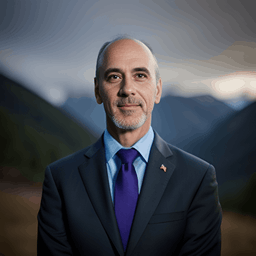
MachineLearningTutorials.org
Brief Machine Learning tutorials with examples.
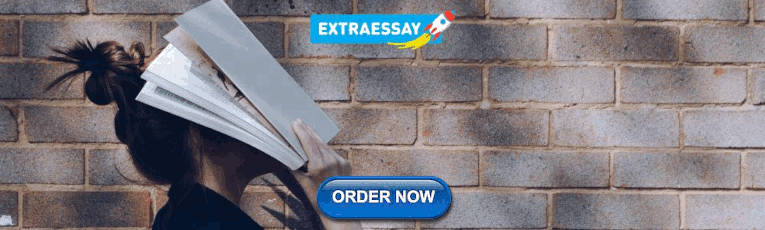
Python bytearray() Function: A Comprehensive Tutorial (With Examples)
In Python, the bytearray() function is a powerful tool that allows you to create a mutable sequence of bytes. This function is particularly useful when you need to manipulate binary data, work with low-level data structures, or modify existing byte sequences. In this comprehensive tutorial, we’ll dive deep into the functionality of the bytearray() function. We’ll cover its syntax, parameters, use cases, and provide multiple examples to help you understand how to use it effectively.
Table of Contents
- Introduction to bytearray()
- Syntax of bytearray()
- Parameters of bytearray()
- Creating a bytearray()
- Modifying bytearray() Elements
- Converting bytearray() to Other Types
- Common Use Cases
- Example 1: Manipulating Binary Data
- Example 2: Network Communication
1. Introduction to bytearray()
In Python, a bytearray() is a mutable sequence of bytes. A byte is the fundamental unit of data storage and manipulation in computers. Unlike strings, which are sequences of characters, bytearrays allow you to work with binary data directly. This is particularly useful when dealing with files, network protocols, cryptographic operations, and various other scenarios where data needs to be manipulated at the byte level.
2. Syntax of bytearray()
The syntax for creating a bytearray using the bytearray() function is as follows:
- source (optional): This parameter can be an iterable (such as a list, tuple, or string) containing integers in the range 0 to 255. Each integer represents a byte value. If this parameter is omitted, an empty bytearray is created.
- encoding (optional): The character encoding to be used when converting a string to bytes. If not provided, the default encoding (usually UTF-8) is used.
- errors (optional): Specifies how encoding and decoding errors should be handled. Common values include 'strict' , 'ignore' , and 'replace' .
3. Parameters of bytearray()
Let’s take a closer look at the parameters of the bytearray() function:
- source (optional): The source parameter can be an iterable, such as a list, tuple, or string, containing integer values in the range of 0 to 255. This parameter is used to initialize the contents of the bytearray. If omitted, an empty bytearray is created.
- encoding (optional): This parameter is only applicable when the source parameter is a string. It specifies the character encoding to be used when converting the string to bytes. Common encodings include 'utf-8' , 'ascii' , 'latin-1' , etc. If not provided, the default encoding (usually UTF-8) is used.
- errors (optional): When the encoding parameter is specified, this parameter determines how encoding and decoding errors should be handled. Possible values include:
- 'strict' : Raises an exception on errors (default).
- 'ignore' : Ignores errors and continues decoding.
- 'replace' : Replaces erroneous characters with a replacement character.
4. Creating a bytearray()
Creating a bytearray using the bytearray() function involves passing an iterable (or nothing) containing integer values in the range of 0 to 255. Let’s explore some examples:
Example 1: Creating an Empty bytearray()
In this example, we create an empty bytearray with no initial values.
Example 2: Creating a bytearray() from an Iterable
In this example, we create a bytearray by passing a list of ASCII values corresponding to the characters ‘A’, ‘B’, ‘C’, and ‘D’. The resulting bytearray contains the binary representation of these ASCII values.
5. Modifying bytearray() Elements
One of the key advantages of using a bytearray() is its mutability. You can modify individual elements within the bytearray just like you would with a list. This allows you to manipulate binary data efficiently.
Let’s explore how to modify elements within a bytearray() using indexing:
In this example, we modify the second element of the bytearray to change the value from 66 (ASCII value of ‘B’) to 69 (ASCII value of ‘E’).
6. Converting bytearray() to Other Types
While bytearrays are useful for binary data manipulation, you might sometimes need to convert them to other types for specific operations. You can convert a bytearray to different types, such as bytes, strings, or hexadecimal strings.
Converting bytearray() to Bytes
To convert a bytearray() to bytes, you can use the built-in bytes() constructor:
In this example, the bytes() constructor converts the bytearray to bytes.
Converting bytearray() to String
To convert a bytearray() to a string, you can use the decode() method with the appropriate encoding:
Here, we decode the bytearray using the UTF-8 encoding to obtain the string representation.
Converting bytearray() to Hexadecimal String
To convert a bytearray() to a hexadecimal string, you can use the binascii module:
In this example, we use the hexlify() function from the binascii module to convert the bytearray to a hexadecimal string.
7. Common Use Cases
The bytearray() function finds its application in various scenarios. Some common use cases include:
- File Manipulation: Bytearrays are often used when reading and writing binary files. They allow efficient modification of file contents at the byte level.
- Network Communication: When working with network protocols, bytearrays can represent packets of data that need to be sent or received.
- Cryptography: Cryptographic operations often involve manipulating binary data. Bytearrays are useful for performing encryption, decryption, and hashing operations.
- Data Serialization: Bytearrays can be used to serialize complex data structures into binary form, which can then be saved to disk or transmitted over a network.
- Image Processing: Image data is often represented as binary data. Bytearrays can be used to manipulate image pixel values directly.
- Low-Level Operations: In certain cases, you might need to work with memory-mapped files or low-level data structures. Bytearrays provide a convenient way to interact with memory.
8. Example 1: Manipulating Binary Data
Let’s consider an example where we read binary data from a file, perform some manipulations on the data, and write the modified data back to the file. In this example, we’ll reverse the byte order of the data.
In this example, the reverse_bytes_in_file() function reads the binary data from the specified file, reverses the byte order using the reverse() method of the bytearray , and then writes the modified data back to the file.
9. Example 2: Network Communication
Let’s consider a simple example of sending and receiving data over a network using the socket module and bytearray() . In this example, we’ll create a simple server and client to exchange messages.
In this example, the server sends a message to the client using a bytearray, and the client receives and decodes the message. This showcases how bytearrays can be used to exchange binary data over a network.
10. Conclusion
In this tutorial, we’ve explored the functionality of the bytearray() function in Python. We’ve covered its syntax, parameters, and various use cases. With bytearrays, you can work with binary data efficiently, whether you’re manipulating files, communicating over networks, performing cryptographic operations, or working with low-level data structures. The ability to modify individual elements within a bytearray makes it a powerful tool for manipulating binary data at the byte level. By understanding the concepts presented in this tutorial, you’ll be well-equipped to leverage the capabilities of bytearrays in your Python projects.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Skip to primary navigation
- Skip to main content
- Skip to primary sidebar

PythonForBeginners.com
Learn By Example
Bytearray in Python
Author: Aditya Raj Last Updated: July 8, 2021
You must have studied different data types in python such as strings and numeric data types like integers and floating point numbers. In this article you will learn about another data type called bytearray in python programming language. You will study the underlying concepts behind bytearray in python and will implement different types of operations on bytearray objects to understand the concepts.
What is bytearray in Python?
A bytearray in python is an array of bytes that can hold data in a machine readable format. When any data is saved in the secondary storage, it is encoded according to a certain type of encoding such as ASCII, UTF-8 and UTF-16 for strings, PNG, JPG and JPEG for images and mp3 and wav for audio files and is turned into a byte object. When we access the data again using python read file operation, it is decoded into the corresponding text, image or audio. Thus byte objects contain data that are machine readable and bytearray objects are arrays of bytes.
How to create bytearray objects in Python?
We can create a bytearray object in python using bytearray() method. The bytearray() function takes three parameters as input all of which are optional. The object which has to be converted to bytearray is passed as the first parameter. Second and third parameters are used only when the first parameter is string. In this case, the second parameter is the encoding format of the string and the third parameter is the name of the error response which is executed when the encoding fails. The bytearray() function returns a bytearray object. In the next sections, we will understand the working of bytearray() function by creating bytes objects from different data objects.
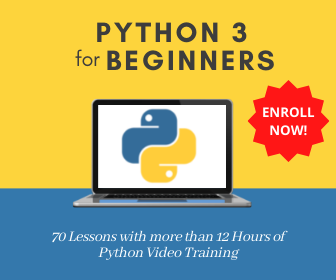
Create a bytearray object
To create a bytearray object of a given size, we can give the desired size of bytearray as input to the bytearray() function. After successful execution, it returns the bytearray object of given size initialized to zeros as follows.
To convert a string to bytearray object, we pass the string as first input and encoding type as second input argument to the bytearray() function. It then returns the bytearray of string as follows.
We can convert a list of integers to bytearray using bytearray() function in python. The bytearray() function takes the list of integers between 0 and 255 as input and returns the corresponding bytearray object as follows.
For integer values which are not between 0 to 255, the bytearray function raises ValueError as follows.
We can handle the above exception using python try-except as follows.
Operations on bytearray objects
Although byte objects are immutable, bytearray objects are mutable and can be modified and they almost behave as python lists. Following are some common operations on bytearray objects.
Bytearray supports indexing and slicing. We can use indices to get the data at a particular index or we can slice a bytearray to get data between two indices as follows.
As bytearray objects are mutable, we can also modify the bytearray objects using indexing and slicing as follows.
We can also insert data into a bytearray object at a given index using insert() method as follows.
We can append data into a bytearray object using the append() method as follows.
We can also delete data at a specific index or between two indices using del statement as follows.
In this article, we have studied the bytearray data structure in python. We have also converted different data types to bytearray and have performed operations like appending data to a bytearray, insertion of data and deletion of data from a bytearray using built in functions.Stay tuned for more informative articles.
Recommended Python Training
Course: Python 3 For Beginners
Over 15 hours of video content with guided instruction for beginners. Learn how to create real world applications and master the basics.
More Python Topics

Python bytearray(): Manipulating Low-Level Data Efficiently

The Python bytearray() function converts strings or collections of integers into a mutable sequence of bytes. It provides developers the usual methods Python affords to both mutable and byte data types. Python’s bytearray() built-in allows for high-efficiency manipulation of data in several common situations.
- 1 Rationale
- 2 Basic Use & Syntax
- 3 Byte Strings vs. Byte Arrays
- 4 Mutable vs. Immutable
- 5.1 Escaped Character Sequences
- 5.2 Default Values
- 6 Final Thoughts
The Python bytearray() function’s powerful features do come with some responsibility. Developers must be mindful of encodings, be aware of source data format, and have a basic working knowledge of common character sets like ASCII .
In this article, you’ll learn the rationale, common use cases, advanced use cases, and potential pitfalls of Python’s bytearray() built-in function. There’s even a section with some notable quirks at the bottom.
TL;DR — Python’s bytearray() function converts strings and sequences of integers into bytes to provide developers the ability to efficiently update (mutate) data without additional memory allocation.
The bytearray object was introduced in Python 2 and has served as a ‘ mutable counterpart ‘ to the bytes object since. Unlike the bytes object, the bytearray object doesn’t come with a literal notational representation and must be instantiated directly.
For example, b'string' provides literal representation for a bytes object whereas developers must use the syntax bytearray('string', encoding='ascii') to create a new bytearray object. I regard this as only a minor inconvenience given the benefits Python’s bytearray data type provides developers.
Data like strings are regarded as immutable (more on that below) meaning they can’t be changed directly. To change (mutate) a string, developers must copy the string and assigned the new version to an object located in memory. Consider the following example:
Note that our string has been changed but now has a different id (memory location.) That means that a is now a different object in memory and our original object is garbage. That’s all well-and-good when one is changing around a few strings.
Such easy string manipulation is one of the language features that make Python such a popular programming language . Unfortunately, this approach isn’t efficient for changing large numbers of such data. That’s where the power of the bytearry() function shines.
The official Python documentation describes the intended usage of bytearry() as follows:
The bytearray class is a mutable sequence of integers in the range 0 <= x < 256. It has most of the usual methods of mutable sequences, described in Mutable Sequence Types, as well as most methods that the bytes type has, see Bytes and Bytearray Operations.
The reference to the Bytes and Bytearray Operations documentation should not be passed over either. That reference provides the following elaboration:
Since 2 hexadecimal digits correspond precisely to a single byte, hexadecimal numbers are a commonly used format for describing binary data.
This will make more sense in just a bit when we take a look at cases where the bytearray() function displays escaped character sequences. For now, let’s consider some common cases and basic syntax!
Basic Use & Syntax
The bytearray() function returns a new array of bytes. This can result from an input string or a collection of integers in the range 0-255 (inclusive). If you are familiar with binary notation you may have already guessed that makes perfect accommodation for eight-bit representations .
Python’s built-in bytearray() takes three possible arguments:
- source – the data to be converted to an array of bytes, either a string or a collection of integers.
- encoding – required for strings, optional for collections of integers
- errors – an optional parameter to specify error handling. See here for options .
Let’s take the bytearray() function for a quick spin to demonstrate the basic use of these arguments:
Well, that didn’t go well. Bytes are essential just numerical representations of information. Without knowing how to interpret that information, Python will make a fuss. Arguably, a default encoding could be used but that would cause another set of problems. Let’s see what happens when we let Python know how to interpret the data:
Now we’ve got a bytearray object in memory signifying as much by showing a bytes literal within parenthesis (more on that below.) Let’s take a look at how the bytearray() function handles a series of integer values. Remember, only values in the range of 0 < val < 255 are considered valid here.
Well, that didn’t go well either. When given a series of numbers, Python doesn’t need to know the encoding because it limits the values to 0-255. The character representation of any value in this range is assumed to be of the ISO8859-1 standard, more commonly known as the Latin-1 character set (a superset of the ASCII encoding.) Let’s try this again:
Now we’ve got a bytearray object from a list of integer values! But what can we do with this? This is where the bytearray() function starts demonstrating its utility as a mutable object. We’ll cover what mutable vs. immutable means in just a second. Before we get there, however, let’s stop for a second and consider what the difference between a byte string and a byte array might be.
Byte Strings vs. Byte Arrays
At this point, you may be wondering what the difference between a sequence of bytes and a collection of bytes might be. In a word— mutability . Both represent data in binary format, both can easily handle two-digit hexadecimal inputs, and both rely on developers to be mindful of encodings to ensure proper representation of numerical values and the resulting translations. So what gives?
Bytes are treated with more finality than bytearray objects. That is, they are suited for applications where one doesn’t need to change the data often . For example, a bytes object would be better suited for storing data received from a network transmission. For large transmissions, maybe even as an array of bytes (but not bytearray objects.)
The bytearray object is better suited for situations where frequent manipulation of data is expected . For example, the updating of data received from a network transmission before retransmission. bytearray objects also provide methods afforded to mutable objects such as sizing, slicing, member assignment, insertion, reversal, and many more.
To fully appreciate the difference between Python’s bytes and bytearay objects we’ll need to consider the difference between mutable vs. immutable data types. Don’t worry, it’s a fairly simple concept and with a basic example one that’s easy to grasp!
Mutable vs. Immutable
In Python, strings, numbers, and tuples are immutable—meaning their values can’t be changed. They can be copied or reassigned but not manipulated directly. Data structures like lists, dictionaries , and sets are mutable and allow much more efficient manipulation of their constituent parts.
Specifically, additional memory allocation is minimized when updating part of data contained in the middle of a list whereas updating the middle of a string would require a near-doubling. This describes how byte arrays can be applied in representing immutable data types to improve efficiency in certain contexts.
Let’s review our original TL;DR example from above, this time with a little more explanation:
Here we’ve shown that trying to directly change the text of our string throws a TypeError . Storing that string as a byte array allows direct manipulation of the individual character values. After manipulation, we can then convert the resulting values to a string via the decode() method—ensuring to specify the proper encoding.
Is this an example of efficient use of the bytearray() function? Probably not. Imagine having to update large sequences of textual data, such as in many database applications or large network data consumptions. The ability to manipulate data without allocating additional memory would be essential. That’s where byte arrays really shine.
TL;DR – representing a string as an array of bytes allows one to manipulate it more efficiently. There is an initial overhead cost in memory but a decreasing cost as manipulative operations amass. Always mind your encoding.
The bytearray() function deals with some pretty nuanced utility. This makes no surprise of the sometimes spooky behavior exhibited by this built-in. Below are a few cases where the bytearray() function might behave in ways that might need some explanation. These certainly aren’t bugs but should be kept in mind nonetheless.
Escaped Character Sequences
Given the bytearray() restriction of valid integer arguments to a range of 0-255 one might expect some quicky behavior to arise. A ValueError gets thrown anytime a non-compliant value is attempted—but that’s expected. What’s quirky is how Python will represent ordinal values for non-printable characters. Consider the following:
Note that bytearry() will display escaped characters regardless of how an array of integers is passed in ( \x prefix.) However, note that the characters with integer values matching ordinal values of printable characters are represented as that character. In other words: 1 is displayed as \x01 but 65 is displayed as A since it’s the ordinal value of a printable character. Check out the article on Python’s ord() function to get a feel for that interoperability.
Default Values
The bytearray() can be initialized as an empty array or one with default values by passing a non-iterable integer argument. Consider the following:
Note here that passing the integer value of 5 as an argument initializes the bytearray() object with five values of null —represented by the escaped character sequence \x00 . The documentation covering valid bytearray() arguments isn’t entirely clear on this point in my opinion and can lead to some confusion. Note the last example places the 5 in Python’s bracketed list notation. This results in a bytearray() object of length one with the single escaped character sequence of \x05 . Again, not a bug but certainly quirky. Note : null is not the same as 0 .
Final Thoughts
Python’s bytearray() function provides an easy-to-use utility for memory-efficient manipulation of data. Developers of network applications, large string-processing use cases, and programs that encode and decode large swaths of data can all stand in admiration of its high-level accessibility of low-lever data.
Python certainly isn’t the canonical language for developing memory-efficient applications—at least not directly. The bytearray() function offers the utility that makes a case that, while not the canonical choice, Python is certainly up to the task if needed. For more insight into the handling of binary data check out the article What’s a Byte Stream Anyway ?
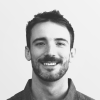
Python Reduce(): A Powerful Functional Programming Made Simple
Have you ever found yourself wondering what all the hype is about functional programming? This ...
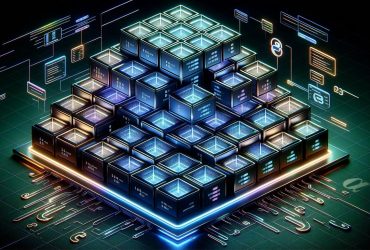
Python’s DefaultDict: Hash Tables Made Easy
Hash Tables do wonders for search operations but can quickly cause syntactic clutter when used ...
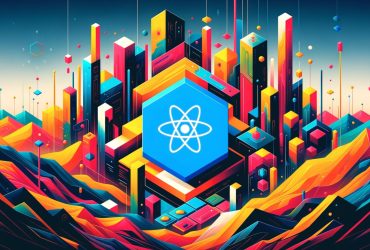
ESLint + React Functional Component Parameter Destructuring
React and ESLint are both amazing tools for building modern applications. Getting them to work ...


Python, C, C++, C#, PowerShell, Android, Visual C++, Java ...
Python – bytearray – A quick walk through with working Examples
A bytearray is also another sequence type in Python; which holds a sequence of bytes. Unlike bytes; bytearray objects are mutable. That means, we can modify the elements in bytearray objects.
Define a bytearray Object
bytearray objects are created using the bytearray class constructor. There is no relevant literal syntax to create bytearray objects.
The syntax of the bytearray constructor is:
Syntax: bytearray([source [, encoding [, errors] ] ])
Note that , all the arguments to the bytearray constructor are optional. If you do not pass any arguments; Python creates a bytearray object with NO elements; that means, an empty instance.
In our previous Article “ Python – Ranges – A walk through with Examples “, we have discussed ranges; which is a collection of integers. Below is the statement, creates bytearray object using elements of a range object: which creates alternate numbers from 1 to 20 (excluding).
Observe that , when we print the elements of a bytearray ; it prints hexadecimal numbers, which is the usual format Python use to display bytearray elements.
Even we can create bytearray object using bytes object. Below statement shows this:
Another interesting thing with bytearray is; it allows to create a bytearray object with zeros filled-in, with a given length. This is very useful when you do not know what values need to be added to the bytearray , but you know the length of it. On the fly, you can add the values, once known. The below statement creates a bytearray object; filled with all zeros:
Access bytearray elements
Like other sequence types, bytearray is also allowed to access its’ elements using the index operator. Index starts from “0” and the index of the last element is (number of elements – 1). It also supports, negative index numbering to access the elements from the end. “-1” is the start index from the end.
If you attempt to give an invalid index value; the Python interpreter throws IndexError . For example , if you attempt to access an element from an empty instance (“ba1”, what we have created from above), will throw an Error:
Below are the statements to access byetearray object’s first and last elements.
Modify bytearray elements
As mentioned above, bytearray is mutable. That means it allows to modify its’ elements. We can use the index operator and “=” sign to assign/modify bytearray elements.
From above, “ ba4 ” bytearray object, contains all zeros. Now we will modify one of its’ elements and display the result.
Observe that , “A” (ASCII value is 65) was added at the 4th position (index value is 3) and shown in the result when we display it.
I gave a very quick introduction about bytearray . We will discuss more sequence types in the upcoming Articles.
2 thoughts on “ Python – bytearray – A quick walk through with working Examples ”
- Pingback: Python – memoryview – A Quick Overview with Examples - CodeSteps
- Pingback: Python – memoryview – A Quick Overview with Examples – CodeSteps
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Python bytearray() Method
The bytearray() method returns a bytearray object, which is an array of the given bytes. The bytearray class is a mutable sequence of integers in the range of 0 to 256.
Parameters:
- If the source is a string, it must be with the encoding parameter.
- If the source is an integer, the array will have that size and will be initialized with null bytes.
- If the source is an object conforming to the buffer interface, a read-only buffer of the object will be used to initialize the bytes array.
- If the source is the iterable object, it must have integer elements only in the range 0 to 256.
- encoding: (Optional) The encoding of the string if the source is a string.
- errors: (Optional) The action to take if the encoding conversion fails.
Return Value:
Returns an array of bytes.
The following example converts integer to bytearrays.
If the source argument is a string and no encoding method is specified, a TypeError exception is returned.
The encoding method needs to be specified to return a bytearray object of the string, as shown below.
An iterable can also be converted to a bytearray object, as shown below.
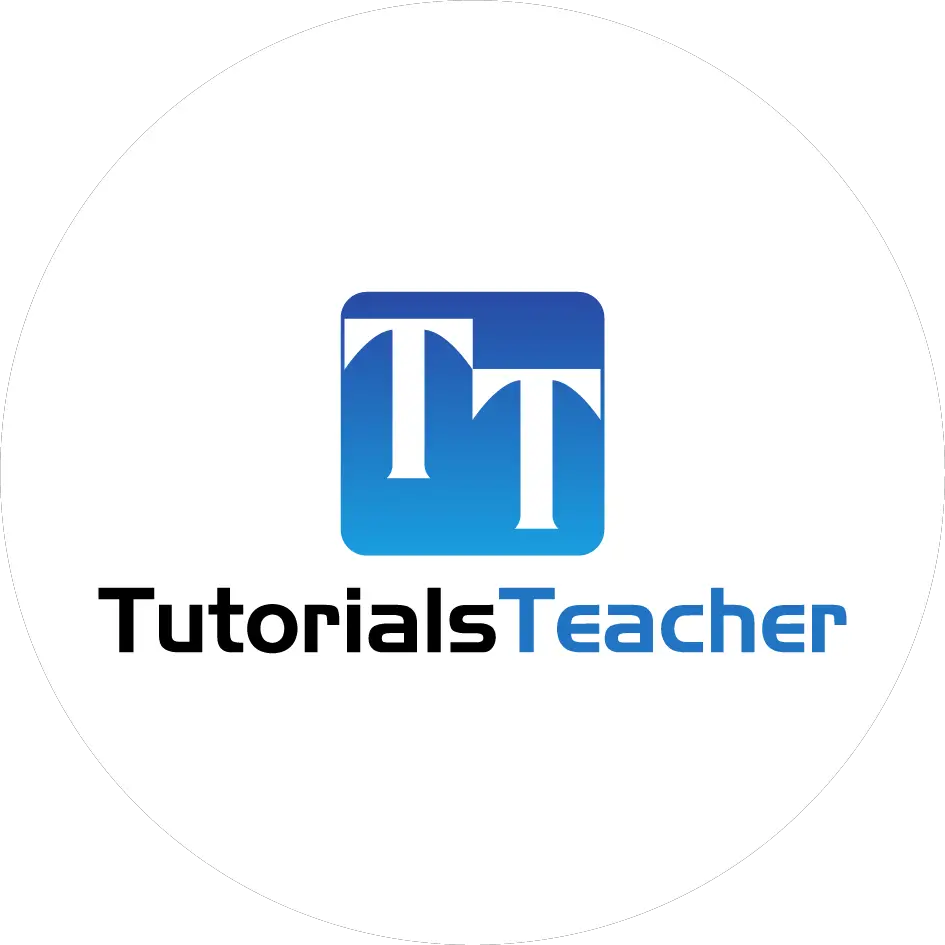
We are a team of passionate developers, educators, and technology enthusiasts who, with their combined expertise and experience, create in -depth, comprehensive, and easy to understand tutorials.We focus on a blend of theoretical explanations and practical examples to encourages hands - on learning. Visit About Us page for more information.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, built-in functions.
- Python abs()
- Python any()
- Python all()
- Python ascii()
- Python bin()
- Python bool()
Python bytearray()
- Python callable()
Python bytes()
- Python chr()
- Python compile()
- Python classmethod()
- Python complex()
- Python delattr()
- Python dict()
- Python dir()
- Python divmod()
- Python enumerate()
- Python staticmethod()
- Python filter()
- Python eval()
- Python float()
- Python format()
- Python frozenset()
- Python getattr()
- Python globals()
- Python exec()
- Python hasattr()
- Python help()
- Python hex()
- Python hash()
- Python input()
- Python id()
- Python isinstance()
- Python int()
- Python issubclass()
- Python iter()
- Python list() Function
- Python locals()
- Python len()
- Python max()
- Python min()
- Python map()
- Python next()
Python memoryview()
- Python object()
- Python oct()
- Python ord()
Python open()
- Python pow()
- Python print()
- Python property()
- Python range()
- Python repr()
- Python reversed()
- Python round()
- Python set()
- Python setattr()
- Python slice()
- Python sorted()
Python str()
- Python sum()
- Python tuple() Function
- Python type()
- Python vars()
- Python zip()
- Python __import__()
- Python super()
Python Tutorials
- Python String encode()
- Python Programming Built-in Functions
The bytearray() method returns a bytearray object which is an array of the given bytes.
bytearray() Syntax
The syntax of bytearray() method is:
bytearray() method returns a bytearray object (i.e. array of bytes) which is mutable (can be modified) sequence of integers in the range 0 <= x < 256 .
If you want the immutable version, use the bytes() method.
bytearray() Parameters
bytearray() takes three optional parameters:
- source (Optional) - source to initialize the array of bytes.
- encoding (Optional) - if the source is a string , the encoding of the string.
- errors (Optional) - if the source is a string, the action to take when the encoding conversion fails (Read more: String encoding )
The source parameter can be used to initialize the byte array in the following ways:
bytearray() Return Value
The bytearray() method returns an array of bytes of the given size and initialization values.
Example 1: Array of bytes from a string
Example 2: array of bytes of given integer size, example 3: array of bytes from an iterable list.
Sorry about that.
Python References
Python Library
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python | bytearray() function
- Convert Bytearray To Bytes In Python
- numpy.byte_bounds() function – Python
- numpy.fromiter() function – Python
- Python str() function
- Python Array length
- numpy.frombuffer() function – Python
- numpy.dtype.base() function - Python
- Numpy recarray.byteswap() function | Python
- Python: Operations on Numpy Arrays
- Python slice() function
- NumPy Array in Python
- Python bytes() method
- Array in Python | Set 2 (Important Functions)
- Python | Numpy ndarray.item()
- hex() function in Python
- Python Arrays
- numpy.matrix.A() function - Python
- p5.js byte() function
- Ruby | StringIO bytes function
- Adding new column to existing DataFrame in Pandas
- Python map() function
- Read JSON file using Python
- How to get column names in Pandas dataframe
- Taking input in Python
- Read a file line by line in Python
- Enumerate() in Python
- Dictionaries in Python
- Iterate over a list in Python
- Different ways to create Pandas Dataframe
bytearray() method returns a bytearray object in Python which is an array of given bytes. It gives a mutable sequence of integers in the range 0 <= x < 256.
Syntax of bytearray() Function in Python
Python bytearray() function has the following syntax:
Syntax: bytearray(source, encoding, errors) Parameters: source [optional] : Initializes the array of bytes encoding [optional] : Encoding of the string errors [optional] : Takes action when encoding fails Returns: Returns an array of bytes of the given size. source parameter can be used to initialize the array in few different ways. Let’s discuss each one by one with help of examples.
Python bytearray() Function Examples
Let us see a few examples, for a better understanding of the bytearray() function in Python.
bytearray() Function on String
In this example, we are taking a Python String and performing the bytearray() function on it. The bytearray() encodes the string and converts it to byte array object in python using str.encode().
bytearray() Function on an Integer
In this example, an integer is passed to the bytesrray() function, which creates an array of that size and initialized with null bytes and return byte array object in Python.
bytearray() Function on a byte Object
In this example, we take a byte object. The read-only buffer will be used to initialize the bytes array and return the byte array object in Python.
bytearray() Function on a List of Integers
In this example, We take a Python List of integers which is passed as a parameter to the bytearray() function.
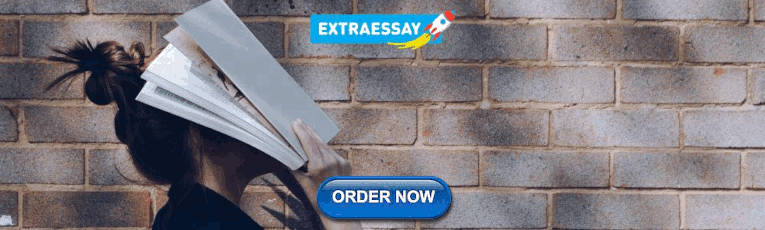
bytearray() Function with no Parameters
If no source is provided to the bytearray() function, an array of size 0 is created and byte array object is returned of that empty array.
Please Login to comment...
Similar reads.
- Python-Built-in-functions

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
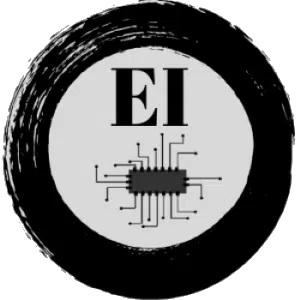
Python ByteArray: Everything you need to know!
In this article, let us learn about the ByteArray data structure in Python and learn how and when to use it in our Python programs.
For those of you in a hurry here is the short version of the answer.
ByteArray in a Nutshell
ByteArray is a data structure in Python that can be used when we wish to store a collection of bytes in an ordered manner in a contiguous area of memory .
ByteArray comes under binary data types.
You can use the bytearray() constructor to create a ByteArray object as shown below
Here the syntax we have used is
Depending on the type of data we wish to convert into an array of bytes, the ByteArray class gives us 4 different constructors are shown in the table below.
Examples of how to use each one are given further down in the article.
The table below shows the most commonly used methods in the ByteArray class
Examples of how to use these above methods, along with some more useful methods are given later on in the article .
If you are a visual learner, we made a YouTube video on ByteArray which you can find below!
If the above answer feels insufficient do not worry as this section was meant to be a quick reference for those who are already familiar with the topic. Read on for a more complete answer where we have explained everything you need to know about ByteArrays with the help of examples!
Feel free to skip to your topic of interest using the table of contents below.
What are ByteArrays and When to use them?
As explained previously, ByteArray is just a data structure in Python. Each data structure is useful in solving a particular kind of problem.
The ByteArray data structure is very useful when we wish to store a collection of bytes in
- an ordered manner and
- in a contiguous area of memory.
But when will the above properties of ByteArrays come in handy?
ByteArrays can be used in the following situations where we need to be sending and receiving
- numerical data
- string data
- images in RGB format and
- other binary files
via a communication channel like USB, Ethernet, Wi-Fi, etc. to another device.
For example, say you have a USB microphone recording. That microphone is sending in audio to your python script. You can receive such audio data in a ByteArray object and process it by decoding it.
Similarly, you can also use ByteArrays to send out audio to a loudspeaker.
ByteArrays can also be used as a buffer for sending data from your Python script to a Python library for data processing.
For example, say you are writing an image recognition library that processes images. As we know everything in computers is basically stored in 0’s and 1’s. You can receive these images as a ByteArray object and do the necessary processing.
ByteArrays are a good way to pack and process binary data.
This is because ByteArrays store the data given in a contiguous block of memory and hence are very efficient in the above scenarios when it comes to speed.
Discussion on “What ByteArrays are” is never complete without comparison with its cousin “Lists” which is what we will address next!
How ByteArrays differ from Lists?
Lists can have data placed in different areas of memory and this makes it very inefficient to transmit data . This is because we constantly need to update the addresses of where the next piece of data is and this will cause unnecessary delays in transmission.
ByteArrays, on the other hand, have their data placed in contiguous areas of memory and this makes it very efficient to transmit data, as we can simply increment the address to get the next byte of data
If the above explanation raises more questions than answers, I suggest you read our other article on lists given below.
Python Lists: Everything You Need To Know!
Now that we are talking about similar datastructures, it is worth mentioning the “ immutable brother ” of bytearrays , i.e the Bytes datastructure. As with Lists we have an entire article dedicated to Bytes which you can find in the link below.
Python Bytes: Everything you need to know!
If the term immutable feels new to you, I suggest heading over to our article that breaks down this concept of mutability and immutability for you. The link below will take you there!
Mutable and Immutable Data Types in python explain using examples
Now that we have learned what ByteArrays are and when to use them, let us go ahead and learn how to use the ByteArray data structure in our code!
Initializing a ByteArray object
ByteArray class gives us a variety of constructors to choose from depending upon the type of data we wish to store in our ByteArray object. They are listed in the table below
Storing List of Integers (Iterable of ints)
Lists of integers can be used to represent not just Mathematical problems, but also multimedia data like audio, pictures, etc. This is because all data can be boiled down to a list of integers.
Hence integer-list is the most common datatype you will see being used with the ByteArray class.
You can use the bytearray(iterable_of_ints) constructor to create a ByteArray object from a list of integers as shown in the example below
As we can see,
- 1 becomes 0x01
- 2 becomes 0x02
Let us take another quick example to learn an important concept
This time our program crashed with an error.
So why the list [1, 2, 3, 4] work but the list [1, 2, 3, 300] doesn’t work?
This is because a single byte can only contain integers in the range (0, 255) and the integer 300 is outside this range.
Hexadecimals and Bytes
1 Byte = 8 Bits
and 8 bits can be arranged in 2 8 = 256 different combinations which give us numbers from 0 to 255.
If you find this concept hard to grasp I recommend researching and learning how hexadecimal numbers work.
Now that we have covered how to convert the most common type of data, i.e. integers to ByteArrays, next let us address the 2nd most common datatype: “Strings”!
Storing a String
When working with strings we can use the following syntax to create the byte array from a string.
For example
Here the string “Python” is encoded using the “utf-8” encoding and then passed into the bytearray.
How does encoding work?
As we know computers treat everything as 0’s and 1’s. So to represent the letter ‘A’ we can use the byte 0x41, ‘B’ with 0x42, and so on as shown in the table below.
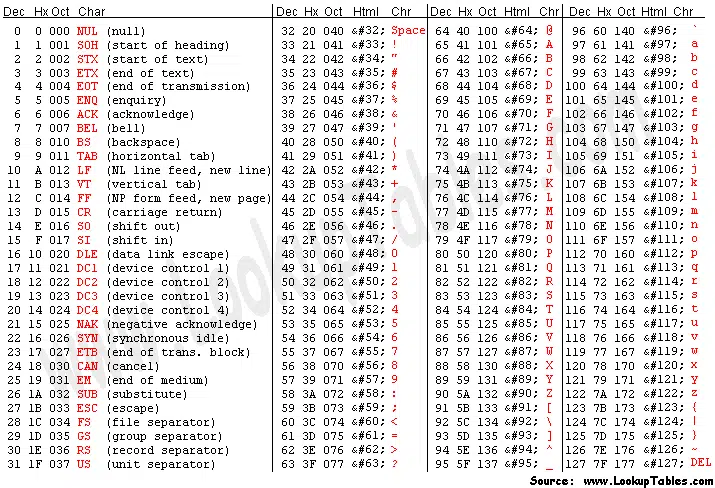
This type of mapping integers to characters is called ASCII encoding. But it is not the only encoding available out there. The latest ones are utf-8 and utf-16 encodings which simply use different numbers to map to different symbols.
Covering different types of encoding is out of the scope of this article, so if you are interested to learn more, I suggest googling!
Let us take another example to see how to use the “errors” parameter.
Notice I have used the Euro character “€” which is not part of ASCII encoding and it got replaced with the “?” (question mark) symbol. The most common options for the “error” parameter are shown in the table below.
The code below shows the usage of “ignore” and “strict” options
As you can see,
- when we use the ignore option, the character which cannot be encoded, the “€” symbol is simply ignored
- when we use the strict option, we get an error!
If you wish to see more examples on how to use strings with ByteArrays , head over to the article linked below.
Using Strings With ByteArray in Python
Now that we have learned how to use the string constructor of the ByteArray class, next, let us have a look at how you can convert an object of the Bytes class into ByteArray class.
Converting Bytes into ByteArray
Let’s assume you are working with a read-only image and you wish to modify it, then first you need to make an editable copy. Situations like this are where our next constructor comes in.
As mentioned previously, the Bytes class is just an immutable version of the ByteArray class, i.e. an object of the Bytes class cannot be modified once created.
This is illustrated in the code below.
- we have a bytes object named mybytes
- if we try to change the 2nd byte we get a TypeError with the error message ” ‘bytes’ object does not support item assignment”
- so we make an editable copy using ByteArray class
- then we can edit the array
Here the syntax used is
You can find a comprehensive comparison between the 2 datastructures here
bytearray vs bytes, Similarities & Differences Explained!
So far we have constructed ByteArray object from already available data, to end this section let us see how to create an empty ByteArray object to which we can add stuff later on.
Creating Placeholders
If we wish to create an empty ByteArray class without any elements you can use the following syntax
As you can see the array is empty.
If we wish to create a ByteArray class with X number of elements all initialized to zeros, we can use the following syntax
As you can see the array has 10 bytes, all of which are zeros.
Now that we have learned how to create/construct ByteArray objects, the next step is to learn how to manipulate values in an existing ByteArray object.
Manipulating contents of a ByteArray object
Accessing items in a bytearray object.
We can access the elements of the bytearray using the index operator , i.e. the square brackets “[ ]”. The index always starts from 0 and the index of the last element is the total number of elements minus 1. Hence accessing elements of ByteArray class works the exact same way as the Lists class.
Let us see a simple example
Here the value at index-1 is the integer 1 and hence it is printed.
We can use the same syntax to modify elements if needed
As you can see, we have changed the element at index-1 to 3.
Let us next see how to
- add elements
- remove elements and
- make a copy of a ByteArray
If you are familiar with Lists then all the methods mentioned in this section will be very familiar to you.
Adding Elements
ByteArray provides 3 methods for adding elements to an existing ByteAray object. They are
- append() method
- extend() method and
- insert() method
The append() method
To add an element to the end of a ByteArray, we use the append() method as shown in the following example
As you can see the element “3” got added to the end of our array.
The syntax to use with the append() method is
The extend() method
If we wish to add all the elements of one ByteArray object to another ByteArray object we use the extend() method as shown in the following example
As you can see, all the elements from myOtherArray (“4”, “5”, and “6”) got added to the end of the 1st array.
The syntax to use with the extend() method is
You can find more examples for append() and extend() methods in the following article.
ByteArray: Append & Extend, Explained with Examples!
The insert() method
If we wish to add a specific element at a particular index then we can use the insert method as shown in the following example.
As you can see element “2” is inserted at index-1 between elements “1” and “3”.
The syntax to use with the insert() method is
Removing Elements
The ByteArray class gives 3 methods for removing elements from ByteArrays. They are
- remove() method
- pop() method and
- clear() method
The remove() method
The remove() method removes the 1st occurrence of a given element from the ByteArray object as shown in the following example.
If you look closely you can see only the first “1” is removed and not the 2nd one.
The syntax for the remove() method is
But what if we wish to remove the 2nd “1” instead of the 1st? That is where the pop() method comes in!
The pop() method
The pop() method deletes the element at the specified index from the ByteArray object as shown in the following example.
As you can see, “1” which is the element at index-3 is removed and returned after calling the pop() method leaving just “1”, “2” and “3” in myArray.
The syntax to use with the pop() method is
If the index is not provided, the last element of the ByteArray is removed and returned. I leave it to you to experiment and see what happens when the pop() method is used without an index!
Advanced: What does “pop” mean?
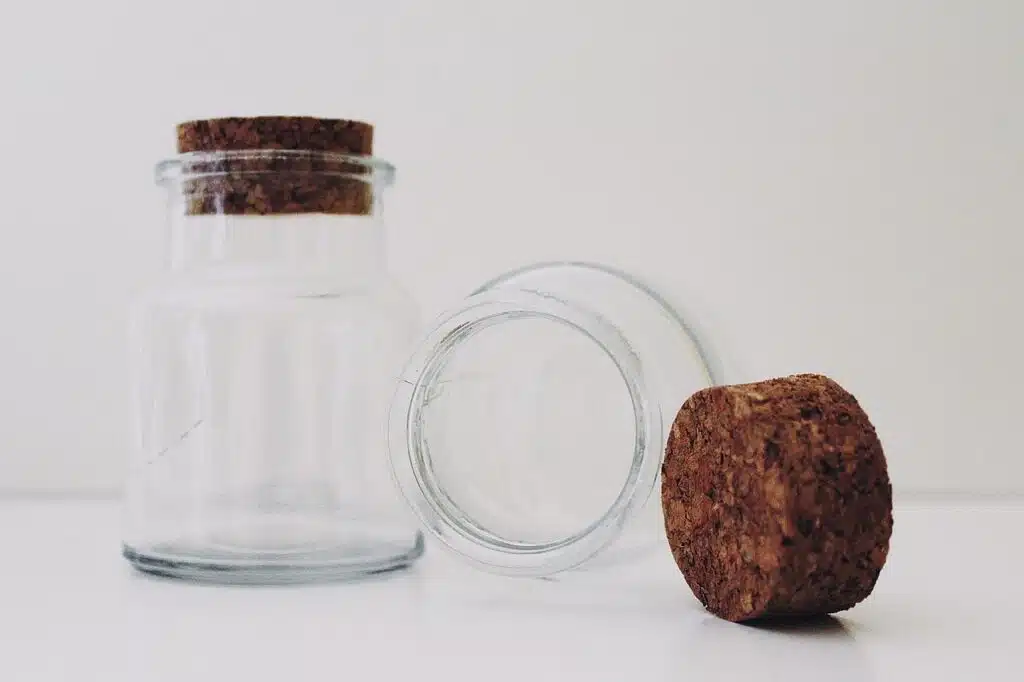
The word “pop” comes from the sound made by the cork as you open a bottle like the one in the pic above.
I assume you have played the game of “Jenga”. If you are sliding a block “in”, we call that “pushing” and whenever you take a block “out” we call that “popping”.
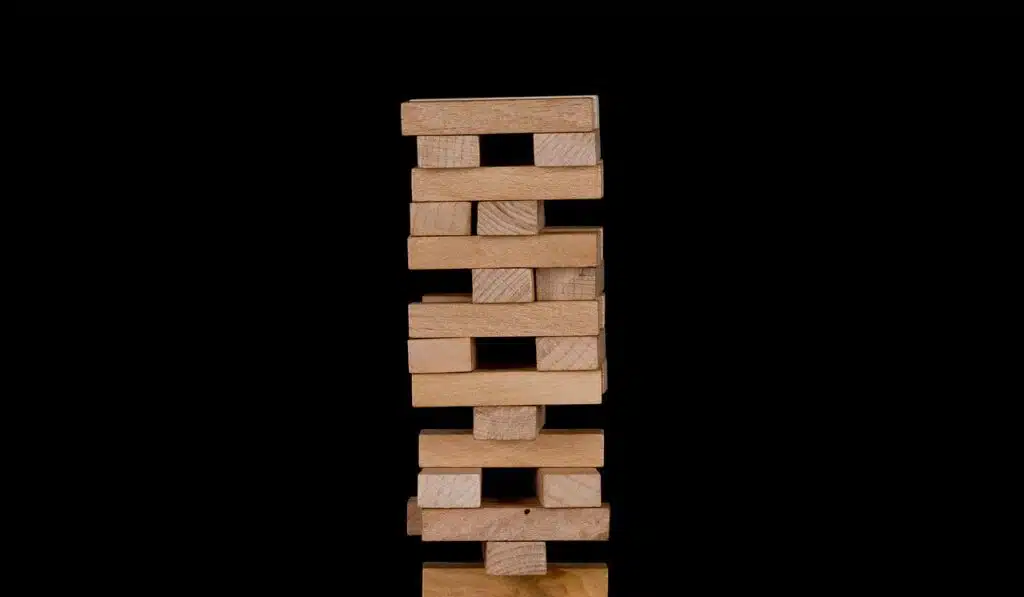
But how does that relate to python lists? Similar to the blocks of wood, the items in the list are stored blocks of memory locations. Hence we use the word “pop” to refer to a situation where we take items out of those memory locations!
The clear() method
The clear() method clears all the elements from the ByteArray object making it empty as shown in the following example.
As you can see, after the call to the clear() method, myArray() has no elements left!
The syntax to use with the clear() method is
Copying ByteArrays
If you are new to python, if you wish to create a copy, intuitively you will try something like this
The problem with this method is we are not creating a copy, instead, we are just making 2 names for the same object, so if we change one the other will also change as shown below.
So if we really wish to create a copy we need to use the copy() method which will return a copy of the given ByteArray as shown below.
As you can see we used the copy() method and now we can edit each copy independently.
The syntax to use with the copy() method is
Now we know how to create and manipulate ByteArrays, the next step would be to learn how to loop through elements of a ByteArray object.
Looping through a ByteArray Object
Since ByteArray is iterable, we can iterate over it using loops just like any other iterable like Lists as shown in the following example.
This will give the following output
Nice and simple!
Methods in a class are like spells in magic, if we learn some it will definitely make our life easier. Let us go ahead and learn some methods/spells that come with the ByteArray class!
Other Useful ByteArray Methods
The count() method.
The count() method returns the number of non-overlapping occurrences of a given subsection in ByteArray.
In simpler words, we can get the number of times a sequence of bytes occurs within a given ByteArray using this method.
As you can see, there are 2 ‘abc’ s in the string ‘abcd abc’ and hence we get the output as 2.
Make sure that the sequence we are searching for is also in the same ByteArray or Bytes format.
The syntax used with the count method is
The reverse() method
As the name suggests, the reverse() method is used to reverse the byte arrays in Python.
As you can see the order of the bytes has been reversed!
The syntax to use with the reverse() method is
The find() method
The find() method finds the first occurrence of the byte given to it. For example
As you can see, the element “3” is at index-2, and hence find() returns 2.
If the element is not present in the given ByteArray object, the find() method returns -1 as shown below
There is another method named index() which works almost the same as the find() method. The only difference between find() and index is that while find() returns -1 when the item is not found, the index will throw an exception instead as shown below.
Next, let us have a look at some ASCII-related methods.
The isdigit() method
This method is used to check whether the string contains only digits or not. For example
Here the string ‘123’ is a digit and hence we got True as a result.
The isalpha() method
This method is complementary to the isdigit() method and it checks if the string is only made up of alphabets as shown below.
As you can see, the string ‘python’ is only made up of alphabets and hence we got True as output.
So what will happen if a mixture of digits and alphabets are given to isalpha() method or to isdigit() method? I leave it to you to experiment and figure it out!
The istitle() method
This method checks whether a given text is in Title Case (each word starts with an uppercase letter, and the rest of the words are in lowercase) as shown below.
As you can see the 1st string returned True and the 2nd string returned False.
The lower() method
As the name implies, this method returns a ByteArray object with all the characters transformed to lowercase as shown below.
With that example, I will end this article!
I hope I was able to quench your thirst for knowledge about the ByteArray class and I hope you found this article useful!
Feel free to share it with your friends and colleagues.
Here are some more articles from our website that might interest you.
Thanks to Sarthak Jaryal for his contributions to writing this article.
Related Articles
Python Bytes: Everything You Need to Know!
Python: “[:]” Notation Explained with Examples!
Exceptions in Python: Everything You Need To Know!
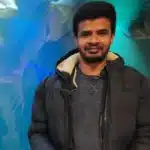
Python Enhancement Proposals
- Python »
- PEP Index »
PEP 3137 – Immutable Bytes and Mutable Buffer
Introduction, literal notations, pep 3118 buffer api, constructors, comparisons, str() and repr(), bytes and the str type, the basestring type.
After releasing Python 3.0a1 with a mutable bytes type, pressure mounted to add a way to represent immutable bytes. Gregory P. Smith proposed a patch that would allow making a bytes object temporarily immutable by requesting that the data be locked using the new buffer API from PEP 3118 . This did not seem the right approach to me.
Jeffrey Yasskin, with the help of Adam Hupp, then prepared a patch to make the bytes type immutable (by crudely removing all mutating APIs) and fix the fall-out in the test suite. This showed that there aren’t all that many places that depend on the mutability of bytes, with the exception of code that builds up a return value from small pieces.
Thinking through the consequences, and noticing that using the array module as an ersatz mutable bytes type is far from ideal, and recalling a proposal put forward earlier by Talin, I floated the suggestion to have both a mutable and an immutable bytes type. (This had been brought up before, but until seeing the evidence of Jeffrey’s patch I wasn’t open to the suggestion.)
Moreover, a possible implementation strategy became clear: use the old PyString implementation, stripped down to remove locale support and implicit conversions to/from Unicode, for the immutable bytes type, and keep the new PyBytes implementation as the mutable bytes type.
The ensuing discussion made it clear that the idea is welcome but needs to be specified more precisely. Hence this PEP.
One advantage of having an immutable bytes type is that code objects can use these. It also makes it possible to efficiently create hash tables using bytes for keys; this may be useful when parsing protocols like HTTP or SMTP which are based on bytes representing text.
Porting code that manipulates binary data (or encoded text) in Python 2.x will be easier using the new design than using the original 3.0 design with mutable bytes; simply replace str with bytes and change ‘…’ literals into b’…’ literals.
I propose the following type names at the Python level:
- bytes is an immutable array of bytes (PyString)
- bytearray is a mutable array of bytes (PyBytes)
- memoryview is a bytes view on another object (PyMemory)
The old type named buffer is so similar to the new type memoryview , introduce by PEP 3118 , that it is redundant. The rest of this PEP doesn’t discuss the functionality of memoryview ; it is just mentioned here to justify getting rid of the old buffer type. (An earlier version of this PEP proposed buffer as the new name for PyBytes; in the end this name was deemed to confusing given the many other uses of the word buffer.)
While eventually it makes sense to change the C API names, this PEP maintains the old C API names, which should be familiar to all.
Here’s a simple ASCII-art table summarizing the type names in various Python versions:
The b’…’ notation introduced in Python 3.0a1 returns an immutable bytes object, whatever variation is used. To create a mutable array of bytes, use bytearray(b’…’) or bytearray([…]). The latter form takes a list of integers in range(256).
Functionality
Both bytes and bytearray implement the PEP 3118 buffer API. The bytes type only implements read-only requests; the bytearray type allows writable and data-locked requests as well. The element data type is always ‘B’ (i.e. unsigned byte).
There are four forms of constructors, applicable to both bytes and bytearray:
- bytes(<bytes>) , bytes(<bytearray>) , bytearray(<bytes>) , bytearray(<bytearray>) : simple copying constructors, with the note that bytes(<bytes>) might return its (immutable) argument, but bytearray(<bytearray>) always makes a copy.
- bytes(<str>, <encoding>[, <errors>]) , bytearray(<str>, <encoding>[, <errors>]) : encode a text string. Note that the str.encode() method returns an immutable bytes object. The <encoding> argument is mandatory; <errors> is optional. <encoding> and <errors>, if given, must be str instances.
- bytes(<memory view>) , bytearray(<memory view>) : construct a bytes or bytearray object from anything that implements the PEP 3118 buffer API.
- bytes(<iterable of ints>) , bytearray(<iterable of ints>) : construct a bytes or bytearray object from a stream of integers in range(256).
- bytes(<int>) , bytearray(<int>) : construct a zero-initialized bytes or bytearray object of a given length.
The bytes and bytearray types are comparable with each other and orderable, so that e.g. b’abc’ == bytearray(b’abc’) < b’abd’.
Comparing either type to a str object for equality returns False regardless of the contents of either operand. Ordering comparisons with str raise TypeError. This is all conformant to the standard rules for comparison and ordering between objects of incompatible types.
( Note: in Python 3.0a1, comparing a bytes instance with a str instance would raise TypeError, on the premise that this would catch the occasional mistake quicker, especially in code ported from Python 2.x. However, a long discussion on the python-3000 list pointed out so many problems with this that it is clearly a bad idea, to be rolled back in 3.0a2 regardless of the fate of the rest of this PEP.)
Slicing a bytes object returns a bytes object. Slicing a bytearray object returns a bytearray object.
Slice assignment to a bytearray object accepts anything that implements the PEP 3118 buffer API, or an iterable of integers in range(256).
Indexing bytes and bytearray returns small ints (like the bytes type in 3.0a1, and like lists or array.array(‘B’)).
Assignment to an item of a bytearray object accepts an int in range(256). (To assign from a bytes sequence, use a slice assignment.)
The str() and repr() functions return the same thing for these objects. The repr() of a bytes object returns a b’…’ style literal. The repr() of a bytearray returns a string of the form “bytearray(b’…’)”.
The following operators are implemented by the bytes and bytearray types, except where mentioned:
- b1 + b2 : concatenation. With mixed bytes/bytearray operands, the return type is that of the first argument (this seems arbitrary until you consider how += works).
- b1 += b2 : mutates b1 if it is a bytearray object.
- b * n , n * b : repetition; n must be an integer.
- b *= n : mutates b if it is a bytearray object.
- b1 in b2 , b1 not in b2 : substring test; b1 can be any object implementing the PEP 3118 buffer API.
- i in b , i not in b : single-byte membership test; i must be an integer (if it is a length-1 bytes array, it is considered to be a substring test, with the same outcome).
- len(b) : the number of bytes.
- hash(b) : the hash value; only implemented by the bytes type.
Note that the % operator is not implemented. It does not appear worth the complexity.
The following methods are implemented by bytes as well as bytearray, with similar semantics. They accept anything that implements the PEP 3118 buffer API for bytes arguments, and return the same type as the object whose method is called (“self”):
This is exactly the set of methods present on the str type in Python 2.x, with the exclusion of .encode(). The signatures and semantics are the same too. However, whenever character classes like letter, whitespace, lower case are used, the ASCII definitions of these classes are used. (The Python 2.x str type uses the definitions from the current locale, settable through the locale module.) The .encode() method is left out because of the more strict definitions of encoding and decoding in Python 3000: encoding always takes a Unicode string and returns a bytes sequence, and decoding always takes a bytes sequence and returns a Unicode string.
In addition, both types implement the class method .fromhex() , which constructs an object from a string containing hexadecimal values (with or without spaces between the bytes).
The bytearray type implements these additional methods from the MutableSequence ABC (see PEP 3119 ):
.extend(), .insert(), .append(), .reverse(), .pop(), .remove().
Like the bytes type in Python 3.0a1, and unlike the relationship between str and unicode in Python 2.x, attempts to mix bytes (or bytearray) objects and str objects without specifying an encoding will raise a TypeError exception. (However, comparing bytes/bytearray and str objects for equality will simply return False; see the section on Comparisons above.)
Conversions between bytes or bytearray objects and str objects must always be explicit, using an encoding. There are two equivalent APIs: str(b, <encoding>[, <errors>]) is equivalent to b.decode(<encoding>[, <errors>]) , and bytes(s, <encoding>[, <errors>]) is equivalent to s.encode(<encoding>[, <errors>]) .
There is one exception: we can convert from bytes (or bytearray) to str without specifying an encoding by writing str(b) . This produces the same result as repr(b) . This exception is necessary because of the general promise that any object can be printed, and printing is just a special case of conversion to str. There is however no promise that printing a bytes object interprets the individual bytes as characters (unlike in Python 2.x).
The str type currently implements the PEP 3118 buffer API. While this is perhaps occasionally convenient, it is also potentially confusing, because the bytes accessed via the buffer API represent a platform-depending encoding: depending on the platform byte order and a compile-time configuration option, the encoding could be UTF-16-BE, UTF-16-LE, UTF-32-BE, or UTF-32-LE. Worse, a different implementation of the str type might completely change the bytes representation, e.g. to UTF-8, or even make it impossible to access the data as a contiguous array of bytes at all. Therefore, the PEP 3118 buffer API will be removed from the str type.
The basestring type will be removed from the language. Code that used to say isinstance(x, basestring) should be changed to use isinstance(x, str) instead.
Left as an exercise for the reader.
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-3137.rst
Last modified: 2023-09-09 17:39:29 GMT
Python Concepts/Bytes objects and Bytearrays
- 1 Objective
- 3.1 bytes object displayed
- 3.2 bytes object initialized
- 3.3 bytes object as iterable
- 4.1.1 Method int.to_bytes(length, ....)
- 4.2.1 from str containing international text
- 4.2.2 from str containing hexadecimal digits
- 4.2.3.1 Examples of characters encoded with 'utf-8'
- 4.2.3.2 A disadvantage of 'utf-8'
- 5.1.1 Class method int.from_bytes(bytes, ....)
- 5.2.1 to str containing international text
- 5.2.2 to str containing hexadecimal digits
- 6.1 Creating and using a translation table:
- 7 bytes objects and disk files
- 8.1 bytearray displayed
- 8.2 bytearray initialized
- 8.3 bytearray as iterable
- 9.1.1 Method int.to_bytes(length, ....)
- 9.2.1 from str containing international text
- 9.2.2 from str containing hexadecimal digits
- 10.1.1 Class method int.from_bytes(bytes, ....)
- 10.2.1 to str containing international text
- 10.2.2 to str containing hexadecimal digits
- 11.1 bytearray.find(sub[, start[, end]])
- 11.2 bytearray.join(iterable)
- 11.3 bytearray.partition(sep)
- 11.4 bytearray.center(width[, fillbyte])
- 11.5 bytearray.split(sep=None, maxsplit=-1)
- 11.6 bytearray.strip([chars])
- 11.7 bytearray.isspace()
- 12 Assignments
- 13 Further Reading or Review
- 14 References
Objective [ edit | edit source ]
Lesson [ edit | edit source ], bytes objects [ edit | edit source ], conversion to bytes object [ edit | edit source ].
Encoding standard 'utf-8' is a good choice for default encoding because:
- 2, 3 or 4 bytes are used only if necessary,
- it doesn't depend on byte ordering, big or little, and
- arbitrary binary data is not likely to conform to the above specification.
Examples of characters encoded with 'utf-8' [ edit | edit source ]
The following code examines chr(0x10006), encoded in 4 bytes:
Theoretically 21 payload bits can contain '\U001FFFFF' but the standard stops at '\U0010FFFF':
A disadvantage of 'utf-8' [ edit | edit source ]
A bytes object produced with encoding 'utf-8' can contain the null byte b'\x00'. This could cause a problem if you are sending a stream of bytes through a filter that interprets b'\x00' as end of data. Standard 'utf-8' never produces b'\xFF'. If your bytes object must not contain b'\x00' after encoding, you could convert the null byte to b'\xFF', then convert b'\xFF' to b'\x00' before decoding:
Conversion from bytes object [ edit | edit source ]
Operations with methods on bytes objects [ edit | edit source ], bytes objects and disk files [ edit | edit source ], bytearrays [ edit | edit source ], conversion to bytearray [ edit | edit source ], conversion from bytearray [ edit | edit source ], operations with methods on bytearrays [ edit | edit source ], assignments [ edit | edit source ], further reading or review [ edit | edit source ], references [ edit | edit source ].
Navigation menu
Python bytes vs bytearray
What’s the difference between bytes() and bytearray().
The difference between bytes() and bytearray() is that bytes() returns an immutable and bytearray() returns a mutable object. So you can modify a bytearray but not bytes type.
Here’s a minimal example that nicely demonstrates the difference of the two functions:
You create two variables a and b . The former is a bytes and the latter a bytearray —both encoding the same data.
However, if you try to modify the bytes object, Python raises a TypeError: 'bytes' object does not support item assignment .
You resolve the error by using a bytearray object instead — if you modify it using item assignment such as b[0] = 1 , the error disappears.
The reason is that unlike bytes , the bytearray type is mutable.
🌍 Recommended Tutorial : Python Mutable vs Immutable Objects
Let’s recap both functions quickly. Afterward, we will do a performance comparison in case you wondered which is faster!
Python bytes()

Python’s built-in bytes(source) function creates an immutable bytes object initialized as defined in the function argument source .
A bytes object is like a string but it uses only byte characters consisting of a sequence of 8-bit integers in the range 0<=x<256 .
The returned byte object is immutable—you cannot change it after creation. If you plan to change the contents, use the bytearray() method to create a mutable bytearray object.
🌍 Recommended Tutorial: Python bytes() Built-in Function
Python bytearray()

Python’s built-in bytearray() function takes an iterable such as a list of integers between 0 and 256, converts them to bytes between 00000000 and 11111111 , and returns a new array of bytes as a bytearray class.
🌍 Recommended Tutorial: Python bytearray() Built-in Function
Performance bytes() vs bytearray()
🛑 Attention : My initial performance measurement is flawed. Keep reading to see ASL97’s comments and corrected performance evaluation!
Creating a large bytes object (e.g., 100 million bytes) using bytes() is faster than creating a bytearray with bytearray() because the mutability of the latter comes at a performance cost.
In our experiment creating objects of 100 million bytes, we have seen a performance speedup of 3x using bytes() compared to bytearray() .
Here’s the code of the simple experiment setup:
I ran the experiment multiple times on my Win Intel Core i7 CPU with 8GB of RAM and obtained the following output:
So the difference is roughly 3-4x in performance: bytes() is 300-400% faster than bytearray() .
However, don’t let this tiny performance edge fool you! 👇
Edit : User ASL97 submitted another variation of this test where the opposite happened:
ASL97 correctly hinted that “To avoid run to run variation, it is advisable to increase n by a factor of 10, bytearray is faster when done correctly even with the initial overhead of converting bytes to bytearray, b'x' * n vs bytearray(b'x') * n .”
Also, I would consider ASL97’s performance test to be superior due to his feedback:
The provided test on that page is not measuring what it seem to be suggesting . For bytes, it is measuring the time to create the byte object and converting bytes to bytes which is basically a do nothing. For bytearray, it is measuring the time to create the same byte object and converting bytes to bytearray
Thanks for the feedback! ♥️
In most cases, it’s best to optimize for readability and suitability for the problem:
- Do you need to change the data structure holding bytes after creation? Use the mutable bytearray() .
- Do you create the data structure once and then only read its information? Use the immutable bytes() .
Don’t do premature optimization!
👉 Recommended Tutorial : Premature Optimization is the Root of All Evil
Where to Go From Here?
Thanks for reading the whole tutorial—I hope you got some valuable pieces of knowledge out of it.
If you want to keep improving your coding skills and become a Python pro, check out our free email academy . We have cheat sheets too!
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He’s the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. He’s a computer science enthusiast, freelancer , and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
TheDeveloperBlog.com
Home | Contact Us
C-Sharp | Java | Python | Swift | GO | WPF | Ruby | Scala | F# | JavaScript | SQL | PHP | Angular | HTML
- Python bytes, bytearray Examples (memoryview)
For: We use the for-loop to iterate over the bytearray's elements. This is the same as how we use a list.
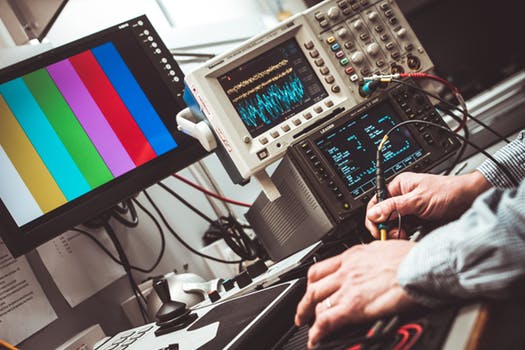
Related Links:
- Python global and nonlocal
- Python not: If Not True
- Python Convert Decimal Binary Octal and Hexadecimal
- Python Tkinter Scale
- Python Tkinter Scrollbar
- Python Tkinter Text
- Python History
- Python Number: random, float and divmod
- Python Tkinter Toplevel
- Python Tkinter Spinbox
- Python Tkinter PanedWindow
- Python Tkinter LabelFrame
- Python Tkinter MessageBox
- Python Website Blocker
- Python Console Programs: Input and Print
- Python Display Calendar
- Python Check Number Odd or Even
- Python readline Example: Read Next Line
- Python Anagram Find Method
- Python Any: Any Versus All, List Performance
- Python Filename With Date Example (date.today)
- Python Find String: index and count
- Python filter (Lambda Removes From List or Range)
- Python ASCII Value of Character
- Python Sum Example
- Python make simple Calculator
- Python Add Two Matrices
- Python Multiply Two Matrices
- Python SyntaxError (invalid syntax)
- Python Transpose Matrix
- Python Remove Punctuation from String
- Python Dictionary items() method with Examples
- Python Dictionary keys() method with Examples
- Python Textwrap Wrap Example
- Python Dictionary popitem() method with Examples
- Python Dictionary pop() method with Examples
- Python HTML: HTMLParser, Read Markup
- Python Tkinter Tutorial
- Python Array Examples
- Python ord, chr Built Ins
- Python Dictionary setdefault() method with Examples
- Python Dictionary update() method with Examples
- Python Dictionary values() method with Examples
- Python complex() function with Examples
- Python delattr() function with Examples
- Python dir() function with Examples
- Python divmod() function with Examples
- Python Loops
- Python for loop
- Python while loop
- Python enumerate() function with Examples
- Python break
- Python continue
- Python dict() function with Examples
- Python pass
- Python Strings
- Python Lists
- Python Tuples
- Python Sets
- Python Built-in Functions
- Python filter() function with Examples
- Python dict Keyword (Copy Dictionary)
- Python Dictionary Order Benchmark
- Python Dictionary String Key Performance
- Python 2D Array: Create 2D Array of Integers
- Python Divmod Examples, Modulo Operator
- bin() in Python | Python bin() Function with Examples
- Python Oops Concept
- Python Object Classes
- Python Constructors
- Python hash() function with Examples
- Python Pandas | Python Pandas Tutorial
- Python Class Examples: Init and Self
- Python help() function with Examples
- Python IndentationError (unexpected indent)
- Python Index and Count (Search List)
- Python min() function with Examples
- Python classmethod and staticmethod Use
- Python set() function with Examples
- Python hex() function with Examples
- Python id() function with Examples
- Python sorted() function with Examples
- Python next() function with Examples
- Python Compound Interest
- Python List insert() method with Examples
- Python Datetime Methods: Date, Timedelta
- Python setattr() function with Examples
- Python 2D List Examples
- Python Pandas Data operations
- Python Def Methods and Arguments (callable)
- Python slice() function with Examples
- Python Remove HTML Tags
- Python input() function with Examples
- Python enumerate (For Index, Element)
- Python Display the multiplication Table
- Python int() function with Examples
- Python Error: Try, Except and Raise
- Python isinstance() function with Examples
- Python oct() function with Examples
- Python startswith, endswith Examples
- Python List append() method with Examples
- Python NumPy Examples (array, random, arange)
- Python Replace Example
- Python List clear() method with Examples
- Python List copy() method with Examples
- Python Lower Dictionary: String Performance
- Python Lower and Upper: Capitalize String
- Python Dictionary Examples
- Python map Examples
- Python Len (String Length)
- Python Padding Examples: ljust, rjust
- Python Type: setattr and getattr Examples
- Python String List Examples
- Python String
- Python Remove Duplicates From List
- Python If Examples: Elif, Else
- Python Programs | Python Programming Examples
- Python List count() method with Examples
- Python List extend() method with Examples
- Python List index() method with Examples
- Python List pop() method with Examples
- Python Palindrome Method: Detect Words, Sentences
- Python Path: os.path Examples
- Python List remove() method with Examples
- Python List reverse() method with Examples
- Top 50+ Python Interview Questions (2021)
- Python List sort() method with Examples
- Python sort word in Alphabetic Order
- abs() in Python | Python abs() Function with Examples
- Python String | encode() method with Examples
- all() in Python | Python all() Function with Examples
- any() in Python | Python any() Function with Examples
- Python Built In Functions
- ascii() in Python | Python ascii() Function with Examples
- bool() in Python | Python bool() Function with Examples
- bytearray() in Python | Python bytearray() Function with Examples
- Python Caesar Cipher
- bytes() in Python | Python bytes() Function with Examples
- Python Sum of Natural Numbers
- callable() in Python | Python callable() Function with Examples
- Python Set add() method with Examples
- Python Set discard() method with Examples
- Python Set pop() method with Examples
- Python math.floor, import math Examples
- Python Return Keyword (Return Multiple Values)
- Python while Loop Examples
- Python Math Examples
- Python Reverse String
- Python max, min Examples
- Python pass Statement
- Python Set remove() method with Examples
- Python Dictionary
- Python Functions
- Python String | capitalize() method with Examples
- Python String | casefold() method with Examples
- Python re.sub, subn Methods
- Python subprocess Examples: subprocess.run
- Python Tkinter Checkbutton
- Python Tkinter Entry
- Python String | center() method with Examples
- Python Substring Examples
- Python pow Example, Power Operator
- Python Lambda
- Python Files I/O
- Python Modules
- Python String | count() method with Examples
- Python String | endswith() method with Examples
- Python String | expandtabs() method with Examples
- Python Prime Number Method
- Python String | find() method with Examples
- Python String | format() method with Examples
- Python String | index() method with Examples
- Python String | isalnum() method with Examples
- Python String | isalpha() method with Examples
- Python String | isdecimal() method with Examples
- Python Pandas Sorting
- Python String | isdigit() method with Examples
- Python Convert Types
- Python String | isidentifier() method with Examples
- Python Pandas Add column to DataFrame columns
- Python String | islower() method with Examples
- Python Pandas Reading Files
- Python Right String Part
- Python IOError Fix, os.path.exists
- Python Punctuation and Whitespace (string.punctuation)
- Python isalnum: String Is Alphanumeric
- Python Pandas Series
- Python Pandas DataFrame
- Python Recursion Example
- Python ROT13 Method
- Python StringIO Examples and Benchmark
- Python Import Syntax Examples: Modules, NameError
- Python in Keyword
- Python iter Example: next
- Python Round Up and Down (Math Round)
- Python List Comprehension
- Python Collection Module
- Python Math Module
- Python OS Module
- Python Random Module
- Python Statistics Module
- Python String Equals: casefold
- Python Sys Module
- Top 10 Python IDEs | Python IDEs
- Python Arrays
- Python Magic Method
- Python Stack and Queue
- Python MySQL Environment Setup
- Python MySQL Database Connection
- Python MySQL Creating New Database
- Python MySQL Creating Tables
- Python Word Count Method (re.findall)
- Python String Literal: F, R Strings
- Python MySQL Update Operation
- Python MySQL Join Operation
- Python Armstrong Number
- Learn Python Tutorial
- Python Factorial Number using Recursion
- Python Features
- Python Comments
- Python if else
- Python Translate and Maketrans Examples
- Python Website Blocker | Building Python Script
- Python Itertools Module: Cycle and Repeat
- Python Operators
- Python Int Example
- Python join Example: Combine Strings From List
- Python Read CSV File
- Python Write CSV File
- Python Read Excel File
- Python Write Excel File
- Python json: Import JSON, load and dumps
- Python Lambda Expressions
- Python Print the Fibonacci sequence
- Python format Example (Format Literal)
- Python Namedtuple Example
- Python SciPy Tutorial
- Python Applications
- Python KeyError Fix: Use Dictionary get
- Python Resize List: Slice and Append
- Python String | translate() method with Examples
- Python Copy List (Slice Entire List)
- Python None: TypeError, NoneType Has No Length
- Python MySQL Performing Transactions
- Python String | isnumeric() method with Examples
- Python MongoDB Example
- Python String | isprintable() method with Examples
- Python Tkinter Canvas
- Python String | isspace() method with Examples
- Python Tkinter Frame
- Python Tkinter Label
- Python Tkinter Listbox
- Python String | istitle() method with Examples
- Python Website Blocker | Script Deployment on Linux
- Python Website Blocker | Script Deployment on Windows
- Python String | isupper() method with Examples
- Python String split() method with Examples
- Python Slice Examples: Start, Stop and Step
- Python String | join() method with Examples
- Python String | ljust() method with Examples
- Python Sort by File Size
- Python Arithmetic Operations
- Python String | lower() method with Examples
- Python Exception Handling | Python try except
- Python Date
- Python Regex | Regular Expression
- Python Sending Email using SMTP
- Python Command Line Arguments
- Python List Comprehension Examples
- Python Assert Keyword
- Python Set Examples
- Python Fibonacci Sequence
- Python Maze Pathfinding Example
- Python Memoize: Dictionary, functools.lru_cache
- Python Timeit, Repeat Examples
- Python Strip Examples
- Python asyncio Example: yield from asyncio.sleep
- Python String Between, Before and After Methods
- Python bool Use (Returns True or False)
- Python Counter Example
- Python frozenset: Immutable Sets
- Python Generator Examples: Yield, Expressions
- Python CSV: csv.reader and Sniffer
- Python globals, locals, vars and dir
- Python abs: Absolute Value
- Python gzip: Compression Examples
- Python Function Display Calendar
- Python Display Fibonacci Sequence Recursion
- Python String | lstrip() method with Examples
- Python del Operator (Remove at Index or Key)
- Python String | partition() method with Examples
- Python String | replace() method with Examples
- Python Zip Examples: Zip Objects
- Python String | rfind() method with Examples
- Python String | rindex() method with Examples
- Python String rjust() method with Examples
- Python String rpartition() method with Examples
- Python String rsplit() method with Examples
- Python Area Of Triangle
- Python Quadratic Equation
- Python swap two Variables
- Python Generate Random Number
- Python Convert Kilometers to Miles
- Python Convert Celsius to Fahrenheit
- Python Check Number Positive Negative or Zero
- Python Check Leap Year
- Python Check Prime Number
- Top 40 Python Pandas Interview Questions (2021)
- Python Check Armstrong Number
- Python SQLite Example
- Python Tkinter Button
- Python Find LCM
- Python Find HCF
- Python Tuple Examples
- Python String | rstrip() method with Examples
- Python String splitlines() method with Examples
- Python String | startswith() method with Examples
- Python String | swapcase() method with Examples
- Python Truncate String
- Python String | upper() method with Examples
- Python for: Loop Over String Characters
- Python String | zfill() method with Examples
- Python Sort Examples: Sorted List, Dictionary
- Python XML: Expat, StartElementHandler
- Python Urllib Usage: Urlopen, UrlParse
- Python File Handling (with open, write)
- Python Example
- Python variables
- Python Random Numbers: randint, random.choice
- Python assert, O Option
- Python Data Types
- Python keywords
- Python literals
- Python MySQL Insert Operation
- Python MySQL Read Operation
- Python ascii Example
- Python ASCII Table Generator: chr
- Python Range: For Loop, Create List From Range
- Python re.match Performance
- Python re.match, search Examples
- Python Tkinter Menubutton
- Python Tkinter Menu
- Python Tkinter Message
- Python Tkinter Radiobutton
- Python List Examples
- Python Split String Examples
Related Links
The QByteArray class provides an array of bytes. More …
def __init__()
def __getitem__()
def __len__()
def __mgetitem__()
def __msetitem__()
def __reduce__()
def __repr__()
def __setitem__()
def __str__()
def append()
def assign()
def capacity()
def cbegin()
def chopped()
def clear()
def compare()
def contains()
def count()
def endsWith()
def erase()
def first()
def front()
def indexOf()
def insert()
def isEmpty()
def isLower()
def isNull()
def isSharedWith()
def isUpper()
def isValidUtf8()
def lastIndexOf()
def leftJustified()
def length()
def __ne__()
def __add__()
def __iadd__()
def __lt__()
def __le__()
def __eq__()
def __gt__()
def __ge__()
def operator[]()
def percentDecoded()
def prepend()
def push_back()
def push_front()
def remove()
def removeAt()
def removeFirst()
def removeLast()
def repeated()
def replace()
def reserve()
def resize()
def right()
def rightJustified()
def setNum()
def setRawData()
def shrink_to_fit()
def simplified()
def sliced()
def split()
def squeeze()
def startsWith()
def toBase64()
def toDouble()
def toFloat()
def toHex()
def toInt()
def toLong()
def toLongLong()
def toLower()
def toPercentEncoding()
def toShort()
def toStdString()
def toUInt()
def toULong()
def toULongLong()
def toUShort()
def toUpper()
def trimmed()
def truncate()
Static functions #
def fromBase64()
def fromBase64Encoding()
def fromHex()
def fromPercentEncoding()
def fromRawData()
def fromStdString()
def number()
This documentation may contain snippets that were automatically translated from C++ to Python. We always welcome contributions to the snippet translation. If you see an issue with the translation, you can also let us know by creating a ticket on https:/bugreports.qt.io/projects/PYSIDE
Detailed Description #
This section contains snippets that were automatically translated from C++ to Python and may contain errors.
QByteArray can be used to store both raw bytes (including ‘\0’s) and traditional 8-bit ‘\0’-terminated strings. Using QByteArray is much more convenient than using const char * . Behind the scenes, it always ensures that the data is followed by a ‘\0’ terminator, and uses implicit sharing (copy-on-write) to reduce memory usage and avoid needless copying of data.
In addition to QByteArray , Qt also provides the QString class to store string data. For most purposes, QString is the class you want to use. It understands its content as Unicode text (encoded using UTF-16) where QByteArray aims to avoid assumptions about the encoding or semantics of the bytes it stores (aside from a few legacy cases where it uses ASCII). Furthermore, QString is used throughout in the Qt API. The two main cases where QByteArray is appropriate are when you need to store raw binary data, and when memory conservation is critical (e.g., with Qt for Embedded Linux).
One way to initialize a QByteArray is simply to pass a const char * to its constructor. For example, the following code creates a byte array of size 5 containing the data “Hello”:
ba = QByteArray ( "Hello" )
Although the size() is 5, the byte array also maintains an extra ‘\0’ byte at the end so that if a function is used that asks for a pointer to the underlying data (e.g. a call to data() ), the data pointed to is guaranteed to be ‘\0’-terminated.
QByteArray makes a deep copy of the const char * data, so you can modify it later without experiencing side effects. (If, for example for performance reasons, you don’t want to take a deep copy of the data, use fromRawData() instead.)
Another approach is to set the size of the array using resize() and to initialize the data byte by byte. QByteArray uses 0-based indexes, just like C++ arrays. To access the byte at a particular index position, you can use operator[](). On non-const byte arrays, operator[]() returns a reference to a byte that can be used on the left side of an assignment. For example:
ba = QByteArray () ba . resize ( 5 ) ba [ 0 ] = 0x3c ba [ 1 ] = 0xb8 ba [ 2 ] = 0x64 ba [ 3 ] = 0x18 ba [ 4 ] = 0xca
For read-only access, an alternative syntax is to use at() :
for i in range ( 0 , ba . size ()): if ba . at ( i ) >= 'a' and ba . at ( i ) <= 'f' : print ( "Found character in range [a-f]" )
at() can be faster than operator[](), because it never causes a deep copy to occur.
To extract many bytes at a time, use first() , last() , or sliced() .
A QByteArray can embed ‘\0’ bytes. The size() function always returns the size of the whole array, including embedded ‘\0’ bytes, but excluding the terminating ‘\0’ added by QByteArray . For example:
ba1 = QByteArray ( "ca \0 r \0 t" ) ba1 . size () # Returns 2. ba1 . constData () # Returns "ca" with terminating \0. ba2 = QByteArray ( "ca \0 r \0 t" , 3 ) ba2 . size () # Returns 3. ba2 . constData () # Returns "ca\0" with terminating \0. ba3 = QByteArray ( "ca \0 r \0 t" , 4 ) ba3 . size () # Returns 4. ba3 . constData () # Returns "ca\0r" with terminating \0. cart = { 'c' , 'a' , ' \0 ' , 'r' , ' \0 ' , 't' } ba4 = QByteArray ( QByteArray . fromRawData ( cart , 6 )) ba4 . size () # Returns 6. ba4 . constData () # Returns "ca\0r\0t" without terminating \0.
If you want to obtain the length of the data up to and excluding the first ‘\0’ byte, call qstrlen() on the byte array.
After a call to resize() , newly allocated bytes have undefined values. To set all the bytes to a particular value, call fill() .
To obtain a pointer to the actual bytes, call data() or constData() . These functions return a pointer to the beginning of the data. The pointer is guaranteed to remain valid until a non-const function is called on the QByteArray . It is also guaranteed that the data ends with a ‘\0’ byte unless the QByteArray was created from raw data . This ‘\0’ byte is automatically provided by QByteArray and is not counted in size() .
QByteArray provides the following basic functions for modifying the byte data: append() , prepend() , insert() , replace() , and remove() . For example:
x = QByteArray ( "and" ) x . prepend ( "rock " ) # x == "rock and" x . append ( " roll" ) # x == "rock and roll" x . replace ( 5 , 3 , "" ) # x == "rock roll"
In the above example the replace() function’s first two arguments are the position from which to start replacing and the number of bytes that should be replaced.
When data-modifying functions increase the size of the array, they may lead to reallocation of memory for the QByteArray object. When this happens, QByteArray expands by more than it immediately needs so as to have space for further expansion without reallocation until the size of the array has greatly increased.
The insert() , remove() and, when replacing a sub-array with one of different size, replace() functions can be slow ( linear time ) for large arrays, because they require moving many bytes in the array by at least one position in memory.
If you are building a QByteArray gradually and know in advance approximately how many bytes the QByteArray will contain, you can call reserve() , asking QByteArray to preallocate a certain amount of memory. You can also call capacity() to find out how much memory the QByteArray actually has allocated.
Note that using non-const operators and functions can cause QByteArray to do a deep copy of the data, due to implicit sharing .
QByteArray provides STL-style iterators ( const_iterator and iterator ). In practice, iterators are handy when working with generic algorithms provided by the C++ standard library.
Iterators and references to individual QByteArray elements are subject to stability issues. They are often invalidated when a QByteArray -modifying operation (e.g. insert() or remove() ) is called. When stability and iterator-like functionality is required, you should use indexes instead of iterators as they are not tied to QByteArray ‘s internal state and thus do not get invalidated.
Iterators over a QByteArray , and references to individual bytes within one, cannot be relied on to remain valid when any non-const method of the QByteArray is called. Accessing such an iterator or reference after the call to a non-const method leads to undefined behavior. When stability for iterator-like functionality is required, you should use indexes instead of iterators as they are not tied to QByteArray ‘s internal state and thus do not get invalidated.
If you want to find all occurrences of a particular byte or sequence of bytes in a QByteArray , use indexOf() or lastIndexOf() . The former searches forward starting from a given index position, the latter searches backward. Both return the index position of the byte sequence if they find it; otherwise, they return -1. For example, here’s a typical loop that finds all occurrences of a particular string:
ba = QByteArray ( "We must be , very " ) j = 0 while ( j = ba . indexOf ( "<b>" , j )) != - 1 : print ( "Found <b> tag at index position " , j ) j = j + 1
If you simply want to check whether a QByteArray contains a particular byte sequence, use contains() . If you want to find out how many times a particular byte sequence occurs in the byte array, use count(). If you want to replace all occurrences of a particular value with another, use one of the two-parameter replace() overloads.
QByteArray s can be compared using overloaded operators such as operator<(), operator<=(), operator==(), operator>=(), and so on. The comparison is based exclusively on the numeric values of the bytes and is very fast, but is not what a human would expect. localeAwareCompare() is a better choice for sorting user-interface strings.
For historical reasons, QByteArray distinguishes between a null byte array and an empty byte array. A null byte array is a byte array that is initialized using QByteArray ‘s default constructor or by passing (const char *)0 to the constructor. An empty byte array is any byte array with size 0. A null byte array is always empty, but an empty byte array isn’t necessarily null:
QByteArray () . isNull () # returns true QByteArray () . isEmpty () # returns true QByteArray ( "" ) . isNull () # returns false QByteArray ( "" ) . isEmpty () # returns true QByteArray ( "abc" ) . isNull () # returns false QByteArray ( "abc" ) . isEmpty () # returns false
All functions except isNull() treat null byte arrays the same as empty byte arrays. For example, data() returns a valid pointer ( not nullptr) to a ‘\0’ byte for a null byte array and QByteArray() compares equal to QByteArray (“”). We recommend that you always use isEmpty() and avoid isNull() .
Maximum size and out-of-memory conditions #
The maximum size of QByteArray depends on the architecture. Most 64-bit systems can allocate more than 2 GB of memory, with a typical limit of 2^63 bytes. The actual value also depends on the overhead required for managing the data block. As a result, you can expect the maximum size of 2 GB minus overhead on 32-bit platforms, and 2^63 bytes minus overhead on 64-bit platforms. The number of elements that can be stored in a QByteArray is this maximum size.
When memory allocation fails, QByteArray throws a std::bad_alloc exception if the application is being compiled with exception support. Out of memory conditions in Qt containers are the only case where Qt will throw exceptions. If exceptions are disabled, then running out of memory is undefined behavior.
Note that the operating system may impose further limits on applications holding a lot of allocated memory, especially large, contiguous blocks. Such considerations, the configuration of such behavior or any mitigation are outside the scope of the QByteArray API.
C locale and ASCII functions #
QByteArray generally handles data as bytes, without presuming any semantics; where it does presume semantics, it uses the C locale and ASCII encoding. Standard Unicode encodings are supported by QString , other encodings may be supported using QStringEncoder and QStringDecoder to convert to Unicode. For locale-specific interpretation of text, use QLocale or QString .
C Strings #
Traditional C strings, also known as ‘\0’-terminated strings, are sequences of bytes, specified by a start-point and implicitly including each byte up to, but not including, the first ‘\0’ byte thereafter. Methods that accept such a pointer, without a length, will interpret it as this sequence of bytes. Such a sequence, by construction, cannot contain a ‘\0’ byte.
Other overloads accept a start-pointer and a byte-count; these use the given number of bytes, following the start address, regardless of whether any of them happen to be ‘\0’ bytes. In some cases, where there is no overload taking only a pointer, passing a length of -1 will cause the method to use the offset of the first ‘\0’ byte after the pointer as the length; a length of -1 should only be passed if the method explicitly says it does this (in which case it is typically a default argument).
Spacing Characters #
A frequent requirement is to remove spacing characters from a byte array ( '\n' , '\t' , ' ' , etc.). If you want to remove spacing from both ends of a QByteArray , use trimmed() . If you want to also replace each run of spacing characters with a single space character within the byte array, use simplified() . Only ASCII spacing characters are recognized for these purposes.
Number-String Conversions #
Functions that perform conversions between numeric data types and string representations are performed in the C locale, regardless of the user’s locale settings. Use QLocale to perform locale-aware conversions between numbers and strings.
Character Case #
In QByteArray , the notion of uppercase and lowercase and of case-independent comparison is limited to ASCII. Non-ASCII characters are treated as caseless, since their case depends on encoding. This affects functions that support a case insensitive option or that change the case of their arguments. Functions that this affects include compare() , isLower() , isUpper() , toLower() and toUpper() .
This issue does not apply to QString s since they represent characters using Unicode.
See also QByteArrayView QString QBitArray
(inherits enum.Flag ) This enum contains the options available for encoding and decoding Base64. Base64 is defined by RFC 4648, with the following options:
Constant Description QByteArray.Base64Encoding (default) The regular Base64 alphabet, called simply “base64” QByteArray.Base64UrlEncoding An alternate alphabet, called “base64url”, which replaces two characters in the alphabet to be more friendly to URLs. QByteArray.KeepTrailingEquals (default) Keeps the trailing padding equal signs at the end of the encoded data, so the data is always a size multiple of four. QByteArray.OmitTrailingEquals Omits adding the padding equal signs at the end of the encoded data. QByteArray.IgnoreBase64DecodingErrors When decoding Base64-encoded data, ignores errors in the input; invalid characters are simply skipped. This enum value has been added in Qt 5.15. QByteArray.AbortOnBase64DecodingErrors When decoding Base64-encoded data, stops at the first decoding error. This enum value has been added in Qt 5.15.
fromBase64Encoding() and fromBase64() ignore the KeepTrailingEquals and OmitTrailingEquals options. If the IgnoreBase64DecodingErrors option is specified, they will not flag errors in case trailing equal signs are missing or if there are too many of them. If instead the AbortOnBase64DecodingErrors is specified, then the input must either have no padding or have the correct amount of equal signs.
arg__1 – PyByteArray
Constructs an empty byte array.
See also isEmpty()
Constructs a byte array of size size with every byte set to ch .
See also fill()
arg__1 – str
Constructs a byte array containing the first size bytes of array data .
If data is 0, a null byte array is constructed.
If size is negative, data is assumed to point to a ‘\0’-terminated string and its length is determined dynamically.
QByteArray makes a deep copy of the string data.
See also fromRawData()
arg__1 – PyBytes
arg__1 – QByteArray
Constructs a copy of other .
This operation takes constant time , because QByteArray is implicitly shared . This makes returning a QByteArray from a function very fast. If a shared instance is modified, it will be copied (copy-on-write), taking linear time .
See also operator=()
This is an overloaded function.
Appends the first len bytes starting at str to this byte array and returns a reference to this byte array. The bytes appended may include ‘\0’ bytes.
If len is negative, str will be assumed to be a ‘\0’-terminated string and the length to be copied will be determined automatically using qstrlen() .
If len is zero or str is null, nothing is appended to the byte array. Ensure that len is not longer than str .
count – int
Appends count copies of byte ch to this byte array and returns a reference to this byte array.
If count is negative or zero nothing is appended to the byte array.
a – QByteArray
Appends the byte array ba onto the end of this byte array.
x = QByteArray ( "free" ) y = QByteArray ( "dom" ) x . append ( y ) # x == "freedom"
This is the same as insert( size() , ba ).
Note: QByteArray is an implicitly shared class. Consequently, if you append to an empty byte array, then the byte array will just share the data held in ba . In this case, no copying of data is done, taking constant time . If a shared instance is modified, it will be copied (copy-on-write), taking linear time .
If the byte array being appended to is not empty, a deep copy of the data is performed, taking linear time .
The append() function is typically very fast ( constant time ), because QByteArray preallocates extra space at the end of the data, so it can grow without reallocating the entire array each time.
See also operator+=() prepend() insert()
Appends the byte ch to this byte array.
a – QByteArrayView
Appends data to this byte array.
v – QByteArrayView
Replaces the contents of this byte array with a copy of v and returns a reference to this byte array.
The size of this byte array will be equal to the size of v .
This function only allocates memory if the size of v exceeds the capacity of this byte array or this byte array is shared.
Replaces the contents of this byte array with n copies of c and returns a reference to this byte array.
The size of this byte array will be equal to n , which has to be non-negative.
This function will only allocate memory if n exceeds the capacity of this byte array or this byte array is shared.
Returns the byte at index position i in the byte array.
i must be a valid index position in the byte array (i.e., 0 <= i < size() ).
See also operator[]()
Returns the last byte in the byte array. Same as at(size() - 1) .
This function is provided for STL compatibility.
Calling this function on an empty byte array constitutes undefined behavior.
front() at() operator[]()
Returns the maximum number of bytes that can be stored in the byte array without forcing a reallocation.
The sole purpose of this function is to provide a means of fine tuning QByteArray ‘s memory usage. In general, you will rarely ever need to call this function. If you want to know how many bytes are in the byte array, call size() .
a statically allocated byte array will report a capacity of 0, even if it’s not empty.
The free space position in the allocated memory block is undefined. In other words, one should not assume that the free memory is always located after the initialized elements.
reserve() squeeze()
Returns a const STL-style iterator pointing to the first byte in the byte-array.
The returned iterator is invalidated on detachment or when the QByteArray is modified.
begin() cend()
Returns a const STL-style iterator pointing just after the last byte in the byte-array.
cbegin() end()
Removes n bytes from the end of the byte array.
If n is greater than size() , the result is an empty byte array.
ba = QByteArray ( "STARTTLS \r\n " ) ba . chop ( 2 ) # ba == "STARTTLS" See also truncate() resize() first()
Clears the contents of the byte array and makes it null.
See also resize() isNull()
cs – CaseSensitivity
Returns an integer less than, equal to, or greater than zero depending on whether this QByteArray sorts before, at the same position as, or after the QByteArrayView bv . The comparison is performed according to case sensitivity cs .
See also operator== Character Case
bv – QByteArrayView
Returns true if this byte array contains an occurrence of the sequence of bytes viewed by bv ; otherwise returns false .
See also indexOf() count()
Returns true if the byte array contains the byte ch ; otherwise returns false .
This function is deprecated.
Use size() or length() instead.
Same as size() .
Returns the number of (potentially overlapping) occurrences of the sequence of bytes viewed by bv in this byte array.
See also contains() indexOf()
Returns the number of occurrences of byte ch in the byte array.
Returns true if this byte array ends with the sequence of bytes viewed by bv ; otherwise returns false .
url = QByteArray ( "http://qt-project.org/doc/qt-5.0/qtdoc/index.html" ) if url . endsWith ( ".html" ): ... See also startsWith() last()
Returns true if this byte array ends with byte ch ; otherwise returns false .
first – str
Removes the character denoted by it from the byte array. Returns an iterator to the character immediately after the erased character.
QByteArray ba = "abcdefg" ; auto it = ba . erase ( ba . cbegin ()); // ba is now "bcdefg" and it points to "b"
Sets every byte in the byte array to ch . If size is different from -1 (the default), the byte array is resized to size size beforehand.
ba = QByteArray ( "Istambul" ) ba . fill ( 'o' ) # ba == "oooooooo" ba . fill ( 'X' , 2 ) # ba == "XX" See also resize()
base64 – QByteArray
options – Combination of Base64Option
Returns a decoded copy of the Base64 array base64 , using the options defined by options . If options contains IgnoreBase64DecodingErrors (the default), the input is not checked for validity; invalid characters in the input are skipped, enabling the decoding process to continue with subsequent characters. If options contains AbortOnBase64DecodingErrors , then decoding will stop at the first invalid character.
For example:
text = QByteArray . fromBase64 ( "UXQgaXMgZ3JlYXQh" ) text . data () # returns "Qt is great!" QByteArray . fromBase64 ( "PHA+SGVsbG8/PC9wPg==" , QByteArray . Base64Encoding ) # returns "<p>Hello?</p>" QByteArray . fromBase64 ( "PHA-SGVsbG8_PC9wPg==" , QByteArray . Base64UrlEncoding ) # returns "<p>Hello?</p>"
The algorithm used to decode Base64-encoded data is defined in RFC 4648.
Returns the decoded data, or, if the AbortOnBase64DecodingErrors option was passed and the input data was invalid, an empty byte array.
The fromBase64Encoding() function is recommended in new code.
toBase64() fromBase64Encoding()
FromBase64Result
hexEncoded – QByteArray
Returns a decoded copy of the hex encoded array hexEncoded . Input is not checked for validity; invalid characters in the input are skipped, enabling the decoding process to continue with subsequent characters.
text = QByteArray . fromHex ( "517420697320677265617421" ) text . data () # returns "Qt is great!" See also toHex()
pctEncoded – QByteArray
percent – int
Decodes input from URI/URL-style percent-encoding.
Returns a byte array containing the decoded text. The percent parameter allows use of a different character than ‘%’ (for instance, ‘_’ or ‘=’) as the escape character. Equivalent to input. percentDecoded (percent).
text = QByteArray . fromPercentEncoding ( "Qt %20i s %20g reat%33" ) qDebug ( " %s " , text . data ()) # reports "Qt is great!" See also percentDecoded()
Constructs a QByteArray that uses the first size bytes of the data array. The bytes are not copied. The QByteArray will contain the data pointer. The caller guarantees that data will not be deleted or modified as long as this QByteArray and any copies of it exist that have not been modified. In other words, because QByteArray is an implicitly shared class and the instance returned by this function contains the data pointer, the caller must not delete data or modify it directly as long as the returned QByteArray and any copies exist. However, QByteArray does not take ownership of data , so the QByteArray destructor will never delete the raw data , even when the last QByteArray referring to data is destroyed.
A subsequent attempt to modify the contents of the returned QByteArray or any copy made from it will cause it to create a deep copy of the data array before doing the modification. This ensures that the raw data array itself will never be modified by QByteArray .
Here is an example of how to read data using a QDataStream on raw data in memory without copying the raw data into a QByteArray :
mydata = { ' \x00 ' , ' \x00 ' , ' \x03 ' , ' \x84 ' , ' \x78 ' , ' \x9c ' , ' \x3b ' , ' \x76 ' , ' \xec ' , ' \x18 ' , ' \xc3 ' , ' \x31 ' , ' \x0a ' , ' \xf1 ' , ' \xcc ' , ' \x99 ' , ... ' \x6d ' , ' \x5b ' data = QByteArray . fromRawData ( mydata , sizeof ( mydata )) in = QDataStream ( data , QIODevice . ReadOnly ) ...
A byte array created with fromRawData() is not ‘\0’-terminated, unless the raw data contains a ‘\0’ byte at position size . While that does not matter for QDataStream or functions like indexOf() , passing the byte array to a function accepting a const char * expected to be ‘\0’-terminated will fail.
setRawData() data() constData()
Returns a copy of the str string as a QByteArray .
See also toStdString() fromStdString()
Returns the first byte in the byte array. Same as at(0) .
back() at() operator[]()
Returns the index position of the start of the first occurrence of the sequence of bytes viewed by bv in this byte array, searching forward from index position from . Returns -1 if no match is found.
x = QByteArray ( "sticky question" ) y = QByteArrayView ( "sti" ) x . indexOf ( y ) # returns 0 x . indexOf ( y , 1 ) # returns 10 x . indexOf ( y , 10 ) # returns 10 x . indexOf ( y , 11 ) # returns -1 See also lastIndexOf() contains() count()
Returns the index position of the start of the first occurrence of the byte ch in this byte array, searching forward from index position from . Returns -1 if no match is found.
ba = QByteArray ( "ABCBA" ) ba . indexOf ( "B" ) # returns 1 ba . indexOf ( "B" , 1 ) # returns 1 ba . indexOf ( "B" , 2 ) # returns 3 ba . indexOf ( "X" ) # returns -1 See also lastIndexOf() contains()
Inserts len bytes, starting at data , at position i in the byte array.
This array grows to accommodate the insertion. If i is beyond the end of the array, the array is first extended with space characters to reach this i .
Inserts count copies of byte ch at index position i in the byte array.
Inserts s at index position i and returns a reference to this byte array.
The function is equivalent to insert(i, QByteArrayView(s))
See also append() prepend() replace() remove()
data – QByteArray
Inserts data at index position i and returns a reference to this byte array.
Inserts byte ch at index position i in the byte array.
data – QByteArrayView
ba = QByteArray ( "Meal" ) ba . insert ( 1 , QByteArrayView ( "ontr" )) # ba == "Montreal"
For large byte arrays, this operation can be slow ( linear time ), because it requires moving all the bytes at indexes i and above by at least one position further in memory.
Returns true if the byte array has size 0; otherwise returns false .
QByteArray () . isEmpty () # returns true QByteArray ( "" ) . isEmpty () # returns true QByteArray ( "abc" ) . isEmpty () # returns false See also size()
Returns true if this byte array is lowercase, that is, if it’s identical to its toLower() folding.
Note that this does not mean that the byte array only contains lowercase letters; only that it contains no ASCII uppercase letters.
See also isUpper() toLower()
Returns true if this byte array is null; otherwise returns false .
QByteArray () . isNull () # returns true QByteArray ( "" ) . isNull () # returns false QByteArray ( "abc" ) . isNull () # returns false
Qt makes a distinction between null byte arrays and empty byte arrays for historical reasons. For most applications, what matters is whether or not a byte array contains any data, and this can be determined using isEmpty() .
other – QByteArray
Returns true if this byte array is uppercase, that is, if it’s identical to its toUpper() folding.
Note that this does not mean that the byte array only contains uppercase letters; only that it contains no ASCII lowercase letters.
See also isLower() toUpper()
Returns true if this byte array contains valid UTF-8 encoded data, or false otherwise.
Returns the index position of the start of the last occurrence of the sequence of bytes viewed by bv in this byte array, searching backward from index position from .
If from is -1, the search starts at the last character; if it is -2, at the next to last character and so on.
Returns -1 if no match is found.
x = QByteArray ( "crazy azimuths" ) y = QByteArrayView ( "az" ) x . lastIndexOf ( y ) # returns 6 x . lastIndexOf ( y , 6 ) # returns 6 x . lastIndexOf ( y , 5 ) # returns 2 x . lastIndexOf ( y , 1 ) # returns -1
When searching for a 0-length bv , the match at the end of the data is excluded from the search by a negative from , even though -1 is normally thought of as searching from the end of the byte array: the match at the end is after the last character, so it is excluded. To include such a final empty match, either give a positive value for from or omit the from parameter entirely.
indexOf() contains() count()
Returns the index position of the start of the last occurrence of byte ch in this byte array, searching backward from index position from . If from is -1 (the default), the search starts at the last byte (at index size() - 1). Returns -1 if no match is found.
ba = QByteArray ( "ABCBA" ) ba . lastIndexOf ( "B" ) # returns 3 ba . lastIndexOf ( "B" , 3 ) # returns 3 ba . lastIndexOf ( "B" , 2 ) # returns 1 ba . lastIndexOf ( "X" ) # returns -1 See also indexOf() contains()
Returns the index position of the start of the last occurrence of the sequence of bytes viewed by bv in this byte array, searching backward from the end of the byte array. Returns -1 if no match is found.
x = QByteArray ( "crazy azimuths" ) y = QByteArrayView ( "az" ) x . lastIndexOf ( y ) # returns 6 x . lastIndexOf ( y , 6 ) # returns 6 x . lastIndexOf ( y , 5 ) # returns 2 x . lastIndexOf ( y , 1 ) # returns -1 See also indexOf() contains() count()
width – int
truncate – bool
Returns a byte array of size width that contains this byte array padded with the fill byte.
If truncate is false and the size() of the byte array is more than width , then the returned byte array is a copy of this byte array.
If truncate is true and the size() of the byte array is more than width , then any bytes in a copy of the byte array after position width are removed, and the copy is returned.
x = QByteArray ( "apple" ) y = x . leftJustified ( 8 , '.' ) # y == "apple..." See also rightJustified()
index – int
arg__1 – int
See also toLongLong()
Returns a byte-array representing the whole number n as text.
Returns a byte array containing a string representing n , using the specified base (ten by default). Bases 2 through 36 are supported, using letters for digits beyond 9: A is ten, B is eleven and so on.
n = 63 QByteArray . number ( n ) # returns "63" QByteArray . number ( n , 16 ) # returns "3f" QByteArray . number ( n , 16 ) . toUpper () # returns "3F"
The format of the number is not localized; the default C locale is used regardless of the user’s locale. Use QLocale to perform locale-aware conversions between numbers and strings.
setNum() toInt()
arg__1 – float
format – int
precision – int
Returns a byte-array representing the floating-point number n as text.
Returns a byte array containing a string representing n , with a given format and precision , with the same meanings as for number(double, char, int) . For example:
ba = QByteArray . number ( 12.3456 , 'E' , 3 ) # ba == 1.235E+01 See also toDouble() FloatingPointPrecisionOption
arg__1 – PyUnicode
a2 – QByteArray
Returns true if byte array a1 is not equal to byte array a2 ; otherwise returns false .
See also compare()
Returns true if this byte array is not equal to the UTF-8 encoding of str ; otherwise returns false .
The comparison is case sensitive.
You can disable this operator by defining QT_NO_CAST_FROM_ASCII when you compile your applications. You then need to call fromUtf8() , fromLatin1() , or fromLocal8Bit() explicitly if you want to convert the byte array to a QString before doing the comparison.
Returns a byte array that is the result of concatenating byte array a1 and ‘\0’-terminated string a2 .
rhs – QByteArray
Returns a byte array that is the result of concatenating byte array a1 and byte array a2 .
See also operator+=()
Returns a byte array that is the result of concatenating byte array a1 and byte a2 .
Appends the byte ch onto the end of this byte array and returns a reference to this byte array.
Appends the byte array ba onto the end of this byte array and returns a reference to this byte array.
x = QByteArray ( "free" ) y = QByteArray ( "dom" ) x += y # x == "freedom"
This operation typically does not suffer from allocation overhead, because QByteArray preallocates extra space at the end of the data so that it may grow without reallocating for each append operation.
See also append() prepend()
Returns true if byte array a1 is lexically less than byte array a2 ; otherwise returns false .
Returns true if this byte array is lexically less than the UTF-8 encoding of str ; otherwise returns false .
Returns true if byte array a1 is lexically less than the ‘\0’-terminated string a2 ; otherwise returns false .
Returns true if this byte array is lexically less than or equal to the UTF-8 encoding of str ; otherwise returns false .
Returns true if byte array a1 is lexically less than or equal to byte array a2 ; otherwise returns false .
Returns true if byte array a1 is equal to byte array a2 ; otherwise returns false .
Returns true if this byte array is equal to the UTF-8 encoding of str ; otherwise returns false .
Returns true if byte array a1 is lexically greater than byte array a2 ; otherwise returns false .
Returns true if this byte array is lexically greater than the UTF-8 encoding of str ; otherwise returns false .
Returns true if this byte array is greater than or equal to the UTF-8 encoding of str ; otherwise returns false .
Returns true if byte array a1 is lexically greater than or equal to byte array a2 ; otherwise returns false .
Same as at( i ).
Decodes URI/URL-style percent-encoding.
Returns a byte array containing the decoded text. The percent parameter allows use of a different character than ‘%’ (for instance, ‘_’ or ‘=’) as the escape character.
encoded = QByteArray ( "Qt %20i s %20g reat%33" ) decoded = encoded . percentDecoded () # Set to "Qt is great!"
Given invalid input (such as a string containing the sequence “%G5”, which is not a valid hexadecimal number) the output will be invalid as well. As an example: the sequence “%G5” could be decoded to ‘W’.
toPercentEncoding() fromPercentEncoding()
Prepends the byte array view ba to this byte array and returns a reference to this byte array.
This operation is typically very fast ( constant time ), because QByteArray preallocates extra space at the beginning of the data, so it can grow without reallocating the entire array each time.
x = QByteArray ( "ship" ) y = QByteArray ( "air" ) x . prepend ( y ) # x == "airship"
This is the same as insert(0, ba ).
See also append() insert()
Prepends the byte ch to this byte array.
Prepends ba to this byte array.
Prepends len bytes starting at str to this byte array. The bytes prepended may include ‘\0’ bytes.
Prepends count copies of byte ch to this byte array.
Same as append( str ).
Same as prepend( str ).
Removes len bytes from the array, starting at index position pos , and returns a reference to the array.
If pos is out of range, nothing happens. If pos is valid, but pos + len is larger than the size of the array, the array is truncated at position pos .
ba = QByteArray ( "Montreal" ) ba . remove ( 1 , 4 ) # ba == "Meal"
Element removal will preserve the array’s capacity and not reduce the amount of allocated memory. To shed extra capacity and free as much memory as possible, call squeeze() after the last change to the array’s size.
See also insert() replace() squeeze()
Removes the character at index pos . If pos is out of bounds (i.e. pos >= size() ) this function does nothing.
See also remove()
Removes the first character in this byte array. If the byte array is empty, this function does nothing.
Removes the last character in this byte array. If the byte array is empty, this function does nothing.
times – int
Returns a copy of this byte array repeated the specified number of times .
If times is less than 1, an empty byte array is returned.
ba = QByteArray ( "ab" ) ba . repeated ( 4 ) # returns "abababab"
Replaces len bytes from index position pos with alen bytes starting at position after . The bytes inserted may include ‘\0’ bytes.
s – QByteArrayView
Replaces len bytes from index position pos with the byte array after , and returns a reference to this byte array.
x = QByteArray ( "Say yes!" ) y = QByteArray ( "no" ) x . replace ( 4 , 3 , y ) # x == "Say no!" See also insert() remove()
before – int
after – int
Replaces every occurrence of the byte before with the byte after .
after – QByteArrayView
Replaces every occurrence of the byte before with the byte array after .
before – str
bsize – int
after – str
asize – int
Replaces every occurrence of the bsize bytes starting at before with the asize bytes starting at after . Since the sizes of the strings are given by bsize and asize , they may contain ‘\0’ bytes and do not need to be ‘\0’-terminated.
before – QByteArrayView
Replaces every occurrence of the byte array before with the byte array after .
ba = QByteArray ( "colour behaviour flavour neighbour" ) ba . replace ( QByteArray ( "ou" ), QByteArray ( "o" )) # ba == "color behavior flavor neighbor"
Attempts to allocate memory for at least size bytes.
If you know in advance how large the byte array will be, you can call this function, and if you call resize() often you are likely to get better performance.
If in doubt about how much space shall be needed, it is usually better to use an upper bound as size , or a high estimate of the most likely size, if a strict upper bound would be much bigger than this. If size is an underestimate, the array will grow as needed once the reserved size is exceeded, which may lead to a larger allocation than your best overestimate would have and will slow the operation that triggers it.
reserve() reserves memory but does not change the size of the byte array. Accessing data beyond the end of the byte array is undefined behavior. If you need to access memory beyond the current end of the array, use resize() .
The sole purpose of this function is to provide a means of fine tuning QByteArray ‘s memory usage. In general, you will rarely ever need to call this function.
See also squeeze() capacity()
Sets the size of the byte array to size bytes.
If size is greater than the current size, the byte array is extended to make it size bytes with the extra bytes added to the end. The new bytes are uninitialized.
If size is less than the current size, bytes beyond position size are excluded from the byte array.
While resize() will grow the capacity if needed, it never shrinks capacity. To shed excess capacity, use squeeze() .
size() truncate() squeeze()
Sets the size of the byte array to newSize bytes.
If newSize is greater than the current size, the byte array is extended to make it newSize bytes with the extra bytes added to the end. The new bytes are initialized to c .
If newSize is less than the current size, bytes beyond position newSize are excluded from the byte array.
Returns a byte array of size width that contains the fill byte followed by this byte array.
If truncate is false and the size of the byte array is more than width , then the returned byte array is a copy of this byte array.
If truncate is true and the size of the byte array is more than width , then the resulting byte array is truncated at position width .
x = QByteArray ( "apple" ) y = x . rightJustified ( 8 , '.' ) # y == "...apple" See also leftJustified()
Represent the floating-point number n as text.
Sets this byte array to a string representing n , with a given format and precision (with the same meanings as for number(double, char, int) ), and returns a reference to this byte array.
See also toDouble() FloatingPointPrecisionOption
Represent the whole number n as text.
Sets this byte array to a string representing n in base base (ten by default) and returns a reference to this byte array. Bases 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
ba = QByteArray () n = 63 ba . setNum ( n ) # ba == "63" ba . setNum ( n , 16 ) # ba == "3f"
number() toInt()
Resets the QByteArray to use the first size bytes of the data array. The bytes are not copied. The QByteArray will contain the data pointer. The caller guarantees that data will not be deleted or modified as long as this QByteArray and any copies of it exist that have not been modified.
This function can be used instead of fromRawData() to re-use existing QByteArray objects to save memory re-allocations.
See also fromRawData() data() constData()
This function is provided for STL compatibility. It is equivalent to squeeze() .
Returns a copy of this byte array that has spacing characters removed from the start and end, and in which each sequence of internal spacing characters is replaced with a single space.
The spacing characters are those for which the standard C++ isspace() function returns true in the C locale; these are the ASCII characters tabulation ‘\t’, line feed ‘\n’, carriage return ‘\r’, vertical tabulation ‘\v’, form feed ‘\f’, and space ‘ ‘.
ba = QByteArray ( " lots \t of \n whitespace \r\n " ) ba = ba . simplified () # ba == "lots of whitespace" See also trimmed() SpecialCharacter Spacing Characters
Returns the number of bytes in this byte array.
The last byte in the byte array is at position size() - 1. In addition, QByteArray ensures that the byte at position size() is always ‘\0’, so that you can use the return value of data() and constData() as arguments to functions that expect ‘\0’-terminated strings. If the QByteArray object was created from a raw data that didn’t include the trailing ‘\0’-termination byte, then QByteArray doesn’t add it automatically unless a deep copy is created.
ba = QByteArray ( "Hello" ) n = ba . size () # n == 5 ba . data ()[ 0 ] # returns 'H' ba . data ()[ 4 ] # returns 'o' ba . data ()[ 5 ] # returns '\0' See also isEmpty() resize()
.list of QByteArray
Splits the byte array into subarrays wherever sep occurs, and returns the list of those arrays. If sep does not match anywhere in the byte array, split() returns a single-element list containing this byte array.
Releases any memory not required to store the array’s data.
See also reserve() capacity()
Returns true if this byte array starts with byte ch ; otherwise returns false .
Returns true if this byte array starts with the sequence of bytes viewed by bv ; otherwise returns false .
url = QByteArray ( "ftp://ftp.qt-project.org/" ) if url . startsWith ( "ftp:" ): ... See also endsWith() first()
Swaps byte array other with this byte array. This operation is very fast and never fails.
Returns a copy of the byte array, encoded using the options options .
text = QByteArray ( "Qt is great!" ) text . toBase64 () # returns "UXQgaXMgZ3JlYXQh" text = QByteArray ( "" ) text . toBase64 ( QByteArray . Base64Encoding | QByteArray . OmitTrailingEquals ) # returns "PHA+SGVsbG8/PC9wPg" text . toBase64 ( QByteArray . Base64Encoding ) # returns "PHA+SGVsbG8/PC9wPg==" text . toBase64 ( QByteArray . Base64UrlEncoding ) # returns "PHA-SGVsbG8_PC9wPg==" text . toBase64 ( QByteArray . Base64UrlEncoding | QByteArray . OmitTrailingEquals ) # returns "PHA-SGVsbG8_PC9wPg"
The algorithm used to encode Base64-encoded data is defined in RFC 4648.
See also fromBase64()
Returns the byte array converted to a double value.
Returns an infinity if the conversion overflows or 0.0 if the conversion fails for other reasons (e.g. underflow).
If ok is not None , failure is reported by setting *``ok`` to false , and success by setting *``ok`` to true .
string = QByteArray ( "1234.56" ) ok = bool () a = string . toDouble ( ok ) # a == 1234.56, ok == true string = "1234.56 Volt" a = str . toDouble ( ok ) # a == 0, ok == false
The QByteArray content may only contain valid numerical characters which includes the plus/minus sign, the character e used in scientific notation, and the decimal point. Including the unit or additional characters leads to a conversion error.
The conversion of the number is performed in the default C locale, regardless of the user’s locale. Use QLocale to perform locale-aware conversions between numbers and strings.
This function ignores leading and trailing whitespace.
See also number()
Returns the byte array converted to a float value.
string = QByteArray ( "1234.56" ) ok = bool () a = string . toFloat ( ok ) # a == 1234.56, ok == true string = "1234.56 Volt" a = str . toFloat ( ok ) # a == 0, ok == false
separator – int
Returns a hex encoded copy of the byte array.
The hex encoding uses the numbers 0-9 and the letters a-f.
If separator is not ‘\0’, the separator character is inserted between the hex bytes.
macAddress = QByteArray . fromHex ( "123456abcdef" ) macAddress . toHex ( ':' ) # returns "12:34:56:ab:cd:ef" macAddress . toHex ( 0 ) # returns "123456abcdef" See also fromHex()
Returns the byte array converted to an int using base base , which is ten by default. Bases 0 and 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
If base is 0, the base is determined automatically using the following rules: If the byte array begins with “0x”, it is assumed to be hexadecimal (base 16); otherwise, if it begins with “0b”, it is assumed to be binary (base 2); otherwise, if it begins with “0”, it is assumed to be octal (base 8); otherwise it is assumed to be decimal.
Returns 0 if the conversion fails.
str = QByteArray ( "FF" ) ok = bool () hex = str . toInt ( ok , 16 ) # hex == 255, ok == true dec = str . toInt ( ok , 10 ) # dec == 0, ok == false
Support for the “0b” prefix was added in Qt 6.4.
Returns the byte array converted to a long int using base base , which is ten by default. Bases 0 and 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
str = QByteArray ( "FF" ) ok = bool () hex = str . toLong ( ok , 16 ) # hex == 255, ok == true dec = str . toLong ( ok , 10 ) # dec == 0, ok == false
Returns the byte array converted to a long long using base base , which is ten by default. Bases 0 and 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
Returns a copy of the byte array in which each ASCII uppercase letter converted to lowercase.
x = QByteArray ( "Qt by THE QT COMPANY" ) y = x . toLower () # y == "qt by the qt company" See also isLower() toUpper() Character Case
exclude – QByteArray
include – QByteArray
Returns a URI/URL-style percent-encoded copy of this byte array. The percent parameter allows you to override the default ‘%’ character for another.
By default, this function will encode all bytes that are not one of the following:
ALPHA (“a” to “z” and “A” to “Z”) / DIGIT (0 to 9) / “-” / “.” / “_” / “~”
To prevent bytes from being encoded pass them to exclude . To force bytes to be encoded pass them to include . The percent character is always encoded.
QByteArray text = "{a fishy string?}" QByteArray ba = text . toPercentEncoding ( " {} " , "s" ) qDebug ( " %s " , ba . constData ()) # prints "{a fi%73hy %73tring%3F}"
The hex encoding uses the numbers 0-9 and the uppercase letters A-F.
See also fromPercentEncoding() toPercentEncoding()
Returns the byte array converted to a short using base base , which is ten by default. Bases 0 and 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
Returns a std::string object with the data contained in this QByteArray .
This operator is mostly useful to pass a QByteArray to a function that accepts a std::string object.
See also fromStdString() toStdString()
Returns the byte array converted to an unsigned int using base base , which is ten by default. Bases 0 and 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
Returns the byte array converted to an unsigned long int using base base , which is ten by default. Bases 0 and 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
Returns the byte array converted to an unsigned long long using base base , which is ten by default. Bases 0 and 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
Returns the byte array converted to an unsigned short using base base , which is ten by default. Bases 0 and 2 through 36 are supported, using letters for digits beyond 9; A is ten, B is eleven and so on.
Returns a copy of the byte array in which each ASCII lowercase letter converted to uppercase.
x = QByteArray ( "Qt by THE QT COMPANY" ) y = x . toUpper () # y == "QT BY THE QT COMPANY" See also isUpper() toLower() Character Case
Returns a copy of this byte array with spacing characters removed from the start and end.
ba = QByteArray ( " lots \t of \n whitespace \r\n " ) ba = ba . trimmed () # ba == "lots\t of\nwhitespace"
Unlike simplified() , trimmed() leaves internal spacing unchanged.
See also simplified() SpecialCharacter Spacing Characters
Truncates the byte array at index position pos .
If pos is beyond the end of the array, nothing happens.
ba = QByteArray ( "Stockholm" ) ba . truncate ( 5 ) # ba == "Stock" See also chop() resize() first()
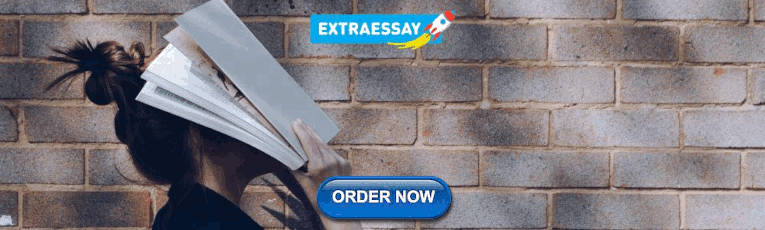
IMAGES
VIDEO
COMMENTS
This type of byte assignment will implicitly produce the integers needed to assign to the bytearray, instead of e.g. d[0]. If the length of the slice is smaller than the assignment, the array will grow as in my example. (*that's just the way to slice in Python, the emphasis is just because I forgot about it in the question.)
Bytestrings (and strings in general) are immutable objects in Python. Once you create them, you can't change them. Instead, you have to create a new one that happens to have some of the old content. (For instance, with a basic string, newString = oldString[:offset] + newChar + oldString[offset+1:] or the like.)
In Python, the bytearray() function is a powerful tool that allows you to create a mutable sequence of bytes. This function is particularly useful when you need to manipulate binary data, work with low-level data structures, or modify existing byte sequences.
7921515. I guess the root of my question is why a bytearray index is being represented by a string. A bytearray object should be mutable and individual elements should be integers. - digitaladdictions. Apr 18, 2014 at 3:27. So, use bytearray not b"". b = bytearray ('\xff'); b [0] = '\xee'. - senior_pimiento.
We can convert a list of integers to bytearray using bytearray () function in python. The bytearray () function takes the list of integers between 0 and 255 as input and returns the corresponding bytearray object as follows. myList=[1,2,56,78,90] print ("The List is:",myList) myObj=bytearray (myList) print ("The bytearray object is:",myObj)
💡 Problem Formulation: In this article, we explore how to modify a single byte within a Python bytearray. A bytearray is a mutable sequence of integers in the range 0 <= x < 256. ... May be less efficient than the direct assignment method. Method 4: Using the struct Module. Versatile for binary data. Potentially complex for simple use cases ...
The bytearray object is better suited for situations where frequent manipulation of data is expected. For example, the updating of data received from a network transmission before retransmission. bytearray objects also provide methods afforded to mutable objects such as sizing, slicing, member assignment, insertion, reversal, and many more.
A bytearray is also another sequence type in Python; which holds a sequence of bytes. Unlike bytes; bytearray objects are mutable. That means, we can modify the elements in bytearray objects.. Define a bytearray Object. bytearray objects are created using the bytearray class constructor. There is no relevant literal syntax to create bytearray objects.. The syntax of the bytearray constructor is:
Python bytearray() Method. The bytearray() ... Parameters: source: (Optional) An integer or iterable to convert it to a byte array. If the source is a string, it must be with the encoding parameter. If the source is an integer, the array will have that size and will be initialized with null bytes.
bytearray() Parameters. bytearray() takes three optional parameters: source (Optional) - source to initialize the array of bytes. encoding (Optional) - if the source is a string, the encoding of the string. errors (Optional) - if the source is a string, the action to take when the encoding conversion fails (Read more: String encoding) The source parameter can be used to initialize the byte ...
Python bytearray () function has the following syntax: Syntax: bytearray (source, encoding, errors) Parameters: source[optional]: Initializes the array of bytes. encoding[optional]: Encoding of the string. errors[optional]: Takes action when encoding fails. Returns: Returns an array of bytes of the given size.
ByteArray is a data structure in Python that can be used when we wish to store a collection of bytes in an ordered manner in a contiguous area of memory. ByteArray comes under binary data types. You can use the bytearray () constructor to create a ByteArray object as shown below. >>> numbers = [1, 2, 3, 4]
Slice assignment to a bytearray object accepts anything that implements the PEP 3118 buffer API, or an iterable of integers in range(256). Indexing. Indexing bytes and bytearray returns small ints (like the bytes type in 3.0a1, and like lists or array.array('B')). Assignment to an item of a bytearray object accepts an int in range(256).
One byte is a memory location with a size of 8 bits. A bytes object is an immutable sequence of bytes, conceptually similar to a string. Because each byte must fit into 8 bits, each member of a bytes object is an unsigned int that satisfies. The bytes object is important because data written to disk is written as a stream of bytes, and because ...
bytes() 0.011996746063232422. bytearray() 0.042777299880981445. So the difference is roughly 3-4x in performance: bytes() is 300-400% faster than bytearray(). However, don't let this tiny performance edge fool you! Edit: User ASL97 submitted another variation of this test where the opposite happened: ~ $ cat test2.py.
It is an immutable array of bytes. Buffer protocol: Bytearray, bytes and memoryview act upon the buffer protocol. They all share similar syntax with small differences. Python program that creates bytes object elements = [5, 10, 0, 0, 100] # Create immutable bytes object. data = bytes (elements) # Loop over bytes. for d in data: print (d) Output ...
Step 1 We create a list of 6 integers. Each number in the list is between 0 and 255 (inclusive). Step 2 Here we create a bytearray from the list—we use the bytearray built-in function. Step 3 We modify the first 2 elements in the bytearray—this cannot be done with a bytes object. Then we loop over the result.
Bytes, Bytearray. Python supports a range of types to store sequences. There are six sequence types: strings, byte sequences (bytes objects), byte arrays (bytearray objects), lists, tuples, and range objects. Strings contain Unicode characters. Their literals are written in single or double quotes : 'python', "data".
Although the size() is 5, the byte array also maintains an extra '\0' byte at the end so that if a function is used that asks for a pointer to the underlying data (e.g. a call to data()), the data pointed to is guaranteed to be '\0'-terminated.. QByteArray makes a deep copy of the const char * data, so you can modify it later without experiencing side effects.
I know this is a very basic question about Python, so if you could point me in the right direction... python; python-3.x; arrays; Share. Improve this question. Follow ... you're using a byte array (the concept) even if you're not using the bytearray type. Either of the two methods in my answer should solve your problem.
bytearray is a sequence-type, and it supports slice-based operations. The "insert at position i" idiom with slices goes like this x[i:i] = <a compatible sequence>. So, for the fist position: >>> a bytearray(b'\x00\x00\x00\x01') >>> b bytearray(b'\x00\x00\x00\x02') >>> a[0:0] = b >>> a bytearray(b'\x00\x00\x00\x02\x00\x00\x00\x01') For the third ...
Sorry if my English is bad, I speak Korean as mother tongue. I was writing code that changes bytearray partially. And what I was trying to is to give name for memoryview of some sections of bytearray, and update bytearry with that memoryview. roughly like: