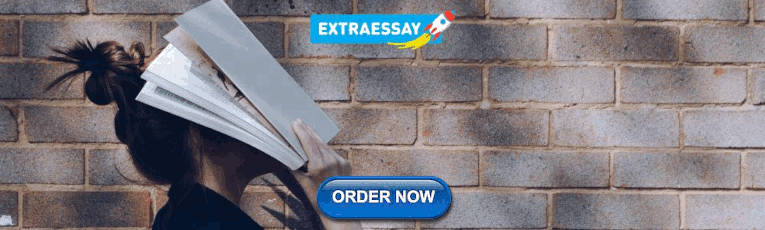
When to use const in C++? Part II: member variables
Just make everything const that you can! That’s the bare minimum you could do for your compiler!
This is a piece of advice, many senior developers tend to repeat to juniors, while so often even the preaching ones - we - fail to follow this rule.
In this series of articles, we discuss about:
In the first episode, we covered const functions and const local variables. Today we’ll speak about the members.
Originally, I didn’t plan this post. I simply wanted to speak about const variables regardless if they have a local scope or if they are members of an object.
Then I saw this tweet from Tina Ulbrich who I met at C++OnSea2020 and I was horrified. Yet another thing in C++, I had no idea about and something I’ve been doing considering that it’s a good practice.
Truth to be told, I didn’t do anything harmful, but that’s only by chance.
Ok, let’s get to it.
Why would you have const members at the first place?
Because you might want to signal that they are immutable, that their values should never change. Some would claim that you have private members for that purpose and you simply should not expose a setter for such members, then there is no need to explicitly make them const .
I get you, you’re right. In an ideal world.
But even if you are a strong believer of the Single Responsibility Principle and small classes, there is a fair chance that others later will change your code, your class will grow, and someone might accidentally change the value inside, plus you haven’t given the compiler a hint for optimization due to immutability.
To me, these are good reasons to make a member const. At least to show the intention.
But unfortunately, there are some implications.
The first is that classes a const member are not assignable:
If you think about it, it makes perfect sense. A variable is something you cannot change after initialization. And when you want to assign a new value to an object, thus to its members, it’s not possible anymore.
As such it also makes it impossible to use move semantics, for the same reason.
From the error messages, you can see that the corresponding special functions, such as the assignment operator or the move assignment operator were deleted.
Let’s implement the assignment operator. It will compile, but what the heck would you do?
Do you skip assigning to the const members? Not so great, either you depend on that value somewhere, or you should not store the value.
And you cannot assign to a const variable, can you? For a matter of fact, you can…
As you cannot cast the constness away from value, you have to turn the member value into a temporary non-const pointer and then you free to rampage.
Is this worth it?
You have your const member, fine. You have the assignment working, fine. Then if anyone comes later and wants to do the same “magic” outside of the special functions, for sure, it would be a red flag in a code review.
Speaking of special functions. Would move semantics work? Well, replace the assignment with this:
You’ll see that it’s still a copy assignment taking place as the the rule of 5 applies. If you implement one special function, you have to implement all of them. The rest is not generated.
In fact, what we have seen is rather dangerous. You think, you have a move and you’re efficient due to having a const member as using move semantics, but in fact, you are using the old copy assignment.
Yet, performance-wise, it seems hard to make a verdict. I ran a couple of tests in QuickBench and there is no significant difference between the above version and the one with non-const member and generated special assignment operator. On low optimization levels (None-O1) it depends on the compiler and its version. With higher optimization levels set there seems to be no difference.
Conclusions
Having const local variables is good. Having const members… It’s not so obvious. We lose the copy assignment and the move semantics as const members cannot be changed anymore.
With “clever” code, we can run a circle around the problem, but then we have to implement all the special functions. For what?
No performance gain. Less readability in the special functions and for slightly higher confidence that nobody will change the value of that member.
Do you think it’s worth it?
Stay tuned, next time we’ll discuss const return types.
If you want to learn more details about How to use const in C++ , check out my book on Leanpub !
Further Reading
When to use const in c++ part i: functions and local variables.
Just make everything const that you can! That’s the bare minimum you could do for your compiler! This is a piece of advice, many senior developers tend to repeat to juniors, while so often even th...
When to use const in C++? Part III: return types
When to use const in c++ part iv: parameters.
Don't Make Me Think by Steve Krug
What do you want from a job, that is the question
Comments powered by Disqus .
Trending Tags
C++ Operator Overloading Guidelines
One of the nice features of C++ is that you can give special meanings to operators, when they are used with user-defined classes. This is called operator overloading . You can implement C++ operator overloads by providing special member-functions on your classes that follow a particular naming convention. For example, to overload the + operator for your class, you would provide a member-function named operator+ on your class.
- = (assignment operator)
- + - * (binary arithmetic operators)
- += -= *= (compound assignment operators)
- == != (comparison operators)
Here are some guidelines for implementing these operators. These guidelines are very important to follow, so definitely get in the habit early.
Assignment Operator =
The assignment operator has a signature like this: class MyClass { public: ... MyClass & operator=(const MyClass &rhs); ... } MyClass a, b; ... b = a; // Same as b.operator=(a);
Notice that the = operator takes a const-reference to the right hand side of the assignment. The reason for this should be obvious, since we don't want to change that value; we only want to change what's on the left hand side.
- e = 42 assigns 42 to e , then returns e as the result
- The value of e is then assigned to d , and then d is returned as the result
- The value of d is then assigned to c , and then c is returned as the result
Now, in order to support operator chaining, the assignment operator must return some value. The value that should be returned is a reference to the left-hand side of the assignment.
Notice that the returned reference is not declared const . This can be a bit confusing, because it allows you to write crazy stuff like this: MyClass a, b, c; ... (a = b) = c; // What?? At first glance, you might want to prevent situations like this, by having operator= return a const reference. However, statements like this will work with primitive types. And, even worse, some tools actually rely on this behavior. Therefore, it is important to return a non- const reference from your operator= . The rule of thumb is, "If it's good enough for int s, it's good enough for user-defined data-types."
So, for the hypothetical MyClass assignment operator, you would do something like this: // Take a const-reference to the right-hand side of the assignment. // Return a non-const reference to the left-hand side. MyClass& MyClass::operator=(const MyClass &rhs) { ... // Do the assignment operation! return *this; // Return a reference to myself. } Remember, this is a pointer to the object that the member function is being called on. Since a = b is treated as a.operator=(b) , you can see why it makes sense to return the object that the function is called on; object a is the left-hand side.
But, the member function needs to return a reference to the object, not a pointer to the object. So, it returns *this , which returns what this points at (i.e. the object), not the pointer itself. (In C++, instances are turned into references, and vice versa, pretty much automatically, so even though *this is an instance, C++ implicitly converts it into a reference to the instance.)
Now, one more very important point about the assignment operator:
YOU MUST CHECK FOR SELF-ASSIGNMENT!
This is especially important when your class does its own memory allocation. Here is why: The typical sequence of operations within an assignment operator is usually something like this: MyClass& MyClass::operator=(const MyClass &rhs) { // 1. Deallocate any memory that MyClass is using internally // 2. Allocate some memory to hold the contents of rhs // 3. Copy the values from rhs into this instance // 4. Return *this } Now, what happens when you do something like this: MyClass mc; ... mc = mc; // BLAMMO. You can hopefully see that this would wreak havoc on your program. Because mc is on the left-hand side and on the right-hand side, the first thing that happens is that mc releases any memory it holds internally. But, this is where the values were going to be copied from, since mc is also on the right-hand side! So, you can see that this completely messes up the rest of the assignment operator's internals.
The easy way to avoid this is to CHECK FOR SELF-ASSIGNMENT. There are many ways to answer the question, "Are these two instances the same?" But, for our purposes, just compare the two objects' addresses. If they are the same, then don't do assignment. If they are different, then do the assignment.
So, the correct and safe version of the MyClass assignment operator would be this: MyClass& MyClass::operator=(const MyClass &rhs) { // Check for self-assignment! if (this == &rhs) // Same object? return *this; // Yes, so skip assignment, and just return *this. ... // Deallocate, allocate new space, copy values... return *this; } Or, you can simplify this a bit by doing: MyClass& MyClass::operator=(const MyClass &rhs) { // Only do assignment if RHS is a different object from this. if (this != &rhs) { ... // Deallocate, allocate new space, copy values... } return *this; } Remember that in the comparison, this is a pointer to the object being called, and &rhs is a pointer to the object being passed in as the argument. So, you can see that we avoid the dangers of self-assignment with this check.
- Take a const-reference for the argument (the right-hand side of the assignment).
- Return a reference to the left-hand side, to support safe and reasonable operator chaining. (Do this by returning *this .)
- Check for self-assignment, by comparing the pointers ( this to &rhs ).
Compound Assignment Operators += -= *=
I discuss these before the arithmetic operators for a very specific reason, but we will get to that in a moment. The important point is that these are destructive operators, because they update or replace the values on the left-hand side of the assignment. So, you write: MyClass a, b; ... a += b; // Same as a.operator+=(b) In this case, the values within a are modified by the += operator.
How those values are modified isn't very important - obviously, what MyClass represents will dictate what these operators mean.
The member function signature for such an operator should be like this: MyClass & MyClass::operator+=(const MyClass &rhs) { ... } We have already covered the reason why rhs is a const-reference. And, the implementation of such an operation should also be straightforward.
But, you will notice that the operator returns a MyClass -reference, and a non-const one at that. This is so you can do things like this: MyClass mc; ... (mc += 5) += 3;
Don't ask me why somebody would want to do this, but just like the normal assignment operator, this is allowed by the primitive data types. Our user-defined datatypes should match the same general characteristics of the primitive data types when it comes to operators, to make sure that everything works as expected.
This is very straightforward to do. Just write your compound assignment operator implementation, and return *this at the end, just like for the regular assignment operator. So, you would end up with something like this: MyClass & MyClass::operator+=(const MyClass &rhs) { ... // Do the compound assignment work. return *this; }
As one last note, in general you should beware of self-assignment with compound assignment operators as well. Fortunately, none of the C++ track's labs require you to worry about this, but you should always give it some thought when you are working on your own classes.
Binary Arithmetic Operators + - *
The binary arithmetic operators are interesting because they don't modify either operand - they actually return a new value from the two arguments. You might think this is going to be an annoying bit of extra work, but here is the secret:
Define your binary arithmetic operators using your compound assignment operators.
There, I just saved you a bunch of time on your homeworks.
So, you have implemented your += operator, and now you want to implement the + operator. The function signature should be like this: // Add this instance's value to other, and return a new instance // with the result. const MyClass MyClass::operator+(const MyClass &other) const { MyClass result = *this; // Make a copy of myself. Same as MyClass result(*this); result += other; // Use += to add other to the copy. return result; // All done! } Simple!
Actually, this explicitly spells out all of the steps, and if you want, you can combine them all into a single statement, like so: // Add this instance's value to other, and return a new instance // with the result. const MyClass MyClass::operator+(const MyClass &other) const { return MyClass(*this) += other; } This creates an unnamed instance of MyClass , which is a copy of *this . Then, the += operator is called on the temporary value, and then returns it.
If that last statement doesn't make sense to you yet, then stick with the other way, which spells out all of the steps. But, if you understand exactly what is going on, then you can use that approach.
You will notice that the + operator returns a const instance, not a const reference. This is so that people can't write strange statements like this: MyClass a, b, c; ... (a + b) = c; // Wuh...? This statement would basically do nothing, but if the + operator returns a non- const value, it will compile! So, we want to return a const instance, so that such madness will not even be allowed to compile.
- Implement the compound assignment operators from scratch, and then define the binary arithmetic operators in terms of the corresponding compound assignment operators.
- Return a const instance, to prevent worthless and confusing assignment operations that shouldn't be allowed.
Comparison Operators == and !=
The comparison operators are very simple. Define == first, using a function signature like this: bool MyClass::operator==(const MyClass &other) const { ... // Compare the values, and return a bool result. } The internals are very obvious and straightforward, and the bool return-value is also very obvious.
The important point here is that the != operator can also be defined in terms of the == operator, and you should do this to save effort. You can do something like this: bool MyClass::operator!=(const MyClass &other) const { return !(*this == other); } That way you get to reuse the hard work you did on implementing your == operator. Also, your code is far less likely to exhibit inconsistencies between == and != , since one is implemented in terms of the other.
- Windows Programming
- UNIX/Linux Programming
- General C++ Programming
- assignment constructor for const member
assignment constructor for const member

Assignment operators
Assignment operators modify the value of the object.
Explanation
copy assignment operator replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is a special member function, described in copy assignment operator .
move assignment operator replaces the contents of the object a with the contents of b , avoiding copying if possible ( b may be modified). For class types, this is a special member function, described in move assignment operator . (since C++11)
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
compound assignment operators replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
Builtin direct assignment
The direct assignment expressions have the form
For the built-in operator, lhs may have any non-const scalar type and rhs must be implicitly convertible to the type of lhs .
The direct assignment operator expects a modifiable lvalue as its left operand and an rvalue expression or a braced-init-list (since C++11) as its right operand, and returns an lvalue identifying the left operand after modification.
For non-class types, the right operand is first implicitly converted to the cv-unqualified type of the left operand, and then its value is copied into the object identified by left operand.
When the left operand has reference type, the assignment operator modifies the referred-to object.
If the left and the right operands identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same)
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2, where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
Builtin compound assignment
The compound assignment expressions have the form
The behavior of every builtin compound-assignment expression E1 op = E2 (where E1 is a modifiable lvalue expression and E2 is an rvalue expression or a braced-init-list (since C++11) ) is exactly the same as the behavior of the expression E1 = E1 op E2 , except that the expression E1 is evaluated only once and that it behaves as a single operation with respect to indeterminately-sequenced function calls (e.g. in f ( a + = b, g ( ) ) , the += is either not started at all or is completed as seen from inside g ( ) ).
In overload resolution against user-defined operators , for every pair A1 and A2, where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2, where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
Operator precedence
Operator overloading
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Copy Constructor vs Assignment Operator in C++
- How to Create Custom Assignment Operator in C++?
- Assignment Operators In C++
- Why copy constructor argument should be const in C++?
- Advanced C++ | Virtual Copy Constructor
- Move Assignment Operator in C++ 11
- Self assignment check in assignment operator
- Is assignment operator inherited?
- Copy Constructor in C++
- How to Implement Move Assignment Operator in C++?
- Default Assignment Operator and References in C++
- Can a constructor be private in C++ ?
- When is a Copy Constructor Called in C++?
- C++ Assignment Operator Overloading
- std::move in Utility in C++ | Move Semantics, Move Constructors and Move Assignment Operators
- C++ Interview questions based on constructors/ Destructors.
- Assignment Operators in C
- Copy Constructor in Python
- Copy Constructor in Java
- Constructors in Objective-C
- Vector in C++ STL
- Initialize a vector in C++ (7 different ways)
- Map in C++ Standard Template Library (STL)
- std::sort() in C++ STL
- Inheritance in C++
- The C++ Standard Template Library (STL)
- Object Oriented Programming in C++
- C++ Classes and Objects
- Virtual Function in C++
- Set in C++ Standard Template Library (STL)
Copy constructor and Assignment operator are similar as they are both used to initialize one object using another object. But, there are some basic differences between them:
Consider the following C++ program.
Explanation: Here, t2 = t1; calls the assignment operator , same as t2.operator=(t1); and Test t3 = t1; calls the copy constructor , same as Test t3(t1);
Must Read: When is a Copy Constructor Called in C++?
Please Login to comment...
Similar reads.
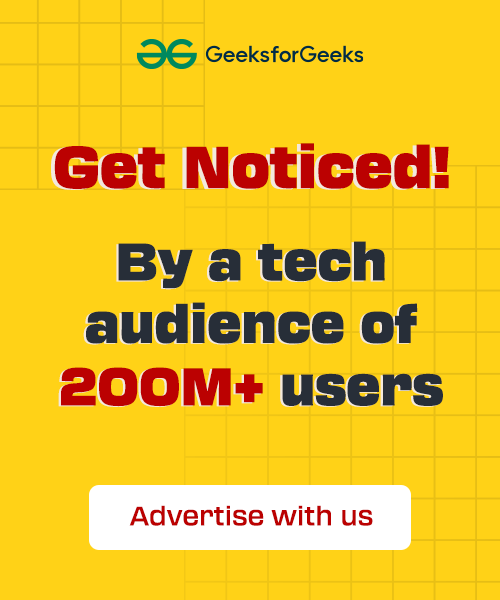
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Copy assignment operator.
A copy assignment operator is a non-template non-static member function with the name operator = that can be called with an argument of the same class type and copies the content of the argument without mutating the argument.
[ edit ] Syntax
For the formal copy assignment operator syntax, see function declaration . The syntax list below only demonstrates a subset of all valid copy assignment operator syntaxes.
[ edit ] Explanation
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type, the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M & or const volatile M & .
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) .
Due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument.
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
The implicitly-declared (or defaulted on its first declaration) copy assignment operator has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used or needed for constant evaluation (since C++14) . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types, the operator performs member-wise copy assignment of the object's direct bases and non-static data members, in their initialization order, using built-in assignment for the scalars, memberwise copy-assignment for arrays, and copy assignment operator for class types (called non-virtually).
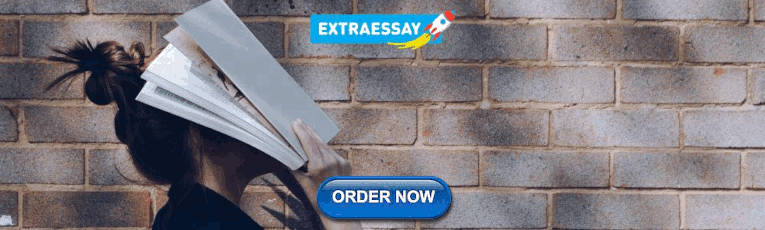
[ edit ] Deleted copy assignment operator
An implicitly-declared or explicitly-defaulted (since C++11) copy assignment operator for class T is undefined (until C++11) defined as deleted (since C++11) if any of the following conditions is satisfied:
- T has a non-static data member of a const-qualified non-class type (or possibly multi-dimensional array thereof).
- T has a non-static data member of a reference type.
- T has a potentially constructed subobject of class type M (or possibly multi-dimensional array thereof) such that the overload resolution as applied to find M 's copy assignment operator
- does not result in a usable candidate, or
- in the case of the subobject being a variant member , selects a non-trivial function.
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- it is not user-provided (meaning, it is implicitly-defined or defaulted);
- T has no virtual member functions;
- T has no virtual base classes;
- the copy assignment operator selected for every direct base of T is trivial;
- the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial.
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Eligible copy assignment operator
Triviality of eligible copy assignment operators determines whether the class is a trivially copyable type .
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move ), and selects the copy assignment if the argument is an lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
See assignment operator overloading for additional detail on the expected behavior of a user-defined copy-assignment operator.
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- converting constructor
- copy constructor
- copy elision
- default constructor
- aggregate initialization
- constant initialization
- copy initialization
- default initialization
- direct initialization
- initializer list
- list initialization
- reference initialization
- value initialization
- zero initialization
- move assignment
- move constructor
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 2 February 2024, at 15:13.
- This page has been accessed 1,333,785 times.
- Privacy policy
- About cppreference.com
- Disclaimers

This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C++ At Work
Copy Constructors, Assignment Operators, and More
Paul DiLascia
Code download available at: CAtWork0509.exe (276 KB) Browse the Code Online
Q I have a simple C++ problem. I want my copy constructor and assignment operator to do the same thing. Can you tell me the best way to accomplish this?
A At first glance this seems like a simple question with a simple answer: just write a copy constructor that calls operator=.
Or, alternatively, write a common copy method and call it from both your copy constructor and operator=, like so:
This code works fine for many classes, but there's more here than meets the eye. In particular, what happens if your class contains instances of other classes as members? To find out, I wrote the test program in Figure 1 . It has a main class, CMainClass, which contains an instance of another class, CMember. Both classes have a copy constructor and assignment operator, with the copy constructor for CMainClass calling operator= as in the first snippet. The code is sprinkled with printf statements to show which methods are called when. To exercise the constructors, cctest first creates an instance of CMainClass using the default ctor, then creates another instance using the copy constructor:
Figure 1 Copy Constructors and Assignment Operators
If you compile and run cctest, you'll see the following printf messages when cctest constructs obj2:
The member object m_obj got initialized twice! First by the default constructor, and again via assignment. Hey, what's going on?
In C++, assignment and copy construction are different because the copy constructor initializes uninitialized memory, whereas assignment starts with an existing initialized object. If your class contains instances of other classes as data members, the copy constructor must first construct these data members before it calls operator=. The result is that these members get initialized twice, as cctest shows. Got it? It's the same thing that happens with the default constructor when you initialize members using assignment instead of initializers. For example:
As opposed to:
Using assignment, m_obj is initialized twice; with the initializer syntax, only once. So, what's the solution to avoid extra initializations during copy construction? While it goes against your instinct to reuse code, this is one situation where it's best to implement your copy constructor and assignment operator separately, even if they do the same thing. Calling operator= from your copy constructor will certainly work, but it's not the most efficient implementation. My observation about initializers suggests a better way:
Now the main copy ctor calls the member object's copy ctor using an initializer, and m_obj is initialized just once by its copy ctor. In general, copy ctors should invoke the copy ctors of their members. Likewise for assignment. And, I may as well add, the same goes for base classes: your derived copy ctor and assignment operators should invoke the corresponding base class methods. Of course, there are always times when you may want to do something different because you know how your code works—but what I've described are the general rules, which are to be broken only when you have a compelling reason. If you have common tasks to perform after the basic objects have been initialized, you can put them in a common initialization method and call it from your constructors and operator=.
Q Can you tell me how to call a Visual C++® class from C#, and what syntax I need to use for this?
Sunil Peddi
Q I have an application that is written in both C# (the GUI) and in classic C++ (some business logic). Now I need to call from a DLL written in C++ a function (or a method) in a DLL written in Visual C++ .NET. This one calls another DLL written in C#. The Visual C++ .NET DLL acts like a proxy. Is this possible? I was able to use LoadLibrary to call a function present in the Visual C++ .NET DLL, and I can receive a return value, but when I try to pass some parameters to the function in the Visual C++ .NET DLL, I get the following error:
How can I resolve this problem?
Giuseppe Dattilo
A I get a lot of questions about interoperability between the Microsoft® .NET Framework and native C++, so I don't mind revisiting this well-covered topic yet again. There are two directions you can go: calling the Framework from C++ or calling C++ from the Framework. I won't go into COM interop here as that's a separate issue best saved for another day.
Let's start with the easiest one first: calling the Framework from C++. The simplest and easiest way to call the Framework from your C++ program is to use the Managed Extensions. These Microsoft-specific C++ language extensions are designed to make calling the Framework as easy as including a couple of files and then using the classes as if they were written in C++. Here's a very simple C++ program that calls the Framework's Console class:
To use the Managed Extensions, all you need to do is import <mscorlib.dll> and whatever .NET assemblies contain the classes you plan to use. Don't forget to compile with /clr:
Your C++ code can use managed classes more or less as if they were ordinary C++ classes. For example, you can create Framework objects with operator new, and access them using C++ pointer syntax, as shown in the following:
Here, the String s is declared as pointer-to-String because String::Format returns a new String object.
The "Hello, world" and date/time programs seem childishly simple—and they are—but just remember that however complex your program is, however many .NET assemblies and classes you use, the basic idea is the same: use <mscorlib.dll> and whatever other assemblies you need, then create managed objects with new, and use pointer syntax to access them.
So much for calling the Framework from C++. What about going the other way, calling C++ from the Framework? Here the road forks into two options, depending on whether you want to call extern C functions or C++ class member functions. Again, I'll take the simpler case first: calling C functions from .NET. The easiest thing to do here is use P/Invoke. With P/Invoke, you declare the external functions as static methods of a class, using the DllImport attribute to specify that the function lives in an external DLL. In C# it looks like this:
This tells the compiler that MessageBox is a function in user32.dll that takes an IntPtr (HWND), two Strings, and an int. You can then call it from your C# program like so:
Of course, you don't need P/Invoke for MessageBox since the .NET Framework already has a MessageBox class, but there are plenty of API functions that aren't supported directly by the Framework, and then you need P/Invoke. And, of course, you can use P/Invoke to call C functions in your own DLLs. I've used C# in the example, but P/Invoke works with any .NET-based language like Visual Basic® .NET or JScript®.NET. The names are the same, only the syntax is different.
Note that I used IntPtr to declare the HWND. I could have got away with int, but you should always use IntPtr for any kind of handle such as HWND, HANDLE, or HDC. IntPtr will default to 32 or 64 bits depending on the platform, so you never have to worry about the size of the handle.
DllImport has various modifiers you can use to specify details about the imported function. In this example, CharSet=CharSet.Auto tells the Framework to pass Strings as Unicode or Ansi, depending on the target operating system. Another little-known modifier you can use is CallingConvention. Recall that in C, there are different calling conventions, which are the rules that specify how the compiler should pass arguments and return values from one function to another across the stack. The default CallingConvention for DllImport is CallingConvention.Winapi. This is actually a pseudo-convention that uses the default convention for the target platform; for example, StdCall (in which the callee cleans the stack) on Windows® platforms and CDecl (in which the caller cleans the stack) on Windows CE .NET. CDecl is also used with varargs functions like printf.
The calling convention is where Giuseppe ran into trouble. C++ uses yet a third calling convention: thiscall. With this convention, the compiler uses the hardware register ECX to pass the "this" pointer to class member functions that don't have variable arguments. Without knowing the exact details of Giuseppe's program, it sounds from the error message that he's trying to call a C++ member function that expects thiscall from a C# program that's using StdCall—oops!
Aside from calling conventions, another interoperability issue when calling C++ methods from the Framework is linkage: C and C++ use different forms of linkage because C++ requires name-mangling to support function overloading. That's why you have to use extern "C" when you declare C functions in C++ programs: so the compiler won't mangle the name. In Windows, the entire windows.h file (now winuser.h) is enclosed in extern "C" brackets.
While there may be a way to call C++ member functions in a DLL directly using P/Invoke and DllImport with the exact mangled names and CallingConvention=ThisCall, it's not something to attempt if you're in your right mind. The proper way to call C++ classes from managed code—option number two—is to wrap your C++ classes in managed wrappers. Wrapping can be tedious if you have lots of classes, but it's really the only way to go. Say you have a C++ class CWidget and you want to wrap it so .NET clients can use it. The basic formula looks something like this:
The pattern is the same for any class. You write a managed (__gc) class that holds a pointer to the native class, you write a constructor and destructor that allocate and destroy the instance, and you write wrapper methods that call the corresponding native C++ member functions. You don't have to wrap all the member functions, only the ones you want to expose to the managed world.
Figure 2 shows a simple but concrete example in full detail. CPerson is a class that holds the name of a person, with member functions GetName and SetName to change the name. Figure 3 shows the managed wrapper for CPerson. In the example, I converted Get/SetName to a property, so .NET-based programmers can use the property syntax. In C#, using it looks like this:
Figure 3 Managed Person Class
Figure 2 Native CPerson Class
Using properties is purely a matter of style; I could equally well have exposed two methods, GetName and SetName, as in the native class. But properties feel more like .NET. The wrapper class is an assembly like any other, but one that links with the native DLL. This is one of the cool benefits of the Managed Extensions: You can link directly with native C/C++ code. If you download and compile the source for my CPerson example, you'll see that the makefile generates two separate DLLs: person.dll implements a normal native DLL and mperson.dll is the managed assembly that wraps it. There are also two test programs: testcpp.exe, a native C++ program that calls the native person.dll and testcs.exe, which is written in C# and calls the managed wrapper mperson.dll (which in turn calls the native person.dll).
Figure 4** Interop Highway **
I've used a very simple example to highlight the fact that there are fundamentally only a few main highways across the border between the managed and native worlds (see Figure 4 ). If your C++ classes are at all complex, the biggest interop problem you'll encounter is converting parameters between native and managed types, a process called marshaling. The Managed Extensions do an admirable job of making this as painless as possible (for example, automatically converting primitive types and Strings), but there are times where you have to know something about what you're doing.
For example, you can't pass the address of a managed object or subobject to a native function without pinning it first. That's because managed objects live in the managed heap, which the garbage collector is free to rearrange. If the garbage collector moves an object, it can update all the managed references to that object—but it knows nothing of raw native pointers that live outside the managed world. That's what __pin is for; it tells the garbage collector: don't move this object. For strings, the Framework has a special function PtrToStringChars that returns a pinned pointer to the native characters. (Incidentally, for those curious-minded souls, PtrToStringChars is the only function as of this date defined in <vcclr.h>. Figure 5 shows the code.) I used PtrToStringChars in MPerson to set the Name (see Figure 3 ).
Figure 5 PtrToStringChars
Pinning isn't the only interop problem you'll encounter. Other problems arise if you have to deal with arrays, references, structs, and callbacks, or access a subobject within an object. This is where some of the more advanced techniques come in, such as StructLayout, boxing, __value types, and so on. You also need special code to handle exceptions (native or managed) and callbacks/delegates. But don't let these interop details obscure the big picture. First decide which way you're calling (from managed to native or the other way around), and if you're calling from managed to native, whether to use P/Invoke or a wrapper.
In Visual Studio® 2005 (which some of you may already have as beta bits), the Managed Extensions have been renamed and upgraded to something called C++/CLI. Think of the C++/CLI as Managed Extensions Version 2, or What the Managed Extensions Should Have Been. The changes are mostly a matter of syntax, though there are some important semantic changes, too. In general C++/CLI is designed to highlight rather than blur the distinction between managed and native objects. Using pointer syntax for managed objects was a clever and elegant idea, but in the end perhaps a little too clever because it obscures important differences between managed and native objects. C++/CLI introduces the key notion of handles for managed objects, so instead of using C pointer syntax for managed objects, the CLI uses ^ (hat):
As you no doubt noticed, there's also a gcnew operator to clarify when you're allocating objects on the managed heap as opposed to the native one. This has the added benefit that gcnew doesn't collide with C++ new, which can be overloaded or even redefined as a macro. C++/CLI has many other cool features designed to make interoperability as straightforward and intuitive as possible.
Send your questions and comments for Paul to [email protected] .
Paul DiLascia is a freelance software consultant and Web/UI designer-at-large. He is the author of Windows ++: Writing Reusable Windows Code in C ++ (Addison-Wesley, 1992). In his spare time, Paul develops PixieLib, an MFC class library available from his Web site, www.dilascia.com .
Additional resources

21.12 — Overloading the assignment operator
The copy assignment operator (operator=) is used to copy values from one object to another already existing object .
Related content
As of C++11, C++ also supports “Move assignment”. We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
Copy assignment vs Copy constructor
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects.
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it’s really not all that difficult. Summarizing:
- If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value).
- If a new object does not have to be created before the copying can occur, the assignment operator is used.
Overloading the assignment operator
Overloading the copy assignment operator (operator=) is fairly straightforward, with one specific caveat that we’ll get to. The copy assignment operator must be overloaded as a member function.
This prints:
This should all be pretty straightforward by now. Our overloaded operator= returns *this, so that we can chain multiple assignments together:
Issues due to self-assignment
Here’s where things start to get a little more interesting. C++ allows self-assignment:
This will call f1.operator=(f1), and under the simplistic implementation above, all of the members will be assigned to themselves. In this particular example, the self-assignment causes each member to be assigned to itself, which has no overall impact, other than wasting time. In most cases, a self-assignment doesn’t need to do anything at all!
However, in cases where an assignment operator needs to dynamically assign memory, self-assignment can actually be dangerous:
First, run the program as it is. You’ll see that the program prints “Alex” as it should.
Now run the following program:
You’ll probably get garbage output. What happened?
Consider what happens in the overloaded operator= when the implicit object AND the passed in parameter (str) are both variable alex. In this case, m_data is the same as str.m_data. The first thing that happens is that the function checks to see if the implicit object already has a string. If so, it needs to delete it, so we don’t end up with a memory leak. In this case, m_data is allocated, so the function deletes m_data. But because str is the same as *this, the string that we wanted to copy has been deleted and m_data (and str.m_data) are dangling.
Later on, we allocate new memory to m_data (and str.m_data). So when we subsequently copy the data from str.m_data into m_data, we’re copying garbage, because str.m_data was never initialized.
Detecting and handling self-assignment
Fortunately, we can detect when self-assignment occurs. Here’s an updated implementation of our overloaded operator= for the MyString class:
By checking if the address of our implicit object is the same as the address of the object being passed in as a parameter, we can have our assignment operator just return immediately without doing any other work.
Because this is just a pointer comparison, it should be fast, and does not require operator== to be overloaded.
When not to handle self-assignment
Typically the self-assignment check is skipped for copy constructors. Because the object being copy constructed is newly created, the only case where the newly created object can be equal to the object being copied is when you try to initialize a newly defined object with itself:
In such cases, your compiler should warn you that c is an uninitialized variable.
Second, the self-assignment check may be omitted in classes that can naturally handle self-assignment. Consider this Fraction class assignment operator that has a self-assignment guard:
If the self-assignment guard did not exist, this function would still operate correctly during a self-assignment (because all of the operations done by the function can handle self-assignment properly).
Because self-assignment is a rare event, some prominent C++ gurus recommend omitting the self-assignment guard even in classes that would benefit from it. We do not recommend this, as we believe it’s a better practice to code defensively and then selectively optimize later.
The copy and swap idiom
A better way to handle self-assignment issues is via what’s called the copy and swap idiom. There’s a great writeup of how this idiom works on Stack Overflow .
The implicit copy assignment operator
Unlike other operators, the compiler will provide an implicit public copy assignment operator for your class if you do not provide a user-defined one. This assignment operator does memberwise assignment (which is essentially the same as the memberwise initialization that default copy constructors do).
Just like other constructors and operators, you can prevent assignments from being made by making your copy assignment operator private or using the delete keyword:
Note that if your class has const members, the compiler will instead define the implicit operator= as deleted. This is because const members can’t be assigned, so the compiler will assume your class should not be assignable.
If you want a class with const members to be assignable (for all members that aren’t const), you will need to explicitly overload operator= and manually assign each non-const member.
Copy assignment operator
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . A type with a public copy assignment operator is CopyAssignable .
[ edit ] Syntax
[ edit ] explanation.
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used
- Forcing a copy assignment operator to be generated by the compiler
- Avoiding implicit copy assignment
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B& or const volatile B &
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M& or const volatile M &
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
The implicitly-declared or defaulted copy assignment operator for class T is defined as deleted in any of the following is true:
- T has a non-static data member that is const
- T has a non-static data member of a reference type.
- T has a non-static data member that cannot be copy-assigned (has deleted, inaccessible, or ambiguous copy assignment operator)
- T has direct or virtual base class that cannot be copy-assigned (has deleted, inaccessible, or ambiguous move assignment operator)
- T has a user-declared move constructor
- T has a user-declared move assignment operator
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- The operator is not user-provided (meaning, it is implicitly-defined or defaulted), and if it is defaulted, its signature is the same as implicitly-defined
- T has no virtual member functions
- T has no virtual base classes
- The copy assignment operator selected for every direct base of T is trivial
- The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is not deleted or trivial, it is defined (that is, a function body is generated and compiled) by the compiler. For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either prvalue such as a nameless temporary or xvalue such as the result of std::move ), and selects the copy assignment if the argument is lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
[ edit ] Copy and swap
Copy assignment operator can be expressed in terms of copy constructor, destructor, and the swap() member function, if one is provided:
T & T :: operator = ( T arg ) { // copy/move constructor is called to construct arg swap ( arg ) ; // resources exchanged between *this and arg return * this ; } // destructor is called to release the resources formerly held by *this
For non-throwing swap(), this form provides strong exception guarantee . For rvalue arguments, this form automatically invokes the move constructor, and is sometimes referred to as "unifying assignment operator" (as in, both copy and move).
[ edit ] Example
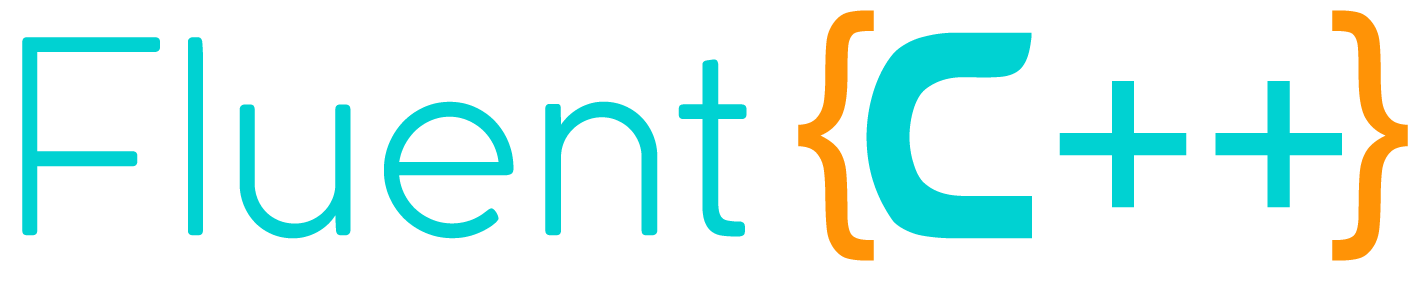
About Jonathan Boccara
Hello, my name is Jonathan Boccara, I'm your host on Fluent C++. I have been a developer for 10 years. My focus is on how to write expressive code . I wrote the book The Legacy Code Programmer's Toolbox . I'm happy to take your feedback, don't hesitate to drop a comment on a post, follow me or get in touch directly !
Jonathan Boccara's blog
Recent Posts
- Usage First, Implementation After: A Principle of Software Development
- Design Patterns VS Design Principles: Factory method
- How to Store an lvalue or an rvalue in the Same Object
- Copy-Paste Developments
- Design Patterns VS Design Principles: Abstract Factory
- How to Generate All the Combinations from Several Collections
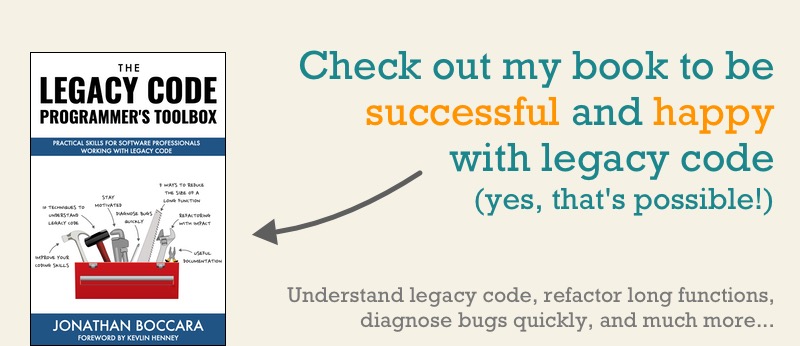
Compiler-generated Functions, Rule of Three and Rule of Five

When you read a class interface that defines some basic functions (constructors, destructors, assignment) but not all of them , don’t you wonder what that code means, and what functions will be available for that class in practice? I often do.
To clarify this type of situation, I suggest we make a recap of what class functions the compiler generates in C++. Being clear on this will let us:
- better understand such code,
- reflect on higher-level questions, such as whether = default makes code more expressive or not, which we’ll explore in the next post.
I went to my compiler and tested out various combinations of user-defined and compiler-defined functions. You’ll find the results synthesized in this article, with some rationale that I took from Effective C++ (item 5 and 6) and Modern Effective C++ (item 17).
Hope you’ll find those results useful.
What functions the compiler can generate
The idea of compiler-generated functions is that, if some functions of a class are so trivial to write that their code would nearly be boilerplate, the compiler will take care of writing them for you.
This feature has been here since C++98, where the compiler would try to generate:
- a default constructor X() , that calls the default constructor of each class member and base class,
- a copy constructor X(X const& other) , that calls a copy constructor on each member and base class,
- a copy assignment operator X& operator=(X const& other) , that calls a copy assignment operator on each class member and base class,
- the destructor ~X() , that calls the destructor of each class member and base class. Note that this default-generated destructor is never virtual (unless it is for a class inheriting from one that has a virtual destructor).
With C++11, the compiler generates 2 new functions related to move semantics:
- a move constructor X(X&& other) , that calls a move constructor of each class member and base class,
- a move assignment operator X& operator=(X&& other) , that calls a move assignment operator on each class member and base class.
Note that other functions have been proposed for automatic generation such as the comparison operators, and something related to this should hit C++20 with the spaceship operator. More on that later.
The Rule of Three and the Rule of Five
It is important to note that the default constructor has different semantics from the rest of the above functions. Indeed, all the other functions deal with the management of the resources inside of the class: how to copy them, how to dispose of them.
If a class holds a handle to a resource such as a database connection or an owning raw pointer (which would be the case in a smart pointer for example), those functions need to pay special care to handle the life cycle of that resource.
The default constructor only initializes the resource, and is closer in semantics to any other constructor that takes values, rather than to those special functions that handle resource life cycle.
Let’s now count the functions in the above bullet points that handle the resource management of the class:
- there are 3 in C++98 (4 minus the default constructor),
- there are 5 in C++11.
Which gives the “Rule of Three” in C++98, and the “Rule of Five” in C++11: let x be 3 in C++98 and 5 in C++11, then we have:
If you need to write the code for one of the x resource management functions, it suggests that your class’s resource handling is not trivial and you certainly need to write the other x – 1. – Rule of x
When the compiler generates them
In some cases, the compiler won’t generate those functions.
If you write any of those functions yourself, the compiler won’t generate it. That’s pretty obvious.
If you don’t write one of the following (and you didn’t write move operations either, see below why):
- a copy constructor,
- a copy-assignement operator,
- a destructor,
the compiler will try to generate them for you. Even if you’ve hand-written the other two. In some cases it may not succeed though, for instance if the class contains a const or reference member, the compiler won’t be able to come up with an operator= .
If you write any of the following:
- a direct constructor X(int, double) ,
- a move constructor,
then the compiler thinks: “the developer made the decision to write a constructor, maybe they don’t want a default one then”, and it doesn’t generate the default constructor. Which makes sense to me in the case of the value constructor, but that I find weird for the copy and move constructor, since like we said, default constructor and copy constructor have different semantics.
- a copy assignment operator,
the compiler thinks “there must be something complex about the resource management of that class if the developer took the time to write one of those”, and it doesn’t generate the move constructor nor the move assignment operator.
You may wonder, why does the compiler only refrain from generating the move functions and not the copy functions? After all, if it feels that the resource handling of the class is beyond its understanding, it shouldn’t generate any of the resource-handling functions, not even the destructor while we’re at it. That’s the rule of 5, isn’t it?
That’s true, and the reason for the observed behaviour is history. C++98 didn’t natively enforce the rule of 3. But C++11, that brought the move functions, also wanted to enforce the rule of 5. But to preserve backward compatibility, C++11 couldn’t remove the copy functions that existing code relied upon, only the move function that didn’t exist yet. This led to that compromise that we could (somewhat approximately) call the “rule of 2”.
Finally, if you write any of the following:
- a move assignment operator,
the compiler still thinks “there must be something complex about the resource management of that class if the developer took the time to write one of those”. But code that contains move operations can’t be pre-C++11. So there is no longer a backward compatibility and the compiler can fully enforce the rule of 5 by refraining from generating any of the 5 resource management functions.
= default and = delete
C++11 brought those two keywords that you can tack on the 6 functions that the compiler can generate.
If you write = default , as in:
Or in an implementation file:
Then you are explicitly asking the compiler to generate that function for you, and it will do it to the best of its abilities. It can fail though, if there is no possible default implementation. For a default constructor, that would be if one of the members of the class doesn’t itself have a default constructor for example.
And if you write = delete , you explicitly ask to remove that function, and the compiler can always satisfy this request. It looks like this:
The Rule of Zero
Now that we’re clear on what makes the compiler generate functions or not, we can move on to higher-level questions. In the next post, we’ll reflect over whether = default make an interface more expressive or not.
One of the aspects of that question will lead us the to Rule of Zero, which is to the Rule of Three and the Rule of Five what Batman Begins is to The Dark Knight and the The Dark Knight Rises, if I may say.
With that said, stay tuned for the next post.

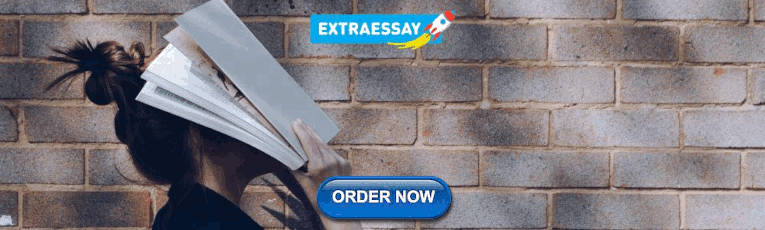
IMAGES
VIDEO
COMMENTS
Fourth, in the rest of my answer I focus on the usage of volatile, as applied to the class members, to see if this might solve the 2nd of these two potential undefined behavior cases: The potential UB in your own solution: // Custom-defined assignment operator. A& operator=(const A& right) {.
Yet, performance-wise, it seems hard to make a verdict. I ran a couple of tests in QuickBench and there is no significant difference between the above version and the one with non-const member and generated special assignment operator. On low optimization levels (None-O1) it depends on the compiler and its version.
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x);. Use the copy constructor. If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you.
Note that none of the following constructors, despite the fact that. they could do the same thing as a copy constructor, are copy. constructors: 1. 2. MyClass( MyClass* other ); MyClass( const MyClass* other ); or my personal favorite way to create an infinite loop in C++: MyClass( MyClass other );
C++ Operator Overloading Guidelines. One of the nice features of C++ is that you can give special meanings to operators, when they are used with user-defined classes. This is called operator overloading. You can implement C++ operator overloads by providing special member-functions on your classes that follow a particular naming convention.
Hi all, is there a good way to define a assignment constructor for const members? Below code doesnot compile for function Foo& operator=(const Foo &f), ... * technically, you could write a copy-assignment operator that doesn't attempt to modify m_val and that will compile, but after such a = b; a and b will not be equal, and that's rarely ...
For the built-in operator, lhs may have any non-const scalar type and rhs must be implicitly convertible to the type of lhs. The direct assignment operator expects a modifiable lvalue as its left operand and an rvalue expression or a braced-init-list (since C++11) as its right operand, and returns an lvalue identifying the left operand after modification.
What is the correct syntax for the above assignment operator? If i is const it can't be assigned, so the implementation of the assignment operator should simply return *this assignment operator should be left as implicitly delete'ed.The default, compiler-generated assignment operator performs a member-wise assignment of each non-static data member.
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current ...
But, there are some basic differences between them: Copy constructor. Assignment operator. It is called when a new object is created from an existing object, as a copy of the existing object. This operator is called when an already initialized object is assigned a new value from another existing object. It creates a separate memory block for ...
Implicitly-declared copy assignment operator. If no user-defined copy assignment operators are provided for a class type, the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T:: operator = (const T &) if all of the following is true:
This topic describes how to write a move constructor and a move assignment operator for a C++ class. A move constructor enables the resources owned by an rvalue object to be moved into an lvalue without copying. For more information about move semantics, see Rvalue Reference Declarator: &&. This topic builds upon the following C++ class ...
9. The assignment operator copies one object into another. Since all objects of the same type share the same static members, there's no reason to copy the static members. const members are another matter. You can't (well, shouldn't) change them, but if two objects have different values for a const member, it might not be a good idea to copy one ...
CFoo::CFoo(const CFoo& rhs) : m_obj(rhs.m_obj) {} Now the main copy ctor calls the member object's copy ctor using an initializer, and m_obj is initialized just once by its copy ctor. In general, copy ctors should invoke the copy ctors of their members. Likewise for assignment.
21.12 — Overloading the assignment operator. Alex November 27, 2023. The copy assignment operator (operator=) is used to copy values from one object to another already existing object. As of C++11, C++ also supports "Move assignment". We discuss move assignment in lesson 22.3 -- Move constructors and move assignment .
const members are ideal in many, many cases. of course, there is the obvious case where a value should not or must not change, but it's also an important restriction for optimization and concurrency -- not every type needs or should have an assignment operator.. if the member needs the behavior of assignment, then the variable must not be const.. when the value/member must not mutate or be ...
Copy assignment operator. A copy assignment operator of class T is a non-template non-static member function with the name operator= that takes exactly one parameter of type T, T&, const T&, volatile T&, or const volatile T&. A type with a public copy assignment operator is CopyAssignable .
If you must supply an operator=() for a class with a const member you must (1) justify such a broken design (2) implement the operator=() so it assigns other members, but not the const ones. @TedLyngmo Presumably the copy constructor should copy name_, since its only other choice would be to make it empty. But if the class does that, it will ...
a copy assignment operator X& operator=(X const& other), ... (X&& other), that calls a move assignment operator on each class member and base class. Note that other functions have been proposed for automatic generation such as the comparison operators, and something related to this should hit C++20 with the spaceship operator. ...
How to overload copy assignment operator for const data members? 0 Discards qualifiers unknown cause (std::bind() / lambda) 2 Overloading the stream insertion operator? Related questions. 1 How to overload copy assignment operator for const data members? ... std::priority_queue<struct with const member&> - 'operator =' function unavailable. 0