Object-Oriented Programming in Java – A Beginner's Guide

Hi, folks! Today we are going to talk about object-oriented programming in Java.
This article will help give you a thorough understanding of the underlying principles of object-oriented programming and its concepts.
Once you understand these concepts, you should have the confidence and ability to develop basic problem-solving applications using object-oriented programming principles in Java.
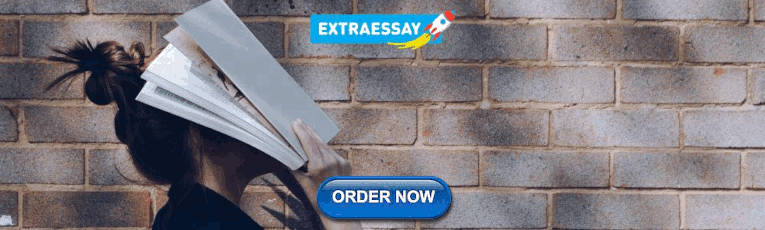
What is Object-Oriented Programming?
Object-oriented programming (OOP) is a fundamental programming paradigm based on the concept of “ objects ” . These objects can contain data in the form of fields (often known as attributes or properties) and code in the form of procedures (often known as methods).
The core concept of the object-oriented approach is to break complex problems into smaller objects.
In this article, we will be looking at the following OOP concepts:
What is Java?
- What is a class?
- What is an object?
- What is a Java Virtual Machine (JVM)?
- How access modifiers work in Java.
- How constructors work in Java.
- How methods work in Java.
- Key principles of OOP.
- Interfaces in Java.
Java is a general-purpose, class-based, object-oriented programming language, which works on different operating systems such as Windows, Mac, and Linux.
You can use Java to develop:
- Desktop applications
- Web applications
- Mobile applications (especially Android apps)
- Web and application servers
- Big data processing
- Embedded systems
And much more.
In Java, every application starts with a class name, and this class must match the file name. When saving a file, save it using the class name and add “ .java ” to the end of the file name.
Let's write a Java program that prints the message “ Hello freeCodeCamp community. My name is ... ” .
We are going to start by creating our first Java file called Main.java, which can be done in any text editor. After creating and saving the file, we are going to use the below lines of code to get the expected output.
Don't worry if you don't understand the above code at the moment. We are going to discuss, step by step, each line of code just below.
For now, I want you to start by noting that every line of code that runs in Java must be in a class.
You may also note that Java is case-sensitive. This means that Java has the ability to distinguish between upper and lower case letters. For example, the variable “ myClass ” and the variable “ myclass ” are two totally different things.
Alright, let's see what that code's doing:
Let's first look at the main() method: public static void main(String[] args) .
This method is required in every Java program, and it is the most important one because it is the entry point of any Java program.
Its syntax is always public static void main(String[] args) . The only thing that can be changed is the name of the string array argument. For example, you can change args to myStringArgs .
What is a Class in Java?
A class is defined as a collection of objects. You can also think of a class as a blueprint from which you can create an individual object.
To create a class, we use the keyword class .
Syntax of a class in Java:
In the above syntax, we have fields (also called variables) and methods, which represent the state and behavior of the object, respectively.
Note that in Java, we use fields to store data, while we use methods to perform operations.
Let's take an example:
We are going to create a class named “ Main ” with a variable “ y ” . The variable “ y ” is going to store the value 2.
Note that a class should always start with an uppercase first letter, and the Java file should match the class name.
What is an Object in Java?
An object is an entity in the real world that can be distinctly identified. Objects have states and behaviors. In other words, they consist of methods and properties to make a particular type of data useful.
An object consists of:
- A unique identity: Each object has a unique identity, even if the state is identical to that of another object.
- State/Properties/Attributes: State tells us how the object looks or what properties it has.
- Behavior: Behavior tells us what the object does.
Examples of object states and behaviors in Java:
Let's look at some real-life examples of the states and behaviors that objects can have.
- Object: car.
- State: color, brand, weight, model.
- Behavior: break, accelerate, turn, change gears.
- Object: house.
- State: address, color, location.
- Behavior: open door, close door, open blinds.
Syntax of an object in Java:
What is the java virtual machine (jvm).
The Java virtual machine (JVM) is a virtual machine that enables a computer to run Java programs.
The JVM has two primary functions, which are:
- To allow Java programs to run on any device or operating system (this is also known as the "Write once, run anywhere" principle).
- And, to manage and optimize program memory.
How Access Modifiers Work in Java
In Java, access modifiers are keywords that set the accessibility of classes, methods, and other members.
These keywords determine whether a field or method in a class can be used or invoked by another method in another class or sub-class.
Access modifiers may also be used to restrict access.
In Java, we have four types of access modifiers, which are:
Let's look at each one in more detail now.
Default Access Modifier
The default access modifier is also called package-private. You use it to make all members within the same package visible, but they can be accessed only within the same package.
Note that when no access modifier is specified or declared for a class, method, or data member, it automatically takes the default access modifier.
Here is an example of how you can use the default access modifier:
Now let's see what this code is doing:
void output() : When there is no access modifier, the program automatically takes the default modifier.
SampleClass obj = new SampleClass(); : This line of code allows the program to access the class with the default access modifier.
obj.output(); : This line of code allows the program to access the class method with the default access modifier.
The output is: Hello World! This is an Introduction to OOP - Beginner's guide. .
Public Access Modifier
The public access modifier allows a class, a method, or a data field to be accessible from any class or package in a Java program. The public access modifier is accessible within the package as well as outside the package.
In general, a public access modifier does not restrict the entity at all.
Here is an example of how the public access modifier can be used:
Now let's see what's going on in that code:
In the above example,
- The public class Car is accessed from the Main class.
- The public variable tireCount is accessed from the Main class.
- The public method display() is accessed from the Main class.
Private Access Modifier
The private access modifier is an access modifier that has the lowest accessibility level. This means that the methods and fields declared as private are not accessible outside the class. They are accessible only within the class which has these private entities as its members.
You may also note that the private entities are not visible even to the subclasses of the class.
Here is an example of what would happen if you try accessing variables and methods declared private, outside the class:
Alright, what's going on here?
- private String activity : The private access modifier makes the variable “activity” a private one.
- SampleClass task = new SampleClass(); : We have created an object of SampleClass.
- task.activity = "We are learning the core concepts of OOP."; : On this line of code we are trying to access the private variable and field from another class (which can never be accessible because of the private access modifier).
When we run the above program, we will get the following error:
This is because we are trying to access the private variable and field from another class.
So, the best way to access these private variables is to use the getter and setter methods.
Getters and setters are used to protect your data, particularly when creating classes. When we create a getter method for each instance variable, the method returns its value while a setter method sets its value.
Let's have a look at how we can use the getters and setters method to access the private variable.
When we run the above program, this is the output:
As we have a private variable named task in the above example, we have used the methods getTask() and setTask() in order to access the variable from the outer class. These methods are called getter and setter in Java.
We have used the setter method ( setTask() ) to assign value to the variable and the getter method ( getTask() ) to access the variable.
To learn more about the this keyword, you can read this article here .
Protected Access Modifier
When methods and data members are declared protected , we can access them within the same package as well as from subclasses.
We can also say that the protected access modifier is somehow similar to the default access modifier. It is just that it has one exception, which is its visibility in subclasses.
Note that classes cannot be declared protected. This access modifier is generally used in a parent-child relationship.
Let's have a look at how we can use the protected access modifier:
What's this code doing?
In this example, the class Test which is present in another package is able to call the multiplyTwoNumbers() method, which is declared protected.
The method is able to do so because the Test class extends class Addition and the protected modifier allows the access of protected members in subclasses (in any packages).
What are Constructors in Java?
A constructor in Java is a method that you use to initialize newly created objects.
Syntax of a constructor in Java:
So what's going on in this code?
- We have started by creating the Main class.
- After that, we have created a class attribute, which is the variable a .
- Third, we have created a class constructor for the Main class.
- After that, we have set the initial value for variable a that we have declared. The variable a will have a value of 9. Our program will just take 3 times 3, which is equal to 9. You are free to assign any value to the variable a . (In programming, the symbol “*” means multiplication).
Every Java program starts its execution in the main() method. So, we have used the public static void main(String[] args) , and that is the point from where the program starts its execution. In other words, the main() method is the entry point of every Java program.
Now I'll explain what every keyword in the main() method does.
The public keyword.
The public keyword is an access modifier . Its role is to specify from where the method can be accessed, and who can access it. So, when we make the main() method public, it makes it globally available. In other words, it becomes accessible to all parts of the program.
The static keyword.
When a method is declared with a static keyword, it is known as a static method. So, the Java main() method is always static so that the compiler can call it without or before the creation of an object of the class.
If the main() method is allowed to be non-static, then the Java Virtual Machine will have to instantiate its class while calling the main() method.
The static keyword is also important as it saves unnecessary memory wasting which would have been used by the object declared only for calling the main() method by the Java Virtual Machine.
The Void keyword.
The void keyword is a keyword used to specify that a method doesn’t return anything. Whenever the main() method is not expected to return anything, then its return type is void. So, this means that as soon as the main() method terminates, the Java program terminates too.
Main is the name of the Java main method. It is the identifier that the Java Virtual Machine looks for as the starting point of the java program.
The String[] args .
This is an array of strings that stores Java command line arguments.
The next step is to create an object of the class Main. We have created a function call that calls the class constructor.
The last step is to print the value of a , which is 9.
How Methods Work in Java
A method is a block of code that performs a specific task. In Java, we use the term method, but in some other programming languages such as C++, the same method is commonly known as a function.
In Java, there are two types of methods:
- User-defined Methods : These are methods that we can create based on our requirements.
- Standard Library Methods : These are built-in methods in Java that are available to use.
Let me give you an example of how you can use methods in Java.
Java methods example 1:
In the above example, we have created a method named divideNumbers() . The method takes two parameters x and y, and we have called the method by passing two arguments firstNumber and secondNumber .
Now that you know some Java basics, let's look at object-oriented programming principles in a bit more depth.
Key Principles of Object-Oriented Programming.
There are the four main principles of the Object-Oriented Programming paradigm. These principles are also known as the pillars of Object-Oriented Programming.
The four main principles of Object-Oriented Programming are:
- Encapsulation (I will also touch briefly on Information Hiding)
- Inheritance
- Abstraction
- Polymorphism
Encapsulation and Information Hiding in Java
Encapsulation is when you wrap up your data under a single unit. In simple terms, it is more or less like a protective shield that prevents the data from being accessed by the code outside this shield.
A simple example of encapsulation is a school bag. A school bag can keep all your items safe in one place, such as your books, pens, pencils, ruler, and more.
Information hiding or data hiding in programming is about protecting data or information from any inadvertent change throughout the program. This is a powerful Object-Oriented Programming feature, and it is closely associated with encapsulation.
The idea behind encapsulation is to ensure that " sensitive " data is hidden from users. To achieve this, you must:
- Declare class variables/attributes as private .
- Provide public get and set methods to access and update the value of a private variable.
As you remember, private variables can only be accessed within the same class and an external class cannot access them. However, they can be accessed if we provide public get and set methods.
Let me give you an additional example that demonstrates how the get and set methods work:
Inheritance in Java
Inheritance allows classes to inherit attributes and methods of other classes. This means that parent classes extend attributes and behaviors to child classes. Inheritance supports reusability.
A simple example that explains the term inheritance is that human beings (in general) inherit certain properties from the class "Human" such as the ability to speak, breathe, eat, drink, and so on.
We group the "inheritance concept" into two categories:
- subclass (child) - the class that inherits from another class.
- superclass (parent) - the class being inherited from.
To inherit from a class, we use the extends keyword.
In the below example, the JerryTheMouse class is created by inheriting the methods and fields from the Animal class.
JerryTheMouse is the subclass and Animal is the superclass.
Abstraction in Java
Abstraction is a concept in object-oriented programming that lets you show only essential attributes and hides unnecessary information in your code. The main purpose of abstraction is to hide unnecessary details from your users.
A simple example to explain abstraction is to think about the process that comes into play when you send an email. When you send an email, complex details such as what happens as soon as it is sent and the protocol that the server uses are hidden from you.
When you send an e-mail, you just need to enter the email address of the receiver, the email subject, type the content, and click send.
You can abstract stuff by using abstract classes or interfaces .
The abstract keyword is a non-access modifier, used for classes and methods:
- Abstract class: is a restricted class that cannot be used to create objects. To access it, it must be inherited from another class.
- Abstract method: A method that doesn't have its body is known as an abstract method. We use the same abstract keyword to create abstract methods.
The body of an abstract method is provided by the subclass (inherited from).
Polymorphism in Java
Polymorphism refers to the ability of an object to take on many forms. Polymorphism normally occurs when we have many classes that are related to each other by inheritance.
Polymorphism is similar to how a person can have different characteristics at the same time.
For instance, a man can be a father, a grandfather, a husband, an employee, and so forth – all at the same time. So, the same person possesses different characteristics or behaviors in different situations.
We are going to create objects Cow and Cat, and call the animalSound() method on each of them.
Inheritance and polymorphism are very useful for code reusability. You can reuse the attributes and methods of an existing class when you create a new class.
Interfaces in Java
An interface is a collection of abstract methods. In other words, an interface is a completely " abstract class " used to group related methods with empty bodies.
An interface specifies what a class can do but not how it can do it.
We have looked at some of the main object-oriented programming concepts in this article. Having a good understanding of these concepts is essential if you want to use them well and write good code.
I hope this article was helpful.
My name is Patrick Cyubahiro, I am a software & web developer, UI/UX designer, technical writer, and Community Builder.
Feel free to connect with me on Twitter: @ Pat_Cyubahiro , or to write to: ampatrickcyubahiro[at]gmail.com
Thanks for reading and happy learning!
Community Builder, Software & web developer, UI/IX Designer, Technical Writer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Java Hello World
- Java JVM, JRE and JDK
- Java Variables and Literals
- Java Data Types
- Java Operators
- Java Input and Output
- Java Expressions & Blocks
- Java Comment
Java Flow Control
- Java if...else
- Java switch Statement
- Java for Loop
- Java for-each Loop
- Java while Loop
- Java break Statement
- Java continue Statement
- Java Arrays
- Multidimensional Array
- Java Copy Array
Java OOP (I)
Java class and objects.
- Java Methods
- Java Method Overloading
- Java Constructor
- Java Strings
- Java Access Modifiers
- Java this keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP (II)
- Java Inheritance
- Java Method Overriding
- Java super Keyword
- Abstract Class & Method
- Java Interfaces
- Java Polymorphism
- Java Encapsulation
Java OOP (III)
- Nested & Inner Class
- Java Static Class
- Java Anonymous Class
- Java Singleton
- Java enum Class
- Java enum Constructor
- Java enum String
Java Reflection
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java List Interface
- Java ArrayList
- Java Vector
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue Interface
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet
- Java EnumSet
- Java LinkedhashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator
- Java ListIterator
- Java I/O Streams
- Java InputStream
- Java OutputStream
- Java FileInputStream
- Java FileOutputStream
- Java ByteArrayInputStream
- Java ByteArrayOutputStream
- Java ObjectInputStream
- Java ObjectOutputStream
- Java BufferedInputStream
- Java BufferedOutputStream
- Java PrintStream
Java Reader/Writer
- Java Reader
- Java Writer
- Java InputStreamReader
- Java OutputStreamWriter
- Java FileReader
- Java FileWriter
- Java BufferedReader
- Java BufferedWriter
- Java StringReader
- Java StringWriter
- Java PrintWriter
Additional Topics
- Java Scanner Class
- Java Type Casting
- Java autoboxing and unboxing
- Java Lambda Expression
- Java Generics
- Java File Class
- Java Wrapper Class
- Java Command Line Arguments
Java Tutorials
Java Abstract Class and Abstract Methods
Java Singleton Class
- Java Nested Static Class
- Java Nested and Inner Class
Java Static Keyword
Java is an object-oriented programming language. The core concept of the object-oriented approach is to break complex problems into smaller objects.
An object is any entity that has a state and behavior . For example, a bicycle is an object. It has
- States : idle, first gear, etc
- Behaviors : braking, accelerating, etc.
Before we learn about objects, let's first know about classes in Java.
A class is a blueprint for the object. Before we create an object, we first need to define the class.
We can think of the class as a sketch (prototype) of a house. It contains all the details about the floors, doors, windows, etc. Based on these descriptions we build the house. House is the object.
Since many houses can be made from the same description, we can create many objects from a class.
- Create a class in Java
We can create a class in Java using the class keyword. For example,
Here, fields ( variables ) and methods represent the state and behavior of the object respectively.
- fields are used to store data
- methods are used to perform some operations
For our bicycle object, we can create the class as
In the above example, we have created a class named Bicycle . It contains a field named gear and a method named braking() .
Here, Bicycle is a prototype. Now, we can create any number of bicycles using the prototype. And, all the bicycles will share the fields and methods of the prototype.
Note : We have used keywords private and public . These are known as access modifiers. To learn more, visit Java access modifiers .
- Java Objects
An object is called an instance of a class. For example, suppose Bicycle is a class then MountainBicycle , SportsBicycle , TouringBicycle , etc can be considered as objects of the class.
Creating an Object in Java
Here is how we can create an object of a class.
We have used the new keyword along with the constructor of the class to create an object. Constructors are similar to methods and have the same name as the class. For example, Bicycle() is the constructor of the Bicycle class. To learn more, visit Java Constructors .
Here, sportsBicycle and touringBicycle are the names of objects. We can use them to access fields and methods of the class.
As you can see, we have created two objects of the class. We can create multiple objects of a single class in Java.
Note : Fields and methods of a class are also called members of the class.
- Access Members of a Class
We can use the name of objects along with the . operator to access members of a class. For example,
In the above example, we have created a class named Bicycle . It includes a field named gear and a method named braking() . Notice the statement,
Here, we have created an object of Bicycle named sportsBicycle . We then use the object to access the field and method of the class.
- sportsBicycle.gear - access the field gear
- sportsBicycle.braking() - access the method braking()
We have mentioned the word method quite a few times. You will learn about Java methods in detail in the next chapter.
Now that we understand what is class and object. Let's see a fully working example.
- Example: Java Class and Objects
In the above program, we have created a class named Lamp . It contains a variable: isOn and two methods: turnOn() and turnOff() .
Inside the Main class, we have created two objects: led and halogen of the Lamp class. We then used the objects to call the methods of the class.
- led.turnOn() - It sets the isOn variable to true and prints the output.
- halogen.turnOff() - It sets the isOn variable to false and prints the output.
The variable isOn defined inside the class is also called an instance variable. It is because when we create an object of the class, it is called an instance of the class. And, each instance will have its own copy of the variable.
That is, led and halogen objects will have their own copy of the isOn variable.
Example: Create objects inside the same class
Note that in the previous example, we have created objects inside another class and accessed the members from that class.
However, we can also create objects inside the same class.
Here, we are creating the object inside the main() method of the same class.
Table of Contents
- Introduction
- Example: Create objects inside a class
Sorry about that.
Related Tutorials
Java Tutorial
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Assignment, Arithmetic, and Unary Operators
The simple assignment operator.
One of the most common operators that you'll encounter is the simple assignment operator " = ". You saw this operator in the Bicycle class; it assigns the value on its right to the operand on its left:
This operator can also be used on objects to assign object references , as discussed in Creating Objects .
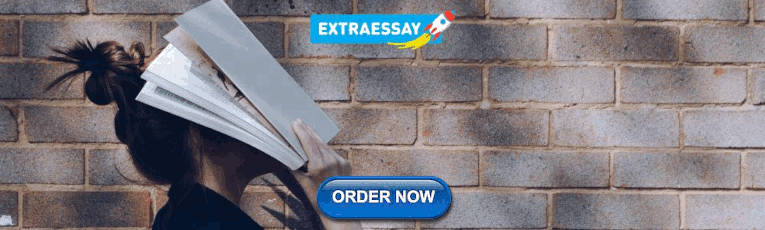
The Arithmetic Operators
The Java programming language provides operators that perform addition, subtraction, multiplication, and division. There's a good chance you'll recognize them by their counterparts in basic mathematics. The only symbol that might look new to you is " % ", which divides one operand by another and returns the remainder as its result.
The following program, ArithmeticDemo , tests the arithmetic operators.
This program prints the following:
You can also combine the arithmetic operators with the simple assignment operator to create compound assignments . For example, x+=1; and x=x+1; both increment the value of x by 1.
The + operator can also be used for concatenating (joining) two strings together, as shown in the following ConcatDemo program:
By the end of this program, the variable thirdString contains "This is a concatenated string.", which gets printed to standard output.
The Unary Operators
The unary operators require only one operand; they perform various operations such as incrementing/decrementing a value by one, negating an expression, or inverting the value of a boolean.
The following program, UnaryDemo , tests the unary operators:
The increment/decrement operators can be applied before (prefix) or after (postfix) the operand. The code result++; and ++result; will both end in result being incremented by one. The only difference is that the prefix version ( ++result ) evaluates to the incremented value, whereas the postfix version ( result++ ) evaluates to the original value. If you are just performing a simple increment/decrement, it doesn't really matter which version you choose. But if you use this operator in part of a larger expression, the one that you choose may make a significant difference.
The following program, PrePostDemo , illustrates the prefix/postfix unary increment operator:
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java - what is oop.
OOP stands for Object-Oriented Programming .
Procedural programming is about writing procedures or methods that perform operations on the data, while object-oriented programming is about creating objects that contain both data and methods.
Object-oriented programming has several advantages over procedural programming:
- OOP is faster and easier to execute
- OOP provides a clear structure for the programs
- OOP helps to keep the Java code DRY "Don't Repeat Yourself", and makes the code easier to maintain, modify and debug
- OOP makes it possible to create full reusable applications with less code and shorter development time
Tip: The "Don't Repeat Yourself" (DRY) principle is about reducing the repetition of code. You should extract out the codes that are common for the application, and place them at a single place and reuse them instead of repeating it.
Java - What are Classes and Objects?
Classes and objects are the two main aspects of object-oriented programming.
Look at the following illustration to see the difference between class and objects:
Another example:
So, a class is a template for objects, and an object is an instance of a class.
When the individual objects are created, they inherit all the variables and methods from the class.
You will learn much more about classes and objects in the next chapter.

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.

Last Minute Java Object Assignment and Object Passing By Value or Reference Explained Tutorial
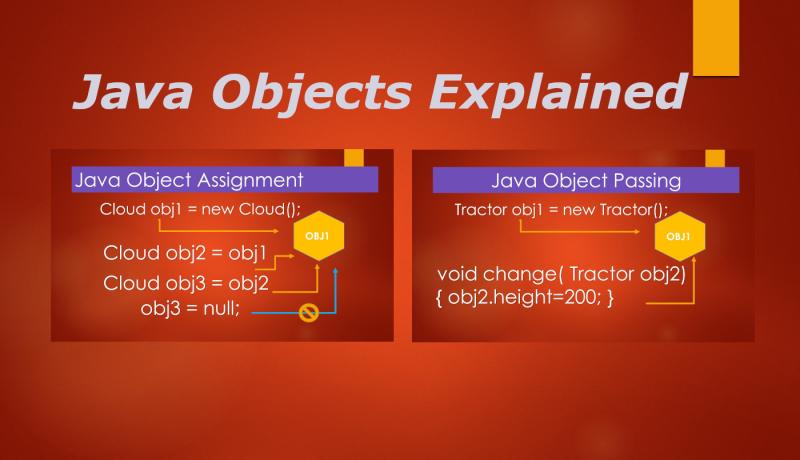
In Java, we create Classes. We create objects of the Classes. Let us know if it is possible to assign a Java Object from one variable to another variable by value or reference in this Last Minute Java Tutorial. We also try to know whether it is possible to pass Java Objects to another method by Value or Reference in this tutorial.
You may also read about Introduction to Java Class Structure .
Java Object Assignment by Value or Reference Explained
In Java, objects are always passed by Reference. Reference is nothing but the starting address of a memory location where the actual object lies. You can create any number of references to just One Object. Let us go by an example.
We create a Class "Cloud" with a single Property called "color". It is a String object. We create 3 objects of the Cloud type.
Notice that all three references obj1, obj2 and obj3 are pointing to the same Cloud object. Thus the above example outputs the same color-property value " RED ". Later, we made the references obj2 and obj3 to point to the null location. Still the original object pointed or referenced by obj1 holds the value. So the final PRINT statement outputs "RED" as usual.
Note : Reference is also called a Variable whether Primitive or Object.
This concludes that a Java object assignment happens by Reference . But the actual value of memory location pointed by References are copied by Value only. So we are actually copying memory locations from one Reference variable to another Reference variable by Value . Interviewers ask this famous Java question just to test your knowledge. If someone asks in C language point of view , say that it is by Reference . If they ask in Java point of view , say that it is by Value.
Java Object Passing by Value or Reference to a Method Explained
You already learnt that Object assignment in Java happens by Reference. Let us try to pass Objects to another method as a parameter or argument. We try to know whether Objects are passed by Value or Reference to another Method using the below example.
In the above example, we have created an object "obj1" of type Tractor. We assigned value of 100 to the property "height". We printed the value before passing the object to another method. Now, we passed the object to another method called "change" from the calling-method " main ". We modified the height property to 200 in the called-method . Now, we tried to print the value of the property in the Calling method. It shows that the Object is modified in the called-method.
Similarly, we can pass Java objects to the Constructor using Pass by Reference or simply Reference .
This concludes that Java Objects are passed to methods by Reference . Here also, we pass the memory location pointed by one Reference variable to another Reference variable of a Called method as a Value only. So, you are actually passing Values (memory locations represented by integer or long data type). But the actual passed-object can only be referenced by a Reference variable. Interviewers take advantage of this tricky logic to confuse you. If someone asks in C language point of view , say that it is by Reference . If they ask in Java point of view , say that it is by Value .
Let us know more about Java Classes with different Constructors and Methods in coming chapters.
Share this Last Minute Java Tutorial explaining Java Object Assignment using Reference and Java Object Passing with Pass by Reference with your friends and colleagues to encourage authors.
Java Class Structure Tutorial
Java Method Signature Rules Tutorial
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Tutorial
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
- Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Differences between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they provide.
Types of Operators:
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
This article explains all that one needs to know regarding Assignment Operators.
Assignment Operators
These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right side operand of the assignment operator is a value. The value on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. This means that the assignment operators have right to left associativity, i.e., the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side value must be declared before using it or should be a constant. The general format of the assignment operator is,
Types of Assignment Operators in Java
The Assignment Operator is generally of two types. They are:
1. Simple Assignment Operator: The Simple Assignment Operator is used with the “=” sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
2. Compound Assignment Operator: The Compound Operator is used where +,-,*, and / is used along with the = operator.
Let’s look at each of the assignment operators and how they operate:
1. (=) operator:
This is the most straightforward assignment operator, which is used to assign the value on the right to the variable on the left. This is the basic definition of an assignment operator and how it functions.
Syntax:
Example:
2. (+=) operator:
This operator is a compound of ‘+’ and ‘=’ operators. It operates by adding the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
Note: The compound assignment operator in Java performs implicit type casting. Let’s consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5 Method 2: x += 4.5 As per the previous example, you might think both of them are equal. But in reality, Method 1 will throw a runtime error stating the “i ncompatible types: possible lossy conversion from double to int “, Method 2 will run without any error and prints 9 as output.
Reason for the Above Calculation
Method 1 will result in a runtime error stating “incompatible types: possible lossy conversion from double to int.” The reason is that the addition of an int and a double results in a double value. Assigning this double value back to the int variable x requires an explicit type casting because it may result in a loss of precision. Without the explicit cast, the compiler throws an error. Method 2 will run without any error and print the value 9 as output. The compound assignment operator += performs an implicit type conversion, also known as an automatic narrowing primitive conversion from double to int . It is equivalent to x = (int) (x + 4.5) , where the result of the addition is explicitly cast to an int . The fractional part of the double value is truncated, and the resulting int value is assigned back to x . It is advisable to use Method 2 ( x += 4.5 ) to avoid runtime errors and to obtain the desired output.
Same automatic narrowing primitive conversion is applicable for other compound assignment operators as well, including -= , *= , /= , and %= .
3. (-=) operator:
This operator is a compound of ‘-‘ and ‘=’ operators. It operates by subtracting the variable’s value on the right from the current value of the variable on the left and then assigning the result to the operand on the left.
4. (*=) operator:
This operator is a compound of ‘*’ and ‘=’ operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
5. (/=) operator:
This operator is a compound of ‘/’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the quotient to the operand on the left.
6. (%=) operator:
This operator is a compound of ‘%’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the remainder to the operand on the left.
Please Login to comment...
Similar reads.
- Java-Operators
- CBSE Exam Format Changed for Class 11-12: Focus On Concept Application Questions
- 10 Best Waze Alternatives in 2024 (Free)
- 10 Best Squarespace Alternatives in 2024 (Free)
- Top 10 Owler Alternatives & Competitors in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
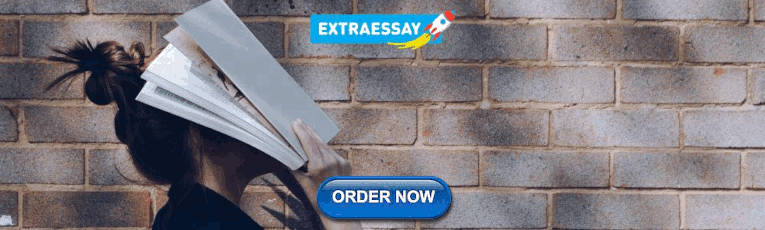
IMAGES
VIDEO
COMMENTS
I am new to Java and I have some questions in mind regarding object assignment. For instance, Test t1 = new Test(); Test t2 = t1; t1.i=1; Assuming variable i is defined inside Test class, am I right to assume both t1 and t2 point to the same object where the modification t1.i=1 affects both t1 and t2?Actually I tested it out and seems like I was right.
Object-oriented programming (OOP) is a fundamental programming paradigm based on the concept of " objects ". These objects can contain data in the form of fields (often known as attributes or properties) and code in the form of procedures (often known as methods). The core concept of the object-oriented approach is to break complex problems ...
The Class object that represents the runtime class of this object. See The Java™ Language Specification: 15.8.2 Class Literals; hashCode public int hashCode() ... the class of this object and initializes all its fields with exactly the contents of the corresponding fields of this object, as if by assignment; the contents of the fields are not ...
A class in Java is a set of objects which shares common characteristics/ behavior and common properties/ attributes. It is a user-defined blueprint or prototype from which objects are created. For example, Student is a class while a particular student named Ravi is an object. Properties of Java Classes. Class is not a real-world entity.
In Java, your variables can be split into two categories: Objects, and everything else (int, long, byte, etc). A primitive type (int, long, etc), holds whatever value you assign it. An object variable, by contrast, holds a reference to an object somewhere. So if you assign one object variable to another, you have copied the reference, both A ...
Java Class and Objects. Java is an object-oriented programming language. The core concept of the object-oriented approach is to break complex problems into smaller objects. An object is any entity that has a state and behavior. For example, a bicycle is an object. It has. States: idle, first gear, etc. Behaviors: braking, accelerating, etc.
Referencing an Object's Fields. Object fields are accessed by their name. You must use a name that is unambiguous. You may use a simple name for a field within its own class. For example, we can add a statement within the Rectangle class that prints the width and height: System.out.println("Width and height are: " + width + ", " + height);
The first line creates an object of the Point class, and the second and third lines each create an object of the Rectangle class. Each of these statements has three parts (discussed in detail below): Declaration: The code set in bold are all variable declarations that associate a variable name with an object type.; Instantiation: The new keyword is a Java operator that creates the object.
By the way, all Java objects are polymorphic because each object is an Object at least. We can assign an instance of Animal to the reference variable of Object type and the compiler won't complain: Object object = new Animal(); That's why all Java objects we create already have Object-specific methods, for example toString().
The default toString () method for class Object returns a string consisting of the name of the class of which the object is an instance, the at-sign character `@', and the unsigned hexadecimal representation of the hash code of the object. In other words, it is defined as: Unmute. ×. // Default behavior of toString() is to print class name ...
Java is an object-oriented programming language. Everything in Java is associated with classes and objects, along with its attributes and methods. For example: in real life, a car is an object. The car has attributes, such as weight and color, and methods, such as drive and brake. A Class is like an object constructor, or a "blueprint" for ...
There are 3 modules in this course. This course provides an introduction to the Java language and object-oriented programming, including an overview of Java syntax and how it differs from a language like Python. Students will learn how to write custom Java classes and methods, and how to test their code using unit testing and test-driven ...
This operator can also be used on objects to assign object references, as discussed in Creating Objects. The Arithmetic Operators. The Java programming language provides operators that perform addition, subtraction, multiplication, and division. There's a good chance you'll recognize them by their counterparts in basic mathematics.
As the Object class is the superclass of all Java classes, all Java objects can use the equals() ... Next, let's see which assignment operators we can use in Java. 9.1. The Simple Assignment Operator. The simple assignment operator (=) is a straightforward but important operator in Java. Actually, we've used it many times in previous examples.
Java is one of the most popular programming languages. It is an object-oriented programming language which means that we can create classes, objects, and many more. It also supports inheritance, polymorphism, encapsulation, and many more. It is used in all applications starting from mobile applications to web-based applications.
Object-oriented programming has several advantages over procedural programming: OOP is faster and easier to execute. OOP provides a clear structure for the programs. OOP helps to keep the Java code DRY "Don't Repeat Yourself", and makes the code easier to maintain, modify and debug. OOP makes it possible to create full reusable applications ...
Assigning one object to another just assigns the object reference (a pointer more or less). It does NOT copy member variables etc. You need to read about cloning.From the first paragraph of the wiki for Java clone():. In Java, objects are manipulated through reference variables, and there is no operator for copying an object—the assignment operator duplicates the reference, not the object.
Java Object Passing by Value or Reference to a Method Explained. You already learnt that Object assignment in Java happens by Reference. Let us try to pass Objects to another method as a parameter or argument. We try to know whether Objects are passed by Value or Reference to another Method using the below example.
Java is a statically and strongly typed language. This means: every variable has a type that is known at compile time and you can only assing values of that type (or sub-types, i.e. sub-classes) to this variable. Box b say "I want to have a variable named b and its (static) type is Box ". At run time, however, the object referenced by b can ...
Note: The compound assignment operator in Java performs implicit type casting. Let's consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5. Method 2: x += 4.5.
The javadoc for Class.isInstance(Object obj) gives the definition of assignment compatible:. Specifically, if this Class object represents a declared class, this method returns true if the specified Object argument is an instance of the represented class (or of any of its subclasses); it returns false otherwise.If this Class object represents an array class, this method returns true if the ...