- Mailing List
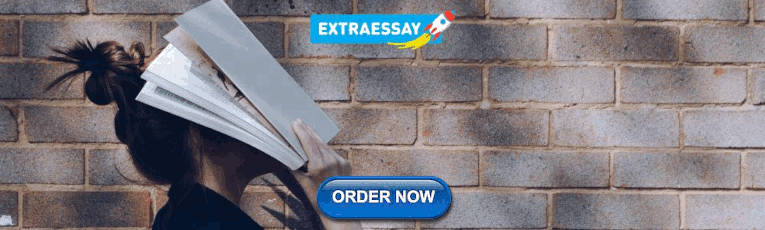
Practical Business Python
Taking care of business, one python script at a time
Creating Powerpoint Presentations with Python
Posted by Chris Moffitt in articles
Introduction
Love it or loathe it, PowerPoint is widely used in most business settings. This article will not debate the merits of PowerPoint but will show you how to use python to remove some of the drudgery of PowerPoint by automating the creation of PowerPoint slides using python.
Fortunately for us, there is an excellent python library for creating and updating PowerPoint files: python-pptx . The API is very well documented so it is pretty easy to use. The only tricky part is understanding the PowerPoint document structure including the various master layouts and elements. Once you understand the basics, it is relatively simple to automate the creation of your own PowerPoint slides. This article will walk through an example of reading in and analyzing some Excel data with pandas, creating tables and building a graph that can be embedded in a PowerPoint file.
PowerPoint File Basics
Python-pptx can create blank PowerPoint files but most people are going to prefer working with a predefined template that you can customize with your own content. Python-pptx’s API supports this process quite simply as long as you know a few things about your template.
Before diving into some code samples, there are two key components you need to understand: Slide Layouts and Placeholders . In the images below you can see an example of two different layouts as well as the template’s placeholders where you can populate your content.
In the image below, you can see that we are using Layout 0 and there is one placeholder on the slide at index 1.
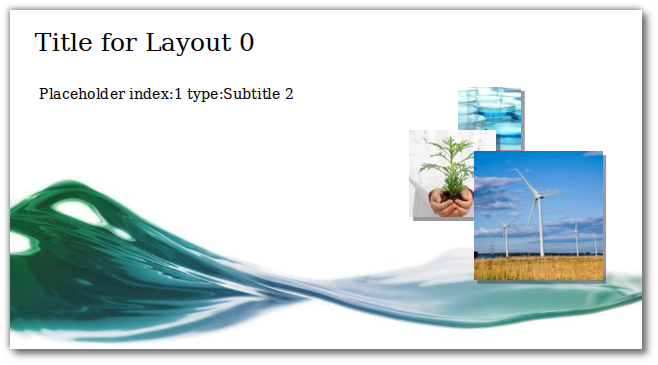
In this image, we use Layout 1 for a completely different look.
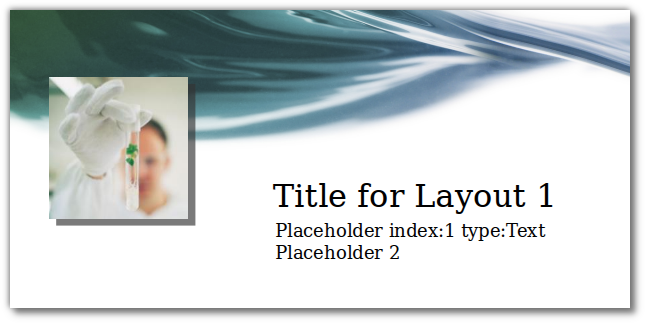
In order to make your life easier with your own templates, I created a simple standalone script that takes a template and marks it up with the various elements.
I won’t explain all the code line by line but you can see analyze_ppt.py on github. Here is the function that does the bulk of the work:
The basic flow of this function is to loop through and create an example of every layout included in the source PowerPoint file. Then on each slide, it will populate the title (if it exists). Finally, it will iterate through all of the placeholders included in the template and show the index of the placeholder as well as the type.
If you want to try it yourself:
Refer to the input and output files to see what you get.
Creating your own PowerPoint
For the dataset and analysis, I will be replicating the analysis in Generating Excel Reports from a Pandas Pivot Table . The article explains the pandas data manipulation in more detail so it will be helpful to make sure you are comfortable with it before going too much deeper into the code.
Let’s get things started with the inputs and basic shell of the program:
After we create our command line args, we read the source Excel file into a pandas DataFrame. Next, we use that DataFrame as an input to create the Pivot_table summary of the data:
Consult the Generating Excel Reports from a Pandas Pivot Table if this does not make sense to you.
The next piece of the analysis is creating a simple bar chart of sales performance by account:
Here is a scaled down version of the image:
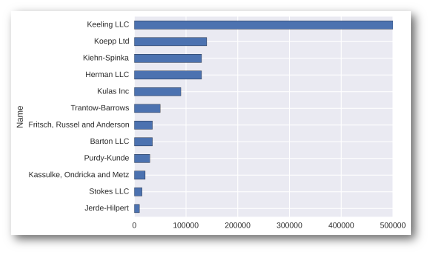
We have a chart and a pivot table completed. Now we are going to embed that information into a new PowerPoint file based on a given PowerPoint template file.
Before I go any farther, there are a couple of things to note. You need to know what layout you would like to use as well as where you want to populate your content. In looking at the output of analyze_ppt.py we know that the title slide is layout 0 and that it has a title attribute and a subtitle at placeholder 1.
Here is the start of the function that we use to create our output PowerPoint:
This code creates a new presentation based on our input file, adds a single slide and populates the title and subtitle on the slide. It looks like this:
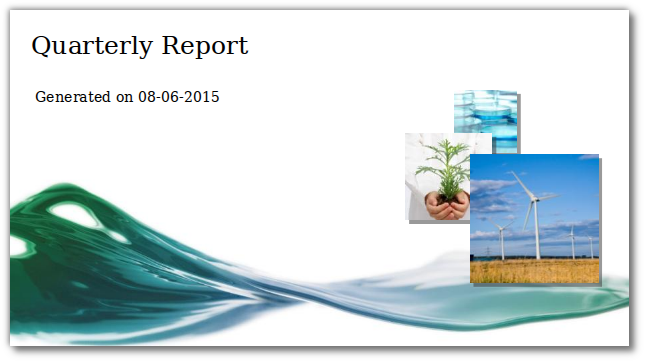
Pretty cool huh?
The next step is to embed our picture into a slide.
From our previous analysis, we know that the graph slide we want to use is layout index 8, so we create a new slide, add a title then add a picture into placeholder 1. The final step adds a subtitle at placeholder 2.
Here is our masterpiece:
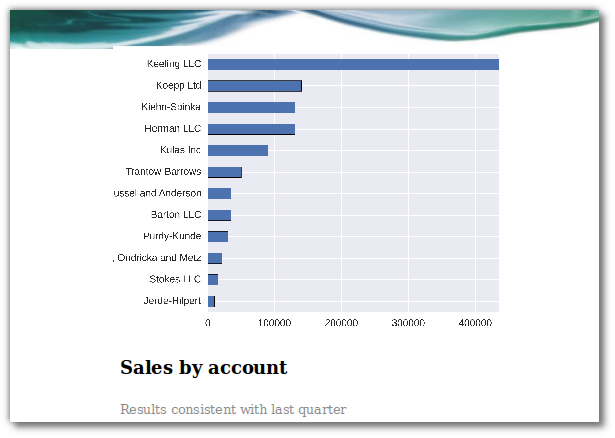
For the final portion of the presentation, we will create a table for each manager with their sales performance.
Here is an image of what we’re going to achieve:
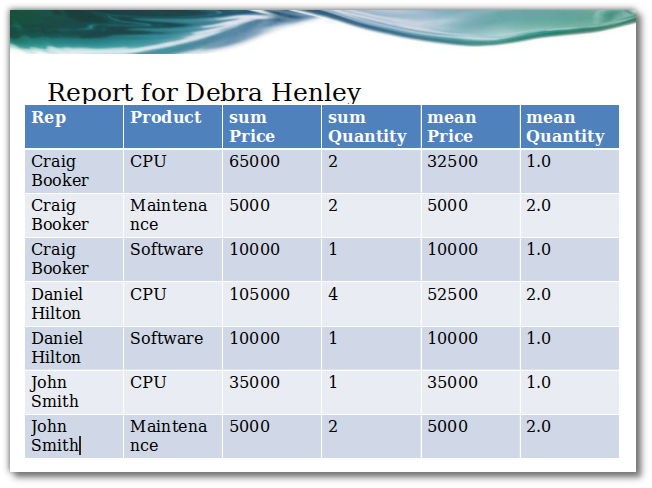
Creating tables in PowerPoint is a good news / bad news story. The good news is that there is an API to create one. The bad news is that you can’t easily convert a pandas DataFrame to a table using the built in API . However, we are very fortunate that someone has already done all the hard work for us and created PandasToPowerPoint .
This excellent piece of code takes a DataFrame and converts it to a PowerPoint compatible table. I have taken the liberty of including a portion of it in my script. The original has more functionality that I am not using so I encourage you to check out the repo and use it in your own code.
The code takes each manager out of the pivot table and builds a simple DataFrame that contains the summary data. Then uses the df_to_table to convert the DataFrame into a PowerPoint compatible table.
If you want to run this on your own, the full code would look something like this:
All of the relevant files are available in the github repository .
One of the things I really enjoy about using python to solve real world business problems is that I am frequently pleasantly surprised at the rich ecosystem of very well thought out python tools already available to help with my problems. In this specific case, PowerPoint is rarely a joy to use but it is a necessity in many environments.
After reading this article, you should know that there is some hope for you next time you are asked to create a bunch of reports in PowerPoint. Keep this article in mind and see if you can find a way to automate away some of the tedium!
- ← Best Practices for Managing Your Code Library
- Adding a Simple GUI to Your Pandas Script →
Subscribe to the mailing list
Submit a topic.
- Suggest a topic for a post
- Pandas Pivot Table Explained
- Common Excel Tasks Demonstrated in Pandas
- Overview of Python Visualization Tools
- Guide to Encoding Categorical Values in Python
- Overview of Pandas Data Types
Article Roadmap
We are a participant in the Amazon Services LLC Associates Program, an affiliate advertising program designed to provide a means for us to earn fees by linking to Amazon.com and affiliated sites.
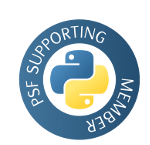
5 Best Ways to Create PowerPoint Files Using Python
💡 Problem Formulation: Automating the creation of PowerPoint presentations is a common task for those who need to generate reports or summaries regularly. For instance, a user may wish to create a presentation summarizing sales data from a CSV file or visualize a project’s progress in a structured format. The desired output is a fully formatted PowerPoint file (.pptx) with various elements like titles, texts, images, and charts, as specified by the input data or customization requirements.
Method 1: Using python-pptx
The python-pptx library provides a comprehensive set of features for creating PowerPoint files (.pptx) in Python. It allows for adding slides, text, images, charts, and more, with a high level of customization. Manipulate slides at a granular level by accessing placeholders, creating bulleted lists, and setting properties like font size or color programmatically.
Here’s an example:
The code snippet above creates a PowerPoint file named python-pptx-presentation.pptx with one slide that includes a title and a subtitle.
In this overview, we create a presentation object, add a new slide with a predefined layout, set text for the title and subtitle placeholders, and then save the presentation. This method gives users the ability to create detailed, professional presentations through code.
Method 2: Using Pandas with python-pptx
This method combines the data manipulation power of Pandas with the presentation capabilities of python-pptx to create PowerPoint files from DataFrame contents. It’s particularly useful for automating the inclusion of tabular data or creating charts based on the DataFrame’s data.
The output is a PowerPoint file named pandas-python-pptx.pptx containing a bar chart representing the quantity of fruits.
This snippet demonstrates using a Pandas DataFrame to generate chart data, which is then used to create a chart in a PowerPoint slide. It showcases the synergy between Pandas for data handling and python-pptx for presentation creation.
Method 3: Using ReportLab with python-pptx
Those seeking to include complex graphics or generate custom visuals can harness the graphic-drawing capabilities of ReportLab with python-pptx. This method leverages ReportLab to create an image, which can then be inserted into a PowerPoint slide.
The output would be a PowerPoint file named reportlab-pptx.pptx containing a slide with a custom bar chart image.
The code above creates a bar chart using ReportLab, saves the chart as an image, and then inserts the image into a PowerPoint slide. This approach is ideal if you need to include bespoke graphics that are not directly supported by python-pptx itself.
Method 4: Using Matplotlib with python-pptx
For those familiar with Matplotlib, this method involves creating a visual plot or chart with Matplotlib, saving it as an image, and then embedding the image into a PowerPoint slide using python-pptx.
The outcome is a PowerPoint file matplotlib-pptx.pptx , with a plot on a slide created by Matplotlib.
In this case, we graph a quadratic function using Matplotlib, save it as an image, and then add that image to a slide in our PowerPoint presentation. This method offers a blend of Matplotlib’s sophisticated plotting tools with the simplicity of python-pptx.
Bonus One-Liner Method 5: Using Officegen
The Officegen package allows for rapid PowerPoint creation with simpler syntax, although with less flexibility compared to python-pptx. It provides functions to add slides, titles, and bullet points.
The outcome is a PowerPoint file officegen-presentation.pptx with a single slide containing a large title.
This snippet uses Officegen to initiate a new presentation, adds a text title to a slide, and saves the presentation. While not as detailed as python-pptx, Officegen is quick for simple presentations.
Summary/Discussion
- Method 1: python-pptx. Full-featured control over presentations. Can be verbose for simple tasks.
- Method 2: Pandas with python-pptx. Ideal for data-driven presentations. Setup can be complex if unfamiliar with data libraries.
- Method 3: ReportLab with python-pptx. Powerful combo for custom graphics. Requires separate handling of graphics and presentation stages.
- Method 4: Matplotlib with python-pptx. Best for users comfortable with Matplotlib. Less direct than using python-pptx alone.
- Bonus Method 5: Officegen. Quick and easy for simple presentations. Limited customization options.
Emily Rosemary Collins is a tech enthusiast with a strong background in computer science, always staying up-to-date with the latest trends and innovations. Apart from her love for technology, Emily enjoys exploring the great outdoors, participating in local community events, and dedicating her free time to painting and photography. Her interests and passion for personal growth make her an engaging conversationalist and a reliable source of knowledge in the ever-evolving world of technology.
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Python | Generate QR Code using pyqrcode module
- Python | Launch a Web Browser using webbrowser module
- Memory profiling in Python using memory_profiler
- How to Clone webpage Using pywebcopy in Python?
- Python Script to create random jokes using pyjokes
- Introduction to Social Networks using NetworkX in Python
- Plotting Data on Google Map using Python's pygmaps package
- Perform addition and subtraction using CherryPy
- Port scanner using 'python-nmap'
- Pathlib module in Python
- Visual TimeTable using pdfschedule in Python
- Generating Word Cloud in Python | Set 2
- Conversion between binary, hexadecimal and decimal numbers using Coden module
- Draw a happy face using Arcade Library in Python
- Hiding and encrypting passwords in Python?
- Create temporary files and directories using tempfile
- Taking Screenshots using pyscreenshot in Python
- Send Message on Instagram Using Instabot module in Python
- Download Instagram Reel using Python
Creating and updating PowerPoint Presentations in Python using python – pptx
python-pptx is library used to create/edit a PowerPoint (.pptx) files. This won’t work on MS office 2003 and previous versions. We can add shapes, paragraphs, texts and slides and much more thing using this library.
Installation: Open the command prompt on your system and write given below command:
Let’s see some of its usage:
Example 1: Creating new PowerPoint file with title and subtitle slide.
Example 2: Adding Text-Box in PowerPoint.
Example 3: PowerPoint (.pptx) file to Text (.txt) file conversion.
Example 4: Inserting image into the PowerPoint file.
Example 5: Adding Charts to the PowerPoint file.
Example 6: Adding tables to the PowerPoint file.
Please Login to comment...
Similar reads.
- python-modules
- python-utility
- How to Organize Your Digital Files with Cloud Storage and Automation
- 10 Best Blender Alternatives for 3D Modeling in 2024
- How to Transfer Photos From iPhone to iPhone
- What are Tiktok AI Avatars?
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
A python library for creating PowerPoint slides with Plotly charts
jonboone1/plotlyPowerpoint
Folders and files, repository files navigation, plotly & powerpoint.
A library used to create powerpoint slides including plotly charts. Use this to automate powerpoint creation including certiain charts/visualizations.
Getting Started
Below is a quick tutorial taking you through how to setup and use this library. I will use public data to showcase how it works.
Step 1 - Install Dependencies
I have yet to figure out how to install required packages with the install of this library. Therefore, please ensure you have installed the following packages. For plotly, please refer to the getting started page to learn how to install everything properly.
- plotly.express
- plotly.graph_objects
- plotly.subplots
- scipy.stats
Additionally, if you want to be able to visualize plotly charts, you may have to install additonal requirements. Refer to the plotly getting started page and scroll down for your proper IDE (Jupyter Notebook, Lab, etc.).
Step 2 - Install Package
Step 3 - prepare your powerpoint.
The main function of this library is meant to generate slides which include a plotly chart. In order to do this, you must prepare a powerpoint template with the proper layout. You can design any layout you desire, but make sure that each element you desire to fill with python is created as a placeholder. You can use a powerpoint template I've included in my source code to make things easy (assets/powerpoint_templates/template.pptx).
In order to do this, open up your desired powerpoint and go into the slide master. Insert a new slide or use a current one already created. I recommend to drag this to be first in the order, this will make your life easier later down the road. Now insert the proper elements and arragne them as you please. For the chart, insert an image placeholder and make the apsect ratio similar to a laptop screen. Most images created are in landscape view but not too wide.

Step 4 - Load Library and Prepare Data
If you want to follow along with my tutorial, feel free and copy/paste my code. However, ensure you have pydataset installed before you do.
Step 5 - Find Your Powerpoint Elements
In order for this library to know where to place each element, you must give it each element index. See the following code to learn how to do this:
You will need this information later on.
Step 6 - Set Template
Now that we have our powerpoint template, we need to tell the library where to find this file. Do this with the following:
Step 7 - Define Charts
Here is where the work is done. Creating slides with charts is done by defining an array of dictionary objects. Each object represents one slide. Everything must be represented as a string except your dataframe object. Additionally, this library automatically groups every dataframe down to the level needed for the specific chart. Therefore, you don't have to create a new dataframe for each chart. As long as your df has the right data in it, this function will do the rest.
As a start, I will define two basic charts:
I will go into more detail on each one of these variables in a later section.
Step 8 - Run Function
This will output a file called output.pptx . I suggest you do not make this your final file, as each time you run this function you will overwrite the powerpoint. Create a copy of this file and start to create your analysis/report there. From here, you can use output.pptx as a slide library, where you can include or delete any chart you create.
- Jupyter Notebook 52.7%
- Python 47.3%
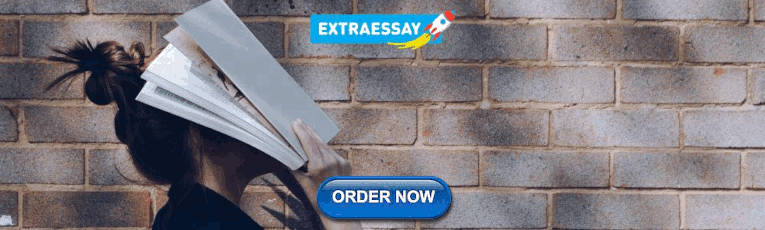
plotlyPowerpoint 1.2.17
pip install plotlyPowerpoint Copy PIP instructions
Released: Nov 22, 2022
A library using Plotly and Powerpoint to easily generate slides with plotly charts in them
Verified details
Maintainers.
Unverified details
Project links, github statistics.
- Open issues:
View statistics for this project via Libraries.io , or by using our public dataset on Google BigQuery
License: MIT License
Author: Jon Boone
Requires: Python >=3.6
Classifiers
- OSI Approved :: MIT License
- OS Independent
- Python :: 3
Project description
Plotly & powerpoint.
A library used to create powerpoint slides including plotly charts. Use this to automate powerpoint creation including certiain charts/visualizations.
Getting Started
Below is a quick tutorial taking you through how to setup and use this library. I will use public data to showcase how it works.
Step 1 - Install Dependencies
I have yet to figure out how to install required packages with the install of this library. Therefore, please ensure you have installed the following packages. For plotly, please refer to the getting started page to learn how to install everything properly.
- plotly.express
- plotly.graph_objects
- plotly.subplots
- scipy.stats
Additionally, if you want to be able to visualize plotly charts, you may have to install additonal requirements. Refer to the plotly getting started page and scroll down for your proper IDE (Jupyter Notebook, Lab, etc.).
Step 2 - Install Package
Step 3 - prepare your powerpoint.
The main function of this library is meant to generate slides which include a plotly chart. In order to do this, you must prepare a powerpoint template with the proper layout. You can design any layout you desire, but make sure that each element you desire to fill with python is created as a placeholder. You can use a powerpoint template I've included in my source code to make things easy (assets/powerpoint_templates/template.pptx).
In order to do this, open up your desired powerpoint and go into the slide master. Insert a new slide or use a current one already created. I recommend to drag this to be first in the order, this will make your life easier later down the road. Now insert the proper elements and arragne them as you please. For the chart, insert an image placeholder and make the apsect ratio similar to a laptop screen. Most images created are in landscape view but not too wide.
Step 4 - Load Library and Prepare Data
If you want to follow along with my tutorial, feel free and copy/paste my code. However, ensure you have pydataset installed before you do.
Step 5 - Find Your Powerpoint Elements
In order for this library to know where to place each element, you must give it each element index. See the following code to learn how to do this:
You will need this information later on.
Step 6 - Set Template
Now that we have our powerpoint template, we need to tell the library where to find this file. Do this with the following:
Step 7 - Define Charts
Here is where the work is done. Creating slides with charts is done by defining an array of dictionary objects. Each object represents one slide. Everything must be represented as a string except your dataframe object. Additionally, this library automatically groups every dataframe down to the level needed for the specific chart. Therefore, you don't have to create a new dataframe for each chart. As long as your df has the right data in it, this function will do the rest.
As a start, I will define two basic charts:
I will go into more detail on each one of these variables in a later section.
Step 8 - Run Function
This will output a file called output.pptx . I suggest you do not make this your final file, as each time you run this function you will overwrite the powerpoint. Create a copy of this file and start to create your analysis/report there. From here, you can use output.pptx as a slide library, where you can include or delete any chart you create.
Project details
Release history release notifications | rss feed.
Nov 22, 2022
Nov 3, 2022
Oct 26, 2022
Oct 25, 2022
Aug 24, 2022
Aug 23, 2022
Aug 11, 2022
Aug 10, 2022
Jun 7, 2021
Jun 5, 2021
Jun 4, 2021
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages .
Source Distribution
Uploaded Nov 22, 2022 Source
Built Distribution
Uploaded Nov 22, 2022 Python 3
Hashes for plotlyPowerpoint-1.2.17.tar.gz
Hashes for plotlypowerpoint-1.2.17-py3-none-any.whl.
- português (Brasil)
Supported by

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
How to create powerpoint files using Python
Introduction.
We’ve all had to make PowerPoint presentations at some point in our lives. Most often we’ve used Microsoft’s PowerPoint or Google Slides.
But what if you don’t have membership or access to the internet? Or what if you just wanted to do it the “programmers” way?
Well, worry not for Python’s got your back!
In this article you’ll learn how to create a PowerPoint file and add some content to it with the help of Python. So let’s get started!
Getting Started
Throughout this walkthrough, we’ll be using the python-pptx package. This package supports different python versions ranging from 2.6 to 3.6.
So, make sure you have the right version of Python installed on your computer first.
Next, open your terminal and type −
Once the module is successfully installed, you are all set to start coding!
Importing the Modules
Before we get into the main aspects of it, we must first import the right modules to utilise the various features of the package.
So, let’s import the presentation class that contains all the required methods to create a PowerPoint.
Now, we are all set to create a presentation.
Creating a Presentation
Let us now create an object of the Presentation class to access its various methods.
Next, we need to select a layout for the presentation.

As you can see, there are nine different layouts. In the pptx module, each layout is numbered from 0 to 8. So, “Title Slide” is 0 and the “Picture with Caption” is 8.
So, let us first add a title slide.
Now, we have created a layout and added a slide to our presentation.
Let us now add some content to the first slide.
In the above lines, we first add a title to the “first slide” and a subtitle using the placeholder.
Now, let us save the presentation. We can do this using the save command.
If you run the program, it will save the PowerPoint presentation in the directory where your program is saved.

You have successfully created your PowerPoint presentation.
Creating a second slide and adding some content
Firstly, you’ll need to import additional methods to add content.
Let us first create and add the second slide.
Adding title for the next slide,
Now, we must create a textbox and move its layout to suit our needs.
Let us position it and adjust its margins in inches.
The above line of code will place a textbox 3 Inches from left and 1.5 Inches from the top with a width of 3 Inches and height of 1 Inch.
Once we have the layout and position fixed, time to create a textframe to add content to.
Now to add a paragraph of content,
Finally, save the presentation again using the save method.

That’s it! You can now create your own presentation with the help of Python.
And there are a lot more features within the pptx package that allows you to completely customise your presentation from A-Z just the way you do it in GUI.
You can add images, build charts, display statistics and a lot more.
You can go through python-pptx official documentation for more syntaxes and features.

Related Articles
- How to convert PDF files to Excel files using Python?
- How to remove swap files using Python?
- How to Create a list of files, folders, and subfolders in Excel using Python?
- How to rename multiple files recursively using Python?
- How to add audio files using Python kivy
- Tips for Using PowerPoint Presentation More Efficiently
- How to close all the opened files using Python?
- How to touch all the files recursively using Python?
- How to ignore hidden files using os.listdir() in Python?
- How to remove hidden files and folders using Python?
- How to read text files using LINECACHE in Python
- PowerPoint Alternatives
- How to copy files to a new directory using Python?
- How are files added to a tar file using Python?
- How are files added to a zip file using Python?
Kickstart Your Career
Get certified by completing the course

Python Tutorial
Python oops, python mysql, python mongodb, python sqlite, python questions, python tkinter (gui), python web blocker, related tutorials, python programs.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

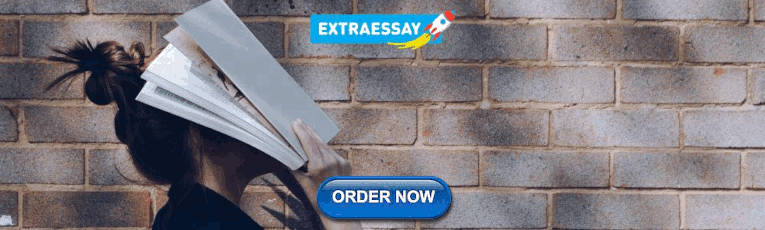
IMAGES
VIDEO
COMMENTS
In looking at the output of. analyze_ppt.py we know that the title slide is layout 0 and that it has a title attribute and a subtitle at placeholder 1. Here is the start of the function that we use to create our output PowerPoint: def create_ppt(input, output, report_data, chart): """ Take the input powerpoint file and use it as the template ...
II. Process Data and Design Slides with Python. You can find the source code with dummy data here: Github. Let us explore all the steps to generate your final report. Steps to create your operational report on PowerPoint — (Image by Author) 1. Data Extraction. Connect to your WMS and extract shipment records.
subtitle.text = "Creating presentations with python-pptx." prs.save('python-pptx-presentation.pptx') The code snippet above creates a PowerPoint file named python-pptx-presentation.pptx with one slide that includes a title and a subtitle. In this overview, we create a presentation object, add a new slide with a predefined layout, set text for ...
pip install python-pptx. Let's see some of its usage: Example 1: Creating new PowerPoint file with title and subtitle slide. Python3. from pptx import Presentation . root = Presentation() first_slide_layout = root.slide_layouts[0] . 0 -> title and subtitle. 5 -> Title only .
python-pptx¶. Release v0.6.22 (Installation)python-pptx is a Python library for creating, reading, and updating PowerPoint (.pptx) files.. A typical use would be generating a PowerPoint presentation from dynamic content such as a database query, analytics output, or a JSON payload, perhaps in response to an HTTP request and downloading the generated PPTX file in response.
Comprehensive Guide. Creating a PowerPoint document using Python allows you to automate the process of generating professional presentations with ease. By leveraging libraries such as Spire ...
python-pptx is a Python library for creating and updating PowerPoint files. This article is going to be a basic introduction to this package. If you want to learn much more about it, this is the official documentation page that you should check. Now let's install the package if you don't have. pip install python-pptx.
Spire.Presentation for Python is a comprehensive PowerPoint compatible API designed for developers to efficiently create, modify, read, and convert PowerPoint files within Python programs. It offers a broad spectrum of functions to manipulate PowerPoint documents without any external dependencies. Spire.Presentation for Python supports a wide ...
The python-pptx can control almost every element in PowerPoint that is available when working with PowerPoint directly. It is possible to start with a PowerPoint template, an existing presentation, or create the entire presentation.
Project description. python-pptx is a Python library for creating, reading, and updating PowerPoint (.pptx) files. A typical use would be generating a PowerPoint presentation from dynamic content such as a database query, analytics output, or a JSON payload, perhaps in response to an HTTP request and downloading the generated PPTX file in ...
Spire.Presentation for Python is a comprehensive PowerPoint compatible API designed for developers to efficiently create, modify, read, and convert PowerPoint files within Python programs. It offers a broad spectrum of functions to manipulate PowerPoint documents without any external dependencies. Spire.Presentation for Python supports a wide ...
Opening a presentation ¶. The simplest way to get started is to open a new presentation without specifying a file to open: from pptx import Presentation prs = Presentation() prs.save('test.pptx') This creates a new presentation from the built-in default template and saves it unchanged to a file named 'test.pptx'. A couple things to note:
This library allows developers to create, modify, and extract information from PowerPoint presentations with just a few lines of code. In this article, we'll explore the different ways to use Python to create PowerPoint presentations and discuss the use of templates to create consistent and professional-looking presentations. Getting Started
In this tutorial I will be showing you how to create POWERPOINT PRESENTATIONS using only Python. This tutorial provides a step-by-step walk-through made to h...
Using python-pptx. To help us automate the PowerPoint presentation report, we would use the Python package called python-pptx. It is a Python package developed to create and update PowerPoint files. To start using the package, we need to install it first with the following code. pip install python-pptx.
4. You can use python-pptx library. It is a Python library for creating and updating PowerPoint (.pptx) files. This includes: Round-trip any Open XML presentation (.pptx file) including all its elements Add slides Populate text placeholders, for example to create a bullet slide Add image to slide at arbitrary position and size Add textbox to a ...
Here is a list of features provided by the python-pptx library: Create Presentations: Generate new PowerPoint presentations or modify existing ones. Slide Layouts: Choose from a variety of predefined slide layouts to structure your presentation. Text Handling: Add titles, subtitles, bullet points, and various text elements to slides.
This allows content from one deck to be pasted into another and be connected with the right new slide layout: In python-pptx, these are prs.slide_layouts[0] through prs.slide_layouts[8] . However, there's no rule they have to appear in this order, it's just a convention followed by the themes provided with PowerPoint.
A python library for creating PowerPoint slides with Plotly charts - jonboone1/plotlyPowerpoint. ... #load presentation prs = Presentation("template.pptx") #set the slide we are after - the first slide in the master layout is at index 0 slide = prs.slides.add_slide(prs.slide_layouts[0]) #print index and name for shape in slide.placeholders ...
Step 4 - Load Library and Prepare Data. If you want to follow along with my tutorial, feel free and copy/paste my code. However, ensure you have pydataset installed before you do. from pptx import Presentation. import pandas as pd. from pydataset import data. import plotlyPowerpoint as pp.
Layout = X.slide_layouts[0] first_slide = X.slides.add_slide(Layout) # Adding first slide. Now, we have created a layout and added a slide to our presentation. Let us now add some content to the first slide. first_slide.shapes.title.text = "Creating a powerpoint using Python". first_slide.placeholders[1].text = "Created by Tutorialpoints".
The images are of variable sizes and I would like to automate the procedure of converting this data into a PowerPoint presentation, with one picture on one slide along with its date and caption as the Title. ... Check out the python-pptx library. Its useful for creating and updating PowerPoint .pptx files. Also for some quick examples in python ...
Verifying the Installation. Once the module is installed, we can verify it by creating an empty Python program file and writing an import statement as follows: File: verify.py. import python-pptx. Now, save the above file and execute it using the following command in a terminal: Syntax: $ python verify.py.