Eloquent: Getting Started
Introduction, generating model classes, table names, primary keys, uuid and ulid keys, database connections, default attribute values, configuring eloquent strictness, collections, chunking results.
- Chunk Using Lazy Collections
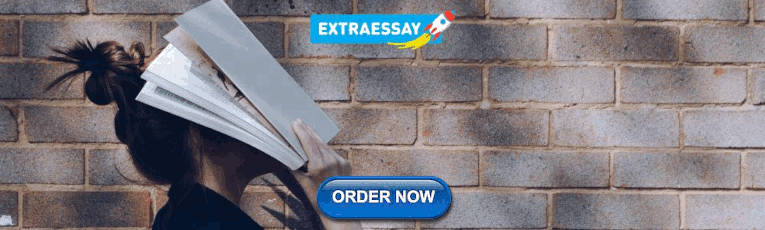
Advanced Subqueries
Retrieving or creating models, retrieving aggregates, mass assignment, soft deleting, querying soft deleted models, pruning models, replicating models, global scopes, local scopes, comparing models, using closures, muting events.
Laravel includes Eloquent, an object-relational mapper (ORM) that makes it enjoyable to interact with your database. When using Eloquent, each database table has a corresponding "Model" that is used to interact with that table. In addition to retrieving records from the database table, Eloquent models allow you to insert, update, and delete records from the table as well.
[!NOTE] Before getting started, be sure to configure a database connection in your application's config/database.php configuration file. For more information on configuring your database, check out the database configuration documentation .
Laravel Bootcamp
If you're new to Laravel, feel free to jump into the Laravel Bootcamp . The Laravel Bootcamp will walk you through building your first Laravel application using Eloquent. It's a great way to get a tour of everything that Laravel and Eloquent have to offer.
To get started, let's create an Eloquent model. Models typically live in the app\Models directory and extend the Illuminate\Database\Eloquent\Model class. You may use the make:model Artisan command to generate a new model:
If you would like to generate a database migration when you generate the model, you may use the --migration or -m option:
You may generate various other types of classes when generating a model, such as factories, seeders, policies, controllers, and form requests. In addition, these options may be combined to create multiple classes at once:
Inspecting Models
Sometimes it can be difficult to determine all of a model's available attributes and relationships just by skimming its code. Instead, try the model:show Artisan command, which provides a convenient overview of all the model's attributes and relations:
Eloquent Model Conventions
Models generated by the make:model command will be placed in the app/Models directory. Let's examine a basic model class and discuss some of Eloquent's key conventions:
After glancing at the example above, you may have noticed that we did not tell Eloquent which database table corresponds to our Flight model. By convention, the "snake case", plural name of the class will be used as the table name unless another name is explicitly specified. So, in this case, Eloquent will assume the Flight model stores records in the flights table, while an AirTrafficController model would store records in an air_traffic_controllers table.
If your model's corresponding database table does not fit this convention, you may manually specify the model's table name by defining a table property on the model:
Eloquent will also assume that each model's corresponding database table has a primary key column named id . If necessary, you may define a protected $primaryKey property on your model to specify a different column that serves as your model's primary key:
In addition, Eloquent assumes that the primary key is an incrementing integer value, which means that Eloquent will automatically cast the primary key to an integer. If you wish to use a non-incrementing or a non-numeric primary key you must define a public $incrementing property on your model that is set to false :
If your model's primary key is not an integer, you should define a protected $keyType property on your model. This property should have a value of string :
"Composite" Primary Keys
Eloquent requires each model to have at least one uniquely identifying "ID" that can serve as its primary key. "Composite" primary keys are not supported by Eloquent models. However, you are free to add additional multi-column, unique indexes to your database tables in addition to the table's uniquely identifying primary key.
Instead of using auto-incrementing integers as your Eloquent model's primary keys, you may choose to use UUIDs instead. UUIDs are universally unique alpha-numeric identifiers that are 36 characters long.
If you would like a model to use a UUID key instead of an auto-incrementing integer key, you may use the Illuminate\Database\Eloquent\Concerns\HasUuids trait on the model. Of course, you should ensure that the model has a UUID equivalent primary key column :
By default, The HasUuids trait will generate "ordered" UUIDs for your models. These UUIDs are more efficient for indexed database storage because they can be sorted lexicographically.
You can override the UUID generation process for a given model by defining a newUniqueId method on the model. In addition, you may specify which columns should receive UUIDs by defining a uniqueIds method on the model:
If you wish, you may choose to utilize "ULIDs" instead of UUIDs. ULIDs are similar to UUIDs; however, they are only 26 characters in length. Like ordered UUIDs, ULIDs are lexicographically sortable for efficient database indexing. To utilize ULIDs, you should use the Illuminate\Database\Eloquent\Concerns\HasUlids trait on your model. You should also ensure that the model has a ULID equivalent primary key column :
By default, Eloquent expects created_at and updated_at columns to exist on your model's corresponding database table. Eloquent will automatically set these column's values when models are created or updated. If you do not want these columns to be automatically managed by Eloquent, you should define a $timestamps property on your model with a value of false :
If you need to customize the format of your model's timestamps, set the $dateFormat property on your model. This property determines how date attributes are stored in the database as well as their format when the model is serialized to an array or JSON:
If you need to customize the names of the columns used to store the timestamps, you may define CREATED_AT and UPDATED_AT constants on your model:
If you would like to perform model operations without the model having its updated_at timestamp modified, you may operate on the model within a closure given to the withoutTimestamps method:
By default, all Eloquent models will use the default database connection that is configured for your application. If you would like to specify a different connection that should be used when interacting with a particular model, you should define a $connection property on the model:
By default, a newly instantiated model instance will not contain any attribute values. If you would like to define the default values for some of your model's attributes, you may define an $attributes property on your model. Attribute values placed in the $attributes array should be in their raw, "storable" format as if they were just read from the database:
Laravel offers several methods that allow you to configure Eloquent's behavior and "strictness" in a variety of situations.
First, the preventLazyLoading method accepts an optional boolean argument that indicates if lazy loading should be prevented. For example, you may wish to only disable lazy loading in non-production environments so that your production environment will continue to function normally even if a lazy loaded relationship is accidentally present in production code. Typically, this method should be invoked in the boot method of your application's AppServiceProvider :
Also, you may instruct Laravel to throw an exception when attempting to fill an unfillable attribute by invoking the preventSilentlyDiscardingAttributes method. This can help prevent unexpected errors during local development when attempting to set an attribute that has not been added to the model's fillable array:
Retrieving Models
Once you have created a model and its associated database table , you are ready to start retrieving data from your database. You can think of each Eloquent model as a powerful query builder allowing you to fluently query the database table associated with the model. The model's all method will retrieve all of the records from the model's associated database table:
Building Queries
The Eloquent all method will return all of the results in the model's table. However, since each Eloquent model serves as a query builder , you may add additional constraints to queries and then invoke the get method to retrieve the results:
[!NOTE] Since Eloquent models are query builders, you should review all of the methods provided by Laravel's query builder . You may use any of these methods when writing your Eloquent queries.
Refreshing Models
If you already have an instance of an Eloquent model that was retrieved from the database, you can "refresh" the model using the fresh and refresh methods. The fresh method will re-retrieve the model from the database. The existing model instance will not be affected:
The refresh method will re-hydrate the existing model using fresh data from the database. In addition, all of its loaded relationships will be refreshed as well:
As we have seen, Eloquent methods like all and get retrieve multiple records from the database. However, these methods don't return a plain PHP array. Instead, an instance of Illuminate\Database\Eloquent\Collection is returned.
The Eloquent Collection class extends Laravel's base Illuminate\Support\Collection class, which provides a variety of helpful methods for interacting with data collections. For example, the reject method may be used to remove models from a collection based on the results of an invoked closure:
In addition to the methods provided by Laravel's base collection class, the Eloquent collection class provides a few extra methods that are specifically intended for interacting with collections of Eloquent models.
Since all of Laravel's collections implement PHP's iterable interfaces, you may loop over collections as if they were an array:
Your application may run out of memory if you attempt to load tens of thousands of Eloquent records via the all or get methods. Instead of using these methods, the chunk method may be used to process large numbers of models more efficiently.
The chunk method will retrieve a subset of Eloquent models, passing them to a closure for processing. Since only the current chunk of Eloquent models is retrieved at a time, the chunk method will provide significantly reduced memory usage when working with a large number of models:
The first argument passed to the chunk method is the number of records you wish to receive per "chunk". The closure passed as the second argument will be invoked for each chunk that is retrieved from the database. A database query will be executed to retrieve each chunk of records passed to the closure.
If you are filtering the results of the chunk method based on a column that you will also be updating while iterating over the results, you should use the chunkById method. Using the chunk method in these scenarios could lead to unexpected and inconsistent results. Internally, the chunkById method will always retrieve models with an id column greater than the last model in the previous chunk:
Chunking Using Lazy Collections
The lazy method works similarly to the chunk method in the sense that, behind the scenes, it executes the query in chunks. However, instead of passing each chunk directly into a callback as is, the lazy method returns a flattened LazyCollection of Eloquent models, which lets you interact with the results as a single stream:
If you are filtering the results of the lazy method based on a column that you will also be updating while iterating over the results, you should use the lazyById method. Internally, the lazyById method will always retrieve models with an id column greater than the last model in the previous chunk:
You may filter the results based on the descending order of the id using the lazyByIdDesc method.
Similar to the lazy method, the cursor method may be used to significantly reduce your application's memory consumption when iterating through tens of thousands of Eloquent model records.
The cursor method will only execute a single database query; however, the individual Eloquent models will not be hydrated until they are actually iterated over. Therefore, only one Eloquent model is kept in memory at any given time while iterating over the cursor.
[!WARNING] Since the cursor method only ever holds a single Eloquent model in memory at a time, it cannot eager load relationships. If you need to eager load relationships, consider using the lazy method instead.
Internally, the cursor method uses PHP generators to implement this functionality:
The cursor returns an Illuminate\Support\LazyCollection instance. Lazy collections allow you to use many of the collection methods available on typical Laravel collections while only loading a single model into memory at a time:
Although the cursor method uses far less memory than a regular query (by only holding a single Eloquent model in memory at a time), it will still eventually run out of memory. This is due to PHP's PDO driver internally caching all raw query results in its buffer . If you're dealing with a very large number of Eloquent records, consider using the lazy method instead.
Subquery Selects
Eloquent also offers advanced subquery support, which allows you to pull information from related tables in a single query. For example, let's imagine that we have a table of flight destinations and a table of flights to destinations. The flights table contains an arrived_at column which indicates when the flight arrived at the destination.
Using the subquery functionality available to the query builder's select and addSelect methods, we can select all of the destinations and the name of the flight that most recently arrived at that destination using a single query:
Subquery Ordering
In addition, the query builder's orderBy function supports subqueries. Continuing to use our flight example, we may use this functionality to sort all destinations based on when the last flight arrived at that destination. Again, this may be done while executing a single database query:
Retrieving Single Models / Aggregates
In addition to retrieving all of the records matching a given query, you may also retrieve single records using the find , first , or firstWhere methods. Instead of returning a collection of models, these methods return a single model instance:
Sometimes you may wish to perform some other action if no results are found. The findOr and firstOr methods will return a single model instance or, if no results are found, execute the given closure. The value returned by the closure will be considered the result of the method:
Not Found Exceptions
Sometimes you may wish to throw an exception if a model is not found. This is particularly useful in routes or controllers. The findOrFail and firstOrFail methods will retrieve the first result of the query; however, if no result is found, an Illuminate\Database\Eloquent\ModelNotFoundException will be thrown:
If the ModelNotFoundException is not caught, a 404 HTTP response is automatically sent back to the client:
The firstOrCreate method will attempt to locate a database record using the given column / value pairs. If the model can not be found in the database, a record will be inserted with the attributes resulting from merging the first array argument with the optional second array argument:
The firstOrNew method, like firstOrCreate , will attempt to locate a record in the database matching the given attributes. However, if a model is not found, a new model instance will be returned. Note that the model returned by firstOrNew has not yet been persisted to the database. You will need to manually call the save method to persist it:
When interacting with Eloquent models, you may also use the count , sum , max , and other aggregate methods provided by the Laravel query builder . As you might expect, these methods return a scalar value instead of an Eloquent model instance:
Inserting and Updating Models
Of course, when using Eloquent, we don't only need to retrieve models from the database. We also need to insert new records. Thankfully, Eloquent makes it simple. To insert a new record into the database, you should instantiate a new model instance and set attributes on the model. Then, call the save method on the model instance:
In this example, we assign the name field from the incoming HTTP request to the name attribute of the App\Models\Flight model instance. When we call the save method, a record will be inserted into the database. The model's created_at and updated_at timestamps will automatically be set when the save method is called, so there is no need to set them manually.
Alternatively, you may use the create method to "save" a new model using a single PHP statement. The inserted model instance will be returned to you by the create method:
However, before using the create method, you will need to specify either a fillable or guarded property on your model class. These properties are required because all Eloquent models are protected against mass assignment vulnerabilities by default. To learn more about mass assignment, please consult the mass assignment documentation .
The save method may also be used to update models that already exist in the database. To update a model, you should retrieve it and set any attributes you wish to update. Then, you should call the model's save method. Again, the updated_at timestamp will automatically be updated, so there is no need to manually set its value:
Occasionally, you may need to update an existing model or create a new model if no matching model exists. Like the firstOrCreate method, the updateOrCreate method persists the model, so there's no need to manually call the save method.
In the example below, if a flight exists with a departure location of Oakland and a destination location of San Diego , its price and discounted columns will be updated. If no such flight exists, a new flight will be created which has the attributes resulting from merging the first argument array with the second argument array:
Mass Updates
Updates can also be performed against models that match a given query. In this example, all flights that are active and have a destination of San Diego will be marked as delayed:
The update method expects an array of column and value pairs representing the columns that should be updated. The update method returns the number of affected rows.
[!WARNING] When issuing a mass update via Eloquent, the saving , saved , updating , and updated model events will not be fired for the updated models. This is because the models are never actually retrieved when issuing a mass update.
Examining Attribute Changes
Eloquent provides the isDirty , isClean , and wasChanged methods to examine the internal state of your model and determine how its attributes have changed from when the model was originally retrieved.
The isDirty method determines if any of the model's attributes have been changed since the model was retrieved. You may pass a specific attribute name or an array of attributes to the isDirty method to determine if any of the attributes are "dirty". The isClean method will determine if an attribute has remained unchanged since the model was retrieved. This method also accepts an optional attribute argument:
The wasChanged method determines if any attributes were changed when the model was last saved within the current request cycle. If needed, you may pass an attribute name to see if a particular attribute was changed:
The getOriginal method returns an array containing the original attributes of the model regardless of any changes to the model since it was retrieved. If needed, you may pass a specific attribute name to get the original value of a particular attribute:
You may use the create method to "save" a new model using a single PHP statement. The inserted model instance will be returned to you by the method:
However, before using the create method, you will need to specify either a fillable or guarded property on your model class. These properties are required because all Eloquent models are protected against mass assignment vulnerabilities by default.
A mass assignment vulnerability occurs when a user passes an unexpected HTTP request field and that field changes a column in your database that you did not expect. For example, a malicious user might send an is_admin parameter through an HTTP request, which is then passed to your model's create method, allowing the user to escalate themselves to an administrator.
So, to get started, you should define which model attributes you want to make mass assignable. You may do this using the $fillable property on the model. For example, let's make the name attribute of our Flight model mass assignable:
Once you have specified which attributes are mass assignable, you may use the create method to insert a new record in the database. The create method returns the newly created model instance:
If you already have a model instance, you may use the fill method to populate it with an array of attributes:
Mass Assignment and JSON Columns
When assigning JSON columns, each column's mass assignable key must be specified in your model's $fillable array. For security, Laravel does not support updating nested JSON attributes when using the guarded property:
Allowing Mass Assignment
If you would like to make all of your attributes mass assignable, you may define your model's $guarded property as an empty array. If you choose to unguard your model, you should take special care to always hand-craft the arrays passed to Eloquent's fill , create , and update methods:
Mass Assignment Exceptions
By default, attributes that are not included in the $fillable array are silently discarded when performing mass-assignment operations. In production, this is expected behavior; however, during local development it can lead to confusion as to why model changes are not taking effect.
If you wish, you may instruct Laravel to throw an exception when attempting to fill an unfillable attribute by invoking the preventSilentlyDiscardingAttributes method. Typically, this method should be invoked in the boot method of your application's AppServiceProvider class:
Eloquent's upsert method may be used to update or create records in a single, atomic operation. The method's first argument consists of the values to insert or update, while the second argument lists the column(s) that uniquely identify records within the associated table. The method's third and final argument is an array of the columns that should be updated if a matching record already exists in the database. The upsert method will automatically set the created_at and updated_at timestamps if timestamps are enabled on the model:
[!WARNING] All databases except SQL Server require the columns in the second argument of the upsert method to have a "primary" or "unique" index. In addition, the MySQL database driver ignores the second argument of the upsert method and always uses the "primary" and "unique" indexes of the table to detect existing records.
Deleting Models
To delete a model, you may call the delete method on the model instance:
You may call the truncate method to delete all of the model's associated database records. The truncate operation will also reset any auto-incrementing IDs on the model's associated table:
Deleting an Existing Model by its Primary Key
In the example above, we are retrieving the model from the database before calling the delete method. However, if you know the primary key of the model, you may delete the model without explicitly retrieving it by calling the destroy method. In addition to accepting the single primary key, the destroy method will accept multiple primary keys, an array of primary keys, or a collection of primary keys:
[!WARNING] The destroy method loads each model individually and calls the delete method so that the deleting and deleted events are properly dispatched for each model.
Deleting Models Using Queries
Of course, you may build an Eloquent query to delete all models matching your query's criteria. In this example, we will delete all flights that are marked as inactive. Like mass updates, mass deletes will not dispatch model events for the models that are deleted:
[!WARNING] When executing a mass delete statement via Eloquent, the deleting and deleted model events will not be dispatched for the deleted models. This is because the models are never actually retrieved when executing the delete statement.
In addition to actually removing records from your database, Eloquent can also "soft delete" models. When models are soft deleted, they are not actually removed from your database. Instead, a deleted_at attribute is set on the model indicating the date and time at which the model was "deleted". To enable soft deletes for a model, add the Illuminate\Database\Eloquent\SoftDeletes trait to the model:
[!NOTE] The SoftDeletes trait will automatically cast the deleted_at attribute to a DateTime / Carbon instance for you.
You should also add the deleted_at column to your database table. The Laravel schema builder contains a helper method to create this column:
Now, when you call the delete method on the model, the deleted_at column will be set to the current date and time. However, the model's database record will be left in the table. When querying a model that uses soft deletes, the soft deleted models will automatically be excluded from all query results.
To determine if a given model instance has been soft deleted, you may use the trashed method:
Restoring Soft Deleted Models
Sometimes you may wish to "un-delete" a soft deleted model. To restore a soft deleted model, you may call the restore method on a model instance. The restore method will set the model's deleted_at column to null :
You may also use the restore method in a query to restore multiple models. Again, like other "mass" operations, this will not dispatch any model events for the models that are restored:
The restore method may also be used when building relationship queries:
Permanently Deleting Models
Sometimes you may need to truly remove a model from your database. You may use the forceDelete method to permanently remove a soft deleted model from the database table:
You may also use the forceDelete method when building Eloquent relationship queries:
Including Soft Deleted Models
As noted above, soft deleted models will automatically be excluded from query results. However, you may force soft deleted models to be included in a query's results by calling the withTrashed method on the query:
The withTrashed method may also be called when building a relationship query:
Retrieving Only Soft Deleted Models
The onlyTrashed method will retrieve only soft deleted models:
Sometimes you may want to periodically delete models that are no longer needed. To accomplish this, you may add the Illuminate\Database\Eloquent\Prunable or Illuminate\Database\Eloquent\MassPrunable trait to the models you would like to periodically prune. After adding one of the traits to the model, implement a prunable method which returns an Eloquent query builder that resolves the models that are no longer needed:
When marking models as Prunable , you may also define a pruning method on the model. This method will be called before the model is deleted. This method can be useful for deleting any additional resources associated with the model, such as stored files, before the model is permanently removed from the database:
After configuring your prunable model, you should schedule the model:prune Artisan command in your application's routes/console.php file. You are free to choose the appropriate interval at which this command should be run:
Behind the scenes, the model:prune command will automatically detect "Prunable" models within your application's app/Models directory. If your models are in a different location, you may use the --model option to specify the model class names:
If you wish to exclude certain models from being pruned while pruning all other detected models, you may use the --except option:
You may test your prunable query by executing the model:prune command with the --pretend option. When pretending, the model:prune command will simply report how many records would be pruned if the command were to actually run:
[!WARNING] Soft deleting models will be permanently deleted ( forceDelete ) if they match the prunable query.
Mass Pruning
When models are marked with the Illuminate\Database\Eloquent\MassPrunable trait, models are deleted from the database using mass-deletion queries. Therefore, the pruning method will not be invoked, nor will the deleting and deleted model events be dispatched. This is because the models are never actually retrieved before deletion, thus making the pruning process much more efficient:
You may create an unsaved copy of an existing model instance using the replicate method. This method is particularly useful when you have model instances that share many of the same attributes:
To exclude one or more attributes from being replicated to the new model, you may pass an array to the replicate method:
Query Scopes
Global scopes allow you to add constraints to all queries for a given model. Laravel's own soft delete functionality utilizes global scopes to only retrieve "non-deleted" models from the database. Writing your own global scopes can provide a convenient, easy way to make sure every query for a given model receives certain constraints.
Generating Scopes
To generate a new global scope, you may invoke the make:scope Artisan command, which will place the generated scope in your application's app/Models/Scopes directory:
Writing Global Scopes
Writing a global scope is simple. First, use the make:scope command to generate a class that implements the Illuminate\Database\Eloquent\Scope interface. The Scope interface requires you to implement one method: apply . The apply method may add where constraints or other types of clauses to the query as needed:
[!NOTE] If your global scope is adding columns to the select clause of the query, you should use the addSelect method instead of select . This will prevent the unintentional replacement of the query's existing select clause.
Applying Global Scopes
To assign a global scope to a model, you may simply place the ScopedBy attribute on the model:
Or, you may manually register the global scope by overriding the model's booted method and invoke the model's addGlobalScope method. The addGlobalScope method accepts an instance of your scope as its only argument:
After adding the scope in the example above to the App\Models\User model, a call to the User::all() method will execute the following SQL query:
Anonymous Global Scopes
Eloquent also allows you to define global scopes using closures, which is particularly useful for simple scopes that do not warrant a separate class of their own. When defining a global scope using a closure, you should provide a scope name of your own choosing as the first argument to the addGlobalScope method:
Removing Global Scopes
If you would like to remove a global scope for a given query, you may use the withoutGlobalScope method. This method accepts the class name of the global scope as its only argument:
Or, if you defined the global scope using a closure, you should pass the string name that you assigned to the global scope:
If you would like to remove several or even all of the query's global scopes, you may use the withoutGlobalScopes method:
Local scopes allow you to define common sets of query constraints that you may easily re-use throughout your application. For example, you may need to frequently retrieve all users that are considered "popular". To define a scope, prefix an Eloquent model method with scope .
Scopes should always return the same query builder instance or void :
Utilizing a Local Scope
Once the scope has been defined, you may call the scope methods when querying the model. However, you should not include the scope prefix when calling the method. You can even chain calls to various scopes:
Combining multiple Eloquent model scopes via an or query operator may require the use of closures to achieve the correct logical grouping :
However, since this can be cumbersome, Laravel provides a "higher order" orWhere method that allows you to fluently chain scopes together without the use of closures:
Dynamic Scopes
Sometimes you may wish to define a scope that accepts parameters. To get started, just add your additional parameters to your scope method's signature. Scope parameters should be defined after the $query parameter:
Once the expected arguments have been added to your scope method's signature, you may pass the arguments when calling the scope:
Sometimes you may need to determine if two models are the "same" or not. The is and isNot methods may be used to quickly verify two models have the same primary key, table, and database connection or not:
The is and isNot methods are also available when using the belongsTo , hasOne , morphTo , and morphOne relationships . This method is particularly helpful when you would like to compare a related model without issuing a query to retrieve that model:
[!NOTE] Want to broadcast your Eloquent events directly to your client-side application? Check out Laravel's model event broadcasting .
Eloquent models dispatch several events, allowing you to hook into the following moments in a model's lifecycle: retrieved , creating , created , updating , updated , saving , saved , deleting , deleted , trashed , forceDeleting , forceDeleted , restoring , restored , and replicating .
The retrieved event will dispatch when an existing model is retrieved from the database. When a new model is saved for the first time, the creating and created events will dispatch. The updating / updated events will dispatch when an existing model is modified and the save method is called. The saving / saved events will dispatch when a model is created or updated - even if the model's attributes have not been changed. Event names ending with -ing are dispatched before any changes to the model are persisted, while events ending with -ed are dispatched after the changes to the model are persisted.
To start listening to model events, define a $dispatchesEvents property on your Eloquent model. This property maps various points of the Eloquent model's lifecycle to your own event classes . Each model event class should expect to receive an instance of the affected model via its constructor:
After defining and mapping your Eloquent events, you may use event listeners to handle the events.
[!WARNING] When issuing a mass update or delete query via Eloquent, the saved , updated , deleting , and deleted model events will not be dispatched for the affected models. This is because the models are never actually retrieved when performing mass updates or deletes.
Instead of using custom event classes, you may register closures that execute when various model events are dispatched. Typically, you should register these closures in the booted method of your model:
If needed, you may utilize queueable anonymous event listeners when registering model events. This will instruct Laravel to execute the model event listener in the background using your application's queue :
Defining Observers
If you are listening for many events on a given model, you may use observers to group all of your listeners into a single class. Observer classes have method names which reflect the Eloquent events you wish to listen for. Each of these methods receives the affected model as their only argument. The make:observer Artisan command is the easiest way to create a new observer class:
This command will place the new observer in your app/Observers directory. If this directory does not exist, Artisan will create it for you. Your fresh observer will look like the following:
To register an observer, you may place the ObservedBy attribute on the corresponding model:
Or, you may manually register an observer by invoking the observe method on the model you wish to observe. You may register observers in the boot method of your application's AppServiceProvider class:
[!NOTE] There are additional events an observer can listen to, such as saving and retrieved . These events are described within the events documentation.
Observers and Database Transactions
When models are being created within a database transaction, you may want to instruct an observer to only execute its event handlers after the database transaction is committed. You may accomplish this by implementing the ShouldHandleEventsAfterCommit interface on your observer. If a database transaction is not in progress, the event handlers will execute immediately:
You may occasionally need to temporarily "mute" all events fired by a model. You may achieve this using the withoutEvents method. The withoutEvents method accepts a closure as its only argument. Any code executed within this closure will not dispatch model events, and any value returned by the closure will be returned by the withoutEvents method:
Saving a Single Model Without Events
Sometimes you may wish to "save" a given model without dispatching any events. You may accomplish this using the saveQuietly method:
You may also "update", "delete", "soft delete", "restore", and "replicate" a given model without dispatching any events:
Navigating the Hazards of Mass Assignment in Laravel
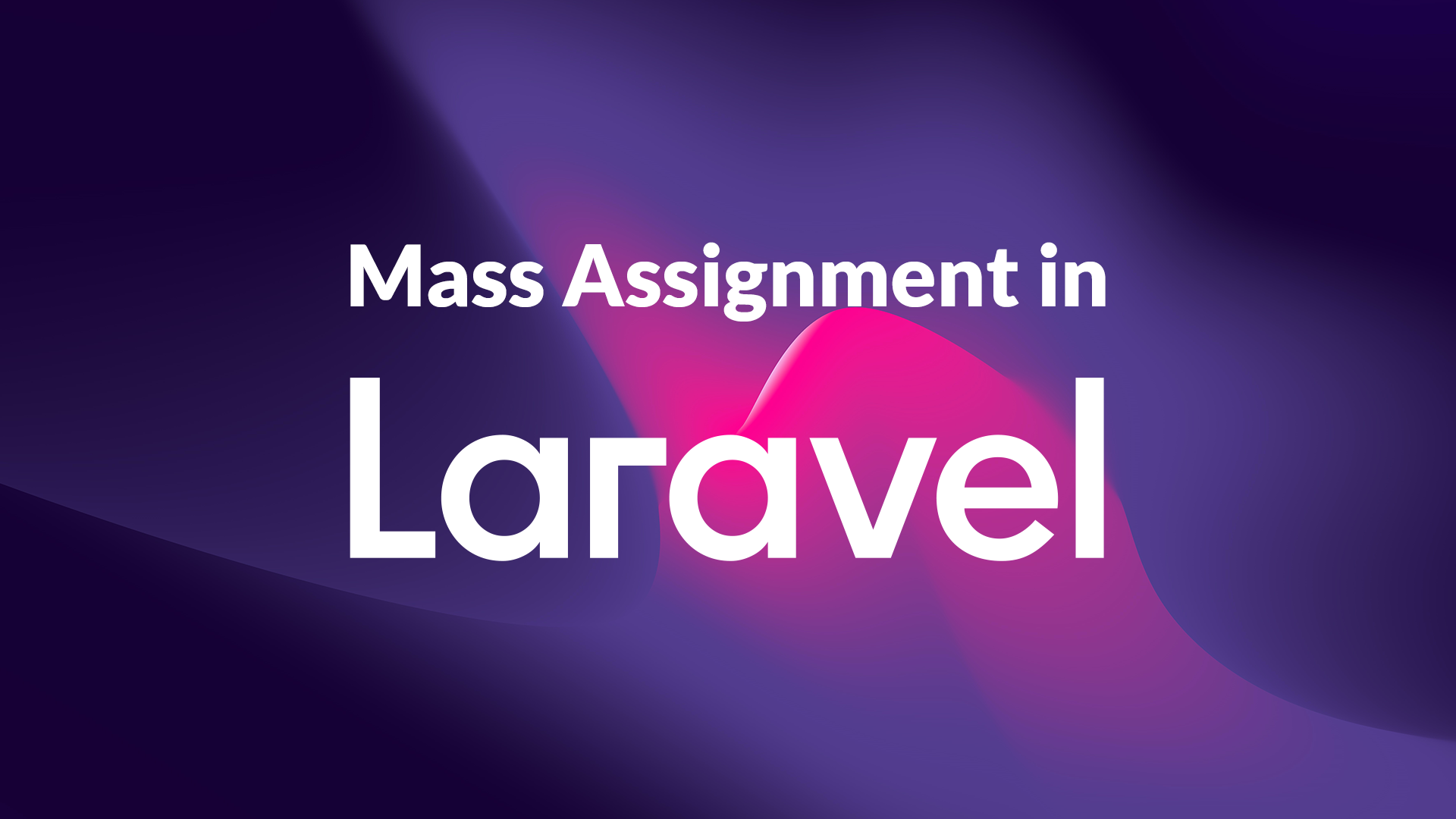
Introduction
Laravel, a popular PHP web application framework, empowers developers with a wide array of tools and features to build robust and scalable applications. Among these features is "Mass Assignment," a convenient way to populate model attributes using an array of data. While this technique streamlines development, it comes with certain pitfalls that developers must be aware of to ensure data security and application integrity.
Understanding Mass Assignment
Mass Assignment allows developers to quickly assign values to a model's attributes by passing an associative array to the model's constructor or the create() method. This feature enhances code readability and reduces the need for repetitive property assignments. For instance, creating a new User instance and populating its attributes can be done in one line using mass assignment.
Pitfalls of Mass Assignment
Overexposing Vulnerabilities: One of the most critical pitfalls of mass assignment is the risk of exposing sensitive attributes. If not handled carefully, attackers might manipulate the input array to update attributes that should not be accessible, such as isAdmin or isSuperUser . Laravel provides protection against this vulnerability through the fillable and guarded properties, which allow developers to specify which attributes can be mass-assigned.
Unintended Overwrites: Mass Assignment can lead to unintended attribute overwrites. If attributes are not properly guarded or fillable, users could potentially update attributes they shouldn't have access to. This can result in data inconsistencies and unexpected behavior. Careful configuration of the model's fillable and guarded properties can mitigate this risk.
Bypassing Business Logic: Mass Assignment might allow data to bypass important business logic and validation. In some cases, certain attributes should only be updated under specific conditions. By blindly using mass assignment, developers might inadvertently allow invalid or incorrect data to enter the system, leading to incorrect outcomes.
Complex Relationships: When dealing with models that have complex relationships, mass assignment can become more intricate. Nested relationships, polymorphic associations, and multi-level structures require careful handling to ensure data consistency and proper relationships. Failing to manage these complexities might result in erroneous database entries.
Best Practices for Secure Mass Assignment
Use the fillable and guarded Properties: Explicitly define the fillable or guarded properties in your model to control which attributes can be mass-assigned. Use fillable to specify attributes that are safe for mass assignment, and use guarded to protect sensitive attributes.
Leverage Mutators and Accessors: Utilize mutator and accessor methods to modify or retrieve attribute values before they are saved to or retrieved from the database. This provides an additional layer of control over the data and allows you to enforce custom validation and logic.
Validate Input Data: Always validate input data, regardless of whether it's being used for mass assignment. Laravel's validation features can ensure that the data adheres to expected formats and rules before it is processed.
Use Explicit Attribute Assignment: In scenarios where you need to handle a limited number of attributes, consider manually assigning them using individual setters. This approach provides more control over what data is being assigned and avoids unintended overwrites.
Mass Assignment is a powerful feature in Laravel that accelerates development by simplifying attribute assignment. However, developers must tread cautiously to avoid the potential pitfalls associated with security vulnerabilities, unintended overwrites, and bypassing critical business logic. By adhering to best practices, such as defining fillable and guarded properties, leveraging mutators, and validating input data, developers can harness the benefits of mass assignment while maintaining data integrity and application security.
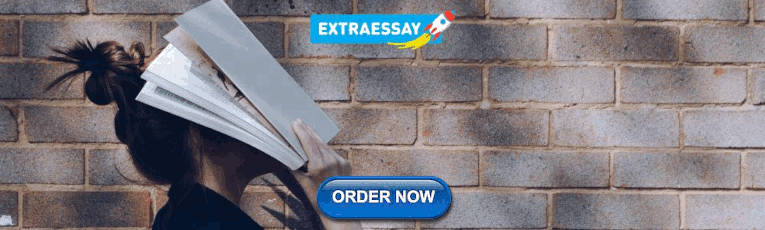
Kontaktperson
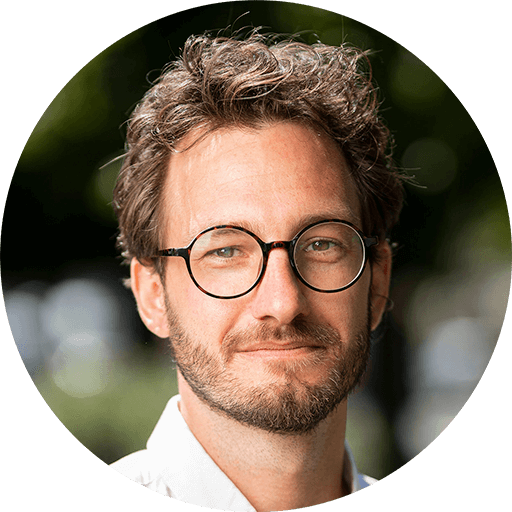
Ola Ebbesson
Fler inlägg från bloggen.
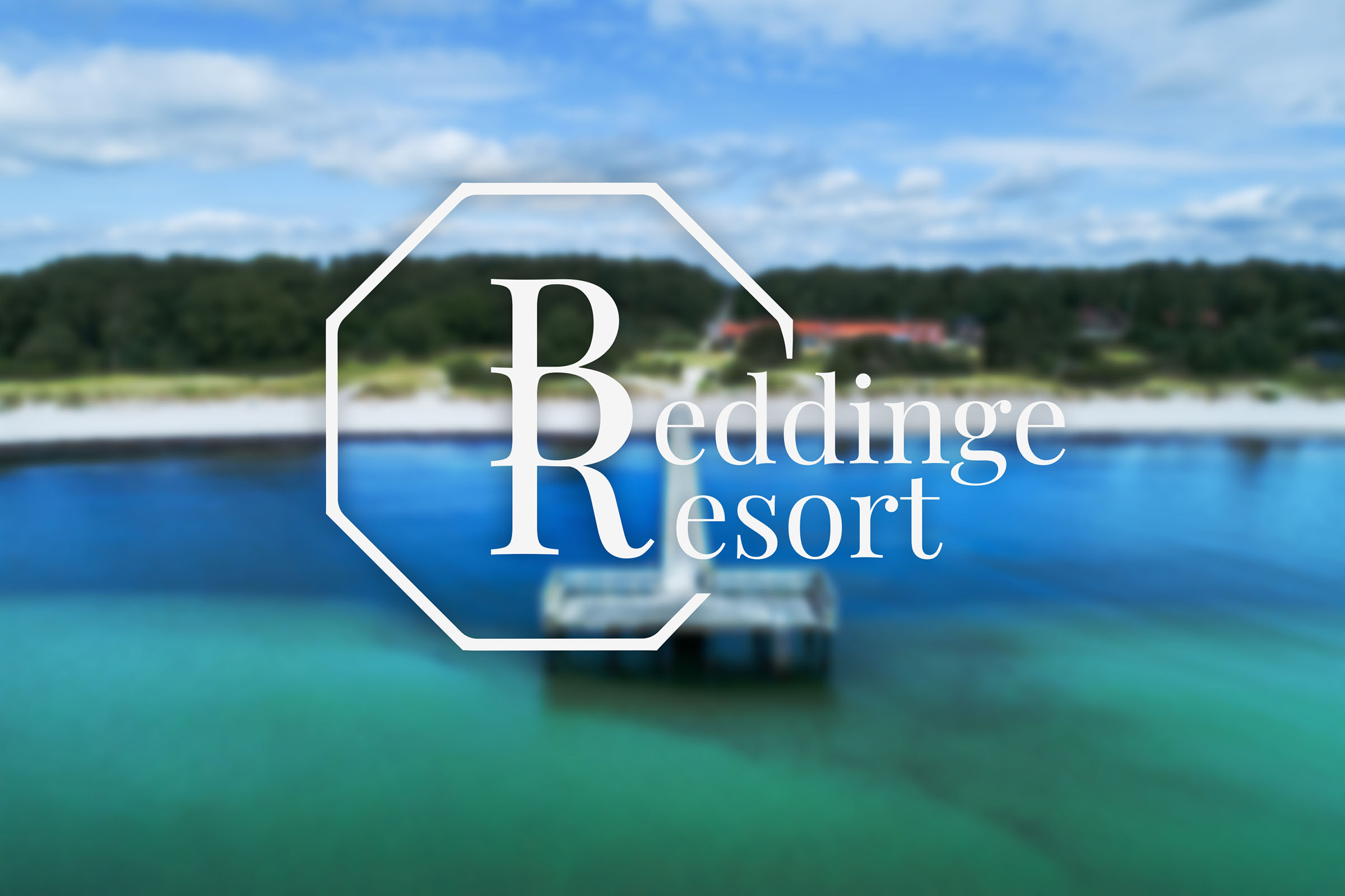
Vi lanserar webbsida och bokningsplattform för Beddinge Resort!
Plattformen fungerar som en bro mellan de boendes uthyrning och besökarnas bokning. Man skulle kunna säga en hybrid mellan Airbnb och traditionella hotell.
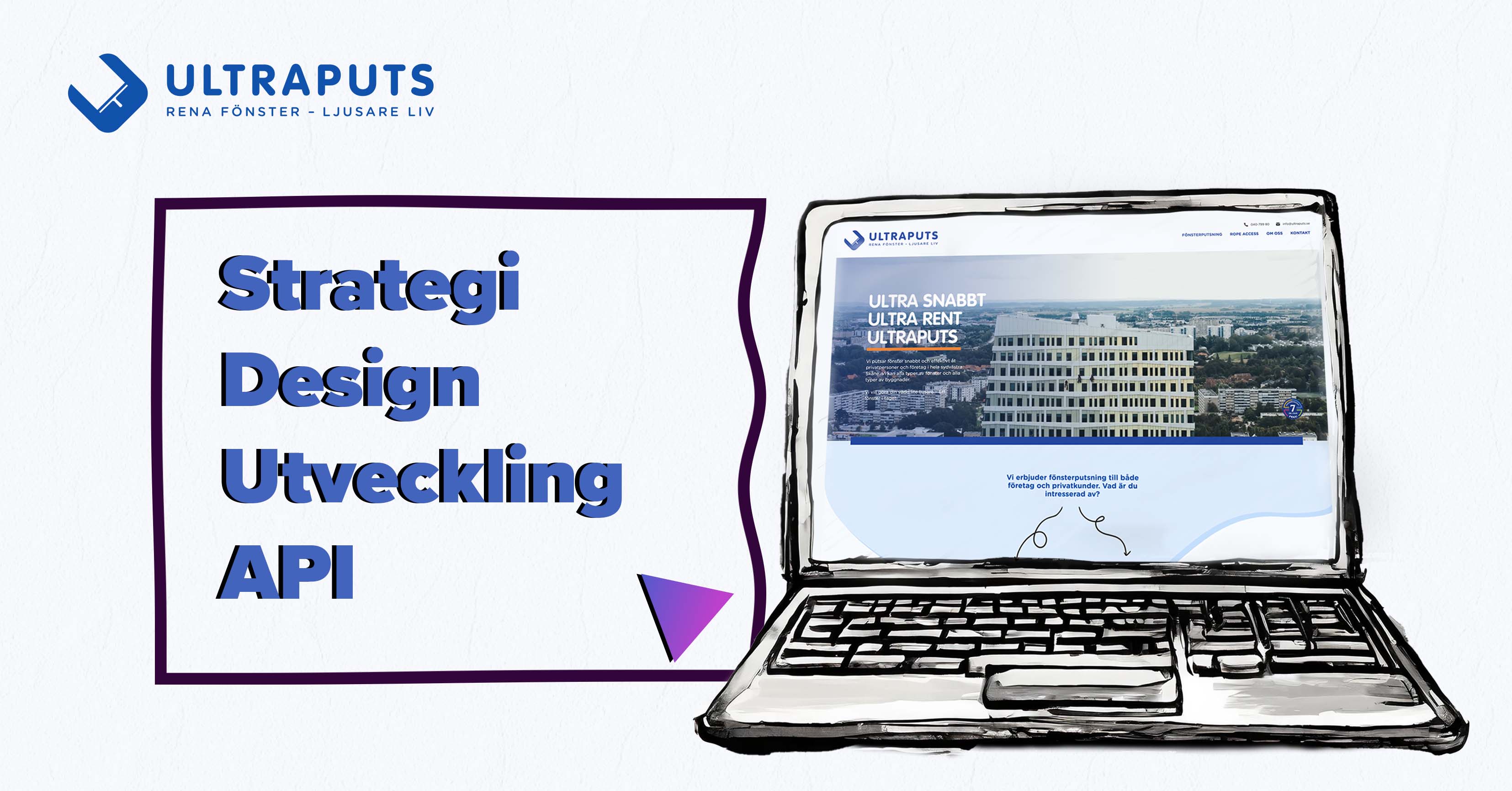
Vi lanserar Ultraputs nya webb
Efter att ha finslipat och färdigställt de sista detaljerna har vi nu lanserat Ultraputs nya webb. Strategi, Design, Uteckling och API koppling mot CRM-system.
- General Solutions
- Ruby On Rails
- Jackson (JSON Object Mapper)
- GSON (JSON Object Mapper)
- JSON-Lib (JSON Object Mapper)
- Flexjson (JSON Object Mapper)
- References and future reading
- Microservices Security
- Microservices based Security Arch Doc
- Mobile Application Security
- Multifactor Authentication
- NPM Security
- Network Segmentation
- NodeJS Docker
- Nodejs Security
- OS Command Injection Defense
- PHP Configuration
- Password Storage
- Prototype Pollution Prevention
- Query Parameterization
- REST Assessment
- REST Security
- Ruby on Rails
- SAML Security
- SQL Injection Prevention
- Secrets Management
- Secure Cloud Architecture
- Secure Product Design
- Securing Cascading Style Sheets
- Server Side Request Forgery Prevention
- Session Management
- TLS Cipher String
- Third Party Javascript Management
- Threat Modeling
- Transaction Authorization
- Transport Layer Protection
- Transport Layer Security
- Unvalidated Redirects and Forwards
- User Privacy Protection
- Virtual Patching
- Vulnerability Disclosure
- Vulnerable Dependency Management
- Web Service Security
- XML External Entity Prevention
- XML Security
- XSS Filter Evasion
Mass Assignment Cheat Sheet ¶
Introduction ¶, definition ¶.
Software frameworks sometime allow developers to automatically bind HTTP request parameters into program code variables or objects to make using that framework easier on developers. This can sometimes cause harm.
Attackers can sometimes use this methodology to create new parameters that the developer never intended which in turn creates or overwrites new variable or objects in program code that was not intended.
This is called a Mass Assignment vulnerability.
Alternative Names ¶
Depending on the language/framework in question, this vulnerability can have several alternative names :
- Mass Assignment: Ruby on Rails, NodeJS.
- Autobinding: Spring MVC, ASP NET MVC.
- Object injection: PHP.
Example ¶
Suppose there is a form for editing a user's account information:
Here is the object that the form is binding to:
Here is the controller handling the request:
Here is the typical request:
And here is the exploit in which we set the value of the attribute isAdmin of the instance of the class User :
Exploitability ¶
This functionality becomes exploitable when:
- Attacker can guess common sensitive fields.
- Attacker has access to source code and can review the models for sensitive fields.
- AND the object with sensitive fields has an empty constructor.
GitHub case study ¶
In 2012, GitHub was hacked using mass assignment. A user was able to upload his public key to any organization and thus make any subsequent changes in their repositories. GitHub's Blog Post .
Solutions ¶
- Allow-list the bindable, non-sensitive fields.
- Block-list the non-bindable, sensitive fields.
- Use Data Transfer Objects (DTOs).
General Solutions ¶
An architectural approach is to create Data Transfer Objects and avoid binding input directly to domain objects. Only the fields that are meant to be editable by the user are included in the DTO.
Language & Framework specific solutions ¶
Spring mvc ¶, allow-listing ¶.
Take a look here for the documentation.
Block-listing ¶
Nodejs + mongoose ¶, ruby on rails ¶, django ¶, asp net ¶, php laravel + eloquent ¶, grails ¶, play ¶, jackson (json object mapper) ¶.
Take a look here and here for the documentation.
GSON (JSON Object Mapper) ¶
Take a look here and here for the document.
JSON-Lib (JSON Object Mapper) ¶
Flexjson (json object mapper) ¶, references and future reading ¶.
- Mass Assignment, Rails and You
Tech Blogger Jenn

Understanding Mass Assignment in Laravel

Mass Assignment is when you use the create method to "save" a new model using a single PHP statement.
The model instance is returned using the create method.
Before using the create method you need to specify either a fillable or guarded property on your modal to avoid mass assignment vulnerabilities.
Fillable attributes are those that the User can make or change. Guarded attributes are those that the User cannot make or change.
A mass assignment vulnerability occurs when a user passes an unexpected HTTP request field and that field changes a column in your database that you did not expect. For example, a malicious user might escalate his role to an administrator by sending an is_admin parameter through an HTTP request. This will then be passed to your model's create method.
So you need to define which model attributes you want to make mass assignable. You do this by using the $fillable property in the model. for example let's make the name, and destination of the FLIGHT model mass assignable.
If there are any attributes you would not want to be changed by the user then put them in the
Once you have specified which attributes are mass-assignable, you may use the create method to insert a new record in the database. The create method returns the newly created model instance:
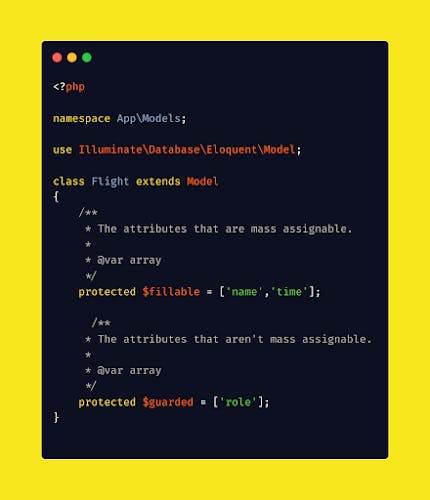
Find tweet here
Press ESC to close
Or check our popular categories....
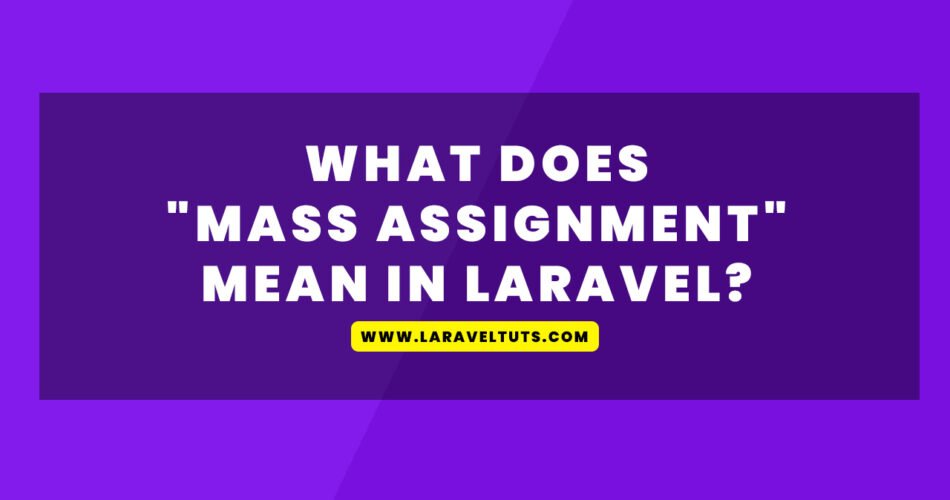
What Does “Mass Assignment” Mean in Laravel?
Today we are going to learn What Does “Mass Assignment” Mean in Laravel? When working with Laravel, a popular PHP framework, developers often encounter the term “Mass Assignment.”
This feature provides a convenient way to handle multiple attribute assignments in a single operation, saving time and effort.
In this article, we will delve into the concept of mass assignment in Laravel and explore how it can be effectively utilized.
Understanding Assignment in Laravel
In Laravel, assignment refers to setting the values of an object’s properties or attributes. For instance, consider a User model with properties like name, email, and password. Assigning values to each property individually can be laborious, especially when dealing with models that possess numerous attributes.
Streamlining Assignments with Mass Assignment
Mass assignment simplifies the process of setting multiple attributes of a model at once by passing an array of values. Laravel accomplishes this through the create() and update() methods provided by the Eloquent ORM.
Let’s examine how mass assignment works in practice. Suppose we have a User model with name, email, and password properties. To create a new user using mass assignment, we can employ the create() method as follows:
With a single line of code, we assign values to all three attributes and successfully create a new user record in the database. Laravel automatically maps the array keys to the corresponding model attributes, simplifying bulk assignments.
Controlling Mass Assignment with Fillable Attributes
While mass assignment provides convenience, it is vital to consider security implications. Certain attributes, like the password field, should not be directly mass assignable to avoid potential vulnerabilities. Laravel addresses this concern by introducing the fillable property within the model.
By defining an array of fillable attributes in the model class, developers can specify which attributes are allowed for mass assignment. For instance:
By employing the fillable property, Laravel ensures that only the listed attributes can be mass assigned, bolstering security. Attempting to assign a non-fillable attribute will result in a MassAssignmentException being thrown.
Additionally, Laravel offers the guarded property as the inverse of fillable . It allows developers to specify attributes that should be guarded against mass assignment. By defining the guarded property as an array of attributes, you can take the “blacklist” approach instead of the “whitelist” approach provided by fillable .
Updating Existing Records with Mass Assignment
Mass assignment is not restricted to creating new records; it can also be utilized for updating existing records. When updating a model, you can leverage the update() method and pass an array of attribute-value pairs. Here’s an example:
In this case, the specified attributes of the user with the ID of 1 will be updated in the database.
Final Thoughts
Mass assignment in Laravel is a powerful feature that simplifies working with models and database records. By allowing multiple attribute assignments in a single operation, developers can save time and effort.
However, it is crucial to be mindful of the security implications and appropriately handle which attributes can be mass assigned using the fillable or guarded property.
Understanding and utilizing mass assignment effectively can significantly enhance the efficiency of your Laravel development workflow.
Categorized in:
Share Article:
I am Nitin Pujari, a full-stack developer and . I created this site to bestow my coding experience with newbie programmers. I love to write on JavaScript, Php, Codeigniter, React, Angular, Vue, and Laravel.
Leave a Reply Cancel reply
Related articles, autocomplete search from database example – laravel 11, add qr code in pdf using dompdf laravel, laravel send welcome email after registration example, laravel convert string to lowercase example, other stories, how to set up file permissions for laravel 10, how to safely remove migration in laravel.
\n If you choose to unguard your model, you should take special care to always hand-craft the arrays passed to Eloquent's fill, create, and update methods: \n \n From Laravel's documentation (emphasis mine) \n In other words: make sure your data is safe first, then you are free to mass assign it. \n","strippedBody":"\nIf you choose to unguard your model, you should take special care to always hand-craft the arrays passed to Eloquent's fill, create, and update methods:\n\nFrom Laravel's documentation (emphasis mine)\nIn other words: make sure your data is safe first, then you are free to mass assign it.\n","bodyInMarkdown":"> If you choose to unguard your model, you should take special care to always **hand-craft** the arrays passed to Eloquent's fill, create, and update methods: \n\nFrom [Laravel's documentation](https:\/\/laravel.com\/docs\/8.x\/eloquent#allowing-mass-assignment) (emphasis mine)\n\nIn other words: make sure your data is safe first, then you are free to mass assign it.","path":"\/comments\/13886","relativePublishDate":"2 years ago","edited":false,"pinned":false,"user":{"id":130804,"username":"raaaahman","avatar":"\/\/unavatar.io\/github\/raaaahman","experience":{"award_count":"0","level":2,"points":"8,550","elite":false,"pointsUntilNextLevel":"1,450"},"achievements":[],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"raaaahman","website":"https:\/\/devindetails.com","employment":"","job_title":"Software Engineer","location":"Aix-en-Provence","flag":"fr","available_for_hire":0},"dateSegments":{"created_diff":"5 years ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@raaaahman"}},"likes":["xbrevi","RayS"],"links":{"like":"\/comments\/13886\/likes"}}],"likes":["Hashim"],"links":{"like":"\/comments\/12933\/likes"}},{"id":16204,"video_id":2011,"parent_id":null,"body":" well i'm new\nis there a problem when i update or create a new row in the table from say phpMyAdmin instead of useing tinker? \n","strippedBody":"well i'm new\nis there a problem when i update or create a new row in the table from say phpMyAdmin instead of useing tinker?\n","bodyInMarkdown":"well i'm new \nis there a problem when i update or create a new row in the table from say phpMyAdmin instead of useing tinker?\n","path":"\/comments\/16204","relativePublishDate":"2 years ago","edited":false,"pinned":false,"user":{"id":228575,"username":"uncle13x","avatar":"\/\/www.gravatar.com\/avatar\/52810e7629b575c9cf72755a596c8670?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-36.png","experience":{"award_count":"0","level":1,"points":"1,010","elite":false,"pointsUntilNextLevel":"3,990"},"achievements":[],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"2 years ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@uncle13x"}},"replies":[{"id":17564,"video_id":2011,"parent_id":16204,"body":" @uncle13x the difference is I think if you use tinker the timestamp (created_at & updated_at) is inserted automatically, while using phpmyadmin you need to insert timestamp manually. \n","strippedBody":"@uncle13x the difference is I think if you use tinker the timestamp (created_at & updated_at) is inserted automatically, while using phpmyadmin you need to insert timestamp manually.\n","bodyInMarkdown":"@uncle13x the difference is I think if you use tinker the timestamp (created_at & updated_at) is inserted automatically, while using phpmyadmin you need to insert timestamp manually.","path":"\/comments\/17564","relativePublishDate":"2 years ago","edited":false,"pinned":false,"user":{"id":199072,"username":"cyrdodi","avatar":"\/\/www.gravatar.com\/avatar\/bdec732e0bc083d32da03c1cfc17b8f9?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-23.png","experience":{"award_count":"0","level":3,"points":"13,580","elite":false,"pointsUntilNextLevel":"1,420"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"3 years ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@cyrdodi"}},"likes":["turanbalayev"],"links":{"like":"\/comments\/17564\/likes"}},{"id":24387,"video_id":2011,"parent_id":16204,"body":" @uncle13x Using thinker you can play with the code and test things like in this video (fill or guard properties of a model). But if you update your data using your phpAdmin you are bypassing code things because you are editing the data directly and not through the code. \n","strippedBody":"@uncle13x Using thinker you can play with the code and test things like in this video (fill or guard properties of a model). But if you update your data using your phpAdmin you are bypassing code things because you are editing the data directly and not through the code.\n","bodyInMarkdown":"@uncle13x Using thinker you can play with the code and test things like in this video (fill or guard properties of a model). But if you update your data using your phpAdmin you are bypassing code things because you are editing the data directly and not through the code.","path":"\/comments\/24387","relativePublishDate":"9 months ago","edited":false,"pinned":false,"user":{"id":258314,"username":"ssulbaran","avatar":"https:\/\/laracasts.nyc3.digitaloceanspaces.com\/members\/avatars\/258314.png?v=982","experience":{"award_count":"0","level":4,"points":"18,390","elite":false,"pointsUntilNextLevel":"1,610"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":true,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"@ssulbaranr","github":"","website":"","employment":"","job_title":"Software Engineer","location":"Argentina","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"1 year ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@ssulbaran"}},"likes":["edriso"],"links":{"like":"\/comments\/24387\/likes"}}],"likes":[],"links":{"like":"\/comments\/16204\/likes"}},{"id":18913,"video_id":2011,"parent_id":null,"body":" At 6:09 and onward, I believe you meant to say you should set $fillable to an empty array, not $guarded to an empty array. \n","strippedBody":"At 6:09 and onward, I believe you meant to say you should set $fillable to an empty array, not $guarded to an empty array.\n","bodyInMarkdown":"At 6:09 and onward, I believe you meant to say you should set $fillable to an empty array, not $guarded to an empty array. ","path":"\/comments\/18913","relativePublishDate":"1 year ago","edited":false,"pinned":false,"user":{"id":247495,"username":"spreadlove5683","avatar":"\/\/www.gravatar.com\/avatar\/ee76177d11febaaddd9a6fc62477cc8c?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-27.png","experience":{"award_count":"0","level":2,"points":"6,610","elite":false,"pointsUntilNextLevel":"3,390"},"achievements":[],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"1 year ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@spreadlove5683"}},"replies":[{"id":19092,"video_id":2011,"parent_id":18913,"body":" @spreadlove5683 Or he meant turn mass assignment protection off entirely and only use it when we know the array's value. \n","strippedBody":"@spreadlove5683 Or he meant turn mass assignment protection off entirely and only use it when we know the array's value.\n","bodyInMarkdown":"@spreadlove5683 Or he meant turn mass assignment protection off entirely and only use it when we know the array's value.","path":"\/comments\/19092","relativePublishDate":"1 year ago","edited":false,"pinned":false,"user":{"id":249913,"username":"littleduck","avatar":"\/\/www.gravatar.com\/avatar\/bf103307e2a8cee57ddfaecf3c83da17?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-20.png","experience":{"award_count":"0","level":2,"points":"5,750","elite":false,"pointsUntilNextLevel":"4,250"},"achievements":[],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"1 year ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@littleduck"}},"likes":[],"links":{"like":"\/comments\/19092\/likes"}}],"likes":["littleduck","RixzZ","parallax"],"links":{"like":"\/comments\/18913\/likes"}},{"id":21618,"video_id":2011,"parent_id":null,"body":" Good day sir Jeff, from philippines here ! :D I would like to ask why Category model don't have mass assign and Post model have it ? \n","strippedBody":"Good day sir Jeff, from philippines here ! :D I would like to ask why Category model don't have mass assign and Post model have it ?\n","bodyInMarkdown":"Good day sir Jeff, from philippines here ! :D I would like to ask why Category model don't have mass assign and Post model have it ? ","path":"\/comments\/21618","relativePublishDate":"1 year ago","edited":false,"pinned":false,"user":{"id":245781,"username":"Jessie2699","avatar":"\/\/www.gravatar.com\/avatar\/fc4884b47c06151d4a2a59f6a74fd4d7?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-18.png","experience":{"award_count":"0","level":2,"points":"5,430","elite":false,"pointsUntilNextLevel":"4,570"},"achievements":[],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"1 year ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@Jessie2699"}},"replies":[],"likes":[],"links":{"like":"\/comments\/21618\/likes"}},{"id":22830,"video_id":2011,"parent_id":null,"body":" @jeffreyway Thanks for your lessons. It 's possible to add subtitle to the videos of this series? I don't speek english very well and sometimes i don't understand your considerations \n","strippedBody":"@jeffreyway Thanks for your lessons. It 's possible to add subtitle to the videos of this series? I don't speek english very well and sometimes i don't understand your considerations\n","bodyInMarkdown":"@jeffreyway Thanks for your lessons. It 's possible to add subtitle to the videos of this series? I don't speek english very well and sometimes i don't understand your considerations","path":"\/comments\/22830","relativePublishDate":"1 year ago","edited":false,"pinned":false,"user":{"id":278364,"username":"Spartaseventy","avatar":"\/\/unavatar.io\/github\/Spartaseventy","experience":{"award_count":"0","level":5,"points":"20,310","elite":false,"pointsUntilNextLevel":"4,690"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":"Nicola Campaniolo","bio":null,"twitter":"","github":"Spartaseventy","website":"","employment":"","job_title":"Web developer","location":"Trapani","flag":"it","available_for_hire":0},"dateSegments":{"created_diff":"1 year ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@Spartaseventy"}},"replies":[],"likes":["ceortega"],"links":{"like":"\/comments\/22830\/likes"}},{"id":23274,"video_id":2011,"parent_id":null,"body":" I find the $guarded = []; part to be very confusing. You set it up as a way to prevent mass assignment entirely, but then you show that it doesn't protect against mass assignment at all, and that you have to just never mass write code that mass assigns. So what is the point of setting guarded to an empty array then? Is that just the equivalent of filling $fillable with all values? So you're basically just making it so that assignment rules dont get in the way of individual assignment? \n","strippedBody":"I find the $guarded = []; part to be very confusing. You set it up as a way to prevent mass assignment entirely, but then you show that it doesn't protect against mass assignment at all, and that you have to just never mass write code that mass assigns. So what is the point of setting guarded to an empty array then? Is that just the equivalent of filling $fillable with all values? So you're basically just making it so that assignment rules dont get in the way of individual assignment?\n","bodyInMarkdown":"I find the $guarded = []; part to be very confusing. You set it up as a way to prevent mass assignment entirely, but then you show that it doesn't protect against mass assignment at all, and that you have to just never mass write code that mass assigns. So what is the point of setting guarded to an empty array then? Is that just the equivalent of filling $fillable with all values? So you're basically just making it so that assignment rules dont get in the way of individual assignment? ","path":"\/comments\/23274","relativePublishDate":"1 year ago","edited":false,"pinned":false,"user":{"id":208917,"username":"JoeBetbeze","avatar":"\/\/www.gravatar.com\/avatar\/37a2bafa0a43257b6eae2334b75c76b1?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-34.png","experience":{"award_count":"0","level":3,"points":"13,580","elite":false,"pointsUntilNextLevel":"1,420"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":true,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"3 years ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@JoeBetbeze"}},"replies":[{"id":23341,"video_id":2011,"parent_id":23274,"body":" @JoeBetbeze it should be $guarded = ['*']; to stop mass assignment \n","strippedBody":"@JoeBetbeze it should be $guarded = ['*']; to stop mass assignment\n","bodyInMarkdown":"@JoeBetbeze it should be $guarded = ['*']; to stop mass assignment","path":"\/comments\/23341","relativePublishDate":"1 year ago","edited":false,"pinned":false,"user":{"id":286119,"username":"muramerci","avatar":"\/\/www.gravatar.com\/avatar\/0c447e5bc3899cc3d2f9c5b52f6fbc99?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-4.png","experience":{"award_count":"0","level":2,"points":"8,470","elite":false,"pointsUntilNextLevel":"1,530"},"achievements":[],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"1 year ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@muramerci"}},"likes":["JoeBetbeze"],"links":{"like":"\/comments\/23341\/likes"}},{"id":23354,"video_id":2011,"parent_id":23274,"body":" @muramerci Ahhh I see. Does an empty array do anything? \n Isn't it the equivalent of setting $fillable = ['*'] \n","strippedBody":"@muramerci Ahhh I see. Does an empty array do anything?\nIsn't it the equivalent of setting $fillable = ['*']\n","bodyInMarkdown":"@muramerci Ahhh I see. Does an empty array do anything? \n\nIsn't it the equivalent of setting $fillable = ['*']","path":"\/comments\/23354","relativePublishDate":"1 year ago","edited":true,"pinned":false,"user":{"id":208917,"username":"JoeBetbeze","avatar":"\/\/www.gravatar.com\/avatar\/37a2bafa0a43257b6eae2334b75c76b1?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-34.png","experience":{"award_count":"0","level":3,"points":"13,580","elite":false,"pointsUntilNextLevel":"1,420"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":true,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"3 years ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@JoeBetbeze"}},"likes":[],"links":{"like":"\/comments\/23354\/likes"}},{"id":24388,"video_id":2011,"parent_id":23274,"body":" @JoeBetbeze In one hand the $guard says to the model "everything in this list wont be fillable, protect them! but if it not here in this list, you can fill it". On the other hand with $fillable, it says to the model "Every field in the model cant be fill except those you specify in this list." (btw the list means the array). \n Thats why $guard=[] means every field is available to fill because you are telling the is no field to "protect". \n","strippedBody":"@JoeBetbeze In one hand the $guard says to the model "everything in this list wont be fillable, protect them! but if it not here in this list, you can fill it". On the other hand with $fillable, it says to the model "Every field in the model cant be fill except those you specify in this list." (btw the list means the array).\nThats why $guard=[] means every field is available to fill because you are telling the is no field to "protect".\n","bodyInMarkdown":"@JoeBetbeze In one hand the $guard says to the model \"everything in this list wont be fillable, protect them! but if it not here in this list, you can fill it\". On the other hand with $fillable, it says to the model \"Every field in the model cant be fill except those you specify in this list.\" (btw the list means the array).\n\nThats why $guard=[] means every field is available to fill because you are telling the is no field to \"protect\".","path":"\/comments\/24388","relativePublishDate":"9 months ago","edited":false,"pinned":false,"user":{"id":258314,"username":"ssulbaran","avatar":"https:\/\/laracasts.nyc3.digitaloceanspaces.com\/members\/avatars\/258314.png?v=982","experience":{"award_count":"0","level":4,"points":"18,390","elite":false,"pointsUntilNextLevel":"1,610"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":true,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"@ssulbaranr","github":"","website":"","employment":"","job_title":"Software Engineer","location":"Argentina","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"1 year ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@ssulbaran"}},"likes":["JoeBetbeze"],"links":{"like":"\/comments\/24388\/likes"}},{"id":24416,"video_id":2011,"parent_id":23274,"body":" @ssulbaran Thanks. Is there any point or have you ever seen anyone use both at the same time? \n For instance if you had user_id, name, title as columns, and then explicitely typed 2 of them in fillable and 1 in guarded? \n","strippedBody":"@ssulbaran Thanks. Is there any point or have you ever seen anyone use both at the same time?\nFor instance if you had user_id, name, title as columns, and then explicitely typed 2 of them in fillable and 1 in guarded?\n","bodyInMarkdown":"@ssulbaran Thanks. Is there any point or have you ever seen anyone use both at the same time? \n\nFor instance if you had user_id, name, title as columns, and then explicitely typed 2 of them in fillable and 1 in guarded? ","path":"\/comments\/24416","relativePublishDate":"9 months ago","edited":false,"pinned":false,"user":{"id":208917,"username":"JoeBetbeze","avatar":"\/\/www.gravatar.com\/avatar\/37a2bafa0a43257b6eae2334b75c76b1?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-34.png","experience":{"award_count":"0","level":3,"points":"13,580","elite":false,"pointsUntilNextLevel":"1,420"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":true,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"3 years ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@JoeBetbeze"}},"likes":[],"links":{"like":"\/comments\/24416\/likes"}},{"id":24551,"video_id":2011,"parent_id":23274,"body":" @JoeBetbeze \n To put it simply: $guarded = [] (i.e. empty) tells Laravel that you do not want it to protect the app you are designing from mass assignment vulnerabilities. In other words, you are fully taking on the responsibility yourself of preventing anything from being mass-assignable. \n So Jeffrey is showing us here how to use mass-assignment "within tinker" while developing an app\/site (a way to quickly whip up a new 'whatever you need'), but he is also showing us that the "best" way to avoid such potential vulnerabilities is to never use mass-assignment "in the app itself" with anything that is even remotely user generated or user editable. In other words, $guarded = [*] will certainly kill mass-assignment for anything in the app, but it will also prevent you from using mass-assignment within tinker, where it is strictly within full control. Tinker provides a massive productivity boost for developers, so you probably don't want to artificially limit its abilities that way. \n My own suggestion would be to use either a populated $fillable or populated $guarded property in your apps for however long it takes to gain full confidence in your ability to recognize mass-assignment-based code (at which point, maybe you then decide to turn off that protection with an empty $guarded array, so you can still use fully developer-controlled mass-assignment in tinker or elsewhere). \n In other words, be patient through the learning process, especially with yourself. Don't sweat the small stuff. And then of course there's Rule #2: It's ALL small stuff (if you allow it to be). ;) \n","strippedBody":"@JoeBetbeze\nTo put it simply: $guarded = [] (i.e. empty) tells Laravel that you do not want it to protect the app you are designing from mass assignment vulnerabilities. In other words, you are fully taking on the responsibility yourself of preventing anything from being mass-assignable.\nSo Jeffrey is showing us here how to use mass-assignment "within tinker" while developing an app\/site (a way to quickly whip up a new 'whatever you need'), but he is also showing us that the "best" way to avoid such potential vulnerabilities is to never use mass-assignment "in the app itself" with anything that is even remotely user generated or user editable. In other words, $guarded = [*] will certainly kill mass-assignment for anything in the app, but it will also prevent you from using mass-assignment within tinker, where it is strictly within full control. Tinker provides a massive productivity boost for developers, so you probably don't want to artificially limit its abilities that way.\nMy own suggestion would be to use either a populated $fillable or populated $guarded property in your apps for however long it takes to gain full confidence in your ability to recognize mass-assignment-based code (at which point, maybe you then decide to turn off that protection with an empty $guarded array, so you can still use fully developer-controlled mass-assignment in tinker or elsewhere).\nIn other words, be patient through the learning process, especially with yourself. Don't sweat the small stuff. And then of course there's Rule #2: It's ALL small stuff (if you allow it to be). ;)\n","bodyInMarkdown":"@JoeBetbeze \n\nTo put it simply: $guarded = [] (i.e. empty) tells Laravel that you do not want it to protect the app you are designing from mass assignment vulnerabilities. In other words, you are fully taking on the responsibility yourself of preventing anything from being mass-assignable.\n\nSo Jeffrey is showing us here how to use mass-assignment \"within tinker\" while developing an app\/site (a way to quickly whip up a new 'whatever you need'), but he is also showing us that the \"best\" way to avoid such potential vulnerabilities is to never use mass-assignment \"in the app itself\" with anything that is even remotely user generated or user editable. In other words, $guarded = [*] will certainly kill mass-assignment for anything in the app, but it will also prevent you from using mass-assignment within tinker, where it is strictly within full control. Tinker provides a massive productivity boost for developers, so you probably don't want to artificially limit its abilities that way.\n\nMy own suggestion would be to use either a populated $fillable or populated $guarded property in your apps for however long it takes to gain full confidence in your ability to recognize mass-assignment-based code (at which point, maybe you then decide to turn off that protection with an empty $guarded array, so you can still use fully developer-controlled mass-assignment in tinker or elsewhere).\n\nIn other words, be patient through the learning process, especially with yourself. Don't sweat the small stuff. And then of course there's Rule #2: It's **ALL** small stuff (if you allow it to be). ;)","path":"\/comments\/24551","relativePublishDate":"9 months ago","edited":true,"pinned":false,"user":{"id":150183,"username":"RayS","avatar":"\/\/unavatar.io\/github\/LTIOfficial","experience":{"award_count":"0","level":45,"points":"222,070","elite":false,"pointsUntilNextLevel":"2,930"},"achievements":[{"id":9,"name":"Laracasts Master","description":"Earned once 1000 Laracasts lessons have been completed.","level":"advanced","icon":"laracasts-master.svg"},{"id":11,"name":"Laracasts Veteran","description":"Earned once your experience points passes 100,000.","level":"intermediate","icon":"laracasts-veteran.svg"},{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":true,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":"Ray Seeger","bio":"I'm the founder\/developer of Linguistic Team International, the all-volunteer translation house for The Venus Project & Zeitgeist Movement.","twitter":"","github":"LTIOfficial","website":"https:\/\/thevenusproject.com","employment":"Earth","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"4 years ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@RayS"}},"likes":["codelando"],"links":{"like":"\/comments\/24551\/likes"}}],"likes":["parallax","RayS","johnloydlao"],"links":{"like":"\/comments\/23274\/likes"}},{"id":25544,"video_id":2011,"parent_id":null,"body":" I found $guarded = [] confusing, and based on the other comments I'm not alone. I believe the confusion is when he says "disable this feature" it's not clear that he is referring to mass assignment protection . I was under the assumption that it completely disabled mass assignment so it was confusing that he then shows it still working. \n","strippedBody":"I found $guarded = [] confusing, and based on the other comments I'm not alone. I believe the confusion is when he says "disable this feature" it's not clear that he is referring to mass assignment protection. I was under the assumption that it completely disabled mass assignment so it was confusing that he then shows it still working.\n","bodyInMarkdown":"I found `$guarded = []` confusing, and based on the other comments I'm not alone. I believe the confusion is when he says \"disable this feature\" it's not clear that he is referring to mass assignment _protection_. I was under the assumption that it completely disabled _mass assignment_ so it was confusing that he then shows it still working.","path":"\/comments\/25544","relativePublishDate":"7 months ago","edited":false,"pinned":false,"user":{"id":53721,"username":"sirmontegu","avatar":"\/\/www.gravatar.com\/avatar\/8ed5eebbf019b84031d15febe168cd27?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-17.png","experience":{"award_count":"0","level":7,"points":"33,910","elite":false,"pointsUntilNextLevel":"1,090"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":true,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"7 years ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@sirmontegu"}},"replies":[],"likes":[],"links":{"like":"\/comments\/25544\/likes"}},{"id":25683,"video_id":2011,"parent_id":null,"body":" So utterly confused about what is the proper thing to do here. Some elaboration on this would be helpful. \n","strippedBody":"So utterly confused about what is the proper thing to do here. Some elaboration on this would be helpful.\n","bodyInMarkdown":"So utterly confused about what is the proper thing to do here. Some elaboration on this would be helpful.","path":"\/comments\/25683","relativePublishDate":"6 months ago","edited":false,"pinned":false,"user":{"id":289703,"username":"tripp","avatar":"\/\/www.gravatar.com\/avatar\/a419ef437ced3aba62eba0a4106eaf02?s=100&d=https%3A%2F%2Fs3.amazonaws.com%2Flaracasts%2Fimages%2Fforum%2Favatars%2Fdefault-avatar-9.png","experience":{"award_count":"0","level":2,"points":"5,010","elite":false,"pointsUntilNextLevel":"4,990"},"achievements":[],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":null,"bio":null,"twitter":"","github":"","website":"","employment":"","job_title":"Software Engineer","location":"","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"1 year ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@tripp"}},"replies":[{"id":27586,"video_id":2011,"parent_id":25683,"body":" @tripp As Jeffrey said, there is no proper way here, I may add if you work for yourself you can choose the approach that suits you, and if you're working in a company\/team you follow their practice \n","strippedBody":"@tripp As Jeffrey said, there is no proper way here, I may add if you work for yourself you can choose the approach that suits you, and if you're working in a company\/team you follow their practice\n","bodyInMarkdown":"@tripp As Jeffrey said, there is no proper way here, I may add if you work for yourself you can choose the approach that suits you, and if you're working in a company\/team you follow their practice","path":"\/comments\/27586","relativePublishDate":"3 months ago","edited":true,"pinned":false,"user":{"id":322905,"username":"ailyye","avatar":"\/\/unavatar.io\/github\/ilyes-msr","experience":{"award_count":"0","level":4,"points":"15,300","elite":false,"pointsUntilNextLevel":"4,700"},"achievements":[{"id":16,"name":"Ten Thousand Strong","description":"Earned once your experience points hits 10,000.","level":"intermediate","icon":"ten-thousand-strong.svg"}],"reported":null,"staff":false,"subscribed":false,"ai":false,"elite":0,"leaderboardPosition":null,"profile":{"full_name":"Ali Ilyes Mansour","bio":"Web Developer","twitter":"AliIlyesMansour","github":"ilyes-msr","website":"","employment":"","job_title":"Software Engineer","location":"Algiers, Algeria","flag":null,"available_for_hire":0},"dateSegments":{"created_diff":"9 months ago"},"settings":{"show_profile":true},"links":{"profile":"https:\/\/laracasts.com\/@ailyye"}},"likes":[],"links":{"like":"\/comments\/27586\/likes"}}],"likes":[],"links":{"like":"\/comments\/25683\/likes"}}],"links":{"first":"https:\/\/laracasts.com\/series\/laravel-8-from-scratch\/episodes\/22?page=1","last":"https:\/\/laracasts.com\/series\/laravel-8-from-scratch\/episodes\/22?page=1","prev":null,"next":null},"meta":{"current_page":1,"from":1,"last_page":1,"links":[{"url":null,"label":"« Previous","active":false},{"url":"https:\/\/laracasts.com\/series\/laravel-8-from-scratch\/episodes\/22?page=1","label":"1","active":true},{"url":null,"label":"More »","active":false}],"path":"https:\/\/laracasts.com\/series\/laravel-8-from-scratch\/episodes\/22","per_page":15,"to":12,"total":12},"mentionableUsernames":[],"newestCommentId":[]},"position":22},"url":"\/series\/laravel-8-from-scratch\/episodes\/22","version":"adf6249ef1a61d73731015142bc82fb8"}" data-v-app> Series Overview Laravel 8 From Scratch I Prerequisites and Setup 01 An Animated Introduction to MVC Episode 1 2m 40s 02 Initial Environment Setup and Composer Episode 2 5m 51s 03 The Laravel Installer Tool Episode 3 2m 25s 04 Why Do We Use Tools Episode 4 2m 37s II The Basics 05 How a Route Loads a View Episode 5 4m 32s 06 Include CSS and JavaScript Episode 6 2m 54s 07 Make a Route and Link to it Episode 7 6m 25s 08 Store Blog Posts as HTML Files Episode 8 8m 09s 09 Route Wildcard Constraints Episode 9 3m 59s 10 Use Caching for Expensive Operations Episode 10 4m 10s 11 Use the Filesystem Class to Read a Directory Episode 11 14m 00s 12 Find a Composer Package for Post Metadata Episode 12 16m 19s 13 Collection Sorting and Caching Refresher Episode 13 4m 51s III Blade 14 Blade: The Absolute Basics Episode 14 7m 56s 15 Blade Layouts Two Ways Episode 15 8m 05s 16 A Few Tweaks and Consideration Episode 16 3m 50s IV Working With Databases 17 Environment Files and Database Connections Episode 17 4m 46s 18 Migrations: The Absolute Basics Episode 18 7m 13s 19 Eloquent and the Active Record Pattern Episode 19 6m 16s 20 Make a Post Model and Migration Episode 20 6m 34s 21 Eloquent Updates and HTML Escaping Episode 21 4m 42s 3 Ways to Mitigate Mass Assignment Vulnerabilities Episode 22 8m 54s 23 Route Model Binding Episode 23 5m 29s 24 Your First Eloquent Relationship Episode 24 8m 26s 25 Show All Posts Associated With a Category Episode 25 5m 09s 26 Clockwork, and the N+1 Problem Episode 26 4m 40s 27 Database Seeding Saves Time Episode 27 9m 42s 28 Turbo Boost With Factories Episode 28 10m 45s 29 View All Posts By An Author Episode 29 8m 59s 30 Eager Load Relationships on an Existing Model Episode 30 4m 17s V Integrate the Design 31 Convert the HTML and CSS to Blade Episode 31 9m 52s 32 Blade Components and CSS Grids Episode 32 14m 12s 33 Convert the Blog Post Page Episode 33 5m 37s 34 A Small JavaScript Dropdown Detour Episode 34 21m 11s 35 How to Extract a Dropdown Blade Component Episode 35 12m 04s 36 Quick Tweaks and Clean-Up Episode 36 6m 42s VI Search 37 Search (The Messy Way) Episode 37 5m 04s 38 Search (The Cleaner Way) Episode 38 9m 51s VII Filtering 39 Advanced Eloquent Query Constraints Episode 39 11m 39s 40 Extract a Category Dropdown Blade Component Episode 40 5m 13s 41 Author Filtering Episode 41 4m 24s 42 Merge Category and Search Queries Episode 42 7m 22s 43 Fix a Confusing Eloquent Query Bug Episode 43 3m 59s VIII Pagination 44 Laughably Simple Pagination Episode 44 11m 40s IX Forms and Authentication 45 Build a Register User Page Episode 45 18m 03s 46 Automatic Password Hashing With Mutators Episode 46 6m 24s 47 Failed Validation and Old Input Data Episode 47 9m 52s 48 Show a Success Flash Message Episode 48 6m 54s 49 Login and Logout Episode 49 10m 53s 50 Build the Log In Page Episode 50 11m 01s 51 Laravel Breeze Quick Peek Episode 51 8m 59s X Comments 52 Write the Markup for a Post Comment Episode 52 6m 43s 53 Table Consistency and Foreign Key Constraints Episode 53 7m 37s 54 Make the Comments Section Dynamic Episode 54 8m 41s 55 Design the Comment Form Episode 55 8m 36s 56 Activate the Comment Form Episode 56 12m 36s 57 Some Light Chapter Clean Up Episode 57 4m 48s Newsletters and APIs 58 Mailchimp API Tinkering Episode 58 10m 33s 59 Make the Newsletter Form Work Episode 59 4m 17s 60 Extract a Newsletter Service Episode 60 10m 35s 61 Toy Chests and Contracts Episode 61 19m 19s Admin Section 62 Limit Access to Only Admins Episode 62 8m 36s 63 Create the Publish Post Form Episode 63 11m 27s 64 Validate and Store Post Thumbnails Episode 64 10m 51s 65 Extract Form-Specific Blade Components Episode 65 9m 37s 66 Extend the Admin Layout Episode 66 14m 54s 67 Create a Form to Edit and Delete Posts Episode 67 20m 28s 68 Group and Store Validation Logic Episode 68 7m 35s 69 All About Authorization Episode 69 13m 49s Conclusion 70 Goodbye and Next Steps Episode 70 2m 22s // find anything Sign In Get Started for Free 3 Ways to Mitigate Mass A... Episode 22 This series has been archived. We instead recommend: 30 Days to Learn Laravel. A series is marked as archived when the content is no longer up-to-date or relevant to most viewers. Dedicated Server: AMD-Technologie eignen sich ideal für anspruchsvolle Workloads und rechenintensiven Anforderungen ads via Carbon Toggle Episode List Laravel 8 From Scratch — Ep 22 3 Ways to Mitigate Mass Assignment Vulnerabilities Episode 22 Run Time 8m 54s Published Apr 14th, 2021 Topic Laravel Version Laravel 8 Mark as Complete Add to Watchlist Jeffrey Way Your Instructor Today Visit Website Hi, I'm Jeffrey. I'm the creator of Laracasts and spend most of my days building the site and thinking of new ways to teach confusing concepts. I live in Orlando, Florida with my wife and two kids. About This Episode Published Apr 14th, 2021 In this lesson, we'll discuss everything you need to know about mass assignment vulnerabilities. As you'll see, Laravel provides a couple ways to specify which attributes may or may not be mass assigned. However, there's a third option at the conclusion of this video that is equally valid. Download this video. Download series wallpaper. Discuss This Lesson Write a reply. Level 14 Hlaing_Min_Than Posted 3 years ago Ten Thousand Strong Earned once your experience points hits 10,000. this series should come out when i've learned laravel 6 haha.this steps were so tough when i was learned.nowadays,jeff can make it easy.. good job 9 Reply Level 33 neilstee Posted 3 years ago Laracasts Tutor Earned once your "Best Reply" award count is 100 or more. Chatty Cathy Earned once you have achieved 500 forum replies. Laracasts Veteran Earned once your experience points passes 100,000. Ten Thousand Strong Earned once your experience points hits 10,000. I think all Laravel developers will agree that they had experienced this Mass Assignment issue in their lives and up to this date 😅 I honestly like the $guarded approach because you can easily see what columns should always be protected for Mass Assignments alongside a validated data from Validator (double protection!) 13 Reply Level 2 Liakat2889 Posted 10 months ago @neilstee Hey, If I use guarded what is the best of below: protected $guarded = []; protected $guarded = ['*']; protected $guarded = ['id']; protected $guarded = ['id','created_at','updated_at']; 1 Reply Level 45 Subscriber RayS Posted 9 months ago Laracasts Master Earned once 1000 Laracasts lessons have been completed. Laracasts Veteran Earned once your experience points passes 100,000. Ten Thousand Strong Earned once your experience points hits 10,000. @Liakat2889 Like most things, it very much depends on the details/context. If we only talk about the fields in this example blog, and you choose to populate the $guarded array, then protected $guarded = [id]; is probably sufficient. But now imagine that the blog you are developing allows users to choose 'when' to publicly publish their own posts, so you might have something like an additional $table->timestamp('published_at')->nullable(); field in your database migration and you don't want any users potentially messing with anyone's published_at dates. In that case, you might want to add that to your $guarded fields. protected $guarded = ['id', 'published_at']; As for `protected $guarded = [*];, that would have the same result are an empty $fillable array. In other words, nothing would be able to get through to the database via mass-assignment, even if it was otherwise fully protected from user input/editing. Having said all of that, keep in mind that Jeffrey has not yet covered how to verify/validate/sanitize user input data so that it cannot do the kinds of things that both $fillable and $guarded are designed to help protect against. That information should help everyone much better understand why some developers choose to turn this Laravel feature off with an empty array on the guarded property. I Hope some of this helps, brother. :) 2 Reply Level 7 Subscriber kurucu Posted 3 years ago Ten Thousand Strong Earned once your experience points hits 10,000. Slightly off topic (very off topic) but the tools, themes etc used for these videos are so clean. I know they are chosen for specifically that reason (it's a tutorial after all), but it might be quite nice to allow the teacher to capture the tools, themes, or other setup being used in the video blurb? Any ideas what Jeffrey's using as a terminal and editor here, and how he's set them up? More on topic: I prefer to use "fillable". Yes, it's a pain sometimes but I'd prefer a little developer pain over a hole in my security later. If I do miss an attribute, it's caught during testing and easy to remedy. It's much harder to prove (without an audit) that all requests are validated and filtered for appropriate attributes, for every unguarded mass assignment. 4 Reply Level 50 Subscriber JeffreyWay Laracasts Owner | Posted 3 years ago Laracasts Tutor Earned once your "Best Reply" award count is 100 or more. Top 50 Earned once your experience points ranks in the top 50 of all Laracasts users. Chatty Cathy Earned once you have achieved 500 forum replies. Laracasts Veteran Earned once your experience points passes 100,000. Ten Thousand Strong Earned once your experience points hits 10,000. Have a look here: https://laracasts.com/uses In this series, I'm using: PHPStorm with the Nord theme and almost every hidden (menu bars, etc.) iTerm 11 Reply Level 1 zerokool110 Posted 2 years ago iTerm changed my life! hahaha. jokes aside. it changed my life! 0 Reply Level 5 Subscriber wondarar Posted 3 years ago Ten Thousand Strong Earned once your experience points hits 10,000. While following I thought I got it but when we reach the end of this chapter I suddenly don't know what to do. What is best practice for a newbie like me? I'm building my blog following Jeff by each chapter and the only one posting / creating a blog post will be me. So I assume the right one is fillable with the properties (name, excerpt, ....)? What is best practive for other forms, like contact/message, comment....? I'm confused... 4 Reply Level 50 Subscriber JeffreyWay Laracasts Owner | Posted 3 years ago Laracasts Tutor Earned once your "Best Reply" award count is 100 or more. Top 50 Earned once your experience points ranks in the top 50 of all Laracasts users. Chatty Cathy Earned once you have achieved 500 forum replies. Laracasts Veteran Earned once your experience points passes 100,000. Ten Thousand Strong Earned once your experience points hits 10,000. My point in the video is there is no best or right way to do it. Some teams insist on being explicit about your fillable attributes, while others disable it entirely. What I generally do is disable mass assignment protection entirely, and then make an internal rule that we never pass request()->all() to an Eloquent query method. 8 Reply Level 3 Hashim Posted 2 years ago Ten Thousand Strong Earned once your experience points hits 10,000. So to clarify the create() method is vulnerable to mass execution vulns whereas the update() method is not? 1 Reply Level 4 ericknyoto Posted 2 years ago Ten Thousand Strong Earned once your experience points hits 10,000. I think that is the same. 1 Reply Level 2 raaaahman Posted 2 years ago If you choose to unguard your model, you should take special care to always hand-craft the arrays passed to Eloquent's fill, create, and update methods:
From Laravel's documentation (emphasis mine)
In other words: make sure your data is safe first, then you are free to mass assign it.

well i'm new is there a problem when i update or create a new row in the table from say phpMyAdmin instead of useing tinker?

Ten Thousand Strong
Earned once your experience points hits 10,000.
@uncle13x the difference is I think if you use tinker the timestamp (created_at & updated_at) is inserted automatically, while using phpmyadmin you need to insert timestamp manually.
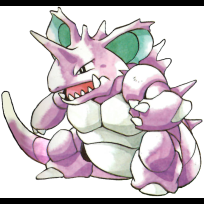
@uncle13x Using thinker you can play with the code and test things like in this video (fill or guard properties of a model). But if you update your data using your phpAdmin you are bypassing code things because you are editing the data directly and not through the code.

At 6:09 and onward, I believe you meant to say you should set $fillable to an empty array, not $guarded to an empty array.

@spreadlove5683 Or he meant turn mass assignment protection off entirely and only use it when we know the array's value.

Good day sir Jeff, from philippines here ! :D I would like to ask why Category model don't have mass assign and Post model have it ?
@jeffreyway Thanks for your lessons. It 's possible to add subtitle to the videos of this series? I don't speek english very well and sometimes i don't understand your considerations

I find the $guarded = []; part to be very confusing. You set it up as a way to prevent mass assignment entirely, but then you show that it doesn't protect against mass assignment at all, and that you have to just never mass write code that mass assigns. So what is the point of setting guarded to an empty array then? Is that just the equivalent of filling $fillable with all values? So you're basically just making it so that assignment rules dont get in the way of individual assignment?

@JoeBetbeze it should be $guarded = ['*']; to stop mass assignment
@muramerci Ahhh I see. Does an empty array do anything?
Isn't it the equivalent of setting $fillable = ['*']
@JoeBetbeze In one hand the $guard says to the model "everything in this list wont be fillable, protect them! but if it not here in this list, you can fill it". On the other hand with $fillable, it says to the model "Every field in the model cant be fill except those you specify in this list." (btw the list means the array).
Thats why $guard=[] means every field is available to fill because you are telling the is no field to "protect".
@ssulbaran Thanks. Is there any point or have you ever seen anyone use both at the same time?
For instance if you had user_id, name, title as columns, and then explicitely typed 2 of them in fillable and 1 in guarded?
Laracasts Master
Earned once 1000 Laracasts lessons have been completed.
Laracasts Veteran
Earned once your experience points passes 100,000.
@JoeBetbeze
To put it simply: $guarded = [] (i.e. empty) tells Laravel that you do not want it to protect the app you are designing from mass assignment vulnerabilities. In other words, you are fully taking on the responsibility yourself of preventing anything from being mass-assignable.
So Jeffrey is showing us here how to use mass-assignment "within tinker" while developing an app/site (a way to quickly whip up a new 'whatever you need'), but he is also showing us that the "best" way to avoid such potential vulnerabilities is to never use mass-assignment "in the app itself" with anything that is even remotely user generated or user editable. In other words, $guarded = [*] will certainly kill mass-assignment for anything in the app, but it will also prevent you from using mass-assignment within tinker, where it is strictly within full control. Tinker provides a massive productivity boost for developers, so you probably don't want to artificially limit its abilities that way.
My own suggestion would be to use either a populated $fillable or populated $guarded property in your apps for however long it takes to gain full confidence in your ability to recognize mass-assignment-based code (at which point, maybe you then decide to turn off that protection with an empty $guarded array, so you can still use fully developer-controlled mass-assignment in tinker or elsewhere).
In other words, be patient through the learning process, especially with yourself. Don't sweat the small stuff. And then of course there's Rule #2: It's ALL small stuff (if you allow it to be). ;)

I found $guarded = [] confusing, and based on the other comments I'm not alone. I believe the confusion is when he says "disable this feature" it's not clear that he is referring to mass assignment protection . I was under the assumption that it completely disabled mass assignment so it was confusing that he then shows it still working.

So utterly confused about what is the proper thing to do here. Some elaboration on this would be helpful.
@tripp As Jeffrey said, there is no proper way here, I may add if you work for yourself you can choose the approach that suits you, and if you're working in a company/team you follow their practice
Forgot Your Password?
Have a question.
Hey! If you have a code-related question, please instead use the forum. Or, if you'd like to sign up with Paypal, though we don't officially offer that option on the sign up page, you can manually pay for a year's subscription ($99) here , and we'll set you up right away!
- Home // is where the PHP is
- Topics // just browsing?
- Series // it's what you're here for
- Path // walk the path
- Larabits // got five minutes?
- Discussions // let it all out
Reply to Post
* You may use Markdown with GitHub-flavored code blocks.
Mass Assignment Vulnerabilities and Validation in Laravel
driesvints, elkdev liked this article
Introduction
The following article is an excerpt from my ebook Battle Ready Laravel , which is a guide to auditing, testing, fixing, and improving your Laravel applications.
Validation is a really important part of any web application and can help strengthen your app's security. But, it's also something that is often ignored or forgotten about (at least on the projects that I've audited and been brought on board to work on).
In this article, we're going to briefly look at different things to look out for when auditing your app's security, or adding new validation. We'll also look at how you can use " Enlightn " to detect potential mass assignment vulnerabilities.
Mass Assignment Analysis with Enlightn
What is enlightn.
A great tool that we can use to get insight into our project is Enlightn .
Enlightn is a CLI (command-line interface) application that you can run to get recommendations about how to improve the performance and security of your Laravel projects. Not only does it provide some static analysis tooling, it also performs dynamic analysis using tooling that was built specifically to analyse Laravel projects. So the results that it generates can be incredibly useful.
At the time of writing, Enlightn offers a free version and paid versions. The free version offers 64 checks, whereas the paid versions offer 128 checks.
One useful thing about Enlightn is that you can install it on your production servers (which is recommended by the official documentation) as well as your development environment. It doesn't incur any overhead on your application, so it shouldn't affect your project's performance and can provide additional analysis into your server's configuration.
Using the Mass Assignment Analyzer
One of the useful analysis that Enlightn performs is the "Mass Assignment Analyzer". It scans through your application's code to find potential mass assignment vulnerabilities.
Mass assignment vulnerabilities can be exploited by malicious users to change the state of data in your database that isn't meant to be changed. To understand this issue, let's take a quick look at a potential vulnerability that I have come across in projects in the past.
Assume that we have a User model that has several fields: id , name , email , password , is_admin , created_at , and updated_at .
Imagine our project's user interface has a form a user can use to update the user. The form only has two fields: name and password . The controller method to handle this form and update the user might like something like the following:
The code above would work , however it would be susceptible to being exploited. For example, if a malicious user tried passing an is_admin field in the request body, they would be able to change a field that wasn't supposed to be able to be changed. In this particular case, this could lead to a permission escalation vulnerability that would allow a non-admin to make themselves an admin. As you can imagine, this could cause data protection issues and could lead to user data being leaked.
The Enlightn documentation provides several examples of the types of mass assignment usages it can detect:
It's important to remember that this becomes less of an issue if you have the $fillable field defined on your models. For example, if we wanted to state that only the name and email fields could be updated when mass assigning values, we could update the user model to look like so:
This would mean that if a malicious user was to pass the is_admin field in the request, it would be ignored when trying to assign the value to the User model.
However, I would recommend completely avoiding using the all method when mass assigning and only ever use the validated , safe , or only methods on the Request . By doing this, you can always have confidence that you explicitly defined the fields that you're assigning. For example, if we wanted to update our controller to use only , it may look something like this:
If we want to update our code to use the validated or safe methods, we'll first want to create a form request class. In this example, we'll create a UpdateUserRequest :
We can change our controller method to use the form request by type hinting it in the update method's signature and use the validated method:
Alternatively, we can also use the safe method like so:
Checking Validation
When auditing your application, you'll want to check through each of your controller methods to ensure that the request data is correctly validated. As we've already covered in the Mass Assignment Analysis example that Enlightn provides, we know that all data that is used in our controller should be validated and that we should never trust data provided by a user.
Whenever you're checking a controller, you must ask yourself " Am I sure that this request data has been validated and is safe to store? ". As we briefly covered earlier, it's important to remember that client-side validation isn't a substitute for server-side validation; both should be used together. For example, in past projects I have seen no server-side validation for "date" fields because the form provides a date picker, so the original developer thought that this would be enough to deter users from sending any other data than a date. As a result, this meant that different types of data could be passed to this field that could potentially be stored in the database (either by mistake or maliciously).
Applying the Basic Rules
When validating a field, I try to apply these four types of rules as a bare minimum:
- Is the field required? - Are we expecting this field to always be present in the request? If so, we can apply the required rule. If not, we can use the nullable or sometimes rule.
- What data type is the field? - Are we expecting an email, string, integer, boolean, or file? If so, we can apply email , string , integer , boolean or files rules.
- Is there a minimum value (or length) this field can be? - For example, if we were adding a price filter for a product listing page, we wouldn't want to allow a user to set the price range to -1 and would want it to go no lower than 0.
- Is there a maximum field (or length) this field can be? - For example, if we have a "create" form for a product page, we might not want the titles to be any longer than 100 characters.
So you can think of your validation rules as being written using the following format:
By applying these rules, not only can it add some basic standard for your security measures, it can also improve the readability of the code and the quality of submitted data.
For example, let's assume that we have these fields in a request:
Although you might be able to guess what these fields are and their data types, you probably wouldn't be able to answer the 4 questions above for each one. However, if we were to apply the four questions to these fields and apply the necessary rules, the fields might look like so in our request:
Now that we've added these rules, we have more information about the four fields in our request and what to expect when working with them in our controllers. This can make development much easier for users that work on the code after you because they can have a clearer understanding of what the request contains.
Applying these four questions to all of your request fields can be extremely valuable. If you find any requests that don't already have this validation, or if you find any fields in a request missing any of these rules, I'd recommend adding them. But it's worth noting that these are only a bare minimum and that in a majority of cases you'll want to use extra rules to ensure more safety.
Checking for Empty Validation
An issue I've found quite often with projects that I have audited is the use of empty rules for validating a field. To understand this a little better, let's take a look at a basic example of a controller that is storing a user in the database:
The store method in the UserController is taking the validated data (in this case, the name and email ), creating a user with them, then returning a redirect response to the users 'show' page. There isn't any problem with this so far.
However, let's say that this was originally written several months ago and that we now want to add a new twitter_handle field. In some projects that I have audited, I have come across fields added to the validation but without any rules applied like so:
This means that the twitter_handle field can now be passed in the request and stored. This can be a dangerous move because it circumvents the purpose of validating the data before it is stored.
It is possible that the developer did this so that they could build the feature quickly and then forgot to add the rules before committing their code. It may also be possible that the developer didn't think it was necessary. However, as we've covered, all data should be validated on the server-side so that we're always sure the data we're working with is acceptable.
If you come across empty validation rule sets like this, you will likely want to add the rules to make sure the field is covered. You may also want to check the database to ensure that no invalid data has been stored.
Hopefully, this post should have given you a brief insight into some of the things to look for when auditing your Laravel application's validation.
If you enjoyed reading this post, I'd love to hear about it. Likewise, if you have any feedback to improve the future ones, I'd also love to hear that too.
You might also be interested in checking out my 220+ page ebook " Battle Ready Laravel " which covers similar topics in more depth.
If you're interested in getting updated each time I publish a new post, feel free to sign up for my newsletter .
Keep on building awesome stuff! 🚀
Other articles you might like
Laravel under the hood - the strategy pattern.
Hello 👋 Wikipedia: In computer programming, the strategy pattern (also known as the policy patter...
How to become Fluent in Laravel
Fluency in Laravel can take several forms. One of them is using the Fluent class hidden inside the L...
Filter Eloquent models with multiple optional filters
Often we need to filter eloquent models when displaying to a view. If we have a small number of filt...
We'd like to thank these amazing companies for supporting us

Your logo here?
The Laravel portal for problem solving, knowledge sharing and community building.
The community

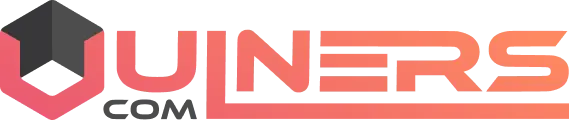
- Basic search
- Lucene search
- Search by product
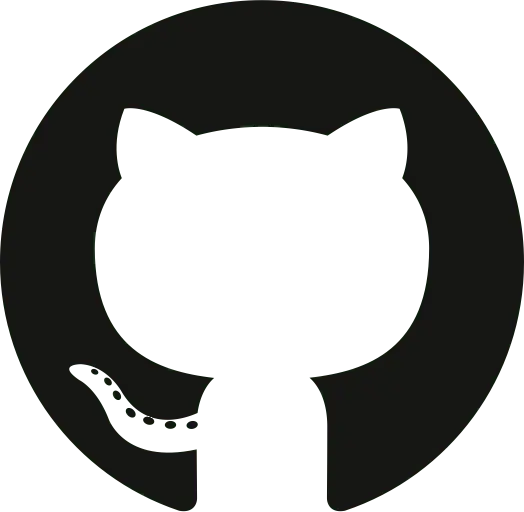
Laravel Risk of mass-assignment vulnerabilities
Laravel 4.1.29 improves the column quoting for all database drivers. This protects your application from some mass assignment vulnerabilities when not using the fillable property on models. If you are using the fillable property on your models to protect against mass assignment, your application is not vulnerable. However, if you are using guarded and are passing a user controlled array into an “update” or “save” type function, you should upgrade to 4.1.29 immediately as your application may be at risk of mass assignment.
github.com/advisories/GHSA-rj3w-99gc-8j58
github.com/FriendsOfPHP/security-advisories/blob/master/laravel/framework/2014-05-20.yaml
github.com/laravel/framework/commit/c942a1bc30999e3d7a5567f264bfc99c77843919
laravel.com/docs/5.3/upgrade#upgrade-4.1.29
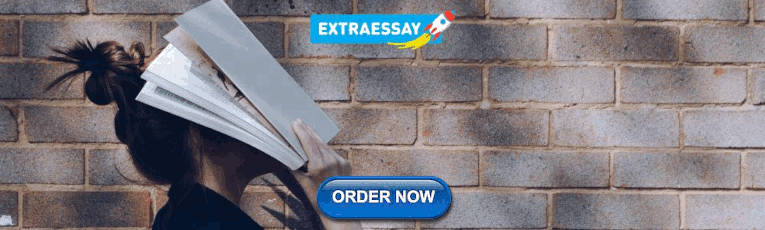
COMMENTS
39. Mass assignment is a process of sending an array of data that will be saved to the specified model at once. In general, you don't need to save data on your model on one by one basis, but rather in a single process. Mass assignment is good, but there are certain security problems behind it.
Laravel includes Eloquent, an object-relational mapper (ORM) that makes it enjoyable to interact with your database. When using Eloquent, each database table has a corresponding "Model" that is used to interact with that table. ... To learn more about mass assignment, please consult the mass assignment documentation. Updates. The save method ...
Since Professional Ajax, 1st Edition, I've been blessed to take part in other book projects: Professional Ajax 2nd Edition, and Beginning JavaScript 3rd and 4th editions. We can use mass assignment to easily create and update records in the database. It is dangerous, however, so in this lesson I'll teach you how to use mass assignment safely.
Laravel wants to ensure you're aware of this potential issue. Luckily, we have two solutions to change this default behavior. One solution is to use the fillable property, which is an array of fields that you want to allow for mass assignment
Laravel Eloquent ORM provides a simple API to work with database. It is an implementation of Active Record pattern. Each database table is mapped to a Model class which is used for interacting with that table.. Mass Assignment Let's break down this word. Mass means large number; Assignment means the assignment operator in programming; In order to make developers life easier, Eloquent ORM ...
Mass assignment is a Laravel feature that allows you to assign multiple attributes to a model at once. This can be useful when saving data to the database, as it can save you time and effort.
Mass assignment in Laravel is a powerful feature that simplifies working with models and database records. By allowing multiple attribute assignments in a single operation, developers can save ...
Illuminate\Database\Eloquent\MassAssignmentException with message 'Add [title] to fillable property to allow mass assignment on [App\Models\Post].' How to allow Mass Assignment in Laravel: As Laravel doesn't support Mass assignment by default. So, if you need it, you can allow it in three different ways.
Mass Assignment is a powerful feature in Laravel that accelerates development by simplifying attribute assignment. However, developers must tread cautiously to avoid the potential pitfalls associated with security vulnerabilities, unintended overwrites, and bypassing critical business logic.
Mass Assignment Cheat Sheet is a concise guide to help developers prevent and mitigate the risks of mass assignment vulnerabilities in web applications. It covers the definition, impact, detection, and prevention of this common security flaw. Learn how to protect your data from unauthorized manipulation with OWASP best practices.
Laravel Form ExplainationThis Laravel tutorial teaches you the fundamentals of Laravel 10🌟 Premium Laravel Course 🌟New and Updated In Depth Laravel 10 cour...
Understanding Mass Assignment in Laravel. Jenniline Ebai · Nov 26, 2022 · 2 min read. Mass Assignment is when you use the create method to "save" a new model using a single PHP statement. The model instance is returned using the create method.
Short Version. Mass assignment is when you send an array of values to be saved all in on go, rather than go through each value one by one. This saves up development time, looks more elegant and requires fewer lines of code. This also opens up to mass assignment vulnerabilities which allows a user to pass unintended request fields which may be ...
Mass assignment simplifies the process of setting multiple attributes of a model at once by passing an array of values. Laravel accomplishes this through the create() and update() methods provided by the Eloquent ORM. Let's examine how mass assignment works in practice. Suppose we have a User model with name, email, and password properties.
Published Apr 14th, 2021. In this lesson, we'll discuss everything you need to know about mass assignment vulnerabilities. As you'll see, Laravel provides a couple ways to specify which attributes may or may not be mass assigned. However, there's a third option at the conclusion of this video that is equally valid. Download this video.
Using the Mass Assignment Analyzer. One of the useful analysis that Enlightn performs is the "Mass Assignment Analyzer". It scans through your application's code to find potential mass assignment vulnerabilities. Mass assignment vulnerabilities can be exploited by malicious users to change the state of data in your database that isn't meant to ...
Without mass assignment protection a user who knows about the underlying application architecture could inject values into fields they're not expected to have access to, e.g if your User field has an is_admin value they could change their is_admin to 1 instead of 0. Mass assignment protection is only required when working with unsanitized user ...
Mass Assignment Vulnerabilities in Laravel Applications. Eloquent like many other ORMs have a nice feature that allows assigning properties to an object without having to assign each value ...
📺 Checkout https://tallpad.com for more videos
Notice we just added parts to the fillable property, and then used Eloquent's attribute setting hook set[Field]Attribute() to perform the assignment. This makes it possible to have the Car api look about right:
Laravel 4.1.29 improves the column quoting for all database drivers. This protects your application from some mass assignment vulnerabilities when not using the fillable property on models. If you are using the fillable property on your models to protect against mass assignment, your application is not vulnerable.
mass-assignment; laravel-models; Share. Improve this question. Follow edited Mar 20, 2022 at 6:39. usersuser. asked Mar 20, 2022 at 5:11. usersuser usersuser. 167 1 1 silver badge 14 14 bronze badges. Add a comment | 1 Answer Sorted by: Reset to default ...