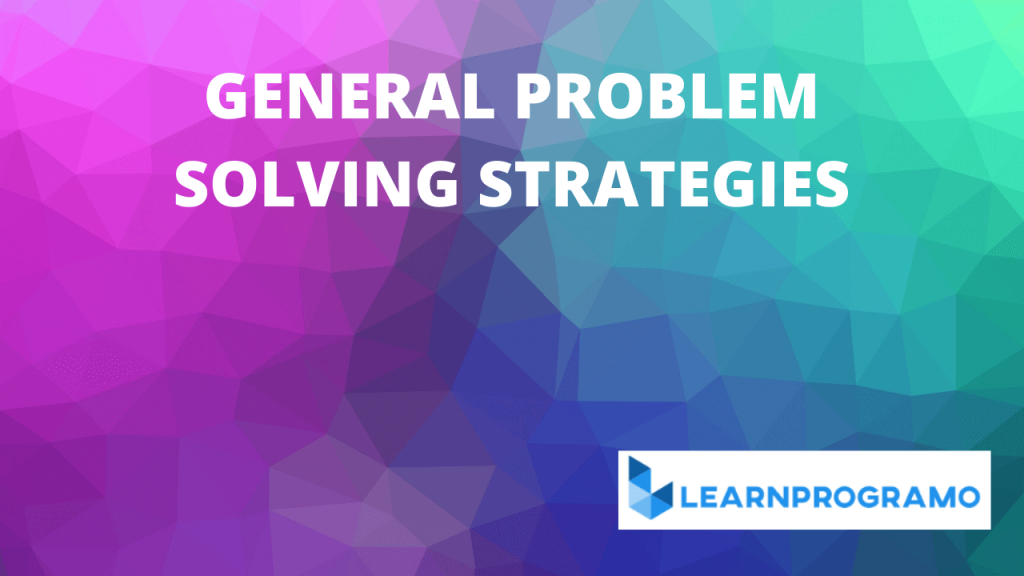
There are a number of general and powerful computational strategies that are repeatedly used in various guises in computer science.
Often it is possible to phrase a problem in terms of one of these strategies and achieve considerable gains in computational efficiency.
1. Divide and Conquer:
The most widely known and used strategy, where the basic idea is to break down the original problem into two or more sub-problems, which is presumably easier or more efficient to solve.
The Splitting can be carried on further so that eventually we have many sub-problems, so small that further splitting is no necessary to solve them. We shall see many examples of this strategy and discuss the gain in efficiency due to its application.
2. Binary Doubling:
This is the reverse of the divide and conquers strategy i.e build-up the solution for a larger problem from solutions and smaller sub-problems.
3. Dynamic Programming:
Another general strategy for problem-solving which is useful when we can build-up the solution as a sequence of the intermediate steps. Problem Solving through programming in C.
The travelling salesman problem falls into this category. The idea here is that a good or optimal solution to a problem can be built-up from good or optimal solutions of the sub-problems.
4. General Search, Back Tracking and Branch-and-Bound:
All of these are variants of the basic dynamic programming strategy but are equally important.
Share This Story, Choose Your Platform!
Related posts.
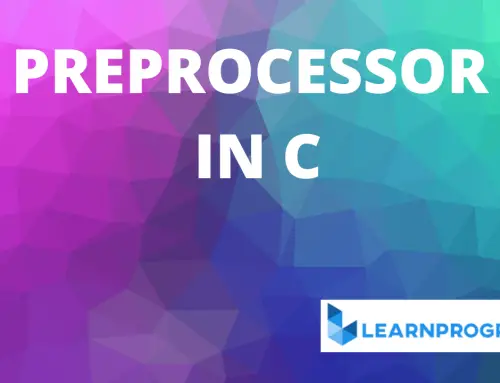
What is Preprocessor in C
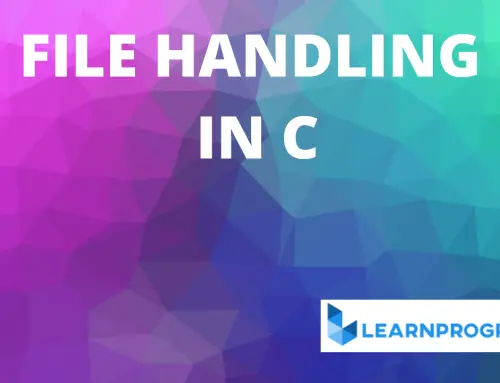
What is File Handling in C
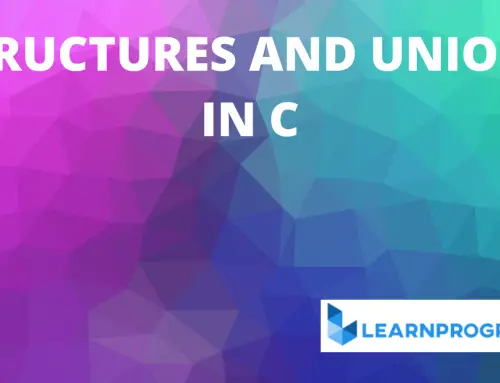
Structures and Unions in C
01. PLAY WITH PRINTF()
02. data type & variables, 03. user input scanf(), 04. apply your conditions, 05. magic of loop, 06. uses of array, 07. fun of function, 08. start with string, 09. pointer de complexity, 10. structur of union, 11. basic of file i/o, 12. math & coding.
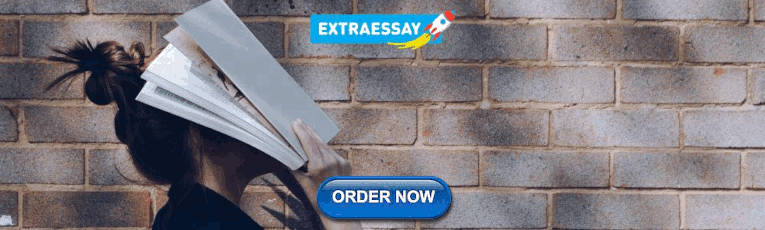
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Collection of HackerRank Solutions in C
MahmoudNageh/hackerrank-c-solutions
Folders and files, repository files navigation, hackerrank-c-solutions.
These questions are a collective team effort. Feel free to use my solutions as inspiration, but please don't literally copy the code.
How to Solve Coding Problems with a Simple Four Step Method
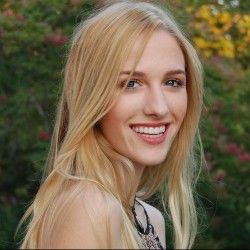
I had fifteen minutes left, and I knew I was going to fail.
I had spent two months studying for my first technical interview.
I thought I was prepared, but as the interview came to a close, it hit me: I had no idea how to solve coding problems.
Of all the tutorials I had taken when I was learning to code, not one of them had included an approach to solving coding problems.
I had to find a method for problem-solving—my career as a developer depended on it.
I immediately began researching methods. And I found one. In fact, what I uncovered was an invaluable strategy. It was a time-tested four-step method that was somehow under the radar in the developer ecosystem.
In this article, I’ll go over this four-step problem-solving method that you can use to start confidently solving coding problems.
Solving coding problems is not only part of the developer job interview process—it’s what a developer does all day. After all, writing code is problem-solving.
A method for solving problems
This method is from the book How to Solve It by George Pólya. It originally came out in 1945 and has sold over one million copies.
His problem-solving method has been used and taught by many programmers, from computer science professors (see Udacity’s Intro to CS course taught by professor David Evans) to modern web development teachers like Colt Steele.
Let’s walk through solving a simple coding problem using the four-step problem-solving method. This allows us to see the method in action as we learn it. We'll use JavaScript as our language of choice. Here’s the problem:
Create a function that adds together two numbers and returns that value. There are four steps to the problem-solving method:
- Understand the problem.
- Devise a plan.
- Carry out the plan.
Let’s get started with step one.
Step 1: Understand the problem.
When given a coding problem in an interview, it’s tempting to rush into coding. This is hard to avoid, especially if you have a time limit.
However, try to resist this urge. Make sure you actually understand the problem before you get started with solving it.
Read through the problem. If you’re in an interview, you could read through the problem out loud if that helps you slow down.
As you read through the problem, clarify any part of it you do not understand. If you’re in an interview, you can do this by asking your interviewer questions about the problem description. If you’re on your own, think through and/or Google parts of the question you might not understand.
This first step is vital as we often don’t take the time to fully understand the problem. When you don’t fully understand the problem, you’ll have a much harder time solving it.
To help you better understand the problem, ask yourself:
What are the inputs?
What kinds of inputs will go into this problem? In this example, the inputs are the arguments that our function will take.
Just from reading the problem description so far, we know that the inputs will be numbers. But to be more specific about what the inputs will be, we can ask:
Will the inputs always be just two numbers? What should happen if our function receives as input three numbers?
Here we could ask the interviewer for clarification, or look at the problem description further.
The coding problem might have a note saying, “You should only ever expect two inputs into the function.” If so, you know how to proceed. You can get more specific, as you’ll likely realize that you need to ask more questions on what kinds of inputs you might be receiving.
Will the inputs always be numbers? What should our function do if we receive the inputs “a” and “b”? Clarify whether or not our function will always take in numbers.
Optionally, you could write down possible inputs in a code comment to get a sense of what they’ll look like:
//inputs: 2, 4
What are the outputs?
What will this function return? In this case, the output will be one number that is the result of the two number inputs. Make sure you understand what your outputs will be.
Create some examples.
Once you have a grasp of the problem and know the possible inputs and outputs, you can start working on some concrete examples.
Examples can also be used as sanity checks to test your eventual problem. Most code challenge editors that you’ll work in (whether it’s in an interview or just using a site like Codewars or HackerRank) have examples or test cases already written for you. Even so, writing out your own examples can help you cement your understanding of the problem.
Start with a simple example or two of possible inputs and outputs. Let's return to our addition function.
Let’s call our function “add.”
What’s an example input? Example input might be:
// add(2, 3)
What is the output to this? To write the example output, we can write:
// add(2, 3) ---> 5
This indicates that our function will take in an input of 2 and 3 and return 5 as its output.
Create complex examples.
By walking through more complex examples, you can take the time to look for edge cases you might need to account for.
For example, what should we do if our inputs are strings instead of numbers? What if we have as input two strings, for example, add('a', 'b')?
Your interviewer might possibly tell you to return an error message if there are any inputs that are not numbers. If so, you can add a code comment to handle this case if it helps you remember you need to do this.
Your interviewer might also tell you to assume that your inputs will always be numbers, in which case you don’t need to write any extra code to handle this particular input edge case.
If you don’t have an interviewer and you’re just solving this problem, the problem might say what happens when you enter invalid inputs.
For example, some problems will say, “If there are zero inputs, return undefined.” For cases like this, you can optionally write a comment.
// check if there are no inputs.
// If no inputs, return undefined.
For our purposes, we’ll assume that our inputs will always be numbers. But generally, it’s good to think about edge cases.
Computer science professor Evans says to write what developers call defensive code. Think about what could go wrong and how your code could defend against possible errors.
Before we move on to step 2, let’s summarize step 1, understand the problem:
-Read through the problem.
-What are the inputs?
-What are the outputs?
Create simple examples, then create more complex ones.
2. Devise a plan for solving the problem.
Next, devise a plan for how you’ll solve the problem. As you devise a plan, write it out in pseudocode.
Pseudocode is a plain language description of the steps in an algorithm. In other words, your pseudocode is your step-by-step plan for how to solve the problem.
Write out the steps you need to take to solve the problem. For a more complicated problem, you’d have more steps. For this problem, you could write:
// Create a sum variable.
Add the first input to the second input using the addition operator .
// Store value of both inputs into sum variable.
// Return as output the sum variable. Now you have your step-by-step plan to solve the problem. For more complex problems, professor Evans notes, “Consider systematically how a human solves the problem.” That is, forget about how your code might solve the problem for a moment, and think about how you would solve it as a human. This can help you see the steps more clearly.
3. Carry out the plan (Solve the problem!)

The next step in the problem-solving strategy is to solve the problem. Using your pseudocode as your guide, write out your actual code.
Professor Evans suggests focusing on a simple, mechanical solution. The easier and simpler your solution is, the more likely you can program it correctly.
Taking our pseudocode, we could now write this:
Professor Evans adds, remember not to prematurely optimize. That is, you might be tempted to start saying, “Wait, I’m doing this and it’s going to be inefficient code!”
First, just get out your simple, mechanical solution.
What if you can’t solve the entire problem? What if there's a part of it you still don't know how to solve?
Colt Steele gives great advice here: If you can’t solve part of the problem, ignore that hard part that’s tripping you up. Instead, focus on everything else that you can start writing.
Temporarily ignore that difficult part of the problem you don’t quite understand and write out the other parts. Once this is done, come back to the harder part.
This allows you to get at least some of the problem finished. And often, you’ll realize how to tackle that harder part of the problem once you come back to it.
Step 4: Look back over what you've done.
Once your solution is working, take the time to reflect on it and figure out how to make improvements. This might be the time you refactor your solution into a more efficient one.
As you look at your work, here are some questions Colt Steele suggests you ask yourself to figure out how you can improve your solution:
- Can you derive the result differently? What other approaches are there that are viable?
- Can you understand it at a glance? Does it make sense?
- Can you use the result or method for some other problem?
- Can you improve the performance of your solution?
- Can you think of other ways to refactor?
- How have other people solved this problem?
One way we might refactor our problem to make our code more concise: removing our variable and using an implicit return:
With step 4, your problem might never feel finished. Even great developers still write code that they later look at and want to change. These are guiding questions that can help you.
If you still have time in an interview, you can go through this step and make your solution better. If you are coding on your own, take the time to go over these steps.
When I’m practicing coding on my own, I almost always look at the solutions out there that are more elegant or effective than what I’ve come up with.
Wrapping Up
In this post, we’ve gone over the four-step problem-solving strategy for solving coding problems.
Let's review them here:
- Step 1: understand the problem.
- Step 2: create a step-by-step plan for how you’ll solve it .
- Step 3: carry out the plan and write the actual code.
- Step 4: look back and possibly refactor your solution if it could be better.
Practicing this problem-solving method has immensely helped me in my technical interviews and in my job as a developer. If you don't feel confident when it comes to solving coding problems, just remember that problem-solving is a skill that anyone can get better at with time and practice.
Problem solving through Programming In C
- BE/BTech in all disciplines
- BCA/MCA/M. Sc
- All IT Industries
65741 students have enrolled already!!
In association with

Programmingoneonone - Programs for Everyone
- HackerRank Problems Solutions
- _C solutions
- _C++ Solutions
- _Java Solutions
- _Python Solutions
- _Interview Preparation kit
- _1 Week Solutions
- _1 Month Solutions
- _3 Month Solutions
- _30 Days of Code
- _10 Days of JS
- CS Subjects
- _IoT Tutorials
- DSA Tutorials
- Interview Questions
HackerRank C All Problems solutions
Post a comment.
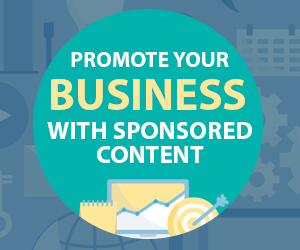
- 10 day of javascript
- 10 days of statistics
- 30 days of code
- Codechef Solutions
- coding problems
- data structure
- hackerrank solutions
- interview prepration kit
- linux shell
Social Plugin
Subscribe us, popular posts.

HackerRank Floyd: City of Blinding Lights problem solution
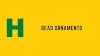
HackerRank Bead Ornaments problem solution

HackerRank Jeanie's Route problem solution

Master in Public Administration
Prepare for greater responsibility across sectors with this flexible two-year program
Key Program Information
Program Length: Two years (varies for students pursuing concurrent degrees)
Degree Awarded: Master in Public Administration
Admission Application Deadline: December 2024
Financial Aid Application Deadline: January 2025
Contact the MPA Program

79 John F. Kennedy Street Littauer Building, Room 126 Cambridge, Massachusetts 02138
Elevate your impact as a public leader
The Master in Public Administration Program is for aspiring leaders with real-world experience and graduate-level coursework in economics, public policy, or management.
The Master in Public Administration (MPA) curriculum is flexible. You create a study plan that reflects your academic interests, focuses on your personal and professional aspirations, and integrates across disciplines.
You may also decide to pursue a concurrent degree in business, law, medicine, or other professional fields. You’ll earn two degrees in less time and acquire even more skills you can use to make a difference in the world.
“HKS provided opportunities for me to expand the narrative of what diversity looks like in foreign policy and foreign service.” —Korde Innis MPA 2023
About the MPA Program
The MPA curriculum is flexible. You’ll create your own 64-credit study plan that reflects your academic interests and professional aspirations.
As an MPA student, you’ll take eight credits in a Policy Area of Concentration as well as four credits in each of these key areas:
- Economics and Quantitative Analysis
- Management, Leadership, and Decision Sciences
- Public Ethics and Political Institutions
You’ll select your remaining credits to support your unique intellectual and professional objectives. You can take classes across academic disciplines at HKS and cross-register into courses at other Harvard graduate schools as well as at MIT Sloan School of Management and The Fletcher School at Tufts University.
Sample Courses
- MLD-223: Negotiating Across Differences
- API-135: Economics of Climate Change and Environmental Policy
- DPI-640: Technology and the Public Interest: From Democracy to Technocracy and Back
Concurrent Degrees
You might consider pursuing a second degree concurrently if you’re interested in how the world’s public challenges can be addressed at the intersection of business, law, medicine, design, or other fields.
Pursuing a concurrent degree reduces coursework and residency requirements and makes it possible to earn two degrees in a shorter amount of time.
Concurrent degree students complete 48 credits at HKS, including four credits in each of the key areas:
Concurrent degree students are not required to fulfill the Policy Area of Concentration requirement.
Degree Requirements
The MPA Program consists of four semesters of full-time coursework in residence at HKS.
To graduate, you must:
- Earn at least 64 credits
- Finish with a GPA of B or better
- Earn a B- or higher in all courses counting towards the distribution requirements
- Matriculate as a full-time, in-residence student and take between 12-20 credits per semester
- Fulfill a Policy Area of Concentration requirement by taking eight credits in one of the policy areas at HKS
- Economics and Quantitative Analysis
HKS faculty members are among the most influential leaders and thinkers in their fields. They have contributed vital research and scholarship to their fields. Served in U.S. administrations. Founded learning labs to assist local communities. Led negotiations to reduce nuclear stockpiles. Reported on human rights abuses. Led efforts to address climate change. Advised governments and companies on gender equality.
They are doers as well as thinkers, shaping public policy and devising entrepreneurial approaches to public problems at the local, national, and international levels.
MPA Faculty Chair

Kessely Hong
Mpa at a glance.
*Statistics are based on a five-year average.
Employment Snapshot: MPA Class of 2023 Employment Sectors

* The number of private sector jobs secured by MPA graduates is, in large part, a reflection of the program’s many concurrent degree graduates.
Featured MPA stories
Elevating the stories of diverse, unsung women.
Jamie Mittleman MPA 2022 launched her COVID-adapted dream job: a platform for women Olympians and Paralympians.
A long military heritage shapes a desire for peace
Clark Yuan MPA 2022 wants unique perspectives to have a seat at the table when critical decisions are being made.
From Santiago to Cambridge to Paris
For Ingrid Olea MPA 2020, a journey that started with a career change has led to remarkable achievements in education policymaking.
Applying to the MPA Program
Prerequisites.
There are specific academic and professional prerequisites required to apply to the MPA Program. You must have:
- A bachelor’s degree with a strong academic record
- Three years of professional work experience by September 1 of the year you would enroll in the program
- Master of Business Administration
- Master of International Business
- Master of International Development
- Master of International Economics
- Master of Science in Engineering
How to Apply
A complete application to the MPA Program includes the following:
- Online application
- Three letters of recommendation
- GRE or GMAT required
- Non-native English speakers who did not earn an undergraduate degree conducted in English must submit results of the TOEFL, IELTS, or Cambridge English exam
- Academic transcripts
- $100 application fee or waiver
Read more about how to apply .
The application for the 2025-2026 academic year will be available in September 2024. There is one admission application deadline and one start date for each degree program per year. You may apply to only one master's degree program per admissions cycle.
Tuition & Fees
The cost of attendance for the 2024-2025 academic year is outlined in Funding Your Master ’ s Education to help you plan financially for our master’s degree programs. Living expense costs are based on residence in Cambridge. The 2025-2026 academic year rates will be published in March 2025. HKS tuition and fees are subject to change without notice.
At HKS, we consider financing your education to be a partnership and are here to help guide you. We encourage you to explore all opportunities for funding .
Learn more about the HKS community
Student life, student stories, admissions & financial aid blog.
We've detected unusual activity from your computer network
To continue, please click the box below to let us know you're not a robot.
Why did this happen?
Please make sure your browser supports JavaScript and cookies and that you are not blocking them from loading. For more information you can review our Terms of Service and Cookie Policy .
For inquiries related to this message please contact our support team and provide the reference ID below.
- Work & Careers
- Life & Arts
Become an FT subscriber
Try unlimited access Only $1 for 4 weeks
Then $75 per month. Complete digital access to quality FT journalism on any device. Cancel anytime during your trial.
- Global news & analysis
- Expert opinion
- Special features
- FirstFT newsletter
- Videos & Podcasts
- Android & iOS app
- FT Edit app
- 10 gift articles per month
Explore more offers.
Standard digital.
- FT Digital Edition
Premium Digital
Print + premium digital, weekend print + standard digital, weekend print + premium digital.
Today's FT newspaper for easy reading on any device. This does not include ft.com or FT App access.
- 10 additional gift articles per month
- Global news & analysis
- Exclusive FT analysis
- Videos & Podcasts
- FT App on Android & iOS
- Everything in Standard Digital
- Premium newsletters
- Weekday Print Edition
- FT Weekend Print delivery
- Everything in Premium Digital
Essential digital access to quality FT journalism on any device. Pay a year upfront and save 20%.
- Everything in Print
Complete digital access to quality FT journalism with expert analysis from industry leaders. Pay a year upfront and save 20%.
Terms & Conditions apply
Explore our full range of subscriptions.
Why the ft.
See why over a million readers pay to read the Financial Times.
International Edition
Windows 11, version 23H2
April 23, 2024—kb5036980 (os builds 22621.3527 and 22631.3527) preview.
- April 9, 2024—KB5036893 (OS Builds 22621.3447 and 22631.3447)
- March 26, 2024—KB5035942 (OS Builds 22621.3374 and 22631.3374) Preview
- March 12, 2024—KB5035853 (OS Builds 22621.3296 and 22631.3296)
- February 29, 2024—KB5034848 (OS Builds 22621.3235 and 22631.3235) Preview
- February 13, 2024—KB5034765 (OS Builds 22621.3155 and 22631.3155)
- January 23, 2024—KB5034204 (OS Builds 22621.3085 and 22631.3085) Preview
- January 9, 2024—KB5034123 (OS Builds 22621.3007 and 22631.3007)
- December 12, 2023—KB5033375 (OS Builds 22621.2861 and 22631.2861)
- December 4, 2023—KB5032288 (OS Builds 22621.2792 and 22631.2792) Preview
- November 14, 2023—KB5032190 (OS Builds 22621.2715 and 22631.2715)
- October 31, 2023—KB5031455 (OS Builds 22621.2506 and 22631.2506) Preview
Windows 11, version 22H2
- October 10, 2023—KB5031354 (OS Build 22621.2428)
- September 26, 2023—KB5030310 (OS Build 22621.2361) Preview
- September 12, 2023—KB5030219 (OS Build 22621.2283)
- August 22, 2023—KB5029351 (OS Build 22621.2215) Preview
- August 8, 2023—KB5029263 (OS Build 22621.2134)
- July 26, 2023—KB5028254 (OS Build 22621.2070) Preview
- July 11, 2023—KB5028185 (OS Build 22621.1992)
- June 27, 2023—KB5027303 (OS Build 22621.1928) Preview
- June 13, 2023—KB5027231 (OS Build 22621.1848)
- May 24, 2023—KB5026446 (OS Build 22621.1778) Preview
- May 9, 2023—KB5026372 (OS Build 22621.1702)
- April 25, 2023—KB5025305 (OS Build 22621.1635) Preview
- April 11, 2023—KB5025239 (OS Build 22621.1555)
- March 28, 2023—KB5023778 (OS Build 22621.1485) Preview
- March 14, 2023—KB5023706 (OS Build 22621.1413)
- February 28, 2023—KB5022913 (OS Build 22621.1344) Preview
- February 14, 2023—KB5022845 (OS Build 22621.1265)
- January 26, 2023—KB5022360 (OS Build 22621.1194) Preview
- January 10, 2023—KB5022303 (OS Build 22621.1105)
- December 13, 2022—KB5021255 (OS Build 22621.963)
- November 29, 2022—KB5020044 (OS Build 22621.900) Preview
- November 8, 2022—KB5019980 (OS Build 22621.819)
- October 25, 2022—KB5018496 (OS Build 22621.755) Preview
- October 18, 2022—KB5019509 (OS Build 22621.675) Out-of-band
- October 11, 2022—KB5018427 (OS Build 22621.674)
- September 30, 2022—KB5017389 (OS Build 22621.608) Preview
- Windows 11, version 21H2
- April 9, 2024—KB5036894 (OS Build 22000.2899)
- March 12, 2024—KB5035854 (OS Build 22000.2836)
- February 13, 2024—KB5034766 (OS Build 22000.2777)
- January 9, 2024—KB5034121 (OS Build 22000.2713)
- December 12, 2023—KB5033369 (OS Build 22000.2652)
- November 14, 2023—KB5032192 (OS Build 22000.2600)
- October 10, 2023—KB5031358 (OS Build 22000.2538)
- September 26, 2023—KB5030301 (OS Build 22000.2482) Preview
- September 12, 2023—KB5030217 (OS Build 22000.2416)
- August 22, 2023—KB5029332 (OS Build 22000.2360) Preview
- August 8, 2023—KB5029253 (OS Build 22000.2295)
- July 25, 2023—KB5028245 (OS Build 22000.2245) Preview
- July 11, 2023—KB5028182 (OS Build 22000.2176)
- June 28, 2023—KB5027292 (OS Build 22000.2124) Preview
- June 13, 2023—KB5027223 (OS Build 22000.2057)
- May 23, 2023—KB5026436 (OS Build 22000.2003) Preview
- May 9, 2023—KB5026368 (OS Build 22000.1936)
- April 25, 2023—KB5025298 (OS Build 22000.1880) Preview
- April 11, 2023—KB5025224 (OS Build 22000.1817)
- March 28, 2023—KB5023774 (OS Build 22000.1761) Preview
- March 14, 2023—KB5023698 (OS Build 22000.1696)
- February 21, 2023—KB5022905 (OS Build 22000.1641) Preview
- February 14, 2023—KB5022836 (OS Build 22000.1574)
- January 19, 2023—KB5019274 (OS Build 22000.1516) Preview
- January 10, 2023—KB5022287 (OS Build 22000.1455)
- December 13, 2022—KB5021234 (OS Build 22000.1335)
- November 15, 2022—KB5019157 (OS Build 22000.1281) Preview
- November 8, 2022—KB5019961 (OS Build 22000.1219)
- October 25, 2022—KB5018483 (OS Build 22000.1165) Preview
- October 17, 2022—KB5020387 (OS Build 22000.1100) Out-of-band
- October 11, 2022—KB5018418 (OS Build 22000.1098)
- September 20, 2022—KB5017383 (OS Build 22000.1042) Preview
- September 13, 2022—KB5017328 (OS Build 22000.978)
- August 25, 2022—KB5016691 (OS Build 22000.918) Preview
- August 9, 2022—KB5016629 (OS Build 22000.856)
- July 21, 2022—KB5015882 (OS Build 22000.832) Preview
- July 12, 2022—KB5015814 (OS Build 22000.795)
- June 23, 2022—KB5014668 (OS Build 22000.778) Preview
- June 20, 2022—KB5016138 (OS Build 22000.740) Out-of-band
- June 14, 2022—KB5014697 (OS Build 22000.739)
- May 24, 2022—KB5014019 (OS Build 22000.708) Preview
- May 10, 2022—KB5013943 (OS Build 22000.675)
- April 25, 2022—KB5012643 (OS Build 22000.652) Preview
- April 12, 2022—KB5012592 (OS Build 22000.613)
- March 28, 2022—KB5011563 (OS Build 22000.593) Preview
- March 8, 2022—KB5011493 (OS Build 22000.556)
- February 15, 2022—KB5010414 (OS Build 22000.527) Preview
- February 8, 2022—KB5010386 (OS Build 22000.493)
- January 25, 2022—KB5008353 (OS Build 22000.469) Preview
- January 17, 2022—KB5010795 (OS Build 22000.438) Out-of-band
- January 11, 2022—KB5009566 (OS Build 22000.434)
- December 14, 2021—KB5008215 (OS Build 22000.376)
- November 22, 2021—KB5007262 (OS Build 22000.348) Preview
- November 9, 2021—KB5007215 (OS Build 22000.318)
- October 21, 2021—KB5006746 (OS Build 22000.282) Preview
- October 12, 2021—KB5006674 (OS Build 22000.258)
Release Date:
OS Builds 22621.3527 and 22631.3527
2/27/24 IMPORTANT: New dates for the end of non-security updates for Windows 11, version 22H2
The new end date is June 24, 2025 for Windows 11, version 22H2 Enterprise and Education editions. Home and Pro editions of version 22H2 will receive non-security preview updates until June, 26, 2024.
After these dates, only cumulative monthly security updates will continue for the supported editions of Windows 11, version 22H2. The initial date communicated for this change was February 27, 2024. Based on user feedback, this date has been changed so more customers can take advantage of our continuous innovations .
For information about Windows update terminology, see the article about the types of Windows updates and the monthly quality update types . For an overview of Windows 11, version 23H2, see its update history page .
Note Follow @WindowsUpdate to find out when new content is published to the Windows release health dashboard.
New! The Recommended section of the Start menu will show some Microsoft Store apps. These apps come from a small set of curated developers. This will help you to discover some of the great apps that are available. If you want to turn this off, go to Settings > Personalization > Start . Turn off the toggle for Show recommendations for tips, app promotions, and more .
New! In the coming weeks, your most frequently used apps might appear in the Recommended section of the Start menu. This applies to apps that you have not already pinned to the Start menu or the taskbar.
New! This update improves the Widgets icons on the taskbar. They are no longer pixelated or fuzzy. This update also starts the rollout of a larger set of animated icons.
New! This update affects Widgets on the lock screen. They are more reliable and have improved quality. This update also supports more visuals and a more customized experience for you.
This update affects the touch keyboard. It makes the Japanese 106 keyboard layout appear as expected when you sign in.
This update addresses an issue that affects Settings. It stops responding when you dismiss a flyout menu.
Improvements
Note: To view the list of addressed issues, click or tap the OS name to expand the collapsible section.
Important: Use EKB KB5027397 to update to Windows 11, version 23H2.
This non-security update includes quality improvements. Key changes include:
This build includes all the improvements in Windows 11, version 22H2.
No additional issues are documented for this release.
This non-security update includes quality improvements. When you install this KB:
This update adds a new mobile device management (MDM) policy called “AllowScreenRecorder.” It affects the Snipping Tool. IT admins can use this policy to turn off screen recording in the app.
This update adds support for Arm64 .msi files using a Group Policy Object (GPO). You can now use the Group Policy Management Console (GPMC) to add Arm64 .msi files. You can also use a GPO to install these files on Arm64 machines.
This update addresses an issue that affects the netstat -c command. It fails to perform effective port exhaustion troubleshooting.
This update addresses an issue that affects a low latency network. The speed of data on the network degrades significantly. This occurs when you turn on timestamps for a Transmission Control Protocol (TCP) connection.
This update addresses a race condition that might stop a machine from starting up. This occurs when you set up a bootloader to start up multiple OSes.
This update addresses an issue that affects an accelerator backing store management path. A memory leak occurs that affects some devices.
This update affects media allocations. It improves their memory granularity for some hardware setups. This lowers overcommitment. Also, performance is more efficient.
This update affects Windows Subsystem for Linux 2 (WSL2). Intermittent name resolution fails in a split DNS setup.
This update addresses an issue that affects universal printers. The system creates duplicate print queues for them.
This update makes Country and Operator Settings Asset (COSA) profiles up to date for some mobile operators.
This update addresses an issue that affects the container networking Address Resolution Protocol (ARP). It returns the wrong Virtual Subnet ID (VSID) for external ports.
This update addresses a memory allocation issue in the Host Networking Service (HNS). The issue causes high memory consumption. It also affects service and pod deployment and scaling.
This update addresses an issue that occurs when you elevate from a normal user to an Administrator to run an application. When you use a PIN to sign in, the app will not run.
This update affects hypervisor-protected code integrity (HVCI). It accepts drivers that are now compatible.
This update includes quarterly changes to the Windows Kernel Vulnerable Driver Blocklist file, DriverSiPolicy.p7b . It adds to the list of drivers that are at risk for Bring Your Own Vulnerable Driver (BYOVD) attacks.
This update addresses an issue that affects Protected Process Light (PPL) protections. You can bypass them.
This update addresses an issue that affects Bluetooth Advanced Audio Distribution Profile (A2DP) hardware offload. A stop error occurs on PCs that support it.
This update addresses an issue that affects the Distributed Transaction Coordinator (DTC). A memory leak occurs when it retrieves mappings.
This update addresses an issue that affects Windows Local Administrator Password Solution (LAPS). Its Post Authentication Actions (PAA) do not occur at the end of the grace period. Instead, they occur at restart.
This update addresses an issue that affects the Resilient File System (ReFS). A high load might make the system unresponsive. Also, signing in might be slow.
If you installed earlier updates, only the new updates contained in this package will be downloaded and installed on your device.
Windows 11 servicing stack update - 22621.3522 and 22631.3522
This update makes quality improvements to the servicing stack, which is the component that installs Windows updates. Servicing stack updates (SSU) ensure that you have a robust and reliable servicing stack so that your devices can receive and install Microsoft updates.
Known issues in this update
How to get this update.
Before installing this update
Microsoft combines the latest servicing stack update (SSU) for your operating system with the latest cumulative update (LCU). For general information about SSUs, see Servicing stack updates and Servicing Stack Updates (SSU): Frequently Asked Questions .
Install this update
If you want to remove the LCU
To remove the LCU after installing the combined SSU and LCU package, use the DISM/Remove-Package command line option with the LCU package name as the argument. You can find the package name by using this command: DISM /online /get-packages .
Running Windows Update Standalone Installer ( wusa.exe ) with the /uninstall switch on the combined package will not work because the combined package contains the SSU. You cannot remove the SSU from the system after installation.
File information
For a list of the files that are provided in this update, download the file information for cumulative update 5036980 .
For a list of the files that are provided in the servicing stack update, download the file information for the SSU - versions 22621.3522 and 22631.3522 .

Need more help?
Want more options.
Explore subscription benefits, browse training courses, learn how to secure your device, and more.
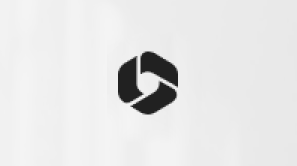
Microsoft 365 subscription benefits
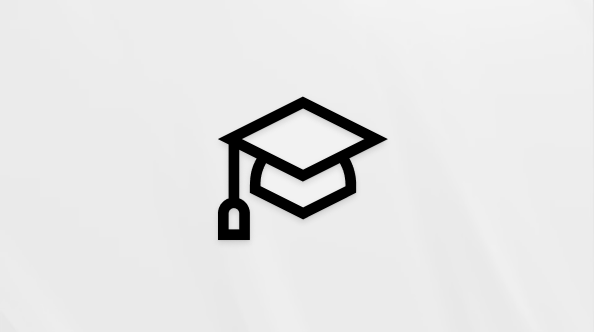
Microsoft 365 training
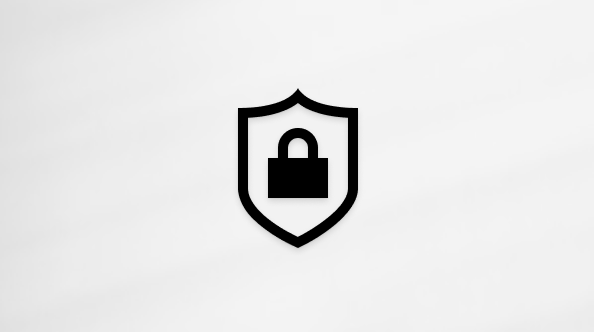
Microsoft security
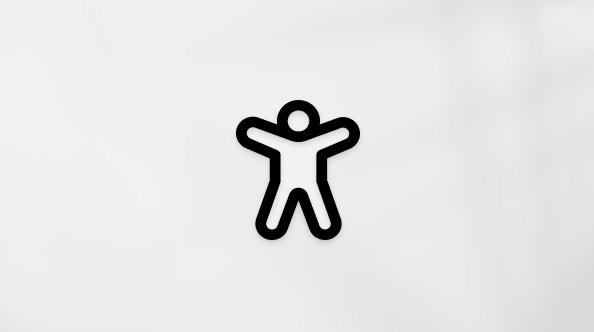
Accessibility center
Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.

Ask the Microsoft Community
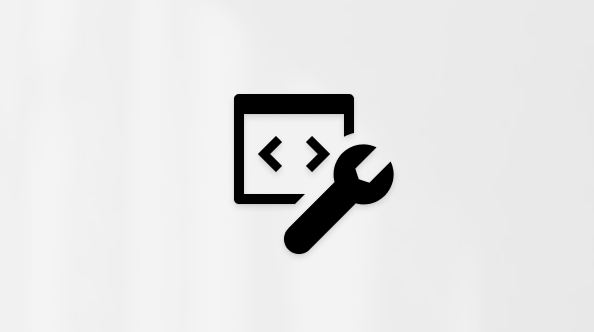
Microsoft Tech Community
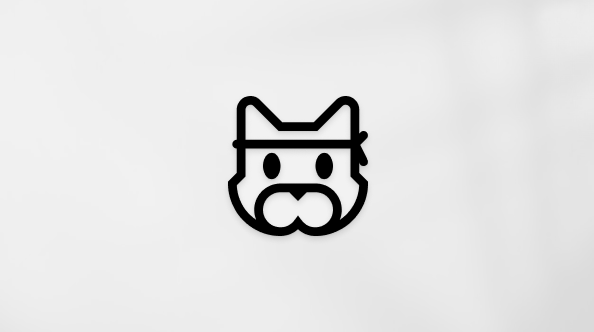
Windows Insiders
Microsoft 365 Insiders
Was this information helpful?
Thank you for your feedback.
- Election 2024
- Entertainment
- Newsletters
- Photography
- Personal Finance
- AP Investigations
- AP Buyline Personal Finance
- AP Buyline Shopping
- Press Releases
- Israel-Hamas War
- Russia-Ukraine War
- Global elections
- Asia Pacific
- Latin America
- Middle East
- Election Results
- Delegate Tracker
- AP & Elections
- Auto Racing
- 2024 Paris Olympic Games
- Movie reviews
- Book reviews
- Personal finance
- Financial Markets
- Business Highlights
- Financial wellness
- Artificial Intelligence
- Social Media
74-year-old Ohio woman charged in armed robbery of credit union was scam victim, family says
This image made from Fairfield Township, Ohio, Police Department body camera video shows Ann Mayers waiting outside of her home with police on Friday, April 19, 2024, in Hamilton, Ohio. The 74-year-old woman charged in the armed robbery of an Ohio credit union last week is a victim of an online scam who may have been trying to solve her financial problems, according to her relatives. Mayers, who had no previous run-ins with the law, faces counts of aggravated robbery with a firearm and tampering with evidence in Friday’s robbery in Fairfield Township. (Fairfield Township Police Department via AP)
- Copy Link copied
FAIRFIELD TOWNSHIP, Ohio (AP) — A 74-year-old woman charged in the armed robbery of an Ohio credit union last week is a victim of an online scam who may have been trying to solve her financial problems, according to her relatives.
Ann Mayers, who had no previous run-ins with the law, faces counts of aggravated robbery with a firearm and tampering with evidence in Friday’s robbery in Fairfield Township, north of Cincinnati. She remained jailed Wednesday on $100,000 bond pending an initial court appearance, and court records don’t list an attorney for her.
Officers arrested Mayers at her Hamilton home shortly after the robbery, Fairfield police said in a Facebook post . A handgun was found in her car, which police allege she used in the robbery.
Authorities later learned that Mayers might have been a scam victim and are looking into the claims. Her relatives told detectives that she had been sending money to an unidentified individual, The Columbus Dispatch reported Wednesday.
“In that aspect, some may see her as a ‘victim,’” Sgt. Brandon McCroskey told the newspaper. “Unfortunately, Ann chose to victimize several other people in the bank by robbing it with a firearm as a remedy for her situation.”
If what her relatives say is true, McCroskey called Mayers’ situation “very sad and unfortunate.” He said she reportedly spoke with family members about robbing banks in the days leading up to the holdup, but they didn’t take her comments seriously.
Scams against seniors have become increasingly common over the last 10 to 15 years, according to experts. Among them are so-called grandparent scams in which callers claim to be anyone from a victim’s grandchild to a police officer and tells the victim something terrible happened and that their younger relative needs money.
The scammers seek to exploit older people’s love for their family and, experts note, they can succeed in part because they tap into the abundance of personal information available about people online and use it make seniors believe the calls are legitimate.
An 81-year-old Ohio man was charged with murder this month after he fatally shot an Uber driver because he wrongly assumed she was part of a scheme to extract $12,000 in supposed bond money for a relative, authorities have said.
The driver was a victim of the same con, summoned by the grifters to the man’s house to retrieve a purported package for delivery. The man later told investigators he believed Hall had arrived to get the money the scammers wanted.
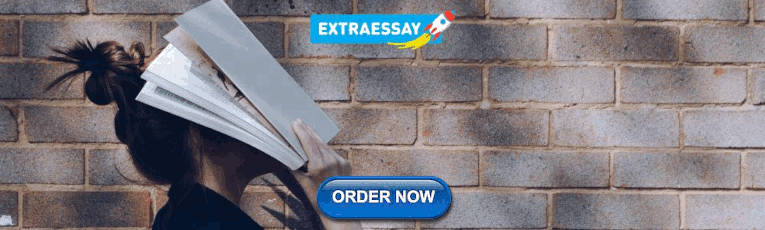
IMAGES
VIDEO
COMMENTS
Solve Challenge. Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews.
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
Q2: Write a Program to find the Sum of two numbers entered by the user. In this problem, you have to write a program that adds two numbers and prints their sum on the console screen. For Example, Input: Enter two numbers A and B : 5 2. Output: Sum of A and B is: 7.
C Programs: Practicing and solving problems is the best way to learn anything. Here, we have provided 100+ C programming examples in different categories like basic C Programs, Fibonacci series in C, String, Array, Base Conversion, Pattern Printing, Pointers, etc. These C programs are the most asked interview questions from basic to advanced level.
Matrix (2D array) Add two matrices. Scalar matrix multiplication. Multiply two matrices. Check if two matrices are equal. Sum of diagonal elements of matrix. Interchange diagonal of matrix. Find upper triangular matrix. Find sum of lower triangular matrix.
Practice C. Practice C problems, a great starting point if you really want to understand fundamental programming constructs. 4.4 (1758 reviews) 33 Problems Beginner level. 29.2k Learners.
Learn C Programming and solve online C Programming Practice Question with CodeChef. Click here and get all aspects of C Programming Language from the basics concepts to advance level for all sort of competitive exams. ... Dynamic programming tutorials here are more diverse with more problems, more solutions, and more information than most of ...
Use C to kickstart your journey in the world of logic building, basic data structures and algorithms. Courses. All Roadmaps 9 roadmaps. Learn Python 7 courses. Learn C++ 11 courses. Learn C 8 courses. Learn Java 9 courses. Data Structures and Algorithms 4 courses. Data Analytics 3 courses. Web ...
Specialization - 4 course series. This specialization develops strong programming fundamentals for learners who want to solve complex problems by writing computer programs. Through four courses, you will learn to develop algorithms in a systematic way and read and write the C code to implement them. This will prepare you to pursue a career in ...
This self-readable and student-friendly text provides a strong programming foundation to solve problems with C language through its well-supported structured programming methodology, rich set of operators and data types. It is designed to help students build efficient and compact programs. The book, now in its second edition, is an extended version of Dr. M.T. Somashekara's previous book ...
Learners enrolled: 29073. ABOUT THE COURSE : This course is aimed at enabling the students to. Formulate simple algorithms for arithmetic and logical problems. Translate the algorithms to programs (in C language) Test and execute the programs and correct syntax and logical errors. Implement conditional branching, iteration and recursion.
The best way to learn C programming is by practicing and solving the C programs (C problems). We have 1000+ C programs with solutions which are categorized below. Practice these C programs to learn and enhance your C problem-solving skills.
Practice these C programs to learn and enhance your C problem-solving skills. If you have mastered programming, you must have experienced the beginning of problem solving or solving problems, which is a set of prohibited questions that measure your understanding and programming thinking. Sometimes it is not sufficient just to cope with problems ...
Note: Practice C Programs for problem solving through programming in C. Problem Solving Steps. Problem-solving is a creative process which defines systematization and mechanization. There are a number of steps that can be taken to raise the level of one's performance in problem-solving. A problem-solving technique follows certain steps in ...
1. Write a C program to get the indices of two numbers in a given array of integers. This will enable you to get the sum of two numbers equal to a specific target. Expected Output: Original Array: 4 2 1 5 Target Value: 7 Indices of the two numbers whose sum equal to target value: 7 1 3. Click me to see the solution. 2.
03. user input scanf() problem [13-15] 04. apply your conditions
Boost your coding interview skills and confidence by practicing real interview questions with LeetCode. Our platform offers a range of essential problems for practice, as well as the latest questions being asked by top-tier companies.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
In this post, we've gone over the four-step problem-solving strategy for solving coding problems. Let's review them here: Step 1: understand the problem. Step 2: create a step-by-step plan for how you'll solve it. Step 3: carry out the plan and write the actual code.
Jones and Harrow present programming concepts in the context of solving problems. Each chapter introduces a problem first, and then covers the C language elements needed to solve it. Students can see how a program is built from its simplest beginning to its final polished form. This book introduces beginning programming concepts using the C language.
Problem solving through Programming In C. ABOUT THE COURSE This course is aimed at enabling the students to. ·formulate simple algorithms for arithmetic and logical problems·translate the algorithms to programs (in C language)·test and execute the programs and correct syntax and logical errors·implement conditional branching, iteration and ...
Here are HackerRank C All Problems solutions with practical programs and code in C Programming language. if you need help, comment with your queries and questions in the comment section on particular problem solutions. HackerRank C All Problems Solutions;
Share your videos with friends, family, and the world
You might consider pursuing a second degree concurrently if you're interested in how the world's public challenges can be addressed at the intersection of business, law, medicine, design, or other fields.. Pursuing a concurrent degree reduces coursework and residency requirements and makes it possible to earn two degrees in a shorter amount of time.
The new presidential helicopter has been demoted to backup duty because Lockheed Martin Corp. still can't figure out how to keep it from scorching the White House's South Lawn.
"The danger of trying to copy 20th century models from other countries is that you're missing what the actual problem is." The more immediate challenge is convincing consumers to try longer ...
This update addresses an issue that affects the netstat -c command. It fails to perform effective port exhaustion troubleshooting. This update addresses an issue that affects a low latency network. The speed of data on the network degrades significantly. This occurs when you turn on timestamps for a Transmission Control Protocol (TCP) connection.
An 81-year-old Ohio man was charged with murder this month after he fatally shot an Uber driver because he wrongly assumed she was part of a scheme to extract $12,000 in supposed bond money for a relative, authorities have said.. The driver was a victim of the same con, summoned by the grifters to the man's house to retrieve a purported package for delivery.