no-unsafe-assignment
Disallow assigning a value with type any to variables and properties.
Extending "plugin:@typescript-eslint/ recommended-type-checked " in an ESLint configuration enables this rule.
This rule requires type information to run.
The any type in TypeScript is a dangerous "escape hatch" from the type system. Using any disables many type checking rules and is generally best used only as a last resort or when prototyping code.
Despite your best intentions, the any type can sometimes leak into your codebase. Assigning an any typed value to a variable can be hard to pick up on, particularly if it leaks in from an external library.
This rule disallows assigning any to a variable, and assigning any[] to an array destructuring.
This rule also compares generic type argument types to ensure you don't pass an unsafe any in a generic position to a receiver that's expecting a specific type. For example, it will error if you assign Set<any> to a variable declared as Set<string> .
Try this rule in the playground ↗
- ❌ Incorrect
There are cases where the rule allows assignment of any to unknown .
Example of any to unknown assignment that are allowed:
This rule is not configurable.
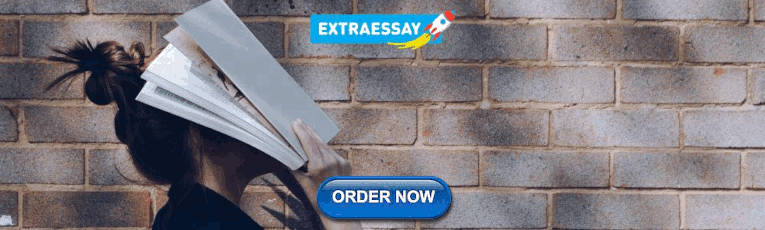
When Not To Use It
If your codebase has many existing any s or areas of unsafe code, it may be difficult to enable this rule. It may be easier to skip the no-unsafe-* rules pending increasing type safety in unsafe areas of your project. You might consider using ESLint disable comments for those specific situations instead of completely disabling this rule.
Related To
- no-explicit-any
- no-unsafe-argument
- no-unsafe-call
- no-unsafe-member-access
- no-unsafe-return
Resources
- Rule source
- Test source
- When Not To Use It
TypeScript ESLint: Unsafe assignment of an any value [Fix]
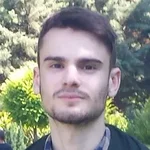
Last updated: Feb 29, 2024 Reading time · 5 min
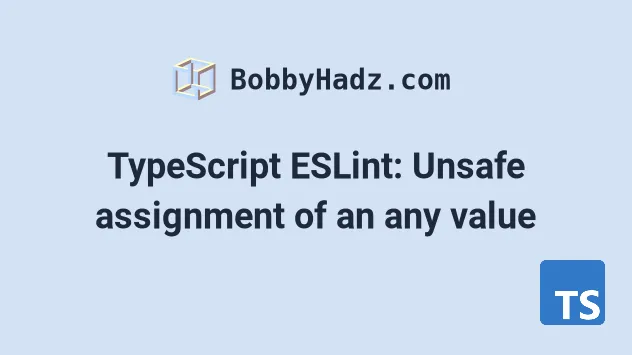
# TypeScript ESLint: Unsafe assignment of an any value
The error "@typescript-eslint/no-unsafe-assignment: Unsafe assignment of an any value." occurs when you assign a value with a type of any to a variable or a property.
To solve the error, set the variable to a specific type or disable the ESLint rule.
Here are some examples of when the ESLint error is raised.
All of the assignments above cause the error because the ESLint rule prevents you from assigning a value with an any type to a variable.
The any type effectively turns off type checking and should be used sparingly.
This article addresses 2 similar ESLint errors:
- @typescript-eslint/no-unsafe-assignment: Unsafe assignment of an any value.
- Unexpected any. Specify a different type. eslint@typescript-eslint/no-explicit-any
# Disabling the @typescript-eslint/no-unsafe-assignment ESLint rule
One way to get around the ESLint error is to disable the rule.
For example, the following comment disables the rule for 1 line.
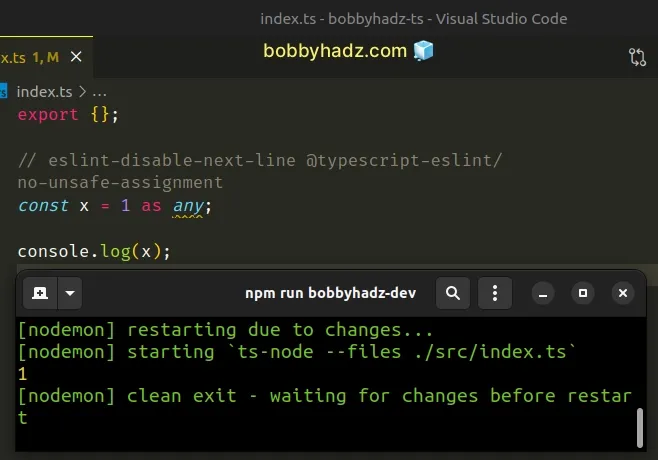
If you need to disable the @typescript-eslint/no-explicit-any rule for a single line, use the following comment.
If you need to disable multiple rules for a line, separate them by a comma.
If you need to disable the rule for the entire file, use the following comment.
If you need to disable the @typescript-eslint/no-explicit-any rule for the entire file, use the following comment instead.
You can disable both rules for the entire file by using the following comment.
If you want to disable the rules globally, add the following 2 rules to your .eslintrc.js file.
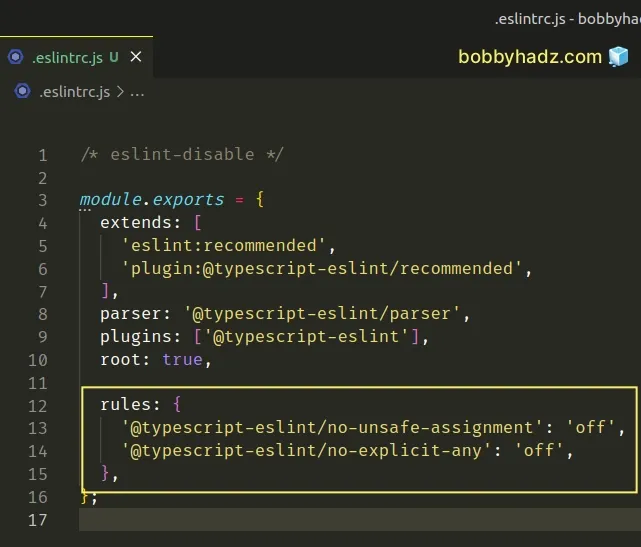
If you use a .eslintrc.json file, make sure to double-quote the keys and values.
# Setting the variable or property to unknown instead of any
Alternatively, you can set the variable or property to unknown to resolve the ESLint error.
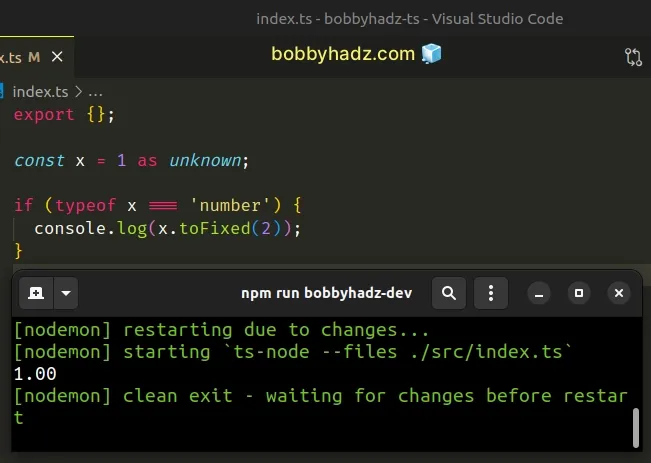
The unknown type is the type-safe counterpart of any .
When working with the unknown type, we basically tell TypeScript that we're going to get this value, but we don't know its type.
We are going to check with a couple of if statements to track the type down and use it safely.
I have written a detailed guide on how to check the type of a variable in TypeScript .
When using the unknown type, you have to use an if statement as a type guard to check the type of the variable before you are able to use any type-specific methods (e.g. string, array, object, etc).
# The error commonly occurs when parsing a JSON string
The error commonly occurs when parsing a JSON string with the JSON.parse() method.
The result variable stores a value of any type because TypeScript doesn't know the type of the value that is being parsed.
One way to resolve the issue is to use a type predicate .
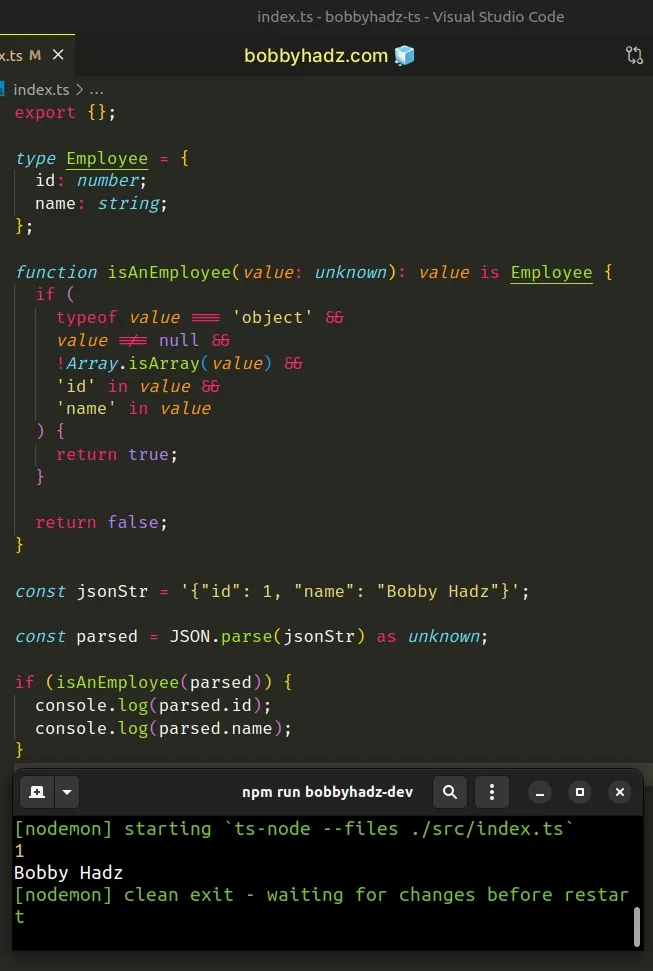
The value is Employee syntax is called a type predicate.
Our function basically checks if the passed-in value is compatible with an object of type Employee .
Notice that in the if block in which we called the isAnEmployee() function, the parsed variable is typed as Employee and we can access the id and name properties without getting TypeScript or ESLint errors.
I've written a detailed guide on how to check if a value is an object .
# Resolve the issue by typing the variable explicitly
You can also resolve the issue by typing the variable explicitly and removing the any type.
Here is an example of typing an object.
And here is an example of typing an array of objects.
You might have to use a type assertion, e.g. when parsing a JSON string.
In some rare cases, you might have to widen the type to unknown before using a type assertion to set a more specific type.
I've written detailed guides on:
- How to initialize a typed Empty Object in TypeScript
- Declare an Empty Array for a typed Variable in TypeScript
- How to add Elements to an Array in TypeScript
- Check if an Array contains a Value in TypeScript
- Check if a Value is an Array (of type) in TypeScript
- How to declare an Array of Objects in TypeScript
- How to declare a Two-dimensional Array in TypeScript
- Declare Array of Numbers, Strings or Booleans in TypeScript
- Create an Object based on an Interface in TypeScript
- Create a Type from an object's Keys or Values in TypeScript
- ESLint: Expected property shorthand object-shorthand [Fixed]
- 'X' should be listed in the project's dependencies, not devDependencies
- ESLint: Unexpected lexical declaration in case block [Fixed]
- ESLint couldn't find the config 'prettier' to extend from
- Import in body of module reorder to top eslint import/first
- ESLint: A form label must be associated with a control
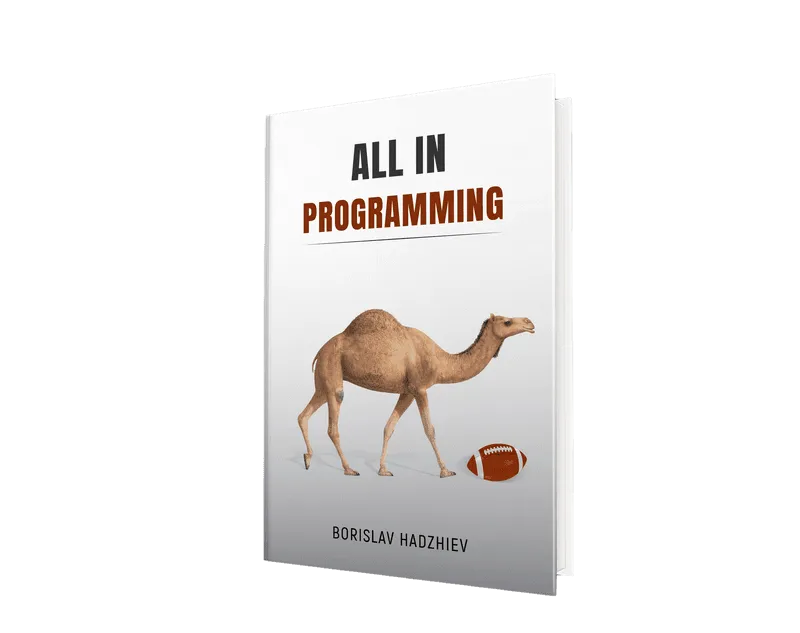
Borislav Hadzhiev
Web Developer
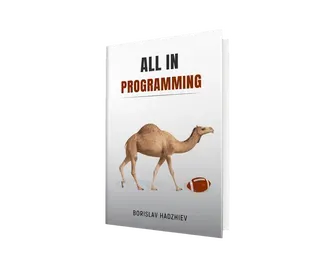
Copyright © 2024 Borislav Hadzhiev
- Datadog Site
- Serverless for AWS Lambda
- Autodiscovery
- Datadog Operator
- Assigning Tags
- Unified Service Tagging
- Service Catalog
- Session Replay
- Continuous Testing
- Browser Tests
- Private Locations
- Incident Management
- Database Monitoring
- Cloud Security Management
- Software Composition Analysis
- Workflow Automation
- Learning Center
- Standard Attributes
- Amazon Linux
- Rocky Linux
- From Source
- Architecture
- Supported Platforms
- Advanced Configurations
- Configuration Files
- Status Page
- Network Traffic
- Proxy Configuration
- FIPS Compliance
- Dual Shipping
- Secrets Management
- Remote Configuration
- Fleet Automation
- Upgrade to Agent v7
- Upgrade to Agent v6
- Upgrade Between Agent Minor Versions
- Container Hostname Detection
- Agent Flare
- Agent Check Status
- Permission Issues
- Integrations Issues
- Site Issues
- Autodiscovery Issues
- Windows Container Issues
- Agent Runtime Configuration
- High CPU or Memory Consumption
- Data Security
- Getting Started
- OTLP Metrics Types
- Configuration
- Integrations
- Resource Attribute Mapping
- Metrics Mapping
- Infrastructure Host Mapping
- Hostname Mapping
- Ingestion Sampling
- OTLP Ingestion by the Agent
- W3C Trace Context Propagation
- OpenTelemetry API Support
- Correlate RUM and Traces
- Correlate Logs and Traces
- Troubleshooting
- Visualizing OTLP Histograms as Heatmaps
- Migrate to OpenTelemetry Collector version 0.95.0+
- Producing Delta Temporality Metrics
- Sending Data from OpenTelemetry Demo
- OAuth2 in Datadog
- Authorization Endpoints
- Datagram Format
- Unix Domain Socket
- High Throughput Data
- Data Aggregation
- DogStatsD Mapper
- Writing a Custom Agent Check
- Writing a Custom OpenMetrics Check
- Create an Agent-based Integration
- Create an API Integration
- Create a Log Pipeline
- Integration Assets Reference
- Build a Marketplace Offering
- Create a Tile
- Create an Integration Dashboard
- Create a Recommended Monitor
- Create a Cloud SIEM Detection Rule
- OAuth for Integrations
- Install Agent Integration Developer Tool
- UI Extensions
- Submission - Agent Check
- Submission - DogStatsD
- Submission - API
- JetBrains IDEs
- Visual Studio
- Account Management
- Components: Common
- Components: Azure
- Components: AWS
- AWS Accounts
- Azure Accounts
- Dashboard List
- Interpolation
- Correlations
- Scheduled Reports
- Template Variables
- Configure Monitors
- Audit Trail
- Error Tracking
- Integration
- Live Process
- Network Performance
- Process Check
- Real User Monitoring
- Service Check
- Search Monitors
- Monitor Status
- Check Summary
- Monitor Settings
- Host and Container Maps
- Infrastructure List
- Container Images View
- Orchestrator Explorer
- Kubernetes Resource Utilization
- Increase Process Retention
- Cloud Resources Schema
- Metric Type Modifiers
- Historical Metrics Ingestion
- Submission - Powershell
- OTLP Metric Types
- Metrics Types
- Distributions
- Metrics Units
- Advanced Filtering
- Metrics Without Limits™
- Impact Analysis
- Faulty Deployment Detection
- Managing Incidents
- Natural Language Querying
- Investigating a Service
- Adding Entries to Service Catalog
- Adding Metadata
- Service Catalog API
- Service Scorecards
- Exploring APIs
- Assigning Owners
- Monitoring APIs
- Adding Entries to API Catalog
- Adding Metadata to APIs
- API Catalog API
- Endpoint Discovery from APM
- Custom Grouping
- Identify Suspect Commits
- Monitor-based SLOs
- Metric-based SLOs
- Time Slice SLOs
- Error Budget Alerts
- Burn Rate Alerts
- Incident Details
- Incident Settings
- Incident Analytics
- Datadog Clipboard
- Ingest Events
- Arithmetic Processor
- Date Remapper
- Category Processor
- Grok Parser
- Lookup Processor
- Service Remapper
- Status Remapper
- String Builder Processor
- Navigate the Explorer
- Customization
- Notifications
- Saved Views
- Triaging & Notifying
- Create a Case
- View and Manage
- Enterprise Configuration
- Build Workflows
- Authentication
- Trigger Workflows
- Workflow Logic
- Data Transformation
- HTTP Requests
- Save and Reuse Actions
- Connections
- Actions Catalog
- Embedded Apps
- Log collection
- Tag extraction
- Data Collected
- Installation
- Further Configuration
- Integrations & Autodiscovery
- Prometheus & OpenMetrics
- Control plane monitoring
- Data collected
- Data security
- Commands & Options
- Cluster Checks
- Endpoint Checks
- Admission Controller
- AWS Fargate
- Duplicate hosts
- Cluster Agent
- HPA and Metrics Provider
- Lambda Metrics
- Distributed Tracing
- Log Collection
- Advanced Configuration
- Continuous Profiler
- Securing Functions
- Deployment Tracking
- Libraries & Integrations
- Enhanced Metrics
- Linux - Code
- Linux - Container
- Windows - Code
- Azure Container Apps
- Google Cloud Run
- Overview Page
- Network Analytics
- Network Map
- DNS Monitoring
- SNMP Metrics
- NetFlow Monitoring
- Network Device Topology Map
- Google Cloud
- Custom Costs
- SaaS Cost Integrations
- Tag Pipelines
- Container Cost Allocation
- Cost Recommendations
- APM Terms and Concepts
- Automatic Instrumentation
- Custom Instrumentation
- Library Compatibility
- Library Configuration
- Configuration at Runtime
- Trace Context Propagation
- Serverless Application Tracing
- Proxy Tracing
- Span Tag Semantics
- Trace Metrics
- Runtime Metrics
- Ingestion Mechanisms
- Ingestion Controls
- Generate Metrics
- Trace Retention
- Usage Metrics
- Correlate DBM and Traces
- Correlate Synthetics and Traces
- Correlate Profiles and Traces
- Search Spans
- Query Syntax
- Span Facets
- Span Visualizations
- Trace Queries
- Request Flow Map
- Service Page
- Resource Page
- Service Map
- APM Monitors
- Expression Language
- Error Tracking Explorer
- Exception Replay
- Tracer Startup Logs
- Tracer Debug Logs
- Connection Errors
- Agent Rate Limits
- Agent APM metrics
- Agent Resource Usage
- Correlated Logs
- PHP 5 Deep Call Stacks
- .NET diagnostic tool
- APM Quantization
- Supported Language and Tracer Versions
- Profile Types
- Profile Visualizations
- Investigate Slow Traces or Endpoints
- Compare Profiles
- Setup Architectures
- Self-hosted
- Google Cloud SQL
- Autonomous Database
- Connecting DBM and Traces
- Exploring Database Hosts
- Exploring Query Metrics
- Exploring Query Samples
- Data Jobs Monitoring
- Monitoring Page Performance
- Monitoring Resource Performance
- Collecting Browser Errors
- Tracking User Actions
- Frustration Signals
- Crash Reporting
- Mobile Vitals
- Web View Tracking
- Integrated Libraries
- Sankey Diagrams
- Funnel Analysis
- Retention Analysis
- Generate Custom Metrics
- Connect RUM and Traces
- Search RUM Events
- Search Syntax
- Watchdog Insights for RUM
- Feature Flag Tracking
- Track Browser Errors
- Track Mobile Errors
- Multistep API Tests
- Recording Steps
- Test Results
- Advanced Options for Steps
- Authentication in Browser Tests
- Dimensioning
- Search Test Batches
- Search Test Runs
- Test Coverage
- Browser Test
- Test Summary
- APM Integration
- Testing Multiple Environments
- Testing With Proxy, Firewall, or VPN
- Azure DevOps Extension
- CircleCI Orb
- GitHub Actions
- Results Explorer
- AWS CodePipeline
- Custom Commands
- Custom Tags and Measures
- Custom Pipelines API
- Search and Manage
- Search Test Runs or Pipeline Executions
- CI Providers
- Java and JVM Languages
- JavaScript and TypeScript
- JUnit Report Uploads
- Tests in Containers
- Developer Workflows
- Code Coverage
- Instrument Browser Tests with RUM
- Instrument Swift Tests with RUM
- How It Works
- CircleCI Orbs
- Generic CI Providers
- Static Analysis Rules
- GitHub Pull Requests
- Deployment events
- Incident events
- Suppressions
- Security Inbox
- Threat Intelligence
- Account Takeover Protection
- Log Detection Rules
- Signal Correlation Rules
- Security Signals
- Investigator
- CSM Enterprise
- CSM Cloud Workload Security
- Detection Rules
- Investigate Security Signals
- Creating Custom Agent Rules
- CWS Events Formats
- Manage Compliance Rules
- Create Custom Rules
- Manage Compliance Posture
- Explore Misconfigurations
- Signals Explorer
- Identity Risks
- Vulnerabilities
- Mute Issues
- Automate Security Workflows
- Create Jira Issues
- Severity Scoring
- Terms and Concepts
- Using Single Step Instrumentation
- Using Datadog Tracing libraries
- Enabling ASM for Serverless
- Code Security
- User Monitoring and Protection
- Custom Detection Rules
- In-App WAF Rules
- Trace Qualification
- Threat Overview
- API Security Inventory
- Splunk HTTP Event Collector
- Splunk Forwarders (TCP)
- Sumo Logic Hosted Collector
- Datadog Agent
- Update Existing Pipelines
- Best Practices for Scaling Observability Pipelines
- React Native
- OpenTelemetry
- Other Integrations
- Pipeline Scanner
- Attributes and Aliasing
- Rehydrate from Archives
- PCI Compliance
- Connect Logs and Traces
- Search Logs
- Advanced Search
- Transactions
- Log Side Panel
- Watchdog Insights for Logs
- Track Browser and Mobile Errors
- Track Backend Errors
- Manage Data Collection
- Switching Between Orgs
- User Management
- Login Methods
- Custom Organization Landing Page
- Service Accounts
- IP Allowlist
- Granular Access
- Permissions
- User Group Mapping
- Active Directory
- API and Application Keys
- Team Management
- Multi-Factor Authentication
- Cost Details
- Usage Details
- Product Allotments
- Multi-org Accounts
- Log Management
- Synthetic Monitoring
- HIPAA Compliance
- Library Rules
- Investigate Sensitive Data Issues
- Regular Expression Syntax
Avoid assigning a value with type any
ID: typescript-best-practices/no-unsafe-assignment
Language: TypeScript
Severity: Warning
Category: Error Prone
Description
The any type in TypeScript is dangerously broad, leading to unexpected behavior. Using any should be avoided.
Non-Compliant Code Examples
Compliant code examples.
Seamless integrations. Try Datadog Code Analysis
Try this rule and analyze your code with Datadog Code Analysis
How to use this rule.
- Create a static-analysis.datadog.yml with the content above at the root of your repository
- Use our free IDE integrations or add Code Analysis scans to your CI pipelines
- Get feedback on your code
For more information, please read the Code Analysis documentation
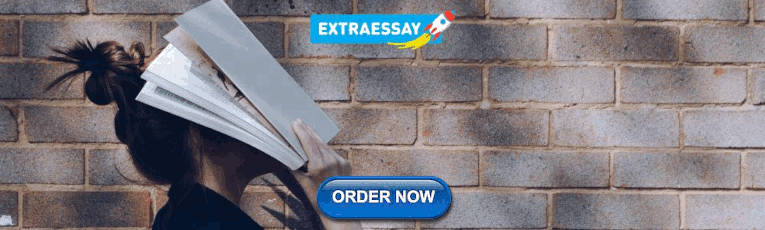
VS Code Extension
Identify code vulnerabilities directly in your VS Code editor
JetBrains Plugin
Identify code vulnerabilities directly in JetBrains products
Use Datadog Code Analysis to catch code issues at every step of your development process
Request a personalized demo
Get started with datadog.
no-unsafe-negation
Disallow negating the left operand of relational operators
Using the recommended config from @eslint/js in a configuration file enables this rule
Some problems reported by this rule are manually fixable by editor suggestions
Just as developers might type -a + b when they mean -(a + b) for the negative of a sum, they might type !key in object by mistake when they almost certainly mean !(key in object) to test that a key is not in an object. !obj instanceof Ctor is similar.
Rule Details
This rule disallows negating the left operand of the following relational operators:
- in operator .
- instanceof operator .
Examples of incorrect code for this rule:
Examples of correct code for this rule:
For rare situations when negating the left operand is intended, this rule allows an exception. If the whole negation is explicitly wrapped in parentheses, the rule will not report a problem.
This rule has an object option:
- "enforceForOrderingRelations": false (default) allows negation of the left-hand side of ordering relational operators ( < , > , <= , >= )
- "enforceForOrderingRelations": true disallows negation of the left-hand side of ordering relational operators
enforceForOrderingRelations
With this option set to true the rule is additionally enforced for:
- < operator.
- > operator.
- <= operator.
- >= operator.
The purpose is to avoid expressions such as ! a < b (which is equivalent to (a ? 0 : 1) < b ) when what is really intended is !(a < b) .
Examples of additional incorrect code for this rule with the { "enforceForOrderingRelations": true } option:
When Not To Use It
If you don’t want to notify unsafe logical negations, then it’s safe to disable this rule.
Handled by TypeScript
It is safe to disable this rule when using TypeScript because TypeScript's compiler enforces this check.
This rule was introduced in ESLint v3.3.0.
- Rule source
- Tests source
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[no-unsafe-assignment] option to ignore object destructuring or shorthand? #3867
JounQin commented Sep 11, 2021
The text was updated successfully, but these errors were encountered:
bradzacher commented Sep 11, 2021
Sorry, something went wrong.
JounQin commented Sep 12, 2021 • edited
Bradzacher commented sep 12, 2021.
- ❤️ 1 reaction
JounQin commented Sep 12, 2021
No branches or pull requests
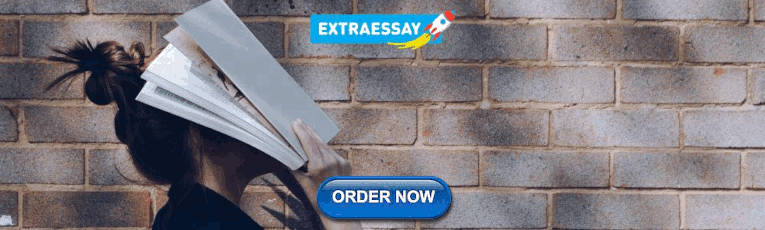
IMAGES
VIDEO
COMMENTS
no-unsafe-assignment. Disallow assigning a value with type any to variables and properties. Extending "plugin:@typescript-eslint/ recommended-type-checked " in an ESLint configuration enables this rule. This rule requires type information to run. The any type in TypeScript is a dangerous "escape hatch" from the type system.
TypeScript offers no real type safety anyway, just development-time type hints, and the TS inference is just so delightful! But yes, for a more complex solution, please declare your interfaces first, and make sure your server and client share/agree on them.
Our function basically checks if the passed-in value is compatible with an object of type Employee.. Notice that in the if block in which we called the isAnEmployee() function, the parsed variable is typed as Employee and we can access the id and name properties without getting TypeScript or ESLint errors.. I've written a detailed guide on how to check if a value is an object.
Thanks for contributing an answer to Stack Overflow! Please be sure to answer the question.Provide details and share your research! But avoid …. Asking for help, clarification, or responding to other answers.
\n. If your codebase has many existing anys or areas of unsafe code, it may be difficult to enable this rule.\nIt may be easier to skip the no-unsafe-* rules pending increasing type safety in unsafe areas of your project.\nYou might consider using ESLint disable comments for those specific situations instead of completely disabling this rule. \n
Thanks for the detailed reply! I think the "correct" solution to this problem is more strict options in TypeScript itself. It's a bit odd IMO that you need a separate tool to find lurking any when there's noImplicityAny.The rules that overlap with the compiler should be in the compiler.
In this regard, unknown and any are the same. The difference being that unknown ensures you don't do things that are unsafe. Not being able to convert past object is a known issue that they haven't solved just yet.
Datadog, the leading service for cloud-scale monitoring.
(feat(eslint-plugin): add rule no-unsafe-assignment #1694) Considering also handling x += 1, etc. Probably should. Using any in arithmetic expression. At this point, this should be implicitly handled by any of the previous rules. const x = 1 * any caught by no-unsafe-assignment; return 1 * any caught by no-unsafe-return
TypeScript is a language for application-scale JavaScript development. TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. Members Online
//error: Unsafe assignment of an `any` value. const foo = /** @type {string} */ (require ('foo')) Additional Info according to the docs , this syntax is equivalent to a cast, not a type annotation. therefore no-unsafe-assignment should allow it, to be consistent with how it works with .ts files
If you don't want to notify unsafe logical negations, then it's safe to disable this rule. Handled by TypeScript. It is safe to disable this rule when using TypeScript because TypeScript's compiler enforces this check. Version. This rule was introduced in ESLint v3.3.0. Resources. Rule source; Tests source
I have tried restarting my IDE and the issue persists. I have updated to the latest version of the packages. I have read the FAQ and my problem is not listed. Repro { "rules": { "@typescript-eslint...
The fact that LinksFunction is being only used as a type in the code you are showing means that the TypeScript compiler could still succeed in compiling your modules under certain circumstances, despite the missing type definitions.