Static Variables in Java – Why and How to Use Static Methods

Static variables and static methods are two important concepts in Java.
Whenever a variable is declared as static, this means there is only one copy of it for the entire class, rather than each instance having its own copy. A static method means it can be called without creating an instance of the class.
Static variables and methods in Java provide several advantages, including memory efficiency, global access, object independence, performance, and code organization.
In this article, you will learn how static variables work in Java, as well as why and how to use static methods.
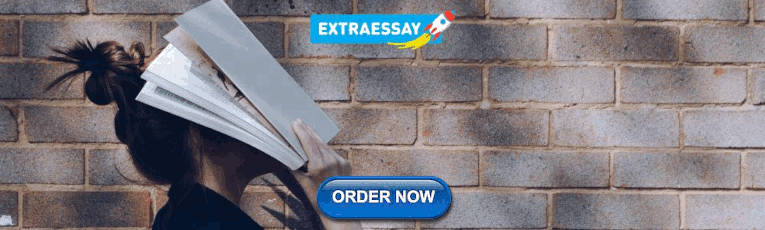
The Static Keyword in Java
The static keyword is one of the most essential features in the Java programming language. We use it to define class-level variables and methods.
Here is an example of how to use the static keyword:
As you can see above, we declared the count variable as a static variable, while we declared the printCount method as a static method.
When a variable is declared static in Java programming, it means that the variable belongs to the class itself rather than to any specific instance of the class. This means that there is only one copy of the variable in memory, regardless of how many instances of the class are created.
Here's an example. Say we have a Department class that has a static variable called numberOfWorker . We declare and increment the static variable at the constructor level to show the value of the static variable whenever the class object is created.
The results of the above code show that as we create new Department objects, the static variable numberOfWorker retains its value.
When we print out the value of numberOfWorker in the console, we can see that it retains its value across all instances of the Department class. This is because there is only one copy of the variable in memory, and any changes to the variable will be reflected across all instances of the class.
We can also use the static keyword to define static methods.
Static methods are methods that belong to the class rather than to any specific instance of the class. Static methods can be called directly on the class itself without needing to create an instance of the class first. See the code below:
In the above code, the Calculation class has two static methods. The declared static methods can be called directly on the Calculation class without creating an instance of the class first. That is to say, you do not need to create an object of the Calculation class before you access the static add and multiply classes.
The main() method in Java is an example of a static method. The main() method is a special static method that is the entry point for Java applications. The Math class in Java also provides many static methods that perform mathematical operations.
The above code shows that the entry point for Java applications is a static method. It also shows that the max() method is a static method of the Math class and does not require an instance of the Math class to be created.
As you can see, static methods can be useful in providing utility functions that do not necessitate the creation of a class object.
The static keyword is a powerful tool in Java that can help solve many programming challenges. It aids in memory consumption management, improves code consistency, and helps speed up applications.
To prevent unforeseen issues from cropping up in the code, it is crucial to use the static keyword wisely and be aware of its limitations.
Code that relies heavily on static variables and methods can be harder to test because it introduces dependencies between different parts of the program. Static variables and methods can introduce hidden dependencies between different parts of the program, making it harder to reason about how changes in one part of the code might affect other parts.
Code that relies heavily on static variables can also be less flexible and harder to extend over time. Static variables can also lead to concurrency issues if multiple threads access and modify the same variable at the same time.
Lastly, if a static variable is not properly released or disposed of when it is no longer needed, it can lead to memory leaks and other performance issues over time.
By using static variables and methods appropriately, you can create efficient and maintainable code that will be easier to work with over time.
Happy coding!
Technical Writer and Software Developer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Static variable in c.
Last Updated on April 20, 2023 by Prepbytes
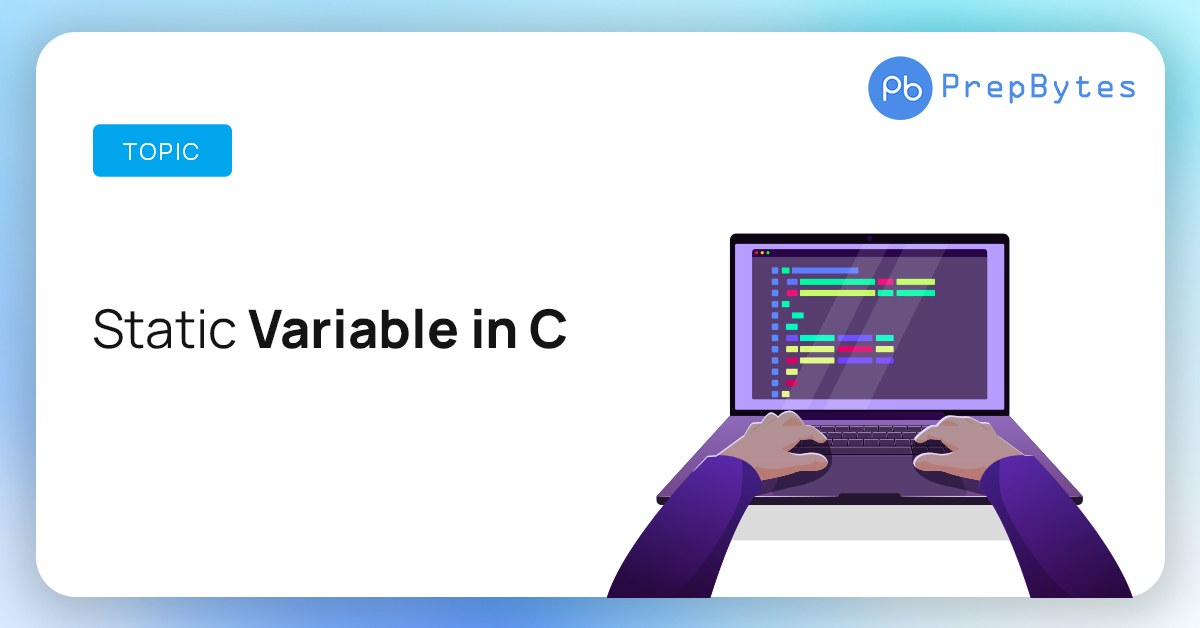
In the C programming language, variables play a crucial role in storing data values that can be used throughout the program. Among the different types of variables available, the static variable in C is one of the most commonly used ones. This article aims to provide a detailed overview of a static variable in C, including its syntax, properties of a static variable in C, differences from global variables, and some use cases of a static variable in C. So, without any further delay, let’s move on to our next section.
What is Static Variable in C?
A static variable in C is a special type of variable that has its value stored in memory for the entire lifespan of a program. The value of a static variable is initialized only once at the time of program loading and it retains its value until the program terminates.
When a static variable in C is declared, the compiler allocates memory for it in a data segment instead of a stack, which is used for automatic variables. This means that the value of a static variable persists between function calls, unlike automatic variables that disappear once the function call completes.
A static variable can have either global or local scope. When declared inside a function, a static variable retains its value between function calls. On the other hand, when declared outside any function, it becomes a global variable and can be accessed by all functions in the program.
Syntax of Static Variable in C
The syntax for declaring a static variable in C programming language is as follows:
In this syntax, "data_type" refers to the data type of the variable being declared (e.g., int, float, double, etc.), and "variable_name" is the name given to the variable.
For example, to declare a static integer variable named "count", we use the following statement:
Properties of Static Variable in C
The properties of static variables in the C programming language are as follows:
- Lifetime: A static variable has a lifetime that extends throughout the entire execution of the program.
- Scope: A static variable can have either global or local scope. If declared inside a function, it has local scope and if declared outside of any function, it becomes a global variable.
- Memory allocation: A static variable’s memory is allocated in the data segment of the program’s memory, rather than the stack.
- Initialization: A static variable is initialized only once during program execution, at the time of program loading.
- Default initialization: If a static variable is not explicitly initialized, it is automatically initialized to zero (0) or NULL, depending on its data type.
- Thread safety: Static variables are not thread-safe by default. If multiple threads access the same static variable simultaneously, it can lead to race conditions and unexpected behavior.
Examples of Static Variable in C
Below are examples demonstrating the use of a static variable in C.
Local Scope Static Variable in C Here is an example of using a local scope static variable in C:
Code Implementation:
Explanation: In this example, the countCalls() function uses a static variable named count. The count variable is initialized to 0 when the program is loaded, and its value persists between function calls. Each time the countCalls() function is called, the value of the count is incremented and printed to the console.
Global Scope Static Variable in C Here is an example of using a global static variable in C:
Explanation: In this example, the count variable is declared as a global static variable outside of any function, meaning it can be accessed and modified by any function in the file. The countCalls() function increments the count variable each time it is called and prints its value to the console.
Difference Between Static Global and Static Local Variable
Here is the table showing the difference between static global and static local variables in C:
Uses of Static Variable in C
Here are some common uses of a static variable in C:
- Maintaining state across function calls – By retaining their values between function calls, static variables can be used to maintain state information, such as counting the number of times a function has been called or tracking the progress of a loop.
- Caching frequently accessed data – Static variable in C can be used to store data that is expensive to compute and frequently accessed, such as the result of a complex calculation or the contents of a file.
- Sharing data between related functions – Global static variable in C can be used to share data between related functions within a file, without exposing the data to other files.
- Implementing persistent storage – Static variable in C can be used to store data across program executions, allowing the program to resume where it left off after being shut down.
- Improving performance – By retaining their values between function calls, static variables can reduce the amount of time and resources needed to compute a value or perform an operation.
Conclusion In conclusion, the static variable in C is an important feature. It allows for the creation of variables that persist throughout the program’s execution, reducing the need for recomputing values and optimizing performance. While they can be useful, it’s important to keep in mind the potential issues that can arise when using static variables in a multi-threaded environment or when accessing them outside of their defined scope. Overall, understanding the properties and proper usage of static variables is key to writing efficient and effective C code.
Here are some frequently asked questions on a static variable in C.
Q1: Can we modify a static variable in C by another function? Ans: Yes, a static variable in C can be modified by any function that has access to it.
Q2: Can we access a static variable in C outside of the function in which it is defined? Ans: No, a static variable in C can only be accessed within the scope in which it is defined.
Q3: Can we use a static variable in a multi-file program? Ans: Yes, a static variable in C can be used in a multi-file program as long as it is declared in a header file and included in all source files that use it.
Q4: What is the difference between a constant variable and a static variable in C? Ans: A static variable’s value can be modified during program execution, while a constant variable’s value cannot be modified.
Q5: How can I ensure thread safety when dealing with static variables in a multi-threaded environment? Ans: To ensure thread safety, you can use synchronization techniques like mutexes or semaphores to ensure that only one thread can access the static variable at a time.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, assignment operator in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c.

15.6 — Static member variables
In the lesson 7.4 -- Introduction to global variables , we introduced global variables, and in lesson 7.10 -- Static local variables , we introduced static local variables. Both of these types of variables have static duration, meaning they are created at the start of the program, and destroyed at the end of the program. Such variables keep their values even if they go out of scope.
For example:
This program prints:
Note that static local variable s_id has kept its value across multiple function calls.
Class types bring two more uses for the static keyword: static member variables, and static member functions. Fortunately, these uses are fairly straightforward. We’ll talk about static member variables in this lesson, and static member functions in the next.
Static member variables
Before we go into the static keyword as applied to member variables, first consider the following class:
When we instantiate a class object, each object gets its own copy of all normal member variables. In this case, because we have declared two Something class objects, we end up with two copies of value : first.value , and second.value . first.value is distinct from second.value . Consequently, the program above prints:
Member variables of a class can be made static by using the static keyword. Unlike normal member variables, static member variables are shared by all objects of the class. Consider the following program, similar to the above:
This program produces the following output:
Because s_value is a static member variable, s_value is shared between all objects of the class. Consequently, first.s_value is the same variable as second.s_value . The above program shows that the value we set using first can be accessed using second !
Static members are not associated with class objects
Although you can access static members through objects of the class (as shown with first.s_value and second.s_value in the example above), static members exist even if no objects of the class have been instantiated! This makes sense: they are created at the start of the program and destroyed at the end of the program, so their lifetime is not bound to a class object like a normal member.
Essentially, static members are global variables that live inside the scope region of the class. There is very little difference between a static member of a class and a normal variable inside a namespace.
Key insight
Static members are global variables that live inside the scope region of the class.
Because static member s_value exists independently of any class objects, it can be accessed directly using the class name and the scope resolution operator (in this case, Something::s_value ):
In the above snippet, s_value is referenced by class name Something rather than through an object. Note that we have not even instantiated an object of type Something , but we are still able to access and use Something::s_value . This is the preferred method for accessing static members.
Best practice
Access static members using the class name and the scope resolution operator (::).
Defining and initializing static member variables
When we declare a static member variable inside a class type, we’re telling the compiler about the existence of a static member variable, but not actually defining it (much like a forward declaration). Because static member variables are essentially global variables, you must explicitly define (and optionally initialize) the static member outside of the class, in the global scope.
In the example above, we do so via this line:
This line serves two purposes: it instantiates the static member variable (just like a global variable), and initializes it. In this case, we’re providing the initialization value 1 . If no initializer is provided, static member variables are zero-initialized by default.
Note that this static member definition is not subject to access controls: you can define and initialize the value even if it’s declared as private (or protected) in the class.
If the class is defined in a header (.h) file, the static member definition is usually placed in the associated code file for the class (e.g. Something.cpp ). If the class is defined in a source (.cpp) file, the static member definition is usually placed directly underneath the class. Do not put the static member definition in a header file (much like a global variable, if that header file gets included more than once, you’ll end up with multiple definitions, which will cause a compile error).
Initialization of static member variables inside the class definition
There are a few shortcuts to the above. First, when the static member is a constant integral type (which includes char and bool ) or a const enum, the static member can be initialized inside the class definition:
In the above example, because the static member variable is a const int, no explicit definition line is needed. This shortcut is allowed because these specific const types are compile-time constants.
In lesson 7.9 -- Sharing global constants across multiple files (using inline variables) , we introduced inline variables, which are variables that are allowed to have multiple definitions. C++17 allows static members to be inline variables:
Such variables can be initialized inside the class definition regardless of whether they are constant or not. This is the preferred method of defining and initializing static members.
Because constexpr members are implicitly inline (as of C++17), static constexpr members can also be initialized inside the class definition without explicit use of the inline keyword:
Make your static members inline or constexpr so they can be initialized inside the class definition.
An example of static member variables
Why use static variables inside classes? One use is to assign a unique ID to every instance of the class. Here’s an example:
Because s_idGenerator is shared by all Something objects, when a new Something object is created, the constructor initializes m_id with the current value of s_idGenerator and then increments the value for the next object. This guarantees that each instantiated Something object receives a unique id (incremented in the order of creation).
Giving each object a unique ID can help when debugging, as it can be used to differentiate objects that otherwise have identical data. This is particularly true when working with arrays of data.
Static members variables are also useful when the class needs to utilize a lookup table (e.g. an array used to store a set of pre-calculated values). By making the lookup table static, only one copy exists for all objects, rather than making a copy for each object instantiated. This can save substantial amounts of memory.
Only static members may use type deduction ( auto and CTAD)
A static member may use auto to deduce its type from the initializer, or Class Template Argument Deduction (CTAD) to deduce template type arguments from the initializer.
Non-static members may not use auto or CTAD.
The reasons for this distinction being made are quite complicated, but boil down to there being certain cases that can occur with non-static members that lead to ambiguity or non-intuitive results. This does not occur for static members. Thus non-static members are restricted from using these features, whereas static members are not.
Static Variables and Static Class Members - 2020

- Persistence: it remains in memory until the end of the program.
- File scope: it can be seen only withing a file where it's defined.
- Visibility: if it is defined within a function/block, it's scope is limited to the function/block. It cannot be accessed outside of the function/block.
- Class: static members exist as members of the class rather than as an instance in each object of the class. So, this keyword is not available in a static member function. Such functions may access only static data members. There is only a single instance of each static data member for the entire class: A static data member : class variable A non-static data member : instance variable
- Static member function: it can only access static member data, or other static member functions while non-static member functions can access all data members of the class: static and non-static.
This is one of the frequently asked questions during the interview. Most of the candidates get the first one, and some with the second, third, and may be 4th ones as well. But rarely they address the 5th one:
- A variable declared static within the body of a function maintains its value between invocations of the function.
- A variable declared static within a module (but outside the body of a function) is accessible by all functions within that module. However, it is not accessible by functions from other modules.
- static members exist as members of the class rather than as an instance in each object of the class. There is only a single instance of each static data member for the entire class.
- Non-static member functions can access all data members of the class: static and non-static. Static member functions can only operate on the static data members.
- C functions declared static within a module may only be called by other functions within that module (file scope).
Static object is an object that persists from the time it's constructed until the end of the program. So, stack and heap objects are excluded. But global objects, objects at namespace scope, objects declared static inside classes/functions, and objects declared at file scope are included in static objects . Static objects are destroyed when the program stops running.
Variables defined outside a function or by using the keyword static have static storage duration. They persist for the entire running time of a program.
- external linkage
- internal linkage
Since the static variables stay the same throughout the life cycle of the program, they are easy to deal with for the memory system and they are allocated in a fixed block of memory.
Here is an example of static variables with different duration.
All the static variables persist until program terminates. The variable d has local scope and no linkage - it's no use outside of f() . But c remains in memory even when the f() function is not being executed. By saying that c is static , we are saying that we want to allocate it once, and only once, at some point before the first time that f() is called, and that we do not want to deallocate it as long as our program runs.
Both a and b can be accessed from the point of declaration until the end of the file. But a can be used in other files because it has external linkage.
All static duration variables have the following initialization characteristics:
- An uninitialized static variable set to 0 .
- A static variable can be initialized only with a constant expression .
The const in C++ has gives a little bit of twist to the default storage classes. While a global variable has external linkage by default, a const global has internal linkage by default. In other words, C++ treats a global const definition as if the static had been used as in the following code.
So, the "const int a = 10" becomes "static const int a = 10". However, if global const had external linkage as regular variables do, the const declaration would be an error because we can define a global variable in one file only. In other words, only one file can contain the preceding declaration, and the other files have to provide reference declarations using the extern keyword. Note that only the declarations without the extern can initialize values.
However, if we want to make a constant have external linkage, we can use the extern keyword to override the default internal linkage:
Here, we should use the extern keyword to declare the constant in all files that use the constant. That's the difference between regular external variables and constant. For regular external variables, we don't use the keyword extern when we define a variable, but we use extern in other files using that variable.
An extern declaration does not define the variable unless it is also initialized in the same statement.
For extern "C" , please visit extern "C" .
Dynamic memory is controlled by the new and delete operators, not by scope and linkage rules. So, dynamic memory can be allocated from one function and freed from another function.
Although the storage schemes don't apply to dynamic memory, then do apply to automatic and static pointer variables used to keep track of dynamic memory.
Let's look at the following line of code:
The 40 bytes of memory allocated by new remains in memory until the delete frees it. But the pointer ptr passes from existence when the function containing this declaration terminates.
If we want to have the 40 bytes of allocated memory available from another function, we need to pass or return its address to that function.
However, a statement that uses new to set ptr has to be in a function because static storage variables can only be initialized with constant expressions as shown in the following example.
See Memory Allocation .
Static members exist as members of the class rather than as an instance in each object of the class. So, this keyword is not available in a static member function. Such functions may access only static data members. There is a single instance of each static data member for the entire class, which should be initialized, usually in the source file that implements the class member functions. Because the member is initialized outside the class definition, we must use fully qualified name when we initialize it:
Here is the real life example, Car.h:
Implementation file, Car.cpp:
The code initializes the static id member to 100. Note again that we cannot initialize a static member variable inside the class declaration. That's because the declaration is a description of how memory is to be allocated, but it doesn't allocate memory. We allocate and initialize memory by creating an object using that format.
In the case of a static class member, we initialize the static member independently, with a separate statement outside the class declaration. That's because the static class member is stored separately rather than as part of an object.
The exception to the initialization of a static data member inside the class declaration is if the static data member is a const of integral or enumeration type.
The following example shows an illegal access to non-static member from a static function.
In function f(), x=z is an error because f(), a static function, is trying to access non-static member x.
So, the fix should be like this:
The following code shows that a non-static members can not be used as default arguments.
Here are some characteristics of static member functions :
- A static member function can only access static member data , static member functions and data and functions outside the class . So, we must take note not to use static member function in the same manner as non-static member function, as non-static member function can access all of the above including the static data member.
- We must first understand the concept of static data while learning the context of static functions. It is possible to declare a data member of a class as static irrespective of it being a public or a private type in class definition. If a data is declared as static, then the static data is created and initialized only once. Non-static data members are created again and again. For each separate object of the class, the static data is created and initialized only once. As in the concept of static data, all objects of the class in static functions share the variables. This applies to all objects of the class.
- A non-static member function can be called only after instantiating the class as an object. This is not the case with static member functions. A static member function can be called, even when a class is not instantiated.
- A static member function cannot have access to the this pointer of the class.
- A non-static member function can be declared as virtual but care must be taken not to declare a static member function as virtual.
Singleton design pattern is a good example of static member function and static member variable. In this pattern, we put constructor in private section not in public section of a class. So, we can not access the constructor to make an instance of the class. Instead, we put a public function which is static function. The getInstance() will make an instance only once. Note that if this method is not static, there is no way to invoke the getInstance() even though it is public method. That's because we do not have any instance of Singleton .
External linkage means that a symbol in one translation unit can be accesses from the other translation units. Unless we take special steps, the functions and global variables in our .cpp will have external linkage.
Here is the code that can access the My_CONSTANT , My_NAME , and My_Function() without using public interfaces, thus breaking encapsulation:
One way of avoid this linkage issue is using static keyword:
Though we can avoid the external linkage problem by using static keyword, it still has issue of polluting global namespace. So, the batter solution is to use anonymous namespace as shown below:
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization
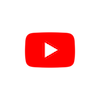
Sponsor Open Source development activities and free contents for everyone.
C++ Tutorials
How to Initialize Static Variables in C++ Class
- How to Initialize Static Variables in …
Initialize Static Variables in C++
Difference between constant and static variables.
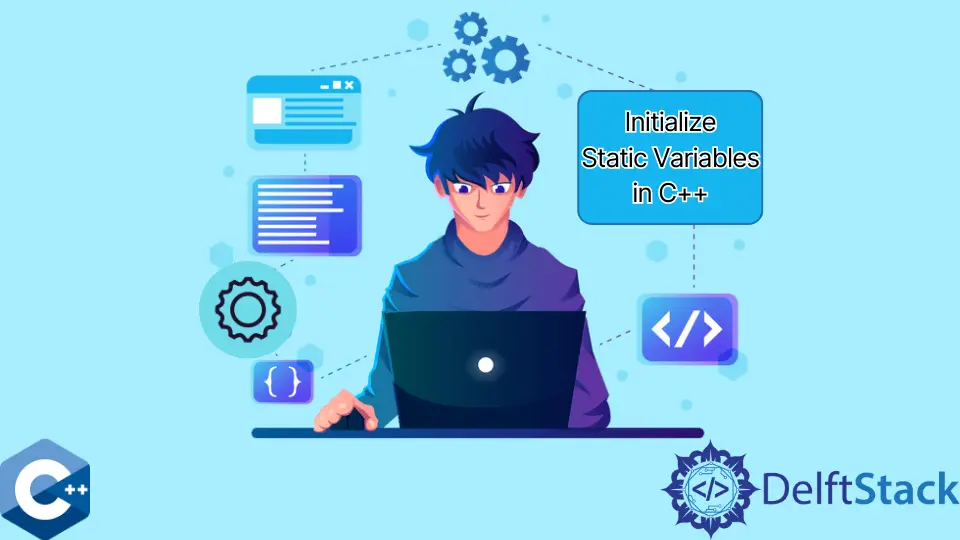
We will learn in this short article how to initialize static variables in C++.
The initialization of static variables in a C++ class is the process of assigning values to the static variables.
All class objects have access to a static member. If no other initialization is present, all static data is initialized to zero when the first object is created.
Although we cannot include it in the class definition, we can initialize it outside the class by redeclaring the static variable and determining its class affiliation using the scope resolution operator. Usually, The initialization can be done in two ways.
- Implicitly, by defining them as constants.
- Explicitly by using the “static” keyword and assigning values.
We should use implicit initialization only when we are sure that all static variables have been initialized beforehand. Otherwise, we should use explicit initialization with the static keyword.
Code Example:
Run Demo Code
In C++, a variable is a name given to a storage location in the computer’s memory that can store different data types, such as numbers and text. These names are called identifiers .
The data stored in the variable may change over time. This type of variable is called dynamic or dynamic-typed .
The other variable type is called constant or constant-typed . The data stored in this kind of variable never changes over time, and constants are typically used to define an object’s properties and parameters at the beginning of a program or function.
Constants are declared with the const keyword and can only be initialized at declaration time or inside a function. The static keyword creates the variable only once, and all functions within the same file can access the variable.
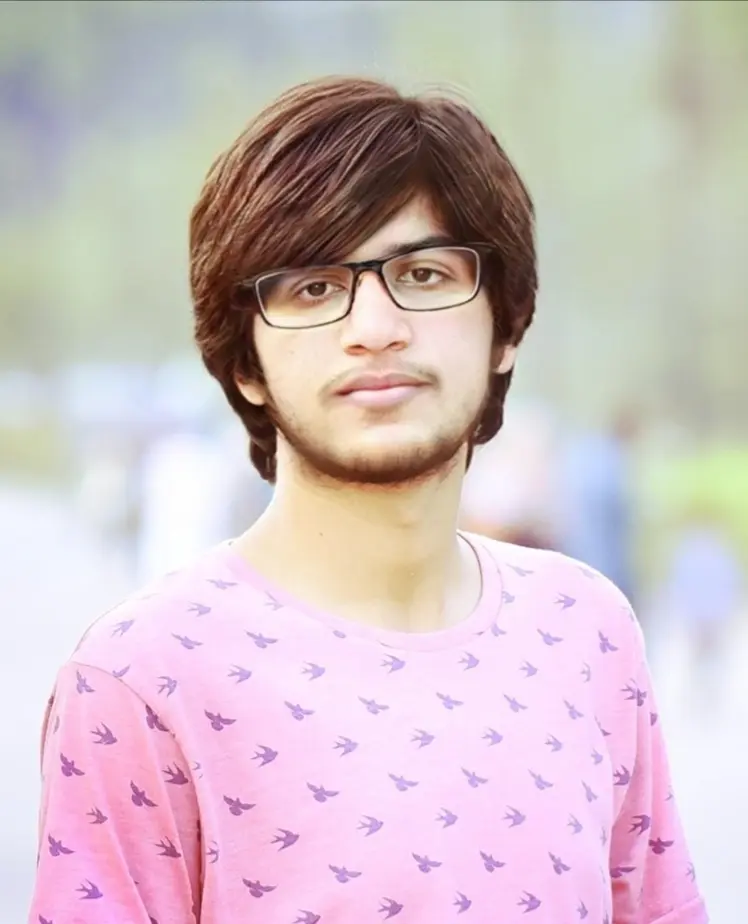
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Related Article - C++ Class
- How to Get Class Name in C++
- Point and Line Class in C++
- Class Template Inheritance in C++
- Difference Between Structure and Class in C++
- Wrapper Class in C++
Rating Action Commentary
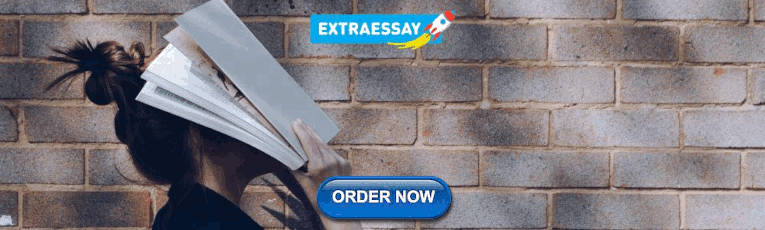
Fitch Rates Golub Capital Partners Static 2024-1, Ltd.; Publishes New Issue Report
Thu 18 Apr, 2024 - 2:43 PM ET
Related Content: Golub Capital Partners Static 2024-1, Ltd.
Fitch Ratings - Austin - 18 Apr 2024: Fitch Ratings has assigned ratings and Rating Outlooks to Golub Capital Partners Static 2024-1, Ltd.
- Subordinated
VIEW ADDITIONAL RATING DETAILS
Transaction Summary
Golub Capital Partners Static 2024-1, Ltd. (the issuer) is an arbitrage cash flow collateralized loan obligation (CLO) that will be managed by OPAL BSL LLC. Net proceeds from the issuance of the secured and subordinated notes will provide financing on a portfolio of approximately $725 million of primarily first lien senior secured leveraged loans.
KEY RATING DRIVERS
Asset Credit Quality (Negative): The average credit quality of the indicative portfolio is 'B/B-', which is in line with that of recent CLOs. Issuers rated in the 'B' rating category denote a highly speculative credit quality; however, the notes benefit from appropriate credit enhancement and standard CLO structural features.
Asset Security (Positive): The indicative portfolio consists of 100% first-lien senior secured loans and has a weighted average recovery assumption of 76.77%.
Portfolio Composition (Neutral): The largest three industries constitute 46.8% of the portfolio balance in aggregate, while the top five obligors represent 7.09% of the portfolio balance in aggregate. The level of diversity by industry, and obligor concentrations is relatively, and at the margin, more concentrated than with other recent U.S. CLOs.
Portfolio Management (Neutral): The transaction does not have a reinvestment period; however, the issuer has the ability to extend the weighted average life of the portfolio as a result of maturity amendments. Fitch's analysis was based on a stressed portfolio incorporating potential maturity amendments on the underlying loans as well as a one-notch downgrade on the Fitch Issuer Default Rating Equivalency Rating for assets with a Negative Outlook on the driving rating of the obligor. The shorter risk horizon means the transaction is less vulnerable to underlying price movements, economic conditions and asset performance.
Cash Flow Analysis (Positive): Fitch used a customized proprietary cash flow model to replicate the principal and interest waterfalls and assess the effectiveness of various structural features of the transaction. In Fitch's stress scenarios, the rated notes can withstand default and recovery assumptions consistent with their assigned ratings.
RATING SENSITIVITIES
Factors that could, individually or collectively, lead to negative rating action/downgrade.
Variability in key model assumptions, such as decreases in recovery rates and increases in default rates, could result in a downgrade. Fitch evaluated the notes' sensitivity to potential changes in such a metric. The results under these sensitivity scenarios are as severe as between 'BBB+sf' and 'AA+sf' for class A-1, between 'BBB+sf' and 'AA+sf' for class A-2, between 'BB+sf' and 'A+sf' for class B, between 'B+sf' and 'A-sf' for class C, between less than 'B-sf' and 'BBB-sf' for class D, and between less than 'B-sf' and 'BB+sf' for class E.
Factors that Could, Individually or Collectively, Lead to Positive Rating Action/Upgrade
Upgrade scenarios are not applicable to the class A-1 and class A-2 notes as these notes are in the highest rating category of 'AAAsf'. Variability in key model assumptions, such as increases in recovery rates and decreases in default rates, could result in an upgrade. Fitch evaluated the notes' sensitivity to potential changes in such metrics; the minimum rating results under these sensitivity scenarios are 'AAAsf' for class B, 'AA+sf' for class C, 'A+sf' for class D, and 'BBB+sf' for class E.
USE OF THIRD PARTY DUE DILIGENCE PURSUANT TO SEC RULE 17G -10
Form ABS Due Diligence-15E was not provided to, or reviewed by, Fitch in relation to this rating action.
DATA ADEQUACY
The majority of the underlying assets or risk-presenting entities have ratings or credit opinions from Fitch and/or other nationally recognized statistical rating organizations and/or European Securities and Markets Authority-registered rating agencies. Fitch has relied on the practices of the relevant groups within Fitch and/or other rating agencies to assess the asset portfolio information. Overall, Fitch's assessment of the asset pool information relied upon for its rating analysis according to its applicable rating methodologies indicates that it is adequately reliable.
ESG CONSIDERATIONS
Fitch does not provide ESG relevance scores for Golub Capital Partners Static 2024-1, Ltd. In cases where Fitch does not provide ESG relevance scores in connection with the credit rating of a transaction, programme, instrument or issuer, Fitch will disclose in the key rating drivers any ESG factor which has a significant impact on the rating on an individual basis. For more information on Fitch's ESG Relevance Scores, visit the Fitch Ratings ESG Relevance Scores page.
REFERENCES FOR SUBSTANTIALLY MATERIAL SOURCE CITED AS KEY DRIVER OF RATING
The principal sources of information used in the analysis are described in the Applicable Criteria.
REPRESENTATIONS, WARRANTIES AND ENFORCEMENT MECHANISMS
A description of the transaction's representations, warranties and enforcement mechanisms (RW&Es) that are disclosed in the offering document and which relate to the underlying asset pool was not prepared for this transaction. Offering Documents for this market sector typically do not include RW&Es that are available to investors and that relate to the asset pool underlying the trust. Therefore, Fitch credit reports for this market sector will not typically include descriptions of RW&Es. For further information, please see Fitch's Special Report titled 'Representations, Warranties and Enforcement Mechanisms in Global Structured Finance Transactions'.
Additional information is available on www.fitchratings.com
PARTICIPATION STATUS
The rated entity (and/or its agents) or, in the case of structured finance, one or more of the transaction parties participated in the rating process except that the following issuer(s), if any, did not participate in the rating process, or provide additional information, beyond the issuer’s available public disclosure.
APPLICABLE CRITERIA
- Structured Finance and Covered Bonds Country Risk Rating Criteria (pub. 07 Jul 2023)
- CLOs and Corporate CDOs Rating Criteria (pub. 21 Jul 2023) (including rating assumption sensitivity)
- Structured Finance and Covered Bonds Counterparty Rating Criteria (pub. 28 Nov 2023)
- Global Structured Finance Rating Criteria (pub. 19 Jan 2024) (including rating assumption sensitivity)
- Structured Finance and Covered Bonds Interest Rate Stresses Rating Criteria (pub. 05 Apr 2024)
APPLICABLE MODELS
Numbers in parentheses accompanying applicable model(s) contain hyperlinks to criteria providing description of model(s).
- CLO – Fitch Stressed Portfolio Model, v2.4.0 ( 1 )
- Global CLO Cash Flow Model, v1.3.7 ( 1 )
- Portfolio Credit Model, v2.16.2 ( 1 )
ADDITIONAL DISCLOSURES
- Dodd-Frank Rating Information Disclosure Form
- Solicitation Status
- Endorsement Policy
ENDORSEMENT STATUS
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- How to find out the caller function in JavaScript?
- How to measure time taken by a function to execute using JavaScript ?
- How to include a JavaScript file in another JavaScript file ?
- How to Remove an Entry by Key in JavaScript Object?
- What is a typical use case for anonymous functions in JavaScript ?
- How to call function from it name stored in a string using JavaScript ?
- Explain the MUL() function in JavaScript ?
- How to check whether a number is NaN or finite in JavaScript ?
- How to create a function that invokes the provided function with its arguments transformed in JavaScript ?
- How to negate a predicate function in JavaScript ?
- How to create a new object from the specified object, where all the keys are in lowercase in JavaScript?
- What is the difference between call and apply in JavaScript ?
- How to declare the optional function parameters in JavaScript ?
- How to get the index of the function in an array of functions which executed the fastest in JavaScript ?
- What is the purpose of setTimeout() function in JavaScript ?
- How to iterate over a callback n times in JavaScript ?
- How to create a function that invokes each provided function with the arguments it receives using JavaScript ?
- JavaScript Passing parameters to a callback function
- How to write a function in JavaScript ?
How to create Static Variables in JavaScript ?
To create a static variable in JavaScript, you can use a closure or a function scope to encapsulate the variable within a function. This way, the variable maintains its state across multiple invocations of the function.
- Static keyword in JavaScript: The static keyword is used to define a static method or property of a class. To call the static method we do not need to create an instance or object of the class.
- Static variable in JavaScript: We used the static keyword to make a variable static just like the constant variable is defined using the const keyword. It is set at the run time and such type of variable works as a global variable. We can use the static variable anywhere. The value of the static variable can be reassigned, unlike the constant variable.
Why do we create a static variable in JavaScript?
In JavaScript, we simulate static variables using closures or other patterns to share state among instances, persist data between calls, or encapsulate information. These “static-like” variables enhance modularity and encapsulation, providing a way to maintain a state without relying on global variables.
Example 1: In the below example, we will create a static variable and display it on the JavaScript console.
Example 2: Static variable is called using this keyword.
Please Login to comment...
Similar reads.
- javascript-functions
- JavaScript-Questions
- Web Technologies
- 10 Ways to Use Microsoft OneNote for Note-Taking
- 10 Best Yellow.ai Alternatives & Competitors in 2024
- 10 Best Online Collaboration Tools 2024
- 10 Best Autodesk Maya Alternatives for 3D Animation and Modeling in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
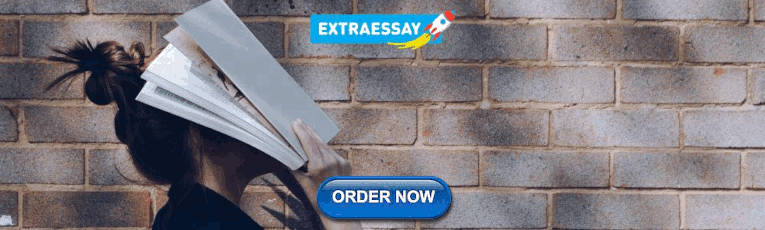
IMAGES
VIDEO
COMMENTS
As others have said, a static variable at function scope is initialized only once. So the assignment doesn't happen on subsequent calls to the function. Unlike other local variables, a static local is not defined on the stack but in the data segment, probably in the same location as global variables. Globals are also initialized at application startup (they have to, since they don't live ...
The static keyword is one of the most essential features in the Java programming language. We use it to define class-level variables and methods. Here is an example of how to use the static keyword: public class StaticKeywordExample { private static int count = 0; // static variable public static void printCount() { // static method System.out ...
Syntax: static data_type var_name = var_value; Following are some interesting facts about static variables in C: 1) A static int variable remains in memory while the program is running. A normal or auto variable is destroyed when a function call where the variable was declared is over. For example, we can use static int to count the number of ...
2. As stated by the other answers, you should set your initial value like so: private static String foo = "initial value"; Additionally, if you want to access this variable from anywhere, you need to reference it in a static context, like so: Foo.foo. where Foo is the class name, and foo is the variable name.
2. The Anatomy of the static Keyword. In the Java programming language, the keyword static means that the particular member belongs to a type itself, rather than to an instance of that type. This means we'll create only one instance of that static member that's shared across all instances of the class. We can apply the keyword to variables ...
Static Variables in Java with Examples. When a variable is declared as static, then a single copy of the variable is created and shared among all objects at the class level. Static variables are, essentially, global variables. All instances of the class share the same static variable. Important points for static variables:
Static variable. In computer programming, a static variable is a variable that has been allocated "statically", meaning that its lifetime (or "extent") is the entire run of the program. This is in contrast to shorter-lived automatic variables, whose storage is stack allocated and deallocated on the call stack; and in contrast to objects, whose ...
Assigning values to static final variables in Java. In Java, non-static final variables can be assigned a value either in constructor or with the declaration. But, static final variables cannot be assigned value in constructor; they must be assigned a value with their declaration. For example, following program works fine.
At a high level, the JVM performs the following steps: First, the class is loaded and linked. Then, the "initialize" phase of this process processes the static variable initialization. Finally, the main method associated with the class is called. In the next section, we'll look at class variable initialization. 3.
The syntax for declaring a static variable in C programming language is as follows: static data_type variable_name; In this syntax, "data_type" refers to the data type of the variable being declared (e.g., int, float, double, etc.), and "variable_name" is the name given to the variable. For example, to declare a static integer variable named ...
15.6 — Static member variables. Alex March 13, 2024. In the lesson 7.4 -- Introduction to global variables, we introduced global variables, and in lesson 7.10 -- Static local variables, we introduced static local variables. Both of these types of variables have static duration, meaning they are created at the start of the program, and ...
Unlike non-static variables, such variables can be accessed directly in static and non-static methods. ... reflects when we access them using other * objects */ //Assigning the value to static variable using object ob1 ob1.var1=88; ob1.var2="I'm Object1"; /* This will overwrite the value of var1 because var1 has a single * copy shared among ...
C++ Tutorial: Static Variables and Static Class Members - Static object is an object that persists from the time it's constructed until the end of the program. So, stack and heap objects are excluded. But global objects, objects at namespace scope, objects declared static inside classes/functions, and objects declared at file scope are included in static objects.
The initialization of static variables in a C++ class is the process of assigning values to the static variables. All class objects have access to a static member. If no other initialization is present, all static data is initialized to zero when the first object is created. Although we cannot include it in the class definition, we can ...
Output: 6 7. Reason: static variable is initialised only once (unlike auto variable) and further definition of static variable would be bypassed during runtime. And if it is not initialised manually, it is initialised by value 0 automatically. So, void foo() {. static int x = 5; // assigns value of 5 only once.
An article for Mid level JavaScript Developers. Static variables are attached to class instead of instance and its value will be shared between the different instances. To use a static variable or static function, we use the static keyword. * A static variable is a class property that is used in a class and not on the instance of the class ...
Static Single Assignment was presented in 1988 by Barry K. Rosen, Mark N, Wegman, and F. Kenneth Zadeck.. In compiler design, Static Single Assignment ( shortened SSA) is a means of structuring the IR (intermediate representation) such that every variable is allotted a value only once and every variable is defined before it's use. The prime use of SSA is it simplifies and improves the ...
Static Variables: Variables in a function, Variables in a class Static Members of Class: Class objects and Functions in a class Let us now look at each one of these uses of static in detail. Static Variables. Static variables in a Function: When a variable is declared as static, space for it gets allocated for the lifetime of the program.Even if the function is called multiple times, space for ...
Spring does not allow to inject value into static variables. A workaround is to create a non static setter to assign your value into the static variable: private static String SVN_URL; @Value("${SVN_URL}") public void setSvnUrl(String svnUrl) {. SVN_URL = svnUrl;
Golub Capital Partners Static 2024-1, Ltd. (the issuer) is an arbitrage cash flow collateralized loan obligation (CLO) that will be managed by OPAL BSL LLC. Net proceeds from the issuance of the secured and subordinated notes will provide financing on a portfolio of approximately $725 million of primarily first lien senior secured leveraged loans.
You can access static fields and properties and methods from all non static methods. You can also add an instance member mapping a static member. Instances of a static thing can't exist in addition to the static existence itself. So you can write this: class myClass. {. public string a = "hello"; static public string b = "world"; public string ...
To create a static variable in JavaScript, you can use a closure or a function scope to encapsulate the variable within a function. This way, the variable maintains its state across multiple invocations of the function. Static keyword in JavaScript: The static keyword is used to define a static method or property of a class.