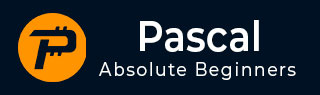
- Pascal Tutorial
- Pascal - Home
- Pascal - Overview
- Pascal - Environment Setup
- Pascal - Program Structure
- Pascal - Basic Syntax
- Pascal - Data Types
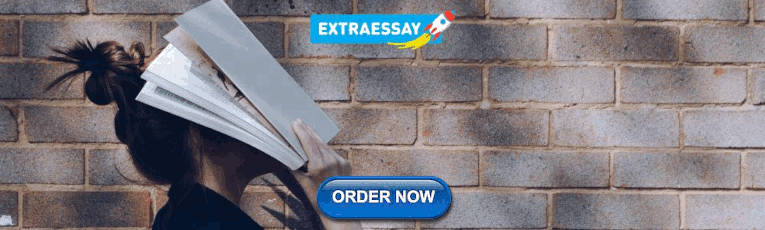
Pascal - Variable Types
- Pascal - Constants
- Pascal - Operators
- Pascal - Decision Making
- Pascal - Loops
- Pascal - Functions
- Pascal - Procedures
- Pascal - Variable Scope
- Pascal - Strings
- Pascal - Booleans
- Pascal - Arrays
- Pascal - Pointers
- Pascal - Records
- Pascal - Variants
- Pascal - Sets
- Pascal - File Handling
- Pascal - Memory
- Pascal - Units
- Pascal - Date & Time
- Pascal - Objects
- Pascal - Classes
- Pascal Useful Resources
- Pascal - Quick Guide
- Pascal - Useful Resources
- Pascal - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
A variable is nothing but a name given to a storage area that our programs can manipulate. Each variable in Pascal has a specific type, which determines the size and layout of the variable's memory; the range of values that can be stored within that memory; and the set of operations that can be applied to the variable.
The name of a variable can be composed of letters, digits, and the underscore character. It must begin with either a letter or an underscore. Pascal is not case-sensitive , so uppercase and lowercase letters mean same here. Based on the basic types explained in previous chapter, there will be following basic variable types −
Basic Variables in Pascal
Pascal programming language also allows defining various other types of variables, which we will cover in subsequent chapters like Pointer, Array, Records, Sets, and Files, etc. For this chapter, let us study only basic variable types.
Variable Declaration in Pascal
All variables must be declared before we use them in Pascal program. All variable declarations are followed by the var keyword. A declaration specifies a list of variables, followed by a colon (:) and the type. Syntax of variable declaration is −
Here, type must be a valid Pascal data type including character, integer, real, boolean, or any user-defined data type, etc., and variable_list may consist of one or more identifier names separated by commas. Some valid variable declarations are shown here −
In the previous tutorial, we have discussed that Pascal allows declaring a type. A type can be identified by a name or identifier. This type can be used to define variables of that type. For example,
Now, the types so defined can be used in variable declarations −
Please note the difference between type declaration and var declaration. Type declaration indicates the category or class of the types such as integer, real, etc., whereas the variable specification indicates the type of values a variable may take. You can compare type declaration in Pascal with typedef in C. Most importantly, the variable name refers to the memory location where the value of the variable is going to be stored. This is not so with the type declaration.
Variable Initialization in Pascal
Variables are assigned a value with a colon and the equal sign, followed by a constant expression. The general form of assigning a value is −
By default, variables in Pascal are not initialized with zero. They may contain rubbish values. So it is a better practice to initialize variables in a program. Variables can be initialized (assigned an initial value) in their declaration. The initialization is followed by the var keyword and the syntax of initialization is as follows −
Some examples are −
Let us look at an example, which makes use of various types of variables discussed so far −
When the above code is compiled and executed, it produces the following result −
Enumerated Variables
You have seen how to use simple variable types like integer, real and boolean. Now, let's see variables of enumerated type, which can be defined as −
When you have declared an enumerated type, you can declare variables of that type. For example,
The following example illustrates the concept −
Subrange Variables
Subrange variables are declared as −
Examples of subrange variables are −
The following program illustrates the concept −
Data Type Assignments and Compatibility
Table 4-1 data type assignment type of variable/parameter type of assignment-compatible expression real , single , shortreal real , single , shortreal , double , longreal , any integer type double , longreal real , single , shortreal , double , longreal , any integer type integer , integer16 , integer32 integer , integer16 , integer32 boolean boolean char char enumerated same enumerated type subrange base type of the subrange record record of the same type array array with the same type set set with compatible base type pointer pointer to an identical type, univ_ptr, string assignments, fixed- and variable-length strings, table 4-2 fixed- and variable-length string assignments type of string type of assignment-compatible expression array of char varying string, constant string, and array of char if the arrays have the same length varying varying string, constant string, array of char , and char, null strings, table 4-3 null string assignments assignment description varying := ''; the compiler assigns the null string to the variable-length string. the length of the variable-length string equals zero. array of char := ''; the compiler assigns a string of blanks to the character array. the length of the resulting string is the number of elements in the source character array. char := ''; it is illegal to assign a null string to a char variable. use chr(0) instead. string concatenation in a string concatenation expression such as: s := 'hello' + '' + s; '' is treated as the additive identity (as nothing)., string constants, arithmetic operators, table 4-4 operator operation operands result + addition integer or real integer or real - subtraction integer or real integer or real * multiplication integer or real integer or real / division integer or real real div truncated division integer integer mod modulo integer integer arithmetic operators, the mod operator, bit operators, table 4-5 bit operators operator operation operands result ~ bitwise not integer integer & bitwise and integer integer | bitwise or integer integer bitwise or (same as | ) integer integer, boolean operators, table 4-6 boolean operators operator operation operands result and conjunction boolean boolean and then similar to boolean and boolean boolean not negation boolean boolean or disjunction boolean boolean or else similar to boolean or boolean boolean, the and then operator.
Note - You cannot insert comments between the and and the then operators.
The or else Operator
Note - You cannot insert comments between the or and the else operators.
Set Operators
Table 4-7 set operators operator operation operands result + set union any set type same as operands - set difference any set type same as operands * set intersection any set type same as operands in member of a specified set 2nd arg:any set type 1st arg:base type of 2nd arg boolean, relational operators, table 4-8 relational operators operator operation operand results = equal any real, integer , boolean, char , record, array, set, or pointer type boolean <> not equal any real, integer, boolean, char, record, array, set, or pointer type boolean < less than any real, integer, boolean, char, string, or set type boolean <= less than or equal any real, integer, boolean, char, string, or set type boolean > greater than any real, integer, boolean, char, string, or set type boolean >= greater than or equal any real, integer, boolean, char, string, or set type boolean, relational operators on sets, the = and <> operators on records and arrays, string operators, precedence of operators, table 4-9 precedence of operators operators precedence ~, not , highest * , / , div , mod , and , & , . | , , + , - , or , . = , <> , < , <= , > , >= , in , . or else , and then lowest.
You are using an outdated browser. Upgrade your browser today or install Google Chrome Frame to better experience this site.
Learn X in Y minutes
Where x=pascal.
Get the code: learnpascal.pas
Pascal is an imperative and procedural programming language, which Niklaus Wirth designed in 1968–69 and published in 1970, as a small, efficient language intended to encourage good programming practices using structured programming and data structuring. It is named in honor of the French mathematician, philosopher and physicist Blaise Pascal. source : wikipedia
To compile and run a pascal program you could use a free pascal compiler. Download Here
Got a suggestion? A correction, perhaps? Open an Issue on the GitHub Repo, or make a pull request yourself!
Originally contributed by Ganesha Danu, and updated by 6 contributors .
Pascal Programming/Expressions and Branches
In this chapter you will learn
- to distinguish between statements and expressions, and
- how to program branches.
- 1.1 Classification
- 1.2 Semicolon
- 2.1 Using expressions
- 2.2 Linking expressions
- 2.3 Comparisons
- 2.4 Calculations
- 2.5 Operator precedence
- 3.1 Conditional statement
- 3.2 Alternative statement
- 3.3 Relevance
Statement [ edit | edit source ]
Before we get know “expressions”, let’s define “statements” more precisely, shall we: A statement tells the computer to change something. All statements in some way or other change the program state. Program state refers to a whole conglomerate of individual states, including but not limited to:
- the values variables have, or
- in general the program’s designated memory contents, but also
- (implicitly) which statement is currently processed.
The last metric is stored in an invisible variable, the program counter . The PC always points to the currently processed statement. Imagine pointing with your finger to one source code line (or, more precisely, statement): “Here we are!” After a statement has successfully been executed, the PC advances to the effect that it points to the next statement. [fn 1] The PC cannot be altered directly, but only implicitly . In this chapter we will learn how.
Classification [ edit | edit source ]
Statements can be categorized into two groups: Elementary and complex statements. Elementary statements are the minimal building blocks of high-level programming languages. In Pascal they are: [fn 2] [fn 3]
- Assignments ( := ), and
- Routine [fn 4] invocations (such as readLn ( x ) and writeLn ( 'Hi!' ) ).
“Complex” statements are:
- Sequences (surrounded by begin and end ),
- branches, and
Semicolon [ edit | edit source ]
Unlike many other programming languages, in Pascal the semicolon ; separates two statements. Lots of programming languages use some symbol to terminate a statement, e. g. the semicolon. Pascal, however, recognized that an extra symbol should not be part of a statement in order to make it an actual statement. The helloWorld program from the second chapter could be written without a semicolon after the writeLn ( … ) , because there is no following statement:
We, however, recommend you to put a semicolon there anyway, even though it is not required. Later in this chapter you will learn one place you most probably (that means not necessarily always) do not want to put a semicolon.
Although a semicolon does not terminate a statement , the program header, constant definitions, variable declarations and some other language constructs are terminated by this symbol. You cannot omit a semicolon at these locations.
Expressions [ edit | edit source ]
Expressions, in contrast to statements, do not change the program state. They are transient values that can be used as part of statements. Examples of expressions are:
- 'D’oh!' , or
- x (where x is the name of a previously declared variable).
Every expression has a type: When an expression is evaluated it results in a value of a certain data type. The expression 42 has the data type integer , 'D’oh!' is a “string type” and the expression merely consisting of a variable’s name, such as x , evaluates to the data type of that variable. Because the data type of an expression is so important, expressions are named after their type. The expression true is a Boolean expression , as is false .
Using expressions [ edit | edit source ]
Expressions appear at many places:
- In the assignment statement ( := ) you write an expression on the RHS . This expression has to have the data type of the variable on the LHS . [fn 5] An assignment makes the transient value of an expression “permanent” by storing it into the variable’s memory block.
- destination becomes output
- “first parameter” becomes 'Hi!'
- call the routine writeLn with the invisible “variables” destination and “first parameter”
- In a constant definition the RHS is also an expression, although – hence their name – it has to be constant . You could not use, for instance, a variable as part of that expression.
Linking expressions [ edit | edit source ]
The power of expressions lies in their capability to link with other expressions. This is done by using special symbols called operators . In the previous chapter we already saw one operator, the equals operator = . Now we can break up such an expression:
As you can you can see in the diagram, an expression can be part of a larger expression. The sub-expressions are linked using the operator symbol = . Sub-expressions that are linked via, or associated with an operator symbol are also called operands .
Comparisons [ edit | edit source ]
Linking expressions via an operand “creates” a new expression which has a data type on its own. While response and 'y' in the example above were both char -expressions, the overall data type of the whole expression is Boolean , because the linking operator is the equal comparison. An equal comparison yields a Boolean expression . Here is a table of relational operators which we can already use with our knowledge:
Using these symbols yield Boolean expressions. The value of the expression will be either true or false depending on the operator’s definition.
All those relational operators require operands on both sides to be of the same data type. [fn 6] Although we can say '$' = 1337 is wrong, that means it should evaluate to the value false , it is nevertheless illegal, because '$' is a char ‑expression and 1337 is an integer ‑expression. Pascal forbids you to compare things/objects that differ in their data type. So, I guess, y’can’t compare apples ’n’ oranges after all. (Note, a few conversion routines will allow you to do some comparisons that are not allowed directly , but by taking a detour. In the next chapter we will see some of them.)
Calculations [ edit | edit source ]
Expressions are also used for calculations, the machine you are using is not called “computer” for no reason. In Standard Pascal you can add, subtract, multiply and divide two numbers, i. e. integer ‑ and real ‑expressions and any combination thereof. The symbols that work for all combinations are:
The division operation has been omitted as it is tricky, and will be explained in a following chapter.
Note, unlike in mathematics, there is no invisible times assumed between two “operands”: You always need to write the “times”, meaning the asterisk * explicitly .
The operator symbols + and - can also appear with one number expression only. It then indicates the positive or negative sign, or – more formally – sign identity or sign inversion respectively.
Operator precedence [ edit | edit source ]
Just like in mathematics, operators have a certain “force” associated with them, in CS we call this operator precedence . You may recall from your primary or secondary education, school or homeschooling, the acronym PEMDAS: It is a mnemonic standing for the initial letters of
- parentheses
- multiplication / division
- addition / subtraction
giving us the correct order to evaluate an arithmetic expression in mathematics. Luckily, Pascal’s operator precedence is just the same, although – to be fair – technically not defined by the word “PEMDAS”. [fn 7]
As you might have guessed it, operator precedence can be overridden on a per-expression basis by using parentheses: In order to evaluate 5 * ( x + 7 ) , the sub-expression x + 7 is evaluated first and that value is then multiplied by 5 , even though multiplication is generally evaluated prior sums or differences.
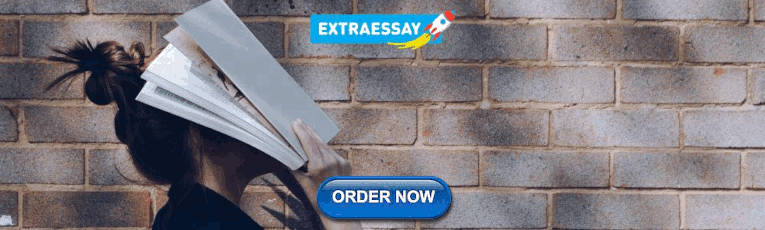
Branches [ edit | edit source ]
Branches are complex statements. Up to this point all programs we wrote were linear: They started at the top and the computer (ideally) executed them line-by-line until the final end . . Branches allow you to choose alternative paths, like at a T‑bone intersection: “Do I turn left or do I turn right?” The general tendency to process the program “downward” remains, but there is (in principle) a choice.
Conditional statement [ edit | edit source ]
Let’s review the program iceCream from the previous chapter. The conditional statement is highlighted:
Now we can say that response = 'y' is a Boolean expression. The words if and then are part of the language construct we call conditional statement . After then comes a statement, in this case a complex statement: begin … end is a sequence and considered to be one statement.
If you remember or can infer from the source code, the statements between begin … end , the writeLn ( 'Awesome!' ) is only executed if the expression response = 'y' evaluated to true . Otherwise, this is skipped as if there was nothing.
Due to this binary nature – yes / no, execute the code or skip it – the expression between if and then has to be a Boolean expression. You cannot write if 1 * 1 then … , since 1 * 1 is an integer -expression. The computer cannot decide based on an integer -expression, whether it shall take a route or not.
Alternative statement [ edit | edit source ]
Let’s expand the program iceCream by giving an alternative response if the user says not to like ice cream. We could do this with another if ‑statement, yet there is a smarter solution for this frequently occurring situation:
The highlighted alternative, the else ‑branch, will only be executed if the supplied Boolean expression evaluated to false . In either case, regardless whether the then ‑branch or the else ‑branch was taken, program execution resumes after the else ‑statement (in this after the end ; in the last line).
Relevance [ edit | edit source ]
Branches and (soon explained) loops are the only method of modifying the PC , “your finger” pointing to the currently executed statement, based on data, an expression, and thus a means of responding to user input. Without them, your programs would be static and do the same over and over again, so pretty boring. Utilizing branches and loops will make your program way more responsive to the given input.
Tasks [ edit | edit source ]
- ↑ This paragraph intentionally uses imprecise terminology to keep things simple. The PC is in fact a processor register (e. g. % eip , extended instruction pointer) and points to the following instruction (not current statement). See Subject: Assembly languages for more details.
- ↑ Jumps ( goto ) have been deliberately banned into the appendix , and are not covered here, yet goto is also an elementary statement.
- ↑ Exception extensions also define raise as an elementary statement.
- ↑ More correctly: procedure calls.
- ↑ Or, a “compatible” data type, e. g. an integer expression can be stored into a variable of the data type real , but not the other way round. As we progress we will learn more about “compatible” types.
- ↑ In the chapter on sets we will expand this statement.
- ↑ To read the technical definition, see § Expressions, subsection 1 “General” in the ISO standard 7185 .
- Book:Pascal Programming
Navigation menu
Once you have declared a variable, you can store values in it. This is called assignment .
To assign a value to a variable, follow this syntax:
Note that unlike other languages, whose assignment operator is just an equals sign, Pascal uses a colon followed by an equals sign, similarly to how it's done in most computer algebra systems.
The expression can either be a single value:
or it can be an arithmetic sequence:
The arithmetic operators in Pascal are:
div and mod only work on integers. / works on both reals and integers but will always yield a real answer. The other operations work on both reals and integers. When mixing integers and reals, the result will always be a real since data loss would result otherwise. This is why Pascal uses two different operations for division and integer division. 7 / 2 = 3.5 (real), but 7 div 2 = 3 (and 7 mod 2 = 1 since that's the remainder).
When the following block of statements executes,
some_real will have a value of 375.0.
Changing one data type to another is referred to as typecasting . Modern Pascal compilers support explicit typecasting in the manner of C, with a slightly different syntax. However, typecasting is usually used in low-level situations and in connection with object-oriented programming, and a beginning programming student will not need to use it. Here is information on typecasting from the GNU Pascal manual .
In Pascal, the minus sign can be used to make a value negative. The plus sign can also be used to make a value positive, but is typically left out since values default to positive.
Do not attempt to use two operators side by side, like in:
This may make perfect sense to you, since you're trying to multiply by negative-2. However, Pascal will be confused — it won't know whether to multiply or subtract. You can avoid this by using parentheses to clarify:
The computer follows an order of operations similar to the one that you follow when you do arithmetic. Multiplication and division ( * / div mod ) come before addition and subtraction ( + - ), and parentheses always take precedence. So, for example, the value of: 3.5*(2+3) will be 17.5 .
Pascal cannot perform standard arithmetic operations on Booleans. There is a special set of Boolean operations. Also, you should not perform arithmetic operations on characters.
Assign a name to a file
Declaration
Source position: systemh.inc line 1257
Description
Assign assigns a name to F , which can be any file type. This call doesn't open the file, it just assigns a name to a file variable, and marks the file as closed.
Note that the filename (including path) can be only 255 characters long.
Code Translation Project
Don't lose in a world of programming languages
Pascal - Assignment: :=
Description.
- var - value receiver
- expression - Expressions
The Borland Pascal procedure assign associates a file variable with an external entity (e. g. a device file or a file on disk). Assign only operates on the program’s internal management data and has otherwise no immediate observable effect.
- 2 application
In non-ISO modes the FPC needs files to be explicitly associated with external entities. This can be achieved with assign . The first parameter is a file variable, the second parameter specifies a path.
Note, you can run this program without any troubles as any user on any platform. Nonexistent path components (e. g. directories), missing privileges to eventually access the file or any path component, or unacceptable characters (usually chr ( 0 ) ) do not pose a problem to assign . It merely operates on internal management data.
application
- Assign is used before operating on any file variable. program assignApplication ( input , output , stdErr ) ; var executable : file of Byte ; begin assign ( executable , paramStr ( 0 )) ; fileMode := 0 ; { 0 = read-only; only relevant for `file of …` variables } reset ( executable ) ; writeLn ( paramStr ( 0 ) , ' has a size of ' , fileSize ( executable ) , ' Bytes.' ) ; end .
- Assign can be used to redirect standard files input / output . Our Hello, World program needs only a few changes as highlighted: program redirectDemo ( input , output , stdErr ) ; const blackhole = {$ifDef Linux} '/dev/null' + {$endIf} {$ifDef Windows} 'nul' + {$endIf} '' ; begin assign ( output , blackhole ) ; rewrite ( output ) ; writeLn ( 'Hello world!' ) ; end .
- Regardless of the utilized string data type , assign can only process paths of up to 255 Bytes . This limitation comes from the internally used fileRec / textRec . Relative paths in conjunction with working directories cannot overcome this. Cf. FPC issue 35185 .
- Since assign only operates on internal management data, specifying illegal paths is accepted. It is even possible to supply an empty string as a path. The specific behavior on a file variable bearing an empty file name depends on the used I/O routine.
- If {$modeSwitch objPas+} (automatically set via {$mode objFPC} or {$mode Delphi} ), the procedure assignFile is available, too. It performs the very same task.
- In Extended Pascal a combination of bindingType and bind are usually used to achieve linking a file variable with an external entity.
- File Handling
Navigation menu
Page actions.
- View source
- In other languages
Personal tools
- Create account
- Documentation
- Recent changes
- Random page
- What links here
- Related changes
- Special pages
- Printable version
- Permanent link
- Page information
- This page was last edited on 3 September 2022, at 18:05.
- Content is available under unless otherwise noted.
- Privacy policy
- About Free Pascal wiki
- Disclaimers
# Programming Assignment 1
A1: live variable analysis and iterative solver, # 1 assignment objectives.
- Implement a live variable analysis for Java.
- Implement a generic iterative solver, which will be used to solve the data-flow problem you defined, i.e., the live variable analysis.
In this first programming assignment, you will implement a live variable analysis and an iterative solver on top of Tai-e. We have built a generic data-flow analysis framework in Tai-e, which provides analysis interfaces, necessary program information (e.g., control-flow graph), commonly used data structures (e.g., representation of data facts), etc., and thus you could easily write new data-flow analyses on top of it. In this assignment, you will implement the key parts of live variable analysis and iterative solver.
In this document (and the documents for other assignments), we only briefly explain some necessary APIs that are related to the assignments, and to learn how other related APIs work, or to get a more comprehensive understanding about the framework, you need to read the source code and the corresponding comments we provided. So please reserve some time for the source code reading and understanding, and this will help improve your ability for rapidly getting familiar with complicated programs.
Now, we suggest that you first setup the Tai-e project for Assignment 1 (in A1/tai-e/ of the assignment repo) by following Setup Tai-e Assignments .
# 2 Implementing Live Variable Analysis
# 2.1 tai-e classes you need to know.
To implement live variable analysis on Tai-e, you need to know the following classes.
pascal.taie.analysis.dataflow.analysis.DataflowAnalysis
This is the interface between concrete data-flow analyses (e.g., live variable analysis and reaching definition) and data-flow analysis solver. It has 7 APIs, and in this assignment, you only need to focus on the first 5 APIs, which correspond to the five key elements in a data-flow analysis ( page 297 of slides for Lecture 4 ), i.e., direction, boundary, initialization, meet operation and transfer function. All APIs of this class are commented in the source code, so that you could get familiar with them by reading the code and comments.
The APIs in this class will be invoked by the data-flow analysis solver you write. Note that in our assignments, the transfer function manipulates statements, not basic blocks, which makes it simpler.
pascal.taie.ir.exp.Exp
This is one of the two key interfaces in Tai-e’s IR (another one, Stmt , will be introduced later), which represents all expressions in the program. This class has many subclasses, corresponding to various expressions. Here we show a tiny part of its class hierarchy:
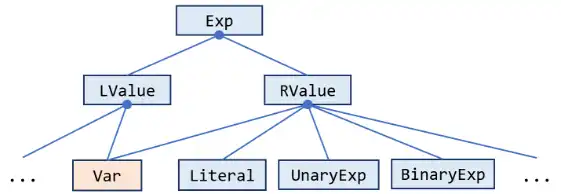
In Tai-e’s IR, we classify all expressions as two kinds, LValue and RValue . LValue represents the expressions that can appear in the left-hand side of an assignment, i.e., variable ( x = …; ), field access ( x.f = …; ), and array access ( x[i] = …; ). Correspondingly, RValue represents the expressions at the right-hand side of assignments, such as literals ( … = 1; ) and binary expressions ( … = a + b; ). Note that some expressions can appear in both side of assignments, e.g., variables (represented by Var in Tai-e, as shown in the figure above). In this assignment, you only need to focus on the Var (of all expressions), as we are doing live variable analysis.
pascal.taie.ir.stmt.Stmt
This is another key interface in Tai-e’s IR, which represents all statements in the program. As each expression belongs to certain statement in a typical programming language, to implement live variable analysis, you need to obtain the variables that are defined and used in a statement. Stmt provides two convenient APIs for these two operations:
- Optional<LValue> getDef()
- List<RValue> getUses()
open in new window and List to wrap the result of getDef() and getUses() .
pascal.taie.analysis.dataflow.fact.SetFact<Var>
open in new window . It provides various set operations, e.g., add/remove elements, intersect/union with other sets, etc. You will use this class to represent the data facts in live variable analysis. Its APIs are commented in the source code, and you should read the source code and decide which APIs to use in this assignment.
pascal.taie.analysis.dataflow.analysis.LiveVariableAnalysis
This class implements DataflowAnalysis and defines live variable analysis. It is incomplete, and you need to finish it as explained in Section 2.2 .
# 2.2 Your Task [Important!]
Your first task is to implement the following four APIs of LiveVariableAnalysis :
- SetFact newBoundaryFact(CFG)
- SetFact newInitialFact()
- void meetInto(SetFact,SetFact)
- boolean transferNode(Stmt,SetFact,SetFact)
which correspond to four parts of live variable analysis algorithm as shown below:
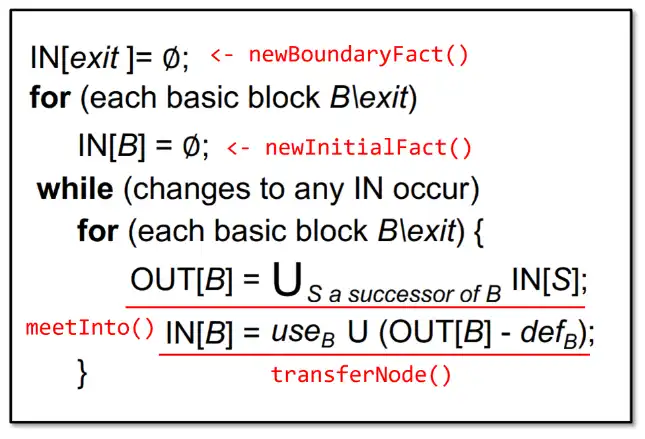
Here, the design of meetInto() might be a bit different from what you expect. It takes two facts as arguments ( fact and target ), and is supposed to meet fact into target . Unlike the equation in the above algorithm which sets OUT[B] to the union of IN facts of all successors of B , meetInto() meets the IN fact of each successor to OUT[B] individually, as illustrated by the following example.
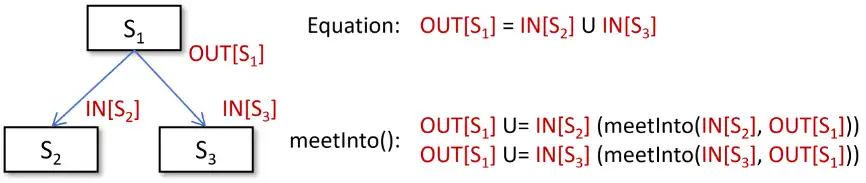
We design meetInto() in this way for efficiency. Firstly, each call to meetInto() for the same control-flow confluence node manipulates the same SetFact object (as OUT fact of the node), thus we can avoid creating many new SetFact objects (for equation OUT[S] = U… , you may need to create multiple new temporary SetFact objects when computing the union of IN facts at each iteration for the same node). In addition, such design enables an optimization, i.e., we can avoid meeting unchanged facts. For example, in some iteration, IN[S2] changes and IN[S3] remains the same. Then unlike the big union equation in the lecture, we only need to meet IN[S2] into OUT[S1] and avoid meeting IN[S3] . This optimization is beyond the scope of this assignment, and you do not need to consider it in your implementation.
To support such meet strategy, you also need to give OUT[S] an initial fact (the same initial value as in IN[S] ) for each statement.
Besides meetInto() , the other APIs to be implemented are straightforward. Note that the APIs of LiveVariableAnalysis are inherited from DataflowAnalysis , which is designed to support various analyses, thus you may not need to use all parameters of these APIs when you implement them in LiveVariableAnalysis .
We have provided code skeletons for all four APIs, and your task is to fill in the parts with comment “ TODO – finish me ”.
# 3 Implementing Iterative Solver
# 3.1 tai-e classes you need to know.
To implement iterative solver on Tai-e, you need to know the following classes.
pascal.taie.analysis.dataflow.fact.DataflowResult
Each object of this class manages the facts of a CFG in a data-flow analysis. You could get/set IN/OUT facts of nodes in a CFG through its APIs.
pascal.taie.analysis.graph.cfg.CFG
This class represents control-flow graphs of the methods in a program. It is iterable, thus you could iterate the nodes of a CFG via a for loop:
You could iterate predecessors/successors of a node by CFG.getPredsOf(Node) and CFG.getSuccsOf(Node) , for example,
For more information about CFG, please read its code and comments.
pascal.taie.analysis.dataflow.solver.Solver
This is the base class for data-flow analysis solvers. As there are different kinds of solvers, e.g., iterative solver and worklist solver (you will implement a worklist solver in the next assignment), we extract their common functionalities to this class. In run time, Tai-e builds CFGs and passes them to Solver.solve(CFG) to start the solver. You may notice that this class has two sets of initialize and doSolve methods, for supporting forward and backward data-flow analyses respectively (despite a bit redundant, such design will lead to a more clean and simpler code structure, and accordingly more straightforward implementation, compared with one analysis for two directions). This class is incomplete, and you need to finish it as explained in Section 3.2 .
pascal.taie.analysis.dataflow.solver.IterativeSolver
This class extends Solver and implements iterative algorithm. It is incomplete too, and to be finished.
# 3.2 Your Task [Important!]
Your second task is to finish two APIs of the solver classes mentioned above:
- Solver.initializeBackward(CFG,DataflowResult)
- IterativeSolver.doSolveBackward(CFG,DataflowResult)
You only need to focus on backward-related methods as live variable analysis is a backward analysis. In initializeBackward() , you should implement the first three lines of the algorithm in Section 2.2 . You should implement the while-loop of iterative algorithm in doSolveBackward() .
Each Solver holds an object of the corresponding data-flow analysis in its field analysis ( LiveVariableAnalysis in this assignment), and you should use it to implement the solver.
# 4 Run and Test Your Implementation
You can run the analysis following the instructions described in document Set Up Tai-e Assignments . In this assignment, Tai-e performs live variable analysis for each method of input class, and outputs the analysis results, i.e., the data-flow information (live variables) in OUT fact of each statement:
Each line starts with the position of the statement, e.g., [0@L4] means that the index of the statement in IR is 0, and it is in line 4 of the source code. Each line ends with the OUT fact of the statement, and it is null as you have not finished the analysis yet. After you implement the analysis, the output should be:
open in new window .
We provide test driver pascal.taie.analysis.dataflow.analysis.LiveVarTest for this assignment, and you could use it to test your implementation as described in Set Up Tai-e Assignments .
# 5 General Requirements
In this assignment, your only goal is correctness. Efficiency is not your concern.
DO NOT distribute the assignment package to any others.
Last but not least, DO NOT plagiarize. The work must be all your own!
# 6 Submission of Assignment
Your submission should be a zip file, which contains your implementation of
- LiveVariableAnalysis.java
- Solver.java
- IterativeSolver.java
# 7 Grading
You can refer to Grade Your Programming Assignments to use our online judge to grade your completed assignments.
The points will be allocated for correctness. We will use your submission to analyze the given test files from the src/test/resources/ directory, as well as other tests of our own, and compare your output to that of our solution.
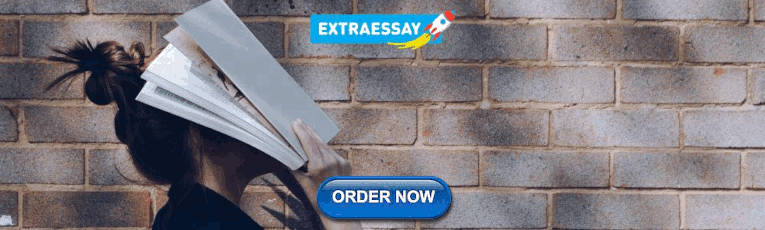
COMMENTS
1E - Assignment and Operations (author: Tao Yue, state: unchanged) Once you have declared a variable, you can store values in it. This is called assignment. To assign a value to a variable, follow this syntax: variable_name := expression; Note that unlike other languages, whose assignment operator is just an equals sign, Pascal uses a colon ...
Variables are declared in a var section . In Pascal every variable has a data type already known at compile-time (and let it be the data type variant ). A variable is declared by a ( identifier, data type identifier) tuple, separated by a colon : var foo: char; According to the data type's space requirements, the appropriate amount of memory ...
Like all programming languages, Pascal provides some means to modify memory. This concept is known as variables. Variables are named chunks of memory. You can use them to store data you cannot predict. Constants, on the other hand, are named pieces of data. You cannot alter them during run-time, but they are hard-coded into the compiled ...
I haven't codified in Pascal for a long time, but the assignation of a variable uses the := operator (assigment operator), = operator is for comparisitions. Otherwise you need to add the main program block, like this: Var current: string; Begin current := '1.6'; End. I hope can be useful for you.
The PChar type can be understood best as the Pascal equivalent of a C-style null-terminated string, i.e. a variable of type PChar is a pointer that points to an array of type Char, which is ended by a null-character (#0). Free Pascal supports initializing of PChar typed constants, or a direct assignment.
Assignment. First, we will learn a new statement, the assignment. Colon equals ( :=) is read as "becomes". In the line x := 10 the variable's value becomes ten. On the left hand side you write a variable name. On the right hand side you put a value. The value has to be valid for the variable's data type.
Specifies a user-defined list. Represents variables, whose values lie within a range. Stores an array of characters. Pascal programming language also allows defining various other types of variables, which we will cover in subsequent chapters like Pointer, Array, Records, Sets, and Files, etc.
15.3 Assignment operators. The assignment operator defines the action of a assignment of one type of variable to another. The result type must match the type of the variable at the left of the assignment statement, the single parameter to the assignment operator must have the same type as the expression at the right of the assignment operator.
In addition to the standard Pascal assignment operator (:=), which simply replaces the value of the variable with the value resulting from the expression on the right of the := operator, Free Pascal supports some C-style constructions.All available constructs are listed in table ().
Therefore is is Highly recommended to always initialize variables before using them. This can be easily done in the declaration of the variables. Given the declaration: Var S : String = 'This is an initialized string'; The value of the variable following will be initialized with the provided value. The following is an even better way of doing this:
Pascal has special rules for assigning fixed- and variable-length strings, null strings, and string constants. Fixed- and Variable-Length Strings When you make an assignment to a fixed-length string, and the source string is shorter than the destination string, the compiler pads the destination string with blanks.
Pascal is an imperative and procedural programming language, which Niklaus Wirth designed in 1968-69 and published in 1970, as a small, efficient language intended to encourage good programming practices using structured programming and data structuring. ... [-2E64+1 .. 2E63-1] Begin int:= 1; // how to assign a value to a variable r:= 3.14 ...
Pascal, however, recognized that an extra symbol should not be part of a statement in order to make it an actual statement. ... This expression has to have the data type of the variable on the LHS. An assignment makes the transient value of an expression "permanent" by storing it into the variable's memory block.
Variable parameters can serve as both input and output, meaning they can be used for passing a value to a routine, and getting a value from it. After procedure xorSwap has been called the variables at the call site will have changed. Assignments to variable parameters have an effect in the scope where the routine is called. Thus, a variable parameter can be considered as an alias for the ...
To assign a value to a variable, follow this syntax: variable_name:=expression; Note that unlike other languages, whose assignment operator is just an equals sign, Pascal uses a colon followed by an equals sign, similarly to how it's done in most computer algebra systems. The expression can either be a single value:
The Wilson and Addyman book ordered the productions alphabetically, and we did the same above. Other authors (Fitzpatrick ) presented the productions grouped by topic (types, statements etc), and other (Jobst ) tried to isolate the LL1 part from the non-LL1 parts.Also the traditional Pascal Grammars also add the definition of identifier, integer, real etc.
Description. Assign assigns a name to F, which can be any file type. This call doesn't open the file, it just assigns a name to a file variable, and marks the file as closed. Note that the filename (including path) can be only 255 characters long.
Pascal - Assignment: :=. The assignment operator is used to assign a value to a variable. it can be used for any data type with the only condition that the first parameter (left side) must be a value receiver, for example a variable. depending on the programming language can be different terms for the assignment of values to the variables, for ...
The Borland Pascal procedure assign associates a file variable with an external entity (e. g. a device file or a file on disk). Assign only operates on the program's internal management data and has otherwise no immediate observable effect.
No problems with Turbo/Borland, Virtual, or Free Pascal (even in Delphi mode). I assume that the Delphis treat (s) as an expression, which can have a value but you cannot assign a value to it. Of course if you write s := 'a'; Delphi works as expected. BTW: I consider it a bad habit, that you put superfluous around variables and expressions.
1 Assignment Objectives. Implement a live variable analysis for Java. Implement a generic iterative solver, which will be used to solve the data-flow problem you defined, i.e., the live variable analysis. In this first programming assignment, you will implement a live variable analysis and an iterative solver on top of Tai-e.