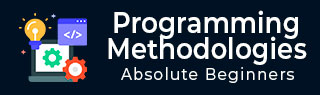
- Programming Methodologies
- Introduction
- Understanding the Problem
- Identifying the Solution
- Applying Modular Techniques
- Writing the Algorithm
- Flowchart Elements
- Using Clear Instructions
- Correct Programming Techniques
- Program Documentation
- Program Maintenance
- Useful Resources
- Quick Guide
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
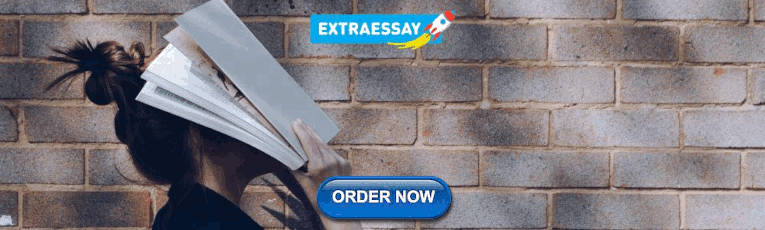
Programming Methodologies - Introduction
When programs are developed to solve real-life problems like inventory management, payroll processing, student admissions, examination result processing, etc. they tend to be huge and complex. The approach to analyzing such complex problems, planning for software development and controlling the development process is called programming methodology .
Types of Programming Methodologies
There are many types of programming methodologies prevalent among software developers −
Procedural Programming
Problem is broken down into procedures, or blocks of code that perform one task each. All procedures taken together form the whole program. It is suitable only for small programs that have low level of complexity.
Example − For a calculator program that does addition, subtraction, multiplication, division, square root and comparison, each of these operations can be developed as separate procedures. In the main program each procedure would be invoked on the basis of user’s choice.
Object-oriented Programming
Here the solution revolves around entities or objects that are part of problem. The solution deals with how to store data related to the entities, how the entities behave and how they interact with each other to give a cohesive solution.
Example − If we have to develop a payroll management system, we will have entities like employees, salary structure, leave rules, etc. around which the solution must be built.
Functional Programming
Here the problem, or the desired solution, is broken down into functional units. Each unit performs its own task and is self-sufficient. These units are then stitched together to form the complete solution.
Example − A payroll processing can have functional units like employee data maintenance, basic salary calculation, gross salary calculation, leave processing, loan repayment processing, etc.
Logical Programming
Here the problem is broken down into logical units rather than functional units. Example: In a school management system, users have very defined roles like class teacher, subject teacher, lab assistant, coordinator, academic in-charge, etc. So the software can be divided into units depending on user roles. Each user can have different interface, permissions, etc.
Software developers may choose one or a combination of more than one of these methodologies to develop a software. Note that in each of the methodologies discussed, problem has to be broken down into smaller units. To do this, developers use any of the following two approaches −
- Top-down approach
- Bottom-up approach
Top-down or Modular Approach
The problem is broken down into smaller units, which may be further broken down into even smaller units. Each unit is called a module . Each module is a self-sufficient unit that has everything necessary to perform its task.
The following illustration shows an example of how you can follow modular approach to create different modules while developing a payroll processing program.
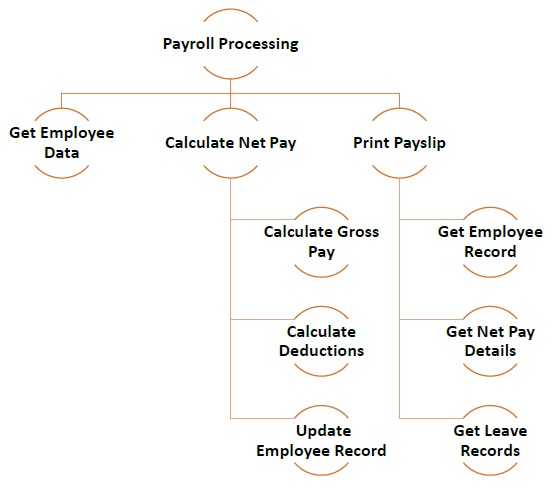
Bottom-up Approach
In bottom-up approach, system design starts with the lowest level of components, which are then interconnected to get higher level components. This process continues till a hierarchy of all system components is generated. However, in real-life scenario it is very difficult to know all lowest level components at the outset. So bottoms up approach is used only for very simple problems.
Let us look at the components of a calculator program.
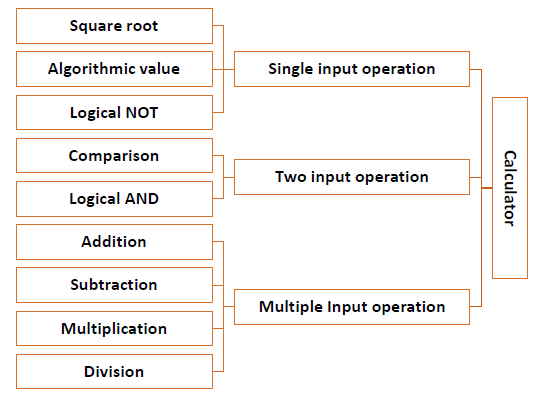
To Continue Learning Please Login
What exactly is a programming paradigm?
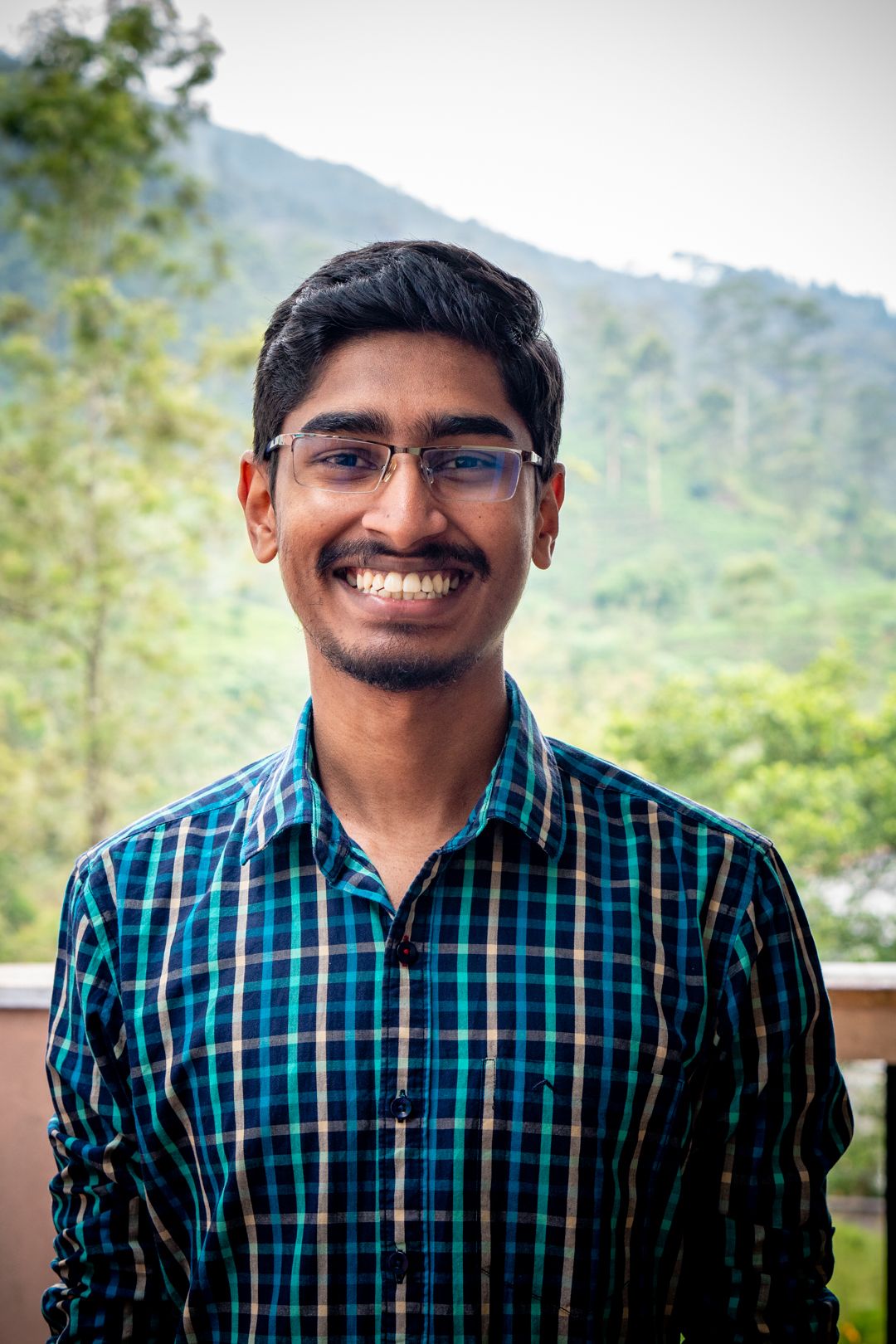
Any fool can write code that a computer can understand. Good programmers write code that humans can understand. ― Martin Fowler
When programming, complexity is always the enemy. Programs with great complexity, with many moving parts and interdependent components, seem initially impressive. However, the ability to translate a real-world problem into a simple or elegant solution requires a deeper understanding.
While developing an application or solving a simple problem, we often say “If I had more time, I would have written a simpler program”. The reason is, we made a program with greater complexity. The less complexity we have, the easier it is to debug and understand. The more complex a program becomes, the harder it is to work on it.
Managing complexity is a programmer’s main concern . So how do programmers deal with complexity? There are many general approaches that reduce complexity in a program or make it more manageable. One of the main approaches is a programming paradigm. Let's dive into programming paradigms!
Introduction to programming paradigms
The term programming paradigm refers to a style of programming . It does not refer to a specific language, but rather it refers to the way you program.
There are lots of programming languages that are well-known but all of them need to follow some strategy when they are implemented. And that strategy is a paradigm.
The types of programming paradigms

Imperative programming paradigm
The word “imperative” comes from the Latin “impero” meaning “I command”.
It’s the same word we get “emperor” from, and that’s quite apt. You’re the emperor. You give the computer little orders to do and it does them one at a time and reports back.
The paradigm consists of several statements, and after the execution of all of them, the result is stored. It’s about writing a list of instructions to tell the computer what to do step by step.
In an imperative programming paradigm, the order of the steps is crucial, because a given step will have different consequences depending on the current values of variables when the step is executed.
To illustrate, let's find the sum of first ten natural numbers in the imperative paradigm approach.
Example in C:
In the above example, we are commanding the computer what to do line by line. Finally, we are storing the value and printing it.
1.1 Procedural programming paradigm
Procedural programming (which is also imperative) allows splitting those instructions into procedures .
NOTE: Procedures aren't functions. The difference between them is that functions return a value, and procedures do not. More specifically, functions are designed to have minimal side effects, and always produce the same output when given the same input. Procedures, on the other hand, do not have any return value. Their primary purpose is to accomplish a given task and cause a desired side effect.
A great example of procedures would be the well known for loop. The for loop's main purpose is to cause side effects and it does not return a value.
To illustrate, let's find the sum of first ten natural numbers in the procedural paradigm approach.
In the example above, we've used a simple for loop to find the summation of the first ten natural numbers.
Languages that support the procedural programming paradigm are:
Procedural programming is often the best choice when:
- There is a complex operation which includes dependencies between operations, and when there is a need for clear visibility of the different application states ('SQL loading', 'SQL loaded', 'Network online', 'No audio hardware', etc). This is usually appropriate for application startup and shutdown (Holligan, 2016).
- The program is very unique and few elements were shared (Holligan, 2016).
- The program is static and not expected to change much over time (Holligan, 2016).
- None or only a few features are expected to be added to the project over time (Holligan, 2016).
Why should you consider learning the procedural programming paradigm?
- It's simple.
- An easier way to keep track of program flow.
- It has the ability to be strongly modular or structured.
- Needs less memory: It's efficient and effective.
1.2 Object-oriented programming paradigm
OOP is the most popular programming paradigm because of its unique advantages like the modularity of the code and the ability to directly associate real-world business problems in terms of code.
Object-oriented programming offers a sustainable way to write spaghetti code. It lets you accrete programs as a series of patches. ― Paul Graham
The key characteristics of object-oriented programming include Class, Abstraction, Encapsulation, Inheritance and Polymorphism.
A class is a template or blueprint from which objects are created.

Objects are instances of classes. Objects have attributes/states and methods/behaviors. Attributes are data associated with the object while methods are actions/functions that the object can perform.

Abstraction separates the interface from implementation. Encapsulation is the process of hiding the internal implementation of an object.
Inheritance enables hierarchical relationships to be represented and refined. Polymorphism allows objects of different types to receive the same message and respond in different ways.
To illustrate, let's find the sum of first ten natural numbers in the object-oriented paradigm approach.
Example in Java:
We have a class Addition that has two states, sum and num which are initialized to zero. We also have a method addValues() which returns the sum of num numbers.
In the Main class, we've created an object, obj of Addition class. Then, we've initialized the num to 10 and we've called addValues() method to get the sum.
Languages that support the object-oriented paradigm:
Object-oriented programming is best used when:
- You have multiple programmers who don’t need to understand each component (Holligan, 2016).
- There is a lot of code that could be shared and reused (Holligan, 2016).
- The project is anticipated to change often and be added to over time (Holligan, 2016).
Why should you consider learning the object-oriented programming paradigm?
- Reuse of code through Inheritance.
- Flexibility through Polymorphism.
- High security with the use of data hiding (Encapsulation) and Abstraction mechanisms.
- Improved software development productivity: An object-oriented programmer can stitch new software objects to make completely new programs (The Saylor Foundation, n.d.).
- Faster development: Reuse enables faster development (The Saylor Foundation, n.d.).
- Lower cost of development: The reuse of software also lowers the cost of development. Typically, more effort is put into the object-oriented analysis and design (OOAD), which lowers the overall cost of development (The Saylor Foundation, n.d.).
- Higher-quality software: Faster development of software and lower cost of development allows more time and resources to be used in the verification of the software. Object-oriented programming tends to result in higher-quality software (The Saylor Foundation, n.d.).
1.3 Parallel processing approach
Parallel processing is the processing of program instructions by dividing them among multiple processors.
A parallel processing system allows many processors to run a program in less time by dividing them up.
Languages that support the Parallel processing approach:
- NESL (one of the oldest ones)
Parallel processing approach is often the best use when:
- You have a system that has more than one CPU or multi-core processors which are commonly found on computers today.
- You need to solve some computational problems that take hours/days to solve even with the benefit of a more powerful microprocessor.
- You work with real-world data that needs more dynamic simulation and modeling.
Why should you consider learning the parallel processing approach?
- Speeds up performance.
- Often used in Artificial Intelligence. Learn more here: Artificial Intelligence and Parallel Processing by Seyed H. Roosta.
- It makes it easy to solve problems since this approach seems to be like a divide and conquer method.
Here are some useful resources to learn more about parallel processing:
- Parallel Programming in C by Paul Gribble
- Introduction to Parallel Programming with MPI and OpenMP by Charles Augustine
- INTRODUCTION TO PARALLEL PROGRAMMING WITH MPI AND OPENMP by Benedikt Steinbusch
2. Declarative programming paradigm
Declarative programming is a style of building programs that expresses the logic of a computation without talking about its control flow.
Declarative programming is a programming paradigm in which the programmer defines what needs to be accomplished by the program without defining how it needs to be implemented. In other words, the approach focuses on what needs to be achieved instead of instructing how to achieve it.
Imagine the president during the state of the union declaring their intentions for what they want to happen. On the other hand, imperative programming would be like a manager of a McDonald's franchise. They are very imperative and as a result, this makes everything important. They, therefore, tell everyone how to do everything down to the simplest of actions.
So the main differences are that imperative tells you how to do something and declarative tells you what to do .
2.1 Logic programming paradigm
The logic programming paradigm takes a declarative approach to problem-solving. It's based on formal logic.
The logic programming paradigm isn't made up of instructions - rather it's made up of facts and clauses. It uses everything it knows and tries to come up with the world where all of those facts and clauses are true.
For instance, Socrates is a man, all men are mortal, and therefore Socrates is mortal.
The following is a simple Prolog program which explains the above instance:
The first line can be read, "Socrates is a man.'' It is a base clause , which represents a simple fact.
The second line can be read, "X is mortal if X is a man;'' in other words, "All men are mortal.'' This is a clause , or rule, for determining when its input X is "mortal.'' (The symbol ":-'', sometimes called a turnstile , is pronounced "if''.) We can test the program by asking the question:
that is, "Is Socrates mortal?'' (The " ?- '' is the computer's prompt for a question). Prolog will respond " yes ''. Another question we may ask is:
That is, "Who (X) is mortal?'' Prolog will respond " X = Socrates ''.
To give you an idea, John is Bill's and Lisa's father. Mary is Bill's and Lisa's mother. Now, if someone asks a question like "who is the father of Bill and Lisa?" or "who is the mother of Bill and Lisa?" we can teach the computer to answer these questions using logic programming.
Example in Prolog:
Example explained:
The above code defines that John is Bill's father.
We're asking Prolog what value of X makes this statement true? X should be Mary to make the statement true. It'll respond X = Mary
Languages that support the logic programming paradigm:
- ALF (algebraic logic functional programming language)
Logic programming paradigm is often the best use when:
- If you're planning to work on projects like theorem proving, expert systems, term rewriting, type systems and automated planning.
Why should you consider learning the logic programming paradigm?
- Easy to implement the code.
- Debugging is easy.
- Since it's structured using true/false statements, we can develop the programs quickly using logic programming.
- As it's based on thinking, expression and implementation, it can be applied in non-computational programs too.
- It supports special forms of knowledge such as meta-level or higher-order knowledge as it can be altered.
2.2 Functional programming paradigm
The functional programming paradigm has been in the limelight for a while now because of JavaScript, a functional programming language that has gained more popularity recently.
The functional programming paradigm has its roots in mathematics and it is language independent. The key principle of this paradigm is the execution of a series of mathematical functions.
You compose your program of short functions. All code is within a function. All variables are scoped to the function.
In the functional programming paradigm, the functions do not modify any values outside the scope of that function and the functions themselves are not affected by any values outside their scope.
To illustrate, let's identify whether the given number is prime or not in the functional programming paradigm.
Example in JavaScript:
In the above example, we've used Math.floor() and Math.sqrt() mathematical functions to solve our problem efficiently. We can solve this problem without using built-in JavaScript mathematical functions, but to run the code efficiently it is recommended to use built-in JS functions.
number is scoped to the function isPrime() and it will not be affected by any values outside its scope. isPrime() function always produces the same output when given the same input.
NOTE: there are no for and while loops in functional programming. Instead, functional programming languages rely on recursion for iteration (Bhadwal, 2019).
Languages that support functional programming paradigm:
Functional programming paradigm is often best used when:
- Working with mathematical computations.
- Working with applications aimed at concurrency or parallelism.
Why should you consider learning the functional programming paradigm?
- Functions can be coded quickly and easily.
- General-purpose functions can be reusable which leads to rapid software development.
- Unit testing is easier.
- Debugging is easier.
- Overall application is less complex since functions are pretty straightforward.
2.3 Database processing approach
This programming methodology is based on data and its movement. Program statements are defined by data rather than hard-coding a series of steps.
A database is an organized collection of structured information, or data, typically stored electronically in a computer system. A database is usually controlled by a database management system (DBMS) ("What is a Database", Oracle, 2019).
To process the data and querying them, databases use tables . Data can then be easily accessed, managed, modified, updated, controlled and organized.
A good database processing approach is crucial to any company or organization. This is because the database stores all the pertinent details about the company such as employee records, transaction records and salary details.
Most databases use Structured Query Language (SQL) for writing and querying data.
Here’s an example in database processing approach (SQL):
The PersonID column is of type int and will hold an integer. The LastName , FirstName , Address , and City columns are of type varchar and will hold characters, and the maximum length for these fields is 255 characters.
The empty Persons table will now look like this:

Database processing approach is often best used when:
- Working with databases to structure them.
- Accessing, modifying, updating data on the database.
- Communicating with servers.
Why are databases important and why should you consider learning database processing approach?
- Massive amount of data is handled by the database: Unlike spreadsheet or other tools, databases are used to store large amount of data daily.
- Accurate: With the help of built-in functionalities in a database, we can easily validate.
- Easy to update data: Data Manipulation Languages (DML) such as SQL are used to update data in a database easily.
- Data integrity: With the help of built-in validity checks, we can ensure the consistency of data.
Programming paradigms reduce the complexity of programs. Every programmer must follow a paradigm approach when implementing their code. Each one has its advantages and disadvantages .
If you're a beginner, I would like to suggest learning object-oriented programming and functional programming first. Understand their concepts and try to apply them in your projects.
For example, if you're learning object-oriented programming, the pillars of object-oriented programming are Encapsulation, Abstraction, Inheritance and Polymorphism. Learn them by doing it. It will help you to understand their concepts on a deeper level, and your code will be less complex and more efficient and effective.
I strongly encourage you to read more related articles on programming paradigms. I hope this article helped you.
Please feel free to let me know if you have any questions.
You can contact and connect with me on Twitter @ThanoshanMV .
Thank you for reading.
Happy Coding!
- Akhil Bhadwal. (2019). Functional Programming: Concepts, Advantages, Disadvantages, and Applications
- Alena Holligan. (2016). When to use OOP over procedural coding
- The Saylor Foundation. (n.d.). Advantages and Disadvantages of Object-Oriented Programming (OOP)
- What is a Database | Oracle. (2019).
System.out.println("Hey there, I am Thanoshan!");
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Enterprise Java
- Web-based Java
- Data & Java
- Project Management
- Visual Basic
- Ruby / Rails
- Java Mobile
- Architecture & Design
- Open Source
- Web Services

Developer.com content and product recommendations are editorially independent. We may make money when you click on links to our partners. Learn More .
The software development methodologies listed in this software development tutorial aim to improve productivity, code quality, and collaboration.
Once upon a time, nearly all software development projects utilized the waterfall model. Much like the assembly line in a factory, this programming methodology requires developers to complete one phase of development before moving on to the next. It is highly structured and does not work well when the project requirements are in flux.
In recent years, developers have begun favoring more iterative processes that make it easier to accommodate changes in the project scope and requirements. Agile software development and its seemingly infinite number of variations have become increasingly common and, according to some surveys, now dominate.
In this guide to software development methodologies, we will take a look at the features of ten of the most popular software development approaches, including Agile , Scrum , Lean, Extreme programming and, yes, even the waterfall method .

Software Development Methodologies
These programming methodologies aim to improve productivity, code quality and collaboration.
Check out these Project Management courses at TechRepublic Academy!
1. Agile Software Development

Image source: Agilemanifesto.org
In 2001, seventeen software developers made history by signing the Agile Manifesto. Since then, agile software development has taken off. The Agile philosophy is based on twelve core principles that emphasize short iterations, continuous delivery, simplicity, retrospection, and collaboration between end-users and developers.
Read: A Deep Dive into Design Thinking with Agile Development
For further learning into Agile, check out this course !
2. Scrum Software Development Methodology
Agile software development comes in many flavors, and Scrum is one of the most popular with 70 percent of respondents to the State of Agile report saying that they practice Scrum or a Scrum hybrid. It is a framework for collaboration that was first invented by Jeff Sutherland in 1993. It divides complex projects into short two-to-four-week sprints, and it emphasizes the values of courage, focus, commitment, respect, and openness.
Read: What is a Scrum Team?
3. Lean Software Development

Although lean development is commonly associated with agile, the principles of lean actually arose from Toyota’s lean manufacturing processes. This development methodology relies on seven key principles: eliminate waste, increase feedback, delay commitment, deliver fast, build integrity in, empower the team and see the whole. Lean first came to the attention of the software development community in 2003 with the publication of Mary and Tom Poppendieck’s book “Lean Software Development: An Agile Toolkit.”
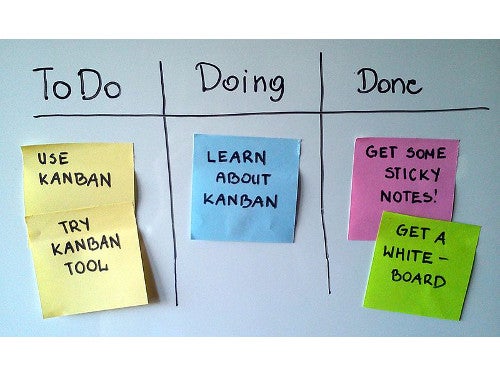
Another variation of agile software development that was inspired by Toyota, Kanban gives developers a visual way to see what work needs to be done and allows them to “pull” in work as they have capacity rather than “pushing” them to complete certain tasks. It relies on three core principles: visualize what you do today, limit the amount of work in progress and enhance flow.
Read more about Kanban in our tutorial: Overview of Kanban for Project Managers and Developers .
5. Rapid Application Development (RAD)
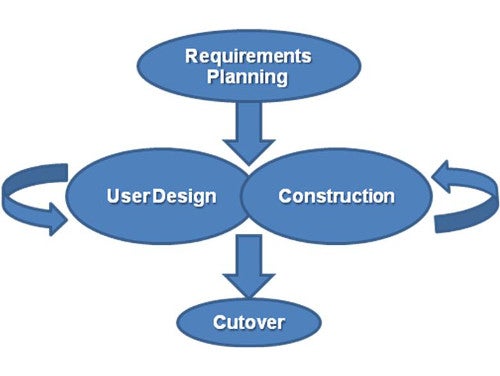
Over the years, several different approaches to software development have used the RAD name. The best-known is probably James Martin’s methodology, which was created at IBM in the 1980s. It can be considered a form of agile, as it emphasizes the ability to adapt to changing requirements and de-emphasizes upfront planning.
6. Test-Driven Development (TDD)

Test-Driven Development is related to both agile software development and extreme programming. Developed by Kent Beck and others, this process requires developers to write a test for any new feature before starting the coding process. It encourages developers to write a minimal amount of code.
7. Extreme Programming
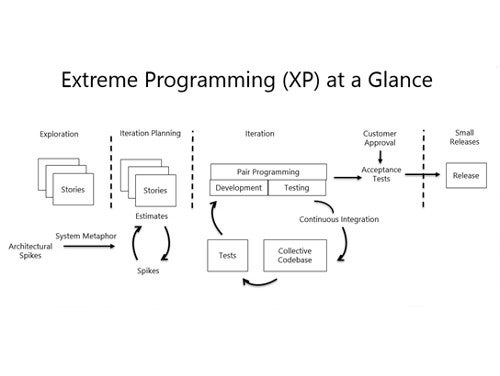
This form of agile software development – known as Extreme Programming or XP – relies heavily on pair programming. Like other agile methodologies, it emphasizes rapid iterations and frequent requirement changes. It was created by Kent Beck, who was one of the signatories of the Agile Manifesto and published a book called “Extreme Programming Explained: Embrace Change” in 1999.
Read: What is Extreme Programming?
8. Rational Unified Process
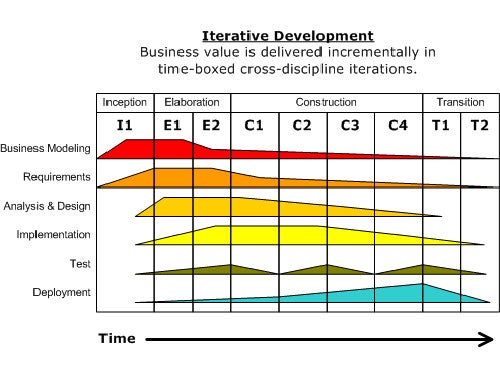
The Rational Unified Process software development methodology is named for the company that invented it—Rational Software, which IBM purchased in 2003. While some programming methodologies are very rigid, the Rational Unified Process aims to be easily tailored to unique situations. It’s an iterative framework that relies heavily on visual models.
9. Spiral Model
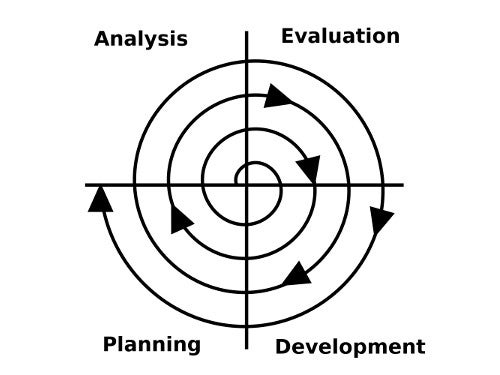
First described in the mid-1980s by Barry Boehm, the spiral model is a risk-driven model that incorporates elements of waterfall, incremental, prototyping and other software development approaches. It says that developers should make decisions based on the level of risk and that they should only do enough work to minimize the risk.
Read: Best Agile Software Strategies
10. Waterfall Model
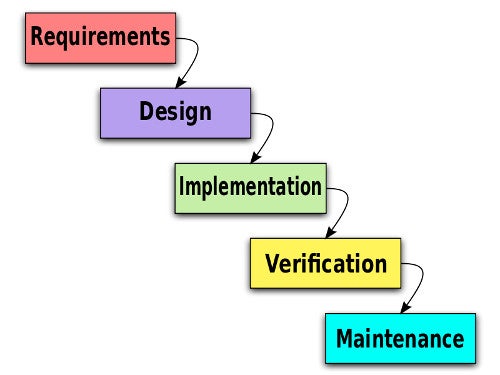
Unlike the other software development methodologies in this slideshows, the waterfall model is sequential rather than iterative. It was the most common method of software development from the early days of computing until very recently. It is best suited to small-scale projects where all of the design requirements can be known upfront.
Read: Best Project Management Tools for Developers
Featured Partners: Project Management Software

Visit website
monday.com Work OS is the project management software that helps you and your team plan, execute, and track projects and workflows in one collaborative space. Manage everything from simple to complex projects more efficiently with the help of visual boards, 200+ ready-made templates, clever no-code automations, and easy integrations. In addition, custom dashboards simplify reporting, so you can evaluate your progress and make data-driven decisions.
Learn more about monday.com

Big ideas aren’t simple to execute. So we’re here to help you tackle any project, no matter how complex. We’re Quickbase. Quickbase helps customers see, connect and control complex projects that reshape our world. Whether it’s raising a skyscraper or coordinating vaccine rollouts, the no-code software platform allows business users to custom fit solutions to the way they work – using information from across the systems they already have.
Learn more about Quickbase

Wrike’s top-notch workflow management software allows you to easily visualize priorities, boost collaboration, and maintain control of your projects. Bonus: you can move seamlessly between apps, without logging in or out. Wrike has more than 400+ integrations with popular platforms such as Google, Dropbox, Microsoft Office, and many more. Automation and AI features strip away time-consuming admin tasks so you can do the best work of your life.
Learn more about Wrike
Get the Free Newsletter!
Subscribe to Developer Insider for top news, trends & analysis
Latest Posts
What is the role of a project manager in software development, how to use optional in java, overview of the jad methodology, microsoft project tips and tricks, how to become a project manager in 2023, related stories.
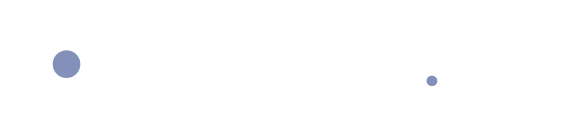
Object-oriented programming (OOP) is a fundamental programming paradigm used by nearly every developer at some point in their career. OOP is the most popular programming paradigm used for software development and is taught as the standard way to code for most of a programmer’s educational career. Another popular programming paradigm is functional programming, but we won’t get into that right now.
Today we will break down the basics of what makes a program object-oriented so that you can start to utilize this paradigm in your algorithms, projects, and interviews.
Now, let’s dive into these OOP concepts and tutorials!
Here’s what will be covered:
What is Object-Oriented Programming?
Building blocks of oop.
- Four principles of OOP
- What to learn next
Object-Oriented Programming (OOP) is a programming paradigm in computer science that relies on the concept of classes and objects . It is used to structure a software program into simple, reusable pieces of code blueprints (usually called classes), which are used to create individual instances of objects. There are many object-oriented programming languages, including JavaScript, C++ , Java , and Python .
OOP languages are not necessarily restricted to the object-oriented programming paradigm. Some languages, such as JavaScript, Python, and PHP, all allow for both procedural and object-oriented programming styles.
A class is an abstract blueprint that creates more specific, concrete objects. Classes often represent broad categories, like Car or Dog that share attributes . These classes define what attributes an instance of this type will have, like color , but not the value of those attributes for a specific object.
Classes can also contain functions called methods that are available only to objects of that type. These functions are defined within the class and perform some action helpful to that specific object type.
For example, our Car class may have a repaint method that changes the color attribute of our car. This function is only helpful to objects of type Car , so we declare it within the Car class, thus making it a method.
Class templates are used as a blueprint to create individual objects . These represent specific examples of the abstract class, like myCar or goldenRetriever . Each object can have unique values to the properties defined in the class.
For example, say we created a class, Car , to contain all the properties a car must have, color , brand , and model . We then create an instance of a Car type object, myCar to represent my specific car. We could then set the value of the properties defined in the class to describe my car without affecting other objects or the class template. We can then reuse this class to represent any number of cars.
Benefits of OOP for software engineering
- OOP models complex things as reproducible, simple structures
- Reusable, OOP objects can be used across programs
- Polymorphism allows for class-specific behavior
- Easier to debug, classes often contain all applicable information to them
- Securely protects sensitive information through encapsulation
How to structure OOP programs
Let’s take a real-world problem and conceptually design an OOP software program.
Imagine running a dog-sitting camp with hundreds of pets where you keep track of the names, ages, and days attended for each pet.
How would you design simple, reusable software to model the dogs?
With hundreds of dogs, it would be inefficient to write unique entries for each dog because you would be writing a lot of redundant code. Below we see what that might look like with objects rufus and fluffy .
As you can see above, there is a lot of duplicated code between both objects. The age() function appears in each object. Since we want the same information for each dog, we can use objects and classes instead.
Grouping related information together to form a class structure makes the code shorter and easier to maintain.
In the dogsitting example, here’s how a programmer could think about organizing an OOP:
- Create a class for all dogs as a blueprint of information and behaviors (methods) that all dogs will have, regardless of type. This is also known as the parent class .
- Create subclasses to represent different subcategories of dogs under the main blueprint. These are also referred to as child classes .
- Add unique attributes and behaviors to the child classes to represent differences
- Create objects from the child class that represent dogs within that subgroup
The diagram below represents how to design an OOP program by grouping the related data and behaviors together to form a simple template and then creating subgroups for specialized data and behavior.
The Dog class is a generic template containing only the structure of data and behaviors common to all dogs as attributes.
We then create two child classes of Dog , HerdingDog and TrackingDog . These have the inherited behaviors of Dog ( bark() ) but also behavior unique to dogs of that subtype.
Finally, we create objects of the HerdingDog type to represent the individual dogs Fluffy and Maisel .
We can also create objects like Rufus that fit under the broad class of Dog but do not fit under either HerdingDog or TrackingDog .
Next, we’ll take a deeper look at each of the fundamental building blocks of an OOP program used above:
In a nutshell, classes are essentially user-defined data types . Classes are where we create a blueprint for the structure of methods and attributes. Individual objects are instantiated from this blueprint.
Classes contain fields for attributes and methods for behaviors. In our Dog class example, attributes include name & birthday , while methods include bark() and updateAttendance() .
Here’s a code snippet demonstrating how to program a Dog class using the JavaScript language.
Remember, the class is a template for modeling a dog, and an object is instantiated from the class representing an individual real-world item.
Enjoying the article? Scroll down to sign up for our free, bi-monthly newsletter.
Objects are, unsurprisingly, a huge part of OOP! Objects are instances of a class created with specific data. For example, in the code snippet below, Rufus is an instance of the Dog class.
When the new class Dog is called:
- A new object is created named rufus
- The constructor runs name & birthday arguments, and assigns values
Programming vocabulary: In JavaScript, objects are a type of variable. This may cause confusion because objects can be declared without a class template in JavaScript, as shown at the beginning. Objects have states and behaviors. The state of an object is defined by data: things like names, birthdates, and other information you’d want to store about a dog. Behaviors are methods the object can undertake.
Attributes are the information that is stored. Attributes are defined in the Class template. When objects are instantiated, individual objects contain data stored in the Attributes field.
The state of an object is defined by the data in the object’s attributes fields. For example, a puppy and a dog might be treated differently at a pet camp. The birthday could define the state of an object and allow the software to handle dogs of different ages differently.
Methods represent behaviors. Methods perform actions; methods might return information about an object or update an object’s data. The method’s code is defined in the class definition.
When individual objects are instantiated, these objects can call the methods defined in the class. In the code snippet below, the bark method is defined in the Dog class, and the bark() method is called on the Rufus object.
Methods often modify, update or delete data. Methods don’t have to update data though. For example, the bark() method doesn’t update any data because barking doesn’t modify any of the attributes of the Dog class: name or birthday .
The updateAttendance() method adds a day the Dog attended the pet-sitting camp. The attendance attribute is important to keep track of for billing Owners at the end of the month.
Methods are how programmers promote reusability and keep functionality encapsulated inside an object. This reusability is a great benefit when debugging. If there’s an error, there’s only one place to find it and fix it instead of many.
The underscore in _attendance denotes that the variable is protected and shouldn’t be modified directly. The updateAttendance() method changes _attendance .
Four Principles of OOP
The four pillars of object-oriented programming are:
- Inheritance: child classes inherit data and behaviors from the parent class
- Encapsulation: containing information in an object, exposing only selected information
- Abstraction: only exposing high-level public methods for accessing an object
- Polymorphism: many methods can do the same task
Inheritance
Inheritance allows classes to inherit features of other classes. Put another way, parent classes extend attributes and behaviors to child classes. Inheritance supports reusability .
If basic attributes and behaviors are defined in a parent class, child classes can be created, extending the functionality of the parent class and adding additional attributes and behaviors.
For example, herding dogs have the unique ability to herd animals. In other words, all herding dogs are dogs, but not all dogs are herding dogs. We represent this difference by creating a child class HerdingDog from the parent class Dog , and then adding the unique herd() behavior.
The benefits of inheritance are programs can create a generic parent class and then create more specific child classes as needed. This simplifies programming because instead of recreating the structure of the Dog class multiple times, child classes automatically gain access to functionalities within their parent class.
In the following code snippet, child class HerdingDog inherits the method bark from the parent class Dog , and the child class adds an additional method, herd() .
Notice that the HerdingDog class does not have a copy of the bark() method. It inherits the bark() method defined in the parent Dog class.
When the code calls fluffy.bark() method, the bark() method walks up the chain of child to parent classes to find where the bark method is defined.
Note: Parent classes are also known as superclasses or base classes. The child class can also be called a subclass, derived class, or extended class.
In JavaScript, inheritance is also known as prototyping . A prototype object is a template for another object to inherit properties and behaviors. There can be multiple prototype object templates, creating a prototype chain.
This is the same concept as the parent/child inheritance. Inheritance is from parent to child. In our example, all three dogs can bark, but only Maisel and Fluffy can herd.
The herd() method is defined in the child HerdingDog class, so the two objects, Maisel and Fluffy , instantiated from the HerdingDog class have access to the herd() method.
Rufus is an object instantiated from the parent class Dog , so Rufus only has access to the bark() method.
Encapsulation
Encapsulation means containing all important information inside an object , and only exposing selected information to the outside world. Attributes and behaviors are defined by code inside the class template.
Then, when an object is instantiated from the class, the data and methods are encapsulated in that object. Encapsulation hides the internal software code implementation inside a class and hides the internal data of inside objects.
Encapsulation requires defining some fields as private and some as public.
- Private/ Internal interface: methods and properties accessible from other methods of the same class.
- Public / External Interface: methods and properties accessible from outside the class.
Let’s use a car as a metaphor for encapsulation. The information the car shares with the outside world, using blinkers to indicate turns, are public interfaces. In contrast, the engine is hidden under the hood.
It’s a private, internal interface. When you’re driving a car down the road, other drivers require information to make decisions, like whether you’re turning left or right. However, exposing internal, private data like the engine temperature would confuse other drivers.
Learn in-demand tech skills in half the time
Mock Interview
Skill Paths
Assessments
Learn to Code
Tech Interview Prep
Generative AI
Data Science
Machine Learning
GitHub Students Scholarship
Early Access Courses
For Individuals
Try for Free
Gift a Subscription
Become an Author
Become an Affiliate
Earn Referral Credits
Cheatsheets
Frequently Asked Questions
Privacy Policy
Cookie Policy
Terms of Service
Business Terms of Service
Data Processing Agreement
Copyright © 2024 Educative, Inc. All rights reserved.
Agile workflow
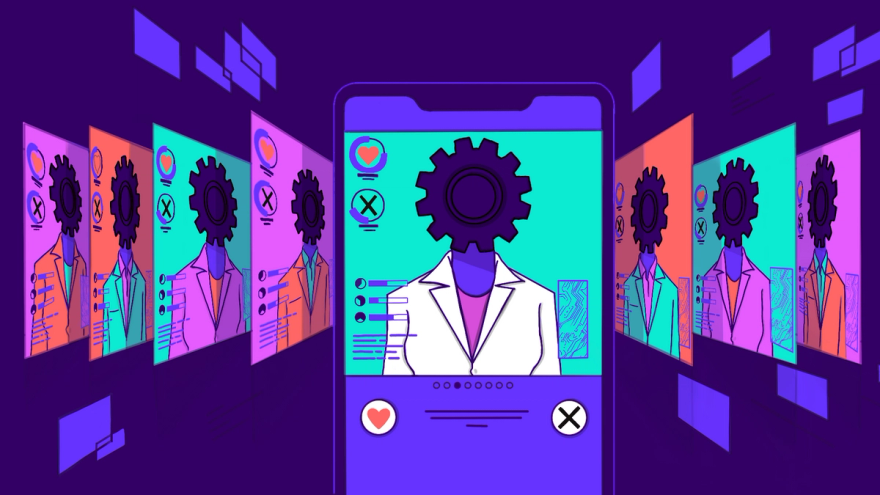
8 Software Development Methodologies Explained

Software development teams are known for using a wide variety of agile methodologies, approaches, and tools to bring value to customers. Depending on the needs of the team and the product's stakeholders, it’s common for teams to deploy and utilize a combination of software development methodologies.
Most dev teams combine methodologies and frameworks to build their own unique approach to product development. You’ll find there are plenty of overlapping principles from one methodology to the next. The key is choosing a system and working as a team to fine-tune and improve that approach so you can continue to reduce waste, maximize efficiency, and master collaboration.
In this post, we’ll outline and compare the following eight software development processes:
1. Agile software development methodology
2. Waterfall methodology
3. Feature driven development (FDD)
4. lean software development methodology, 5. scrum software development methodology, 6. extreme programming (xp), 7. rapid application development (rad), 8. devops deployment methodology.
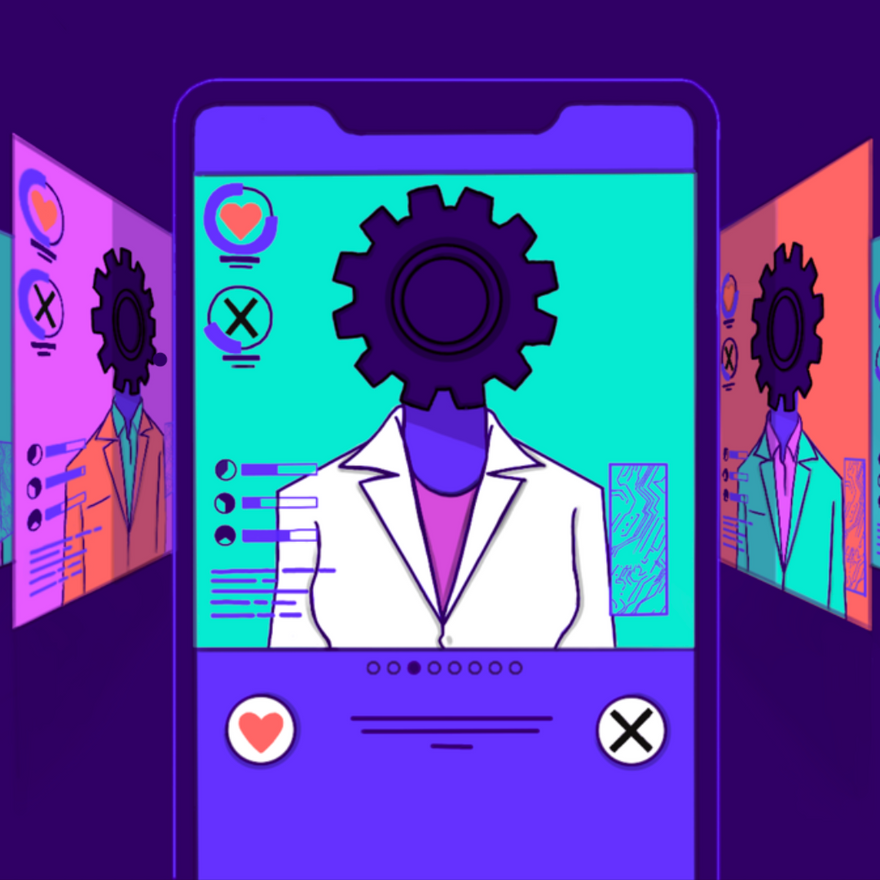
Agile is the most common term used to describe development methods. It’s often used as an umbrella term to label any methodology that’s agile in nature, meaning an iterative process that reduces waste and maximizes efficiency.
Most software development methodologies are agile with a strong emphasis on iteration, collaboration, and efficiency, as opposed to traditional project management. It’s like comparing jazz to classical music. 🎷
Traditional, linear management methods, such as the waterfall method we’ll cover below, are like classical music, led by one conductor who has a set plan for how the music should be played. The agile process, on the other hand, is more like jazz, which comes together through collaboration, experimentation, and iteration between band members. It’s adaptive and evolves with new ideas, situations, and directions.
2. The waterfall methodology
The waterfall approach is a traditional methodology that’s not very common in software development anymore. For many years, the waterfall model was the leading methodology, but its rigid approach couldn’t meet the dynamic needs of software development.
It’s more common to see the waterfall method used for project management rather than product development. At the beginning of a project, project managers gather all of the necessary information and use it to make an informed plan of action up front. Usually, this plan is a linear, step-by-step process with one task feeding into the next, giving it the “waterfall” name.
The approach is plan-driven and rigid, leaving little room for adjustments. It’s more or less the opposite of agile, prioritizing sticking to the plan rather than adapting to new circumstances.
Feature driven development is also considered an older methodology. Although it uses some agile principles, it’s viewed as the predecessor of today’s agile and lean methodologies.
As the name says, this process focuses on frequently implementing client-valued features. It’s an iterative process with all eyes on delivering tangible results to end users. The process is adaptive, improving based on new data and results that are collected regularly to help software developers identify and react to errors.
This kind of focused agile methodology can work for some teams that want a highly structured approach and clear deliverables while still leaving some freedom for iteration.
Lean software development comes from the principles of lean manufacturing. At its core, lean development strives to improve efficiency by eliminating waste. By reducing tasks and activities that don’t add real value, team members can work at optimal efficiency.
The five lean principles provide a workflow that teams use to identify waste and refine processes. Lean is also a guiding mindset that can help people work more efficiently, productively, and effectively.
The philosophies and principles of lean can be applied to agile and other software development methodologies. Lean development provides a clear application for scaling agile practices across large or growing organizations.
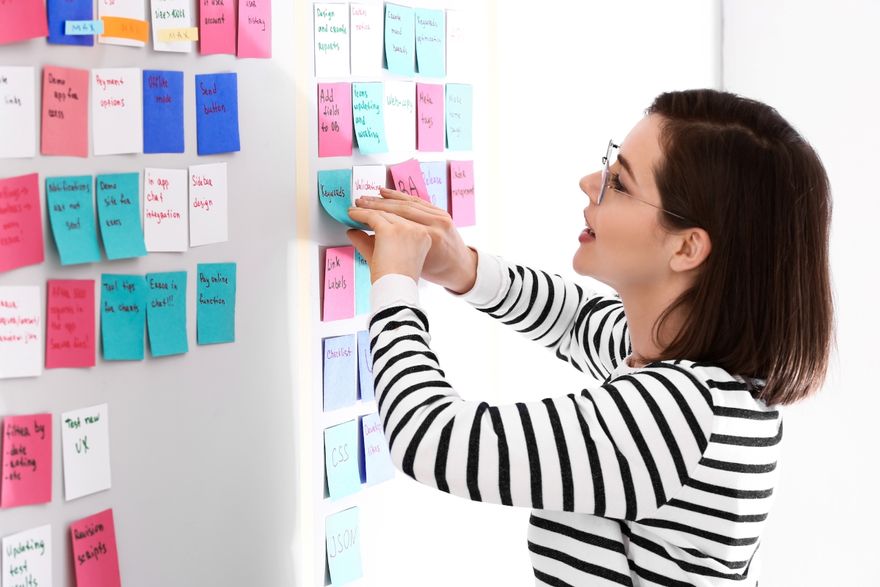
Scrum is a system regularly used by software development teams. Like many software development methodologies, Scrum is agile, focusing on a value-driven approach. The Scrum process is based on empiricism, which is the theory that knowledge comes from hands-on experience and observable facts.
One Scrum takes place over a preset amount of time called a sprint. Usually, the time frame is between two to four weeks and the Scrum is at the beginning of the sprint. The goal of each sprint is to yield an imperfect but progressing version of a product to bring to stakeholders so that feedback can be integrated right away into the next sprint.
The specific goals of each sprint are determined by a product owner who orders and prioritizes backlog items (the artifacts that need completion). The sprint process repeats over and over again with the development team adjusting and iterating based on successes, failures, and stakeholder feedback.
Learn more about Scrum — the complete program planning solution for Jira.
Extreme programming , also called XP, is a methodology based on improving software quality and responsiveness. It’s an agile approach that evolves based on customer requirements; the ultimate goal is producing high-quality results. Quality isn’t just limited to the final product — it applies to every aspect of the work, ensuring a great work experience for developers, programmers, and managers.
Decision-making in extreme programming is based on five values: communication, simplicity, feedback, courage, and respect. The specifics of XP can’t apply to all situations, but the general framework can provide value no matter the context.
Rapid application development (RAD), sometimes called rapid application building (RAB), is an agile methodology that aims to produce quality results at a low-cost investment. The process prioritizes rapid prototyping and frequent iteration.
Rapid application development begins with defining the project requirements. From there, teams design and build imperfect prototypes to bring to stakeholders as soon as possible. Prototyping and building repeat over and over through iterations until a product is complete and meets customer requirements.
This is ideal for smaller projects with a well-defined objective. The process helps developers make quick adjustments based on frequent feedback from stakeholders. It’s all about creating quick prototypes that can get in front of users for constructive feedback as soon as possible. This feedback is pulled into the user design so that development decisions are based on the direct thoughts and concerns of those who will use the product.
The DevOps deployment methodology is a combination of Dev (software development) and Ops (information technology operations). Together, they create a set of practices designed to improve communication and collaboration between the departments responsible for developing a product.
It's an ongoing loop of communication between product developers and Ops teams (IT operations.) Like so many agile processes, it relies on continuous feedback to help teams save time, increase customer satisfaction, improve launch speed, and reduce risks.
The steps of DevOps deployment repeat, aiming to increase customer satisfaction with new features, functionality, and improvements. However, this methodology has some drawbacks. Some customers don’t want continuous updates to their systems once they are satisfied with an end product.
Software development made easy
Most software development teams use a combination of methodologies and frameworks to fit their team size, team dynamics, and the type of work being completed. The key is to use an agile methodology and work together to continually improve your systems as you learn and grow.
Easy Agile is dedicated to helping teams work better together with agile. We design agile apps for Jira with simple, collaborative, and flexible functionality. From team agility with Easy Agile TeamRhythm , to scaled agility with Easy Agile Programs , our apps can help your agile teams deliver better for your customers.
Book a 1:1 demo to learn more about our suite of Jira tools, or contact our team if you have additional questions. We offer a free, 30-day trial, so you can try out our products before making a commitment.
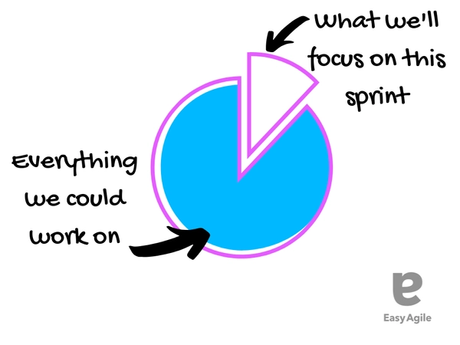
The guide to Agile Ceremonies for Scrum
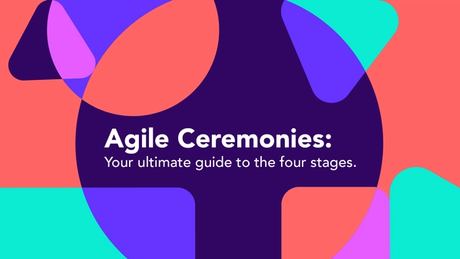
Agile Ceremonies: Your Ultimate Guide To the Four Stages
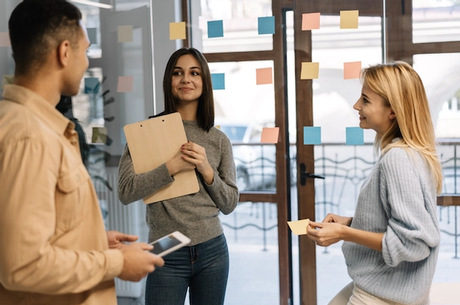
Your Guide To Agile Software Development Life Cycles
Subscribe to our blog.
Keep up with the latest tips and updates.
- Software Development
- Data Science and Business Analytics
- Press Announcements
- Scaler Academy Experience
What is Software Development?
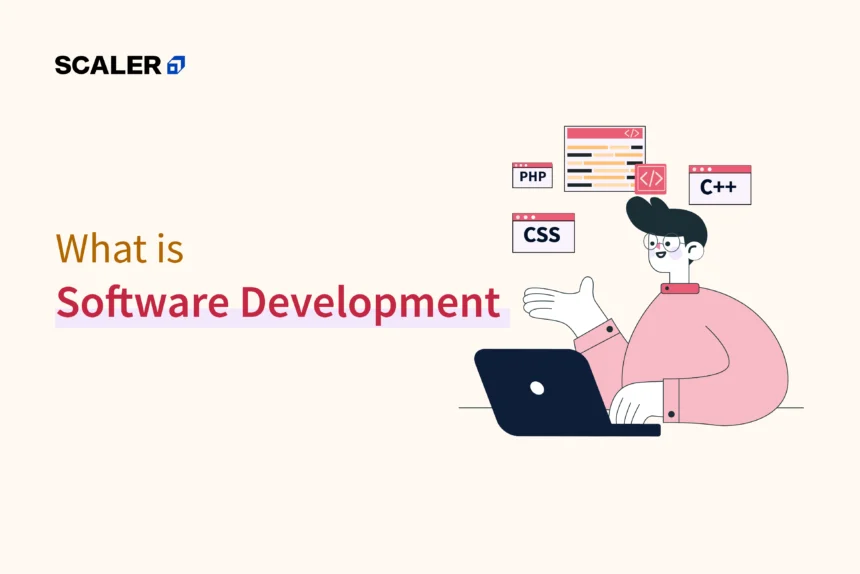
#ezw_tco-2 .ez-toc-title{ font-size: 120%; font-weight: 500; color: #000; } #ezw_tco-2 .ez-toc-widget-container ul.ez-toc-list li.active{ background-color: #ededed; } Contents
Software development is the lifeblood of the digital age. It’s how we design, build, and maintain the software that powers everything from our smartphones to the complex systems running global businesses. The impact of software development is undeniable:
Explosive Growth: The global software market is poised to eclipse $600 billion by 2023 according to the Gartner report on the global software market. This highlights the vast reach and importance of software in our lives.
High Demand, High Reward: Software developers are in high demand, with the U.S. Bureau of Labor Statistics projecting a growth rate of 22% by 2030 – much faster than the average for all occupations.
In this guide, we’ll delve into the world of software development, exploring its significance, the essential skills involved, and how you can launch your career in this dynamic field.
Software development is the process of designing, writing (coding), testing, debugging, and maintaining the source code of computer programs. Picture it like this:
Software developers are the architects, builders, quality control, and renovation crew all in one! They turn ideas into the functional software that shapes our digital experiences.
This covers a lot of ground, from the websites and tools you use online, to the apps on your mobile devices, regular computer programs, big systems for running companies, and even the fun games you play.
Steps in the Software Development Process
Building software isn’t a haphazard process. It generally follows a structured lifecycle known as the Software Development Life Cycle (SDLC). Here’s an overview of its key phases:
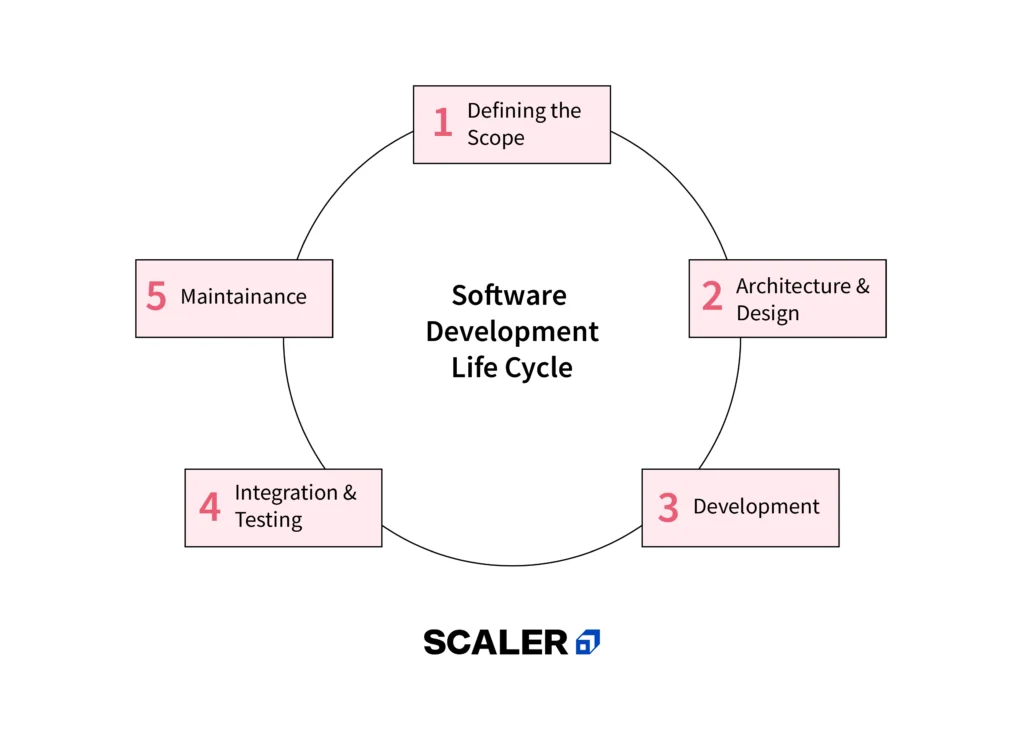
1. Needs Identification
At this early stage, it’s all about figuring out the “why” of the software. What’s the issue you’re trying to solve for users? Will it simplify a business process? Will it create an exciting new entertainment experience? Or will it fill an existing market gap? For instance, an online retailer might recognize that their website’s recommendation engine needs to be improved in order to increase sales.
2. Requirement Analysis
Once the purpose is clear, the team delves into the detailed blueprint. They meticulously gather all the specific requirements the software must fulfill. This includes defining features, how users will interact with the software, security considerations, and necessary performance levels. Continuing our example, the e-commerce company might determine requirements for personalized product suggestions, integration with their inventory system, and the ability to handle a high volume of users.
In this phase, software architects and designers create a structural plan. They’ll choose the right technologies (programming languages, frameworks), decide how the software is broken into modules, and define how different components will interact. For the recommendation engine, designers might opt for a cloud-based solution for scalability and use a machine learning library to power the suggestion algorithm.
4. Development and Implementation
This is where the building happens! Developers use their programming expertise to bring the design to life, writing the code that embodies the application’s logic and functionality. Depending on the project’s complexity, multiple programming languages and tools might be involved. In our recommendation engine example, the team could utilize Python for the machine learning algorithm and a web framework like Flask to integrate it into their website.
A rigorous testing phase is crucial to ensure the software is bug-free and functions as intended. Testers employ techniques like unit testing (checking individual code components), integration testing (how everything works together), and user acceptance testing (getting real-world feedback). In our scenario, testers will verify that the recommendation system suggests relevant products and gracefully handles different scenarios, such as when users have limited browsing history.
6. Deployment and Maintenance
It’s launch time! The software is deployed to its final environment, whether that’s release on an App Store, a live web application, or installation on company systems. However, the journey doesn’t end here. Continuous maintenance and updates are vital for security patches, addressing bugs, and adding new features down the line.
The SDLC can have different models (like Waterfall, and Agile). Real-world development often involves iterations and revisiting these stages.
Types of Software
The world of software is divided into broad categories, each serving a distinct purpose. Let’s explore these main types:
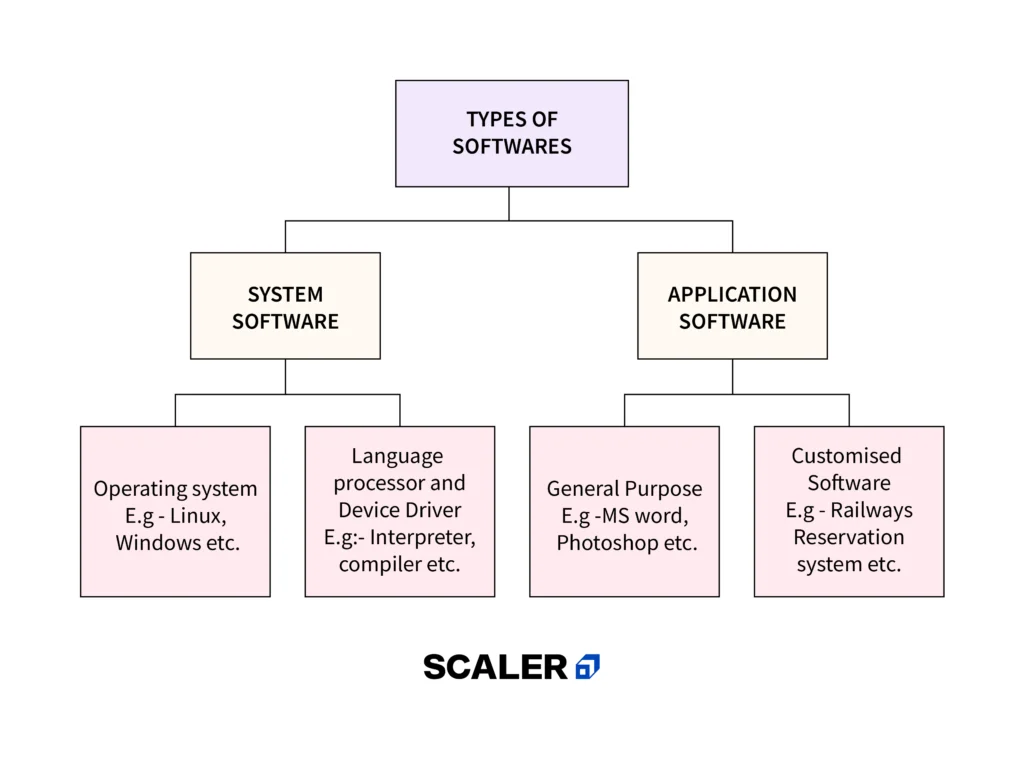
1. System Software
Think of system software as the foundation upon which your computer operates. It includes the operating system (like Windows, macOS, or Linux), which manages your computer’s hardware, provides a user interface, and enables you to run applications. Additionally, system software encompasses device drivers, the programs that allow your computer to communicate with devices like printers and webcams, and essential utilities like antivirus software and disk management tools.
2. Application Software
Application software is what you interact with directly to get things done, whether for work or play. This encompasses the programs you’re familiar with, such as web browsers (Chrome, Firefox), productivity suites (Microsoft Office, Google Workspace), video and photo editing software (Adobe Premiere Pro, Photoshop), games, and music streaming services like Spotify.
3. Programming Languages
Software coders use programming languages to create instructions. Programming languages also create application software and system software. Python, C++, Java, and C# are among the best known programming languages. Python is flexible and easy to learn for beginners, while Java is used in Android apps and enterprise programs. JavaScript makes websites interactive, while C++ is used for high-performance games and systems-level software development.
Important Note
While these categories are helpful, the lines between them can sometimes blur. For example, a web browser, though considered application software, heavily relies on components of your system software to function properly.
Why is Software Development Important?
Software development isn’t just about coding; it’s the engine driving progress in our modern world. Here’s why it matters:
The Fuel of Innovation: Breakthrough technologies like artificial intelligence, self-driving cars, and virtual reality would be impossible without sophisticated software. Software development pushes the boundaries of what’s possible, enabling entirely new industries and ways of interacting with the world.
Streamlining and Automating: From online banking to automated customer service chatbots, software makes processes faster, more convenient, and less prone to human error. This increased efficiency revolutionizes businesses and saves countless hours across various domains.
Adapting to Change: In our rapidly evolving world, software’s ability to be updated and modified is crucial. Whether it’s adapting to new regulations, customer needs, or technology trends, software empowers organizations to stay agile and remain competitive.
Global Reach: The internet and software transcend borders. Software-powered platforms connect people worldwide, facilitating remote work, global collaboration, and the rise of e-commerce, opening up markets once limited by geography.
Software Development’s Impact Across Industries
Let’s consider a few examples of how software changes the game in various sectors:
- Healthcare: Software powers medical imaging tools, analyzes vast datasets to discover new treatments and even assists with robot-guided surgery, ultimately improving patient care.
- Finance: Secure banking platforms, algorithmic trading, and fraud-detection software enable the flow of money while reducing risk.
- Education: Online learning platforms, interactive simulations, and adaptive learning tools make education more accessible and personalized for students of all ages.
- Transportation: Ride-hailing apps, traffic management systems, and developments in self-driving technology all rely on complex software systems.
Key Features of Software Development
Building high-quality software that meets user needs and endures over time requires a focus on these essential features:
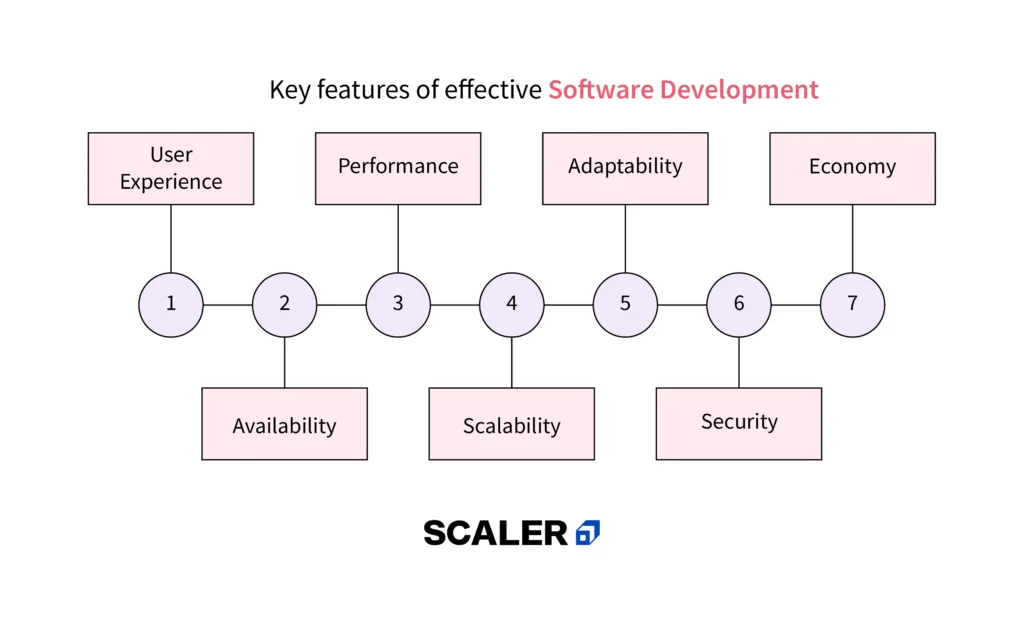
Scalability: The ability of software to handle an increasing workload. Imagine a social media app: good software needs to work effortlessly whether it’s serving a few thousand users or millions. Scalability involves planning for growth and ensuring the system can expand gracefully.
Reliability: Users expect software to work consistently and as intended. Reliability encompasses thorough testing to minimize bugs and crashes, as well as implementing error-handling procedures to provide the best possible experience even when unexpected glitches occur.
Security: With cyberattacks on the rise, protecting user data and preventing unauthorized access is paramount. Secure software development includes careful encryption practices, safeguarding against common vulnerabilities, and constantly staying updated to address potential threats.
Flexibility: User needs change, technologies evolve, and new features are often desired. Flexible software is designed to be adaptable and maintainable. This makes it easier to introduce updates, integrate with other systems, and pivot in response to market changes.
Software Development Methodologies
Think of software development methodologies as different roadmaps to guide a software project from start to finish. Let’s explore some popular approaches:
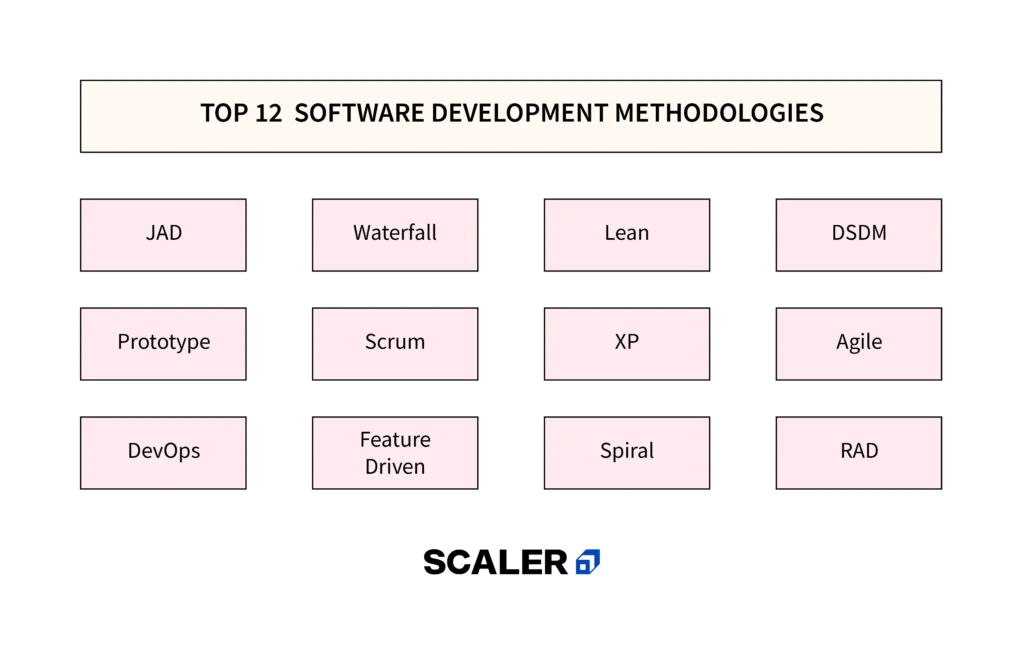
Waterfall: The Traditional Approach
The Waterfall model is linear and sequential. It involves phases like requirements analysis, design, coding, testing, and then deployment. Each phase must be fully complete before moving on to the next. This approach is best suited for projects with very clear, unchanging requirements and a long development timeline.
Agile: Embracing Iteration
Agile methods like Scrum and Kanban focus on short development cycles (sprints), continuous feedback, and adaptability to change. They emphasize collaboration between developers, stakeholders, and end-users. Agile methodologies are well-suited for projects where requirements might shift or evolve, and where delivering working software quickly is a priority.
DevOps: Bridging Development and Operations
DevOps focuses on collaboration between development teams and IT operations. It emphasizes automation, frequent updates, and monitoring to enable continuous delivery and rapid issue resolution. The aim is to ensure faster releases with increased stability. This benefits businesses by allowing them to innovate quickly, delivering updates and features at a much faster pace while maintaining reliability.
Choosing the Right Methodology
The best methodology depends on factors like the project’s size and complexity, the clarity of requirements upfront, company culture, and the desired speed of delivery.
Modern Trends: Hybrid Approaches and Adaptability
Many organizations adopt variations or hybrid approaches, combining elements of different methodologies to suit their specific needs. The ability to adapt the process itself is becoming a key hallmark of successful software development.
Software Development Tools and Solutions
Building software involves a whole toolkit beyond just coding skills. Let’s take a look at some commonly used categories of tools and technologies:
Integrated Development Environments (IDEs)
Think of IDEs as the developer’s powerhouse workspace. They combine a code editor, debugger, and compiler/interpreter, providing a streamlined experience. Popular IDEs include Visual Studio Code, IntelliJ IDEA, PyCharm, and Eclipse. IDEs boost productivity with features like code completion, syntax highlighting, and error checking, simplifying the overall development process.
Version Control Systems (e.g., Git)
Version control systems act like time travel for your code. Git, the most widely used system, allows developers to track every change made, revert to older versions if needed, collaborate effectively, and maintain different branches of code for experimentation. This provides a safety net, facilitates easy collaboration, and streamlines code management.
Project Management Tools (e.g., Jira, Trello)
These tools help organize tasks, set deadlines, and visualize the project’s progress. They often integrate with methodologies like Agile (Jira is particularly popular for this) and provide features like Kanban boards or Gantt charts to keep projects organized and efficient, especially within teams.
Collaboration Platforms (e.g., Slack, Microsoft Teams)
These platforms serve as a communication hub for development teams. They enable real-time messaging, file sharing, and video conferencing for seamless collaboration, reducing email overload and promoting quick problem-solving and knowledge sharing among team members.
Other Important Tools
- Cloud Computing Platforms (AWS, Azure, Google Cloud): Provide on-demand access to computing resources, databases, and a vast array of development tools.
- Testing Frameworks: Tools that help design and execute automated tests to ensure software quality.
- Web Frameworks (Django, Flask, Ruby on Rails): Offer structure and reusable components for building web applications.
Jobs That Use Software Development
- Quality Assurance (QA) Engineer: These professionals meticulously test software to identify bugs and ensure quality before release. They employ both manual and automated testing methods, working closely with developers to enhance the final product.
- Computer Programmer: Computer Programmers focus on the core act of writing code. They use specific programming languages to translate software designs into functional programs.
- Database Administrator (DBA): DBAs are responsible for the design, implementation, security, and performance optimization of database systems. They manage the storage, organization, and retrieval of an organization’s crucial data.
- Senior Systems Analyst: Systems Analysts act as a bridge between business needs and technical solutions. They analyze an organization’s requirements, propose software solutions, and often oversee the development process to ensure the system meets its intended goals.
- Software Engineer: This is a broad term encompassing roles involved in designing, building, testing, and maintaining software applications. Software Engineers might specialize in areas like web development, mobile development, game development, or embedded systems.
Software Development Resources
Online courses and platforms.
- Beginner-friendly: Look for websites that have interactive lessons and guided projects to start with.
- Comprehensive Programs: Look for websites that cover a vast array of languages, frameworks, and specializations.
- Scaler’s Full-Stack Developer Course: A structured program with industry-relevant curriculum, experienced mentors, and strong community support.
Tutorials and Documentation
- Official Sources: Programming language websites (e.g., Python.org) and framework documentation provide the most reliable information.
- Community Hubs: Websites like Stack Overflow are phenomenal for troubleshooting and finding answers to common questions.
Coding Communities
- Online Forums: Engage with fellow learners, seek help, and discuss projects on platforms like Reddit (programming-related subreddits) or Discord servers dedicated to software development.
- Meetups: Network with developers in your area through local meetups and events.
How Scaler Can Help?
Scaler’s comprehensive Full-Stack Developer Course can be an accelerator on your software development journey. Consider the advantages:
- Structured Learning: A well-designed curriculum takes you systematically from core concepts to advanced applications, offering a clear path forward.
- Mentorship: Guidance from industry experts provides valuable insights and helps you overcome roadblocks.
- Projects and Practical Experience: Hands-on building solidifies learning and creates a portfolio to showcase your skills.
- Community and Career Support: Interaction with peers and access to career services can be beneficial as you navigate the journey into software development.
Learning software development is an ongoing process. Even experienced developers continue to explore new technologies and techniques.
- From ubiquitous applications to world-changing inventions that fuel global digitalization efforts in every sector, it is the software development that does all the creating.
- Designing, developing, testing and deploying are among the critical stages of the SDLC (Software Development Life Cycle).
- High-quality software should be scalable, safe and reliable. It must also perform well with good user experience while being easy to understand for other developers.
- With roles ranging from quality assurance engineers to software engineering engineers there are many opportunities available in different industries when it comes to software development.
- Continuous learning and community support are necessary for both beginners and experienced developers because of ever-evolving technologies which help them stay current within this domain of expertise or advance their careers further.
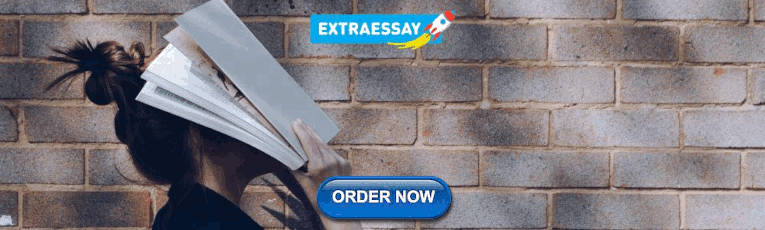
What is meant by a software developer?
A software developer is like an architect and construction crew combined. They design and build the software that powers everything from websites to complex enterprise systems.
What is the full form of SDLC?
SDLC stands for Software Development Life Cycle. It refers to the structured process of creating software, from defining requirements to deployment and maintenance.
Is software development the same as coding?
Coding (writing code) is a major part of software development, but it also involves problem-solving, design, testing, and collaboration.
What does a software developer do?
Software developers design, write code, test, debug, and maintain software applications. Their specific tasks depend on the project and their area of specialization.
What are some software development projects?
Software development projects can range from simple websites or mobile apps to complex enterprise systems, games, and even software for self-driving cars. The possibilities are endless!
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Software Engineering Tutorial
- Software Development Life Cycle
- Waterfall Model
- Software Requirements
- Software Measurement and Metrics
- Software Design Process
- System configuration management
- Software Maintenance
- Software Development Tutorial
- Software Testing Tutorial
- Product Management Tutorial
- Project Management Tutorial
- Agile Methodology
- Selenium Basics
- Introduction to Software Engineering - Software Engineering
- Classification of Software - Software Engineering
- Waterfall Model - Software Engineering
- Iterative Waterfall Model - Software Engineering
- Spiral Model - Software Engineering
- Incremental Process Model - Software Engineering
- Rapid application development model (RAD) - Software Engineering
- RAD Model vs Traditional SDLC - Software Engineering
- Agile Development Models - Software Engineering
- Agile Software Development - Software Engineering
What is Extreme Programming (XP)?
- SDLC V-Model - Software Engineering
- Comparison of different life cycle models in Software Engineering
- User Interface Design - Software Engineering
- Coupling and Cohesion - Software Engineering
- Information System Life Cycle - Software Engineering
- Database Application System Life Cycle - Software Engineering
- Pham-Nordmann-Zhang Model (PNZ model) - Software Engineering
- Software Engineering | Schick-Wolverton software reliability model
- Phases of Project Management Process
- Project size Estimation Techniques - Software Engineering
- System configuration management - Software Engineering
- COCOMO Model - Software Engineering
- Capability Maturity Model (CMM) - Software Engineering
- Integrating Risk Management in SDLC | Set 1
Extreme programming (XP) is one of the most important software development frameworks of Agile models. It is used to improve software quality and responsiveness to customer requirements.
Table of Content
Good Practices in Extreme Programming
Basic principles of extreme programming, applications of extreme programming (xp), extreme programming practices, advantages of extreme programming (xp).
The extreme programming model recommends taking the best practices that have worked well in the past in program development projects to extreme levels.
Some of the good practices that have been recognized in the extreme programming model and suggested to maximize their use are given below:
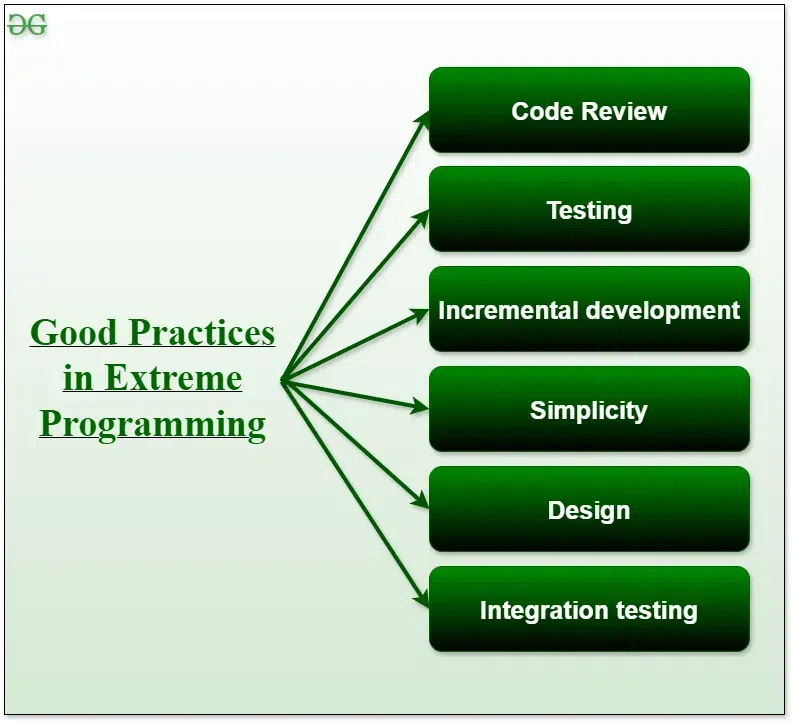
Extreme Programming Good Practices
- Code Review: Code review detects and corrects errors efficiently. It suggests pair programming as coding and reviewing of written code carried out by a pair of programmers who switch their work between them every hour.
- Testing: Testing code helps to remove errors and improves its reliability. XP suggests test-driven development (TDD) to continually write and execute test cases. In the TDD approach, test cases are written even before any code is written.
- Incremental development: Incremental development is very good because customer feedback is gained and based on this development team comes up with new increments every few days after each iteration.
- Simplicity: Simplicity makes it easier to develop good-quality code as well as to test and debug it.
- Design: Good quality design is important to develop good quality software. So, everybody should design daily.
- Integration testing: It helps to identify bugs at the interfaces of different functionalities. Extreme programming suggests that the developers should achieve continuous integration by building and performing integration testing several times a day.
XP is based on the frequent iteration through which the developers implement User Stories. User stories are simple and informal statements of the customer about the functionalities needed. A User Story is a conventional description by the user of a feature of the required system. It does not mention finer details such as the different scenarios that can occur. Based on User stories, the project team proposes Metaphors. Metaphors are a common vision of how the system would work. The development team may decide to build a Spike for some features. A Spike is a very simple program that is constructed to explore the suitability of a solution being proposed. It can be considered similar to a prototype. Some of the basic activities that are followed during software development by using the XP model are given below:
- Coding: The concept of coding which is used in the XP model is slightly different from traditional coding. Here, the coding activity includes drawing diagrams (modeling) that will be transformed into code, scripting a web-based system, and choosing among several alternative solutions.
- Testing: The XP model gives high importance to testing and considers it to be the primary factor in developing fault-free software.
- Listening: The developers need to carefully listen to the customers if they have to develop good quality software. Sometimes programmers may not have the depth knowledge of the system to be developed. So, the programmers should understand properly the functionality of the system and they have to listen to the customers.
- Designing: Without a proper design, a system implementation becomes too complex, and very difficult to understand the solution, thus making maintenance expensive. A good design results elimination of complex dependencies within a system. So, effective use of suitable design is emphasized.
- Feedback: One of the most important aspects of the XP model is to gain feedback to understand the exact customer needs. Frequent contact with the customer makes the development effective.
- Simplicity: The main principle of the XP model is to develop a simple system that will work efficiently in the present time, rather than trying to build something that would take time and may never be used. It focuses on some specific features that are immediately needed, rather than engaging time and effort on speculations of future requirements.
- Pair Programming: XP encourages pair programming where two developers work together at the same workstation. This approach helps in knowledge sharing, reduces errors, and improves code quality.
- Continuous Integration: In XP, developers integrate their code into a shared repository several times a day. This helps to detect and resolve integration issues early on in the development process.
- Refactoring: XP encourages refactoring, which is the process of restructuring existing code to make it more efficient and maintainable. Refactoring helps to keep the codebase clean, organized, and easy to understand.
- Collective Code Ownership: In XP, there is no individual ownership of code. Instead, the entire team is responsible for the codebase. This approach ensures that all team members have a sense of ownership and responsibility towards the code.
- Planning Game: XP follows a planning game, where the customer and the development team collaborate to prioritize and plan development tasks. This approach helps to ensure that the team is working on the most important features and delivers value to the customer.
- On-site Customer: XP requires an on-site customer who works closely with the development team throughout the project. This approach helps to ensure that the customer’s needs are understood and met, and also facilitates communication and feedback.
Some of the projects that are suitable to develop using the XP model are given below:
- Small projects: The XP model is very useful in small projects consisting of small teams as face-to-face meeting is easier to achieve.
- Projects involving new technology or Research projects: This type of project faces changing requirements rapidly and technical problems. So XP model is used to complete this type of project.
- Web development projects: The XP model is well-suited for web development projects as the development process is iterative and requires frequent testing to ensure the system meets the requirements.
- Collaborative projects: The XP model is useful for collaborative projects that require close collaboration between the development team and the customer.
- Projects with tight deadlines: The XP model can be used in projects that have a tight deadline, as it emphasizes simplicity and iterative development.
- Projects with rapidly changing requirements: The XP model is designed to handle rapidly changing requirements, making it suitable for projects where requirements may change frequently.
- Projects where quality is a high priority: The XP model places a strong emphasis on testing and quality assurance, making it a suitable approach for projects where quality is a high priority.
Extreme Programming (XP) is an Agile software development methodology that focuses on delivering high-quality software through frequent and continuous feedback, collaboration, and adaptation. XP emphasizes a close working relationship between the development team, the customer, and stakeholders, with an emphasis on rapid, iterative development and deployment.
Agile development approaches evolved in the 1990s as a reaction to documentation and bureaucracy-based processes, particularly the waterfall approach. Agile approaches are based on some common principles, some of which are:
- Working software is the key measure of progress in a project.
- For progress in a project, therefore software should be developed and delivered rapidly in small increments.
- Even late changes in the requirements should be entertained.
- Face-to-face communication is preferred over documentation.
- Continuous feedback and involvement of customers are necessary for developing good-quality software.
- A simple design that involves and improves with time is a better approach than doing an elaborate design up front for handling all possible scenarios.
- The delivery dates are decided by empowered teams of talented individuals.
Extreme programming is one of the most popular and well-known approaches in the family of agile methods. an XP project starts with user stories which are short descriptions of what scenarios the customers and users would like the system to support. Each story is written on a separate card, so they can be flexibly grouped.
XP, and other agile methods, are suitable for situations where the volume and space of requirements change are high and where requirement risks are considerable.
- Continuous Integration: Code is integrated and tested frequently, with all changes reviewed by the development team.
- Test-Driven Development: Tests are written before code is written, and the code is developed to pass those tests.
- Pair Programming: Developers work together in pairs to write code and review each other’s work.
- Continuous Feedback: Feedback is obtained from customers and stakeholders through frequent demonstrations of working software.
- Simplicity: XP prioritizes simplicity in design and implementation, to reduce complexity and improve maintainability.
- Collective Ownership: All team members are responsible for the code, and anyone can make changes to any part of the codebase.
- Coding Standards: Coding standards are established and followed to ensure consistency and maintainability of the code.
- Sustainable Pace: The pace of work is maintained at a sustainable level, with regular breaks and opportunities for rest and rejuvenation.
- XP is well-suited to projects with rapidly changing requirements, as it emphasizes flexibility and adaptability. It is also well-suited to projects with tight timelines, as it emphasizes rapid development and deployment.
- Refactoring: Code is regularly refactored to improve its design and maintainability, without changing its functionality.
- Small Releases: Software is released in small increments, allowing for frequent feedback and adjustments based on that feedback.
- Customer Involvement: Customers are actively involved in the development process, providing feedback and clarifying requirements.
- On-Site Customer: A representative from the customer’s organization is present with the development team to provide continuous feedback and answer questions.
- Short Iterations: Work is broken down into short iterations, usually one to two weeks in length, to allow for rapid development and frequent feedback.
- Planning Game: The team and customer work together to plan and prioritize the work for each iteration, to deliver the most valuable features first.
- Metaphor: A shared metaphor is used to guide the design and implementation of the system.
- Slipped schedules: Timely delivery is ensured through slipping timetables and doable development cycles.
- Misunderstanding the business and/or domain − Constant contact and explanations are ensured by including the client on the team.
- Canceled projects: Focusing on ongoing customer engagement guarantees open communication with the consumer and prompt problem-solving.
- Staff turnover: Teamwork that is focused on cooperation provides excitement and goodwill. Team spirit is fostered by multidisciplinary cohesion.
- Costs incurred in changes: Extensive and continuing testing ensures that the modifications do not impair the functioning of the system. A functioning system always guarantees that there is enough time to accommodate changes without impairing ongoing operations.
- Business changes: Changes are accepted at any moment since they are seen to be inevitable.
- Production and post-delivery defects: the unit tests to find and repair bugs as soon as possible.
Please Login to comment...
Similar reads.
- Software Engineering
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
.css-s5s6ko{margin-right:42px;color:#F5F4F3;}@media (max-width: 1120px){.css-s5s6ko{margin-right:12px;}} AI that works. Coming June 5, Asana redefines work management—again. .css-1ixh9fn{display:inline-block;}@media (max-width: 480px){.css-1ixh9fn{display:block;margin-top:12px;}} .css-1uaoevr-heading-6{font-size:14px;line-height:24px;font-weight:500;-webkit-text-decoration:underline;text-decoration:underline;color:#F5F4F3;}.css-1uaoevr-heading-6:hover{color:#F5F4F3;} .css-ora5nu-heading-6{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;-webkit-box-pack:start;-ms-flex-pack:start;-webkit-justify-content:flex-start;justify-content:flex-start;color:#0D0E10;-webkit-transition:all 0.3s;transition:all 0.3s;position:relative;font-size:16px;line-height:28px;padding:0;font-size:14px;line-height:24px;font-weight:500;-webkit-text-decoration:underline;text-decoration:underline;color:#F5F4F3;}.css-ora5nu-heading-6:hover{border-bottom:0;color:#CD4848;}.css-ora5nu-heading-6:hover path{fill:#CD4848;}.css-ora5nu-heading-6:hover div{border-color:#CD4848;}.css-ora5nu-heading-6:hover div:before{border-left-color:#CD4848;}.css-ora5nu-heading-6:active{border-bottom:0;background-color:#EBE8E8;color:#0D0E10;}.css-ora5nu-heading-6:active path{fill:#0D0E10;}.css-ora5nu-heading-6:active div{border-color:#0D0E10;}.css-ora5nu-heading-6:active div:before{border-left-color:#0D0E10;}.css-ora5nu-heading-6:hover{color:#F5F4F3;} Get early access .css-1k6cidy{width:11px;height:11px;margin-left:8px;}.css-1k6cidy path{fill:currentColor;}
- Product overview
- All features
- App integrations
CAPABILITIES
- project icon Project management
- Project views
- Custom fields
- Status updates
- goal icon Goals and reporting
- Reporting dashboards
- workflow icon Workflows and automation
- portfolio icon Resource management
- Time tracking
- my-task icon Admin and security
- Admin console
- asana-intelligence icon Asana Intelligence
- list icon Personal
- premium icon Starter
- briefcase icon Advanced
- Goal management
- Organizational planning
- Campaign management
- Creative production
- Marketing strategic planning
- Request tracking
- Resource planning
- Project intake
- View all uses arrow-right icon
- Project plans
- Team goals & objectives
- Team continuity
- Meeting agenda
- View all templates arrow-right icon
- Work management resources Discover best practices, watch webinars, get insights
- What's new Learn about the latest and greatest from Asana
- Customer stories See how the world's best organizations drive work innovation with Asana
- Help Center Get lots of tips, tricks, and advice to get the most from Asana
- Asana Academy Sign up for interactive courses and webinars to learn Asana
- Developers Learn more about building apps on the Asana platform
- Community programs Connect with and learn from Asana customers around the world
- Events Find out about upcoming events near you
- Partners Learn more about our partner programs
- Support Need help? Contact the Asana support team
- Asana for nonprofits Get more information on our nonprofit discount program, and apply.
Featured Reads
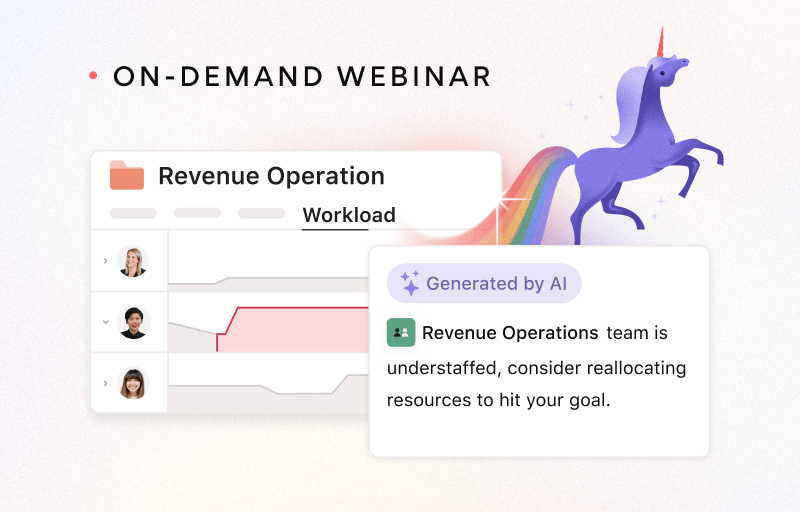
- What is Agile methodology? (A beginner’ ...
What is Agile methodology? (A beginner’s guide)
Agile methodology is a project management framework that breaks projects down into several dynamic phases, commonly known as sprints. In this article, get a high-level overview of Agile project management, plus a few common frameworks to choose the right one for your team.
Scrum, Kanban, waterfall, Agile.
Agile project management isn’t just useful for software project management—all types of teams have been successful with this dynamic methodology. If you’re looking to get started with Agile, you’ve come to the right place.
What is the Agile methodology?
Agile methodology is a project management framework that breaks projects down into several dynamic phases, commonly known as sprints.
The Agile framework is an iterative methodology . After every sprint, teams reflect and look back to see if there was anything that could be improved so they can adjust their strategy for the next sprint.
What is the Agile Manifesto?
The Agile Manifesto is a document that focuses on four values and 12 principles for Agile software development. It was published in February 2001 by 17 software developers who needed an alternative to the more linear product development process .
What are the 4 pillars of Agile?
As outlined in the Agile Manifesto, there are four main values of Agile project management:
Individuals over processes and tools: Agile teams value team collaboration and teamwork over working independently and doing things "by the book.”
Working software over comprehensive documentation: The software that Agile teams develop should work. Additional work, like documentation, is not as important as developing good software.
Customer collaboration over contract negotiation: Customers are extremely important within the Agile methodology. Agile teams allow customers to guide where the software should go. Therefore, customer collaboration is more important than the finer details of contract negotiation.
Responding to change over following a plan: One of the major benefits of Agile project management is that it allows teams to be flexible. This framework allows for teams to quickly shift strategies and workflows without derailing an entire project.
What are the 12 Agile principles?
The four values of Agile are the pillars of Agile methodology. From those values, the team developed 12 principles.
If the four values of Agile are the weight-bearing pillars of a house, then these 12 principles are the rooms you can build within that house. These principles can be easily adapted to fit the needs of your team.
The 12 principles used in Agile methodology are:
Satisfy customers through early, continuous improvement and delivery. When customers receive new updates regularly, they're more likely to see the changes they want within the product. This leads to happier, more satisfied customers—and more recurring revenue.
Welcome changing requirements, even late in the project. The Agile framework is all about adaptability. In iterative processes like Agile, being inflexible causes more harm than good.
Deliver value frequently. Similar to principle #1, delivering value to your customers or stakeholders frequently makes it less likely for them to churn.
Break the silos of your projects. Collaboration is key in the Agile framework. The goal is for people to break out of their own individual projects and collaborate together more frequently .
Build projects around motivated individuals. Agile works best when teams are committed and actively working to achieve a goal.
The most effective way to communicate is face-to-face. If you’re working on a distributed team, spend time communicating in ways that involve face-to-face communication like Zoom calls.
Working software is the primary measure of progress. The most important thing that teams should strive for with the Agile framework is the product. The goal here is to prioritize functional software over everything else.
Maintain a sustainable working pace. Some aspects of Agile can be fast-paced, but it shouldn't be so fast that team members burn out . The goal is to maintain sustainability throughout the project.
Continuous excellence enhances agility . If the team develops excellent code in one sprint, they can continue to build off of it the next. Continually creating great work allows teams to move faster in the future.
Simplicity is essential. Sometimes the simplest solution is the best solution. Agile aims to not overcomplicate things and find simple answers to complex problems.
Self-organizing teams generate the most value. Similar to principle #5, proactive teams become valuable assets to the company as they strive to deliver value.
Regularly reflect and adjust your way of work to boost effectiveness . Retrospective meetings are a common Agile practice. It's a dedicated time for teams to look back and reflect on their performance and adapt their behaviors for the future.
What are the benefits of the Agile development methodology?
You commonly find Agile project management used in application development or other types of software development. This is because software is constantly changing, and the needs of the product have to change with it.
Because of this, linear project management methods like the waterfall model are less effective. Here are a few other reasons why teams use Agile:
Agile methods are adaptable
There's a reason why they call it the Agile methodology. One of the main benefits of using Agile processes in software development is the ability to shift strategies quickly, without disrupting the flow of a project.
Because phases in the traditional waterfall method flow into one another, shifting strategies is challenging and can disrupt the rest of the project roadmap . Since software development is a much more adaptable field, project managing rapid changes in the traditional sense can be challenging. This is part of the reason why Agile project management is favored in software development.
Agile fosters collaborative teamwork
One of the Agile principles states that the most effective way to communicate with your team is face-to-face. Combine this with the principle that encourages teams to break project silos and you have a recipe for collaborative teamwork.
While technology has changed since Agile’s inception and work has shifted to welcome more remote-friendly policies, the idea of working face-to-face still hasn't changed.
Agile methods focus on customer needs
One of the unique aspects of software development is that teams can focus on customer needs much more closely than other industries. With the rise of cloud-based software, teams can get feedback from their actual customers quickly.
Since customer satisfaction is a key driver for software development, it’s easy to see why it was included in the Agile process. By collaborating with customers, Agile teams can prioritize features that focus on customer needs. When those needs change, teams can take an Agile approach and shift to a different project.
Agile methodologies
The Agile framework is an umbrella for several different variations. Here are a few of the most common Agile methodologies.
Kanban is a visual approach to Agile. Teams use online Kanban board tools to represent where certain tasks are in the development process. Tasks are represented by cards on a board, and stages are represented in columns. As team members work on tasks, they move cards from the backlog column to the column that represents the stage the task is in.
This method is a good way for teams to identify roadblocks and to visualize the amount of work that’s getting done.
Scrum is a common Agile methodology for small teams and also involves sprints. The team is led by a Scrum master whose main job is to clear all obstacles for others executing the day-to-day work.
Scrum teams meet daily to discuss active tasks, roadblocks, and anything else that may affect the development team.
Sprint planning: This event kicks off the sprint. Sprint planning outlines what can be delivered in a sprint (and how).
Sprint retrospective : This recurring meeting acts as a sprint review—to iterate on learnings from a previous sprint that will improve and streamline the next one.
Extreme Programming (XP)
Typically used in software development, Extreme Programming (XP) is an Agile framework that outlines values that will allow your team to work together more effectively.
The five values of XP include:
Communication
Similar to daily Scrum standups, there are regular releases and iterations, yet XP is much more technical in its approach. If your dev team needs to quickly release and respond to customer requests, XP focuses on the “how” it will get done.
Adaptive Project Framework (APF)
The Adaptive Project Framework, also known as Adaptive Project Management (APM) grew from the idea that unknown factors can show up at any time during a project. This technique is mainly used for IT projects where more traditional project management techniques don’t apply.
This framework is based on the idea that project resources can change at any time. For example, budgets can change, timelines can shift, or team members working on the project may transition to different teams. APF focuses on the resources that a project has, as opposed to the resources a project needs.
Extreme Project Management (XPM)
This type of project management is often used for very complex projects with a high level of uncertainty. This approach involves constantly adapting processes until they lead to the desired result. This type of project involves many spontaneous changes and it’s normal for teams to switch strategies from one week to the next.
XPM requires a lot of flexibility. This is one of the reasons why each sprint is short—only a few weeks maximum. This methodology allows for frequent changes, trial-and-error approaches to problems, and many iterations of self-correction.
Adaptive Software Development (ASD)
This Agile methodology enables teams to quickly adapt to changing requirements. The main focus of this process is continuous adaptation. The phases of this project type —speculate, collaborate, and learn—allow for continuous learning as the project progresses.
It’s not uncommon for teams running ASD to be in all three phases of ASD at once. Because of its non-linear structure, it’s common for the phases to overlap. Because of the fluidity of this type of management, there’s a higher likelihood that the constant repetition of the three phases helps team members identify and solve problems much quicker than standard project management methods.
Dynamic Systems Development Method (DSDM)
The Dynamic Systems Development Method is an Agile method that focuses on a full project lifecycle. Because of this, DSDM has a more rigorous structure and foundation, unlike other Agile methods.
There are four main phases of DSDM:
Feasibility and business study
Functional mode or prototype iteration
Design and build iteration
Implementation
Feature Driven Development (FDD)
Feature Driven Development blends different Agile best practices. While still an iterative method of project management, this model focuses more on the exact features of a software that the team is working to develop. Feature-driven development relies heavily on customer input, as the features the team prioritizes are the features that the customers need.
This model also allows teams to update projects frequently. If there is an error, it's quick to cycle through and implement a fix as the phases of this framework are constantly moving.
Organize Agile processes with Asana
You’ll often hear software development teams refer to the Agile process—but any team can run Agile. If you’re looking for a more flexible project management framework, try Agile.
Related resources
Smooth product launches are simpler than you think
How Asana uses work management to streamline project intake processes
6 ways to develop adaptability in the workplace and embrace change
How to run more effective stand-up meetings
- Daily Crossword
- Word Puzzle
- Word Finder
- Word of the Day
- Synonym of the Day
- Word of the Year
- Language stories
- All featured
- Gender and sexuality
- All pop culture
- Writing hub
- Grammar essentials
- Commonly confused
- All writing tips
- Pop culture
- Writing tips
Advertisement
methodology
[ meth- uh - dol - uh -jee ]
- a set or system of methods, principles, and rules for regulating a given discipline, as in the arts or sciences.
- the underlying principles and rules of organization of a philosophical system or inquiry procedure.
- the study of the principles underlying the organization of the various sciences and the conduct of scientific inquiry.
- Education. a branch of pedagogics dealing with analysis and evaluation of subjects to be taught and of the methods of teaching them.
/ ˌmɛθəˈdɒlədʒɪ; ˌmɛθədəˈlɒdʒɪkəl /
- the system of methods and principles used in a particular discipline
- the branch of philosophy concerned with the science of method and procedure
Discover More
Derived forms.
- ˌmethodoˈlogically , adverb
- ˌmethodˈologist , noun
- methodological , adjective
Other Words From
- meth·od·o·log·i·cal [ meth-, uh, -dl-, oj, -i-k, uh, l ] , adjective
- meth·od·ol·o·gist noun
Word History and Origins
Origin of methodology 1
Example Sentences
In the global race for a Covid vaccine, different researchers are trying a variety of methodologies and platforms.
The issue, he added, is that there isn’t a clear methodology or adjudication system for publishers or platforms to dispute Chrome’s decision making over what constitutes a “heavy” ad.
Firefox has been an aggressive champion of consumer privacy and not necessarily a friend to digital marketers, most of whom would have preferred to keep third-party cookies and existing tracking and targeting methodologies intact.
Before you dive in, it may help to read our summary of the state of the race, or at least skim our very detailed methodology guide.
We work with Google to find the most advanced and highest impact advertising strategies, as well as new advertising features, and we reveal some of our new methodologies which we normally do not share.
Geisbert was also quick to mention how the methodology of the study could be affecting the current results.
Germane and relevant in their way, but wielding a different methodology.
The UN methodology affords its team a little more flexibility.
“Food Chains” shows how the CIW is using a completely new methodology—contract law—to make a difference in the growing fields.
Alt cert critics often argue that there are flaws in the methodology of some of these studies.
The next two Partes contain a discussion of the methodology of note-taking and are not directly bibliographical in nature.
I bring together here different studies relating more or less directly to questions of scientific methodology.
Some expense for the development of computer systems and computer systems methods is justifiable as an investment in methodology.
The arguments used by these despisers of methodology are strong enough in all appearance.
The study of these processes of historical construction forms the second half of Methodology.
Related Words
What is agile?

Quick on your feet: think for a minute of your favorite athlete. Unless this person is a bodybuilder, strength and sheer power are only part of the story. For most sportsmen and women, real success on the playing field comes with a certain hard-to-teach nimbleness—the ability to quickly take in new information and adjust strategy to achieve a specific result. Part of the appeal of sports is the excitement that comes with constant change , and—discounting the vicissitudes of luck—the result comes down to how athletes apply their abilities in response.
Get to know and directly engage with senior McKinsey experts on agile
Aaron De Smet and Sherina Ebrahim are senior partners in McKinsey’s New Jersey office; Christopher Handscomb is a partner in the London office, where Shail Thaker is a senior partner. Other experts include Krish Krishnakanthan , a senior partner in the Stamford office; and David Pralong , a senior partner in the Charlotte office.
Change is also a constant in business (and, yes, life). Agile, in business, is a way of working that seeks to go with the flow of inevitable change rather than work against it. The Agile Manifesto , developed in 2001 as a way of optimizing software development, prioritizes individuals over processes, working prototypes over thorough documentation, customer collaboration over closed-door meetings, and swift response to change over following a set plan. In the years since its inception, agile has conferred competitive advantage to the organizations that have applied it, in and out of the IT department.
As our business, social, economic, and political environments become increasingly volatile, the only way to meet the challenges of rapidly changing times is to change with them . Read on to learn more about agile and how to adopt an agile mindset.
Learn more about McKinsey’s People & Organizational Performance Practice.
What is an agile organization?
Let’s go back to sports for a minute. Maybe you’re a great free throw shooter in basketball. You make nine out of ten shots when you’re by yourself in your driveway, shooting around for fun. But when you meet friends for a pickup game at a nearby court, your shot is off. People keep jumping in front of you when you’re trying to line up, and maybe the sun is shining in your eyes from an unfavorable angle. You make maybe a couple of shots the whole game. The following week, disappointed by your performance on the court, you decide to make a change. Rather than doubling your practice time shooting in your driveway, you mix up your routine, practicing your shot at a couple of different courts at different times of day. Maybe you also ask a friend to run some defense drills with you so you can get used to shooting under pressure. Maybe you add a layup to your shot practice. This is a shift to an agile approach—and increases the likelihood that you’ll perform better the next time you play pickup.
Traditional organizations are—much like you shooting free throws in your driveway—optimized to operate in static, siloed situations of structural hierarchy. Planning is linear, and execution is controlled. The organization’s skeletal structure is strong but frequently rigid and slow moving.
Agile organizations are different. They’re designed for rapid change. An agile organization is a technology-enabled network of teams with a people-centered culture that operates in rapid-learning and fast-decision cycles. Agility adds speed and adaptability to stability, creating a competitive advantage in uncertain conditions.
What is kanban and what is scrum?
Kanban and scrum are two organizational frameworks that fall under the umbrella of agile.
Kanban originated in the manufacturing plants of postwar Japan. Kanban, which is Japanese for “signboard,” was first developed to prioritize “just in time” delivery—that is, meeting demand rather than creating a surplus of products before they’re needed. With kanban, project managers create lanes of work that are required to deliver a product. A basic kanban board would have vertical lanes for processes—these processes could be “to do,” “doing,” “done,” and “deployed.” A product or assignment would move horizontally through the board.
The idea of scrum was invented by two of the original developers of agile methodology. A team of five to nine people is led by a scrum leader and product owner. The team sets its own commitments and engages in ceremonies like daily stand-up meetings and sprint planning, uniting in a shared goal.
Scrums, kanban, and other agile product management frameworks are not set in stone. They’re designed to be adapted and adjusted to fit the requirements of the project. One critical component of agile is the kaizen philosophy— a pillar of the Toyota production model —which is one of continuous improvement. With agile methodologies, the point is to learn from each iteration and adjust the process based on what’s learned.
What are the hallmarks of an agile organization?
A team or organization of any size or industry can be agile. But regardless of the details, all agile groups have five things in common .
North Star embodied across the organization
Agile organizations set a shared purpose and vision for the organization that helps people feel personally invested—that’s a North Star. This helps align teams with sometimes wildly varied remits and processes.
Network of empowered teams
Agile organizations typically replace top-down structures with flexible, scalable networks of teams. Agile networks should operate with high standards of alignment, accountability, expertise, transparency, and collaboration. Regardless of the configuration of the network, team members should feel a sense of ownership over their work and see a clear connection between their work and the business’s North Star.
Rapid decision and learning cycles
Agile teams work in short cycles—or sprints—then learn from them by collecting feedback from users to apply to a future sprint. This quick-cycle model accelerates the pace throughout the organization, prioritizing quarterly cycles and dynamic management systems—such as objectives and key results (OKRs)—over annual planning.
Dynamic people model that ignites passion
An agile culture puts people at the center, seeking to create value for a much wider range of stakeholders, including employees, investors, partners, and communities. Making change personally meaningful for employees can build transformational momentum.
Next-generation enabling technology
Radical rethinking of an organizational model requires a fresh look at the technologies that enable processes. These include, for example, real-time communication and work management tools that support continually evolving operating processes.
Agility looks a little different for every organization. But the advantages in stability and dynamism that the above trademarks confer are the same—and are critical to succeeding in today’s rapidly changing competitive environment.

Introducing McKinsey Explainers : Direct answers to complex questions
How should an organization implement an agile transformation.
According to a McKinsey survey on agile transformations , the best way to go about an agile transformation is for an entire organization to transition to agile, rather than just individual departments or teams. This is ambitious but possible: New Zealand–based digital-services and telecommunications company Spark NZ managed to flip the entire organization to an agile operating model in less than a year.
Any enterprise-wide agile transformation needs to be both comprehensive and iterative: comprehensive in the sense that it addresses strategy, structure, people, process, and technology and iterative in its acceptance that things will change along the way.
Transformations vary according to the size, industry, and scope of the business, but there are a common set of elements that can be divided into two components .
- The first phase of an agile transformation involves designing and piloting the new agile operating model. This usually starts with building the top team’s understanding and aspirations, creating a blueprint for how agile will add value, and implementing pilots.
- The second phase is about improving the process and creating more agile cells throughout the organization. Here, a significant amount of time is required from key leaders, as well as willingness to role model new mindsets. The best way to accomplish this phase is to recognize that not everything can be planned for, and implementation requires continuous measurement and adjustment.
Culture is a critical part of any agile transformation. Agile is a mindset; it’s not something an organization does— it’s something an organization is . Getting this transition right is key to overall success.
Agile is a mindset; it’s not something an organization does —it’s something an organization is .
Who should lead an agile transformation?
The single most important trait for the leader of an agile organization is an agile mindset, or inner agility . Simply put, inner agility is a comfortable relationship with change and uncertainty. And research has shown that a leader’s mindset, and how that mindset shapes organizational culture, can make or break a successful agile transformation.
Leaders need three sets of capabilities for agile transformations:
Leaders must evolve new mindsets and behaviors.
For most of us, the natural impulse is to react. Research shows that people spend most of their time in a reactive mindset—reacting to challenges, circumstances, or other people. Because of this natural tendency, traditional organizations were designed to run on the reactive.
Agile organizations, by contrast, run on creative mindsets built on curiosity. A culture of innovation, collaboration, and value creation helps nurture the ability to flexibly respond to unexpected change. Creative mindsets also help members of an organization, at all levels, tap into their core passions and purposes .
Roche, a legacy biotech company, recognized the importance of a mindset shift at the leadership level. When the organization decided to build an agile culture , it invited more than 1,000 leaders to a four-day immersion program designed to enable leaders to shift from a reactive mindset to a creative one. Today, agility has been widely deployed within Roche, engaging tens of thousands of people in applying agile mindsets.
Leaders must help teams work in new and more effective ways.
Agility spells change for both leaders and their teams. Leaders need to give more autonomy and flexibility to their teams and trust them to do the right thing. For their part, teams should embrace a design-thinking mentality and build toward working more efficiently, assuming more responsibility for the outcomes of their projects and being more accountable to customers.
Leaders must cocreate an agile organizational purpose, design, and culture.
A critical organization-level skill for leaders is the ability to distill and communicate a clear, shared, and compelling purpose, or North Star. Next, leaders need to design the strategy and operating model of the organization based on agile principles and practices. Finally, leaders need to shape a new culture across the organization, based on creative mindsets of discovery, partnership, and abundance.
For tips on how to develop agile leaders, see “ Leading agile transformation: The new capabilities leaders need to build 21st-century organizations .”
How can we build inner agility?
Inner agility can feel counterintuitive. Our impulse as humans is to simplify and solve problems by applying our expertise. But complex problems require complex solutions, and sometimes those solutions are beyond our expertise. Recognizing that our solutions aren’t working can feel like failure—but it doesn’t have to. To train themselves to address problems in a more agile way, leaders need to learn to think beyond their normal ways of solving problems.
Here are five ways to build inner agility :
Pause to move faster
This can be tough for leaders used to moving quickly. But pausing in the middle of the fray can create space for clearer judgment, original thinking, and purposeful action. This can take many forms: one CEO McKinsey has worked with takes a ten-minute walk outside the office without his cell phone. Others do breathing exercises between meetings. These practices can help leaders interrupt habits to create space for something different.
Embrace your ignorance
Being a know-it-all no longer works. The world is changing so fast that new ideas can come from anywhere. Competitors you’ve never heard of can suddenly reshape your industry. As change accelerates, listening and thinking from a place of not knowing is crucial to encouraging the discovery of original, surprising, breakthrough ideas.
Radically reframe the questions
We too frequently interrogate our ideas, asking ourselves questions we already know the answers to—and worse, questions whose answers confirm what we already believe. Instead, seek to ask truly challenging, open-ended questions. Those types of questions allow your employees and stakeholders to creatively discuss and describe what they’re seeing, and potentially unblock existing mental frameworks.
Set direction, not destination
In the increasing complexity of our era, solutions are rarely straightforward. Instead of setting a path from one point to another, share a purposeful vision with your team. Then join your team in heading toward a general goal, and in exploring and experimenting together to reach common goals.
Test your solutions—and yourself
Ideas may not work out as planned. But quick, cheap failures allow you to see what works and what doesn’t—and avoid major, costly disasters.
In times of stress, we often feel ourselves challenged. Rather than falling back on old habits, inner agility enables us to embrace complexity and use it to grow stronger.
What is deliberate calm?
Deliberate calm is a mindset that helps leaders keep a cool head during a crisis and steer their ships through a storm. It’s not something that comes naturally: in times of uncertainty, the human brain is wired to react rather than stay calm. The ability to step back and choose actions suited to a given situation is a skill that must be cultivated. In their 2022 book Deliberate Calm , McKinsey veterans Jacqueline Brassey , Aaron De Smet , and Michiel Kruyt describe their personal self-mastery practices to offer lessons in effective leadership through crises.
One important lesson? Not all crises are created equal. Inspired by the thinking of Harvard Business School professor Herman “Dutch” Leonard, De Smet differentiates between routine emergencies and crises of uncertainty. Routine emergencies can be dealt with using past experiences and training. But crises of uncertainty are different. In these moments, where you don’t know how deep the rabbit hole goes, you can’t fall back on what you know. “If you are in an uncertain situation,” says De Smet, “the most important thing you can do is calm down. Take a breath. Take stock. ‘Is the thing I’m about to do the right thing to do?’ And in many cases, the answer is no. If you were in a truly uncertain environment, if you’re in new territory, the thing you would normally do might not be the right thing.”
For more from De Smet and his coauthors, check out their discussion on The McKinsey Podcast , their Author Talks interview , or order Deliberate Calm .
What is an agile transformation office?
Establishing an agile transformation office (ATO) can help improve the odds that an agile transformation will be successful. Embedded within an existing organizational structure, an ATO shapes and manages the transformation, brings the organization along, and—crucially—helps it achieve lasting cultural change.
To set up an ATO for success, an organization has to make three design decisions :
Agree on the ATO’s purpose and mandate
An ATO needs a purpose just as an agile organization needs a North Star. This step links an ATO specifically to the “why” of the transformation. An ATO’s mandate can include driving the transformation strategy, building capabilities, championing change, coaching senior leaders, managing interdependencies, and creating and refining best practices.
Define the ATO’s place within the organization
While an ATO’s reporting lines will depend on the organization, usually the leader of successful ATOs reports to the CEO or one of the CEO’s direct reports. This ensures tight alignment and support from top leadership.
Determine the ATO’s roles and responsibilities
Regardless of an ATO’s size or mandate, the following core capabilities should be managed by a strong transformation leader:
Execution leaders own the transformation road map, assessing and adjusting it on an ongoing basis.
Methodology owners gather lessons from the transformation and refine and evolve agile practices and behaviors.
Agile coaches guide teams through their transformations, helping to instill an agile culture and mindset.
Change management and communications experts maintain lines of communication through periods of change.
As agile principles become the norm across industries, an ATO can help usher in an agile transformation regardless of the design choices an organization makes in setting it up.
Can remote teams practice agile?
Agile teams typically rely on the camaraderie, community, and trust made possible by co-location. The remote work necessitated by the COVID-19 crisis has put this idea—along with many other assumptions about work and office culture—to the test.
While the shift was sudden, talented agile teams working through the crisis proved that productivity can be maintained with the right technology in place. Here are some targeted actions agile leaders can take to recalibrate their processes and sustain an agile culture with remote teams.
Revisit team norms
Tools to ease teams into remote work abound—these include virtual whiteboards, instant chat, and videoconferencing. But they still represent a change from the in-person tools agile teams usually rely on for ceremonies. Team members need to help one another quickly get up to speed on how best to shift to virtual. Teams also need to make extra effort to capture the collective view, a special challenge when working remotely.
Cultivate bonding and morale
In the absence of in-person bonding activities, like lunches or spontaneous coffees, team members can bond virtually, such as by showing each other around their homes on a video call, introducing pets or family members, or sharing music or other personal interests. Being social is important in the virtual space, as well as in person, to nurture team cohesion.
Adapt coaching and development
Team leaders who normally do one-on-one coaching over coffee should transition as seamlessly as possible to remote coaching—coffee and all.
Establish a single source of truth
In person, agile team processes are usually informal. Teams make decisions with everyone in the room, so there’s not usually much need to record these discussions. In the virtual space, however, people might be absent or distracted, so it’s important to document team discussions in a way that can be referenced later.
Adjust to asynchronous collaboration
Messaging boards and chats can be useful in coordinating agile teams working remotely. But they should be used carefully, as they can also lead to team members feeling isolated.
Adapt a leadership approach
When working with remote teams, leaders need to be deliberate in guiding team members and interacting with external customers and stakeholders. Simply put, they need to show—in tone and approach—that everyone is in this together.
Now that it seems likely that remote work is here to stay , it’s all the more important that teams reinforce productivity by purposefully working to sustain an agile culture.
How can public-sector organizations benefit from agile?
The pandemic era and its attendant sociopolitical and economic crises have placed new pressures on public-sector organizations. In these situations of urgency, agile can help public-sector organizations better serve citizens by being more responsive.
Compared with the private sector, where agile has had a clear impact on the overall health of organizations , the public sector doesn’t immediately seem to be a great candidate for agile methodology. Government processes are often slower moving than their private-sector counterparts, and agencies are frequently in competition for funding, which can discourage collaboration. Finally, public-sector organizations are usually hierarchical; agile methodology works best in flat organizational structures.
But according to McKinsey analysis and experience, agile tools can still have an impact on public-sector productivity . As with any agile transformation, the approach should be tailored to each specific department, team, and organization.
Government entities, for example, might focus on short-term, results-driven management styles. OKRs and quarterly business reviews (QBRs) are agile concepts that can transform planning and resource allocation for governments. Agencies, for their part, can benefit from increased collaboration and cross-pollination made possible by agile operating models.
For a more in-depth exploration of these topics, see McKinsey’s People & Organizational Performance Practice. Also check out agile-related job opportunities if you’re interested in working at McKinsey.
Articles referenced include:
- “ When things get rocky, practice deliberate calm ,” November 10, 2022, Jacqueline Brassey and Aaron De Smet
- “ In pursuit of value—not work ,” October 24, 2022, Elena Chong, Christopher Handscomb , Tomasz Maj, Rishi Markenday, Leslie Morse, and Dave West
- “ Why an agile transformation office is your ticket to real and lasting impact ,” June 30, 2021, Amit Anand, Khushpreet Kaur , Noor Narula, and Belkis Vasquez-McCall
- “ The impact of agility: How to shape your organization to compete ,” May 25, 2021, Wouter Aghina , Christopher Handscomb , Olli Salo , and Shail Thaker
- “ Doing vs being: Practical lessons on building an agile culture ,” August 4, 2020, Nikola Jurisic , Michael Lurie , Philippine Risch , and Olli Salo
- “ All in: From recovery to agility at Spark New Zealand ,” McKinsey Quarterly , June 11, 2019, Simon Moutter, Jolie Hodson, Joe McCollum, David Pralong , Jason Inacio , and Tom Fleming
- “ The journey to an agile organization ,” May 10, 2019, Daniel Brosseau , Sherina Ebrahim , Christopher Handscomb , and Shail Thaker
- “ Leading agile transformation: The new capabilities leaders need to build 21st-century organizations ,” October 1, 2018, Aaron De Smet , Michael Lurie , and Andrew St. George
- “ Agile with a capital ‘A’: A guide to the principles and pitfalls of agile development ,” February 12, 2018, Hugo Sarrazin, Belkis Vasquez-McCall , and Simon London
- “ The five trademarks of agile organizations ,” January 22, 2018, Wouter Aghina , Karin Ahlback, Aaron De Smet , Gerald Lackey, Michael Lurie , Monica Murarka, and Christopher Handscomb
- “ A business leader’s guide to agile ,” July 25, 2017, Santiago Comella-Dorda , Krish Krishnakanthan , Jeff Maurone, and Gayatri Shenai
- “ ING’s agile transformation ,” McKinsey Quarterly , January 10, 2017, Peter Jacobs, Bart Schlatmann, and Deepak Mahadevan

Want to know more about agile?
Related articles.
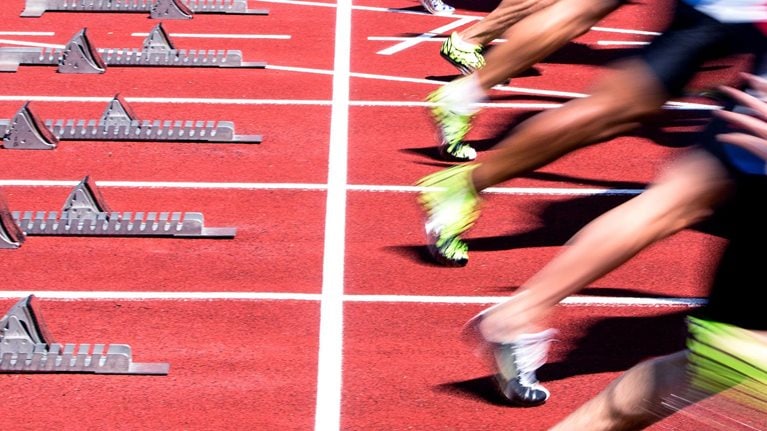
Beyond the anecdote: True drivers of digital-delivery performance

Why an agile transformation office is your ticket to real and lasting impact

The impact of agility: How to shape your organization to compete
Members-only Content
- Monthly Member Events
- Event Session Videos
- Experience Reports
- Research Papers
- Share a Community Event
- Submit an Article to the Blog
- Submit a Member Initiative
- Promote a Training Event
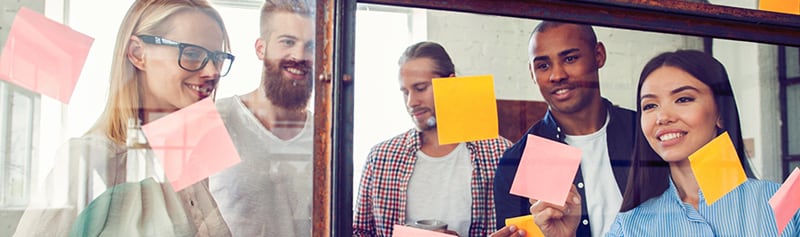
Become an Agile Alliance member!
Your membership enables us to offer a wealth of resources, present renowned international events, support global community groups, and so much more! And, while you’re supporting our non-profit mission, you’ll also gain access to a range of valuable member benefits. Learn more
- Join Us Today
- Member Portal
- Membership FAQs
- Terms and Conditions
- Corporate Members
Agile Conferences
- XP 2024 in Bolzano
- Agile Executive Forum
- Agile2024 European Experience
- All Agile Alliance Events
- Past Conferences
- Become an Event Sponsor
Virtual Events
- Member Events Calendar
- Agile MiniCon
- BYOC Lean Coffee
- Agile Tech Talks
- Member Meet & Greet
- Agile Coaching Network
- Full Events Calendar
- Community Events
- Community Events Calendar
- Agile Training Calendar
- Sponsored Meetup Groups
- Submit a Non-profit Event
- Submit a For-profit Training
- Event Funding Request
- Global Events Calendars
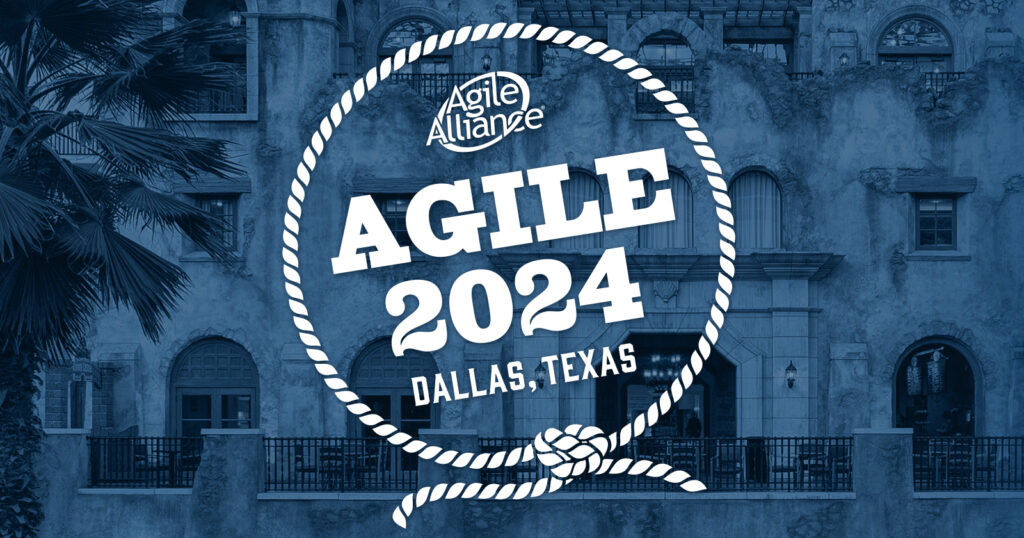
- Events Calendar
- BYOC – Lean Coffee
- Member Meet & Greet
- Agile Training
- View All Events
- Submit an Event
- Meetup Groups
- Past Conferences & Events
Agile Essentials is designed to bring you up to speed on the basic concepts and principles of Agile with articles, videos, glossary terms, and more.
Agile Essentials
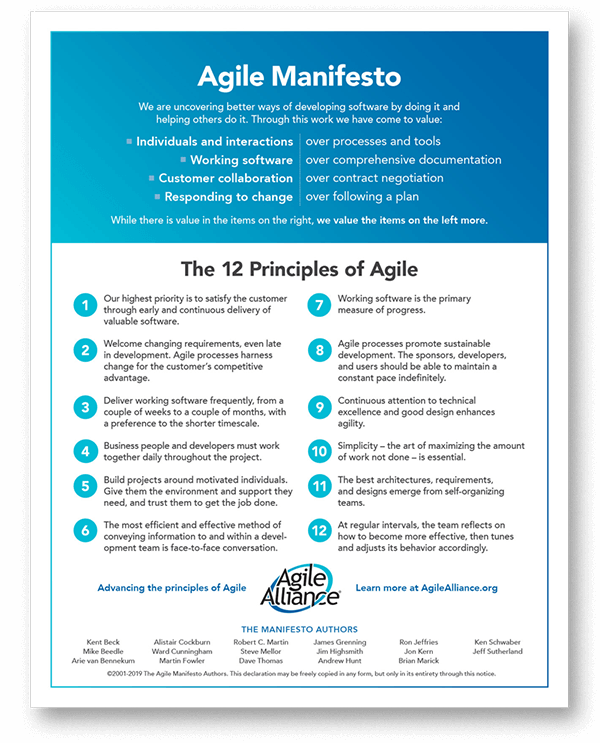
Download the Agile Manifesto
To download a free PDF copy of the Agile Manifesto and 12 Principles of Agile, simply sign-up for our newsletter. Agile Alliance members can download it for free.
- Agile Essentials Overview
- Agile Manifesto
- 12 Principles Behind the Manifesto
- A Short History of Agile
- Subway Map to Agile Practices
- Agile Glossary
- Introductory Videos
Recent Blog Posts
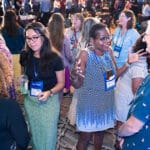
Finding your community at Agile Alliance
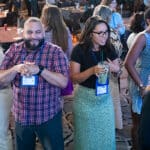
Ten reasons for joining us at Agile2024
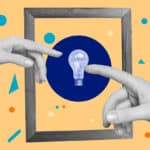
How the Agile Alliance board makes decisions
View all blog posts
Agile Resources
The new agile resource guide.
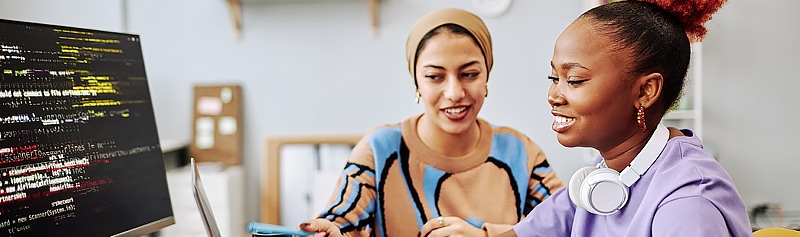
Find Agile services and products from our member companies in our new Agile Resource Guide . Many listings in the guide feature exclusive offers just for Agile Alliance members. View the guide
- Remote Working Guide
- Event Sessions
- Content Library
Sustainability Manifesto
The Agile Sustainability Initiative has created the Agile Sustainability Manifesto in an effort to grow awareness about sustainability within the Agile community and inspire a more sustainable way of working. Read and sign now
MEMBER INITIATIVES
- Agile Sustainability Initiative
- Principle 12 Initiative
- Agile in Color Initiative
- Agile Coach Camp Worldwide
- Agile Coaching Ethics
View all initiatives
Your Community
Global development.
- LATAM Community
- India Community
Global Affiliates
- Community Groups
- Community Services
- Member Initiatives
- LATAM Community Development
- India Community Development
- Volunteer Signup
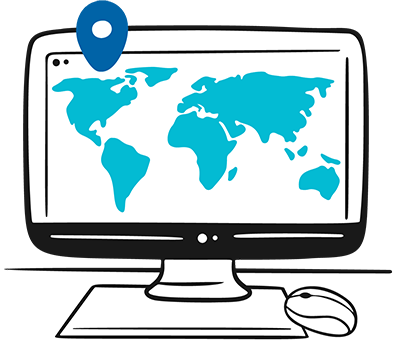
OUR POLICIES
Become a sponsor.
Being an Agile Alliance sponsor is a great way to introduce your company to our members to build awareness around your products and services. The Call for Agile2024 Sponsorships is now open, and there are great options and opportunities still available! Learn more >
- About Agile Alliance
- Code of Conduct
- Board of Directors
- Agile Alliance Brazil
- Agile Alliance New Zealand
- Policies, Reports & Bylaws
- Logo and Media Files
- Become a Sponsor
Extreme Programming
> just getting started with agile learn the essentials at agile2024, what is extreme programming.
Extreme Programming (XP) is an agile software development framework that aims to produce higher quality software and higher quality of life for the development team. XP is the most specific of the agile frameworks regarding appropriate engineering practices for software development.
When Applicable
The general characteristics where XP is appropriate were described by Don Wells on www.extremeprogramming.org :
- Dynamically changing software requirements
- Risks caused by fixed-time projects using new technology
- Small, co-located extended development team
- The technology you are using allows for automated unit and functional tests
Due to XP’s specificity when it comes to its full set of software engineering practices, there are several situations where you may not want to fully practice XP. The post When is XP Not Appropriate on the C2 Wiki is probably a good place to start to find examples where you may not want to use XP.
While you can’t use the entire XP framework in many situations, that shouldn’t stop you from using as many of the practices as possible given your context.
The five values of XP are communication, simplicity, feedback, courage, and respect which are described in more detail below.
Communication
Software development is inherently a team sport that relies on communication to transfer knowledge from one team member to everyone else on the team. XP stresses the importance of the appropriate kind of communication – face-to-face discussion with the aid of a whiteboard or other drawing mechanism.
Simplicity means “what is the simplest thing that will work?” The purpose of this is to avoid waste and do only absolutely necessary things such as keep the design of the system as simple as possible so that it is easier to maintain, support, and revise. Simplicity also means addressing only the requirements that you know about; don’t try to predict the future.
Through constant feedback about their previous efforts, teams can identify areas for improvement and revise their practices. Feedback also supports simple design. Your team builds something, gathers feedback on your design and implementation, and then adjusts your product going forward.
Kent Beck defined courage as “effective action in the face of fear” (Extreme Programming Explained P. 20). This definition shows a preference for action based on other principles so that the results aren’t harmful to the team. You need courage to raise organizational issues that reduce your team’s effectiveness. You need courage to stop doing something that doesn’t work and try something else. You need courage to accept and act on feedback, even when it’s difficult to accept.
The members of your team need to respect each other in order to communicate with each other, provide and accept feedback that honors your relationship, and work together to identify simple designs and solutions.
The core of XP is the interconnected set of software development practices listed below. While it is possible to do these practices in isolation, many teams have found some practices reinforce the others and should be done in conjunction to fully eliminate the risks you often face in software development.
The XP Practices have changed a bit since they were initially introduced. The original twelve practices are listed below. If you would like more information about how these practices were originally described, you can visit http://ronjeffries.com/xprog/what-is-extreme-programming/ .
- The Planning Game
- Small Releases
- Simple Design
- Refactoring
Pair Programming
- Collective Ownership
Continuous Integration
- 40-hour week
- On-site Customer
- Coding Standard
Below are the descriptions of practices as described in the second edition of Extreme Programming Explained Embrace Change. These descriptions include refinements based on the experiences of many who practice extreme programming and reflect a more practical set of practices.
Sit Together
Since communication is one of the five values of XP, and most people agree that face-to-face conversation is the best form of communication, have your team sit together in the same space without barriers to communication, such as cubicle walls.
A cross-functional group of people with the necessary roles for a product form a single team. This means people with a need as well as all the people who play some part in satisfying that need all work together on a daily basis to accomplish a specific outcome.
Informative Workspace
Set up your team space to facilitate face-to-face communication, allow people to have some privacy when they need it, and make the work of the team transparent to each other and to interested parties outside the team. Utilize Information Radiators to actively communicate up-to-date information.
Energized Work
You are most effective at software development and all knowledge work when you are focused and free from distractions.
Energized work means taking steps to make sure you are able physically and mentally to get into a focused state. This means do not overwork yourself (or let others overwork you). It also means staying healthy, and showing respect to your teammates to keep them healthy.
Pair Programming means all production software is developed by two people sitting at the same machine. The idea behind this practice is that two brains and four eyes are better than one brain and two eyes. You effectively get a continuous code review and quicker response to nagging problems that may stop one person dead in their tracks.
Teams that have used pair programming have found that it improves quality and does not actually take twice as long because they are able to work through problems quickly and they stay more focused on the task at hand, thereby creating less code to accomplish the same thing.
Describe what the product should do in terms meaningful to customers and users. These stories are intended to be short descriptions of things users want to be able to do with the product that can be used for planning and serve as reminders for more detailed conversations when the team gets around to realizing that particular story.
Weekly Cycle
The Weekly Cycle is synonymous with an iteration . In the case of XP, the team meets on the first day of the week to reflect on progress to date, the customer picks the stories they would like delivered in that week, and the team determines how they will approach those stories. The goal by the end of the week is to have running tested features that realize the selected stories.
The intent behind the time-boxed delivery period is to produce something to show to the customer for feedback.
Quarterly Cycle
The Quarterly Cycle is synonymous with a release. The purpose is to keep the detailed work of each weekly cycle in the context of the overall project. The customer lays out the overall plan for the team in terms of features desired within a particular quarter, which provides the team with a view of the forest while they are in the trees, and it also helps the customer to work with other stakeholders who may need some idea of when features will be available.
Remember when planning a quarterly cycle the information about any particular story is at a relatively high level, the order of story delivery within a Quarterly Cycle can change and the stories included in the Quarterly Cycle may change. If you are able to revisit the plan on a weekly basis following each weekly cycle, you can keep everyone informed as soon as those changes become apparent to keep surprises to a minimum.
The idea behind slack in XP terms is to add some low-priority tasks or stories in your weekly and quarterly cycles that can be dropped if the team gets behind on more important tasks or stories. Put another way, account for the inherent variability in estimates to make sure you leave yourself a good chance of meeting your forecasts.
Ten-Minute Build
The goal of the Ten-Minute Build is to automatically build the whole system and run all of the tests in ten minutes. The founders of XP suggested a 10-minute time frame because if a team has a build that takes longer than that, it is less likely to be run on a frequent basis, thus introducing a longer time between errors.
This practice encourages your team to automate your build process so that you are more likely to do it on a regular basis and to use that automated build process to run all of your tests.
This practice supports the practice of Continuous Integration and is supported by the practice of Test First Development .
Continuous Integration is a practice where code changes are immediately tested when they are added to a larger code base. The benefit of this practice is you can catch and fix integration issues sooner.
Most teams dread the code integration step because of the inherent discovery of conflicts and issues that result. Most teams take the approach of “If it hurts, avoid it as long as possible”.
Practitioners of XP suggest “if it hurts, do it more often”.
The reasoning behind that approach is that if you experience problems every time you integrate code, and it takes a while to find where the problems are, perhaps you should integrate more often so that if there are problems, they are much easier to find because there are fewer changes incorporated into the build.
This practice requires some extra discipline and is highly dependent on Ten Minute Build and Test First Development.
Test-First Programming
Instead of following the normal path of:
develop code -> write tests -> run tests
The practice of Test-First Programming follows the path of:
Write failing automated test -> Run failing test -> develop code to make test pass -> run test -> repeat
As with Continuous Integration, Test-First Programming reduces the feedback cycle for developers to identify and resolve issues, thereby decreasing the number of bugs that get introduced into production.
Incremental Design
The practice of Incremental Design suggests that you do a little bit of work upfront to understand the proper breadth-wise perspective of the system design, and then dive into the details of a particular aspect of that design when you deliver specific features. This approach reduces the cost of changes and allows you to make design decisions when necessary based on the most current information available.
The practice of Refactoring was originally listed among the 12 core but was incorporated into the practice of Incremental Design. Refactoring is an excellent practice to use to keep the design simple, and one of the most recommended uses of refactoring is to remove duplication of processes.
Although Extreme Programming specifies particular practices for your team to follow, it does not really establish specific roles for the people on your team.
Depending on which source you read, there is either no guidance, or there is a description of how roles typically found in more traditional projects behave on Extreme Programming projects. Here are the four most common roles associated with Extreme Programming:
The Customer
The Customer role is responsible for making all of the business decisions regarding the project including:
- What should the system do (What features are included and what do they accomplish)?
- How do we know when the system is done (what are our acceptance criteria)?
- How much do we have to spend (what is the available funding, what is the business case)?
- What should we do next (in what order do we deliver these features)?
The XP Customer is expected to be actively engaged in the project and ideally becomes part of the team.
The XP Customer is assumed to be a single person, however, experience has shown that one person cannot adequately provide all of the business-related information about a project. Your team needs to make sure that you get a complete picture of the business perspective, but have some means of dealing with conflicts in that information so that you can get clear direction.
The Developer
Because XP does not have much need for role definition, everyone on the team (with the exception of the customer and a couple of secondary roles listed below) is labeled a developer. Developers are responsible for realizing the stories identified by the Customer. Because different projects require a different mix of skills, and because the XP method relies on a cross-functional team providing the appropriate mix of skills, the creators of XP felt no need for further role definition.
The Tracker
Some teams may have a tracker as part of their team. This is often one of the developers who spend part of their time each week filling this extra role. The main purpose of this role is to keep track of relevant metrics that the team feels are necessary to track their progress and to identify areas for improvement. Key metrics that your team may track include velocity, reasons for changes to velocity, amount of overtime worked, and passing and failing tests.
This is not a required role for your team and is generally only established if your team determines a true need for keeping track of several metrics.
If your team is just getting started applying XP, you may find it helpful to include a Coach on your team. This is usually an outside consultant or someone from elsewhere in your organization who has used XP before and is included in your team to help mentor the other team members on the XP Practices and to help your team maintain your self-discipline.
The main value of the coach is that they have gone through it before and can help your team avoid mistakes that most new teams make.
To describe XP in terms of a lifecycle it is probably most appropriate to revisit the concept of the Weekly Cycle and Quarterly Cycle. First, start off by describing the desired results of the project by having customers define a set of stories. As these stories are being created, the team estimates the size of each story. This size estimate, along with relative benefit as estimated by the customer can provide an indication of relative value which the customer can use to determine the priority of the stories. If the team identifies some stories that they are unable to estimate because they don’t understand all of the technical considerations involved, they can introduce a spike to do some focused research on that particular story or a common aspect of multiple stories. Spikes are short, time-boxed time frames set aside for the purposes of doing research on a particular aspect of the project. Spikes can occur before regular iterations start or alongside ongoing iterations. Next, the entire team gets together to create a release plan that everyone feels is reasonable. This release plan is a first pass at what stories will be delivered in a particular quarter or release. The stories delivered should be based on what value they provide and considerations about how various stories support each other. Then the team launches into a series of weekly cycles. At the beginning of each weekly cycle, the team (including the customer) gets together to decide which stories will be realized during that week. The team then breaks those stories into tasks to be completed within that week. At the end of the week, the team and customer review progress to date, and the customer can decide whether the project should continue, or if a sufficient value has been delivered.
XP was first used on the Chrysler Comprehensive Compensation (C3) program which was initiated in the mid-’90s and switched to an XP project when Kent Beck was brought on to the project to improve the performance of the system. He wound up adding a couple of other folks, including Ron Jeffries to the team and changing the way the team approached development. This project helped to bring the XP methodology into focus and the several books written by people who were on the project helped spread knowledge about and adaptation of this approach.
Primary Contributions
XP’s primary contribution to the software development world is an interdependent collection of engineering practices that teams can use to be more effective and produce higher-quality code. Many teams adopting agile start by using a different framework and when they identify the need for more disciplined engineering practices they adopt several if not all of the engineering practices espoused by XP.
An additional, and equally important, contribution of XP is the focus on practice excellence. The method prescribes a small number of absolutely essential practices and encourages teams to perform those practices as well as they possibly can, almost to the extreme. This is where the name comes from. Not because the practices themselves are necessarily radical (although some consider some of them pretty far out) but rather because teams continuously focus so intently on continuously improving their ability to perform those few practices.
Further Reading
- Extreme Programming: A gentle introduction . By Don Wells
- Extreme Programming Explained – Embrace Change 2nd Edition by Kent Beck
- Extreme Programming Pocket Guide by Chromatic
< View all Glossary Terms | Provide feedback on this term
Thank you to our Annual Partners
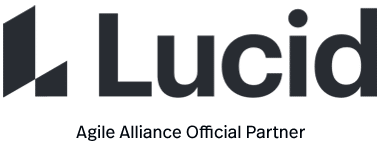
Join us today!
Agile Alliance offers many online and in-person events and workshops for our members. If you’re not currently a member, you can join now to take advantage of our many members-only resources and programs.
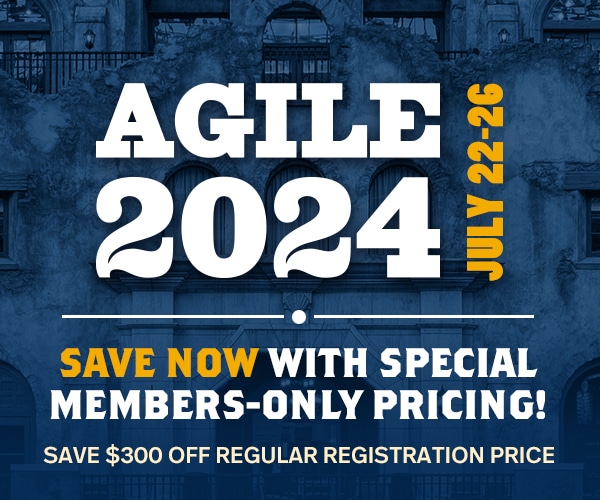
Get the latest Agile news!
- Phone This field is for validation purposes and should be left unchanged.
By subscribing, you acknowledge the Agile Alliance Privacy Policy , and agree to receive our emails.
Additional Agile Glossary Terms
Acceptance testing, heartbeat retrospective, product backlog, help us keep the definitions updated, discover the many benefits of membership.
Your membership enables Agile Alliance to offer a wealth of first-rate resources, present renowned international events, support global community groups, and more — all geared toward helping Agile practitioners reach their full potential and deliver innovative, Agile solutions.
Thank you to our valued Agile Alliance Annual Partners
Our new Annual Partner Program offers a new and exciting level of engagement beyond event sponsorship.
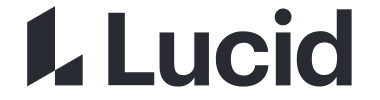
Our Cornerstone Corporate Supporting Members
Our Corporate Supporting Members are vital to the mission of Agile Alliance. Click here to view all corporate members.
©2024 Agile Alliance | All Rights Reserved | Privacy Policy
©2024 Agile Alliance All Rights Reserved | Privacy Policy
- Welcome back!
Not yet a member? Sign up now
- Renew Membership
- Agile Alliance Events
- Agile en Español
- Agile en Chile
- Resources Overview
- Agile Books
- Content Library by Category
- Content Standards
- Privacy Policy
- Cookie Policy
Privacy Overview
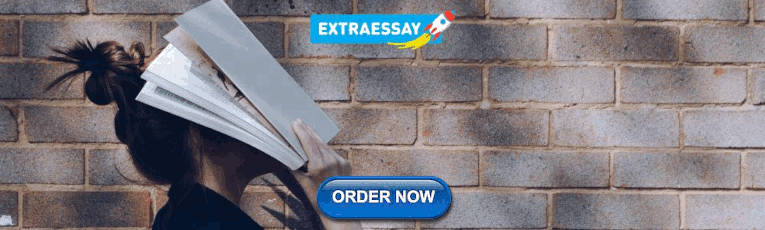
IMAGES
VIDEO
COMMENTS
Programming Methodologies - Introduction - When programs are developed to solve real-life problems like inventory management, payroll processing, student admissions, examination result processing, etc. they tend to be huge and complex. The approach to analyzing such complex problems, planning for software development and controlling the deve.
PROGRAMMING METHODOLOGY Making a Science Out of an Art by David Gries and Fred B. Schneider It doesn 't take too long for an intel-ligent, scientifically oriented person to ... can be used so simply to define the assignment statement. Other statements are defined similarly. For example, sequencing of two state-25 ments SO and S\ is defined ...
Software Development Methodologies are defined as a process in which the software developers design, develop and test the new computer programs and it is also used in the betterment of the software projects. ... XP or Extreme programming is also used to define the agile methodology whose main objective is to develop a fully functional product ...
This programming methodology is based on data and its movement. Program statements are defined by data rather than hard-coding a series of steps. A database is an organized collection of structured information, or data, typically stored electronically in a computer system. A database is usually controlled by a database management system (DBMS ...
2. Scrum Software Development Methodology. Agile software development comes in many flavors, and Scrum is one of the most popular with 70 percent of respondents to the State of Agile report saying that they practice Scrum or a Scrum hybrid. It is a framework for collaboration that was first invented by Jeff Sutherland in 1993.
A method in object-oriented programming (OOP) is a procedure associated with an object, and generally also a message. An object consists of state data and behavior; these compose an interface, which specifies how the object may be used. A method is a behavior of an object parametrized by a user. Data is represented as properties of the object ...
The birthday could define the state of an object and allow the software to handle dogs of different ages differently. Methods. Methods represent behaviors. Methods perform actions; methods might return information about an object or update an object's data. The method's code is defined in the class definition.
Programming is the mental process of thinking up instructions to give to a machine (like a computer). Coding is the process of transforming those ideas into a written language that a computer can understand. Over the past century, humans have been trying to figure out how to best communicate with computers through different programming languages.
The four phases of the spiral methodology are: Planning: The developers define their objectives at a given stage of development. Risk analysis: The developers predict risks and try to devise solutions for them. Engineering: The developers design and develop the product based on the previous phases.
The software development life cycle (SDLC) is the process of planning, writing, modifying, and maintaining software. Developers use the methodology as they design and write modern software for computers, cloud deployment, mobile phones, video games, and more. Adhering to the SDLC methodology helps to optimize the final outcome.
Most graphical signal processing environments do not define a language in any strict sense. In fact, ... DevOps is a newer programming methodology, which has sprung from the concept of agile development incorporating quicker turnaround and advocating a shortening of the development lifecycle. That being said, if DevOps is implemented without ...
Lean development provides a clear application for scaling agile practices across large or growing organizations. 5. Scrum software development methodology. Scrum is a system regularly used by software development teams. Like many software development methodologies, Scrum is agile, focusing on a value-driven approach.
method: In object-oriented programming , a method is a programmed procedure that is defined as part of a class and included in any object of that class. A class (and thus an object) can have more than one method. A method in an object can only have access to the data known to that object, which ensures data integrity among the set of objects ...
Extreme programming (XP) is a software development methodology intended to improve software quality and responsiveness to changing customer requirements. As a type of agile software development, it advocates frequent releases in short development cycles, intended to improve productivity and introduce checkpoints at which new customer requirements can be adopted.
Software development is the process of designing, writing (coding), testing, debugging, and maintaining the source code of computer programs. Picture it like this: Software developers are the architects, builders, quality control, and renovation crew all in one! They turn ideas into the functional software that shapes our digital experiences.
Extreme Programming (XP) is an Agile software development methodology that focuses on delivering high-quality software through frequent and continuous feedback, collaboration, and adaptation. XP emphasizes a close working relationship between the development team, the customer, and stakeholders, with an emphasis on rapid, iterative development ...
Summary. Agile methodology is a project management framework that breaks projects down into several dynamic phases, commonly known as sprints. In this article, get a high-level overview of Agile project management, plus a few common frameworks to choose the right one for your team. Scrum, Kanban, waterfall, Agile.
Scrum is an empirical process, where decisions are based on observation, experience and experimentation. Scrum has three pillars: transparency, inspection and adaptation. This supports the concept of working iteratively. Think of Empiricism as working through small experiments, learning from that work and adapting both what you are doing and ...
A programming methodology is concerned with: (1) the analysis of a problem by developing algorithms based on modern programming techniques, (2) designing programs in appropriate languages and (3) implementation on a suitable platform. Chapter 5 completes the programming methodology for the partition method for a power series expansion that ...
Methodology definition: a set or system of methods, principles, and rules for regulating a given discipline, as in the arts or sciences.. See examples of METHODOLOGY used in a sentence.
Change is also a constant in business (and, yes, life). Agile, in business, is a way of working that seeks to go with the flow of inevitable change rather than work against it. The Agile Manifesto, developed in 2001 as a way of optimizing software development, prioritizes individuals over processes, working prototypes over thorough documentation, customer collaboration over closed-door ...
What is Extreme Programming? Extreme Programming (XP) is an agile software development framework that aims to produce higher quality software and higher quality of life for the development team. XP is the most specific of the agile frameworks regarding appropriate engineering practices for software development.