- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Exercises - Basic to Advanced Java Practice Set with Solutions
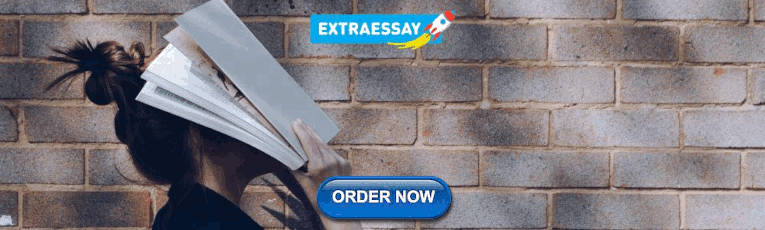
1. Write Hello World Program in Java
2. write a program in java to add two numbers., 3. write a program to swap two numbers, 4. write a java program to convert integer numbers and binary numbers..
- 5. Write a Program to Find Factorial of a Number in Java
- 6. Write a Java Program to Add two Complex Numbers
7. Write a Program to Calculate Simple Interest in Java
- 8. Write a Program to Print the Pascal’s Triangle in Java
9. Write a Program to Find Sum of Fibonacci Series Number
10. write a program to print pyramid number pattern in java., 11. write a java program to print pattern., 12. write a java program to print pattern., 13. java program to print patterns., 14. write a java program to compute the sum of array elements., 15. write a java program to find the largest element in array, 16. write java program to find the tranpose of matrix, 17. java array program for array rotation, 18. java array program to remove duplicate elements from an array, 19. java array program to remove all occurrences of an element in an array, 20. java program to check whether a string is a palindrome, 21. java string program to check anagram, 22. java string program to reverse a string, 23. java string program to remove leading zeros, 24. write a java program for linear search., 25. write a binary search program in java., 26. java program for bubble sort..
- 27. Write a Program for Insertion Sort in Java
28. Java Program for Selection Sort.
29. java program for merge sort., 30. java program for quicksort., java exercises – basic to advanced java practice programs with solutions.
Java is one of the most popular languages because of its robust and secure nature. But, programmers often find it difficult to find a platform for Java Practice Online. In this article, we have provided Java Practice Programs. That covers various Java Core Topics that can help users with Java Practice.
Take a look at our Java Exercises to practice and develop your Java programming skills. Our Java programming exercises Practice Questions from all the major topics like loops, object-oriented programming, exception handling, and many more.
Table of Content
Pattern Programs in Java
Array programs in java, string programs in java, java practice problems for searching algorithms, practice problems in java sorting algorithms.
- Practice More Java Problems
-768.webp)
Java Practice Programs
The solution to the Problem is mentioned below:
Click Here for the Solution
5. write a program to find factorial of a number in java., 6. write a java program to add two complex numbers., 8. write a program to print the pascal’s triangle in java.
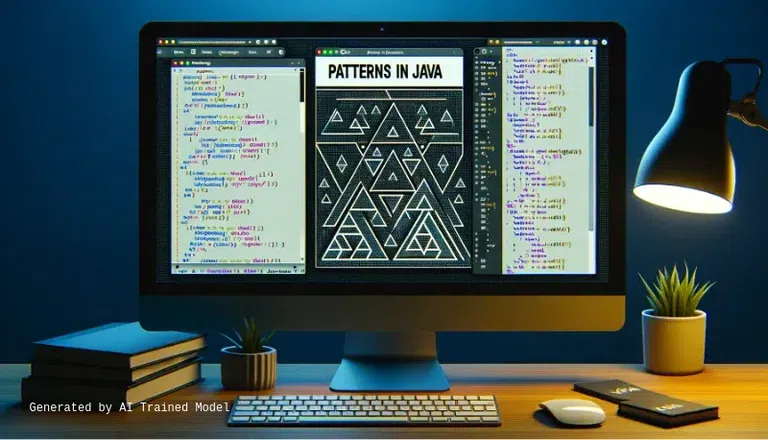
Time Complexity: O(N) Space Complexity: O(N)
Time Complexity: O(logN) Space Complexity: O(N)
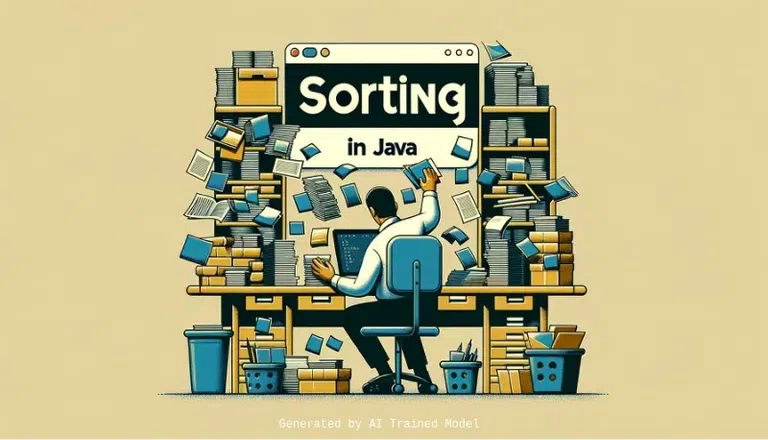
Time Complexity: O(N 2 ) Space Complexity: O(1)
27. Write a Program for Insertion Sort in Java.
Time Complexity: O(N logN) Space Complexity: O(N)
Time Complexity: O(N logN) Space Complexity: O(1)
After completing these Java exercises you are a step closer to becoming an advanced Java programmer. We hope these exercises have helped you understand Java better and you can solve beginner to advanced-level questions on Java programming.
Solving these Java programming exercise questions will not only help you master theory concepts but also grasp their practical applications, which is very useful in job interviews.
More Java Practice Exercises
Java Array Exercise Java String Exercise Java Collection Exercise To Practice Java Online please check our Practice Portal. <- Click Here
FAQ in Java Exercise
1. how to do java projects for beginners.
To do Java projects you need to know the fundamentals of Java programming. Then you need to select the desired Java project you want to work on. Plan and execute the code to finish the project. Some beginner-level Java projects include: Reversing a String Number Guessing Game Creating a Calculator Simple Banking Application Basic Android Application
2. Is Java easy for beginners?
As a programming language, Java is considered moderately easy to learn. It is unique from other languages due to its lengthy syntax. As a beginner, you can learn beginner to advanced Java in 6 to 18 months.
3. Why Java is used?
Java provides many advantages and uses, some of which are: Platform-independent Robust and secure Object-oriented Popular & in-demand Vast ecosystem
Please Login to comment...
Similar reads.
- Java-Arrays
- java-basics
- Java-Data Types
- Java-Functions
- Java-Library
- Java-Object Oriented
- Java-Output
- Java-Strings
- Output of Java Program
- How to Use ChatGPT with Bing for Free?
- 7 Best Movavi Video Editor Alternatives in 2024
- How to Edit Comment on Instagram
- 10 Best AI Grammar Checkers and Rewording Tools
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Java Coding Practice
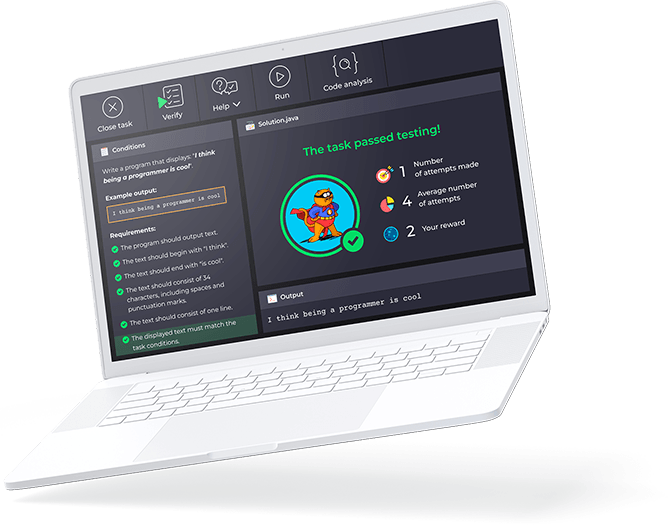
What kind of Java practice exercises are there?
How to solve these java coding challenges, why codegym is the best platform for your java code practice.
- Tons of versatile Java coding tasks for learners with any background: from Java Syntax and Core Java topics to Multithreading and Java Collections
- The support from the CodeGym team and the global community of learners (“Help” section, programming forum, and chat)
- The modern tool for coding practice: with an automatic check of your solutions, hints on resolving the tasks, and advice on how to improve your coding style
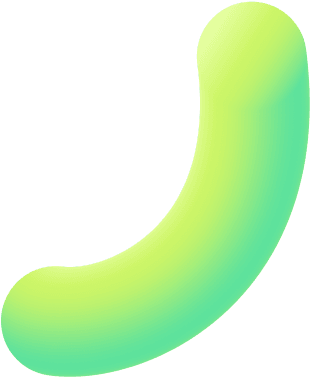
Click on any topic to practice Java online right away
Practice java code online with codegym.
In Java programming, commands are essential instructions that tell the computer what to do. These commands are written in a specific way so the computer can understand and execute them. Every program in Java is a set of commands. At the beginning of your Java programming practice , it’s good to know a few basic principles:
- In Java, each command ends with a semicolon;
- A command can't exist on its own: it’s a part of a method, and method is part of a class;
- Method (procedure, function) is a sequence of commands. Methods define the behavior of an object.
Here is an example of the command:
The command System.out.println("Hello, World!"); tells the computer to display the text inside the quotation marks.
If you want to display a number and not text, then you do not need to put quotation marks. You can simply write the number. Or an arithmetic operation. For example:
Command to display the number 1.
A command in which two numbers are summed and their sum (10) is displayed.
As we discussed in the basic rules, a command cannot exist on its own in Java. It must be within a method, and a method must be within a class. Here is the simplest program that prints the string "Hello, World!".
We have a class called HelloWorld , a method called main() , and the command System.out.println("Hello, World!") . You may not understand everything in the code yet, but that's okay! You'll learn more about it later. The good news is that you can already write your first program with the knowledge you've gained.
Attention! You can add comments in your code. Comments in Java are lines of code that are ignored by the compiler, but you can mark with them your code to make it clear for you and other programmers.
Single-line comments start with two forward slashes (//) and end at the end of the line. In example above we have a comment //here we print the text out
You can read the theory on this topic here , here , and here . But try practicing first!
Explore the Java coding exercises for practicing with commands below. First, read the conditions, scroll down to the Solution box, and type your solution. Then, click Verify (above the Conditions box) to check the correctness of your program.
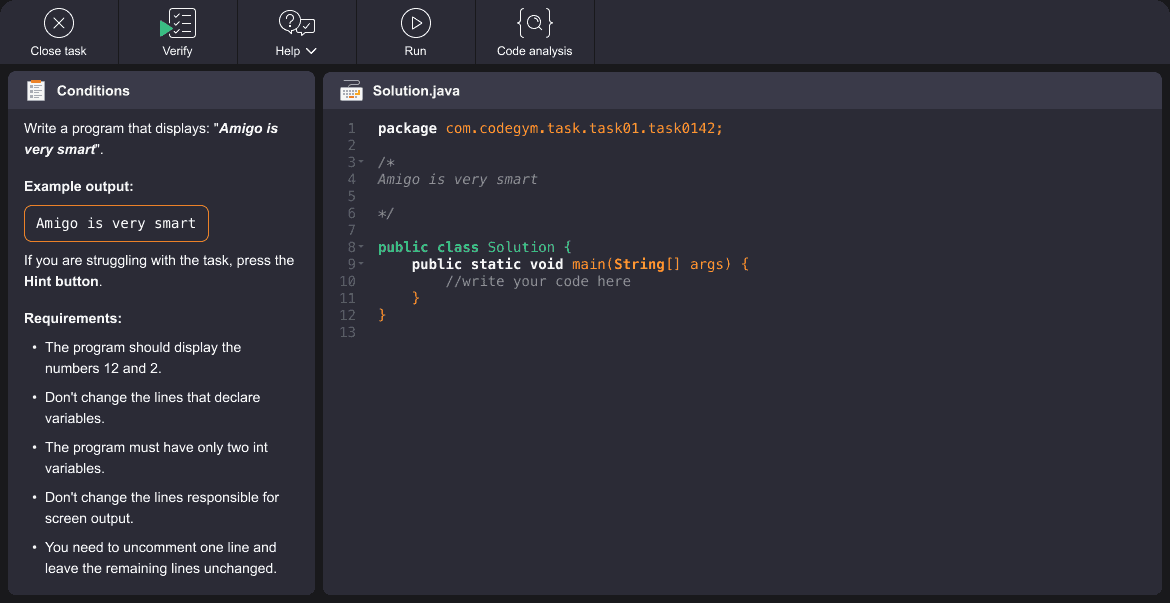
The two main types in Java are String and int. We store strings/text in String, and integers (whole numbers) in int. We have already used strings and integers in previous examples without explicit declaration, by specifying them directly in the System.out.println() operator.
In the first case “I am a string” is a String in the second case 5 is an integer of type int. However, most often, in order to manipulate data, variables must be declared before being used in the program. To do this, you need to specify the type of the variable and its name. You can also set a variable to a specific value, or you can do this later. Example:
Here we declared a variable called a but didn't give it any value, declared a variable b and gave it the value 5 , declared a string called s and gave it the value Hello, World!
Attention! In Java, the = sign is not an equals sign, but an assignment operator. That is, the variable (you can imagine it as an empty box) is assigned the value that is on the right (you can imagine that this value was put in the empty box).
We created an integer variable named a with the first command and assigned it the value 5 with the second command.
Before moving on to practice, let's look at an example program where we will declare variables and assign values to them:
In the program, we first declared an int variable named a but did not immediately assign it a value. Then we declared an int variable named b and "put" the value 5 in it. Then we declared a string named s and assigned it the value "Hello, World!" After that, we assigned the value 2 to the variable a that we declared earlier, and then we printed the variable a, the sum of the variables a and b, and the variable s to the screen
This program will display the following:
We already know how to print to the console, but how do we read from it? For this, we use the Scanner class. To use Scanner, we first need to create an instance of the class. We can do this with the following code:
Once we have created an instance of Scanner, we can use the next() method to read input from the console or nextInt() if we should read an integer.
The following code reads a number from the console and prints it to the console:
Here we first import a library scanner, then ask a user to enter a number. Later we created a scanner to read the user's input and print the input out.
This code will print the following output in case of user’s input is 5:
More information about the topic you could read here , here , and here .
See the exercises on Types and keyboard input to practice Java coding:
Conditions and If statements in Java allow your program to make decisions. For example, you can use them to check if a user has entered a valid password, or to determine whether a number is even or odd. For this purpose, there’s an 'if/else statement' in Java.
The syntax for an if statement is as follows:
Here could be one or more conditions in if and zero or one condition in else.
Here's a simple example:
In this example, we check if the variable "age" is greater than or equal to 18. If it is, we print "You are an adult." If not, we print "You are a minor."
Here are some Java practice exercises to understand Conditions and If statements:
In Java, a "boolean" is a data type that can have one of two values: true or false. Here's a simple example:
The output of this program is here:
In addition to representing true or false values, booleans in Java can be combined using logical operators. Here, we introduce the logical AND (&&) and logical OR (||) operators.
- && (AND) returns true if both operands are true. In our example, isBothFunAndEasy is true because Java is fun (isJavaFun is true) and coding is not easy (isCodingEasy is false).
- || (OR) returns true if at least one operand is true. In our example, isEitherFunOrEasy is true because Java is fun (isJavaFun is true), even though coding is not easy (isCodingEasy is false).
- The NOT operator (!) is unary, meaning it operates on a single boolean value. It negates the value, so !isCodingEasy is true because it reverses the false value of isCodingEasy.
So the output of this program is:
More information about the topic you could read here , and here .
Here are some Java exercises to practice booleans:
With loops, you can execute any command or a block of commands multiple times. The construction of the while loop is:
Loops are essential in programming to execute a block of code repeatedly. Java provides two commonly used loops: while and for.
1. while Loop: The while loop continues executing a block of code as long as a specified condition is true. Firstly, the condition is checked. While it’s true, the body of the loop (commands) is executed. If the condition is always true, the loop will repeat infinitely, and if the condition is false, the commands in a loop will never be executed.
In this example, the code inside the while loop will run repeatedly as long as count is less than or equal to 5.
2. for Loop: The for loop is used for iterating a specific number of times.
In this for loop, we initialize i to 1, specify the condition i <= 5, and increment i by 1 in each iteration. It will print "Count: 1" to "Count: 5."
Here are some Java coding challenges to practice the loops:
An array in Java is a data structure that allows you to store multiple values of the same type under a single variable name. It acts as a container for elements that can be accessed using an index.
What you should know about arrays in Java:
- Indexing: Elements in an array are indexed, starting from 0. You can access elements by specifying their index in square brackets after the array name, like myArray[0] to access the first element.
- Initialization: To use an array, you must declare and initialize it. You specify the array's type and its length. For example, to create an integer array that can hold five values: int[] myArray = new int[5];
- Populating: After initialization, you can populate the array by assigning values to its elements. All elements should be of the same data type. For instance, myArray[0] = 10; myArray[1] = 20;.
- Default Values: Arrays are initialized with default values. For objects, this is null, and for primitive types (int, double, boolean, etc.), it's typically 0, 0.0, or false.
In this example, we create an integer array, assign values to its elements, and access an element using indexing.
In Java, methods are like mini-programs within your main program. They are used to perform specific tasks, making your code more organized and manageable. Methods take a set of instructions and encapsulate them under a single name for easy reuse. Here's how you declare a method:
- public is an access modifier that defines who can use the method. In this case, public means the method can be accessed from anywhere in your program.Read more about modifiers here .
- static means the method belongs to the class itself, rather than an instance of the class. It's used for the main method, allowing it to run without creating an object.
- void indicates that the method doesn't return any value. If it did, you would replace void with the data type of the returned value.
In this example, we have a main method (the entry point of the program) and a customMethod that we've defined. The main method calls customMethod, which prints a message. This illustrates how methods help organize and reuse code in Java, making it more efficient and readable.
In this example, we have a main method that calls the add method with two numbers (5 and 3). The add method calculates the sum and returns it. The result is then printed in the main method.
All composite types in Java consist of simpler ones, up until we end up with primitive types. An example of a primitive type is int, while String is a composite type that stores its data as a table of characters (primitive type char). Here are some examples of primitive types in Java:
- int: Used for storing whole numbers (integers). Example: int age = 25;
- double: Used for storing numbers with a decimal point. Example: double price = 19.99;
- char: Used for storing single characters. Example: char grade = 'A';
- boolean: Used for storing true or false values. Example: boolean isJavaFun = true;
- String: Used for storing text (a sequence of characters). Example: String greeting = "Hello, World!";
Simple types are grouped into composite types, that are called classes. Example:
We declared a composite type Person and stored the data in a String (name) and int variable for an age of a person. Since composite types include many primitive types, they take up more memory than variables of the primitive types.
See the exercises for a coding practice in Java data types:
String is the most popular class in Java programs. Its objects are stored in a memory in a special way. The structure of this class is rather simple: there’s a character array (char array) inside, that stores all the characters of the string.
String class also has many helper classes to simplify working with strings in Java, and a lot of methods. Here’s what you can do while working with strings: compare them, search for substrings, and create new substrings.
Example of comparing strings using the equals() method.
Also you can check if a string contains a substring using the contains() method.
You can create a new substring from an existing string using the substring() method.
More information about the topic you could read here , here , here , here , and here .
Here are some Java programming exercises to practice the strings:
In Java, objects are instances of classes that you can create to represent and work with real-world entities or concepts. Here's how you can create objects:
First, you need to define a class that describes the properties and behaviors of your object. You can then create an object of that class using the new keyword like this:
It invokes the constructor of a class.If the constructor takes arguments, you can pass them within the parentheses. For example, to create an object of class Person with the name "Jane" and age 25, you would write:
Suppose you want to create a simple Person class with a name property and a sayHello method. Here's how you do it:
In this example, we defined a Person class with a name property and a sayHello method. We then created two Person objects (person1 and person2) and used them to represent individuals with different names.
Here are some coding challenges in Java object creation:
Static classes and methods in Java are used to create members that belong to the class itself, rather than to instances of the class. They can be accessed without creating an object of the class.
Static methods and classes are useful when you want to define utility methods or encapsulate related classes within a larger class without requiring an instance of the outer class. They are often used in various Java libraries and frameworks for organizing and providing utility functions.
You declare them with the static modifier.
Static Methods
A static method is a method that belongs to the class rather than any specific instance. You can call a static method using the class name, without creating an object of that class.
In this example, the add method is static. You can directly call it using Calculator.add(5, 3)
Static Classes
In Java, you can also have static nested classes, which are classes defined within another class and marked as static. These static nested classes can be accessed using the outer class's name.
In this example, Student is a static nested class within the School class. You can access it using School.Student.
More information about the topic you could read here , here , here , and here .
See below the exercises on Static classes and methods in our Java coding practice for beginners:
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Questions and Exercises: Generics
- Write a generic method to count the number of elements in a collection that have a specific property (for example, odd integers, prime numbers, palindromes).
- Will the following class compile? If not, why? public final class Algorithm { public static <T> T max(T x, T y) { return x > y ? x : y; } }
- Write a generic method to exchange the positions of two different elements in an array.
- If the compiler erases all type parameters at compile time, why should you use generics?
- What is the following class converted to after type erasure? public class Pair<K, V> { public Pair(K key, V value) { this.key = key; this.value = value; } public K getKey() { return key; } public V getValue() { return value; } public void setKey(K key) { this.key = key; } public void setValue(V value) { this.value = value; } private K key; private V value; }
- What is the following method converted to after type erasure? public static <T extends Comparable<T>> int findFirstGreaterThan(T[] at, T elem) { // ... }
- Will the following method compile? If not, why? public static void print(List<? extends Number> list) { for (Number n : list) System.out.print(n + " "); System.out.println(); }
- Write a generic method to find the maximal element in the range [begin, end) of a list.
- Will the following class compile? If not, why? public class Singleton<T> { public static T getInstance() { if (instance == null) instance = new Singleton<T>(); return instance; } private static T instance = null; }
- Given the following classes: class Shape { /* ... */ } class Circle extends Shape { /* ... */ } class Rectangle extends Shape { /* ... */ } class Node<T> { /* ... */ } Will the following code compile? If not, why? Node<Circle> nc = new Node<>(); Node<Shape> ns = nc;
- Consider this class: class Node<T> implements Comparable<T> { public int compareTo(T obj) { /* ... */ } // ... } Will the following code compile? If not, why? Node<String> node = new Node<>(); Comparable<String> comp = node;
- How do you invoke the following method to find the first integer in a list that is relatively prime to a list of specified integers? public static <T> int findFirst(List<T> list, int begin, int end, UnaryPredicate<T> p) Note that two integers a and b are relatively prime if gcd( a, b ) = 1, where gcd is short for greatest common divisor.
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Welcome to Java! Easy Max Score: 3 Success Rate: 97.05%
Java stdin and stdout i easy java (basic) max score: 5 success rate: 96.88%, java if-else easy java (basic) max score: 10 success rate: 91.33%, java stdin and stdout ii easy java (basic) max score: 10 success rate: 92.62%, java output formatting easy java (basic) max score: 10 success rate: 96.55%, java loops i easy java (basic) max score: 10 success rate: 97.67%, java loops ii easy java (basic) max score: 10 success rate: 97.32%, java datatypes easy java (basic) max score: 10 success rate: 93.66%, java end-of-file easy java (basic) max score: 10 success rate: 97.91%, java static initializer block easy java (basic) max score: 10 success rate: 96.14%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
Browse Course Material
Course info, instructors.
- Adam Marcus
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
Introduction to programming in java, assignments.

You are leaving MIT OpenCourseWare

Java Programs With Examples (Basic to Advanced)
Want to practice Java programming with examples ? Tutorials Freak is your destination for Java codes, whether you are a beginner or an experienced professional. Here, you will find all types of Java programs with examples.
Fine-tune your skills with our comprehensive collection of Java example programs. Each program comes complete with code samples, outputs, and easy-to-understand explanations, making your learning experience both enjoyable and effective.
At Tutorials Freak, we believe in learning by doing. That's why our Java programs are designed not just to showcase theoretical concepts, but to give you hands-on practice.
What sets us apart is our commitment to simplicity. Our explanations are presented in plain, easy-to-understand language, ensuring that complex Java concepts become accessible to all. Say goodbye to confusing jargon and welcome a clear path to Java mastery.
So, what are you waiting for? Start practicing Java programs with examples, and let your coding journey begin! Happy coding!
Hello World Program in Java (Print Hello World in Java)
Java program to add two numbers (java sum / addition), find greatest of three numbers in java (largest number program), prime number program in java (code to check prime or not), java program for fibonacci series (using for, while, recursion, scanner), factorial program in java (find factorial of a number in java), how to find sum of digits of a number in java, how to reverse a number in java program & examples, how to swap two numbers in java programs with/without third variable, even odd program in java (program to check number is even or odd), vowel and consonant program in java, java program for quadratic equation (find roots with 3 ways), find frequency of characters in a string in java (4 ways), remove space from string in java (remove whitespace), string null check in java (string is empty or null) - 5 ways, how to print string in java 6 methods, how to get ascii value of char in java find ascii value, how to convert char to string in java character to string, how to convert char to int in java character to integer conversion, how to convert int to char in java integer to character, how to convert long to int in java long to integer, how to convert int to long in java integer to long program, how to convert boolean to string in java (parse bool to string), how to convert string to boolean in java 3 ways with program, how to convert string to int in java string to integer program, how to convert int to string in java integer to string program, how to convert int to double in java (integer to double), how to convert double to int in java 4 ways, how to convert string to double in java programs, how to convert double to string in java programs, leap year program in java (check leap year or not), check number is positive or negative in java (4 ways), java program to check character is alphabet or not, armstrong number program in java (for loop, recursion), print prime numbers between 1 to n in java (1 to 100), java program for palindrome number (palindrome code), sum of n natural numbers in java (programs & explanation), java multiplication table program (loops, 2d array) 5 ways, find gcd of two numbers in java (hcf program), gcd of three numbers in java (hcf of 3 numbers program, gcd of array in java (gcd of n numbers program), lcm of two numbers in java (lcm program and code), lcm of three numbers in java (easy programs), lcm of n numbers in java (lcm of array of numbers), how to print a to z in java 3 ways to print alphabets, java program to count number of digits in number (integer length), java program to reverse a string (4 methods), how to reverse an array in java array reverse program, how to find power of a number in java 4 programs, how to find factors of a number in java factors program, check disarium number in java (3 easy programs), find keith number in java (easy programs), happy number in java (easy programs with logic), find sunny number in java (easy programs), simple calculator program in java (code, switch, methods, swing), java scientific calculator program (source code, swing, switch), java age calculator program (from date/year of birth), java matrix addition program (add two matrices): 3 ways, java matrix multiplication program (multiply two matrices), java matrix transpose program (3 ways to transpose matrix), top 15 alphabet pattern programs in java (2023), top 14 pyramid pattern programs in java (print pyramids).
- [email protected]
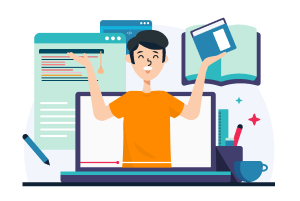
What’s New ?
The Top 10 favtutor Features You Might Have Overlooked

- Don’t have an account Yet? Sign Up
Remember me Forgot your password?
- Already have an Account? Sign In
Lost your password? Please enter your email address. You will receive a link to create a new password.
Back to log-in
By Signing up for Favtutor, you agree to our Terms of Service & Privacy Policy.
Top 25 Java Projects for Beginners to Practice in 2024
- Jan 01, 2024
- 13 Minutes Read
- Why Trust Us We uphold a strict editorial policy that emphasizes factual accuracy, relevance, and impartiality. Our content is crafted by top technical writers with deep knowledge in the fields of computer science and data science, ensuring each piece is meticulously reviewed by a team of seasoned editors to guarantee compliance with the highest standards in educational content creation and publishing.
- By Arkadyuti Bandyopadhyay
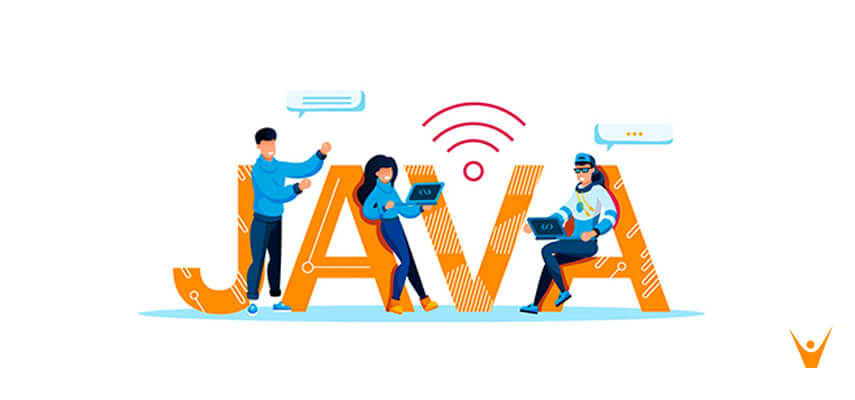
Java is one of the best languages one can learn when stepping into the world of programming. The main reason is that Java is an OOP (Object Oriented Programming) language, making it closer to the real world.
Despite being started in 1992, Java is still being actively used for the development of software across the globe. According to Glassdoor , the national average salary for a Java Developer is around $87,000 in the United States.
Looking at the above statistics you must have understood that becoming a Java developer is not all you have on your plate, applying it to develop and deploy some simple and fun projects is more important to give your resume a push in the software industry.
Before jumping into to practice some amazing Java Projects for Beginners in 2024 , let’s understand briefly why Java is so popular.
Why do Companies prefer Java for building Software?
About 30.55% of professional developers around the world make use of Java programming as their core technology. It is in much demand in real-world applications because of the following reasons:
- Unlike other programming languages, Java uses both a compiler and an interpreter for the conversion of a high-level language into machine code.
- Being an object-oriented programming language, Java is much closer to the real world than most other languages, with concepts like Abstraction, encapsulation, inheritance, and polymorphism.
- Java quickly became a favorite of the software industry because it made the development process more efficient and solved the problem of software distribution across platforms.
- Java’s memory management is quite robust by nature. Java handles garbage collection automatically without the user needing to worry about it.
- Java's usefulness has grown over the years as programmers develop new ways to use it on servers, bringing performance and scalability to a whole new level.
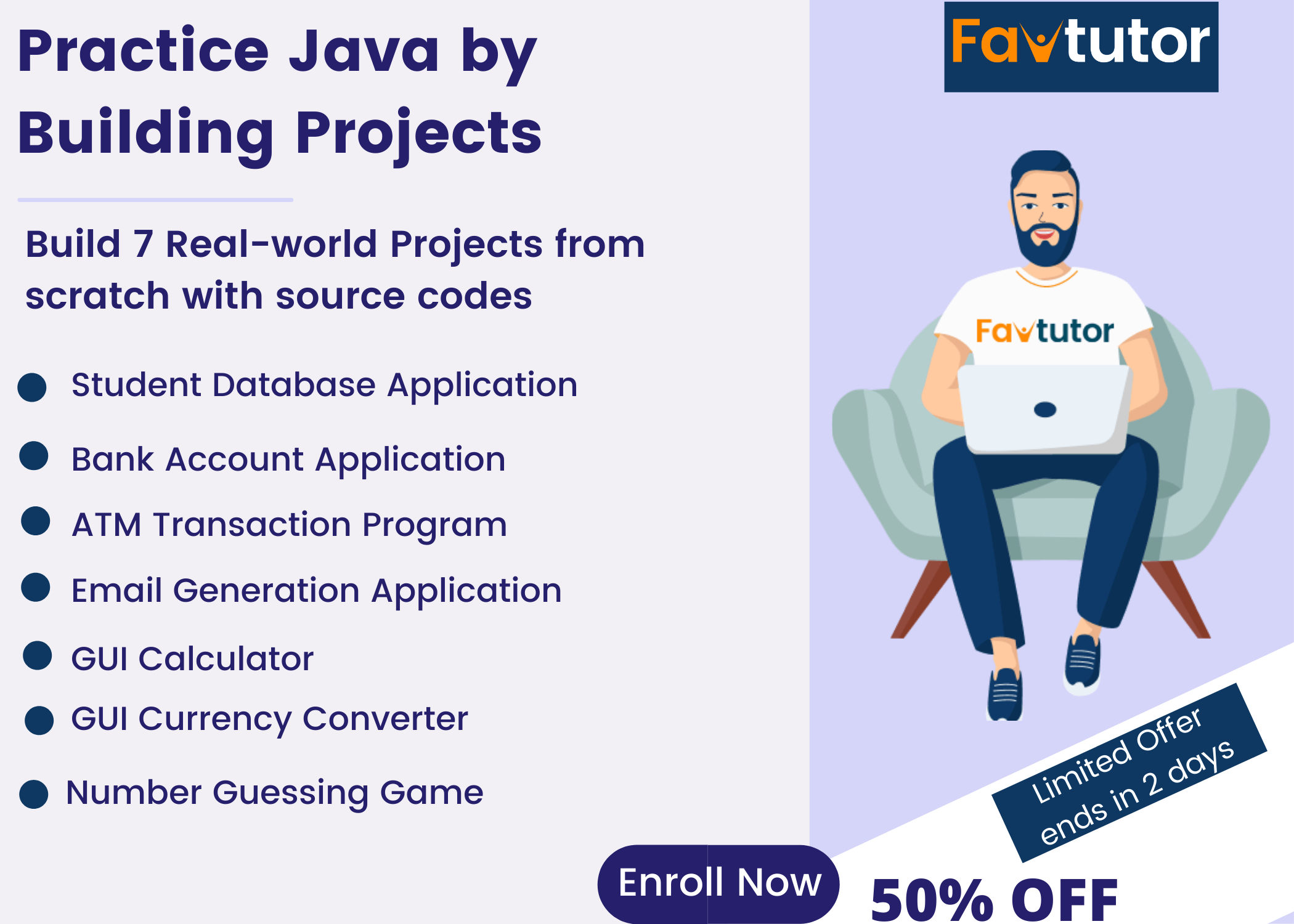
It is often a programmer’s dilemma as to what they can build with the concepts they have learned via some tutorial on the Internet. Good project ideas, especially ones that help boost your portfolio and improve your understanding of the underlying concepts are hard to come by.
Our list suggests some fun Java project ideas for beginners with fresh topics programmers that should get you started.
Why Java Practice Projects are Important?
Java continues to be a programming language that is in much demand. But sadly, just mentioning “I know Java” on your resume isn’t going to be enough, is it? Showing recruiters that you can work your way through Java with some real-world projects is going to look better.
Projects are a way to show that one understands the underlying concepts of the programming language and is ready to apply them to solve real-world problems. Project-based learning helps teach many concepts that the main theory can never teach.
Implementing a few Java practice projects not only demonstrates how Java works behind the scenes but also helps boost the portfolio. These projects don’t have to be very complex; they should be something basic, which puts some of Java’s many features into play.
Best 25 Java Projects for Beginners in 2024
Let's first look at all Java Project Ideas in the list below:
Bank Management Software
Temperature Converter
Electricity Billing System
Supermarket Billing Software
Memory Game
Link Shortener
Chatting Application
Digital Clock
Quizzing app
Email-Client Software
Student Management System
Airline Reservation System
Food Ordering System
Text-based RPG (role-playing game)
Media Player Application
Currency Converter
Brick Breaker Game
Number Guessing Game
Tic-Tac-Toe Game
Word Counter
- Online Quiz Platform
- Health and Fitness Tracker
- Appointment Scheduler
- Online Auction System
- Language Learning App
As a beginner, it might be difficult to come up with ideas for projects, that's why we have decided to curate a list of amazing Java projects for beginners.
1) Bank Management Software
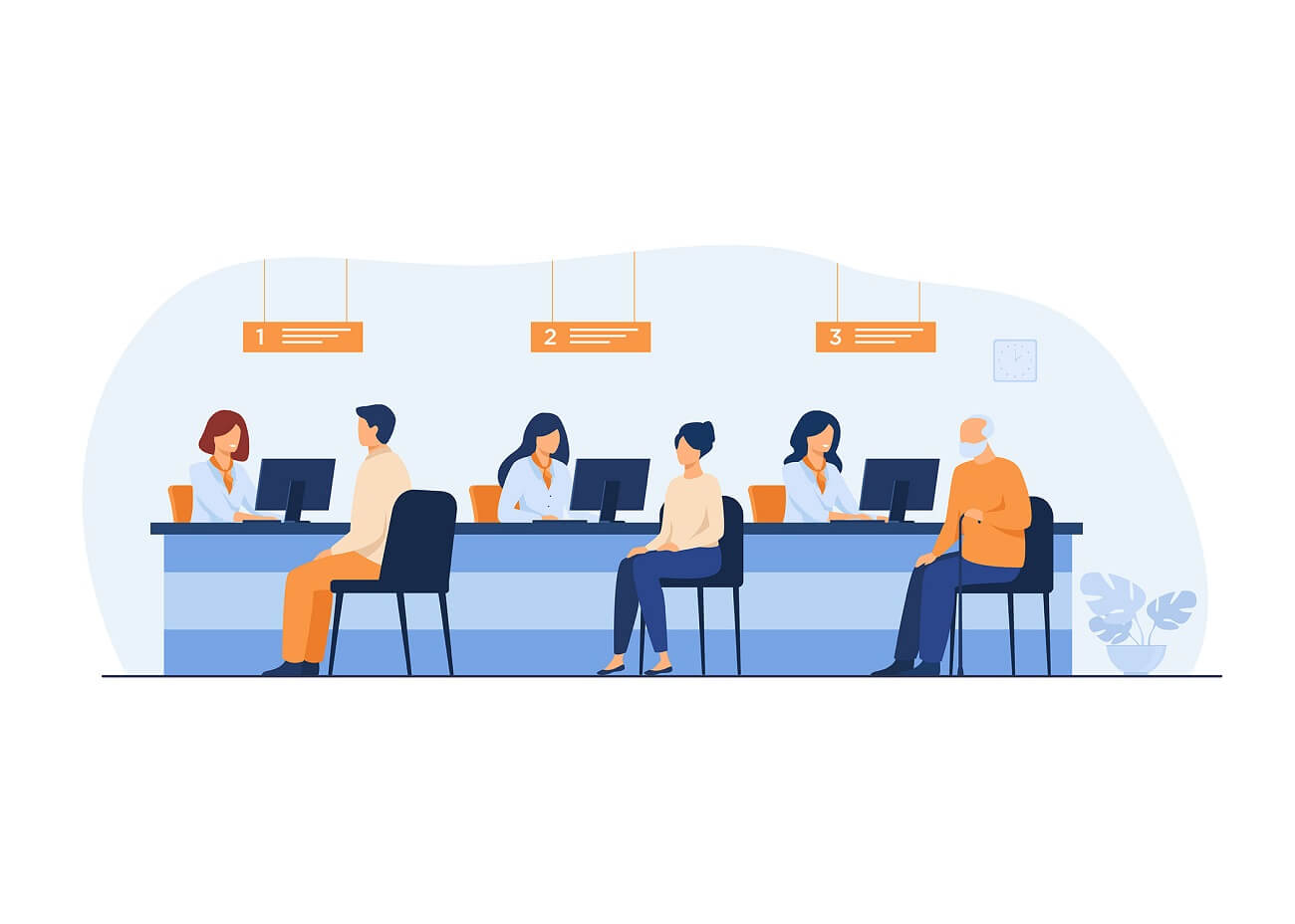
Perhaps the simplest software that you can work with is the one that allows you to deal with bank accounts and transactions regarding it. Designing a robust system that allows you to engage in transactions is something that every beginner should get started with.
The proposed system is a web-based project that allows you to do everything a bank would allow you to do naturally. One should be able to deposit money and withdraw money from a particular account as the user desires.
There should be a validation to allow only a particular amount of cash inflows at any time, as well as to allow withdrawals if the balance is sufficient. There should also be the calculation of interest and its addition to the balance every month.
There can be multiple improvements for this project, including adding support for multiple types of accounts. We started with this because it is still one of the most popular Java projects for beginners.
Source code:
2) Temperature Converter
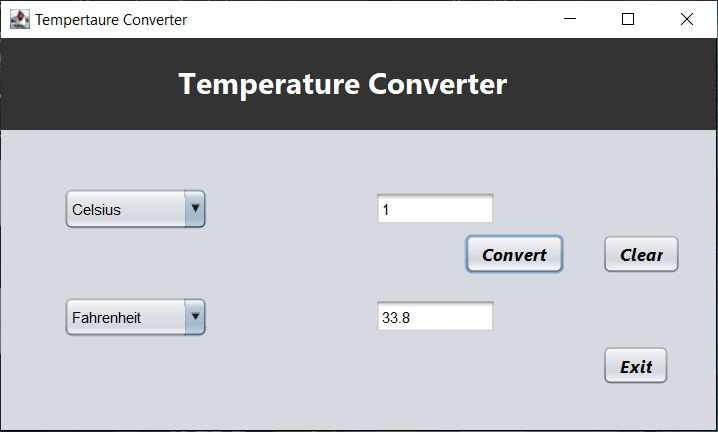
One of the best ways to get started with Java practice projects is by building conversion tools, and what could be simpler than a temperature conversion tool? One already knows the mathematical formula needed for conversion from Fahrenheit to Celsius and from Celsius to Fahrenheit.
In this tool, we have to take input for the value to be converted, do the desired conversion, and then output the converted value. There is also the concept of sanitizing the text being input and displaying an error for incorrect values. There should be a proper error if a non-numerical value is inserted as input.
Source Code:
3) Electricity Billing System
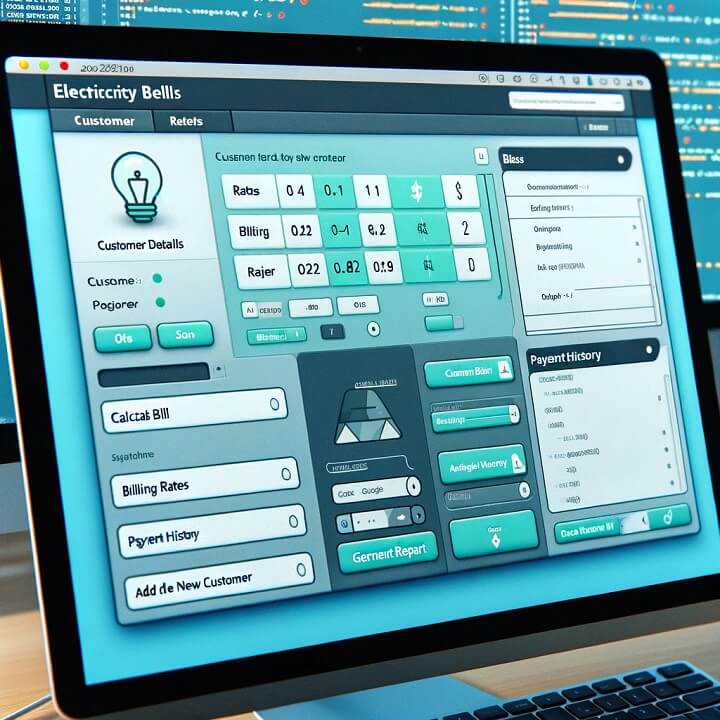
The main task of designing software is to automate something with greater efficiency. In our day-to-day lives, one of the biggest systems that are in dire need of automation is the billing system for electricity.
Till today, bills are generated manually after readings are taken. Work done to automate reading meters and generating bills will go a long way in ensuring work is done in the most efficient way possible while ensuring accuracy in the numbers quoted on the bills.
There are lots of additions that can be done to ensure this remains on top. There aren’t any hard and fast requirements for a flexible mini-project like this one.
4) Supermarket Billing Software
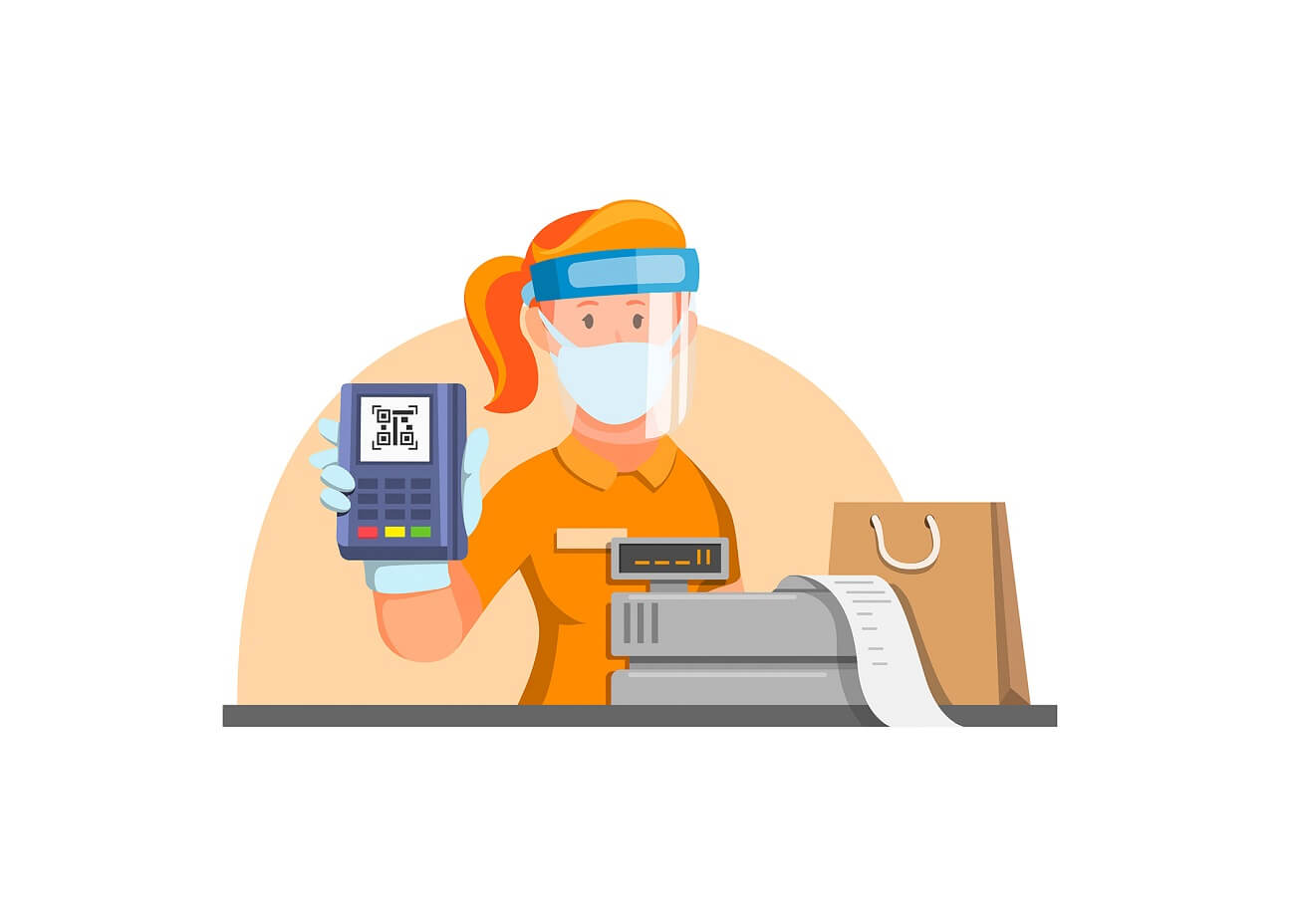
Most supermarkets use software for preparing the final bill before the goods are packed. This software itself can very easily be designed by someone starting in Java. (Note that most of the actual software used for billing in supermarkets uses Java itself).
This billing software sums the amount for individual items and then adds them up to get the final sum that the customer has to pay. The number of individual items and the price of each item should be editable for the user. Also, there should be an option to remove the item from the list altogether.
If one wants to extend their project, one can consider adding an option to export existing bills or implement a way to save previous bills in memory.
5) Memory Game
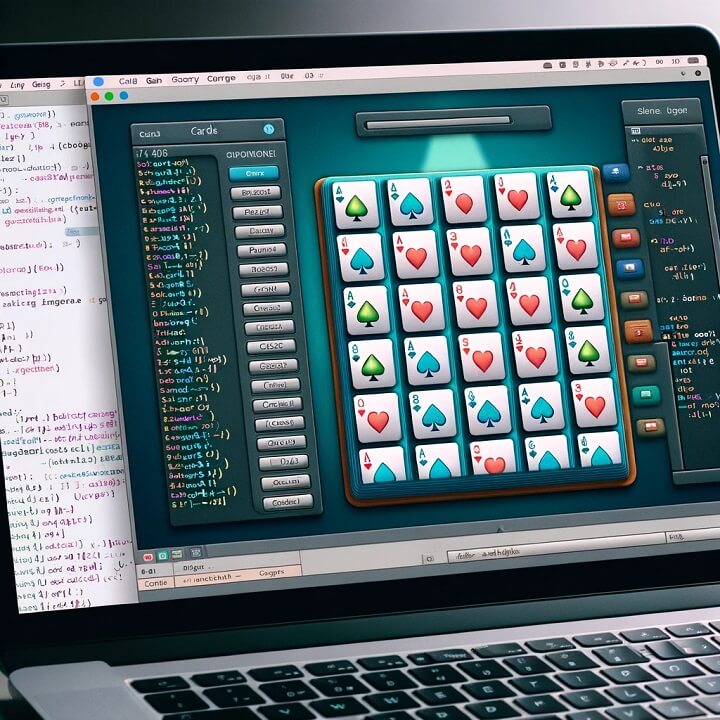
This might be one of the most unique projects that one can come up with while learning the game. Java’s graphics library allows the design of a wide range of small video games that can easily go up on your resume .
In a memory game, a matrix consisting of a large number of smaller boxes will be available, and the user’s task will be to win the game by matching patterns on particular boxes.
When the user clicks one box, it should show its pattern – if the next box clicked on has a similar pattern, both boxes should remain flipped and the score should be added. If the second box flipped does not have a similar pattern, both boxes should stop showing their pattern.
There is a lot of scope in doing this, and a lot of possible improvements. For instance, there can be a way of storing the number of moves required as well as a leaderboard system for hosting folks that have the best memory possible.
6) Link Shortener
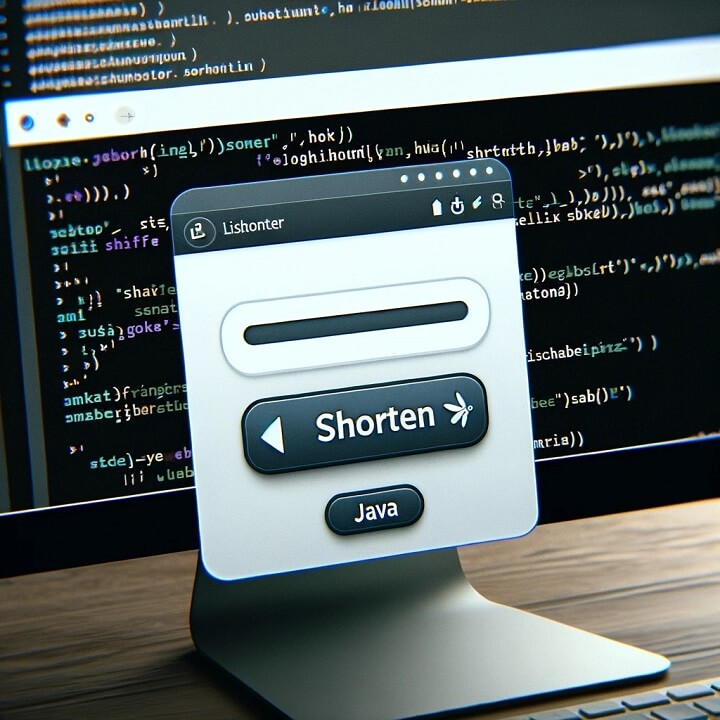
This might seem like something too basic, but it’s something that should exist on your portfolio (if you want to be a good programmer, that is). A good link shortener does wonders in proving not your skills in Java, but your skills in understanding data structures and algorithms.
Hashing is the main concept required for building something, but the challenge is getting it to run on all browsers and point to the actual page instead of showing an error. There should be some validation to check if the link inserted is a proper link or not, and then the appropriate logic should be applied to “shorten” it.
Oh yes, there should be a proper error telling the user that the link entered isn’t a proper link. One improvement that can be done here is adding some sort of history for the application for storing previously shortened links, and for adding an option to add the shortened link to the clipboard directly.
7) Chatting Application
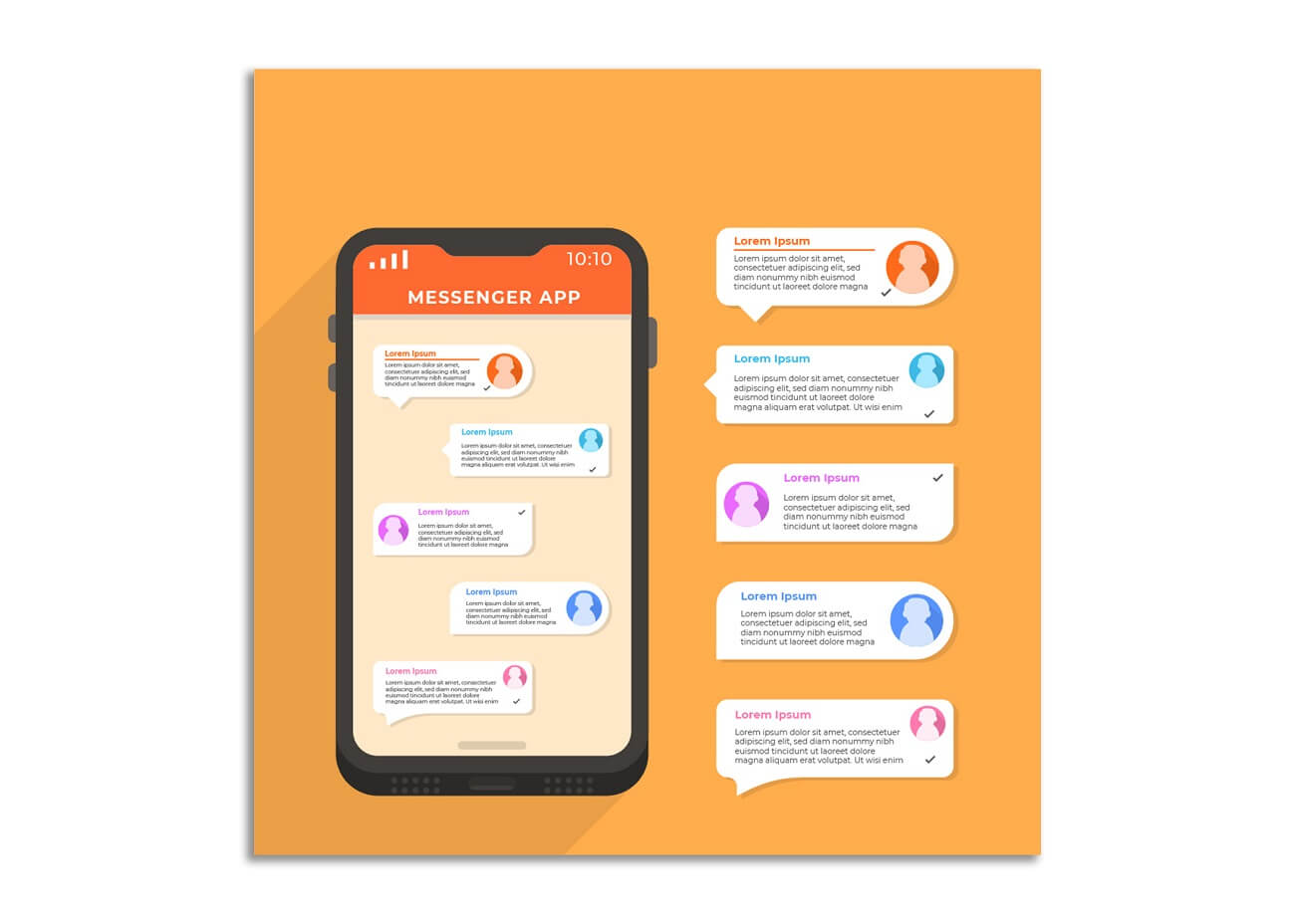
Wondering what a “chatting” application is doing on a list of beginners' projects about Java? Turns out that Java has quite some intense support for network-based libraries too. However, instead of basic request-based communication, this application will need to communicate through sockets.
There are multiple ways to implement this and is probably one of the best ways for people to learn the networking functionality available with Java. A good GUI works wonders in improving the project and improving its appeal to the users.
However, it would be cool if support could be added for file transfer besides normal chat messages. After all, messaging applications would not have come so far if it wasn’t possible to send songs and funny clips to your friends and family, would it?
8) Digital Clock
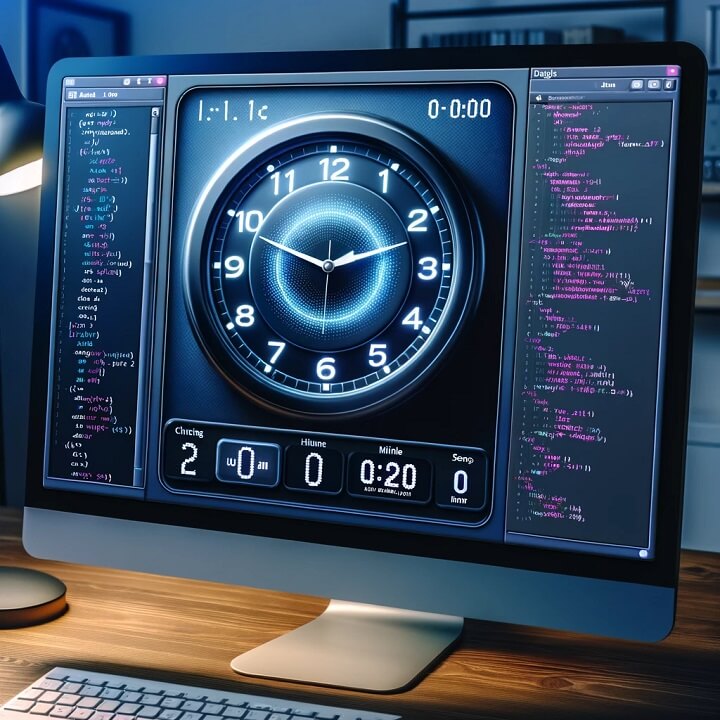
Another simple and easy-to-implement project that you can show off is a digital clock. Some knowledge of UI design is needed here if a visually pleasing design is wanted (which does help a lot!). This requires some event handling as well as periodic execution functions to achieve the desired effect.
Extra features such as other modes, including that of a stopwatch and a counter can be made to improve the project’s output. If that itself isn’t challenging enough, adding the option to move between time zones and show the time according to the time zone selected is certainly going to be a challenge.
9) Quizzing app
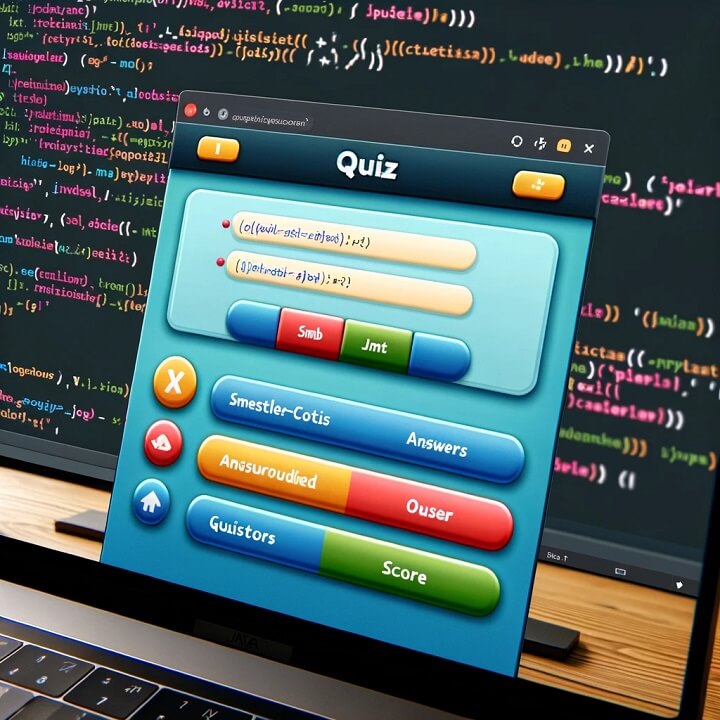
One of the more subtle project to practice that Java allows one to build is apps. Google built Android to be built on top of the Java ecosystem, allowing all features of the Android OS to build an app. One of the best apps to get started is probably a quiz app for distributing questions to friends!
As for extensions, one can be creative when one wants to improve this project. Authentication for users, export a set of questions from the app for use on other apps with the quiz, an online ranking system based on the number of people who have taken the quiz – the possibilities are endless.
Note that the extensions might require some expertise on the Android platform, which might not be taken upon immediately for most Java beginners. So, if you are more into app development, this is an exciting Java project idea to start with.
10) Email-Client Software
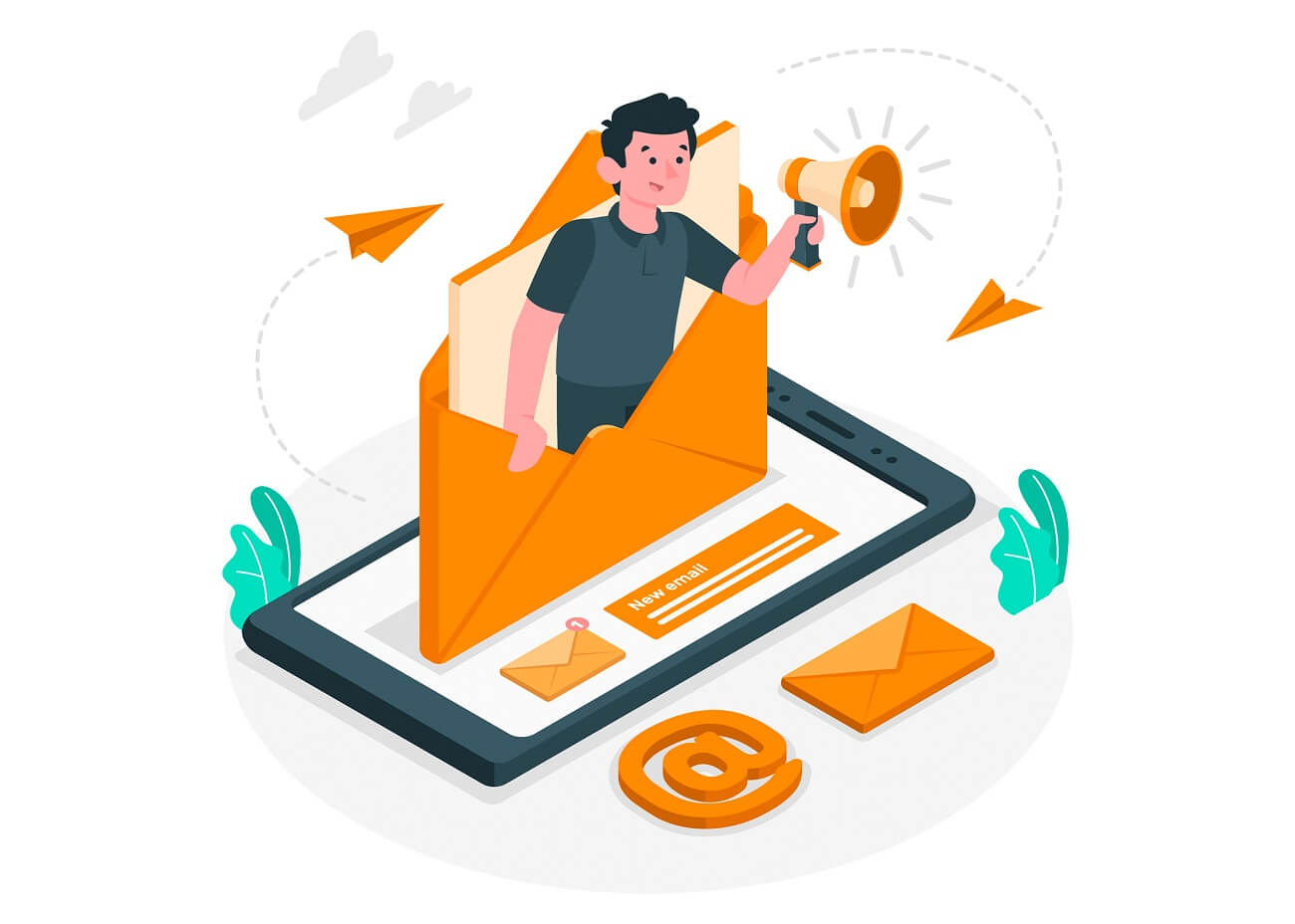
Email has been one of the most important applications of the World Wide Web since its inception. It’s extremely relevant even in today’s world, as most formal conversations take place online via email.
Designing software that allows one to deal with emails not only helps add a decent project to your resume but also allows you to explore some unexplored parts of Java. Designing a proper body with headers and then sending it via a proper channel (SMTP or POP3, depending on the media being sent) is a pretty decent task.
While the main task is to primarily send an email to a destination of the user’s choice, the project can be extended much beyond that. One can add the feature of the creation and storage of drafts for sending them later. And of course, the project becomes a bit more challenging if you need to deal with multimedia as part of the email body as well.
11) Student Management System
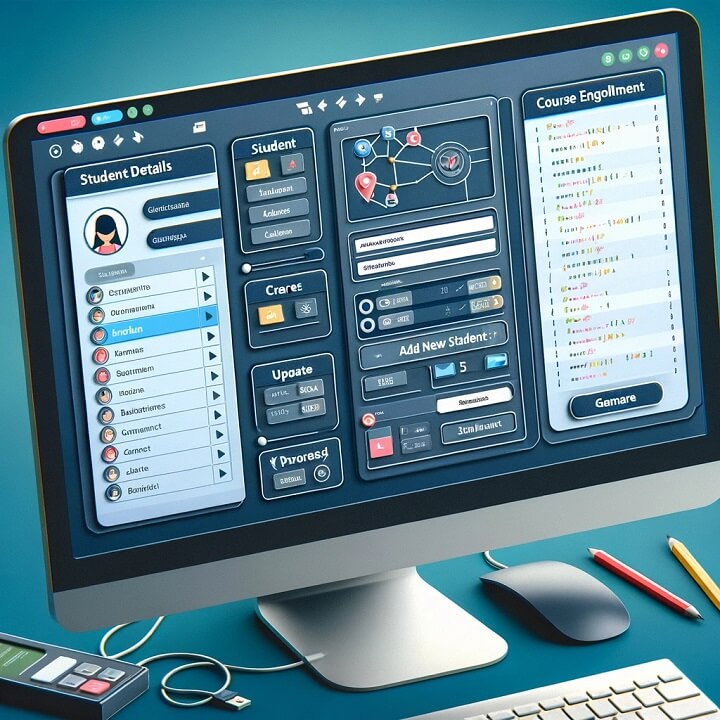
This might be something that most people already have seen in their daily lives. You see your teacher recording your attendance on a sheet, putting in feedback, uploading assignments, etc. Combine everything, and everything should be part of one sweet project to add to your portfolio!
A project as big as this should have its functionality split into three main parts depending on the type of user (which is identified at the time of authentication). These users include:
- Students: Students are designed to be consumers. They log in to access their data, download assignments, upload solutions and projects, respond to feedback provided, and so on.
- Teachers: Teachers are designed to be providers. They log in to provide details about students like feedback, update attendance, communicate with the students, upload assignments, and so on.
- Administrator: Administrators are designed to be the hierarchical elements that form the interconnecting bridge between teachers and students. They are the masters of the system; creating and editing account details, managing the mass inflow of information, checking the status of any submissions, and so on.
This project can be highly customizable and only gets more vibrant once more features are added to improve it. The more, the better.
12) Airline Reservation System
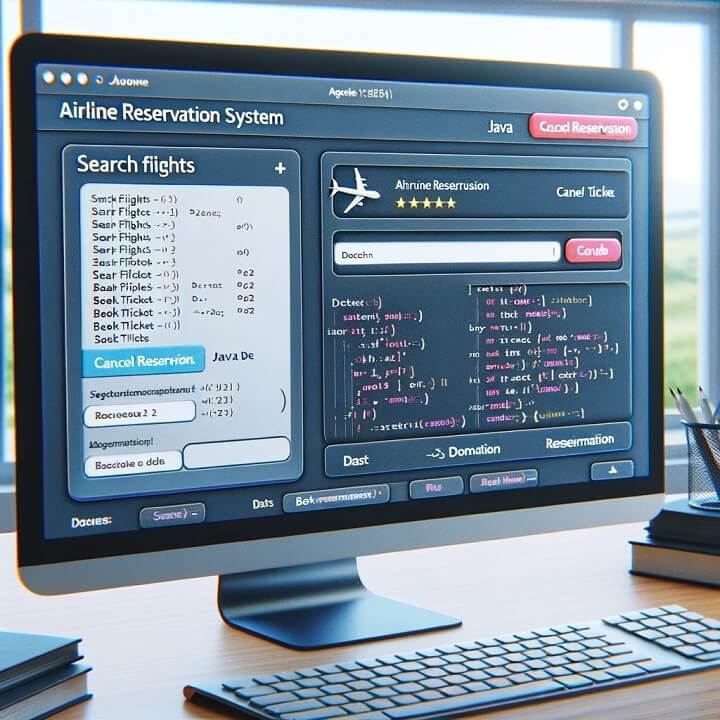
One of the best ways to try out the concepts of OOPs for yourself is with this project, probably. We have seen everyday systems where we make reservations or cancellations for flights, haven’t we?
Designing a similar system as a project can be a very good way to add to the resume! The system should be a web-based one allowing all sorts of functionality that a normal reservation system would have fulfilled.
One should be able to make reservations on certain flights after filling in the requisite data. Reservations should be saved somewhere so that they can be accessed again later for a particular user. One should also be able to cancel reservations provided the reservation to be canceled exists, else an error should be displayed.
This project is a thorough test of your database skills as well, as it requires a lot of data to be dumped and fetched at all times.
13) Food Ordering System
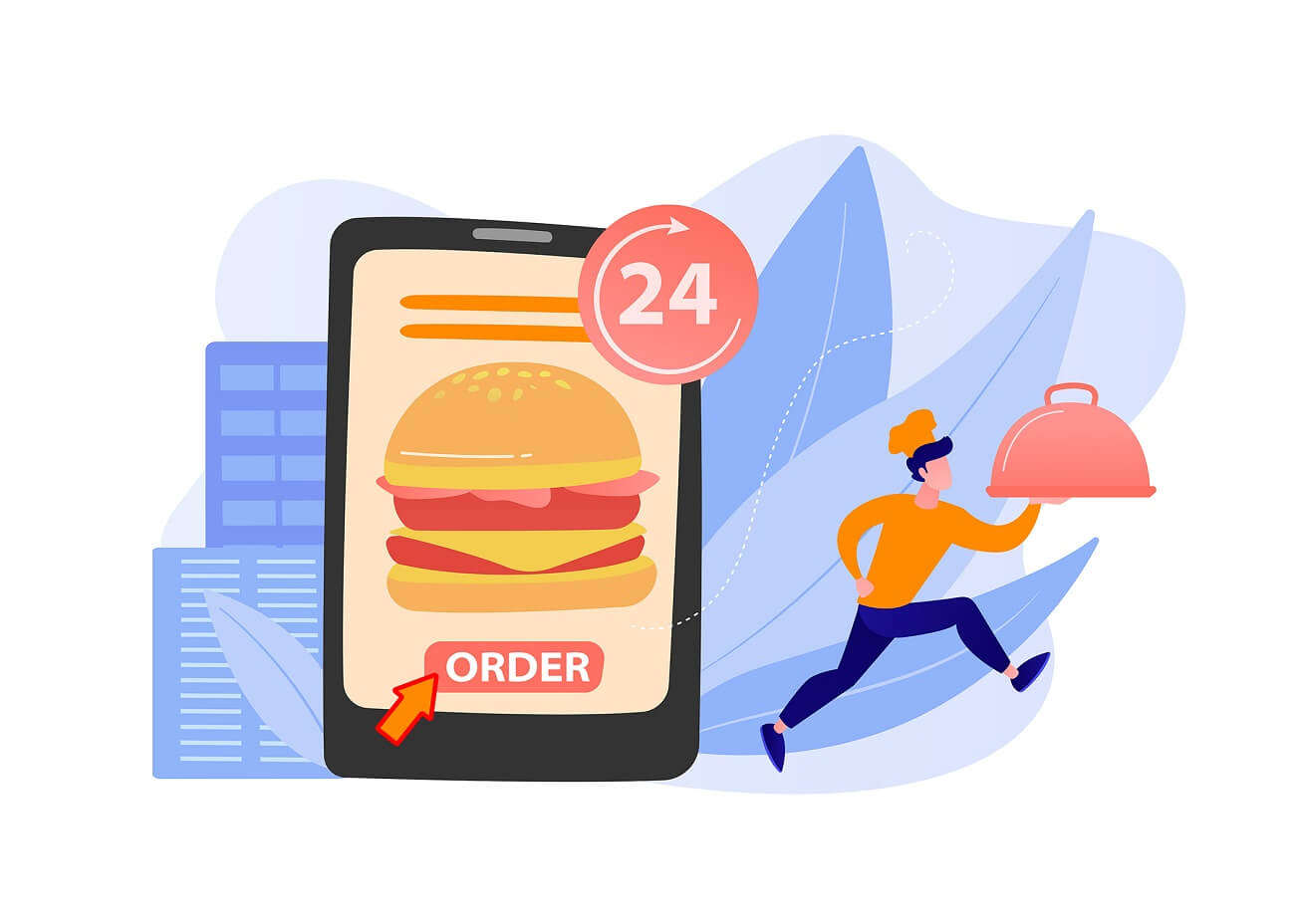
We have all eaten out at restaurants or ordered from outside. It takes a long time for the order to be taken and for our food (and later, our bill) to be delivered, right? One piece of well-designed software can help minimize queue times a lot and thus help increase the number of customers served per day.
The main functionality is to allow the user to order food and send it to the particular restaurant for which the order is being taken and show the user their bill. A sophisticated UI and other small features go a long way in improving this project.
14) Text-based RPG (role-playing game)
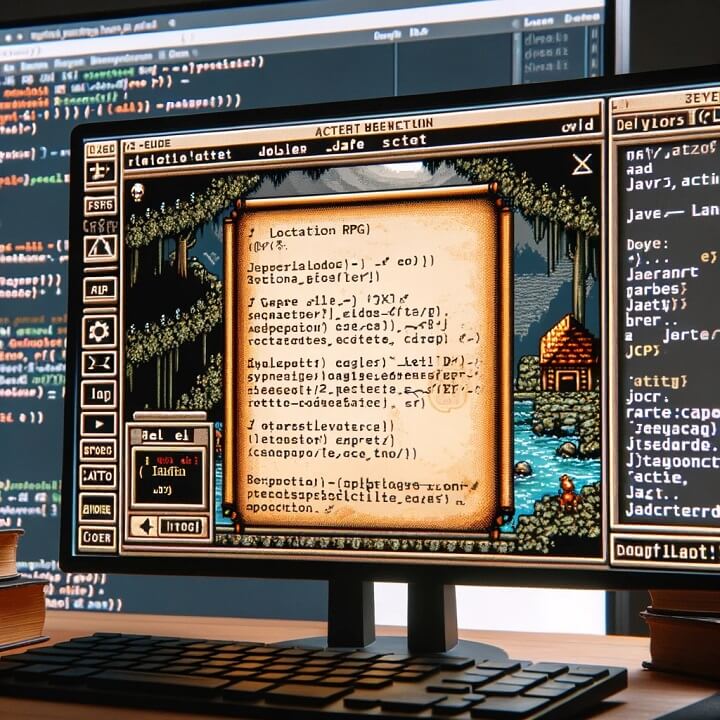
This might probably be one of the coolest things that one can build if they have decided to venture into Java. The idea is to allow the user to navigate their path in a video-game-based setting. The only concept is that users have to choose their next move at every point in the game.
Depending on the outcome of the move, the next choice is presented continuously till one of the conditions to end the game is triggered, which shows the score of the player and how long they survived.
This project is one of the best suggestions for people to start with, as it has less to nothing to implement, yet can be surprisingly complex to deal with. Think your basics about branching instructions and decision-making statements are good enough? This is one sure-shot way to put it to the test.
The flexibility of this project means that one can design the story of this game and its decisions and outcomes in multiple ways possible. It’s totally up to the user as to how they want to proceed with it.
15) Media Player Application
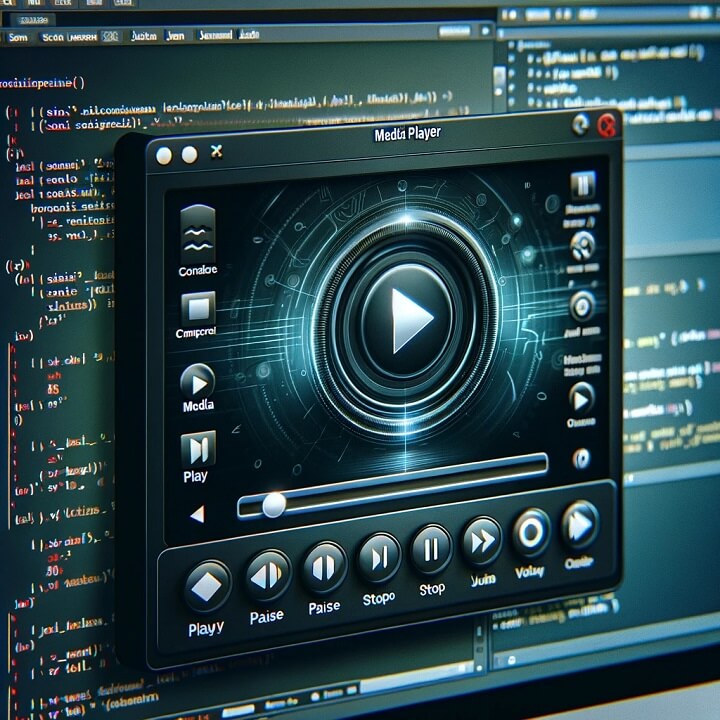
Java is a language of many merits, including the ability to deal with all types of files. We all have used some sort of media player to listen to songs or watch movies, haven’t we? Designing and creating one such media player is going to be tough, but is something that one can take up if one wants to explore more of the Java language.
A media player application may require extensive Java knowledge before it is successfully made, but it can be very rewarding to figure out how to build one. That is why it is an interesting Java project for new programmers.
This is, again, one of the projects that are extremely flexible by nature. Both an Android application and a desktop application can be designed to work as a media player. There’s also a choice to make it play only audio, or play both audio and video (in synchronization!).
Showing details of the video or audio being played as well as the ability to make playlists should also be something up the to-do list as well.
(Note that this project might take some time to implement as it involves a deep understanding of multithreading as well as file handling).
16) Currency Converter
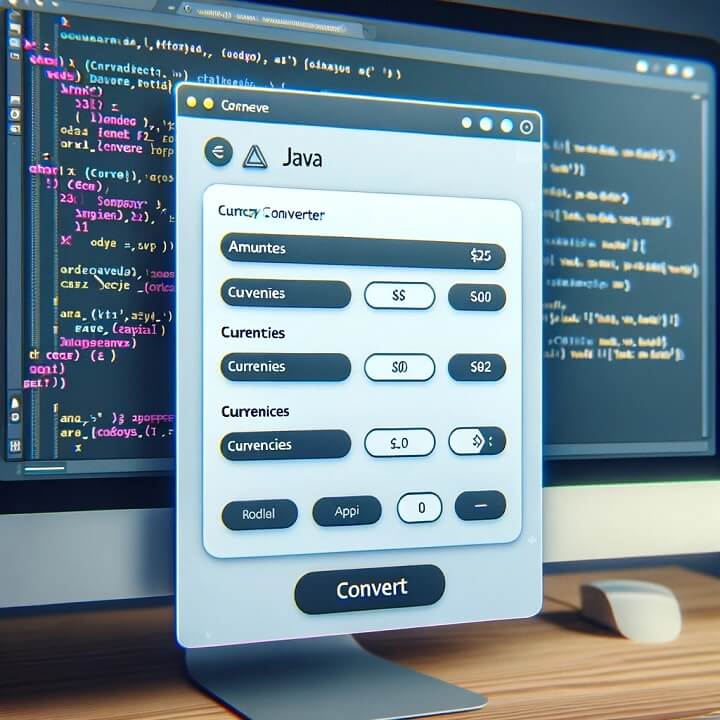
A very basic Java beginner project that is used to convert a currency from one to another. It has a web-based interface you need to just enter the amount, and the currency to which you want to transform, click enter and you get the output.
The real-life use case for this application is many people use this application basically for business, shares, and finance-related areas where the exchange of currency and money transfer happens daily. You can add multiple currencies by adding the corresponding countries with their current exchange prices in the market.
It is a calculator-type application that is developed using the Ajax, Applet, and web features of Java servlets.
17) Brick Breaker Game
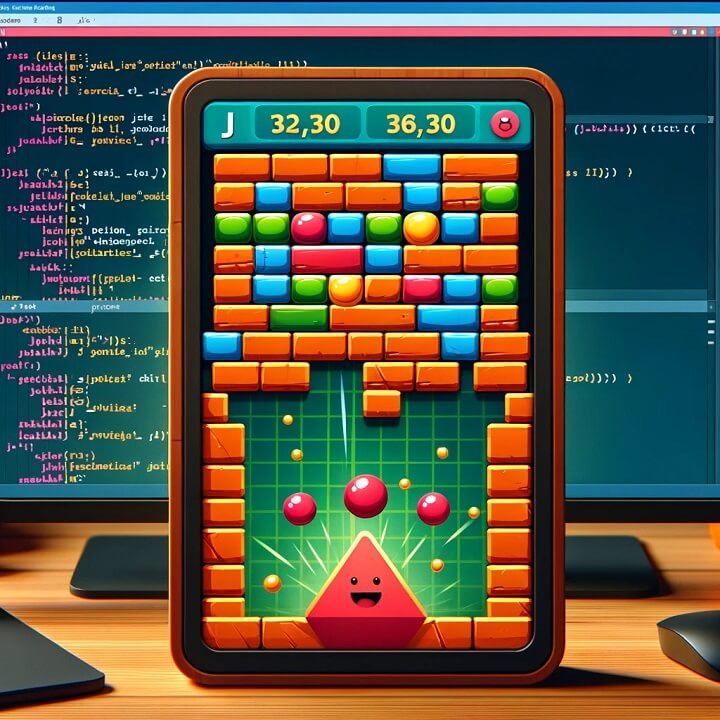
Game applications are the ones that can make your understanding of the Java project better and you would be able to see the essential animation techniques with their integration and can easily experience them. Though this Java project might not be easy for you. Let's understand what this game is about.
This game has a small ball and a small platform at the base and the small ball knocks the bricks taking the help of this small platform at the base. The control of this base is given to the player and the player tries to bounce the ball with this platform.
The number of bricks broken by the ball is the same number of points you scored. i.e., the more you destroy the bricks, the more you score.
If the ball doesn't bounce by the platform then the player will lose the game. This project is beginner-friendly, and one might use this project in their first-year projects list or can experience the implementation for fun purposes.
18) Number Guessing Game
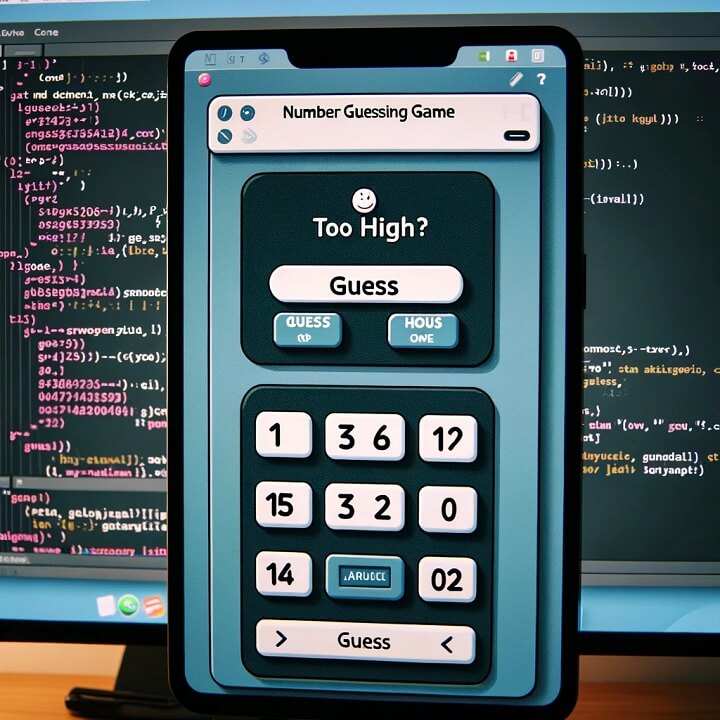
It's a very easy game to develop as a Java Developer and easy to understand as well. The only functionality provided by this application is there will be a range of numbers specified to the users, and users have to just guess the number.
If the number guessed is correct, then the player wins, else loses. You can restrict the count of guessing the numbers for the users to make this more interesting and fun.
This is a web-based application where there will be an input provided to the user and they to just guess the number in the given range and a time clock will be there. If the guessed number is correct: the player Wins ! or Loses!
If the guess value is close to the correct value, you might provide the user with a pop-up of too-close value! You need to know about Random Class in Java to make this game.
19) Tic-Tac-Toe Game
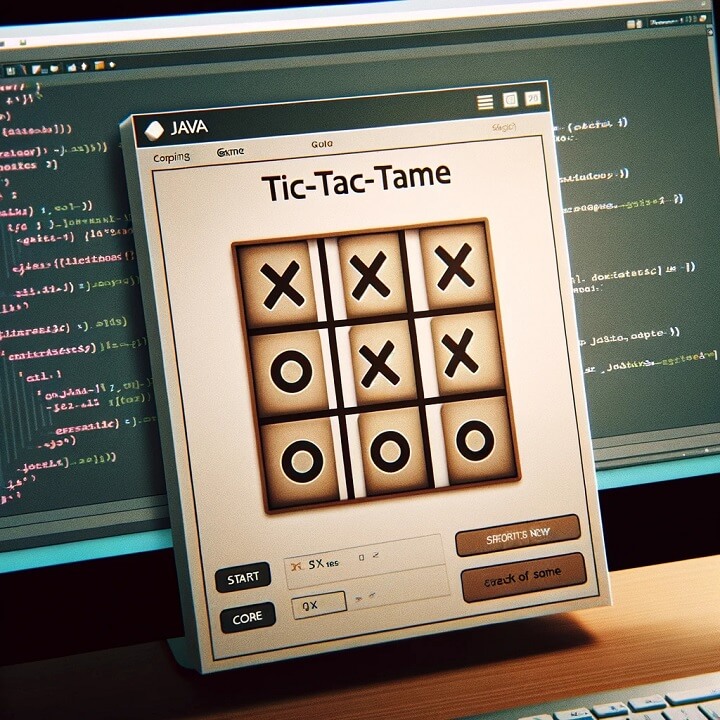
It's a very famous game that you all must be aware of. It is developed using GUI (Graphical User Interface) in Java. Players generally prefer this kind of game when they’re bored and want something to play that is quick and easy.
This game application has a 3x3 box displayed on the screen. The first person who starts the game has to enter either X or O for any one box, followed by the other player entering the other X or O. This continues unless any one of them gets a line cut either diagonally or straight. And the person who finds the line is the winner of the game.
But you first need to have an understanding of Java Swing, Java GUI (Graphical User Interface), and JFrame.
20) Word Counter
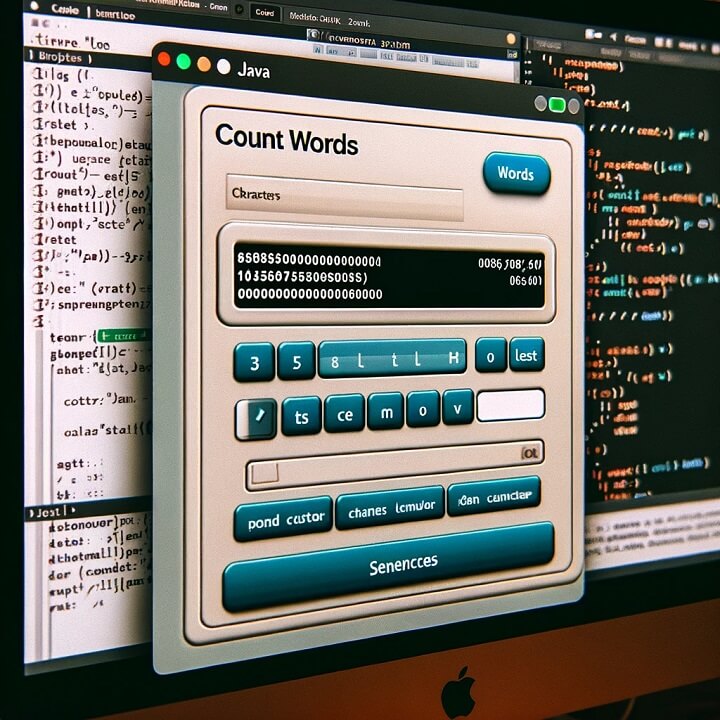
This application tells you the exact number of words used in the pasted paragraph by the user in the input. A simple project for beginners is good to start. It can be built using Swing in Java. This is a counting-word application Java-based project.
Remember, our childhood days when we were asked to write an essay on a given topic where the word length should be 500 or 1000? This application comes with a feature that could help you.
You can further add functionalities like showcasing the number of characters, words, and paragraphs it has. Also, it is completely free to use and there’s no word count limit.
21) Online Quiz Platform
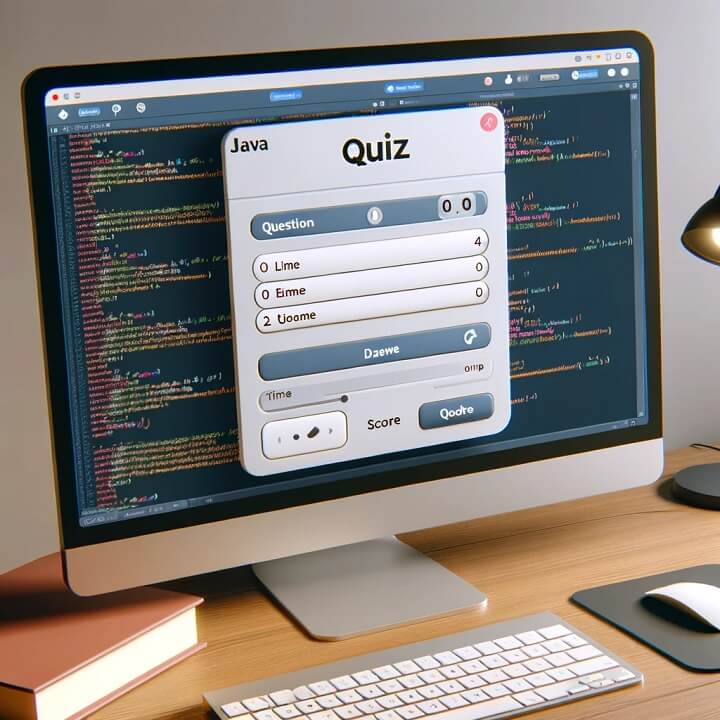
An online quiz platform can be built in Java that helps us access a diverse range of quizzes on various subjects and topics. The platform offers an interactive interface where we can select quizzes, attempt questions, receive instant feedback, and track their progress.
We can add the following features to this quiz platform:
- User Authentication: Users can create accounts, log in securely, and access quizzes based on their preferences.
- Quiz Selection: Users can choose quizzes from multiple categories such as science, mathematics, history, etc.
- Interactive Quiz Interface : Engaging user interface for displaying questions, multiple-choice answers, and providing immediate feedback.
- Scoring and Progress Tracking: Users can view their scores, track their progress, and review past quiz attempts.
- Admin Panel: Administrators can manage quizzes, add new questions, and analyze user statistics.
22) Health and Fitness Tracker
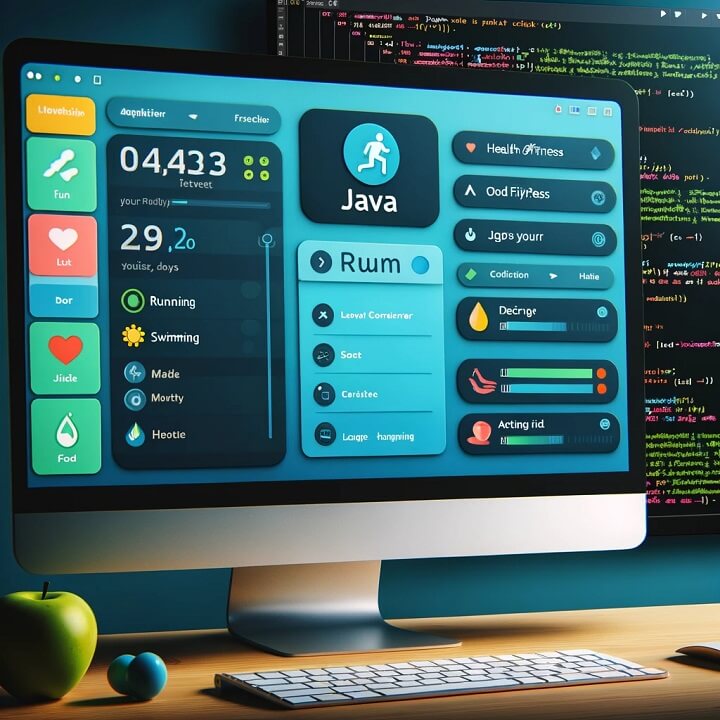
In today’s age when everyone is concerned too much about their health, a Health and Fitness Tracker can be developed in Java that serves as an invaluable tool for individuals striving for a healthier lifestyle.
The Health and Fitness Tracker is a Java-based application designed to assist users in monitoring their daily activities, nutritional intake, and overall fitness progress. It offers a comprehensive dashboard where users can input data and track their health goals.
It can help us in many ways, like:
- Personalized Health Monitoring: Users can tailor the tracker to their specific health needs and goals.
- Motivation and Accountability: The visual representation of progress serves as motivation to stay committed to a healthy lifestyle.
- Data-Driven Insights: Analyze tracked data to gain insights into habits, patterns, and areas for improvement.
23) Appointment Scheduler
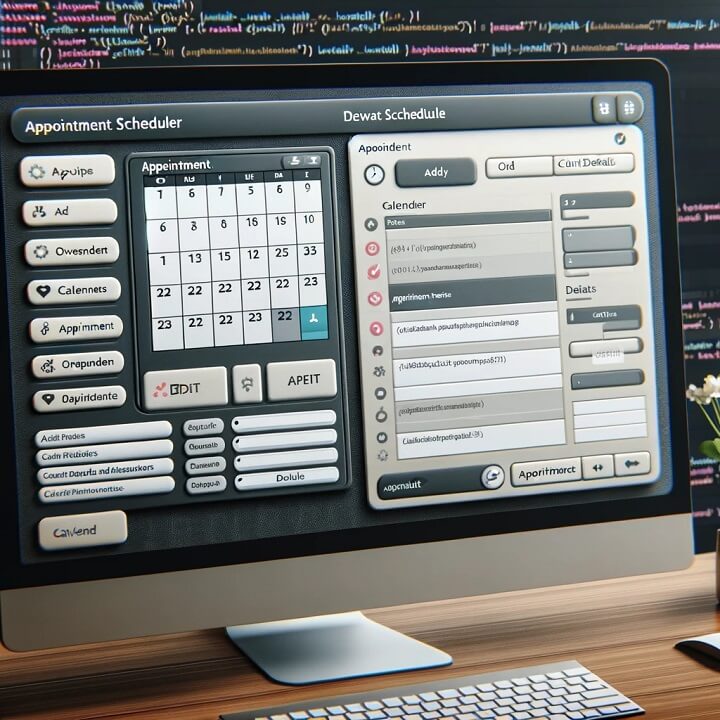
Efficiently managing appointments and schedules is essential in various fields, from healthcare to business. An Appointment Scheduler developed in Java can streamline the process, ensuring organized and optimized time management.
The Appointment Scheduler application in Java provides a user-friendly platform for scheduling and managing appointments. It caters to various industries where appointment booking and management are critical.
We can implement this in simple steps:
- User Authentication and Role Management: Use frameworks like Spring Security for authentication and authorization. Implement different user roles (admin, staff, clients/customers).
- Database Setup: Use MySQL or PostgreSQL to store user details, appointment schedules, and related data.
- Utilize JavaFX for a visually appealing calendar view.
- Implement functionalities to schedule appointments and display them in the calendar.
- Search and Filter Functionality: Implement features for users to search for available slots based on date/time or service type.
24) Online Auction System
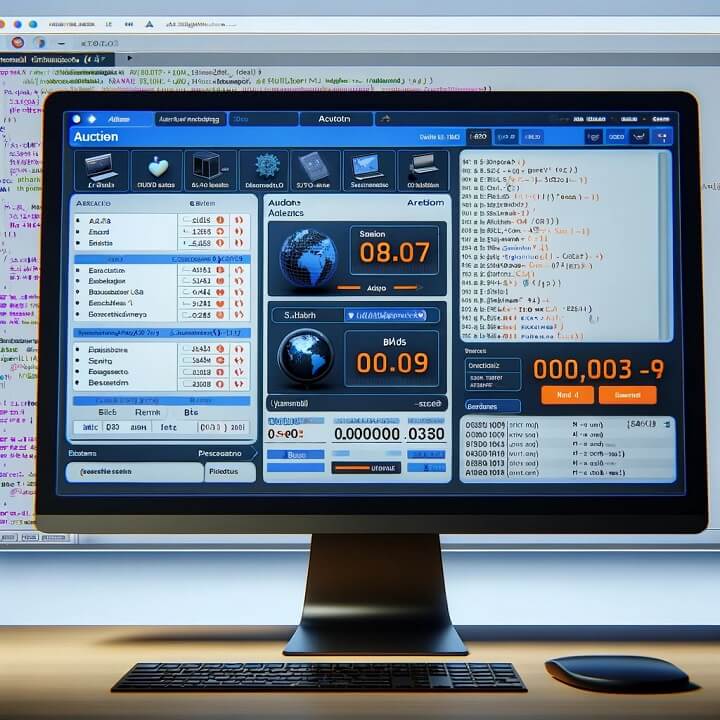
Online auctions have transformed commerce by providing a platform for buying and selling goods. Developing an Online Auction System using Java enables users to engage in exciting bidding experiences. The Online Auction System in Java offers a virtual marketplace where users can bid on items, sellers can list products, and an auctioneer manages the bidding process.
The following features can be implemented in this application:
- User Registration and Authentication: Secure login/signup for buyers, sellers, and admin roles.
- Product Listings: Sellers can add products with descriptions, images, and starting bid prices.
- Bidding Mechanism: Buyers can place bids, receive notifications on higher bids, and track auction progress.
- Auction Management: Admin panel to oversee auctions, close/open bidding, and manage listings.
- Payment Integration: Integration with payment gateways for secure transactions.
- Feedback and Rating : Users can leave feedback and rate sellers/buyers after successful transactions.
25) Language Learning App
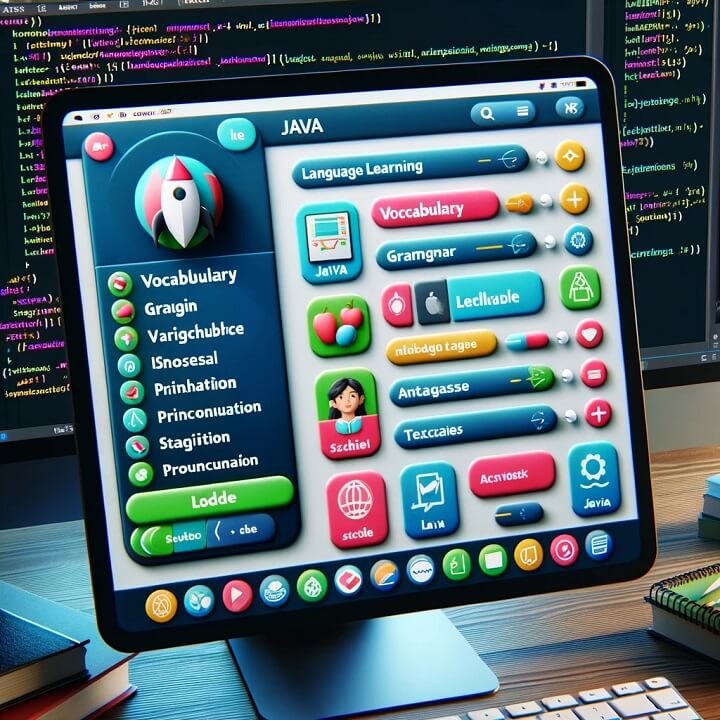
Learning a new language opens doors to new cultures and opportunities. A Language Learning App developed in Java provides an interactive platform for users to master a new language.
The Language Learning App offers a user-friendly interface with various tools and exercises to aid users in language acquisition.
We can follow the below steps to implement it:
- User Registration and Profile Creation: Allow users to register and create profiles, select their target language, and set learning goals.
- Design structured lessons covering vocabulary, grammar, listening, speaking, and writing exercises.
- Create interactive quizzes to assess language proficiency.
- Implement speech recognition features for pronunciation practice.
- Set up community forums for users to interact and practice language skills.
- Implement a system to track user progress with stats and achievements.
- Utilize MySQL or SQLite for database management.
- Develop backend functionalities for user data storage and retrieval.
Which Projects are Best for New Programmers?
It’s a lot of projects, isn’t it? That should be enough to get started with. Of course, even among these projects, some are easier than others. Like always, it’s wise to build stepping stones while learning any language. The mantra is to get started with the easier projects, and then move on to bigger and better ones as one’s understanding of the language improves.
The recommendation for new programmers making a project for the first time is to get started with the projects like the temperature converter, the digital clock, the link shortener, or the memory game . These are the most simple Java project ideas for beginners.
They have pretty straightforward requirements and don’t require a huge amount of time to build or code. Once the beginner projects are pretty much done and dusted, it’s time to move on to slightly bigger and better stuff.
The intermediate projects include stuff like the chatting application, the text-based role-playing game, the supermarket billing software, or the email client software. These projects are slightly more complex and require some time investment before they can be completed.
Projects like the Online quiz platform, appointment scheduler, electricity billing system, or the student management system can be done next. These have requirements that are mostly tailored for different situations, hence it might require some research.
However, if you face problems with any of the above projects, or you need Java homework help , our experts are always ready to help you.
However good of a programmer you are, no one will be convinced about your skills unless you can show off a practical demonstration of the same. Nothing’s better than getting started with a few simple Java projects for beginners to show that you have some hold over the implementation of real-world applications.
Remember, it’s much more difficult to break into the programming world if you don’t have practical experience in coding. Coding a few Java practice projects helps mitigate this, and opens the doors for employment!
Frequently Asked Questions (FAQs)
1) what are some good java projects.
If you are a beginner then you can start with some Java projects like Bank Management Software, Electricity Billing System, Temperature Converter, and Supermarket Billing Software. We can also build a Digital Clock, Quizzing App, Email-Client Software, Student Management System, Airline Management System, or Food Ordering System.
2) Why do we need to build Java projects?
Projects are important for developing core Java skills and it also helps to shine your resume. If you are a beginner, projects are a way to show that you understand the underlying concepts of the programming language and are ready to apply them to solve real-world problems.
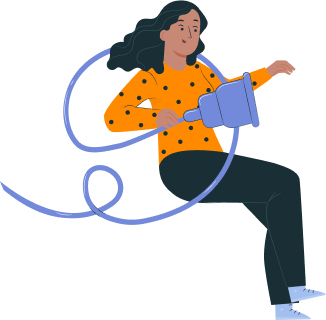
FavTutor - 24x7 Live Coding Help from Expert Tutors!
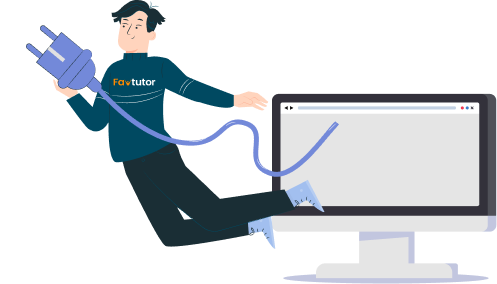
About The Author
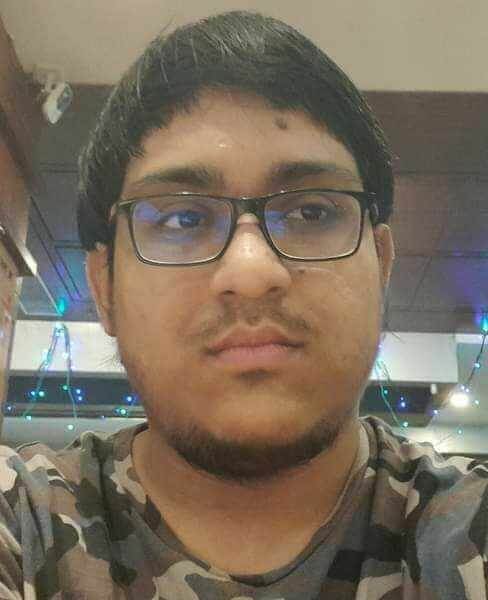
Arkadyuti Bandyopadhyay
More by favtutor blogs, monte carlo simulations in r (with examples), aarthi juryala.
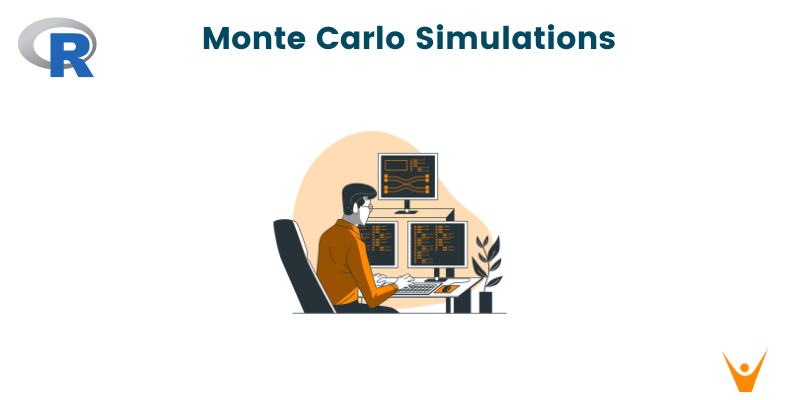
The unlist() Function in R (with Examples)
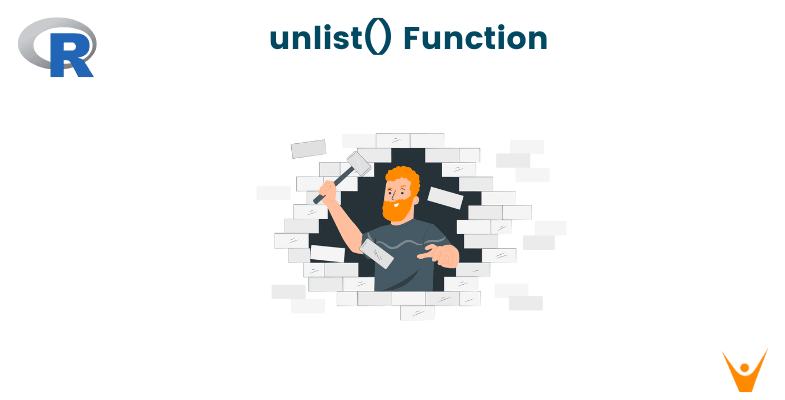
Paired Sample T-Test using R (with Examples)
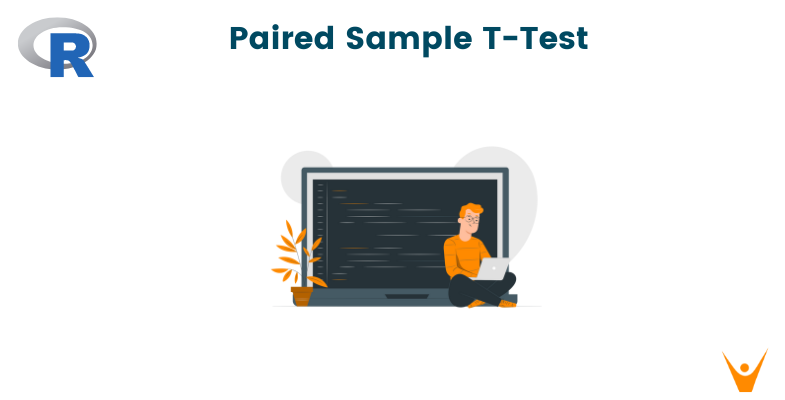
- C Program : Remove Vowels from A String | 2 Ways
- C Program : Remove All Characters in String Except Alphabets
- C Program : Sorting a String in Alphabetical Order – 2 Ways
- C Program To Check If Vowel Or Consonant | 4 Simple Ways
- C Program To Print Number Of Days In A Month | Java Tutoring
- C Program To Check Whether A Number Is Even Or Odd | C Programs
- C Program To Find Reverse Of An Array – C Programs
- C Program To Count The Total Number Of Notes In A Amount | C Programs
- C Program To Check A Number Is Negative, Positive Or Zero | C Programs
- C Program To Input Any Alphabet And Check Whether It Is Vowel Or Consonant
- C Program Inverted Pyramid Star Pattern | 4 Ways – C Programs
- C Program To Check Whether A Character Is Alphabet or Not
- C Program To Check Character Is Uppercase or Lowercase | C Programs
- C Program To Check If Alphabet, Digit or Special Character | C Programs
- C Program To Find Maximum Between Three Numbers | C Programs
- C Program To Check Whether A Year Is Leap Year Or Not | C Programs
- C Program Area Of Triangle | C Programs
- C Program To Check If Triangle Is Valid Or Not | C Programs
- C Program Find Circumference Of A Circle | 3 Ways
- C Program To Calculate Profit or Loss In 2 Ways | C Programs
- C Program Area Of Rectangle | C Programs
- X Star Pattern C Program 3 Simple Ways | C Star Patterns
- C Program Hollow Diamond Star Pattern | C Programs
- C Program To Check Number Is Divisible By 5 and 11 or Not | C Programs
- Mirrored Rhombus Star Pattern Program In c | Patterns
- C Program Area Of Rhombus – 4 Ways | C Programs
- C Program Area Of Isosceles Triangle | C Programs
- C Program Area Of Square | C Programs
- C Program To Find Area Of Semi Circle | C Programs
- C Program To Find Volume of Sphere | C Programs
- C Program Area Of Trapezium – 3 Ways | C Programs
- C Program Area Of Parallelogram | C Programs
- C Program Check A Character Is Upper Case Or Lower Case
- Hollow Rhombus Star Pattern Program In C | Patterns
- C Program To Count Total Number Of Notes in Given Amount
- C Program Area Of Equilateral Triangle | C Programs
- C Program to find the Area Of a Circle
- C Program To Find Volume Of Cone | C Programs
- C Program To Calculate Volume Of Cube | C Programs
- C Program To Calculate Perimeter Of Rectangle | C Programs
- C Program To Calculate Perimeter Of Rhombus | C Programs
- C Program Volume Of Cuboid | C Programs
- C Program To Calculate Perimeter Of Square | C Programs
- C Program To Search All Occurrences Of A Character In String | C Programs
- C Program Count Number Of Words In A String | 4 Ways
- C Program To Left Rotate An Array | C Programs
- C Program To Copy All Elements From An Array | C Programs
- C Program To Toggle Case Of Character Of A String | C Programs
- C Program To Delete Duplicate Elements From An Array | 4 Ways
- C Program Inverted Right Triangle Star Pattern – Pattern Programs
- C Program Volume Of Cylinder | C Programs
- C Square Star Pattern Program – C Pattern Programs | C Programs
- C Program To Remove First Occurrence Of A Character From String
- C Program To Compare Two Strings – 3 Easy Ways | C Programs
- C Program To Search All Occurrences Of A Word In String | C Programs
- C Mirrored Right Triangle Star Pattern Program – Pattern Programs
- C Program To Count Occurrences Of A Word In A Given String | C Programs
- C Program To Find Reverse Of A string | 4 Ways
- C Program To Delete An Element From An Array At Specified Position | C Programs
- C Program To Reverse Words In A String | C Programs
- C Program To Remove Last Occurrence Of A Character From String
- C Programs – 500+ Simple & Basic Programming Examples & Outputs
- C Pyramid Star Pattern Program – Pattern Programs | C
- C Program Replace First Occurrence Of A Character With Another String
- C Program Replace All Occurrences Of A Character With Another In String
- C Program To Remove Repeated Characters From String | 4 Ways
- C Plus Star Pattern Program – Pattern Programs | C
- C Program To Find Last Occurrence Of A Word In A String | C Programs
- Hollow Square Pattern Program in C | C Programs
- C Program To Check A String Is Palindrome Or Not | C Programs
- C Program To Remove Blank Spaces From String | C Programs
- Rhombus Star Pattern Program In C | 4 Multiple Ways
- C Program To Find Last Occurrence Of A Character In A Given String
- C Program To Trim Leading & Trailing White Space Characters From String
- C Program To Copy One String To Another String | 4 Simple Ways
- C Program Number Of Alphabets, Digits & Special Character In String | Programs
- Merge Two Arrays To Third Array C Program | 4 Ways
- C Program To Find Maximum & Minimum Element In Array | C Prorams
- C Program To Sort Even And Odd Elements Of Array | C Programs
- C Program To Search An Element In An Array | C Programs
- C Program Right Triangle Star Pattern | Pattern Programs
- C Program To Count Frequency Of Each Character In String | C Programs
- C Program Count Number Of Vowels & Consonants In A String | 4 Ways
- C Program Find Maximum Between Two Numbers | C Programs
- C Program To Count Frequency Of Each Element In Array | C Programs
- C Program To Trim Trailing White Space Characters From String | C Programs
- C Program To Trim White Space Characters From String | C Programs
- Highest Frequency Character In A String C Program | 4 Ways
- C Program To Find First Occurrence Of A Word In String | C Programs
- C Program To Concatenate Two Strings | 4 Simple Ways
- C Program To Remove First Occurrence Of A Word From String | 4 Ways
- C Program To Insert Element In An Array At Specified Position
- C Program To Convert Lowercase String To Uppercase | 4 Ways
- C Program To Print All Unique Elements In The Array | C Programs
- C Program To Put Even And Odd Elements Of Array Into Two Separate Arrays
- C Program To Count Occurrences Of A Character In String | C Programs
- C Program To Count Number Of Even & Odd Elements In Array | C Programs
- C Program To Sort Array Elements In Ascending Order | 4 Ways
- C Program To Count Number Of Negative Elements In Array
- C Program Hollow Inverted Right Triangle Star Pattern
- C Program To Convert Uppercase String To Lowercase | 4 Ways
- C Program To Print Number Of Days In A Month | 5 Ways
- Diamond Star Pattern C Program – 4 Ways | C Patterns
- C Program To Input Week Number And Print Week Day | 2 Ways
- C Program Hollow Inverted Mirrored Right Triangle
- C Program To Remove All Occurrences Of A Character From String | C Programs
- C Program Count Number of Duplicate Elements in An Array | C Programs
- C Program To Find Sum Of All Array Elements | 4 Simple Ways
- C Program To Find Lowest Frequency Character In A String | C Programs
- C Program To Sort Array Elements In Descending Order | 3 Ways
- 8 Star Pattern – C Program | 4 Multiple Ways
- C Program To Read & Print Elements Of Array | C Programs
- C Program To Replace Last Occurrence Of A Character In String | C Programs
- C Program To Find Length Of A String | 4 Simple Ways
- C Program To Right Rotate An Array | 4 Ways
- C Program To Find First Occurrence Of A Character In A String
- C Program Hollow Mirrored Rhombus Star Pattern | C Programs
- C Program Half Diamond Star Pattern | C Pattern Programs
- C Program Hollow Mirrored Right Triangle Star Pattern
- Hollow Inverted Pyramid Star Pattern Program in C
- C Program To Print All Negative Elements In An Array
- Right Arrow Star Pattern Program In C | 4 Ways
- C Program : Capitalize First & Last Letter of A String | C Programs
- C Program Hollow Right Triangle Star Pattern
- C Program : Check if Two Arrays Are the Same or Not | C Programs
- C Program : Check if Two Strings Are Anagram or Not
- Left Arrow Star Pattern Program in C | C Programs
- C Program Mirrored Half Diamond Star Pattern | C Patterns
- C Program Inverted Mirrored Right Triangle Star Pattern
- Hollow Pyramid Star Pattern Program in C
- C Program : Non – Repeating Characters in A String | C Programs
- C Program : Sum of Positive Square Elements in An Array | C Programs
- C Program : Find Longest Palindrome in An Array | C Programs
- C Program : To Reverse the Elements of An Array | C Programs
- C Program Lower Triangular Matrix or Not | C Programs
- C Program : Maximum Scalar Product of Two Vectors
- C Program Merge Two Sorted Arrays – 3 Ways | C Programs
- C Program : Check If Arrays are Disjoint or Not | C Programs
- C program : Find Median of Two Sorted Arrays | C Programs
- C Program : Convert An Array Into a Zig-Zag Fashion
- C Program : Minimum Scalar Product of Two Vectors | C Programs
- C Program : Find Missing Elements of a Range – 2 Ways | C Programs
- C Program Transpose of a Matrix 2 Ways | C Programs
- C Program Patterns of 0(1+)0 in The Given String | C Programs
- C Program : To Find Maximum Element in A Row | C Programs
- C Program : To Find the Maximum Element in a Column
- C Program : Rotate the Matrix by K Times | C Porgrams
- C Program To Check Upper Triangular Matrix or Not | C Programs
- C Program : Check if An Array Is a Subset of Another Array
- C Program : Non-Repeating Elements of An Array | C Programs
- C Program : Rotate a Given Matrix by 90 Degrees Anticlockwise
- C Program Sum of Each Row and Column of A Matrix | C Programs
Learn Java Java Tutoring is a resource blog on java focused mostly on beginners to learn Java in the simplest way without much effort you can access unlimited programs, interview questions, examples
Java programs – 500+ simple & basic programs with outputs.
in Java Programs , Java Tutorials March 6, 2024 Comments Off on Java Programs – 500+ Simple & Basic Programs With Outputs
Java programs: Basic Java programs with examples & outputs. Here we covered over the list of 500+ Java simple programs for beginners to advance, practice & understood how java programming works. You can take a pdf of each program along with source codes & outputs.
In case if you are looking out for C Programs , you can check out that link.
We covered major Simple to basic Java Programs along with sample solutions for each method. If you need any custom program you can contact us.
All of our Sample Java programs with outputs in pdf format are written by expert authors who had high command on Java programming. Even our Java Tutorials are with rich in-depth content so that newcomers can easily understand.
1. EXECUTION OF A JAVA PROGRAM
Static loading : A block of code would be loaded into the RAM before it executed ( i.e after being loaded into the RAM it may or may not get executed )
Dynamic loading: A block of code would be loaded into the RAM only when it is required to be executed.
Note: Static loading took place in the execution of structured programming languages. EX: c- language
Java follows the Dynamic loading
– JVM would not convert all the statements of the class file into its executable code at a time.
– Once the control comes out from the method, then it is deleted from the RAM and another method of exe type will be loaded as required.
– Once the control comes out from the main ( ), the main ( ) method would also be deleted from the RAM. This is why we are not able to view the exe contents of a class file.
Simple Hello Word Program
Out of 500+ Simple & Basic Java Programs: Hello world is a first-ever program which we published on our site. Of course, Every Java programmer or C programmer will start with a “Hello World Program”. Followed by the rest of the programs in different Categories.
Basic Java Programs – Complete List Here
Advanced simple programming examples with sample outputs, string, array programs.
Sort Programs
Conversion Programs:
Star & Number Pattern Programs
Functions of JVM:
- It converts the required part if the bytecode into its equivalent executable code.
- It loads the executable code into the RAM.
- Executes this code through the local operating system.
- Deletes the executable code from the RAM.
We know that JVM converts the class file into its equivalent executable code. Now if a JVM is in windows environment executable code that is understood by windows environment only.
Similarly, same in the case with UNIX or other or thus JVM ID platform dependent.
Java, With the help of this course, students can now get a confidant to write a basic program to in-depth algorithms in C Programming or Java Programming to understand the basics one must visit the list 500 Java programs to get an idea.
Users can now download the top 100 Basic Java programming examples in a pdf format to practice.
But the platform dependency of the JVM is not considered while saying Java is platform independent because JVM is supplied free of cost through the internet by the sun microsystems.
Platform independence :
Compiled code of a program should be executed in any operating system, irrespective of the as in OS in which that code had been generated. This concept is known as platform independence.
- The birth of oops concept took place with encapsulation.
- Any program contains two parts.
- Date part and Logic part
- Out of data and logic the highest priority we have given to data.
- But in a structured programming language, the data insecurity is high.
- Thus in a process, if securing data in structured prog. lang. the concept of encapsulation came into existence.
Note: In structured programming lang programs, the global variable play a vital role.
But because of these global variables, there is data insecurity in the structured programming lang programs. i.e functions that are not related to some variables will have access to those variables and thus data may get corrupted. In this way data is unsecured.
“This is what people say in general about data insecurity. But this is not the actual reason. The actual concept is as follows”.
Let us assume that, we have a ‘C’ program with a hundred functions. Assume that it is a project. Now if any upgradation is required, then the client i.e the user of this program (s/w) comes to its company and asks the programmers to update it according to his requirement.
Now we should note that it is not guaranteed that the programmers who developed this program will still be working with that company. Hence this project falls into the hands of new programmers.
Automatically it takes a lot of time to study. The project itself before upgrading it. It may not be surprising that the time required for writing the code to upgrade the project may be very less when compared to the time required for studying the project.
Thus maintenance becomes a problem.
If the new programmer adds a new function to the existing code in the way of upgrading it, there is no guarantee that it will not affect the existing functions in the code. This is because of global variables. In this way, data insecurity is created.
- To overcome this problem, programmers developed the concept of encapsulation .
- For example, let us have a struc.prog.lang. program with ten global variables and twenty functions.
- It is sure that all the twenty functions will not use all the global variables .
Three of the global variables may be used only by two functions. But in a structured prog. Lang like ‘C’ it is not possible to restrict the access of global variables by some limited no of functions.
Every function will have access to all the global variables.
To avoid this problem, programmers have designed a way such that the variables and the functions which are associated with or operate on those variables are enclosed in a block and that bock is called a class and that class and that class is given a name, Just as a function is given a name.
Now the variables inside the block cannot be called as the local variable because they cannot be called as global variables because they are confined to a block and not global.
Hence these variables are known as instance variables
_______________________________________________________________
Example 2 :
Therefore a class is nothing but grouping data along with its functionalities.
Note 1: E ncapsulation it’s the concept of binding data along with its corresponding functionalities.
Encapsulations came into existence in order to provide security for the data present inside the program.
Note 2: Any object oriental programming language file looks like a group of classes. Everything is encapsulated. Nothing is outside the class.
- Encapsulation is the backbone of oop languages.
- JAVA supports all the oop concepts ( i.e. encapsulation, polymorphism, inheritance) and hence it is known as an object-oriented programming language.
- C++ breaks the concept of encapsulation because the main ( ) method in a C++ program is declared outside a class. Hence it is not a pure oop language, in fact, it is a poor oop language.
Related Posts !
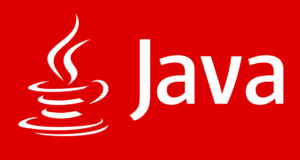
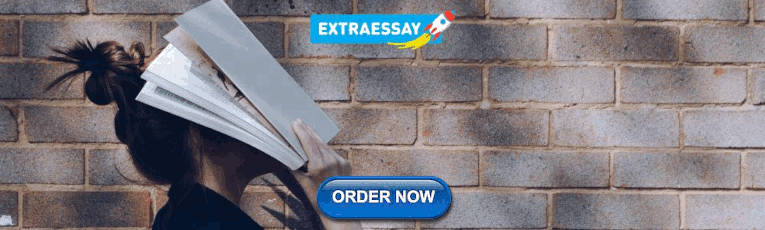
Java Program To Calculate Perimeter Of Rhombus | 3 Ways
April 10, 2024
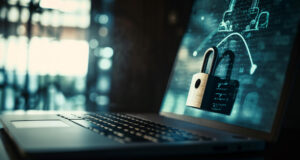
VPN Blocked by Java Security on PC? Here’s How to Fix That
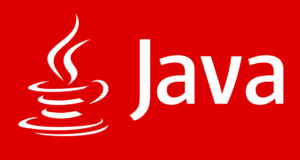
HCF Of Two & N Numbers Java Program | 3 Ways
April 7, 2024
LCM Of Two Numbers Java Program | 5 Ways – Programs
April 4, 2024
Java Program Convert Fahrenheit To Celsius | Vice Versa
April 1, 2024
Java Program Count Vowels In A String | Programs
March 29, 2024
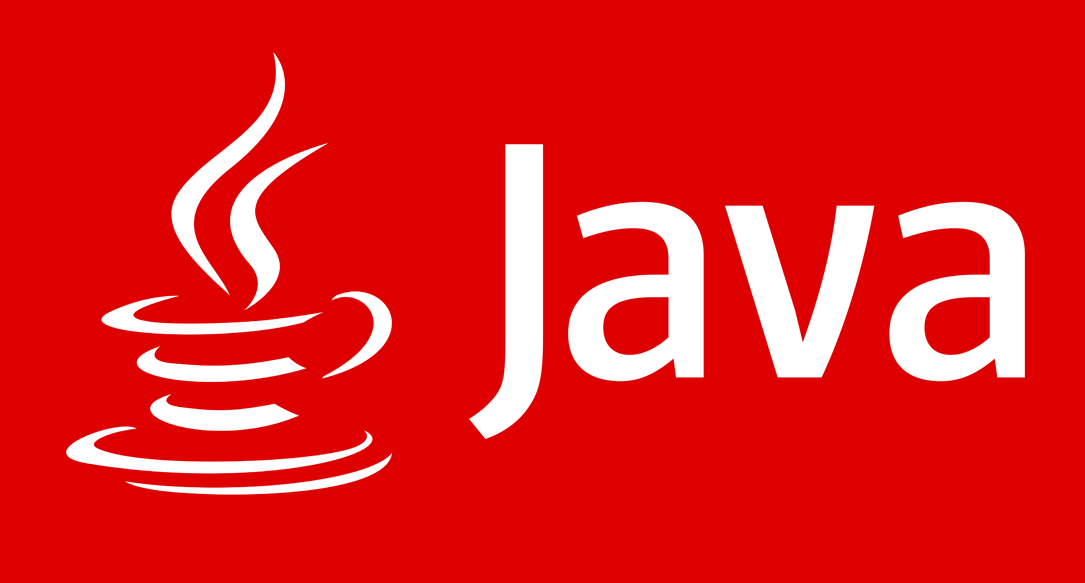
X Star Pattern Java Program – Patterns
Java program to print X star pattern program – We have written the below print/draw ...
Java Solved Programs, Problems with Solutions – Java programming
Java solved programs problems with solutions.
Java Solved programs —-> Java is a powerful general-purpose programming language. It is fast, portable and available in all platforms.
This page contains the Java solved programs/examples with solutions, here we are providing most important programs on each topic . These Java examples cover a wide range of programming areas in Computer Science.
Every example program includes the description of the program, Java code as well as output of the program. All examples are compiled and tested on a Windows system.
These examples can be as simple and basic as “Hello World” program to extremely tough and advanced Java programs. Here is the List of Java solved programs/examples with solutions (category wise) and detailed explanation.
Java Solved Programs by categories…….
Java basic solved programs.
- Java Program to Print Hello World on Screen
- Java program to Swap two numbers using third variable
- Java Program to find Addition of two numbers by input value from keyboard
- Java Program to Swap two numbers without using third variable
- Java Program Swap Two Numbers using function
- Java Program to Check entered input is Prime Number or Not
- Java Program to Check input number is Even or Odd
- Java Program to find Factorial using While loop
- Java Program to find Reverse Number using While Loop
- Java Program to display Fibonacci Series using While Loop
- Java Program to find GCD (HCF) of Two Numbers
- Java Program to Check whether Given Number is Armstrong or Not
- Java Program to find Largest among three Numbers
- Java Program to find given number is Palindrome or Not
- Java Program to Find Sum of Digits of given Number
- Java Program to display n Prime Numbers using Exception Handling
- Java Program to print Armstrong numbers between desired Range
- Java Program to Print Floyd’s Triangle using For Loop
- Java Program to Print Pascal Triangle using Recursion
- Java Program to Perform Mathematical Operations
- Java Program to Make a Simple Calculator using switch case
- Java Program to Calculate Arithmetic Mean of N numbers
- Java program to convert Fahrenheit to Celsius
- Java Program to Convert Celsius to Fahrenheit
- Java Menu Driven Program for If, Switch, While, Do-While and For Loop
- Java program to multiply N numbers without using * operator
Java Number Solved Programs
- Java Program to Print Pascal Triangle using For Loop
- Java program to generate random numbers using Random()
- Java Program to Find GCD(HCF) and LCM of Two Numbers
- Java program to find Reverse of a number
- Java program to find roots of quadratic equation in all cases
Java String Solved Programs
- Java Program to Print Simple String entered by user
- Java Program to Find Length of String using length() method
- Java Program to Compare Two Strings using compareTo() Function
- Java program to find and display all substrings in string
- Java Program to reverse any String
- Java Program to Copy String into Another String
- Java Program to Append(Concatenate) one string to another string
- Java Program to Swap Strings using temp variable
- Java Program to Swap two strings without using temp variable
- Java Program to Delete or Remove Vowels from string (Manual Method)
- Java Program to Sort n Strings in Alphabetical Order
- Java Program to Remove or Delete Vowels from String using Function
- Java Program to Remove or Delete Words from String using Function
- Java Program to Delete or Remove Words from String without Function
- Java Program to Check whether string is palindrome or not using Function
- Java Program to Check String is palindrome or not using String Buffer
- Java Program to Check String is Palindrome or not using Recursion
- Java Program to find Lexicographically smallest and largest substring of length k
- Java Program to Count frequency or occurrence of all characters in string
- Java Program to Count frequency of each characters using Buffered Reader
- Java Program to Count Frequency of Character in String ( 4 Methods )
- Java Program to Count Frequency or Occurrance of each Word in String
Java Arrays Solved Programs
- Java Program for Binary Search on Unsorted Array
- Java Program to input and print n elements in an array
- Java Program for Addition of Two Matrices
- Java Program for Subtraction of Two Matrices
- Java Program to find Transpose Matrix
- Java Program for Multiplication of Two Matrices
- Java Program for Linear Search on unsorted array
- Java Program to Perform Bubble Sort
- Java Program to perform Selection Sort using static function
- Java Program to perform Insertion Sort using function
- Java Program to perform Quick Sort using Static Function
If you found any error or any queries related to the above programs or any questions or reviews , you wanna to ask from us ,you may Contact Us through our contact Page or you can also comment below in the comment section.We will try our best to reach up to you in short interval.
Thanks for reading the post….
No related posts.
nice web but plz add creating account
please solve the qustion
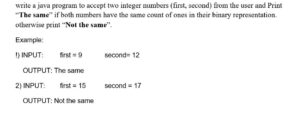
Create a menu-based java program for the following queues operations using linked list :
- Display/Print
Create a menu-based java program for the following queues operations using array:
Design a flowchart of the java program that accepts 3 integer values and display the lowest and the highest given value.
hello i need help
Write a program to input two arrays of size ‘m’ each. Find the sum of the elements of the two arrays in the same index and store it in the 3rd array in reverse order.
wtf………….
Create the class such that it reset the value of the object it is used to null its after uses in all cases using in finally statement in java exception handling
Enter a four-digit number. Break it down into numbers. Compose a new number in which from the original number all even digits less than 8 are increased by 2, and 8 is replaced by 0(java program)
Write a program that reads in from the user a character (ch), an integer (n) and another character (operator). This operator is an indication of the operation applied to (ch) and (n) entered as follows: – When (operator) character entered is ‘p’ or ‘P’, your program displays the character that comes after (ch) entered in (n) positions. – When (operator) character entered is ‘s’ or ‘S’, your program displays the character that precedes (ch) entered with (n) positions. – When (operator) character entered is ‘m’ or ‘M’, your program displays the character resulting of the multiplication of the value of … Read more »
anyone know how to solve that question
implement a multithreaded UDP server and client in java using JDK open source library 1 Client will send a number to server on tcp packet(continuous stream) 2.Server will acknowledge the request with sending back the same number(echo) 3.Client will maintain a data structure which stores un acknowledged numbers from server
class A { int a; String b; boolean c; A()//default { a=100; b=”pawan”; c=true; } void Disp() { System.out.print(a+” “+b+” “+c); } } class B { public static void main(String []arga){ A r=new A(); r.Disp(); } }
public class TicketCost ( public static void main(String[] args) ( I Declare variables int age; double cost; String msge;
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Java Hello World
- Java JVM, JRE and JDK
- Java Variables and Literals
- Java Data Types
- Java Operators
- Java Input and Output
- Java Expressions & Blocks
- Java Comment
Java Flow Control
- Java if...else
- Java switch Statement
- Java for Loop
- Java for-each Loop
- Java while Loop
- Java break Statement
- Java continue Statement
- Java Arrays
- Multidimensional Array
- Java Copy Array
Java OOP (I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructor
- Java Strings
- Java Access Modifiers
- Java this keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP (II)
- Java Inheritance
- Java Method Overriding
- Java super Keyword
- Abstract Class & Method
- Java Interfaces
- Java Polymorphism
- Java Encapsulation
Java OOP (III)
- Nested & Inner Class
- Java Static Class
- Java Anonymous Class
- Java Singleton
- Java enum Class
Java enum Constructor
- Java enum String
Java Reflection
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java List Interface
- Java ArrayList
- Java Vector
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue Interface
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet
- Java EnumSet
- Java LinkedhashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator
- Java ListIterator
- Java I/O Streams
- Java InputStream
- Java OutputStream
- Java FileInputStream
- Java FileOutputStream
- Java ByteArrayInputStream
- Java ByteArrayOutputStream
- Java ObjectInputStream
- Java ObjectOutputStream
- Java BufferedInputStream
- Java BufferedOutputStream
- Java PrintStream
Java Reader/Writer
- Java Reader
- Java Writer
- Java InputStreamReader
- Java OutputStreamWriter
- Java FileReader
- Java FileWriter
- Java BufferedReader
- Java BufferedWriter
- Java StringReader
- Java StringWriter
- Java PrintWriter
Additional Topics
- Java Scanner Class
- Java Type Casting
- Java autoboxing and unboxing
- Java Lambda Expression
- Java Generics
- Java File Class
- Java Wrapper Class
- Java Command Line Arguments
Java Tutorials
Java this Keyword
- Java Singleton Class
- Java Abstract Class and Abstract Methods
Java Constructors
A constructor in Java is similar to a method that is invoked when an object of the class is created.
Unlike Java methods , a constructor has the same name as that of the class and does not have any return type. For example,
Here, Test() is a constructor. It has the same name as that of the class and doesn't have a return type.
- Example: Java Constructor
In the above example, we have created a constructor named Main() .
Inside the constructor, we are initializing the value of the name variable .
Notice the statement creating an object of the Main class.
Here, when the object is created, the Main() constructor is called. And the value of the name variable is initialized.
Hence, the program prints the value of the name variables as Programiz .
Types of Constructor
In Java, constructors can be divided into three types:
- No-Arg Constructor
- Parameterized Constructor
- Default Constructor
1. Java No-Arg Constructors
Similar to methods, a Java constructor may or may not have any parameters (arguments).
If a constructor does not accept any parameters, it is known as a no-argument constructor. For example,
Example: Java Private No-arg Constructor
In the above example, we have created a constructor Main() .
Here, the constructor does not accept any parameters. Hence, it is known as a no-arg constructor.
Notice that we have declared the constructor as private.
Once a constructor is declared private , it cannot be accessed from outside the class.
So, creating objects from outside the class is prohibited using the private constructor.
Here, we are creating the object inside the same class.
Hence, the program is able to access the constructor. To learn more, visit Java Implement Private Constructor .
However, if we want to create objects outside the class, then we need to declare the constructor as public .
Example: Java Public no-arg Constructors
2. java parameterized constructor.
A Java constructor can also accept one or more parameters. Such constructors are known as parameterized constructors (constructors with parameters).
Example: Parameterized Constructor
Here, the constructor takes a single parameter. Notice the expression:
Here, we are passing the single value to the constructor.
Based on the argument passed, the language variable is initialized inside the constructor.
3. Java Default Constructor
If we do not create any constructor, the Java compiler automatically creates a no-arg constructor during the execution of the program.
This constructor is called the default constructor.
Example: Default Constructor
Here, we haven't created any constructors.
Hence, the Java compiler automatically creates the default constructor.
The default constructor initializes any uninitialized instance variables with default values.
To learn more, visit Java Data Types .
In the above program, the variables a and b are initialized with default value 0 and false respectively.
The above program is equivalent to:
- Important Notes on Java Constructors
- Constructors are invoked implicitly when you instantiate objects.
- The two rules for creating a constructor are: 1. The name of the constructor should be the same as the class. 2. A Java constructor must not have a return type.
- If a class doesn't have a constructor, the Java compiler automatically creates a default constructor during run-time. The default constructor initializes instance variables with default values. For example, the int variable will be initialized to 0
- Constructor types: No-Arg Constructor - a constructor that does not accept any arguments Parameterized constructor - a constructor that accepts arguments Default Constructor - a constructor that is automatically created by the Java compiler if it is not explicitly defined.
- A constructor cannot be abstract or static or final .
- A constructor can be overloaded but can not be overridden.
- Constructors Overloading in Java
Similar to Java method overloading , we can also create two or more constructors with different parameters. This is called constructor overloading.
Example: Java Constructor Overloading
In the above example, we have two constructors: Main() and Main(String language) .
Here, both the constructors initialize the value of the variable language with different values.
Based on the parameter passed during object creation, different constructors are called, and different values are assigned.
It is also possible to call one constructor from another constructor. To learn more, visit Java Call One Constructor from Another .
Note : We have used this keyword to specify the variable of the class. To know more about this keyword, visit Java this keyword .
- Enum Constructor
- Constructor Call
- Private Construction Implementation
- Java Access Modifier
Table of Contents
- Introduction
- Java No-Arg Constructors
- Java Parameterized Constructor
- Java Default Constructor
Sorry about that.
Related Tutorials
Java Tutorial
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples.
Java is a popular programming language.
Java is used to develop mobile apps, web apps, desktop apps, games and much more.
Examples in Each Chapter
Our "Try it Yourself" editor makes it easy to learn Java. You can edit Java code and view the result in your browser.
Try it Yourself »
Click on the "Run example" button to see how it works.
We recommend reading this tutorial, in the sequence listed in the left menu.
Java is an object oriented language and some concepts may be new. Take breaks when needed, and go over the examples as many times as needed.
Java Exercises
Test yourself with exercises.
Insert the missing part of the code below to output "Hello World".
Start the Exercise
Advertisement
Test your Java skills with a quiz.
Start Java Quiz
Learn by Examples
Learn by examples! This tutorial supplements all explanations with clarifying examples.
See All Java Examples
My Learning
Track your progress with the free "My Learning" program here at W3Schools.
Log in to your account, and start earning points!
This is an optional feature. You can study at W3Schools without using My Learning.

Java Keywords
Java String Methods
Java Math Methods
Download Java
Download Java from the official Java web site: https://www.oracle.com
Java Exam - Get Your Diploma!
Kickstart your career.
Get certified by completing the course

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
- Corporate Presentation
- Java Tutors
- Python Tutors
- Assembly Tutors
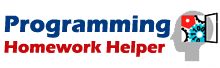
Java Homework Samples
Students pursuing a course in programming can download our Java homework samples right here for free. These samples have been done by experienced and knowledgeable Java experts who are associated with us. They are intended to boost your knowledge and improve your coding skills in Java. You will be surprised by the quality and accuracy of the samples. We can guarantee that you will be enjoying your Java classes after using our samples for revision and practice.
Write simple java and command line to compile and run
Todo list program with multiple priorities, simple animation, quiz program with multiple choice, single word and true or false..., quarterly sales report, polygon class, peer 2 peer networking and webservice, local sequence alignment, interactive crime statistics, implement multiset, implement a shopping list program, draw graphics in text mode, draw character that bounces around screen, dna processing, class to model headphones and junit test, check if graph is a subgraph, binary search tree, ball dodging game, ascii art drawing program, another to do list implementation, another ascii art drawing program, add junit tests, tokenize file, simulate virtual memory, rsa cryptography problems, quiz tester, program to process text in a file, performance testing of trees, multiple recursive functions, multi threaded queues, mini reversi game, knapsack problem, javafx to create bar graph, java estate agent database with swing gui, count the number of characters in a string, calculate statistics for series of values, assembly programming assignment help, database programming assignment help, sql programming assignment help, c programming assignment help, java programming assignment help, scala homework help, cpp programming assignment help, python programming assignment help, prolog homework help.
7 Best Java Homework Help Websites: How to Choose Your Perfect Match?
J ava programming is not a field that could be comprehended that easily; thus, it is no surprise that young learners are in search of programming experts to get help with Java homework and handle their assignments. But how to choose the best alternative when the number of proposals is enormous?
In this article, we are going to talk about the top ‘do my Java assignment’ services that offer Java assignment assistance and dwell upon their features. In the end, based on the results, you will be able to choose the one that meets your demands to the fullest and answer your needs. Here is the list of services that are available today, as well as those that are on everyone's lips:
TOP Java Assignment Help Services: What Makes Them Special?
No need to say that every person is an individual and the thing that suits a particular person could not meet the requirements of another. So, how can we name the best Java assignment help services on the web? - We have collected the top issues students face when searching for Java homework help and found the companies that promise to meet these requirements.
What are these issues, though?
- Pricing . Students are always pressed for budget, and finding services that suit their pockets is vital. Thus, we tried to provide services that are relatively affordable on the market. Of course, professional services can’t be offered too cheaply, so we have chosen the ones that balance professionalism and affordability.
- Programming languages . Not all companies have experts in all possible programming languages. Thus, we tried to choose the ones that offer as many different languages as possible.
- Expert staff . In most cases, students come to a company when they need to place their ‘do my Java homework’ orders ASAP. Thus, a large expert staff is a real benefit for young learners. They want to come to a service, place their order and get a professional to start working on their project in no time.
- Reviews . Of course, everyone wants to get professional help with Java homework from a reputable company that has already completed hundreds of Java assignments for their clients. Thus, we have mentioned only those companies that have earned enough positive feedback from their clients.
- Deadline options. Flexible deadline options are also a benefit for those who are placing their last-minute Java homework help assignments. Well, we also provide services with the most extended deadlines for those who want to save some money and place their projects beforehand.
- Guarantees . This is the must-feature if you want to get quality assistance and stay assured you are totally safe with the company you have chosen. In our list, we have only named companies that provide client-oriented guarantees and always keep their word, as well as offer only professional Java assignment experts.
- Customization . Every service from the list offers Java assistance tailored to clients’ personal needs. There, you won’t find companies that offer pre-completed projects and sell them at half-price.
So, let’s have a closer look at each option so you can choose the one that totally meets your needs.
DoMyAssignments.com
At company service, you can get assistance with academic writing as well as STEM projects. The languages you can get help with are C#, C++, Computer science, Java, Javascript, HTML, PHP, Python, Ruby, and SQL.
The company’s prices start at $30/page for a project that needs to be done in 14+ days.
Guarantees and extra services
The company offers a list of guarantees to make your cooperation as comfortable as possible. So, what can you expect from the service?
- Free revisions . When you get your order, you can ask your expert for revisions if needed. It means that if you see that any of your demands were missed, you can get revisions absolutely for free.
- Money-back guarantee. The company offers professional help, and they are sure about their experts and the quality of their assistance. Still, if you receive a project that does not meet your needs, you can ask for a full refund.
- Confidentiality guarantee . Stay assured that all your personal information is safe and secure, as the company scripts all the information you share with them.
- 100% customized assistance . At this service, you won’t find pre-written codes, all the projects are completed from scratch.
Expert staff
If you want to hire one of the top Java homework experts at DoMyAssignments , you can have a look at their profile, see the latest orders they have completed, and make sure they are the best match for your needs. Also, you can have a look at the samples presented on their website and see how professional their experts are. If you want to hire a professional who completed a particular sample project, you can also turn to a support team and ask if you can fire this expert.
CodingHomeworkHelp.org
CodingHomeworkHelp is rated at 9.61/10 and has 10+ years of experience in the programming assisting field. Here, you can get help with the following coding assignments: MatLab, Computer Science, Java, HTML, C++, Python, R Studio, PHP, JavaScript, and C#.
Free options all clients get
Ordering your project with CodingHomeworkHelp.org, you are to enjoy some other options that will definitely satisfy you.
- Partial payments . If you order a large project, you can pay for it in two parts. Order the first one, get it done, and only then pay for the second one.
- Revisions . As soon as you get your order, you can ask for endless revisions unless your project meets your initial requirements.
- Chat with your expert . When you place your order, you get an opportunity to chat directly with your coding helper. If you have any questions or demands, there is no need to first contact the support team and ask them to contact you to your assistant.
- Code comments . If you have questions concerning your code, you can ask your helper to provide you with the comments that will help you better understand it and be ready to discuss your project with your professor.
The prices start at $20/page if you set a 10+ days deadline. But, with CodingHomeworkHelp.org, you can get a special discount; you can take 20% off your project when registering on the website. That is a really beneficial option that everyone can use.
CWAssignments.com
CWAssignments.com is an assignment helper where you can get professional help with programming and calculations starting at $30/page. Moreover, you can get 20% off your first order.
Working with the company, you are in the right hands and can stay assured that the final draft will definitely be tailored to your needs. How do CWAssignments guarantee their proficiency?
- Money-back guarantee . If you are not satisfied with the final work, if it does not meet your expectations, you can request a refund.
- Privacy policy . The service collects only the data essential to complete your order to make your cooperation effective and legal.
- Security payment system . All the transactions are safe and encrypted to make your personal information secure.
- No AI-generated content . The company does not use any AI tools to complete their orders. When you get your order, you can even ask for the AI detection report to see that your assignment is pure.
With CWAssignments , you can regulate the final cost of your project. As it was mentioned earlier, the prices start at $30/page, but if you set a long-term deadline or ask for help with a Java assignment or with a part of your task, you can save a tidy sum.
DoMyCoding.com
This company has been offering its services on the market for 18+ years and provides assistance with 30+ programming languages, among which are Python, Java, C / C++ / C#, JavaScript, HTML, SQL, etc. Moreover, here, you can get assistance not only with programming but also with calculations.
Pricing and deadlines
With DoMyCoding , you can get help with Java assignments in 8 hours, and their prices start at $30/page with a 14-day deadline.
Guarantees and extra benefits
The service offers a number of guarantees that protect you from getting assistance that does not meet your requirements. Among the guarantees, you can find:
- The money-back guarantee . If your order does not meet your requirements, you will get a full refund of your order.
- Free edits within 7 days . After you get your project, you can request any changes within the 7-day term.
- Payments in parts . If you have a large order, you can pay for it in installments. In this case, you get a part of your order, check if it suits your needs, and then pay for the other part.
- 24/7 support . The service operates 24/7 to answer your questions as well as start working on your projects. Do not hesitate to use this option if you need to place an ASAP order.
- Confidentiality guarantee . The company uses the most secure means to get your payments and protects the personal information you share on the website to the fullest.
More benefits
Here, we also want to pay your attention to the ‘Samples’ section on the website. If you are wondering if a company can handle your assignment or you simply want to make sure they are professionals, have a look at their samples and get answers to your questions.
AssignCode.com
AssignCode is one of the best Java assignment help services that you can entrust with programming, mathematics, biology, engineering, physics, and chemistry. A large professional staff makes this service available to everyone who needs help with one of these disciplines. As with some of the previous companies, AssignCode.com has reviews on different platforms (Reviews.io and Sitejabber) that can help you make your choice.
As with all the reputed services, AssignCode offers guarantees that make their cooperation with clients trustworthy and comfortable. Thus, the company guarantees your satisfaction, confidentiality, client-oriented attitude, and authenticity.
Special offers
Although the company does not offer special prices on an ongoing basis, regular clients can benefit from coupons the service sends them via email. Thus, if you have already worked with the company, make sure to check your email before placing a new one; maybe you have received a special offer that will help you save some cash.
AssignmentShark.com
Reviews about this company you can see on different platforms. Among them are Reviews.io (4.9 out of 5), Sitejabber (4.5 points), and, of course, their own website (9.6 out of 10). The rate of the website speaks for itself.
Pricing
When you place your ‘do my Java homework’ request with AssignmentShark , you are to pay $20/page for the project that needs to be done in at least ten days. Of course, if the due date is closer, the cost will differ. All the prices are presented on the website so that you can come, input all the needed information, and get an approximate calculation.
Professional staff
On the ‘Our experts’ page, you can see the full list of experts. Or, you can use filters to see the professional in the required field.
The company has a quick form on its website for those who want to join their professional staff, which means that they are always in search of new experts to make sure they can provide clients with assistance as soon as the need arises.
Moreover, if one wants to make sure the company offers professional assistance, one can have a look at the latest orders and see how experts provide solutions to clients’ orders.
What do clients get?
Placing orders with the company, one gets a list of inclusive services:
- Free revisions. You can ask for endless revisions until your order fully meets your demands.
- Code comments . Ask your professional to provide comments on the codes in order to understand your project perfectly.
- Source files . If you need the list of references and source files your helper turned to, just ask them to add these to the project.
- Chat with the professional. All the issues can be solved directly with your coding assistant.
- Payment in parts. Large projects can be paid for in parts. When placing your order, let your manager know that you want to pay in parts.
ProgrammingDoer.com
ProgrammingDoer is one more service that offers Java programming help to young learners and has earned a good reputation among previous clients.
The company cherishes its reputation and does its best to let everyone know about their proficiency. Thus, you, as a client, can read what people think about the company on several platforms - on their website as well as at Reviews.io.
What do you get with the company?
Let’s have a look at the list of services the company offers in order to make your cooperation with them as comfortable as possible.
- Free revisions . If you have any comments concerning the final draft, you can ask your professional to revise it for free as many times as needed unless it meets your requirements to the fullest.
- 24/7 assistance . No matter when you realize that you have a programming assignment that should be done in a few days. With ProgrammingDoer, you can place your order 24/7 and get a professional helper as soon as there is an available one.
- Chat with the experts . When you place your order with the company, you get an opportunity to communicate with your coding helper directly to solve all the problems ASAP.
Extra benefits
If you are not sure if the company can handle your assignment the right way, if they have already worked on similar tasks, or if they have an expert in the needed field, you can check this information on your own. First, you can browse the latest orders and see if there is something close to the issue you have. Then, you can have a look at experts’ profiles and see if there is anyone capable of solving similar issues.
Can I hire someone to do my Java homework?
If you are not sure about your Java programming skills, you can always ask a professional coder to help you out. All you need is to find the service that meets your expectations and place your ‘do my Java assignment’ order with them.
What is the typical turnaround time for completing a Java homework assignment?
It depends on the service that offers such assistance as well as on your requirements. Some companies can deliver your project in a few hours, but some may need more time. But, you should mind that fast delivery is more likely to cost you some extra money.
What is the average pricing structure for Java assignment help?
The cost of the help with Java homework basically depends on the following factors: the deadline you set, the complexity level of the assignment, the expert you choose, and the requirements you provide.
How will we communicate and collaborate on my Java homework?
Nowadays, Java assignment help companies provide several ways of communication. In most cases, you can contact your expert via live chat on a company’s website, via email, or a messenger. To see the options, just visit the chosen company’s website and see what they offer.
Regarding the Author:
Nayeli Ellen, a dynamic editor at AcademicHelp, combines her zeal for writing with keen analytical skills. In her comprehensive review titled " Programming Assignment Help: 41 Coding Homework Help Websites ," Nayeli offers an in-depth analysis of numerous online coding homework assistance platforms.
CSC 347 - Concepts of Programming Languages
Scala pragmatics.
Instructor: Stefan Mitsch
Learning Objectives
- Set up a Scala development environment
Java and Scala
- Unzip the Scala homework workspace hw-scala-3.4.1.zip
- Follow the instructions in README.html
- If you have Java installed, check Scala JDK Compatibility
- Install Java LTS (long term support) v8, v11, v17, or v21 or OpenJDK/AdoptOpenJDK (e.g., via homebrew ) v8, v11, v17, or v21
- Install SBT using the windows installer or homebrew
Using Scala
- For real programs and homeworks, use sbt to run tests
- File may only contain object and class declarations object o { val x = ... } class c { ... }
- You can use console to get a REPL within sbt, use :quit to exit the REPL
- In the sbt REPL, you can use import objects import o.* // use x
- For tiny examples, type directly into the REPL val x = ... // use x
- For larger examples, type in a file and :load into the REPL :load x.sc // use x
- File contains declarations just as you would type them in the REPL
- If there are expressions, then the last one is printed out as a value in the REPL
- Do not put snippet files ending in .scala in the SBT directory; SBT expects object and class declarations and will report a compile error
Homework Assignments
- Make sure you are in the right directory : run dir (Windows) or ls (Linux, MacOS) and check that the file build.sbt is listed
- Compile the homework assignments: inside SBT compile or from command line sbt compile
- Run all unit tests: inside SBT test or from command line sbt test
- Run the tests of a single homework assignment: inside SBT testOnly fp1tests or from command line sbt "testOnly fp1tests"
- Run the homework assignment tests whenever a file changes: inside SBT ~testOnly fp1tests
- Run a single test of a single homework assignment: inside SBT testOnly fp1tests -- -n fp1ex05
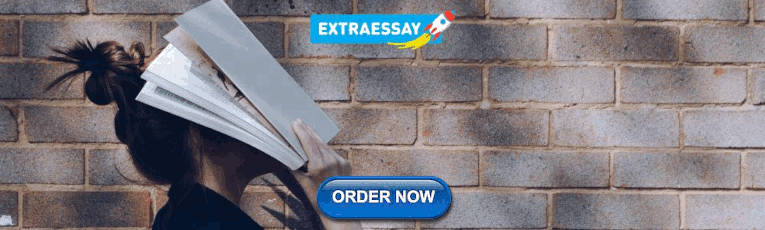
IMAGES
VIDEO
COMMENTS
That covers various Java Core Topics that can help users with Java Practice. Take a look at our Java Exercises to practice and develop your Java programming skills. Our Java programming exercises Practice Questions from all the major topics like loops, object-oriented programming, exception handling, and many more.
Here you have the opportunity to practice the Java programming language concepts by solving the exercises starting from basic to more complex exercises. A sample solution is provided for each exercise. It is recommended to do these exercises by yourself first before checking the solution. Hope, these exercises help you to improve your Java ...
Java Program to Reverse a Number. Java Program to Iterate through each characters of the string. Java Program to Remove elements from the LinkedList. Java Program to Access elements from a LinkedList. This page contains examples of basic concepts of Python programming like loops, functions, native datatypes and so on.
Single-line comments start with two forward slashes (//) and end at the end of the line. In example above we have a comment //here we print the text out. You can read the theory on this topic here, here, and here. But try practicing first! Explore the Java coding exercises for practicing with commands below. First, read the conditions, scroll ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Examples SumOfTwoNumbers(3, 2) 5 SumOfTwoNumbers(-3, -6) -9 SumOfTwoNumbers(7, 3) 10 Notes Don't forget to return the result. If you get stuck on a challenge, find help in the Resources tab. ... Practice Java coding with fun, bite-sized exercises. Earn XP, unlock achievements and level up. It's like Duolingo for learning to code.
Arrow Chevron Down Icon. Java is an open-source, general-purpose programming language known for its versatility and stability. It's used for everything from building websites to operating systems and wearable devices. You can even find Java in outer space, running the Mars rover.
See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases. The Java Tutorials are practical guides for programmers who want to use the Java programming language to create applications. They include hundreds of complete, working examples, and dozens of lessons.
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases.
Java Stdin and Stdout I. Easy Java (Basic) Max Score: 5 Success Rate: 96.88%. Solve Challenge. Java If-Else. Easy Java (Basic) Max Score: 10 Success Rate: 91.33%. Solve Challenge. Java Stdin and Stdout II. Easy Java (Basic) Max Score: 10 Success Rate: 92.62%. Solve Challenge. Java Output Formatting.
BouncingBox.java . DrawGraphics.java . 6 Graphics strikes back! assn06.zip (This ZIP file contains: 6 .java files.) 7 Magic squares Mercury.txt . Luna.txt . Solutions . Course Info Instructors Evan Jones; Adam Marcus; Eugene Wu; Departments Electrical Engineering and Computer Science ...
10 Java code challenges to practice your new skills. 1. Word reversal. For this challenge, the input is a string of words, and the output should be the words in reverse but with the letters in the original order. For example, the string "Dog bites man" should output as "man bites Dog.".
Java Hello World Program. A "Hello, World!" is a simple program that outputs Hello, World! on the screen. Since it's a very simple program, it's often used to introduce a new programming language to a newbie. Let's explore how Java "Hello, World!" program works. Note: You can use our online Java compiler to run Java programs.
Here, you will find all types of Java programs with examples. Fine-tune your skills with our comprehensive collection of Java example programs. Each program comes complete with code samples, outputs, and easy-to-understand explanations, making your learning experience both enjoyable and effective.
9) Quizzing app. One of the more subtle project to practice that Java allows one to build is apps. Google built Android to be built on top of the Java ecosystem, allowing all features of the Android OS to build an app. One of the best apps to get started is probably a quiz app for distributing questions to friends!
Java, With the help of this course, students can now get a confidant to write a basic program to in-depth algorithms in C Programming or Java Programming to understand the basics one must visit the list 500 Java programs to get an idea. Users can now download the top 100 Basic Java programming examples in a pdf format to practice.
IntroductiontoProgrammingUsingJava Version7.0,August2014 (Version 7.0.3, with just a few corrections, May 2018) DavidJ.Eck HobartandWilliamSmithColleges
Java Solved programs —-> Java is a powerful general-purpose programming language. It is fast, portable and available in all platforms. This page contains the Java solved programs/examples with solutions, here we are providing most important programs on each topic.These Java examples cover a wide range of programming areas in Computer Science.
In the above example, we have created a constructor named Main(). Here, the constructor takes a single parameter. Notice the expression: Main obj1 = new Main("Java"); Here, we are passing the single value to the constructor. Based on the argument passed, the language variable is initialized inside the constructor. 3.
System.out.println("Hello World"); Click on the "Run example" button to see how it works. We recommend reading this tutorial, in the sequence listed in the left menu. Java is an object oriented language and some concepts may be new. Take breaks when needed, and go over the examples as many times as needed.
Java Homework Samples. Students pursuing a course in programming can download our Java homework samples right here for free. These samples have been done by experienced and knowledgeable Java experts who are associated with us. They are intended to boost your knowledge and improve your coding skills in Java. You will be surprised by the quality ...
A subreddit for all questions related to programming in any language. I give you the best 200+ assignments I have ever created (Java) I'm a public school teacher who has taught the basics of programming to nearly 2,000 ordinary students over the past fifteen years. I have seen a lot of coding tutorials online, but most of them go too fast!
In this case, you get a part of your order, check if it suits your needs, and then pay for the other part. 24/7 support. The service operates 24/7 to answer your questions as well as start working ...
Java and Scala. Unzip the Scala homework workspace hw-scala-3.4.1.zip; Follow the instructions in README.html; If you have Java installed, check Scala JDK Compatibility; Install Java LTS (long term support) ... For larger examples, type in a file and :load into the REPL:load x.sc // use x