
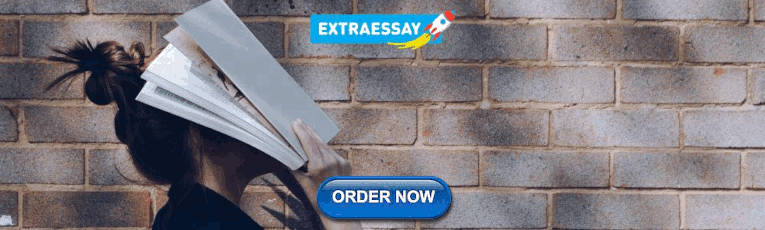
Common Weakness Enumeration
A community-developed list of SW & HW weaknesses that can become vulnerabilities

- About ▼ About New to CWE User Stories History Documents FAQs Glossary Compatibility
- CWE List ▼ Latest Version Downloads Reports Visualizations Archive
- Mapping ▼ Root Cause Mapping Guidance Root Cause Mapping Quick Tips Root Cause Mapping Examples
- Top-N Lists ▼ Top 25 Software Top Hardware Top 10 KEV Weaknesses
- Community ▼ Community Working Groups & Special Interest Groups Board Board Meeting Minutes CWE Discussion List CWE Discussion Archives Contribute Weakness Content to CWE

CWE-587: Assignment of a Fixed Address to a Pointer
Edit custom filter.
C (Undetermined Prevalence)
C++ (Undetermined Prevalence)
C# (Undetermined Prevalence)
Class: Assembly (Undetermined Prevalence)
This code assumes a particular function will always be found at a particular address. It assigns a pointer to that address and calls the function.
The same function may not always be found at the same memory address. This could lead to a crash, or an attacker may alter the memory at the expected address, leading to arbitrary code execution.
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- Pointer Arithmetics in C with Examples
- Applications of Pointers in C
- Passing Pointers to Functions in C
- C - Pointer to Pointer (Double Pointer)
- Chain of Pointers in C with Examples
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- NULL Pointer in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
Pointers are one of the core components of the C programming language. A pointer can be used to store the memory address of other variables, functions, or even other pointers. The use of pointers allows low-level memory access, dynamic memory allocation, and many other functionality in C.
In this article, we will discuss C pointers in detail, their types, uses, advantages, and disadvantages with examples.
What is a Pointer in C?
A pointer is defined as a derived data type that can store the address of other C variables or a memory location. We can access and manipulate the data stored in that memory location using pointers.
As the pointers in C store the memory addresses, their size is independent of the type of data they are pointing to. This size of pointers in C only depends on the system architecture.
Syntax of C Pointers
The syntax of pointers is similar to the variable declaration in C, but we use the ( * ) dereferencing operator in the pointer declaration.
- ptr is the name of the pointer.
- datatype is the type of data it is pointing to.
The above syntax is used to define a pointer to a variable. We can also define pointers to functions, structures, etc.
How to Use Pointers?
The use of pointers in C can be divided into three steps:
- Pointer Declaration
- Pointer Initialization
- Pointer Dereferencing
1. Pointer Declaration
In pointer declaration, we only declare the pointer but do not initialize it. To declare a pointer, we use the ( * ) dereference operator before its name.
The pointer declared here will point to some random memory address as it is not initialized. Such pointers are called wild pointers.
2. Pointer Initialization
Pointer initialization is the process where we assign some initial value to the pointer variable. We generally use the ( & ) addressof operator to get the memory address of a variable and then store it in the pointer variable.
We can also declare and initialize the pointer in a single step. This method is called pointer definition as the pointer is declared and initialized at the same time.
Note: It is recommended that the pointers should always be initialized to some value before starting using it. Otherwise, it may lead to number of errors.
3. Pointer Dereferencing
Dereferencing a pointer is the process of accessing the value stored in the memory address specified in the pointer. We use the same ( * ) dereferencing operator that we used in the pointer declaration.
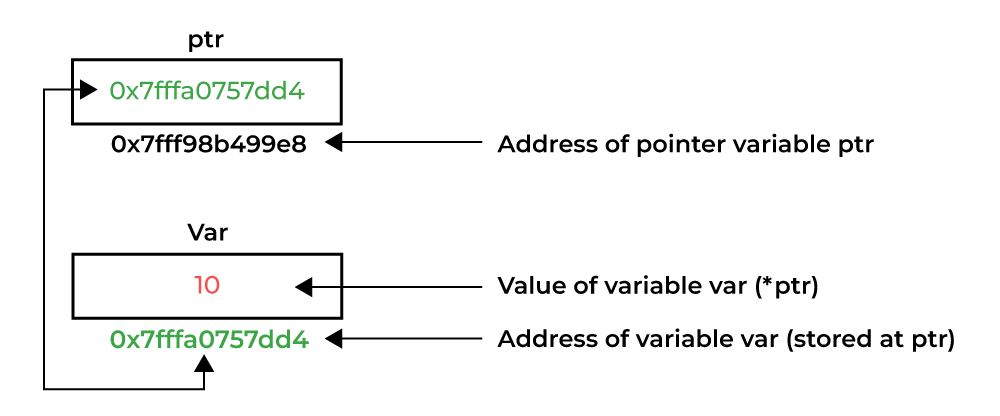
Dereferencing a Pointer in C
C Pointer Example
Types of pointers in c.
Pointers in C can be classified into many different types based on the parameter on which we are defining their types. If we consider the type of variable stored in the memory location pointed by the pointer, then the pointers can be classified into the following types:
1. Integer Pointers
As the name suggests, these are the pointers that point to the integer values.
These pointers are pronounced as Pointer to Integer.
Similarly, a pointer can point to any primitive data type. It can point also point to derived data types such as arrays and user-defined data types such as structures.
2. Array Pointer
Pointers and Array are closely related to each other. Even the array name is the pointer to its first element. They are also known as Pointer to Arrays . We can create a pointer to an array using the given syntax.
Pointer to Arrays exhibits some interesting properties which we discussed later in this article.
3. Structure Pointer
The pointer pointing to the structure type is called Structure Pointer or Pointer to Structure. It can be declared in the same way as we declare the other primitive data types.
In C, structure pointers are used in data structures such as linked lists, trees, etc.
4. Function Pointers
Function pointers point to the functions. They are different from the rest of the pointers in the sense that instead of pointing to the data, they point to the code. Let’s consider a function prototype – int func (int, char) , the function pointer for this function will be
Note: The syntax of the function pointers changes according to the function prototype.
5. Double Pointers
In C language, we can define a pointer that stores the memory address of another pointer. Such pointers are called double-pointers or pointers-to-pointer . Instead of pointing to a data value, they point to another pointer.
Dereferencing Double Pointer
Note: In C, we can create multi-level pointers with any number of levels such as – ***ptr3, ****ptr4, ******ptr5 and so on.
6. NULL Pointer
The Null Pointers are those pointers that do not point to any memory location. They can be created by assigning a NULL value to the pointer. A pointer of any type can be assigned the NULL value.
It is said to be good practice to assign NULL to the pointers currently not in use.
7. Void Pointer
The Void pointers in C are the pointers of type void. It means that they do not have any associated data type. They are also called generic pointers as they can point to any type and can be typecasted to any type.
One of the main properties of void pointers is that they cannot be dereferenced.
8. Wild Pointers
The Wild Pointers are pointers that have not been initialized with something yet. These types of C-pointers can cause problems in our programs and can eventually cause them to crash. If values is updated using wild pointers, they could cause data abort or data corruption.
9. Constant Pointers
In constant pointers, the memory address stored inside the pointer is constant and cannot be modified once it is defined. It will always point to the same memory address.
10. Pointer to Constant
The pointers pointing to a constant value that cannot be modified are called pointers to a constant. Here we can only access the data pointed by the pointer, but cannot modify it. Although, we can change the address stored in the pointer to constant.
Other Types of Pointers in C:
There are also the following types of pointers available to use in C apart from those specified above:
- Far pointer : A far pointer is typically 32-bit that can access memory outside the current segment.
- Dangling pointer : A pointer pointing to a memory location that has been deleted (or freed) is called a dangling pointer.
- Huge pointer : A huge pointer is 32-bit long containing segment address and offset address.
- Complex pointer: Pointers with multiple levels of indirection.
- Near pointer : Near pointer is used to store 16-bit addresses means within the current segment on a 16-bit machine.
- Normalized pointer: It is a 32-bit pointer, which has as much of its value in the segment register as possible.
- File Pointer: The pointer to a FILE data type is called a stream pointer or a file pointer.
Size of Pointers in C
The size of the pointers in C is equal for every pointer type. The size of the pointer does not depend on the type it is pointing to. It only depends on the operating system and CPU architecture. The size of pointers in C is
- 8 bytes for a 64-bit System
- 4 bytes for a 32-bit System
The reason for the same size is that the pointers store the memory addresses, no matter what type they are. As the space required to store the addresses of the different memory locations is the same, the memory required by one pointer type will be equal to the memory required by other pointer types.
How to find the size of pointers in C?
We can find the size of pointers using the sizeof operator as shown in the following program:
Example: C Program to find the size of different pointer types.
As we can see, no matter what the type of pointer it is, the size of each and every pointer is the same.
Now, one may wonder that if the size of all the pointers is the same, then why do we need to declare the pointer type in the declaration? The type declaration is needed in the pointer for dereferencing and pointer arithmetic purposes.
C Pointer Arithmetic
The Pointer Arithmetic refers to the legal or valid arithmetic operations that can be performed on a pointer. It is slightly different from the ones that we generally use for mathematical calculations as only a limited set of operations can be performed on pointers. These operations include:
- Increment in a Pointer
- Decrement in a Pointer
- Addition of integer to a pointer
- Subtraction of integer to a pointer
- Subtracting two pointers of the same type
- Comparison of pointers of the same type.
- Assignment of pointers of the same type.
C Pointers and Arrays
In C programming language, pointers and arrays are closely related. An array name acts like a pointer constant. The value of this pointer constant is the address of the first element. For example, if we have an array named val then val and &val[0] can be used interchangeably.
If we assign this value to a non-constant pointer of the same type, then we can access the elements of the array using this pointer.
Example 1: Accessing Array Elements using Pointer with Array Subscript
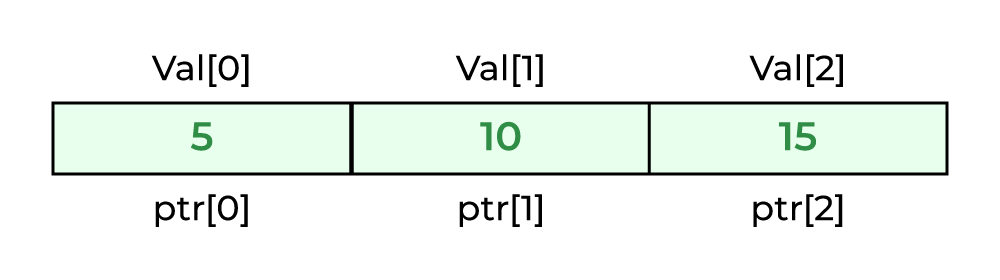
Not only that, as the array elements are stored continuously, we can pointer arithmetic operations such as increment, decrement, addition, and subtraction of integers on pointer to move between array elements.
Example 2: Accessing Array Elements using Pointer Arithmetic
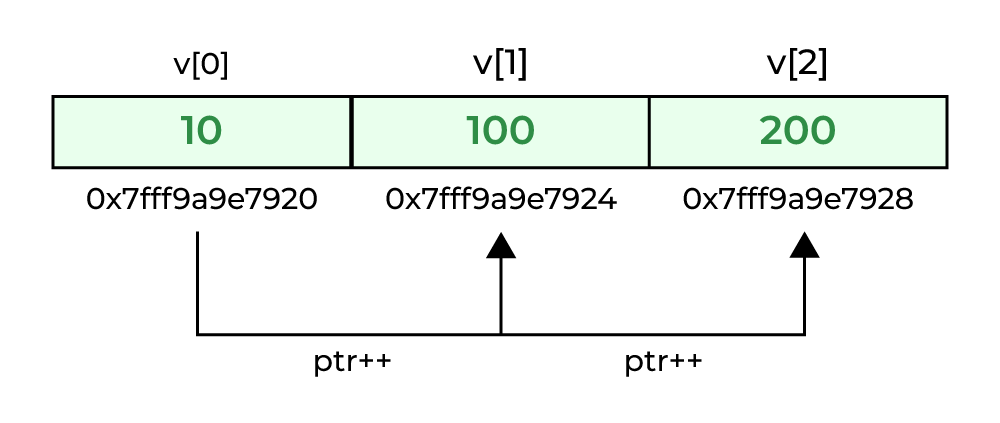
This concept is not limited to the one-dimensional array, we can refer to a multidimensional array element as well using pointers.
To know more about pointers to an array, refer to this article – Pointer to an Array
Uses of Pointers in C
The C pointer is a very powerful tool that is widely used in C programming to perform various useful operations. It is used to achieve the following functionalities in C:
- Pass Arguments by Reference
- Accessing Array Elements
- Return Multiple Values from Function
- Dynamic Memory Allocation
- Implementing Data Structures
- In System-Level Programming where memory addresses are useful.
- In locating the exact value at some memory location.
- To avoid compiler confusion for the same variable name.
- To use in Control Tables.
Advantages of Pointers
Following are the major advantages of pointers in C:
- Pointers are used for dynamic memory allocation and deallocation.
- An Array or a structure can be accessed efficiently with pointers
- Pointers are useful for accessing memory locations.
- Pointers are used to form complex data structures such as linked lists, graphs, trees, etc.
- Pointers reduce the length of the program and its execution time as well.
Disadvantages of Pointers
Pointers are vulnerable to errors and have following disadvantages:
- Memory corruption can occur if an incorrect value is provided to pointers.
- Pointers are a little bit complex to understand.
- Pointers are majorly responsible for memory leaks in C .
- Pointers are comparatively slower than variables in C.
- Uninitialized pointers might cause a segmentation fault.
In conclusion, pointers in C are very capable tools and provide C language with its distinguishing features, such as low-level memory access, referencing, etc. But as powerful as they are, they should be used with responsibility as they are one of the most vulnerable parts of the language.
FAQs on Pointers in C
Q1. define pointers..
Pointers are the variables that can store the memory address of another variable.
Q2. What is the difference between a constant pointer and a pointer to a constant?
A constant pointer points to the fixed memory location, i.e. we cannot change the memory address stored inside the constant pointer. On the other hand, the pointer to a constant point to the memory with a constant value.
Q3. What is pointer to pointer?
A pointer to a pointer (also known as a double pointer) stores the address of another pointer.
Q4. Does pointer size depends on its type?
No, the pointer size does not depend upon its type. It only depends on the operating system and CPU architecture.
Q5. What are the differences between an array and a pointer?
The following table list the differences between an array and a pointer : Pointer Array A pointer is a derived data type that can store the address of other variables. An array is a homogeneous collection of items of any type such as int, char, etc. Pointers are allocated at run time. Arrays are allocated at runtime. The pointer is a single variable. An array is a collection of variables of the same type. Dynamic in Nature Static in Nature.
Q6. Why do we need to specify the type in the pointer declaration?
Type specification in pointer declaration helps the compiler in dereferencing and pointer arithmetic operations.
- Quiz on Pointer Basics
- Quiz on Advanced Pointer
Please Login to comment...
Similar reads.
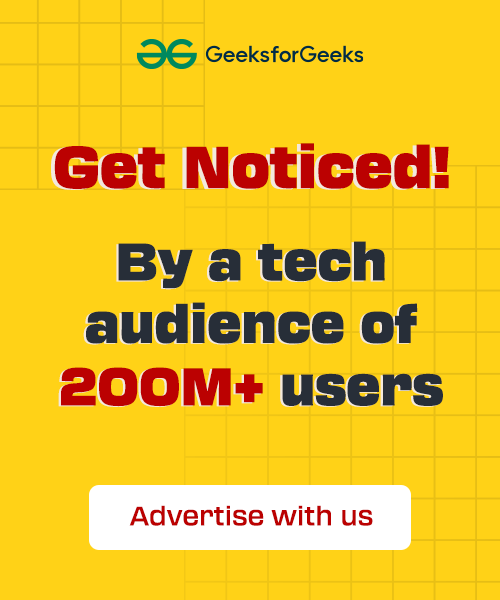
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
What is a Pointer?
Declaring pointers:, de-referencing pointers:, pointer types:, the "address of" operator:, pass by address:, pointers and arrays:, pointer arithmetic.
- A pointer can be incremented (++) or decremented (--)
- An integer may be added to a pointer (+ or +=)
- An integer may be subtracted from a pointer (- or -=)
- One pointer may be subtracted from another
Pass By Address with arrays:

- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
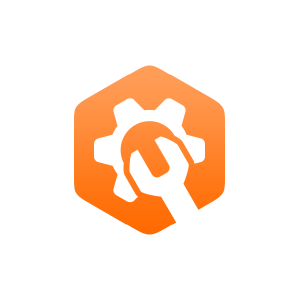
12.1: Address Operator
- Last updated
- Save as PDF
- Page ID 29103
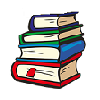
- Patrick McClanahan
- San Joaquin Delta College
Address Operator in C++
"Every variable is assigned a memory location whose address can be retrieved using the address operator &. The address of a memory location is called a pointer. Every variable in an executing program is allocated a section of memory large enough to hold a value of that variable’s type." Thus, whether the variables are global scope and use the data area for storage or local scope and use the stack for storage; you can ask the question at what address in the memory does this variable exist. Given an integer variable named age:
int age = 47;
We can use the address operator [which is the ampersand or &] to determine where it exists (or its address) in the memory by:
This expression is a pointer data type. The concept of an address and a pointer are one in the same. A pointer points to the location in memory because the value of a pointer is the address were the data item resides in the memory.
The address operator is commonly used in two ways:
To do parameter passing by reference
To establish the value of pointers
Both of these items are covered in the supplemental links to this module.
You can print out the value of the address with the following code:
cout << &age;
This will by default print the value in hexadecimal. Some people prefer an integer value and to print it as an integer you will need to cast the address into a long data type:
cout << long(&age);
One additional tidbit, an array’s name is by definition a pointer to the arrays first element. Thus:
int iqs[] = {122, 105, 131, 97};
establishes "iqs" as a pointer to the array.
Definitions
Tony Gaddis, Judy Walters and Godfrey Muganda, Starting Out with C++ Early Objects Sixth Edition (United States of America: Pearson – Addison Wesley, 2008) 597.
Assignment of a Fixed Address to a Pointer
The product sets a pointer to a specific address other than NULL or 0.
CWE Catalog - 4.12
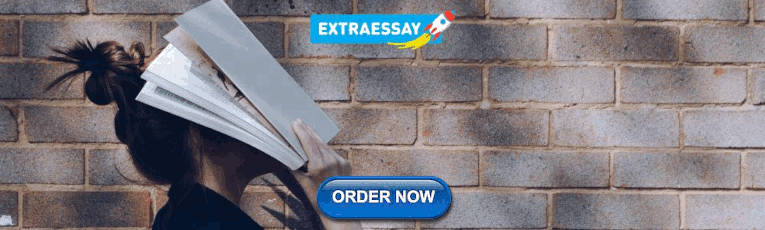
Description
Example one.
Using a fixed address is not portable, because that address will probably not be valid in all environments or platforms.
Demonstrations
The following examples help to illustrate the nature of this weakness and describe methods or techniques which can be used to mitigate the risk.
Note that the examples here are by no means exhaustive and any given weakness may have many subtle varieties, each of which may require different detection methods or runtime controls.
This code assumes a particular function will always be found at a particular address. It assigns a pointer to that address and calls the function.
The same function may not always be found at the same memory address. This could lead to a crash, or an attacker may alter the memory at the expected address, leading to arbitrary code execution.
Weaknesses in this category are related to memory safety.
Weaknesses in this category are related to the rules and recommendations in the Integers (INT) section of the SEI CERT C Coding Standard.
This category identifies Software Fault Patterns (SFPs) within the Glitch in Computation cluster (SFP1).
This view (slice) covers all the elements in CWE.
CWE identifiers in this view (slice) are quality issues that only indirectly make it easier to introduce a vulnerability and/or make the vulnerability more difficult t...
This view contains a selection of weaknesses that represent the variety of weaknesses that are captured in CWE, at a level of abstraction that is likely to be useful t...
Common Weakness Enumeration content on this website is copyright of The MITRE Corporation unless otherwise specified. Use of the Common Weakness Enumeration and the associated references on this website are subject to the Terms of Use as specified by The MITRE Corporation.
404 Not found
C Pointers and Memory Allocation
Other resources, declarations, creating a valid pointer value.
int *ip; int x; x = 42; ip = &x;
Using a pointer
printf("%d %d\n", x, *ip);
Memory Allocation and Deallocation
(void *) malloc(size_t numbytes);
int *ip; ip = (int *) malloc(sizeof(int));
Pointers and arrays
int *ip; ip = (int *) malloc( sizeof(int)*10 ); // allocate 10 ints ip[6] = 42; // set the 7th element to 42 ip[10] = 99; // WRONG: array only has 10 elements (this would corrupted memory!)
Freeing memory, and memory leaks
int *ip; ip = (int *) malloc( sizeof(int)*10 ); // allocate 10 ints ... (use the array, etc.) ip = (int *) malloc( sizeof(int)*100 ); // allocate 100 ints ...
int *ip; ip = (int *) malloc( sizeof(int)*10 ); // allocate 10 ints ... (use the array, etc.) free(ip); // de-allocate old array ip = (int *) malloc( sizeof(int)*100 ); // allocate 100 ints ...
Pointers and arrays as arguments
7.0 Pointers
7.1.0 pointer basics.
int i = 5; ip = &i;
ip = &i;
*ip = &i;
int i = 5; int *ip; ip = &i; // Point ip to the memory address of variable i. *ip = 10; // Change the contents of the variable ip points to to 10.
7.2.0 Pointers and Arrays - Pointer Arithmetic
int a[10]; int *pa = &a[0];
int a[10]; int *pa = a;
int a[10]; int *pa = &a[4];
int a[10]; int *pa = a+4; // Converts to &a[0] + 4 or &a[4]
int a[5] = {1,2,3,4,5}; int *pa = a; int i, total = 0; for(i = 0; i
int a[5] = {1,2,3,4,5}; int *pa = a; pa = pa+3; // Increments the pointer by 3 *pa = *pa+3 // WRONG: Increments the contents of the variable // pointed to by pa by 3
7.3.0 Pointers as function arguments
char function(char a[]); char function(char *a);
void function (char a[], int len) { int k; for(k = 0; k

12.7 — Introduction to pointers
Pointers are one of C++’s historical boogeymen, and a place where many aspiring C++ learners have gotten stuck. However, as you’ll see shortly, pointers are nothing to be scared of.
In fact, pointers behave a lot like lvalue references. But before we explain that further, let’s do some setup.
Related content
If you’re rusty or not familiar with lvalue references, now would be a good time to review them. We cover lvalue references in lessons 12.3 -- Lvalue references , 12.4 -- Lvalue references to const , and 12.5 -- Pass by lvalue reference .
Consider a normal variable, like this one:
Simplifying a bit, when the code generated for this definition is executed, a piece of memory from RAM will be assigned to this object. For the sake of example, let’s say that the variable x is assigned memory address 140 . Whenever we use variable x in an expression or statement, the program will go to memory address 140 to access the value stored there.
The nice thing about variables is that we don’t need to worry about what specific memory addresses are assigned, or how many bytes are required to store the object’s value. We just refer to the variable by its given identifier, and the compiler translates this name into the appropriately assigned memory address. The compiler takes care of all the addressing.
This is also true with references:
Because ref acts as an alias for x , whenever we use ref , the program will go to memory address 140 to access the value. Again the compiler takes care of the addressing, so that we don’t have to think about it.
The address-of operator (&)
Although the memory addresses used by variables aren’t exposed to us by default, we do have access to this information. The address-of operator (&) returns the memory address of its operand. This is pretty straightforward:
On the author’s machine, the above program printed:
In the above example, we use the address-of operator (&) to retrieve the address assigned to variable x and print that address to the console. Memory addresses are typically printed as hexadecimal values (we covered hex in lesson 5.3 -- Numeral systems (decimal, binary, hexadecimal, and octal) ), often without the 0x prefix.
For objects that use more than one byte of memory, address-of will return the memory address of the first byte used by the object.
The & symbol tends to cause confusion because it has different meanings depending on context:
- When following a type name, & denotes an lvalue reference: int& ref .
- When used in a unary context in an expression, & is the address-of operator: std::cout << &x .
- When used in a binary context in an expression, & is the Bitwise AND operator: std::cout << x & y .
The dereference operator (*)
Getting the address of a variable isn’t very useful by itself.
The most useful thing we can do with an address is access the value stored at that address. The dereference operator (*) (also occasionally called the indirection operator ) returns the value at a given memory address as an lvalue:
This program is pretty simple. First we declare a variable x and print its value. Then we print the address of variable x . Finally, we use the dereference operator to get the value at the memory address of variable x (which is just the value of x ), which we print to the console.
Key insight
Given a memory address, we can use the dereference operator (*) to get the value at that address (as an lvalue).
The address-of operator (&) and dereference operator (*) work as opposites: address-of gets the address of an object, and dereference gets the object at an address.
Although the dereference operator looks just like the multiplication operator, you can distinguish them because the dereference operator is unary, whereas the multiplication operator is binary.
Getting the memory address of a variable and then immediately dereferencing that address to get a value isn’t that useful either (after all, we can just use the variable to access the value).
But now that we have the address-of operator (&) and dereference operator (*) added to our toolkits, we’re ready to talk about pointers.
A pointer is an object that holds a memory address (typically of another variable) as its value. This allows us to store the address of some other object to use later.
As an aside…
In modern C++, the pointers we are talking about here are sometimes called “raw pointers” or “dumb pointers”, to help differentiate them from “smart pointers” that were introduced into the language more recently. We cover smart pointers in chapter 22 .
Much like reference types are declared using an ampersand (&) character, pointer types are declared using an asterisk (*):
To create a pointer variable, we simply define a variable with a pointer type:
Note that this asterisk is part of the declaration syntax for pointers, not a use of the dereference operator.
Best practice
When declaring a pointer type, place the asterisk next to the type name.
Although you generally should not declare multiple variables on a single line, if you do, the asterisk has to be included with each variable.
Although this is sometimes used as an argument to not place the asterisk with the type name (instead placing it next to the variable name), it’s a better argument for avoiding defining multiple variables in the same statement.
Pointer initialization
Like normal variables, pointers are not initialized by default. A pointer that has not been initialized is sometimes called a wild pointer . Wild pointers contain a garbage address, and dereferencing a wild pointer will result in undefined behavior. Because of this, you should always initialize your pointers to a known value.
Always initialize your pointers.
Since pointers hold addresses, when we initialize or assign a value to a pointer, that value has to be an address. Typically, pointers are used to hold the address of another variable (which we can get using the address-of operator (&)).
Once we have a pointer holding the address of another object, we can then use the dereference operator (*) to access the value at that address. For example:
This prints:
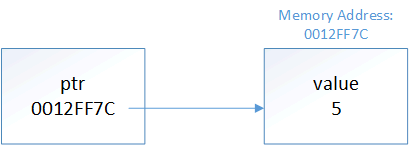
This is where pointers get their name from -- ptr is holding the address of x , so we say that ptr is “pointing to” x .
Author’s note
A note on pointer nomenclature: “X pointer” (where X is some type) is a commonly used shorthand for “pointer to an X”. So when we say, “an integer pointer”, we really mean “a pointer to an integer”. This understanding will be valuable when we talk about const pointers.
Much like the type of a reference has to match the type of object being referred to, the type of the pointer has to match the type of the object being pointed to:
With one exception that we’ll discuss next lesson, initializing a pointer with a literal value is disallowed:
Pointers and assignment
We can use assignment with pointers in two different ways:
- To change what the pointer is pointing at (by assigning the pointer a new address)
- To change the value being pointed at (by assigning the dereferenced pointer a new value)
First, let’s look at a case where a pointer is changed to point at a different object:
The above prints:
In the above example, we define pointer ptr , initialize it with the address of x , and dereference the pointer to print the value being pointed to ( 5 ). We then use the assignment operator to change the address that ptr is holding to the address of y . We then dereference the pointer again to print the value being pointed to (which is now 6 ).
Now let’s look at how we can also use a pointer to change the value being pointed at:
This program prints:
In this example, we define pointer ptr , initialize it with the address of x , and then print the value of both x and *ptr ( 5 ). Because *ptr returns an lvalue, we can use this on the left hand side of an assignment statement, which we do to change the value being pointed at by ptr to 6 . We then print the value of both x and *ptr again to show that the value has been updated as expected.
When we use a pointer without a dereference ( ptr ), we are accessing the address held by the pointer. Modifying this ( ptr = &y ) changes what the pointer is pointing at.
When we dereference a pointer ( *ptr ), we are accessing the object being pointed at. Modifying this ( *ptr = 6; ) changes the value of the object being pointed at.
Pointers behave much like lvalue references
Pointers and lvalue references behave similarly. Consider the following program:
In the above program, we create a normal variable x with value 5 , and then create an lvalue reference and a pointer to x . Next, we use the lvalue reference to change the value from 5 to 6 , and show that we can access that updated value via all three methods. Finally, we use the dereferenced pointer to change the value from 6 to 7 , and again show that we can access the updated value via all three methods.
Thus, pointers and references both provide a way to indirectly access another object. The primary difference is that with pointers, we need to explicitly get the address to point at, and we have to explicitly dereference the pointer to get the value. With references, the address-of and dereference happens implicitly.
There are some other differences between pointers and references worth mentioning:
- References must be initialized, pointers are not required to be initialized (but should be).
- References are not objects, pointers are.
- References can not be reseated (changed to reference something else), pointers can change what they are pointing at.
- References must always be bound to an object, pointers can point to nothing (we’ll see an example of this in the next lesson).
- References are “safe” (outside of dangling references), pointers are inherently dangerous (we’ll also discuss this in the next lesson).
The address-of operator returns a pointer
It’s worth noting that the address-of operator (&) doesn’t return the address of its operand as a literal. Instead, it returns a pointer containing the address of the operand, whose type is derived from the argument (e.g. taking the address of an int will return the address in an int pointer).
We can see this in the following example:
On Visual Studio, this printed:
With gcc, this prints “pi” (pointer to int) instead. Because the result of typeid().name() is compiler-dependent, your compiler may print something different, but it will have the same meaning.
The size of pointers
The size of a pointer is dependent upon the architecture the executable is compiled for -- a 32-bit executable uses 32-bit memory addresses -- consequently, a pointer on a 32-bit machine is 32 bits (4 bytes). With a 64-bit executable, a pointer would be 64 bits (8 bytes). Note that this is true regardless of the size of the object being pointed to:
The size of the pointer is always the same. This is because a pointer is just a memory address, and the number of bits needed to access a memory address is constant.
Dangling pointers
Much like a dangling reference, a dangling pointer is a pointer that is holding the address of an object that is no longer valid (e.g. because it has been destroyed).
Dereferencing a dangling pointer (e.g. in order to print the value being pointed at) will lead to undefined behavior, as you are trying to access an object that is no longer valid.
Perhaps surprisingly, the standard says “Any other use of an invalid pointer value has implementation-defined behavior”. This means that you can assign an invalid pointer a new value, such as nullptr (because this doesn’t use the invalid pointer’s value). However, any other operations that use the invalid pointer’s value (such as copying or incrementing an invalid pointer) will yield implementation-defined behavior.
Dereferencing an invalid pointer will lead to undefined behavior. Any other use of an invalid pointer value is implementation-defined.
Here’s an example of creating a dangling pointer:
The above program will probably print:
But it may not, as the object that ptr was pointing at went out of scope and was destroyed at the end of the inner block, leaving ptr dangling.
Pointers are variables that hold a memory address. They can be dereferenced using the dereference operator (*) to retrieve the value at the address they are holding. Dereferencing a wild or dangling (or null) pointer will result in undefined behavior and will probably crash your application.
Pointers are both more flexible than references and more dangerous. We’ll continue to explore this in the upcoming lessons.
Question #1
What values does this program print? Assume a short is 2 bytes, and a 32-bit machine.
Show Solution
A brief explanation about the 4 and the 2. A 32-bit machine means that pointers will be 32 bits in length, but sizeof() always prints the size in bytes. 32 bits is 4 bytes. Thus the sizeof(ptr) is 4. Because ptr is a pointer to a short, *ptr is a short. The size of a short in this example is 2 bytes. Thus the sizeof(*ptr) is 2.
Question #2
What’s wrong with this snippet of code?
The last line of the above snippet doesn’t compile.
Let’s examine this program in more detail.
The first line contains a standard variable definition, along with an initialization value. Nothing special here.
In the second line, we’re defining a new pointer named ptr , and initializing it with the address of value . Remember that in this context, the asterisk is part of the pointer declaration syntax, not a dereference. So this line is fine.
On line three, the asterisk represents a dereference, which is used to get the value that a pointer is pointing to. So this line says, “retrieve the value that ptr is pointing to (an integer), and overwrite it with the address of value (an address). That doesn’t make any sense -- you can’t assign an address to an integer!
The third line should be:
This correctly assigns the address of variable value to the pointer.
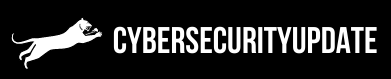
Cyber Security News
Fraudsters exploit telegram’s popularity for toncoin scam, using legitimate github urls for malware, how to spot ai audio deepfakes at election time, dependency confusion vulnerability found in apache project, crushftp file transfer vulnerability lets attackers download system files, nsa launches guidance for secure ai deployment, ncsc announces pwc’s richard horne as new ceo, mitre reveals ivanti breach by nation state actor, friday squid blogging: squid trackers, cwe-587 – assignment of a fixed address to a pointer.
Description
The software sets a pointer to a specific address other than NULL or 0.
Using a fixed address is not portable, because that address will probably not be valid in all environments or platforms.
Modes of Introduction:
– Architecture and Design
Related Weaknesses
CWE-344 CWE-758
Consequences
Integrity, Confidentiality, Availability : Execute Unauthorized Code or Commands
If one executes code at a known location, an attacker might be able to inject code there beforehand.
Availability : DoS: Crash, Exit, or Restart, Reduce Maintainability, Reduce Reliability
If the code is ported to another platform or environment, the pointer is likely to be invalid and cause a crash.
Confidentiality, Integrity : Read Memory, Modify Memory
The data at a known pointer location can be easily read or influenced by an attacker.
Potential Mitigations
Phase: Implementation
Description:
Never set a pointer to a fixed address.
CVE References
More stories, the most dangerous vulnerabilities in apache tomcat and how to protect against them.
Apache Tomcat is an open-source web server and servlet container that is widely used in enterprise environments to run Java...
ZDI-CAN-18333: A Critical Zero-Day Vulnerability in Microsoft Windows
Zero-day vulnerabilities are a serious threat to cybersecurity, as they can be exploited by malicious actors to gain unauthorized access...
CWE-669 – Incorrect Resource Transfer Between Spheres
Description The product does not properly transfer a resource/behavior to another sphere, or improperly imports a resource/behavior from another sphere,...
CWE-67 – Improper Handling of Windows Device Names
Description The software constructs pathnames from user input, but it does not handle or incorrectly handles a pathname containing a...
CWE-670 – Always-Incorrect Control Flow Implementation
Description The code contains a control flow path that does not reflect the algorithm that the path is intended to...
CWE-671 – Lack of Administrator Control over Security
Description The product uses security features in a way that prevents the product's administrator from tailoring security settings to reflect...
On occasion, developers may want to access and work with memory, and with pointers to memory locations, directly. This is necessary, for example, for certain operating system interactions as well as with certain types of time-critical algorithms. To support this capability, C# requires use of the unsafe code construct.
One of C#’s great features is the fact that it is strongly typed and supports type checking throughout the runtime execution. What makes this feature especially beneficial is that it is possible to circumvent this support and manipulate memory and addresses directly. You would do so when working with memory-mapped devices, for example, or if you wanted to implement time-critical algorithms. The key is to designate a portion of the code as unsafe.
Unsafe code is an explicit code block and compilation option, as shown in Listing 23.10 . The unsafe modifier has no effect on the generated CIL code itself, but rather is a directive to the compiler to permit pointer and address manipulation within the unsafe block. Furthermore, unsafe does not imply unmanaged.
You can use unsafe as a modifier to the type or to specific members within the type.
In addition, C# allows unsafe as a statement that flags a code block to allow unsafe code (see Listing 23.11 ).
Code within the unsafe block can include unsafe constructs such as pointers.
When you write unsafe code, your code becomes vulnerable to the possibility of buffer overflows and similar outcomes that may potentially expose security holes. For this reason, it is necessary to explicitly notify the compiler that unsafe code occurs. To accomplish this, set AllowUnsafeBlocks to true in your CSPROJ file, as shown in Listing 23.12 . Alternatively, you can pass the property on the command line when running dotnet build (see Output 23.1 ). Or, if invoking the C# compiler directly, you need the /unsafe switch (see Output 23.2 ).
With Visual Studio, you can activate this feature by checking the Allow Unsafe Code checkbox from the Build tab of the Project Properties window.
The /unsafe switch enables you to directly manipulate memory and execute instructions that are unmanaged. Requiring /unsafe , therefore, makes explicit any exposure to potential security vulnerabilities that such code might introduce. With great power comes great responsibility.
Now that you have marked a code block as unsafe, it is time to look at how to write unsafe code. First, unsafe code allows the declaration of a pointer. Consider the following example:
byte* pData;
Assuming pData is not null , its value points to a location that contains one or more sequential byte s; the value of pData represents the memory address of the byte s. The type specified before the * is the referent type —that is, the type located where the value of the pointer refers. In this example, pData is the pointer and byte is the referent type, as shown in Figure 23.1 .
Because pointers are simply integers that happen to refer to a memory address, they are not subject to garbage collection. C# does not allow referent types other than unmanaged types , which are types that are not reference types, are not generics, and do not contain reference types. Therefore, the following command is not valid:
string* pMessage;
Likewise, this command is not valid:
ServiceStatus* pStatus;
where ServiceStatus is defined as shown in Listing 23.13 . The problem, once again, is that ServiceStatus includes a string field.
In C/C++, multiple pointers within the same declaration are declared as follows:
int *p1, *p2;
Notice the * on p2 ; this makes p2 an int* rather than an int . In contrast, C# always places the * with the data type:
int* p1, p2;
The result is two variables of type int* . The syntax matches that of declaring multiple arrays in a single statement:
int[] array1, array2;
Pointers are an entirely new category of type. Unlike structs, enums, and classes, pointers don’t ultimately derive from System.Object and are not even convertible to System.Object . Instead, they are convertible (explicitly) to System.IntPtr (which can be converted to System.Object ).
In addition to custom structs that contain only unmanaged types, valid referent types include enums, predefined value types ( sbyte , byte , short , ushort , int , uint , long , ulong , char , float , double , decimal , and bool ), and pointer types (such as byte** ). Lastly, valid syntax includes void* pointers, which represent pointers to an unknown type.
Once code defines a pointer, it needs to assign a value before accessing it. Just like reference types, pointers can hold the value null , which is their default value. The value stored by the pointer is the address of a location. Therefore, to assign the pointer, you must first retrieve the address of the data.
You could explicitly cast an int or a long into a pointer, but this rarely occurs without a means of determining the address of a particular data value at execution time. Instead, you need to use the address operator ( & ) to retrieve the address of the value type:
byte* pData = &bytes[0]; // Compile error
The problem is that in a managed environment, data can move, thereby invalidating the address. The resulting error message will be “You can only take the address of [an] unfixed expression inside a fixed statement initializer.” In this case, the byte referenced appears within an array, and an array is a reference type (a movable type). Reference types appear on the heap and are subject to garbage collection or relocation. A similar problem occurs when referring to a value type field on a movable type:
int* a = &"message".Length;
Either way, assigning an address of some data requires that the following criteria are met:
If the data is an unmanaged variable type but is not fixed, use the fixed statement to fix a movable variable.
To retrieve the address of a movable data item, it is necessary to fix, or pin, the data, as demonstrated in Listing 23.14 .
Within the code block of a fixed statement, the assigned data will not move. In this example, bytes will remain at the same address, at least until the end of the fixed statement.
The fixed statement requires the declaration of the pointer variable within its scope. This avoids accessing the variable outside the fixed statement, when the data is no longer fixed. However, as a programmer, you are responsible for ensuring that you do not assign the pointer to another variable that survives beyond the scope of the fixed statement—possibly in an API call, for example. Unsafe code is called “unsafe” for a reason; you must ensure that you use the pointers safely, rather than relying on the runtime to enforce safety on your behalf. Similarly, using ref or out parameters will be problematic for data that will not survive beyond the method call.
Since a string is an invalid referent type, it would appear to be invalid to define pointers to strings. However, as in C++, internally a string is a pointer to the first character of an array of characters, and it is possible to declare pointers to characters using char* . Therefore, C# allows for declaring a pointer of type char* and assigning it to a string within a fixed statement. The fixed statement prevents the movement of the string during the life of the pointer. Similarly, it allows any movable type that supports an implicit conversion to a pointer of another type, given a fixed statement.
You can replace the verbose assignment of &bytes[0] with the abbreviated bytes , as shown in Listing 23.15 .
Depending on the frequency and time needed for their execution, fixed statements may have the potential to cause fragmentation in the heap because the garbage collector cannot compact fixed objects. To reduce this problem, the best practice is to pin blocks early in the execution and to pin fewer large blocks rather than many small blocks. Unfortunately, this preference must be tempered with the practice of pinning as little as possible for as short a time as possible, so as to minimize the chance that a collection will happen during the time that the data is pinned. To some extent, .NET 2.0 reduces this problem through its inclusion of some additional fragmentation-aware code.
Potentially you might need to fix an object in place in one method body and have it remain fixed until another method is called; this is not possible with the fixed statement. If you are in this unfortunate situation, you can use methods on the GCHandle object to fix an object in place indefinitely. You should do so only if it is absolutely necessary, however; fixing an object for a long time makes it highly likely that the garbage collector will be unable to efficiently compact memory.
You should use the fixed statement on an array to prevent the garbage collector from moving the data. However, an alternative is to allocate the array on the call stack. Stack allocated data is not subject to garbage collection or to the finalizer patterns that accompany it. Like referent types, the requirement is that the stackalloc data is an array of unmanaged types. For example, instead of allocating an array of bytes on the heap, you can place it onto the call stack, as shown in Listing 23.16 .
Because the data type is an array of unmanaged types, the runtime can allocate a fixed buffer size for the array and then restore that buffer once the pointer goes out of scope. Specifically, it allocates sizeof(T) * E , where E is the array size and T is the referent type. Given the requirement of using stackalloc only on an array of unmanaged types, the runtime restores the buffer back to the system by simply unwinding the stack, thereby eliminating the complexities of iterating over the f-reachable queue (see the “Garbage Collection” section and discussion of finalization in Chapter 10) and compacting reachable data. Thus, there is no way to explicitly free stackalloc data.
The stack is a precious resource. Although it is small, running out of stack space will have a big effect—namely, the program will crash. For this reason, you should make every effort to avoid running out stack space. If a program does run out of stack space, the best thing that can happen is for the program to shut down/crash immediately. Generally, programs have less than 1MB of stack space (and possibly a lot less). Therefore, take great care to avoid allocating arbitrarily sized buffers on the stack.
Accessing the data stored in a variable of a type referred to by a pointer requires that you dereference the pointer, placing the indirection operator prior to the expression. For example, byte data = *pData; dereferences the location of the byte referred to by pData and produces a variable of type byte . The variable provides read/write access to the single byte at that location.
Using this principle in unsafe code allows the unorthodox behavior of modifying the “immutable” string, as shown in Listing 23.17 with Output 23.3 . In no way is this strategy recommended, even though it does expose the potential of low-level memory manipulation.
In this case, you take the original address and increment it by the size of the referent type ( sizeof(char) ), using the pre-increment operator. Next, you dereference the address using the indirection operator and then assign the location with a different character. Similarly, using the + and – operators on a pointer changes the address by the * sizeof(T) operand, where T is the referent type.
The comparison operators ( == , != , < , > , <= , and >= ) also work to compare pointers. Thus, their use effectively translates to a comparison of address location values.
One restriction on the dereferencing operator is the inability to dereference a void* . The void* data type represents a pointer to an unknown type. Since the data type is unknown, it can’t be dereferenced to produce a variable. Instead, to access the data referenced by a void* , you must convert it to another pointer type and then dereference the latter type.
You can achieve the same behavior as implemented in Listing 23.17 by using the index operator rather than the indirection operator (see Listing 23.18 with Output 23.4 ).
Modifications such as those in Listing 23.17 and Listing 23.18 can lead to unexpected behavior. For example, if you reassigned text to "S5280ft" following the Console.WriteLine() statement and then redisplayed text , the output would still be Smile because the address of two equal string literals is optimized to one string literal referenced by both variables. In spite of the apparent assignment
text = "S5280ft";
after the unsafe code in Listing 23.17 , the internals of the string assignment are an address assignment of the modified "S5280ft" location, so text is never set to the intended value.
Dereferencing a pointer produces a variable of the pointer’s underlying type. You can then access the members of the underlying type using the member access dot operator in the usual way. However, the rules of operator precedence require that *x.y means *(x.y) , which is probably not what you intended. If x is a pointer, the correct code is (*x).y , which is an unpleasant syntax. To make it easier to access members of a dereferenced pointer, C# provides a special member access operator: x->y is a shorthand for (*x).y , as shown in Listing 23.19 with Output 23.5 .
- p.key == item.key) && !currentPage.some(p => p.level > item.level), }" > p.key == item.key) && !currentPage.some(p => p.level > item.level), }" :href="item.href"> Introduction
- p.key == item.key) && !currentPage.some(p => p.level > item.level), }" > p.key == item.key), }" :href="item.href"> {{item.title}}
Firefox is no longer supported on Windows 8.1 and below.
Please download Firefox ESR (Extended Support Release) to use Firefox.
Download Firefox ESR 64-bit
Download Firefox ESR 32-bit
Firefox is no longer supported on macOS 10.14 and below.
Mozilla Foundation Security Advisory 2024-18
Security vulnerabilities fixed in firefox 125.
- Firefox 125
# CVE-2024-3852: GetBoundName in the JIT returned the wrong object
Description.
GetBoundName could return the wrong version of an object when JIT optimizations were applied.
- Bug 1883542
# CVE-2024-3853: Use-after-free if garbage collection runs during realm initialization
A use-after-free could result if a JavaScript realm was in the process of being initialized when a garbage collection started.
- Bug 1884427
# CVE-2024-3854: Out-of-bounds-read after mis-optimized switch statement
In some code patterns the JIT incorrectly optimized switch statements and generated code with out-of-bounds-reads.
- Bug 1884552
# CVE-2024-3855: Incorrect JIT optimization of MSubstr leads to out-of-bounds reads
In certain cases the JIT incorrectly optimized MSubstr operations, which led to out-of-bounds reads.
- Bug 1885828
# CVE-2024-3856: Use-after-free in WASM garbage collection
A use-after-free could occur during WASM execution if garbage collection ran during the creation of an array.
- Bug 1885829
# CVE-2024-3857: Incorrect JITting of arguments led to use-after-free during garbage collection
The JIT created incorrect code for arguments in certain cases. This led to potential use-after-free crashes during garbage collection.
- Bug 1886683
# CVE-2024-3858: Corrupt pointer dereference in js::CheckTracedThing<js::Shape>
It was possible to mutate a JavaScript object so that the JIT could crash while tracing it.
- Bug 1888892
# CVE-2024-3859: Integer-overflow led to out-of-bounds-read in the OpenType sanitizer
On 32-bit versions there were integer-overflows that led to an out-of-bounds-read that potentially could be triggered by a malformed OpenType font.
- Bug 1874489
# CVE-2024-3860: Crash when tracing empty shape lists
An out-of-memory condition during object initialization could result in an empty shape list. If the JIT subsequently traced the object it would crash.
- Bug 1881417
# CVE-2024-3861: Potential use-after-free due to AlignedBuffer self-move
If an AlignedBuffer were assigned to itself, the subsequent self-move could result in an incorrect reference count and later use-after-free.
- Bug 1883158
# CVE-2024-3862: Potential use of uninitialized memory in MarkStack assignment operator on self-assignment
The MarkStack assignment operator, part of the JavaScript engine, could access uninitialized memory if it were used in a self-assignment.
- Bug 1884457
# CVE-2024-3863: Download Protections were bypassed by .xrm-ms files on Windows
The executable file warning was not presented when downloading .xrm-ms files. Note: This issue only affected Windows operating systems. Other operating systems are unaffected.
- Bug 1885855
# CVE-2024-3302: Denial of Service using HTTP/2 CONTINUATION frames
There was no limit to the number of HTTP/2 CONTINUATION frames that would be processed. A server could abuse this to create an Out of Memory condition in the browser.
- Bug 1881183
- VU#421644 - HTTP/2 CONTINUATION frames can be utilized for DoS attacks
# CVE-2024-3864: Memory safety bug fixed in Firefox 125, Firefox ESR 115.10, and Thunderbird 115.10
Memory safety bug present in Firefox 124, Firefox ESR 115.9, and Thunderbird 115.9. This bug showed evidence of memory corruption and we presume that with enough effort this could have been exploited to run arbitrary code.
- Memory safety bug fixed in Firefox 125, Firefox ESR 115.10, and Thunderbird 115.10
# CVE-2024-3865: Memory safety bugs fixed in Firefox 125
Memory safety bugs present in Firefox 124. Some of these bugs showed evidence of memory corruption and we presume that with enough effort some of these could have been exploited to run arbitrary code.
- Memory safety bugs fixed in Firefox 125
FixedAddr -- Assignment of a Fixed Address to a Pointer
Go Up to C++ Audits
Description
FixedAddr checks for the assignment of a fixed address other than NULL or 0 to a pointer.
Using a fixed address is not portable, because that address will probably not be valid in all environments or platforms.
- C++ Audits Configuration dialog box
- C++ Audits pane
- Running C++ Audits
- Source Code Audits and Metrics
- RAD Studio Reference
Navigation menu
Personal tools.
- View source
- View history
RAD Studio 10.4 Sydney
- Libraries Reference
- Code Examples
RAD Studio 10.4 Topics
- FireMonkey Application Platform
- Multi-Device Applications
- Getting Started
- Steps in Developing a Project
- Key Application Types
- Windows Developer's Guide
- Modeling Tools
- IDE Reference and Utilities
- Delphi Reference
- C++ Reference
- Subject Index
In other languages
Previous versions.
- Latest Version
- Tokyo Topics
- Older Versions
- Recent changes
- What links here
- Related changes
- Special pages
- Printable version
- Permanent link
- Page information
- This page was last edited on 4 January 2016, at 08:59.
- Privacy policy
- About RAD Studio
- Disclaimers
- Help Feedback ( QP , email )
- Mobile view
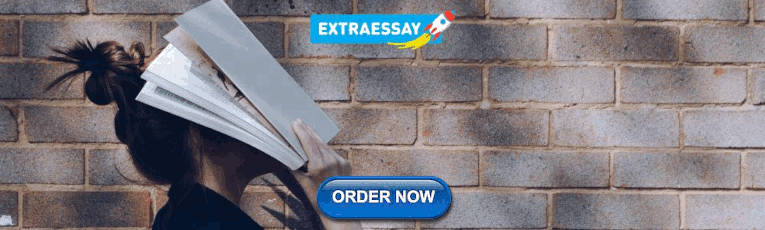
IMAGES
VIDEO
COMMENTS
How do you assign a specific memory address to a pointer? The Special Function Registers in a microcontroller such AVR m128 has fixed addresses, the AVR GCC defines the SFR in the io.h header file, but I want to handle it myself.
You need to split in two instructions: int *p= (int *)0x1234; *p = 10; Not to mention that you can't assign values to random memory addresses that your program doesn't "own". If you try to access the value of a memory address (read or write), the program will likely close. The OS prohibits you.
CWE-587: Assignment of a Fixed Address to a Pointer. Weakness ID: 587. Vulnerability Mapping: ALLOWEDThis CWE ID may be used to map to real-world vulnerabilitiesAbstraction: VariantVariant - a weakness that is linked to a certain type of product, typically involving a specific language or technology. More specific than a Base weakness.
In constant pointers, the memory address stored inside the pointer is constant and cannot be modified once it is defined. It will always point to the same memory address. ... Assignment of pointers of the same type. ... A constant pointer points to the fixed memory location, i.e. we cannot change the memory address stored inside the constant ...
Arrays of pointers. Pointers to pointers have a few uses. The most common use is to dynamically allocate an array of pointers: int** array { new int*[10] }; // allocate an array of 10 int pointers. This works just like a standard dynamically allocated array, except the array elements are of type "pointer to integer" instead of integer.
The null pointer is the only literal number you may assign to a pointer, since it is often used to initialize pointers or to be used as a signal. You may NOT assign arbitrary numbers to pointer variables: int * p = 0; // okay. assignment of null pointer to p int * q; q = 0; // okay. null pointer again. int * z; z = 900; // BAD!
We can use the address operator [which is the ampersand or &] to determine where it exists (or its address) in the memory by: &age. This expression is a pointer data type. The concept of an address and a pointer are one in the same. A pointer points to the location in memory because the value of a pointer is the address were the data item ...
This code assumes a particular function will always be found at a particular address. It assigns a pointer to that address and calls the function. int (*pt2Function) (float, char, char)=0x08040000; int result2 = (*pt2Function) (12, 'a', 'b'); // Here we can inject code to execute. The same function may not always be found at the same memory ...
Chapter 5. Pointers in C. 5.4. Assigning an address to a pointer. Given a variable var of type t and a variable var_ptr of type pointer to t ( t. * ), it possible to assign. var_ptr = &var. The following example shows the declaration and value assignment of pointers (file pointer_example_1.c ): 1.
Line 13 is a pointer assignment. The value from ptr2 your the address of num2 as it was assigned previously. As ampere result, ptr1 contains now also the address of num2. Line 14 assigns to pointer ptr2 an constant NULL defined in the stdio.h included in the first lineage of the program. This constant representing the " empty pointer ". Its ...
One way to create a pointer value is to use the & operator on a variable name. This operator is known as "address-of", because that's what it does -- it provides the address of (in other words, a pointer to) the variable it is applied to. So, the code int *ip; int x; x = 42; ip = &x; will assign 42 to x, and will assign to ip a pointer to x.
int *ptr; // 1) this is declaration without initialisation. int *ptr = &a; // 2) this is declaration **and** initialisation (initialise ptr to the address of variable a) int b = 10; *ptr = b; // 3) this is assignment, assign what pointed by ptr to value of variable b. In 1) the * means that ptr is a pointer to int (but it has not pointed to any ...
7.0 Pointers. A pointer is a variable that holds the address to a variable. The address of a variable is where it is stored in the computer's memory. Pointers allow us to create dynamic data structures, handle variable parameters passed to functions, and they also give us an alternate way of working with arrays.
The size of the pointer is always the same. This is because a pointer is just a memory address, and the number of bits needed to access a memory address is constant. Dangling pointers. Much like a dangling reference, a dangling pointer is a pointer that is holding the address of an object that is no longer valid (e.g. because it has been ...
Description The software sets a pointer to a specific address other than NULL or 0. Using a fixed address is not portable, because that address will probably not be valid in all environments or platforms. Modes of Introduction: - Architecture and Design Related Weaknesses CWE-344 CWE-758 Consequences Integrity, Confidentiality, Availability: Execute Unauthorized […]
CWE (Common weakness enumeration) 587: Assignment of a Fixed Address to a Pointer. CWE (Common weakness enumeration) 587: Assignment of a Fixed Address to a Pointer. Documentation. Documentation. Log in; CVEdetails.com. ... The product sets a pointer to a specific address other than NULL or 0. Created: 2006-12-15 Updated: 2024-02-29 Source ...
Within the code block of a fixed statement, the assigned data will not move. In this example, bytes will remain at the same address, at least until the end of the fixed statement. The fixed statement requires the declaration of the pointer variable within its scope. This avoids accessing the variable outside the fixed statement, when the data is no longer fixed.
FixedAddr checks for the assignment of a fixed address other than NULL or 0 to a pointer. Using a fixed address is not portable, because that address will probably not be valid in all environments or platforms. See Also. C++ Audits Configuration dialog box; C++ Audits pane; Running C++ Audits; Source Code Audits and Metrics
If an AlignedBuffer were assigned to itself, the subsequent self-move could result in an incorrect reference count and later use-after-free. References. Bug 1883158 # CVE-2024-3862: Potential use of uninitialized memory in MarkStack assignment operator on self-assignment Reporter Ronald Crane Impact moderate Description
1. I would add that you can call the placement operator for new if you want an objects constructor called when assigning it at the specified address: int *pLoc = reinterpret_cast<int*>(0x604769); int *address = new (pLoc) int (1234); // init to a value. This is also used for memory caching objects. Create a buffer and then assign an object to it.
FixedAddr checks for the assignment of a fixed address other than NULL or 0 to a pointer. Using a fixed address is not portable, because that address will probably not be valid in all environments or platforms. See Also. C++ Audits Configuration dialog box; C++ Audits pane; Running C++ Audits; Source Code Audits and Metrics
Sorry. As stated by Mat, the proper syntax for a function pointer would be: void (*myFuncPtr)(void) = (void (*)(void)) FUNC; This is often simplified by using a typedef since the C function pointer syntax is somewhat convoluted. Also, you're must be really sure the function to be called is at that same exact address every time your injected DLL ...