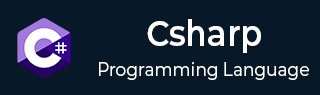
- C# Basic Tutorial
- C# - Overview
- C# - Environment
- C# - Program Structure
- C# - Basic Syntax
- C# - Data Types
- C# - Type Conversion
- C# - Variables
- C# - Constants
- C# - Operators
- C# - Decision Making
- C# - Encapsulation
- C# - Methods
- C# - Nullables
- C# - Arrays
- C# - Strings
- C# - Structure
- C# - Classes
- C# - Inheritance
- C# - Polymorphism
- C# - Operator Overloading
- C# - Interfaces
- C# - Namespaces
- C# - Preprocessor Directives
- C# - Regular Expressions
- C# - Exception Handling
- C# - File I/O
- C# Advanced Tutorial
- C# - Attributes
- C# - Reflection
- C# - Properties
- C# - Indexers
- C# - Delegates
- C# - Events
- C# - Collections
- C# - Generics
- C# - Anonymous Methods
- C# - Unsafe Codes
- C# - Multithreading
- C# Useful Resources
- C# - Questions and Answers
- C# - Quick Guide
- C# - Useful Resources
- C# - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
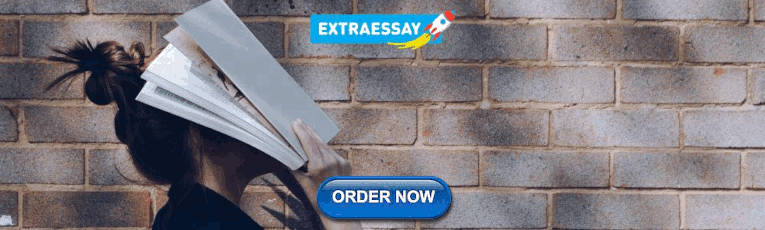
C# - Assignment Operators
There are following assignment operators supported by C# −
The following example demonstrates all the assignment operators available in C# −
When the above code is compiled and executed, it produces the following result −
C# Tutorial
C# examples, c# assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
Try it Yourself »
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, introduction.
- Your First C# Program
- C# Comments
- C# Variables and (Primitive) Data Types
C# Operators
C# Basic Input and Output
- C# Expressions, Statements and Blocks
Flow Control
- C# if, if...else, if...else if and Nested if Statement
C# ternary (? :) Operator
- C# for loop
- C# while and do...while loop
- Nested Loops in C#: for, while, do-while
- C# break Statement
- C# continue Statement
- C# switch Statement
- C# Multidimensional Array
- C# Jagged Array
- C# foreach loop
- C# Class and Object
- C# Access Modifiers
- C# Variable Scope
- C# Constructor
- C# this Keyword
- C# Destructor
- C# static Keyword
- C# Inheritance
- C# abstract class and method
- C# Nested Class
- C# Partial Class and Partial Method
- C# sealed class and method
- C# interface
- C# Polymorphism
- C# Method Overloading
- C# Constructor Overloading
Exception Handling
- C# Exception and Its Types
- C# Exception Handling
- C# Collections
- C# ArrayList
- C# SortedList
- C# Hashtable
- C# Dictionary
- C# Recursion
- C# Lambda Expression
- C# Anonymous Types
- C# Generics
- C# Iterators
- C# Delegates
- C# Indexers
- C# Regular Expressions
Additional Topics
- C# Keywords and Identifiers
- C# Type Conversion
C# Operator Precedence and Associativity
C# Bitwise and Bit Shift Operators
- C# using Directive
- C# Preprocessor Directives
- Namespaces in C# Programming
- C# Nullable Types
- C# yield keyword
- C# Reflection
C# Tutorials
Operators are symbols that are used to perform operations on operands. Operands may be variables and/or constants.
For example , in 2+3 , + is an operator that is used to carry out addition operation, while 2 and 3 are operands.
Operators are used to manipulate variables and values in a program. C# supports a number of operators that are classified based on the type of operations they perform.
1. Basic Assignment Operator
Basic assignment operator (=) is used to assign values to variables. For example,
Here, 50.05 is assigned to x.
Example 1: Basic Assignment Operator
When we run the program, the output will be:
This is a simple example that demonstrates the use of assignment operator.
You might have noticed the use of curly brackets { } in the example. We will discuss about them in string formatting . For now, just keep in mind that {0} is replaced by the first variable that follows the string, {1} is replaced by the second variable and so on.
2. Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations such as addition, subtraction, multiplication, division, etc.
For example,
Example 2: Arithmetic Operators
Arithmetic operations are carried out in the above example. Variables can be replaced by constants in the statements. For example,
3. Relational Operators
Relational operators are used to check the relationship between two operands. If the relationship is true the result will be true , otherwise it will result in false .
Relational operators are used in decision making and loops.
Example 3: Relational Operators
4. logical operators.
Logical operators are used to perform logical operation such as and , or . Logical operators operates on boolean expressions ( true and false ) and returns boolean values. Logical operators are used in decision making and loops.
Here is how the result is evaluated for logical AND and OR operators.
In simple words, the table can be summarized as:
- If one of the operand is true, the OR operator will evaluate it to true .
- If one of the operand is false, the AND operator will evaluate it to false .
Example 4: Logical Operators
5. unary operators.
Unlike other operators, the unary operators operates on a single operand.
Example 5: Unary Operators
The increment (++) and decrement (--) operators can be used as prefix and postfix. If used as prefix, the change in value of variable is seen on the same line and if used as postfix, the change in value of variable is seen on the next line. This will be clear by the example below.
Example 6: Post and Pre Increment operators in C#
We can see the effect of using ++ as prefix and postfix. When ++ is used after the operand, the value is first evaluated and then it is incremented by 1 . Hence the statement
prints 10 instead of 11 . After the value is printed, the value of number is incremented by 1 .
The process is opposite when ++ is used as prefix. The value is incremented before printing. Hence the statement
prints 12 .
The case is same for decrement operator (--) .
6. Ternary Operator
The ternary operator ? : operates on three operands. It is a shorthand for if-then-else statement. Ternary operator can be used as follows:
The ternary operator works as follows:
- If the expression stated by Condition is true , the result of Expression1 is assigned to variable.
- If it is false , the result of Expression2 is assigned to variable.
Example 7: Ternary Operator
To learn more, visit C# ternary operator .
7. Bitwise and Bit Shift Operators
Bitwise and bit shift operators are used to perform bit manipulation operations.
Example 8: Bitwise and Bit Shift Operator
To learn more, visit C# Bitwise and Bit Shift operator .
8. Compound Assignment Operators
Example 9: compound assignment operator.
We will discuss about Lambda operators in later tutorial.
Table of Contents
- Basic Assignment Operator
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Ternary Operator
- Bitwise and Bit Shift Operators
- Compound Assignment Operators
Sorry about that.
Related Tutorials
C# Tutorial
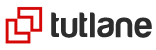
C# Assignment Operators with Examples
In c#, Assignment Operators are useful to assign a new value to the operand, and these operators will work with only one operand.
For example, we can declare and assign a value to the variable using the assignment operator ( = ) like as shown below.
If you observe the above sample, we defined a variable called “ a ” and assigned a new value using an assignment operator ( = ) based on our requirements.
The following table lists the different types of operators available in c# assignment operators.
C# Assignment Operators Example
Following is the example of using assignment Operators in the c# programming language.
If you observe the above example, we defined a variable or operand “ x ” and assigning new values to that variable by using assignment operators in the c# programming language.
Output of C# Assignment Operators Example
When we execute the above c# program, we will get the result as shown below.
This is how we can use assignment operators in c# to assign new values to the variable based on our requirements.
Table of Contents
- Assignment Operators in C# with Examples
- C# Assignment Operator Example
- Output of C# Assignment Operator Example
- Network Programming
- Windows Programming
- Visual Studio
- Visual Basic

Working with C Sharp Math Operators
We discussed C# data types in a previous article and today we will be following this up with a .Net tutorial that shows developers how to work with one of the data types we covered: numeric data types. Specifically, we will be learning how to use C# math operators and perform mathematical operations using C#. We will have plenty of code examples for you to practice along with, so open up your favorite integrated development environment (IDE) or code editor and let’s get started!
If you missed our previous articles in this series, we encourage you to read them. Likewise, if you need to refresh your memory, you can always revisit them by following the links below:
- C# Data Types Explained
- Commenting Best Practices in C#
- C# for Beginners
- Working with Strings in C#
Math Operators in C#
Below you will find code examples showing how to work with the various math and numeric operators in C#. Some of them work in a similar manner to the mathematical operators you are used to from school and every day life. Others, while they may seem similar, work in unexpected and even surprising ways. We will begin with one of the most used operators in all of C# – the assignment operator.
Assignment Operator in C#
You have no doubt seen the assignment operator many times in your life; it is written using the equals sign or = . There are several assignment operators available in C# and the most basic is used to assign a simple value to a variable. The item to the left of the = sign is the variable name, while the value to the right of the = sign is the value that you want to assign to the variable. Here is how you use the basic assignment operator in C# code:
In this code, we create an integer variable named age and assign it the value 44 . This is achieved in the line that says int age = 44; . Next, we use the print statement Console.WriteLine to print some text and the value of our variable to the screen. Running this code in your code editor would result in the following output:
You can also use other variables to assign a value to a variable using the assignment operator, as shown in the following example:
In this example, we have created three variables. The first variable, age , represents my age. The second variable, ageTwo , represents my age when I am twice as old as I am now. Finally, we create a third variable, futureAge , which we assign the value of age + ageTwo – this shows that you can assign the value of one or more variables to another variable with the assignment operator. The result of running this code is:
Another way to assign the value of an existing variable to a new variable would be to simply do the following:
This results in:
The Addition + Operator in C#
The addition operator – or + operator – is used in a similar fashion to its mathematical equivalent you have been using your entire life. You can use it to add two or more numbers to one another when you apply it to an integer value or a floating-point number. Note that there is a difference when you use the + operator on an int versus when you use it on a float . We will discuss that difference momentarily. First, however, check out the following example where we demonstrate how to use the C# mathematical + operator :
The result of this code, which adds the values of two integers together, is:
Another way to use the + operator on an int in C# is by using it to assign a value to a variable. Check out the following code example:
Here, we create a variable named valueOne and assign is the result of the equation 8+12 . The result of running this in your IDE is:
Anytime that you add an integer value to another integer, the result will always be an integer value. However, if you add an integer to a floating-point number, the result is always a float. Try running the following code to see this at work:
Running this code will create the following output:
A final thing to take note of: adding two floating point numbers will, as you might imagine, always result in a float.
The subtraction – operator in C#
The subtraction operator – or – operator – in C# works much as you would expect it to. Its purpose is to perform subtraction in a mathematical equation. Again, it works the same as its math equivalent. Like the + operator , when you subtract an integer from an integer, you always return an integer value. However, subtracting an integer from a floating-point number (or vice versa), the value is always a floating-point number.
Here is how you subtract two integer values from one another with the – operator in C#:
The output of this code is:
Here is some code showing what happens when you subtract an integer from a float or vice versa:
Here, the result of running this code is:
Finally, you can also assign a value to a variable by subtracting two variables from one another and storing the result of the equation in a variable. Here is how that looks in code:
This code adds the result of the equation 12 – 8 – or valueInteger2 – valueInteger1 – to the variable named valueInteger3. The result of running this code in your IDE?
The Mulitplication * Operator in C#
The mutiplication operator – or * operator – performs multiplication on two or more values, just as it does in mathematical equations, though you may also have used x for multiplying numbers in school, as in 4 x 2 = 8 . When you multiply two integers together, your result is always an integer. However, when you multiply an integer by a floating-point number, the result is always a floating-point number. Multiplying two or more decimals, meanwhile, always results in a decimal value.
Here is an example showing how to use the multiplication * operator in C# on two integer values:
Here is the result of running this code:
Likewise, here is some code showing what happens when you multiply an integer by a floating-point number in C#
The outcome of this code snippet is:
Finally, as with the + operator and the – operator , you can of course assign a value to a variable by assigning it the result of two variables multiplied by one another. Here is a sample of that in code:
The result of running this code would give you:
The Division / Operator in C#
The division operator – or / operator – is used to divide two or more numeric values. When using the division operator, be aware that dividing an integer by an integer always results in an integer – even if there is a remainder. In the event of a remainder, C# rounds down. Consider the following code:
Normally, if you divided 7 / 4 , you would expect to get a result of 1 with a remainder of three. However, since we are dividing two integers, all that C# can return is an integer, so you get the following result instead:
If you divide an integer by a floating-point number, you always receive a floating-point number:
The result of this code is:
Finally, you can assign a value to a variable by dividing two numbers and assigning their value to a variable:
This results in the following output:
The Modulo % Operator in C#
If you do want to retrieve the remainder from a division, you are in luck, because C# has a built-in math operator for just such a function: the modulo operator – or % operator . Note that using this mathematical operator only returns the remainder from the division and nothing more. Here is how you use the modulo operator in C#:
Since 7 / 4 equals 1 with a remainder of 3 , using the modulo will result in the output:
Remember – only the remainder is returned when using the modulo operator.
Of course, we can use modulo to create a variable as well:
Other Assignment Operators in C#
In addition to the basic or normal assignment operator we discussed at the beginning of this tutorial, C# also offers a slew of other assignment operators. We do not have space left in this article to cover all of them, so we will have to follow up with another article to show you the rest of them. However, we can show you a few here, and give you a sneak peek of the others. Note that not all assignment operators are mathematical in nature, which is another reason we will not review them here. Some belong to Bitwise and Shift operators.
The remaining C# assignment operators include:
- = operator A regular assignment operator.
- += Addition assignment operator
- -= Subtraction assignment operator
- *= Multiplication assignment operator
- /= Division assignment operator
- %= Modulo assignment operator
Run the following code to see each of these C# assignment operators in action:
The += operator works by basically saying x = x +5 in this example. For instance, we started off by assigning additionAssignmentExample the value of 0 . We then use the += assignment operator to add assign the value of additionAssignmentExample to itself, plus add 5 to it.
The rest of the assignment operators work in a similar fashion, only they subtract , multiply , divide , or return the modulo of the number to the right of the = sign.
More by Author
Codeguru and vbforums developer forums and community, benefits of programming in c++, .net 6 features – part 2, tips for writing clean c# code, news & trends, c# versus c, different types of jit compilers in .net, get the free newsletter.
Subscribe to Developer Insider for top news, trends & analysis
Middleware in ASP.NET Core
CodeGuru covers topics related to Microsoft-related software development, mobile development, database management, and web application programming. In addition to tutorials and how-tos that teach programmers how to code in Microsoft-related languages and frameworks like C# and .Net, we also publish articles on software development tools, the latest in developer news, and advice for project managers. Cloud services such as Microsoft Azure and database options including SQL Server and MSSQL are also frequently covered.
Advertisers
Advertise with TechnologyAdvice on CodeGuru and our other developer-focused platforms.
- Privacy Policy
- California – Do Not Sell My Information
Property of TechnologyAdvice. © 2023 TechnologyAdvice. All Rights Reserved Advertiser Disclosure: Some of the products that appear on this site are from companies from which TechnologyAdvice receives compensation. This compensation may impact how and where products appear on this site including, for example, the order in which they appear. TechnologyAdvice does not include all companies or all types of products available in the marketplace.
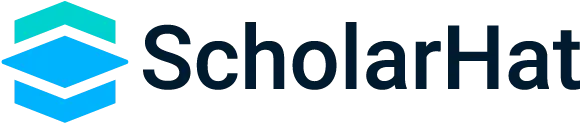
01 Career Opportunities
- Top 50 C# Interview Questions and Answers To Get Hired
02 Beginner
- C# Developer Roadmap
- New features added to C# 5.0
- Introduction to C Sharp
- What is C#: C# for Beginners
- Keywords in C#: Types of Keywords with Examples
- Identifiers in C# - A Beginner's Guide ( With Examples )
- Variables in C#: Types of Variables with Examples
- Scope of Variables in C# : Class Level, Method Level and Block Level Scope
- Data Types in C# with Examples: Value and Reference Data Type
Operators in C#: Arithmetic, Comparison, Logical and More...
- C# Best Practices for Writing Clean and Efficient Code
- Conditional Statements in C#: if , if..else, Nested if, if-else-if
- Switch Statement in C#: Difference between if-else and Switch
- Loops in C#: For, While, Do..While Loops
- Jump Statement in C#: Break, Continue, Goto, Return and Throw
- Method in C#: Learn How to Use Methods in C#
- Method Parameters in C#: Types Explained with Examples
- Arrays in C#: Create, Declare and Initialize the Arrays
03 Intermediate
- Partial Methods in C Sharp
- Difference between int, Int16, Int32 and Int64
- C Sharp Delegates and Plug-in Methods with Delegates
- Difference between c sharp generics and Collections with example
- C Sharp Extension method
- Difference between ref and out parameters
- Difference Between C# Const and ReadOnly and Static
- C Sharp Anonymous Method
- Partial Class, Interface or Struct in C Sharp with example
- Safe Type Casting with IS and AS Operator
- Understanding Type Casting or Type Conversion in C#
- Differences between Object, Var and Dynamic type
- Understanding Delegates in C#
- Properties in C#
- C Sharp Var data type and Anonymous Type: An Overview
- Understanding virtual, override and new keyword in C#
- C Sharp Lambda Expression
- Call By Value and Call by Reference in C#
- Types of Arrays in C#: Single-dimensional, Multi-dimensional and Jagged Array
- Access Modifiers in C# with Program Examples
04 Advanced
- Understanding Collections and Collections Interfaces
- C Sharp Generic delegates Func, Action and Predicate with anonymous method
- Top 20 C# Features Every .NET Developer Must Know in 2024
- Understanding C# Interface
- Errors and Exceptions Handling in C#
- C# Class Abstraction
- Method Overloading and Method Overriding in C#
- Objects and Classes in C#: Examples and Differences
- C# Constructor
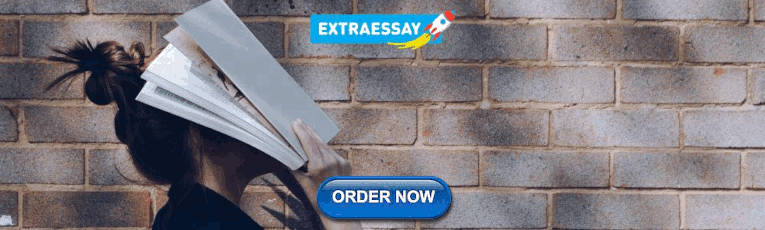
05 Questions
- OOPs Interview Questions and Answers in C#
06 Training Programs
- C# Programming Course
- Full-Stack .NET Developer Certification Training
- .NET Certification Training
- Data Structures and Algorithms Training
- Operators In C#: Arithmet..
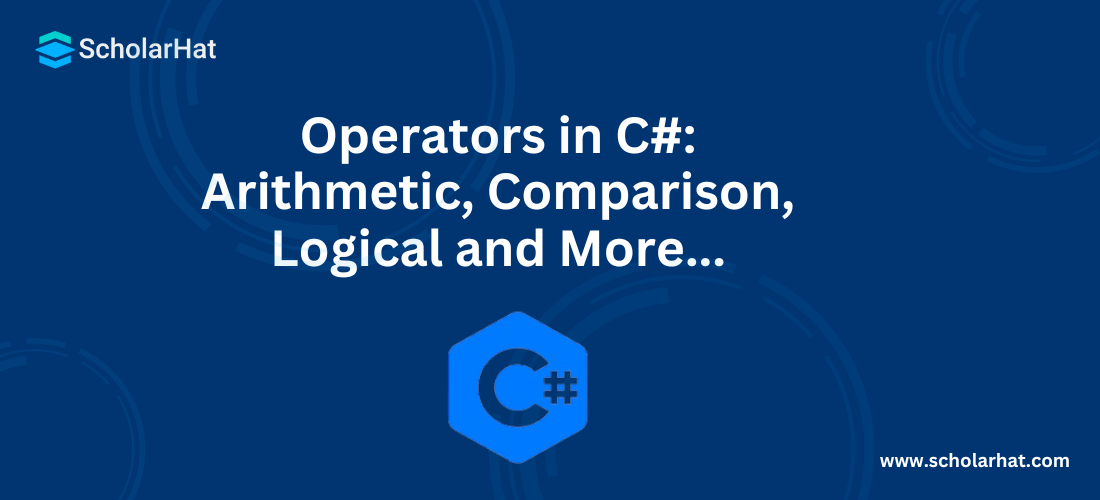
C# Programming For Beginners Free Course
Operators in c#: an overview.
Operators play a crucial role in any Programming Language, including C#. They allow us to perform various operations on data types, making our code more efficient and concise. In this article, we will explore the different types of Operators in C# and understand how they can enhance our coding efficiency.
Different Types of Operators in C# *{padding:0;margin:0;overflow:hidden}html,body{height:100%}img,span{position:absolute;width:100%;top:0;bottom:0;margin:auto}span{height:1.5em;text-align:center;font:48px/1.5 sans-serif;color:white;text-shadow:0 0 0.5em black} ▶ " frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen="">
C# provides a wide range of operators that cater to different requirements. Let's take a look at the different types of operators in C#:
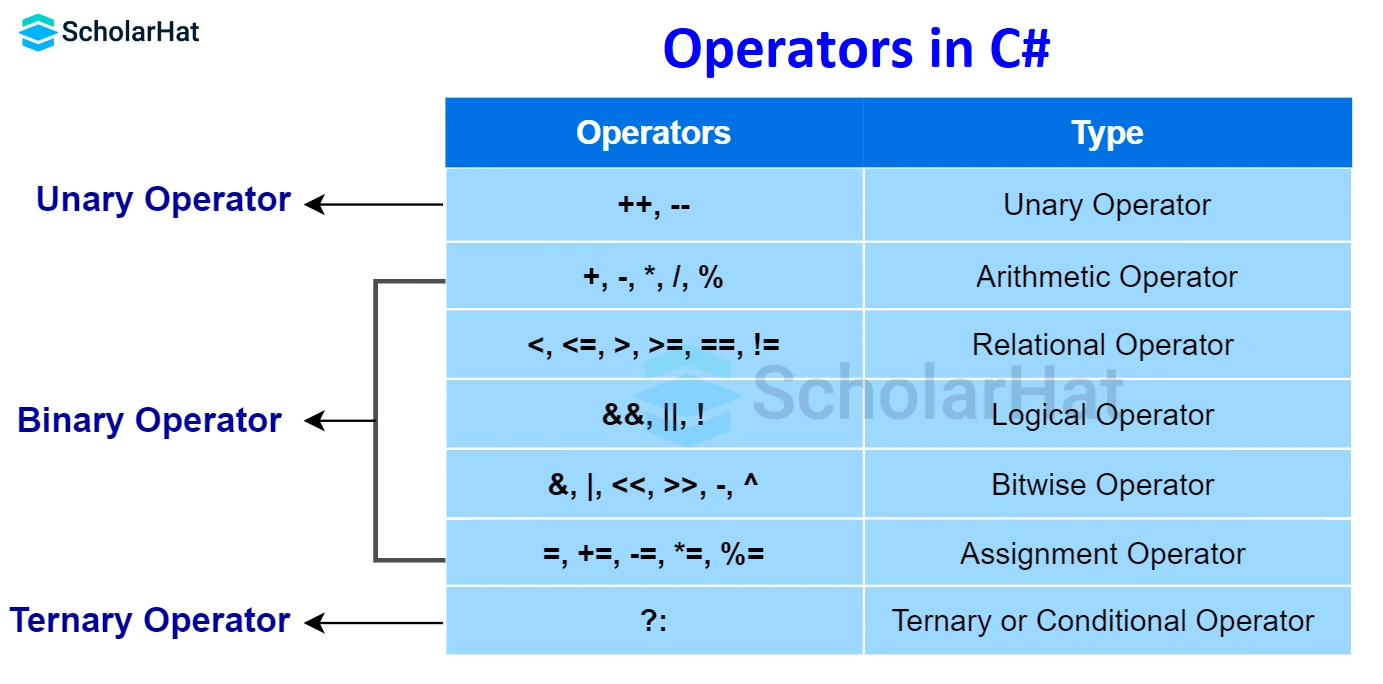
Read More - C# Interview Questions For Freshers
Arithmetic Operators
Arithmetic operators are used to perform mathematical calculations in C#. They include addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). These operators allow us to manipulate numerical values and perform calculations with ease. For example, if we want to add two numbers together, we can simply use the addition operator (+).
Arithmetic Operators Example
Using system; namespace arithmetic { class dnt { static void main (string[] args) { int result; int x = 10 , y = 5 ; result = (x + y); console. writeline ( "addition operator: " + result); result = (x - y); console. writeline ( "subtraction operator: " + result); result = (x * y); console. writeline ( "multiplication operator: " + result); result = (x / y); console. writeline ( "division operator: " + result); result = (x % y); console. writeline ( "modulo operator: " + result); } } } run code >>, explanation.
This C# code in the C# Compiler defines a console application that performs basic arithmetic operations. It initializes two integers (x=10 and y=5), then calculates and prints the results of addition, subtraction, multiplication, division, and modulo operations between these two numbers using appropriate operators.
Read More - C# Interview Questions And Answers
Assignment Operators
Assignment operators are used to assign values to variables in C#. They include the simple assignment operator (=), as well as compound assignment operators such as +=, -=, *=, and /=. These operators not only assign values but also perform an operation simultaneously. For instance, the += operator adds the right-hand operand to the left-hand operand and assigns the result to the left-hand operand.
Assignment Operators Example
This C# code demonstrates various assignment operators and their usage. It initializes an integer variable "x" and then performs operations like addition, subtraction, multiplication, division, modulo, left shift, right shift, bitwise AND, bitwise XOR, and bitwise OR using their corresponding assignment operators, modifying and printing the value of "x" at each step.
Comparison Operators
Comparison operators are used to compare values in C#. They include == (equality), != (inequality), > (greater than), < (less than), >= (greater than or equal to), and <= (less than or equal to). These operators return a Boolean value (true or false) based on the comparison result. For example, if we want to check if two numbers are equal, we can use the equality operator (==).
Comparison Operators: Example
Using system; class program { static void main ( string [] args ) { int num1 = 10 ; int num2 = 5 ; // equal to bool isequal = num1 == num2; console.writeline( $" {num1} == {num2} : {isequal} " ); // not equal to bool isnotequal = num1 = num2; console.writeline( $" {num1} = {num2} : {isnotequal} " ); // greater than bool isgreater = num1 > num2; console.writeline( $" {num1} > {num2} : {isgreater} " ); // less than bool isless = num1 < num2; console.writeline( $" {num1} < {num2} : {isless} " ); // greater than or equal to bool isgreaterorequal = num1 >= num2; console.writeline( $" {num1} >= {num2} : {isgreaterorequal} " ); // less than or equal to bool islessorequal = num1 <= num2; console.writeline( $" {num1} <= {num2} : {islessorequal} " ); } } run code >>.
This C# program compares two integer variables, num1 and num2, using relational operators. It checks if num1 is equal to num2, not equal, greater than, less than, greater than or equal to, and less than or equal to num2. The results of these comparisons are printed on the console.
Logical Operators
Logical operators are used to perform logical operations in C#. They include && (logical AND), || (logical OR), and ! (logical NOT). These operators are commonly used in conditional statements and loops. They allow us to combine multiple conditions and control the flow of our program based on the result. For instance, if we want to check if both condition A and condition B are true, we can use the logical AND operator (&&).
Logical Operators: Example
This C# code in the C# Editor demonstrates the usage of logical operators. It initializes two boolean variables, 'a' and 'b', and then computes and prints the results of logical AND, OR, and NOT operations between these variables. The code shows how these operators combine and negate boolean values to produce logical results.
Bitwise Operators
Bitwise operators are used to perform operations at the bit level in C#. They include &, |, ^, ~, <<, and >>. These operators manipulate individual bits of an operand, allowing us to perform operations such as bitwise AND, bitwise OR, bitwise XOR, bitwise complement, left shift, and right shift. Bitwise operators are particularly useful in scenarios where we need to work with binary data or perform low-level operations.
Unary Operators
Unary operators are used to perform operations on a single operand in C#. They include ++ (increment), -- (decrement), + (positive), - (negative), ! (logical NOT), and ~ (bitwise complement). These operators allow us to modify the value of a variable or change its state based on specific conditions. For example, the increment operator (++) increases the value of a variable by 1.
Unary Operators: Example
This C# code illustrates the post-increment (a++) and post-decrement (a--) operators, as well as the pre-increment (++a) and pre-decrement (--a) operators. It initializes an integer 'a', performs these operations, and prints 'a' and the result of each operation to demonstrate how these operators affect the variable's value.
Ternary Operators
Ternary operators are unique to C# and allow us to write concise conditional expressions. The ternary operator (?:) takes three operands: a condition, a true expression, and a false expression. It evaluates the condition and returns the true expression if the condition is true, otherwise, it returns the false expression. Ternary operators are handy when we need to assign a value to a variable based on a condition in a single line of code.
Ternary Operators: Example
This C# program uses ternary operators to perform conditional operations in a concise way. It checks if 'number' is even or odd, finds the maximum of 'a' and 'b', and determines if a person can vote based on their 'age'. The results are then printed to the console. Ternary operators provide a compact way to write conditional expressions with a true/false outcome.
Precedence of Operators in C#
Operators in C# have different levels of precedence, which determines the order in which they are evaluated. It is essential to understand the precedence rules to avoid unexpected results in our code. For example, multiplication (*) has higher precedence than addition (+), so an expression like 2 + 3 * 4 will evaluate to 14, not 20. By understanding the precedence of operators, we can write code that accurately reflects our intended calculations.
Here is the Precedence of Operators in C#
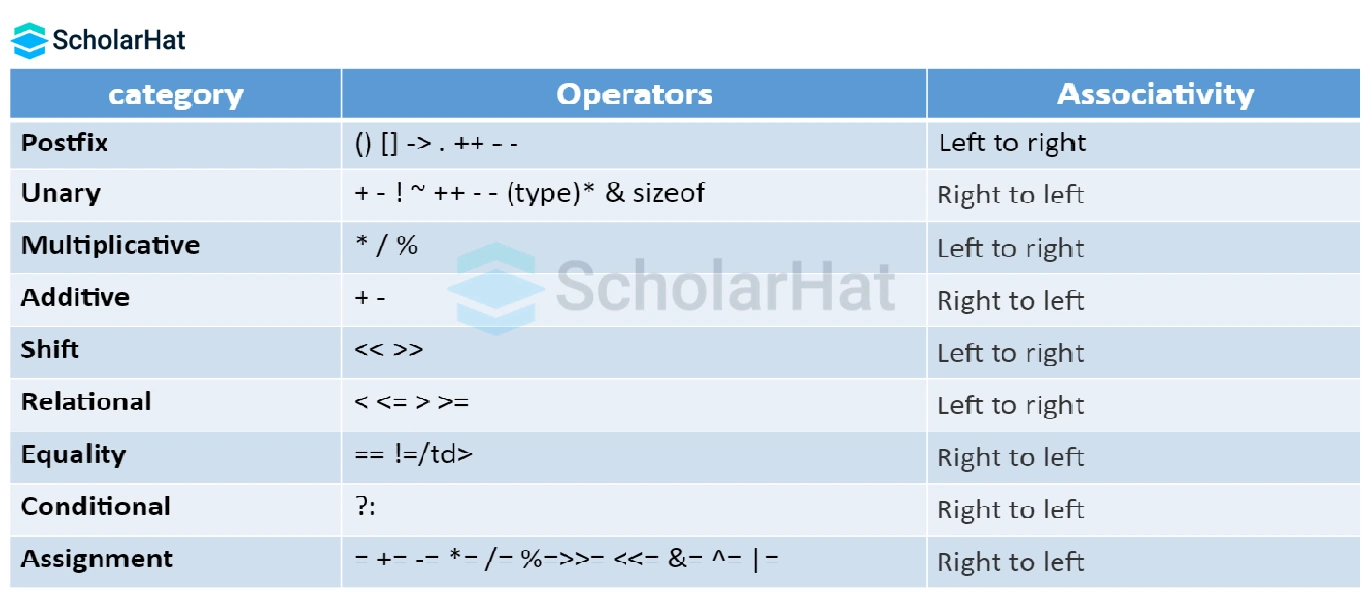
In conclusion, operators are a fundamental aspect of C# programming. They allow us to perform various operations on various data types, enhancing our coding efficiency. By understanding the different types of operators in C#, their functionality, and the precedence rules, we can write code that is concise, efficient, and easy to understand. So, next time you write code in C#, remember to leverage the power of operators and take your coding efficiency to new heights!
Resources for further learning and practice
Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/csharp-certification-training
There are numerous online C# tutorials and courses available that specifically focus on C# language.
Take our free csharp skill challenge to evaluate your skill
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.
GET CHALLENGE
About Author
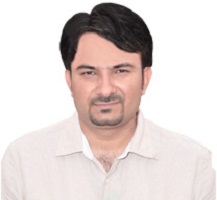
- 22+ Video Courses
- 750+ Hands-On Labs
- 300+ Quick Notes
- 55+ Skill Tests
- 45+ Interview Q&A Courses
- 10+ Real-world Projects
- Career Coaching Sessions
- Email Support
Upcoming Master Classes
We use cookies to make interactions with our websites and services easy and meaningful. Please read our Privacy Policy for more details.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
addition-assignment-operator.md
Latest commit, file metadata and controls, += operator (c# reference).
The addition assignment operator.
An expression using the += assignment operator, such as
is equivalent to
except that x is only evaluated once. The meaning of the + operator depends on the types of x and y (addition for numeric operands, concatenation for string operands, and so forth).
The += operator cannot be overloaded directly, but user-defined types can overload the + operator (see operator ).
The += operator is also used to specify a method that will be called in response to an event; such methods are called event handlers. The use of the += operator in this context is referred to as subscribing to an event . For more information, see How to: Subscribe to and Unsubscribe from Events . and Delegates .
[!code-cs csRefOperators#35 ]
C# Reference C# Programming Guide C# Operators

C# Operators
- What is Operators in C#?
- Arithmetic operators (+, -, *, /, %)
- Comparison operators (==, !=, <, >, <=, >=)
- Logical operators (&&, ||, !)
- Assignment operators (=, +=, -=, *=, /=, %=)
What is Operator?
Operators in C# are symbols that perform operations on operands.
They are used to manipulate and perform calculations on variables and values.
Arithmetic Operators:
- + (Addition): Adds two operands.
- - (Subtraction): Subtracts the second operand from the first.
- * (Multiplication): Multiplies two operands.
- / (Division): Divides the first operand by the second.
- % (Modulus): Returns the remainder of the division of the first operand by the second.
Comparison Operators:
- == (Equality): Returns true if the operands are equal; otherwise, false.
- != (Inequality): Returns true if the operands are not equal; otherwise, false.
- < (Less than): Returns true if the first operand is less than the second; otherwise, false.
- > (Greater than): Returns true if the first operand is greater than the second; otherwise, false.
- <= (Less than or equal to): Returns true if the first operand is less than or equal to the second; otherwise, false.
- >= (Greater than or equal to): Returns true if the first operand is greater than or equal to the second; otherwise, false.
Logical Operators:
- && (Logical AND): Returns true if both operands are true; otherwise, false.
- || (Logical OR): Returns true if either operand is true; otherwise, false.
- ! (Logical NOT): Returns the opposite of the operand's value; if the operand is true, ! returns false, and vice versa.
Assignment Operators:
- = (Assignment): Interchanges the value for right operant to left operand.
- += (Addition assignment): Performs the operation of addition and the obtained value to the left operand is kept and the right operand is skipped.
- -= (Subtraction assignment): Sums up the value of the left operand and the right operand and returns their product to the left operand.
- *= (Multiplication assignment): More to the left, multiplies operands by the value from the right side. The output is assigned on the opposite (left) side.
- /= (Division assignment): Splits the value on the left by an occurrence of the value on the right and assigns the outcome to the left operand.
- %= (Modulus assignment): Performs a modulo operation that is the modulus of the left operand divided by the value of the right operand, and returns it to the left operand.
- Certified ScrumMaster (CSM) Certification
- Certified Scrum Product Owner (CSPO) Certification
- Leading SAFe 6.0 Certification
- Professional Scrum Master-Advanced™ (PSM-A) Training
- SAFe 6.0 Scrum Master (SSM) Certification
- Implementing SAFe 6.0 (SPC) Certification
- SAFe 6.0 Release Train Engineer (RTE) Certification
- SAFe 6.0 Product Owner Product Manager (POPM) Certification
- ICP-ACC Certification
- Agile Master's Program
- Agile Excellence Master's Program
- Kanban Management Professional (KMP I: Kanban System Design) Certification
- Professional Scrum Product Owner I (PSPO I) Training
- View All Courses
Accreditation Bodies
- Project Management Professional (PMP) Certification
- PRINCE2 Certification
- PRINCE2 Foundation Certification
- PRINCE2 Practitioner Certification
- Change Management Training
- Project Management Techniques Training
- Certified Associate in Project Management (CAPM) Certification
- Program Management Professional (PgMP) Certification
- Portfolio Management Professional (PfMP) Certification
- Oracle Primavera P6 Certification
- Project Management Master's Program
- Microsoft Project Training
- Data Science Bootcamp
- Data Engineer Bootcamp
- Data Analyst Bootcamp
- AI Engineer Bootcamp
- Data Science with Python Certification
- Python for Data Science
- Machine Learning with Python
- Data Science with R
- Machine Learning with R
- Deep Learning Certification Training
- Natural Language Processing (NLP)
Enhance your career prospects with our Data Science Training
Embark on a Data Science career with our Data Analyst Bootcamp
Elevate your Data Science career with our AI Engineer Bootcamp
- DevOps Foundation Certification
- Docker with Kubernetes Training
- Certified Kubernetes Administrator (CKA) Certification
- Kubernetes Training
- Docker Training
- DevOps Training
- DevOps Leader Training
- Jenkins Training
- Openstack Training
- Ansible Certification
- Chef Training
- AWS Certified Solutions Architect - Associate
- Multi-Cloud Engineer Bootcamp
- AWS Cloud Practitioner Certification
- Developing on AWS
- AWS DevOps Certification
- Azure Solution Architect Certification
- Azure Fundamentals Certification
- Azure Administrator Certification
- Azure Data Engineer Certification
- Azure Devops Certification
- AWS Cloud Architect Master's Program
- AWS Certified SysOps Administrator Certification
- Azure Security Engineer Certification
- Azure AI Solution Certification Training
Supercharge your career with our Multi-Cloud Engineer Bootcamp
- Full-Stack Developer Bootcamp
- UI/UX Design Bootcamp
- Full-Stack [Java Stack] Bootcamp
- Software Engineer Bootcamp
- Software Engineer Bootcamp (with PMI)
- Front-End Development Bootcamp
- Back-End Development Bootcamp
- React Training
- Node JS Training
- Angular Training (Version 12)
- Javascript Training
- PHP and MySQL Training
Work on real-world projects, build practical developer skills
Hands-on, work experience-based learning
Start building in-demand tech skills
- ITIL 4 Foundation Certification
- ITIL Practitioner Certification
- ISO 14001 Foundation Certification
- ISO 20000 Certification
- ISO 27000 Foundation Certification
- ITIL 4 Specialist: Create, Deliver and Support Training
- ITIL 4 Specialist: Drive Stakeholder Value Training
- ITIL 4 Strategist Direct, Plan and Improve Training
- FAAANG/MAANG Interview Preparation
- Python Certification Training
- Advanced Python Course
- R Programming Language Certification
- Advanced R Course
- Java Training
- Java Deep Dive
- Scala Training
- Advanced Scala
- C# Training
- Microsoft .Net Framework Training
- Tableau Certification
- Data Visualisation with Tableau Certification
- Microsoft Power BI Certification
- TIBCO Spotfire Training
- Data Visualisation with Qlikview Certification
- Sisense BI Certification
- Blockchain Professional Certification
- Blockchain Solutions Architect Certification
- Blockchain Security Engineer Certification
- Blockchain Quality Engineer Certification
- Blockchain 101 Certification
- Hadoop Administration Course
- Big Data and Hadoop Course
- Big Data Analytics Course
- Apache Spark and Scala Training
- Apache Storm Training
- Apache Kafka Training
- Comprehensive Pig Training
- Comprehensive Hive Training
- Android Development Course
- IOS Development Course
- React Native Course
- Ionic Training
- Xamarin Studio Training
- Xamarin Certification
- OpenGL Training
- NativeScript for Mobile App Development
- Selenium Certification Training
- ISTQB Foundation Certification
- ISTQB Advanced Level Security Tester Training
- ISTQB Advanced Level Test Manager Certification
- ISTQB Advanced Level Test Analyst Certification
- ISTQB Advanced Level Technical Test Analyst Certification
- Silk Test Workbench Training
- Automation Testing using TestComplete Training
- Cucumber Training
- Functional Testing Using Ranorex Training
- Teradata Certification Training
- Certified Business Analysis Professional (CBAP®)
- Entry Certificate in Business Analysis™ (ECBA™)
- Certification of Capability in Business Analysis™ (CCBA®)
- Business Case Writing Course
- Professional in Business Analysis (PMI-PBA) Certification
- Agile Business Analysis Certification
- Six Sigma Green Belt Certification
- Six Sigma Black Belt Certification
- Six Sigma Yellow Belt Certification
- CMMIV1.3 Training
- Cyber Security Bootcamp
- Certified Ethical Hacker (CEH v12) Certification
- Certified Information Systems Auditor (CISA) Certification
- Certified Information Security Manager (CISM) Certification
- Certified Information Systems Security Professional (CISSP) Certification
- Cybersecurity Master's Program
- Certified Cloud Security Professional (CCSP) Certification
- Certified Information Privacy Professional - Europe (CIPP-E) Certification
- Control Objectives for Information and Related Technology (COBIT5) Foundation
- Payment Card Industry Security Standards (PCI-DSS) Certification
- Introduction to Forensic
- Digital Marketing Course
- PPC Training
- Web Analytics Course
- Social Media Marketing Course
- Content Marketing Course
- E-Mail Marketing Course
- Display Advertizing Course
- Conversion Optimization Course
- Mobile Marketing Course
- Introduction to the European Union General Data Protection Regulation
- Financial Risk Management (FRM) Level 1 Certification
- Financial Risk Management (FRM) Level 2 Certification
- Risk Management and Internal Controls Training
- Data Protection-Associate
- Credit Risk Management
- Budget Analysis and Forecasting
- International Financial Reporting Standards (IFRS) for SMEs
- Diploma In International Financial Reporting
- Certificate in International Financial Reporting
- Corporate Governance
- Finance for Non-Finance Managers
- Financial Modeling with Excel
- Auditing and Assurance
- MySQL Course
- Redis Certification
- MongoDB Developer Course
- Postgresql Training
- Neo4j Certification
- Mariadb Course
- Hbase Training
- MongoDB Administrator Course
- Conflict Management Training
- Communication Course
- International Certificate In Advanced Leadership Skills
- Soft Skills Training
- Soft Skills for Corporate Career Growth
- Soft Skills Leadership Training
- Building Team Trust Workshop
- CompTIA A+ Certification
- CompTIA Cloud Essentials Certification
- CompTIA Cloud+ Certification
- CompTIA Mobility+ Certification
- CompTIA Network+ Certification
- CompTIA Security+ Certification
- CompTIA Server+ Certification
- CompTIA Project+ Certification
- Master of Business Administration from Golden Gate University Training
- MBA from Deakin Business School with Multiple Specializations
- Master of Business Administration from Jindal Global Business School Training
- Master of Business Administration from upGrad Training
- MS Excel 2010
- Advanced Excel 2013
- Certified Supply Chain Professional
- Software Estimation and Measurement Using IFPUG FPA
- Software Size Estimation and Measurement using IFPUG FPA & SNAP
- Leading and Delivering World Class Product Development Course
- Product Management and Product Marketing for Telecoms IT and Software
- Foundation Certificate in Marketing
- Flow Measurement and Custody Transfer Training Course
C# Tutorial
By knowledgehut ., 1. c# tutorial, 2. c# introduction, 3. java vs c#, 4. ides to run c# program, 5. set environment, 6. first c# program, 7. data types in c#, 8. variables and constants in c#, 9. operators in c#, 10. namespaces in c#, 11. decision making statements in c#, 12. loops in c#, 13. arrays in c#, 14. strings in c#, 15. hashset collection in c#, 16. stack collection in c#, 17. queue collection in c#, 18. linkedlist collection in c#, 19. dictionary collection in c#, 20. sortedlist collection in c#, 21. bitarray collection in c#, 22. arraylist collection in c#, 23. methods in c#, 24. anonymous methods in c#, 25. classes and objects in c#, 26. constructors in c#, 27. static keyword in c#, 28. inheritance in c#, 29. interfaces in c#, 30. abstraction in c#, 31. delegates in c#, 32. structures in c#, 33. preprocessor directives in c#, 34. type conversion in c#, 35. exception handling in c#, 36. polymorphism in c#, 37. nullables in c#, 38. reflection in c#, 39. regular expressions in c#, 40. multithreading in c#, 41. indexers in c#, 42. file handling in c#, 43. attributes in c#, 44. generics in c#, 45. events in c#, 46. synchronization in c#, 47. serialization in c#, 48. properties in c#, 49. enum in c#, 50. unsafe code in c#, operators in c#.
Operators are used to perform mathematical or logical manipulations in a program. There are many types of built-in operators in C#. Some of these are the following: Arithmetic Operators, Relational Operators, Logical Operators, Bitwise Operators, etc.
Details about all these operators are given as follows:
Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations such as addition, subtraction, multiplication, division etc.
A table that shows all the arithmetic operators in C# is as follows: Table: Arithmetic Operators in C#
A program that demonstrates the arithmetic operators in C# is as follows:
Source Code: Program to implement arithmetic operators in C#
The output of the above program is as follows:
Relational Operators
Relational Operators are used for comparison purposes. This includes finding if a variable is greater than another variable, lesser than another variable etc.
A table that shows all the relational operators in C# is as follows: Table: Relational Operators in C#
A program that demonstrates the relational operators in C# is as follows: Source Code: Program to implement relational operators in C#
Logical Operators
The logical operators are used to perform specific logical operations in c#. There are three logical operators namely, logical AND, logical OR and logical NOT.
A table that shows all the logical operators in C# is as follows: Table: Logical Operators in C#
A program that demonstrates the logical operators in C# is as follows:
Source Code: Program to implement logical operators in C#
Bitwise Operators
The bitwise operators work at the level of bits and perform bit-by-bit operation. There are many bitwise operators such as bitwise AND, bitwise OR, bitwise XOR, bitwise NOT etc.
A table that shows all the bitwise operators in C# is as follows:
Table: Bitwise Operators in C#
A program that demonstrates the bitwise operators in C# is as follows: Source Code: Program to implement bitwise operators in C#
Assignment Operators
The assignment operators in C# are used to assign values to variables. There are various shorthand’s to assign values.
A table that shows all the assignment operators in C# is as follows: Table: Assignment Operators in C#
A program that demonstrates the assignment operators in C# is as follows:
Source Code: Program to implement assignment operators in C#
Miscellaneous Operators
There are many miscellaneous operators in C# such as sizeof(), & (address specifier),* (pointer dereference), ?: (conditional expression) etc.
A table that shows all the miscellaneous operators in C# is as follows: Table: Miscellaneous Operators in C#
A program that demonstrates different miscellaneous operators in C# is as follows:
Source Code: Program to implement miscellaneous operators in C#
Operator Precedence in C#
Operator precedence specifies the order in which operators will be evaluated. Some operators have more precedence than others have and are evaluated first. In the table given below, the operators with the higher precedence are given at the top and the precedence decreases further down the table. Table: Operator Precedence in C#
Leave a Reply
Your email address will not be published. Required fields are marked *
austin faith
Avery good write-up. Please let me know what are the types of C# libraries used for AI development.
kariya arti
very satisfied!!
jean-Francois Michaud
Good tutorial. Small question: Say, there is : enum numbers { one, two, three} and a string field_enum ="one" how would I from the variable field_enum have a response with value numbers.one so that it can be treated as an enum and not as a string. making a list from the enum, and loop into the list. is not elegant... and may not work is forced value on field is forced ( one = 100).
Hi Team Knowledge Hut, Thank you for such an informative post like this. I am completely new to this digital marketing field and do not have much idea about this, but your post has become a supportive pillar for me. After reading the blog I would expect to read more about the topic. I wish to get connected with you always to have updates on these sorts of ideas. Regards, Kshitiz
The reason abstraction can be used with this example is because, the triangle, circle. Square etc can be defined as a shape, for example.....shape c = new circle(5,0)...the abstract object c now points at the circle class. Thus hiding implementation
Suggested Tutorials
Swift tutorial.
Introduction to Swift Tutorial
R Programming Tutorial
R Programming
Python Tutorial
Python Tutorial
Get a 1:1 Mentorship call with our Career Advisor
Your Message (Optional)
Subscribe to our newsletter..
- Login Forgot Password
Don't Miss Out on Exclusive Discounts on Courses!
Future-proof your career with the latest in-demand courses.
- Get Job-Ready Digital Skills
- Experience Outcome-Based Immersive Learning
- Get Trained by a Stellar Pool of Industry Experts
- Best-In-Class Industry-Vetted Curriculum
By tapping submit, you agree to KnowledgeHut Privacy Policy and Terms & Conditions
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
?? and ??= operators - the null-coalescing operators
- 9 contributors
The null-coalescing operator ?? returns the value of its left-hand operand if it isn't null ; otherwise, it evaluates the right-hand operand and returns its result. The ?? operator doesn't evaluate its right-hand operand if the left-hand operand evaluates to non-null. The null-coalescing assignment operator ??= assigns the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . The ??= operator doesn't evaluate its right-hand operand if the left-hand operand evaluates to non-null.
The left-hand operand of the ??= operator must be a variable, a property , or an indexer element.
The type of the left-hand operand of the ?? and ??= operators can't be a non-nullable value type. In particular, you can use the null-coalescing operators with unconstrained type parameters:
The null-coalescing operators are right-associative. That is, expressions of the form
are evaluated as
The ?? and ??= operators can be useful in the following scenarios:
In expressions with the null-conditional operators ?. and ?[] , you can use the ?? operator to provide an alternative expression to evaluate in case the result of the expression with null-conditional operations is null :
When you work with nullable value types and need to provide a value of an underlying value type, use the ?? operator to specify the value to provide in case a nullable type value is null :
Use the Nullable<T>.GetValueOrDefault() method if the value to be used when a nullable type value is null should be the default value of the underlying value type.
You can use a throw expression as the right-hand operand of the ?? operator to make the argument-checking code more concise:
The preceding example also demonstrates how to use expression-bodied members to define a property.
You can use the ??= operator to replace the code of the form
with the following code:
Operator overloadability
The operators ?? and ??= can't be overloaded.
C# language specification
For more information about the ?? operator, see The null coalescing operator section of the C# language specification .
For more information about the ??= operator, see the feature proposal note .
- Null check can be simplified (IDE0029, IDE0030, and IDE0270)
- C# operators and expressions
- ?. and ?[] operators
- ?: operator
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
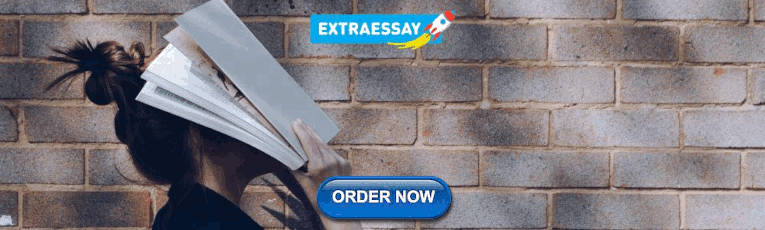
IMAGES
VIDEO
COMMENTS
In this article. The + and += operators are supported by the built-in integral and floating-point numeric types, the string type, and delegate types.. For information about the arithmetic + operator, see the Unary plus and minus operators and Addition operator + sections of the Arithmetic operators article.. String concatenation. When one or both operands are of type string, the + operator ...
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
For more information, see the -and -= operators article. Compound assignment. For a binary operator op, a compound assignment expression of the form. x op= y is equivalent to. x = x op y except that x is only evaluated once. The following example demonstrates the usage of compound assignment with arithmetic operators:
BtnClickHandler2. If you want specific info about += operator, MSDN says: The += operator is also used to specify a method that will be called in response to an event; such methods are called event handlers. The use of the += operator in this context is referred to as subscribing to an event. For more info look at:
Simple assignment operator, Assigns values from right side operands to left side operand. C = A + B assigns value of A + B into C. +=. Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand. C += A is equivalent to C = C + A.
Add Two Numbers C# Examples C# Examples C# Compiler C# Exercises C# Quiz C# Server C# Certificate. C# Assignment Operators Previous Next Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator (=) ...
For example, in 2+3, + is an operator that is used to carry out addition operation, while 2 and 3 are operands. Operators are used to manipulate variables and values in a program. C# supports a number of operators that are classified based on the type of operations they perform.
In c#, Assignment Operators are useful to assign a new value to the operand, and these operators will work with only one operand. For example, we can declare and assign a value to the variable using the assignment operator ( =) like as shown below. int a; a = 10; If you observe the above sample, we defined a variable called " a " and ...
Assignment operators for assigning values to variables. Comparison operators for comparing two values. Logical operators for combining Boolean values. Bitwise operators for manipulating the bits of a number. Arithmetic Operators. C# has the following arithmetic operators: Addition, +, returns the sum of two numbers.
Other Assignment Operators in C#. In addition to the basic or normal assignment operator we discussed at the beginning of this tutorial, C# also offers a slew of other assignment operators. We do not have space left in this article to cover all of them, so we will have to follow up with another article to show you the rest of them ...
Explanation. This C# code demonstrates various assignment operators and their usage. It initializes an integer variable "x" and then performs operations like addition, subtraction, multiplication, division, modulo, left shift, right shift, bitwise AND, bitwise XOR, and bitwise OR using their corresponding assignment operators, modifying and printing the value of "x" at each step.
except that x is only evaluated once. The meaning of the + operator depends on the types of x and y (addition for numeric operands, concatenation for string operands, and so forth).. The += operator cannot be overloaded directly, but user-defined types can overload the + operator (see operator).. The += operator is also used to specify a method that will be called in response to an event; such ...
What is Operator? Operators in C# are symbols that perform operations on operands. They are used to manipulate and perform calculations on variables and values. ... (Addition assignment): Performs the operation of addition and the obtained value to the left operand is kept and the right operand is skipped.-= (Subtraction assignment): Sums up ...
A user-defined type can overload a predefined C# operator. That is, a type can provide the custom implementation of an operation in case one or both of the operands are of that type. The Overloadable operators section shows which C# operators can be overloaded. Use the operator keyword to declare an operator. An operator declaration must ...
There are various shorthand's to assign values.A table that shows all the assignment operators in C# is as follows:Table: Assignment Operators in C#Operator NameOperatorOperator DescriptionAssignment Operator=This operator assigns the values from the right side to the left side operand.Addition Assignment Operator+=This operator adds the ...
Notes for You:: Assignment Operators in C#.Assignment Operator ( = ):- is used to assign a value to a variable or a constant.Short Hand Assignment (SHA) Oper...
1. The Graph class would have a nodes property which should only be able to have nodes added or removed but not set as a whole property. While this is great for encapsulation, there are simpler and more obvious ways to implement it than overloading the += operator. Here is one example: class Graph.
Unlike C++, in C# you can't overload the assignment operator. I'm doing a custom Number class for arithmetic operations with very large numbers and I want it to have the look-and-feel of the built-in numerical types like int, decimal, etc. I've overloaded the arithmetic operators, but the assignment remains... Here's an example:
In expressions with the null-conditional operators ?. and ?[], you can use the ?? operator to provide an alternative expression to evaluate in case the result of the expression with null-conditional operations is null: