Go by Example : Multiple Return Values
Next example: Variadic Functions .
by Mark McGranaghan and Eli Bendersky | source | license
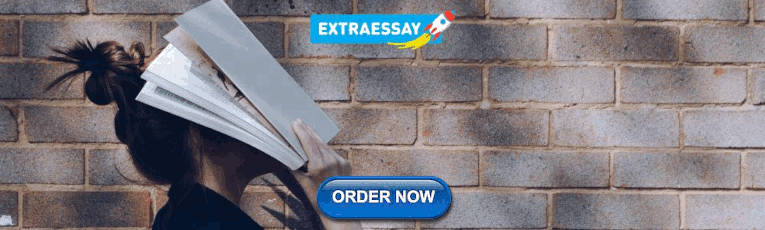
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
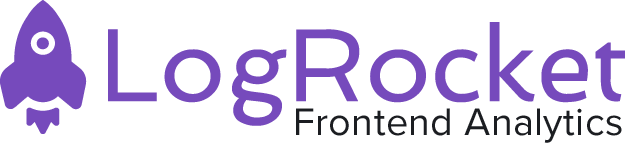
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
Error handling in Go: Best practices
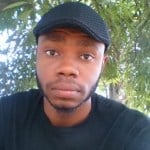
Editor’s note : This article was last updated and validated for accuracy on 18 November 2022.
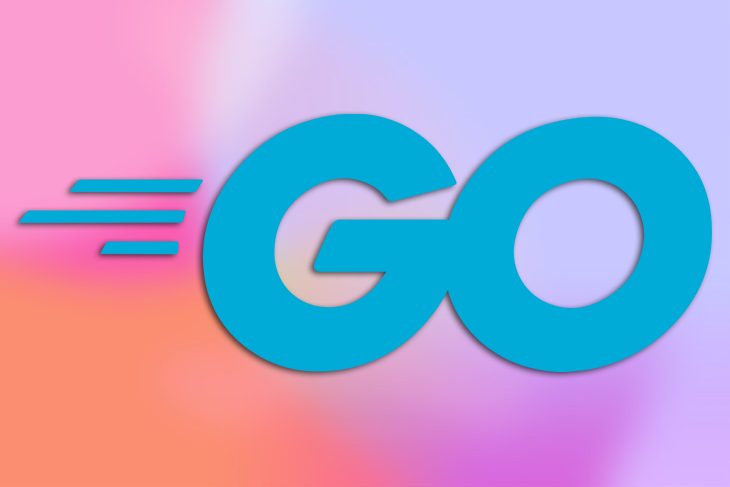
Tackling errors in Go requires a different approach than the conventional methods in other mainstream programming languages, like JavaScript, which uses the try...catch statement, or Python with its try… except block. Oftentimes, developers misapply Go’s features for error handling.
In this article, we’ll consider the best practices that you should follow when handling errors in a Go application. To follow along with this article, you’ll need a basic understanding of how Go works . Let’s get started!
The blank identifier
Handling errors through multiple return values, defer, panic, and recover, error wrapping.
The blank identifier is an anonymous placeholder. You can use it just like any other identifier in a declaration, but it does not introduce a binding. The blank identifier provides a way to ignore left-handed values in an assignment and avoid compiler errors surrounding unused imports and variables in a program.
Assigning errors to the blank identifier instead of properly handling them is unsafe, meaning you have decided to explicitly ignore the value of the defined function:
The rationale behind this is likely that you’re not expecting an error from the function. However, this could create cascading effects in your program. The best practice is to handle errors whenever you can.
One way to handle errors is to take advantage of the fact that functions in Go support multiple return values. Therefore, you can pass an error variable alongside the result of the function you’re defining:
In the code snippet above, we have to return the predefined error variable if we think there’s a chance that our function may fail. error is an interface type declared in Go’s built-in package , and its zero value is nil :
Usually, returning an error means that there is a problem, and returning nil means there were no errors:
Whenever the iterate function is called and err is not equal to nil , the error returned should be handled appropriately.
One option could be to create an instance of a retry or a cleanup mechanism. The only drawback with handling errors this way is that there’s no enforcement from Go’s compiler. You have to decide how the function you created returns the error.
You could define an error struct and place it in the position of the returned values. One way to do this is by using the built-in errorString struct. You can also find the code below in Go’s source code :
In the code sample above, errorString embeds a string , which is returned by the Error method. To create a custom error, you’ll have to define your error struct and use method sets to associate a function to your struct:
The newly created custom error can then be restructured to use the built-in error struct:
One limitation of the built-in error struct is that it does not come with stack traces, making it very difficult to locate where an error occurred. The error could pass through a number of functions before being printed out.
To handle this, you could install the pkg/errors package, which provides basic error handling primitives like stack trace recording, error wrapping, unwrapping, and formatting. To install this package, run the command below in your terminal:
When you need to add stack traces or any other information to make debugging your errors easier, use the New or Errorf functions to provide errors that record your stack trace. Errorf implements the fmt.Formatter interface, which lets you format your errors using the fmt package runes, %s , %v , and %+v :
To print stack traces instead of a plain error message, you have to use %+v instead of %v in the format pattern. The stack traces will look similar to the code sample below:
Although Go doesn’t have exceptions, it has a similar type of mechanism known as defer, panic, and recover . Go’s ideology is that adding exceptions like the try/catch/finally statement in JavaScript would result in complex code and encourage programmers to label too many basic errors, like failing to open a file, as exceptional.
You should not use defer/panic/recover as you would throw/catch/finally . You should reserve it only in cases of unexpected, unrecoverable failure.
Defer is a language mechanism that puts your function call into a stack. Each deferred function is executed in reverse order when the host function finishes, regardless of whether a panic is called or not. The defer mechanism is very useful for cleaning up resources:
The code above would compile as follows:
panic is a built-in function that stops the normal execution flow. When you call panic in your code, it means you’ve decided that your caller can’t solve the problem. Therefore, you should use panic only in rare cases where it’s not safe for your code or anyone integrating your code to continue at that point.
The code sample below demonstrates how panic works:
The sample above would compile as follows:
As shown above, when panic is used and not handled, the execution flow stops, all deferred functions are executed in reverse order, and stack traces are printed.
You can use the built-in recover function to handle panic and return the values passed from a panic call. recover must always be called in a defer function, otherwise, it will return nil :
As you can see in the code sample above, recover prevents the entire execution flow from coming to a halt. We added in a panic function, so the compiler would return the following:
To report an error as a return value, you have to call the recover function in the same goroutine as where the panic function is called, retrieve an error struct from the recover function, and pass it to a variable:
Every deferred function will be executed after a function call but before a return statement. Therefore, you can set a returned variable before a return statement is executed. The code sample above would compile as follows:
Previously, error wrapping in Go was only accessible via packages like pkg/errors . However, Go v1.13 introduced support for error wrapping .
According to the release notes :
An error e can wrap another error w by providing an Unwrap method that returns w . Both e and w are available to programs, allowing e to provide additional context to w or to reinterpret it while still allowing programs to make decisions based on w .
To create wrapped errors, fmt.Errorf has a %w verb, and for inspecting and unwrapping errors, a couple of functions have been added to the error package.
errors.Unwrap basically inspects and exposes the underlying errors in a program. It returns the result of calling the Unwrap method on Err if Err ’s type contains an Unwrap method returning an error. Otherwise, Unwrap returns nil:
Below is an example implementation of the Unwrap method:
With the errors.Is function, you can compare an error value against the sentinel value.
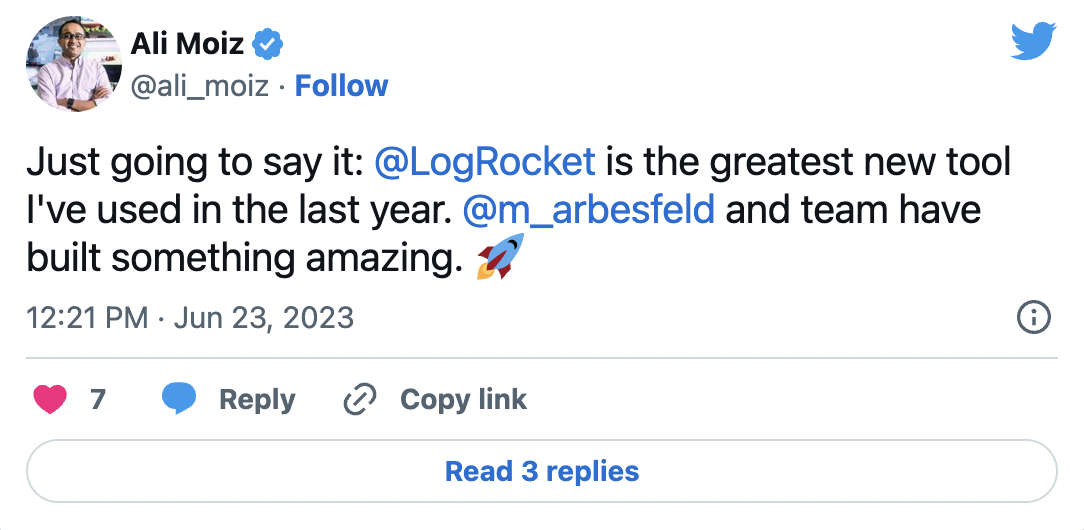
Over 200k developers use LogRocket to create better digital experiences

Instead of comparing the sentinel value to one error, this function compares it to every error in the error chain. It also implements an Is method on an error so that an error can post itself as a sentinel even though it’s not a sentinel value:
In the basic implementation above, Is checks and reports if err or any of the errors in its chain are equal to the target, the sentinel value.
The errors.As function provides a way to cast to a specific error type. It looks for the first error in the error chain that matches the sentinel value, and if found, it sets the sentinel value to that error value, returning true :
You can find this code in Go’s source code . Below is the compiler result:
An error matches the sentinel value if the error’s concrete value is assignable to the value pointed to by the sentinel value. As will panic if the sentinel value is not a non-nil pointer to either a type that implements error or to any interface type. As returns false if err is nil .
The Go community has been making impressive strides as of late with support for various programming concepts and introducing even more concise and easy ways to handle errors. Do you have any ideas on how to handle or work with errors that may appear in your Go program? Let me know in the comments below.
Get set up with LogRocket's modern error tracking in minutes:
- Visit https://logrocket.com/signup/ to get an app ID
Install LogRocket via npm or script tag. LogRocket.init() must be called client-side, not server-side
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
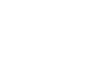
Stop guessing about your digital experience with LogRocket
Recent posts:.
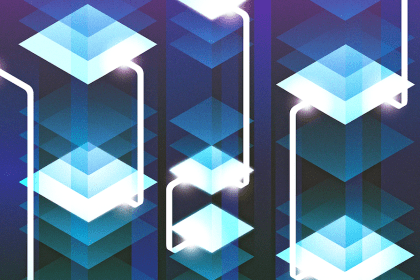
Understanding the CSS revert-layer keyword
In this article, we’ll explore CSS cascade layers — and, specifically, the revert-layer keyword — to help you refine your styling strategy.
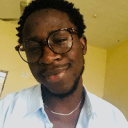
Exploring Nushell, a Rust-powered, cross-platform shell
Nushell is a modern, performant, extensible shell built with Rust. Explore its pros, cons, and how to install and get started with it.
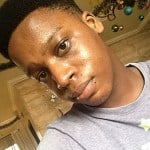
Exploring Zed, an open source code editor written in Rust
The Zed code editor sets itself apart with its lightning-fast performance and cutting-edge collaborative features.
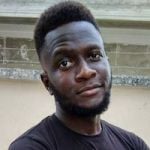
Implementing infinite scroll in Next.js with Server Actions
Infinite scrolling in Next.js no longer requires external libraries — Server Actions let us fetch initial data directly on the server.
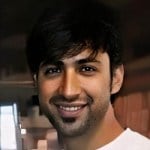
Leave a Reply Cancel reply
[SOLVED] Getting this error messge using go get hugo
go get -u -v github.com/spf13/hugo github.com/spf13/hugo (download) github.com/spf13/hugo/parser
github.com/spf13/hugo/parser
…/go/src/github.com/spf13/hugo/parser/frontmatter.go:55: assignment count mismatch: 2 = 1
Solved this myself by deleting entire folder contents of /go/src/github.com and then go getting.
This issue is closed.
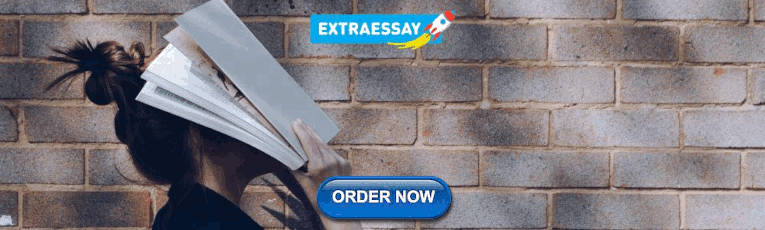
Related Topics
More than 3 years have passed since last update.
Golangで未使用の変数エラーを消す方法
stringconv.Atoi などのerrを二つ目の返り値として返してくれる関数を利用する際、errに代入した後使用しないと実行時にエラーが発生します。
しかしだからといって二つの返り値を変数に格納しないと、割り当てが間違っているという別のエラーが返却されることになります。
そのため必ず返り値を変数に代入する必要があるのですが、未使用エラーを発生させない必要があります。
このような状況の場合、 _ (アンダースコア)を利用するとエラーが発生しなくなります。
これはGo公式では Blank identifier という名称になっているようです。
Register as a new user and use Qiita more conveniently
- You get articles that match your needs
- You can efficiently read back useful information
- You can use dark theme
Source file test / fixedbugs / issue30087.go
View as plain text
Help | Advanced Search
Computer Science > Computer Vision and Pattern Recognition
Title: finematch: aspect-based fine-grained image and text mismatch detection and correction.
Abstract: Recent progress in large-scale pre-training has led to the development of advanced vision-language models (VLMs) with remarkable proficiency in comprehending and generating multimodal content. Despite the impressive ability to perform complex reasoning for VLMs, current models often struggle to effectively and precisely capture the compositional information on both the image and text sides. To address this, we propose FineMatch, a new aspect-based fine-grained text and image matching benchmark, focusing on text and image mismatch detection and correction. This benchmark introduces a novel task for boosting and evaluating the VLMs' compositionality for aspect-based fine-grained text and image matching. In this task, models are required to identify mismatched aspect phrases within a caption, determine the aspect's class, and propose corrections for an image-text pair that may contain between 0 and 3 mismatches. To evaluate the models' performance on this new task, we propose a new evaluation metric named ITM-IoU for which our experiments show a high correlation to human evaluation. In addition, we also provide a comprehensive experimental analysis of existing mainstream VLMs, including fully supervised learning and in-context learning settings. We have found that models trained on FineMatch demonstrate enhanced proficiency in detecting fine-grained text and image mismatches. Moreover, models (e.g., GPT-4V, Gemini Pro Vision) with strong abilities to perform multimodal in-context learning are not as skilled at fine-grained compositional image and text matching analysis. With FineMatch, we are able to build a system for text-to-image generation hallucination detection and correction.
Submission history
Access paper:.
- Other Formats

References & Citations
- Google Scholar
- Semantic Scholar
BibTeX formatted citation

Bibliographic and Citation Tools
Code, data and media associated with this article, recommenders and search tools.
- Institution
arXivLabs: experimental projects with community collaborators
arXivLabs is a framework that allows collaborators to develop and share new arXiv features directly on our website.
Both individuals and organizations that work with arXivLabs have embraced and accepted our values of openness, community, excellence, and user data privacy. arXiv is committed to these values and only works with partners that adhere to them.
Have an idea for a project that will add value for arXiv's community? Learn more about arXivLabs .
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
False positive Assignment count mismatch #2554
ignatov commented Jun 6, 2016
dlsniper commented Jun 6, 2016
Sorry, something went wrong.
dlsniper commented Jun 14, 2016
No branches or pull requests
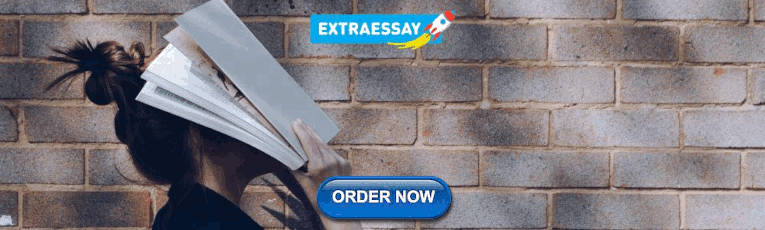
IMAGES
VIDEO
COMMENTS
./main.go:11:5 assignment mismatch: 2 variables but uuid.NewV4() returns 1 values In the environment where I encounter this problem, in Visual Studio Code when I hover with mouse over the call to uuid.NewV4() I see: func uuid.NewV4() (uuid.UUID, error) uuid.NewV4 on pkg.go.dev NewV4 returns random generated UUID. and hover over uuid shows:
The issue is this: Christophe_Meessen: func2() returns three values, and you assign the returned values of func2 to two variables. func2 expects three variables. If you post the definition of func2 we can point out the specific location where this is happening, but it might be more useful for the long term to go through the Go tour as Jakob ...
What version of Go are you using (go version)? $ go version go version go1.15.7 linux/amd64 Does this issue reproduce with the latest release? go1.15.7 is the latest stable at this time. ... assignment mismatch: 1 variables but right side has 2 values What did you see instead? An incorrect statement about a helper function. I arrived at this ...
% cat /tmp/x.go package p func f() string func _() { x := f(), 1, 2 } % go tool compile /tmp/x.go /tmp/x.go:6:4: assignment mismatch: 1 variables but f returns 3 values % Narrator: f does not return 3 values. Been this way back to Go 1.1...
Here we use the 2 different return values from the call with multiple assignment. a, b:= vals fmt. Println (a) fmt. Println (b) If you only want a subset of the returned values, use the blank identifier _. _, c:= vals fmt. Println (c)} $ go run multiple-return-values.go 3 7 7: Accepting a variable number of arguments is another nice feature of ...
# command-line-arguments ./reserved.go:4:6: assignment mismatch: 2 variables but 1 values tip gives -./reserved.go:4:6: assignment mismatch: 2 variable but 1 values This was introduced in commit ae9c822. Author: David Heuschmann [email protected] Date: Sat Sep 15 13:04:59 2018 +0200.
The blank identifier provides a way to ignore left-handed values in an assignment and avoid compiler errors surrounding unused imports and variables in a program. ... Go's ideology is that adding exceptions like the try/catch/finally statement in JavaScript would result in complex code and encourage programmers to label too many basic errors, ...
Learn and network with Go developers from around the world. Go blog The Go project's official blog. Go project ... b // ERROR "assignment mismatch: 1 variable but 2 values" 12 _ = a, b, c // ERROR "assignment mismatch: 1 variable but 3 values" 13 14 _, ...
Assignment Mismatch in Golang | Common Mistake in Golang | Dr Vipin ClassesAbout this video: In this video, I explained about following topics: 1. How to so...
Keep in mind that the Go compiler has no idea about what's going on in your SQL code, so it must be talking about your Go code. That gives you more of a clue about what it's complaining about in that case (i.e. because for one, it doesn't know your query is only returning one field, it's just a string like any other to the Go compiler; and two ...
I don't think the Go compiler is trying to prevent you from doing certain things like ignoring errors. It's just trying to make you do so intentionally. This, to me, seems intentional. ... ./prog.go:12:11: assignment mismatch: 1 variable but executeUpdate returns 2 values. Why doesn't my hello example fail to compile with the same type of ...
Saved searches Use saved searches to filter your results more quickly
Solved this myself by deleting entire folder contents of /go/src/github.com and then go getting. This issue is closed.
しかしだからといって二つの返り値を変数に格納しないと、割り当てが間違っているという別のエラーが返却されることになります。. # command-line-arguments. ./x_cubic.go:13:7: assignment mismatch: 1 variable but strconv.Atoi returns 2 values. そのため必ず返り値を変数に代入する ...
Learn and network with Go developers from around the world. Go blog ... { 10 var a, b = 1 // ERROR "assignment mismatch: 2 variables but 1 value|wrong number of initializations|cannot initialize" 11 _ = 1, 2 // ERROR "assignment mismatch: 1 variable but 2 values |number ...
Saved searches Use saved searches to filter your results more quickly
Reinforcement Learning From Human Feedback (RLHF) has been a critical to the success of the latest generation of generative AI models. In response to the complex nature of the classical RLHF pipeline, direct alignment algorithms such as Direct Preference Optimization (DPO) have emerged as an alternative approach. Although DPO solves the same objective as the standard RLHF setup, there is a ...
Thanks for contributing an answer to Stack Overflow! Please be sure to answer the question.Provide details and share your research! But avoid …. Asking for help, clarification, or responding to other answers.
Recent progress in large-scale pre-training has led to the development of advanced vision-language models (VLMs) with remarkable proficiency in comprehending and generating multimodal content. Despite the impressive ability to perform complex reasoning for VLMs, current models often struggle to effectively and precisely capture the compositional information on both the image and text sides. To ...
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Compile packages and dependencies. Usage: go build [-o output] [-i] [build flags] [packages] Build compiles the packages named by the import paths, along with their dependencies, but it does not install the results. If the arguments to build are a list of .go files, build treats them as a list of source files specifying a single package.