Using @Mock as a Method Parameter with Mockito
As with many other Java developers, I heavily utilise Mockito as a mocking framework for unit testing.
I'll often end up with tests that look like this:
In this case, I want to create a List and check it's returned by the caching layer I'm testing.
However, this is a good example of where inlining our mock calls is bad, because IntelliJ warns us:
This is quite annoying, and pollutes our codebase with warnings that we really want to avoid.
One solution would be to make it a field of the test class, and @Mock it there, but in this case it's a throwaway mock that is only required in this test, not in all tests (therefore wouldn't make as much sense to make a field variable).
Fortunately, we can utilise something my colleague Lewis taught me the other week, and use @Mock as a method parameter when using JUnit5.
This gives us the following test:
This would of course, make our tests more readable if we had many mocks being set up!
Note that you also need to make sure you're using Mockito's mock setup:
This has been tested as of spring-boot-starter-test v2.2.4.RELEASE, and works in Mockito 2+ with JUnit5+.
I have raised a PR to add it to the Mockito 3.x documentation , but not 2.x as per request of the Mockito core team .
This post's permalink is https://www.jvt.me/posts/2020/06/27/mockito-mock-parameter/ and has the following summary:
Using `@Mock` on method parameters to reduce manual mock setups with Mockito.
The canonical URL for this post is https://www.jvt.me/posts/2020/06/27/mockito-mock-parameter/ .
Content for this article is shared under the terms of the Creative Commons Attribution Non Commercial Share Alike 4.0 International , and code is shared under the Apache License 2.0 .
# blogumentation # java # mockito .
This post was filed under articles .
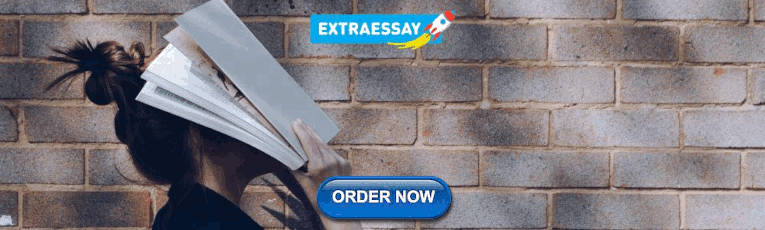
Interactions with this post
Below you can find the interactions that this page has had using WebMention .
Have you written a response to this post? Let me know the URL:
Do you not have a website set up with WebMention capabilities? You can use Comment Parade .
Kohei Nozaki's blog Notes of my experiments -->
- JUnit and Mockito tips
Posted on Friday Jul 03, 2020 at 06:40PM in Technology
In this entry I’ll share some tips about JUnit and Mockito for making unit tests better.
Mockito annotations
Mockito has some annotations that can be used for reducing redundancy of tests:
@InjectMocks
Before looking into the usage of those annotations, let’s assume we have the following production code which consists of 2 classes.
The first one is called MailServer, which has a method called send() that sends an SMTP message which this object receives as the parameter of the method. Note that the MailServer class most probably needs to be mocked out when you want to write a unit test for a class which uses the MailServer class because it really opens a TCP connection to an SMTP server, which is not a preferable thing for unit tests.
The other class is called Messenger, which depends on the MailServer class. This class requires an instance of the MailServer class in its constructor. This class has a method called sendMail(), which has 3 parameters. The responsibility of this method is first constructing an SMTP message based on those 3 parameters and then asking the MailServer object to send the SMTP message. It also does quick error handling which translates IOException into an unchecked one with embedding the content.
Let’s try writing a unit test for the Messenger class. But we don’t want to use the real MailServer class because it really tries to open a connection to an SMTP server. It will make testing harder because in order to test with the real MailServer class, we really need an SMTP server which is up and running. Let’s avoid doing that and try using a mocked version of a MailServer instance for the testing here.
A happy path test case would look like the following:
In the setUp() method, a mock MailServer object is created and injected into the constructor of the Messenger class. And in the test() method, first we create the expected SMTP message which the Messenger class has to create, then we call the sendMail() method and finally we verify that the send() method of the mock MailServer object has been called with the expected SMTP message.
With annotations, the test above can be written as follows:
First we annotate the test class with @ExtendWith(MockitoExtension.class) (Note that it’s a JUnit5 specific annotation, so for JUnit4 tests we need something different). Having the test class annotated with that one, when there is a field annotated with @Mock in the test class, Mockito will automatically create a mock for the field and inject it. And when there is a field annotated with @InjectMocks, Mockito will automatically create a real instance of the declared type and inject the mocks that are created by the @Mock annotation.
This is especially beneficial when many mock objects are needed because it reduces the amount of repetitive mock() method calls and also removes the need for creating the object which gets tested and injecting the mocks into the object.
And also it provides a clean way to create a mock instance of a class which has a parameterized type. When we create a mock instance of the Consumer class, a straightforward way would be the following:
The problem here is that it produces an unchecked assignment warning. Your IDE will complain about it and you will get this warning when the code compiles with -Xlint:unchecked :
With the @Mock annotation, we can get rid of the warning:
There is another useful annotation called @Captor. Let’s see the following test case:
The @Captor annotation creates an object called ArgumentCaptor which captures a method parameter of a method call of a mock object. In order to capture a parameter with an ArgumentCaptor, first we need to call the capture() method in a method call chain of the Mockito.verify() method. Then we can get the captured value with the getValue() method and we can do any assertion against it. It’s especially useful in a situation where checking the equality is not sufficient and a complex verification is needed.
AssertJ is an assertion library for unit tests written in Java. It provides better readability and richer assertions than its older equivalents like the one shipped with JUnit. Let’s see some example code from the official website :
A unique feature of AssertJ is that all of the assertions here begin with the method assertThat() which receives the parameter that gets asserted. After that we specify the conditions the parameter needs to fulfill. An advantage of this approach is that we can specify multiple conditions with method call chain. It’s more readable and less verbose than the old way where repetitive assertTrue() or assertEquals() calls are involved. It also provides rich assertions for widely used classes like List, Set or Map.
Another useful feature of AssertJ is for verifying an unhappy path where an Exception is involved. Let’s remember the production code we used in the Mockito annotation section and consider a situation where the MailServer cannot connect to the SMTP server. Due to that, the send() method throws IOException. In this situation, the Messenger class is expected to catch the IOException and translate it into UncheckedIOException with the SMTP message embedded. A unit test for this can be written as follows with AssertJ:
First we make the mock MailServer instance throw IOException when its send() method is called. After that we pass a lambda expression which calls the sendMail() method to the assertThatThrownBy() method of AssertJ. After that we can do various assertions. What we are checking here is that the sendMail() method throws UncheckedIOException with the SMTP message embedded and it also contains a parent Exception whose class is IOException.
We’ve discussed some tips about Mockito and the basic uses of AssertJ for test cases that are written in JUnit. Both Mockito and AssertJ have extensive documents and rich functionality which greatly helps writing unit tests. I highly recommend checking the references below:
Mockito framework site
AssertJ - fluent assertions java library
Practical Unit Testing: JUnit 5, Mockito, TestNG, AssertJ - book by Tomek Kaczanowski
The pieces of code which are used in this entry can be found on GitHub: https://github.com/nuzayats/junittips
Comments [0]
- ← JPA Builder パターン
- Java Generics wildca... →
Leave a Comment
You're viewing a weblog entry titled JUnit and Mockito tips . If you like this entry you might want to:
This is just one entry in the weblog Kohei Nozaki's blog . Why don't you visit the main page of the weblog?
Related entries
- The State Pattern
- Synchronize access to shared mutable data
- The Singleton Pattern in Java
- Favor composition over inheritance
- Java Generics wildcards
- JPA Builder パターン
- Jersey 1.x ExceptionMapper examples
- JPA Builder Pattern
- Jukito integration with JPA and guice-persist
- Managing multiple JPA persistence units with guice-persist
- In-container JMS consumer/producer example
- PrivateModule, TypeLiteral and AssistedInject - Google Guice techniques for complex scenarios
- jEdit conditional line deleting with regex
- My jEdit setup on OS X
- BeanShell recipies
- Testing guice-persist nested @Transactional behavior
- Java EE ブログエンジン Apache Roller のご紹介
- Adding indexes to tables of Apache James 3
- Notes about using UPSERT on RDBMS
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Most popular Mocking framework for unit tests written in Java
mockito/mockito
Folders and files, repository files navigation.
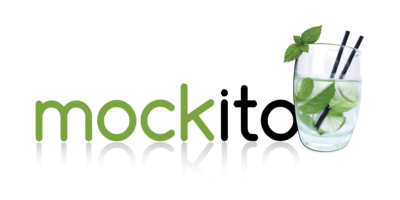
Most popular mocking framework for Java
Current version is 5.x
Still on Mockito 1.x? See what's new in Mockito 2! Mockito 3 does not introduce any breaking API changes, but now requires Java 8 over Java 6 for Mockito 2. Mockito 4 removes deprecated API. Mockito 5 switches the default mockmaker to mockito-inline, and now requires Java 11. Only one major version is supported at a time, and changes are not backported to older versions.
Mockito for enterprise
Available as part of the Tidelift Subscription.
The maintainers of org.mockito:mockito-core and thousands of other packages are working with Tidelift to deliver commercial support and maintenance for the open source dependencies you use to build your applications. Save time, reduce risk, and improve code health, while paying the maintainers of the exact dependencies you use. Learn more.
Development
Mockito publishes every change as a -SNAPSHOT version to a public Sonatype repository. Roughly once a month, we publish a new minor or patch version to Maven Central. For release automation we use Shipkit library ( http://shipkit.org ), Gradle Nexus Publish Plugin . Fully automated releases are awesome, and you should do that for your libraries, too! See the latest release notes and latest documentation . Docs in javadoc.io are available 24h after release. Read also about semantic versioning in Mockito .
Older 1.x and 2.x releases are available in Central Repository and javadoc.io (documentation).
More information
All you want to know about Mockito is hosted at The Mockito Site which is Open Source and likes pull requests , too.
Want to contribute? Take a look at the Contributing Guide .
Enjoy Mockito!
- Search / Ask question on stackoverflow
- Go to the mockito mailing-list (moderated)
- Open a ticket in GitHub issue tracker
How to develop Mockito?
To build locally:
To develop in IntelliJ IDEA you can use built-in Gradle import wizard in IDEA. Alternatively generate the importable IDEA metadata files using:
Then, open the generated *.ipr file in IDEA.
How to release new version?
- Every change on the main development branch is released as -SNAPSHOT version to Sonatype snapshot repo at https://s01.oss.sonatype.org/content/repositories/snapshots/org/mockito/mockito-core .
- In order to release a non-snapshot version to Maven Central push an annotated tag, for example:
- At the moment, you may not create releases from GitHub Web UI . Doing so will make the CI build fail because the CI creates the changelog and posts to GitHub releases. We'll support this in the future.
Security policy
Releases 209, sponsor this project, contributors 288.
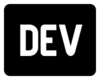
DEV Community

Posted on Jun 1, 2023
How to Avoid Unchecked Casts in Java Programs
Unchecked cast refers to the process of converting a variable of one data type to another data type without checks by the Java compiler.
This operation is unchecked because the compiler does not verify if the operation is valid or safe. Unchecked casts can lead to runtime errors, such as ClassCastException, when the program tries to assign an object to a variable of an incompatible type.
Hence, it is important to avoid unchecked casts in Java programs to prevent potential errors and ensure the program's reliability.
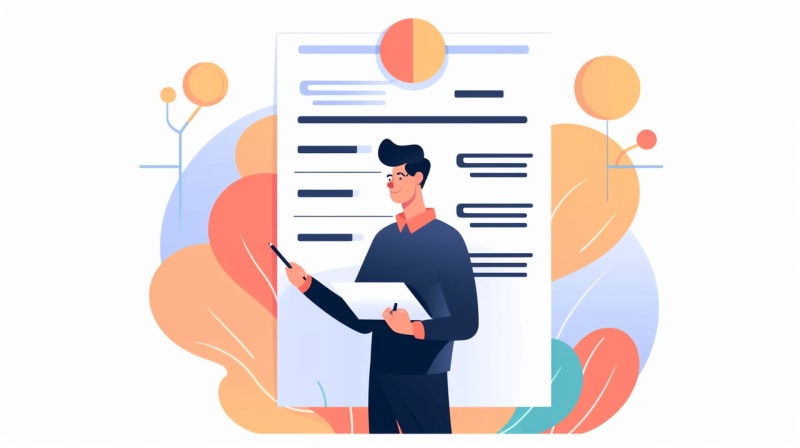
Consequences of Unchecked Casts
In Java programs, unchecked casts can lead to several issues. The most common problem is a ClassCastException at runtime, which occurs when we try to cast an object to a wrong type. This can cause the program to crash or behave unexpectedly.
Unchecked casts also violate the type safety of the Java language, which can lead to bugs that are difficult to detect and debug. Additionally, unchecked casts can make the code less readable and maintainable, as they hide the true type of objects and dependencies between components.
Therefore, it is important to avoid unchecked casts and use other mechanisms, such as generics or polymorphism, to ensure type safety and code quality in Java programs.
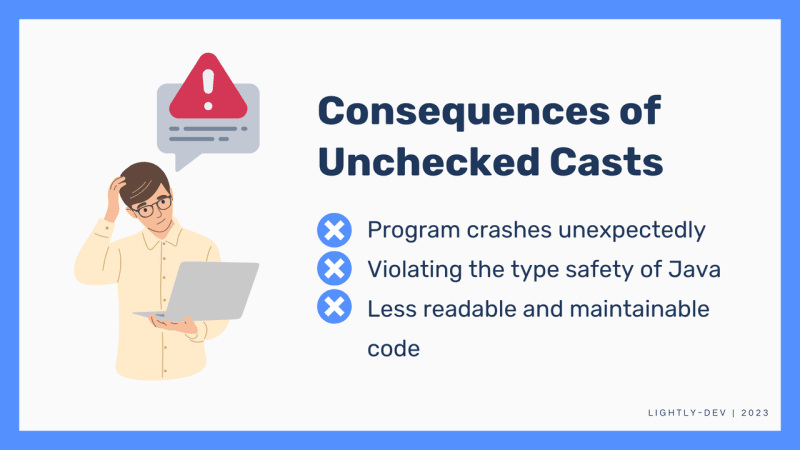
How Unchecked Casts Occur
Unchecked casts in Java programs occur when an object of one type is assigned to a reference of another type without proper type checking. This can happen when a programmer assumes that a reference to a superclass is actually a reference to its subclass and tries to cast it into that subclass. If the assumption is incorrect, the cast will result in a ClassCastException at runtime.
Unchecked casts can also occur when dealing with raw types, which are generic types without any type parameters specified. In such cases, the compiler cannot perform type checking and the programmer must ensure that the proper type conversions are made. Failing to do so can result in unchecked casts and potential runtime errors.
Why unchecked casts are problematic
In Java, unchecked casts allow a programmer to cast any object reference to any other reference type without providing any type information at compile-time. While this flexibility may seem useful, it can lead to serious run-time errors. If the object being casted is not actually of the type specified, a ClassCastException will occur at run-time.
Unchecked casts can cause difficult-to-debug errors in large and complex codebases, as it may not be immediately clear where the error originated. Additionally, unchecked casts can undermine Java's type system, creating code that is harder to read, maintain, and reason about. As a result, avoiding unchecked casts should be a priority when writing Java programs.
Examples of Unchecked Casts in Java
Unchecked casts are a common source of Java program errors. Here are some examples of unchecked casts:
This cast statement above can result in a class cast exception if the object referred to by obj is not a List.
In this case, the cast could fail at runtime if the array contains objects of a type other than String.
Finally, this cast could fail if the object referred to by obj is not a Map.
Using Generics to Avoid Unchecked Casts in Java
In Java, Generics is a powerful feature that allows you to write classes and methods that are parameterized by one or more types. Generics are a way of making your code more type-safe and reusable. With generics, you can define classes and methods that work on a variety of types, without having to write separate code for each type.
Using generics in Java programs has several advantages. It enables type safety at compile-time, which can prevent ClassCastException errors at runtime. With generics, the compiler can detect type mismatches and prevent them from happening, which leads to more robust and reliable code. It also allows for code reuse without sacrificing type safety and improve performance by avoiding unnecessary casting and allowing for more efficient code generation.
Generics allow Java developers to create classes and methods that can work with different data types. For example, a List can be defined to hold any type of object using generics. Here's an example:
In this example, we create a List that holds String objects. We can add String objects to the list and iterate over them using a for-each loop. The use of generics allows us to ensure type safety and avoid unchecked casts. Another example is the Map interface, which can be used to map keys to values of any data type using generics.
Using the instanceof operator to Avoid Unchecked Casts in Java
The instanceof operator is a built-in operator in Java that is used to check whether an object is an instance of a particular class or interface. The operator returns a boolean value - true if the object is an instance of the specified class or interface, and false otherwise.
The instanceof operator is defined as follows:
where object is the object that is being checked, and class/interface is the class or interface that is being tested against.
The instanceof operator can be useful in situations where we need to perform different operations based on the type of an object. It provides a way to check the type of an object at runtime, which can help prevent errors that can occur when performing unchecked casts.
Here are some examples of using the instanceof operator:
In this example, we use the instanceof operator to check whether the object obj is an instance of the String class. If it is, we perform an explicit cast to convert the object to a String and call the toUpperCase() method on it.
In this example, we use the instanceof operator to check whether the List object passed as a parameter is an instance of the ArrayList or LinkedList classes. If it is, we perform an explicit cast to convert the List to the appropriate class and perform different operations on it depending on its type.
Overall, using the instanceof operator can help us write more robust and flexible code. However, it should be used judiciously as it can also make code harder to read and understand.
Using Polymorphism to Avoid Unchecked Casts in Java
Polymorphism is a fundamental concept in object-oriented programming. It refers to the ability of an object or method to take on multiple forms. It allows us to write code that can work with objects of different classes as long as they inherit from a common superclass or implement a common interface. This helps to reduce code duplication and makes our programs more modular and extensible.
Some of the advantages of using polymorphism are:
- Code reusability: We can write code that can work with multiple objects without having to rewrite it for each specific class.
- Flexibility: Polymorphism allows us to write code that can adapt to different types of objects at runtime.
- Ease of maintenance: By using polymorphism, changes made to a superclass or interface are automatically propagated to all its subclasses.
Here are a few examples of how you can use polymorphism to avoid unchecked casts in Java:
Example 1: Shape Hierarchy
In this example, the abstract class Shape defines the common behavior draw(), which is implemented by the concrete classes Circle and Rectangle. By using the Shape reference type, we can invoke the draw() method on different objects without the need for unchecked casts.
Example 2: Polymorphic Method Parameter
In this example, the makeAnimalSound() method accepts an Animal parameter. We can pass different Animal objects, such as Dog or Cat, without the need for unchecked casts. The appropriate implementation of the makeSound() method will be invoked based on the dynamic type of the object.
By utilizing polymorphism in these examples, we achieve type safety and avoid unchecked casts, allowing for cleaner and more flexible code.
Tips to Avoid Unchecked Casts in Java Programs
Unchecked casts in Java programs can introduce runtime errors and compromise type safety. Fortunately, there are several techniques and best practices you can employ to avoid unchecked casts and ensure a more robust codebase. Here are some effective tips to help you write Java programs that are type-safe and free from unchecked cast exceptions.
- Use generic classes, interfaces, and methods to ensure that your code handles compatible types without relying on casting.
- Embrace polymorphism by utilizing abstract classes and interfaces, define common behavior and interact with objects through their common type.
- Check the type of an object using the instanceof operator. This allows you to verify that an object is of the expected type before proceeding with the cast.
- Favor composition over inheritance, where classes contain references to other classes as instance variables.
- Familiarize yourself with design patterns that promote type safety and avoid unchecked casts. Patterns such as Factory Method, Builder, and Strategy provide alternative approaches to object creation and behavior, often eliminating the need for explicit casting.
- Clearly define the contracts and preconditions for your methods. A well-defined contract helps ensure that the method is called with appropriate types, improving overall code safety.
- Refactor your code and improve its overall design. Look for opportunities to apply the aforementioned tips, such as utilizing generics, polymorphism, or design patterns.
Unchecked casts in Java programs can introduce runtime errors and undermine type safety. By adopting practices like using generics, leveraging polymorphism, checking types with instanceof, favoring composition over inheritance, reviewing design patterns, employing design by contract, and improving code design, you can avoid unchecked casts and enhance the robustness of your Java programs. Prioritizing type safety will result in more reliable code and a smoother development process.
Lightly IDE as a Programming Learning Platform
So, you want to learn a new programming language? Don't worry, it's not like climbing Mount Everest. With Lightly IDE, you'll feel like a coding pro in no time. With Lightly IDE , you don't need to be a coding wizard to start programming.
One of its standout features is its intuitive design, which makes it easy to use even if you're a technologically challenged unicorn. With just a few clicks, you can become a programming wizard in Lightly IDE. It's like magic, but with less wands and more code.
If you're looking to dip your toes into the world of programming or just want to pretend like you know what you're doing, Lightly IDE's online Java compiler is the perfect place to start. It's like a playground for programming geniuses in the making! Even if you're a total newbie, this platform will make you feel like a coding superstar in no time.
Read more: How to Avoid Unchecked Casts in Java Programs
Top comments (0)
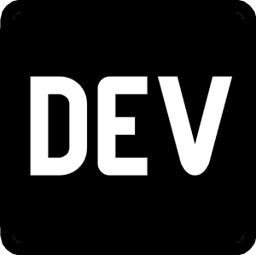
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
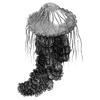
How To Create Ai Bot With Whatsapp
ellalily - Apr 23
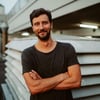
Using eBPF and XDP to Share Default DNS Port Between Multiple Resolvers
Misha Bragin - Apr 23
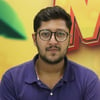
Laravel DUSK
Imran Yahya - Apr 23
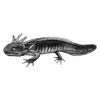
Data Engineering - Practicing for fun
Hiromi - Apr 23
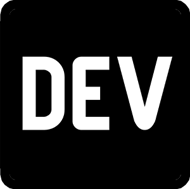
We're a place where coders share, stay up-to-date and grow their careers.
Class MockitoExtension
Constructor summary, method summary, methods inherited from class java.lang. object, constructor details, mockitoextension, method details, supportsparameter, resolveparameter.
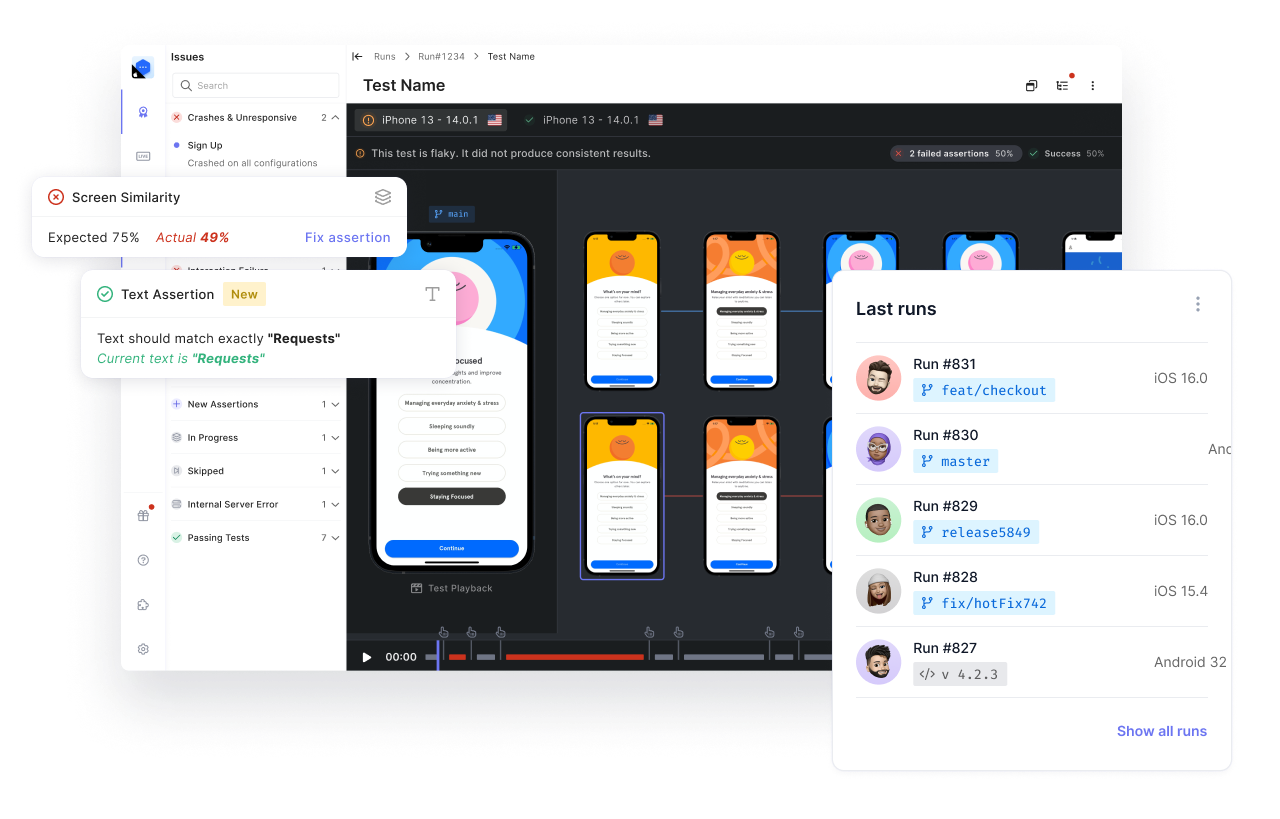
Get true E2E testing in minutes, not months.
How to use mockito to mock a generic class.
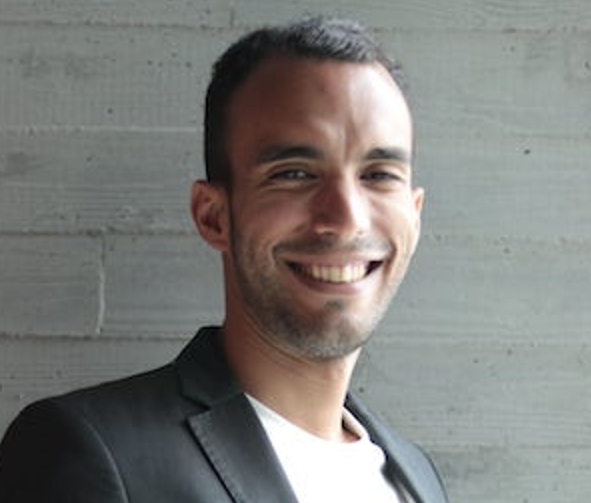
This article will explore how to use the Mockito library to mock a generic class in Java. We will start by explaining what Mockito is and how to use it. Then we will explore the @Mock annotation in Java for mock tests . After that, we will delve into the particulars of using Mockito in a generic class. Finally, we will list the advantages and disadvantages of using a library like Mockito in Java.
Let's jump right in.
.png)
What Is Mockito?
Mockito is a popular Java library that allows you to create mock objects for testing purposes . Mocking is a technique that lets you replace the functionality of an object or class with a simulated version, which can help test how your code interacts with other components.
To mock a generic class with Mockito, you'll need to do the following:
Create a mock object of the generic class using the Mockito.mock() method.
Set up the desired behavior of the mock object using Mockito's when() and thenReturn() methods.
This will cause the mock object to return "Hello, world!" whenever the getValue() method is called.
Use the mock object in your test code like any other object.
Verify that the mock object was used as expected using the Mockito.verify() method.
This will ensure that the getValue() method is called exactly once on the mock object.
Using the @Mock Annotation
The @Mock annotation is a convenient way to create mock objects in Mockito. It allows you to create and inject mock objects into your test classes without manually calling the Mockito.mock() method.
To use the @Mock annotation, you'll need to do the following:
Annotate a field in your test class with @Mock .
This will create a mock object of the specified type and assign it to the field.
Initialize the mock objects in your test class using the MockitoAnnotations.initMocks() method.
This will initialize all fields in your test class annotated with @Mock and assign them their corresponding mock objects.
Use the mock object in your test methods as you would any other object.
The @Mock annotation is a convenient way to create and manage mock objects in your tests and can save you time and boilerplate code when setting up mock objects.
Keep in mind that the @Mock annotation only creates mock objects. It does not set up their behavior. So you'll still need to use the when() and thenReturn() methods or another method of specifying the mock object's behavior, such as the Answer interface or a custom Answer implementation.
Using Mockito on a Generic Class
Using Mockito to mock a generic class can be more complicated than mocking a regular class due to the added complexity of generics. However, with a bit of practice and an understanding of how Mockito works, you should be able to use it to mock generic classes in your tests effectively.
One important thing to keep in mind when mocking generic classes is that Mockito's mock() method uses type erasure to create the mock object. This means that the type information of the generic class is not preserved at runtime, which can cause issues if you try to use the mock object in a way that relies on the specific type parameters of the class.
For example, consider the following generic class:
If you create a mock object of this class using Mockito's mock() method, Mockito will replace the type parameter T with the type Object at runtime. Unfortunately, if you try to call a method on the mock object that takes an argument of type T , you'll get a compile-time error because the mock object doesn't have a corresponding method that takes an Object argument.
To work around this issue, you can use Mockito's @Captor annotation to create an ArgumentCaptor object that can capture the arguments passed to the mock object's methods. You can then use the ArgumentCaptor to access the captured arguments and verify that they have the expected type.
For example:
This code sets up the mock object to return true whenever the setValue() method is called with any argument and then verifies that the setValue() method was called with the correct argument using the ArgumentCaptor .
Mocking Using the Answer Interface
Another option for mocking generic classes is to use Mockito's Answer interface, which allows you to specify a custom behavior for the mock object's methods. This can be useful if you need to perform more complex operations or use the type information of the generic class in your mock object's behavior.
This code sets up the mock object to return the argument passed to the getValue() method as the return value.
To use the Answer interface, you'll need to create a new implementation of the Answer interface and override the answer() method. The answer() method takes an InvocationOnMock object as an argument, representing the method call made on the mock object. You can use the InvocationOnMock object to access the arguments passed to the method and perform any desired operations.
Here's an example of how you might use the Answer interface to mock a generic class:
You can then use this Answer implementation to set up the desired behavior of your mock object like this:
This will cause the mock object to return the transformed argument whenever the getValue() method is called.
.png)
Mockito's Advantages and Disadvantages
Here are some advantages of using Mockito:
- It's easy to use. Mockito has a simple and intuitive API that makes it easy to create and use mock objects in your tests.
- It's widely used. Mockito is a popular library with a large user base, which means it's well tested and has a wealth of documentation and resources available.
- It integrates well with other tools. Mockito works well with other testing tools and libraries, such as JUnit and PowerMock.
- It supports a variety of mock object types. Mockito allows you to create mock objects of various types, including regular classes, interfaces, and final classes.
Some potential disadvantages of using Mockito include the following:
- Mocking generic classes: As mentioned earlier, Mockito's mock() method uses type erasure, making it difficult to mock generic classes in a way that preserves their type information.
- Mocking final classes and methods: Mockito cannot mock final classes or methods, which can be a limitation if you need to test code that relies on these classes or methods.
- Mocking static methods: Mockito does not directly support the mocking of static methods, although this can be done using the PowerMock library in combination with Mockito.
- Mocking complex behavior: While Mockito is good at mocking simple behavior, it can be more challenging to mock complex or nuanced behavior, which may require more manual setup or custom Answer implementations.
Mockito is a powerful tool for creating mock objects in Java to mock generic and regular classes. By using the when() and thenReturn() methods, the @Captor annotation, and the Answer interface, you can set up the desired behavior of your mock objects. This will allow you to test your code in various situations.
However, I advise you to check out Waldo's extensive toolkit for UI testing if you want to make sure that your code is reliable and safe. Even for nondevelopers, it is incredibly accessible and doesn't require any code.
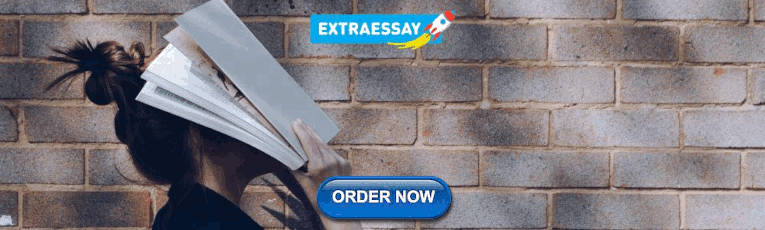
Reproduce, capture, and share bugs fast!
Script your first test in minutes, automated e2e tests for your mobile app.

Appium Tutorial: Your Complete Intro to Mobile Testing
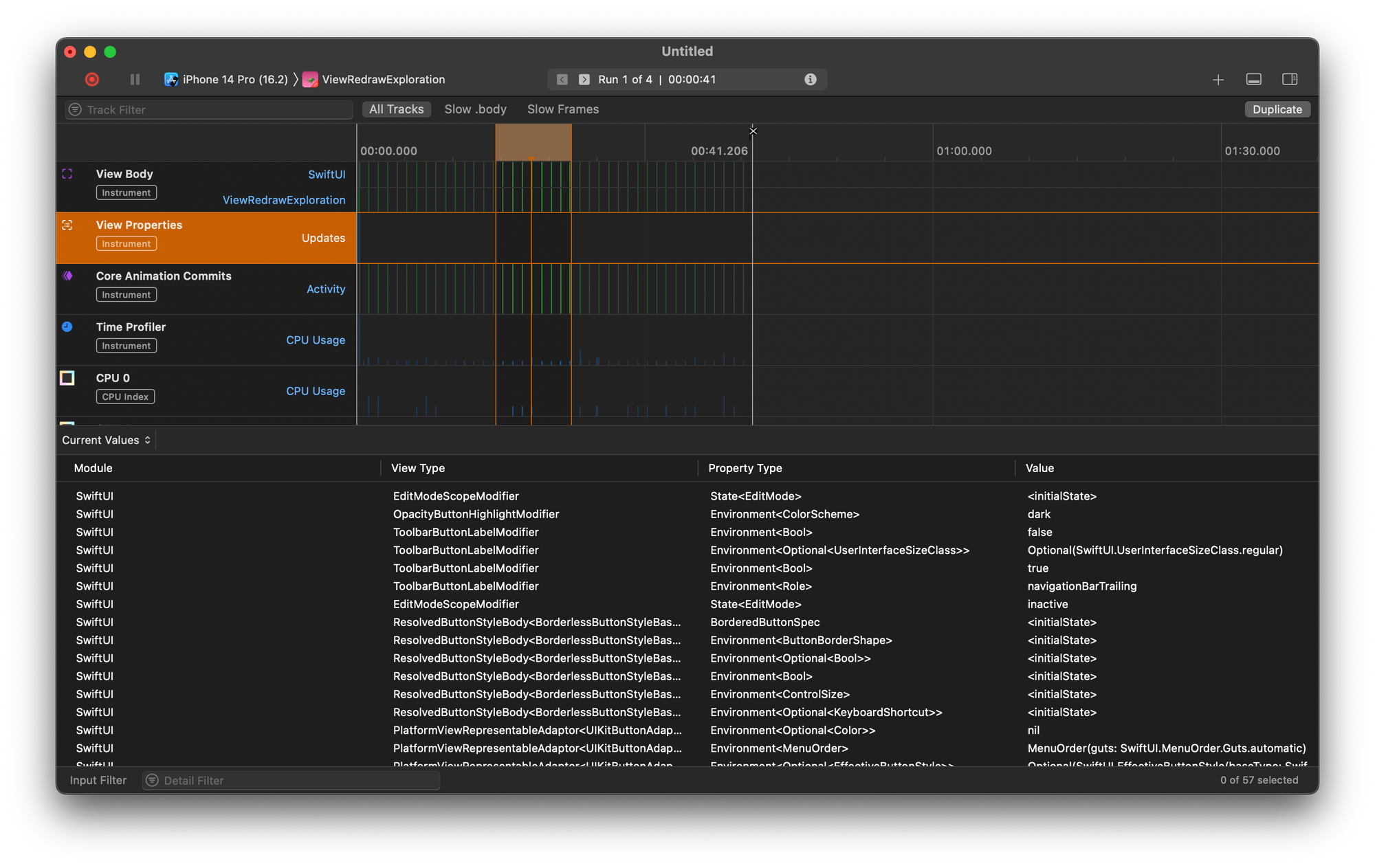
Profiling your SwiftUI apps with instruments
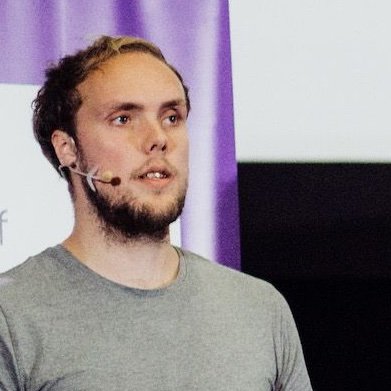
Updating CocoaPods: A Step-By-Step Guide
How to fix this unchecked assignment warning?

I got Warning:(31, 46) Unchecked assignment: 'java.lang.Class' to 'java.lang.Class<? extends PACKAGE_NAME.Block>' warning on the line blockRta.registerSubtype(c); , but I can’t figure out how to fix that without supressing it.
ReflectionHelper.getClasses is a static method to get all the classes in that package name, and its return type is Class[] . Block is an interface. RuntimeTypeAdapterFactory is a class in gson extra, and its source code can be viewed here .
Advertisement
Since ReflectionHelper.getClasses returns an array of the raw type Class , the local-variable type inference will use this raw type Class[] for var blks and in turn, the raw type Class for var c . Using the raw type Class for c allows passing it to registerSubtype(Class<? extends Block>) , without any check, but not without any warning. You can use the method asSubclass to perform a checked conversion, but you have to declare an explicit non-raw variable type, to get rid of the raw type, as otherwise, even the result of the asSubclass invocation will be erased to a raw type by the compiler.
There are two approaches. Change the type of blks :
Then, the type of var c changes automatically to Class<?> .
Or just change the type of c :
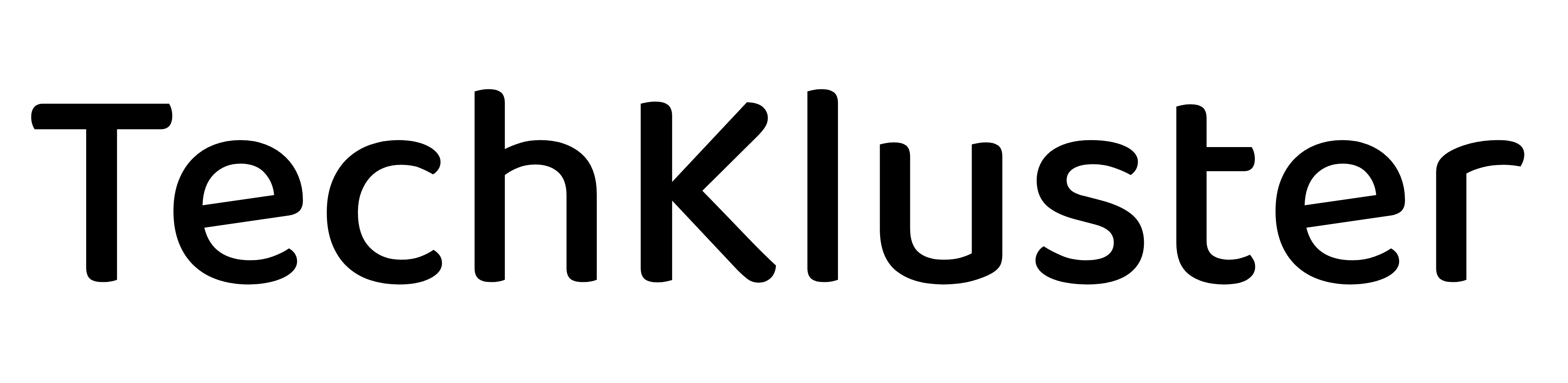
Mocking Exception Throwing using Mockito
- By Syed Wahaj
Table of Contents

Exception handling is an essential aspect of writing robust and reliable code. In the world of Java development, the Mockito framework provides a powerful toolset for creating mock objects and simulating various scenarios, including the throwing of exceptions. In this article, we will delve into how to mock exception throwing using Mockito, a popular Java testing library. We’ll cover the basic concepts, the benefits of using such techniques, and provide relevant code examples.
Understanding Mocking and Mockito
Mocking involves simulating the behavior of objects or components within your codebase. Mockito is a widely-used Java testing library that enables developers to create mock objects, set expectations on their behavior, and verify interactions. One common scenario is mocking the throwing of exceptions during tests, allowing developers to validate the proper handling of exceptional cases.
Benefits of Mocking Exception Throwing
- Isolating Scenarios : Mocking exception throwing lets you isolate specific scenarios within your codebase. By artificially triggering exceptions, you can ensure that your code responds correctly and gracefully to error conditions.
- Test Coverage : Robust exception handling is a crucial aspect of software quality. Mocking exception throwing enhances your test suite’s coverage, ensuring that even rare or edge-case exceptions are accounted for.
- Error Recovery : Through testing exception handling paths, you can identify potential issues and enhance the error recovery mechanisms of your application.
Getting Started with Mockito
Before diving into mocking exception throwing, ensure that you have the Mockito library added to your project’s dependencies. You can typically achieve this by adding the following Maven dependency:
Mocking Exception Throwing: Example
Suppose we have a simple Calculator class that performs division. We want to test how it handles division by zero exceptions. Here’s how we can achieve this using Mockito:
In this example, we’ve created a mock Calculator object using mock() . We then use the when(...).thenThrow(...) syntax to define the behavior when a specific method is invoked. In this case, we’re specifying that when the divide method is called with any value and a divisor of 0.0, it should throw an ArithmeticException .
Advanced Exception Handling Testing
While the previous section demonstrated a basic example of mocking exception throwing, Mockito offers more advanced features for handling exceptions during testing.
Stubbing Multiple Calls
Mockito allows you to stub multiple calls with different behaviors. This can be particularly useful for simulating progressive behavior or sequential exceptions. Let’s take a look at how this can be done:
In this example, the processFile method is stubbed to throw a FileNotFoundException , then an IOException , and finally to return “Processed”. This simulates a scenario where the file processing encounters different exceptions in consecutive attempts.
Verifying Exception Messages
You can use Mockito to verify the messages associated with thrown exceptions. This ensures that the correct messages are being set when exceptions are thrown. Here’s how you can achieve this:
In this example, the createUser method is stubbed to throw an IllegalArgumentException with a specific message. You can then perform assertions to verify that the exception message is being set correctly.
Best Practices
When using Mockito to mock exception throwing, keep the following best practices in mind:
- Be Specific : Mock only the exceptions that are relevant to the test scenario. Over-mocking can make your tests less clear and harder to maintain.
- Combine with Regular Testing : Mocking exception throwing is a powerful tool, but don’t rely solely on it. Regular testing of exception handling paths is still essential.
- Use with Caution : Mocking exception throwing should not replace testing against actual exceptions that could occur in production.
- Document Intent : Clearly document your intentions when mocking exception throwing. Use meaningful method and variable names to enhance code readability.
Mockito’s capabilities extend beyond basic mock object creation, providing a comprehensive toolkit for mocking exception throwing and verifying the handling of exceptional scenarios. By taking advantage of these advanced features, you can craft thorough tests that ensure your code gracefully handles exceptions and exceptional scenarios. As you integrate these practices into your testing approach, you’ll contribute to building more robust, resilient, and high-quality Java applications.
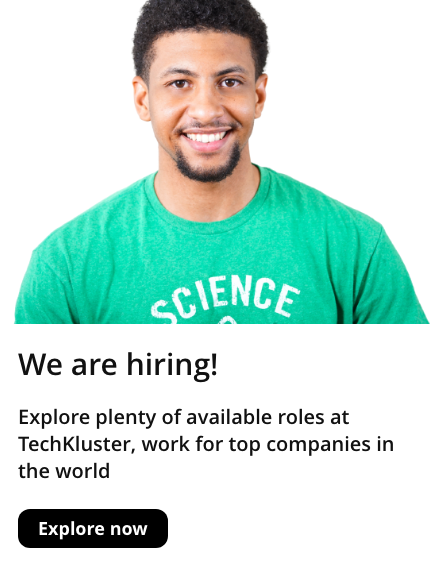
Undefined vs Null in JavaScript
JavaScript, as a dynamically-typed language, provides two distinct primitive values to represent the absence of a meaningful value: undefined and null. Although they might seem
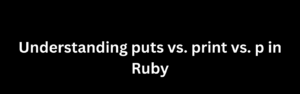
Understanding puts vs. print vs. p in Ruby
Ruby, a dynamic, object-oriented programming language, offers several methods for outputting information to the console. Among the commonly used ones are puts, print, and p.
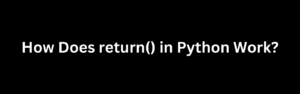
How Does return() in Python Work?
In Python, the return statement plays a fundamental role in functions. It allows a function to send back a result to the caller, thereby terminating
TechKluster
- Schedule a call
- How it works
- Hire Resources
- Apply for Jobs
- CV Writing Tips
- Terms and Conditions
- Privacy Policy
- Frequently Asked Questions
- Customer Support
Remote Job Roles
- Java Developers
- Python Developers
- Angular Developers
- ReactJS Developers
- iOS Developers
- Android Developers
- PHP Developers
- NodeJS Developers
- GCP Developers
- GoLang Developers
- React Native Developers
- Spring Developers
- .Net Developers
- ASP.Net Developers
- Azure Developers
@2023 techkluster
- Swift Developers
- Docker Developers
- Golang Developers
@2022 techkluster
Mocking Generic Types
Java's generic type system does not work well with its dynamic reflection APIs. For jMock, this means that the compiler will warn you about possible static type errors when you mock generic types. The warnings are incorrect. The best way to avoid them is to suppress the warning with an annotation on the variable declaration of the mock object.
Consider, for example, the following generic interface:
In a test, you would want to mock this interface as follows:
However, that is not syntactically correct Java. You actually have to write the following:
Both those lines, though syntactically and semantically correct, generate type safety warnings. To avoid these warnings, you have to annotate the variable declarations with @SuppressWarnings :
Hopefully future versions Java will improve the type system so that this is not necessary.
jMock 2 (Java 1.6)
- Stable: 2.6.1
jMock 1 (Java 1.3+)
- Stable: 1.2.0
Source Repository
Project License
Version Numbering
Documentation
Getting Started
Cheat Sheet
API Documentation
Articles and Papers
jMock 1 Documentation
About Mock Objects
User Support
Mailing Lists
Issue Tracker
News Feed (RSS 2.0)
Development Team
Project hosted by Github
Icons by the Tango Project
Understanding Java unchecked cast Warning
Introduction §
The unchecked cast warning indicates potential ClassCastExceptions may occur if incompatible types exist in a raw type being cast. This article explores when this warning occurs, why it appears, and how to properly address it.
When Does the Warning Occur? §
An unchecked cast warning occurs when casting from a raw type to a parameterized type without type checking at runtime. For example:
Adding incompatible types to the rawMap allows the unchecked cast to still happen:
Retrieving the wrong type from castMap then throws a ClassCastException:
So the warning indicates the potential for this exception.
Here is an updated section with more details and an example on why the compiler warns about unchecked casts in Java:
Why Does the Compiler Warn? §
Using raw types circumvents compile-time type checking, which is why the compiler warns about unchecked casts.
For example, consider the following code:
When we cast the raw Map to Map<String, Integer>, the compiler cannot actually verify that the types are compatible. Since the rawMap only contained strings, the cast seems valid.
However, nothing prevents another developer from adding incompatible types to the rawMap later on:
Now the rawMap contains both Strings and Dates. But since the cast occurred without type checks, this incompatibility goes undetected.
Later on when retrieving values from the casted typedMap, a ClassCastException will be thrown:
So unchecked casts allow incompatible types to go undetected at compile time and end up causing runtime exceptions. The compiler warns to indicate this danger.
Proper use of generics provides compile-time type safety that prevents these issues. But with raw types, unchecked casts can hide bugs until runtime.
How to Address the Warning §
There are a few ways to handle unchecked cast warnings:
1. Avoid Raw Types Entirely §
Ideally, avoid using raw types and favor generic types for type safety:
2. Use @SuppressWarnings Annotation §
Use @SuppressWarnings("unchecked") if certain the cast is safe:
Only suppress warnings if thoroughly reviewed. Use sparingly.
3. Manually Check Types §
Manually check types before accessing the casted collection:
This fails fast if types are incompatible.
Comparison in Java Versions §
Later Java versions add features like generics, diamond operators, and type inference to reduce raw type usage. This provides increased compile-time type safety and decreases unchecked casts.
Conclusion §
Heed unchecked cast warnings to avoid runtime ClassCastExceptions. Use generics, suppress carefully, and check types manually. This results in code that fails fast and makes issues easier to trace.
- Revolutionize Your Data Analysis with Java tablesaw table
- An In-Depth Guide to StringList Java
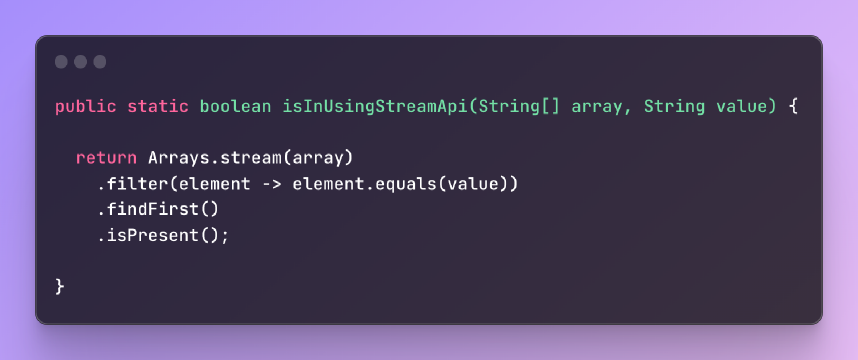
- Computer Science
- Cryptography
- Cyclic Codes
- Programming
- Applications
- Binary Data
- Data Retrieval
- Image Storage
- Language Display
- Operating Systems
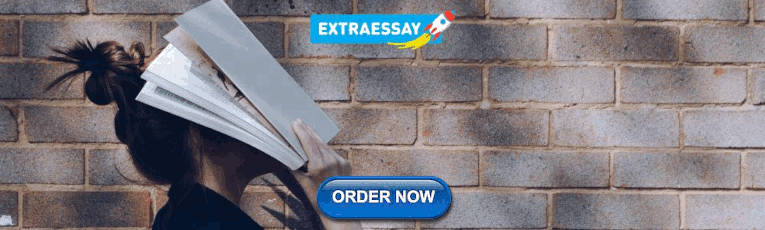
IMAGES
VIDEO
COMMENTS
Map<Integer, String> map = a.getMap(); gets you a warning now: "Unchecked assignment: 'java.util.Map to java.util.Map<java.lang.Integer, java.lang.String>'. Even though the signature of getMap is totally independent of T, and the code is unambiguous regarding the types the Map contains. I know that I can get rid of the warning by reimplementing ...
Mockito.any(TimeUnit.class)) ).thenReturn(future); // <-- warning here. except that I'm getting unchecked warning in the last line: missing type arguments for generic class java.util.concurrent.ScheduledFuture<V>. unchecked method invocation: method thenReturn in interface org.mockito.stubbing.OngoingStubbing is applied to given types.
Now all the warnings go away except one, which is on the mockmethod: Unchecked assignment: java.util.List to java.util.List<String> ... The type of the mock is inferred from the assignment, which ...
However, this is a good example of where inlining our mock calls is bad, because IntelliJ warns us: Unchecked assignment: 'java.util.List' to 'java.util.List<me.jvt.www.api.analytics.model.Page>' This is quite annoying, and pollutes our codebase with warnings that we really want to avoid.
Generally speaking, the name of a mock has nothing to do with the working code. However, it may be helpful in debugging, as we use the mock's name to track down verification errors. To ensure the exception message thrown from an unsuccessful verification includes the provided name of a mock, we'll use assertThatThrownBy.
In this quick tutorial, we'll focus on how to configure a method call to throw an exception with Mockito. For more information on the library, also check out our Mockito series.. Here's the simple dictionary class that we'll use:
The problem here is that it produces an unchecked assignment warning. Your IDE will complain about it and you will get this warning when the code compiles with -Xlint:unchecked : Warning:(35, 49) java: unchecked conversion required: java.util.function.Consumer<java.lang.String> found: java.util.function.Consumer
The "unchecked cast" is a compile-time warning . Simply put, we'll see this warning when casting a raw type to a parameterized type without type checking. An example can explain it straightforwardly. Let's say we have a simple method to return a raw type Map: public class UncheckedCast {. public static Map getRawMap() {.
See what's new in Mockito 2! Mockito 3 does not introduce any breaking API changes, but now requires Java 8 over Java 6 for Mockito 2. Mockito 4 removes deprecated API. Mockito 5 switches the default mockmaker to mockito-inline, and now requires Java 11.
This will result in having all test data within the test method. This will keep your test classes clean as there are no 'floating' mock's. @ExtendWith(MockitoExtension.class) public class SomeClassTest { @Test void someTestMethod(@Mock Foo<Bar> fooMock) { // do something with your mock } }
Unchecked cast refers to the process of converting a variable of one data type to another data type without checks by the Java compiler. This operation is unchecked because the compiler does not verify if the operation is valid or safe. Unchecked casts can lead to runtime errors, such as ClassCastException, when the program tries to assign an ...
Use parameters for initialization of mocks that you use only in that specific test method. In other words, where you would initialize local mocks in JUnit 4 by calling Mockito.mock(Class), use the method parameter. This is especially beneficial when initializing a mock with generics, as you no longer get a warning about "Unchecked assignment".
To mock a generic class with Mockito, you'll need to do the following: Create a mock object of the generic class using the Mockito.mock () method. MyGenericClass<String> mock = Mockito.mock(MyGenericClass.class); Set up the desired behavior of the mock object using Mockito's when () and thenReturn () methods.
Answer. Since ReflectionHelper.getClasses returns an array of the raw type Class, the local-variable type inference will use this raw type Class[] for var blks and in turn, the raw type Class for var c. Using the raw type Class for c allows passing it to registerSubtype(Class<? extends Block>), without any check, but not without any warning.
5.2. Checking Type Conversion Before Using the Raw Type Collection. The warning message " unchecked conversion " implies that we should check the conversion before the assignment. To check the type conversion, we can go through the raw type collection and cast every element to our parameterized type.
Exception handling is an essential aspect of writing robust and reliable code. In the world of Java development, the Mockito framework provides a powerful toolset for creating mock objects and simulating various scenarios, including the throwing of exceptions. In this article, we will delve into how to mock exception throwing using Mockito, a popular Java testing library.
For jMock, this means that the compiler will warn you about possible static type errors when you mock generic types. The warnings are incorrect. The best way to avoid them is to suppress the warning with an annotation on the variable declaration of the mock object. Consider, for example, the following generic interface: Liquid juice(T fruit);
Of course that's an unchecked assignment. You seem to be implementing a heterogeneous map. Java (and any other strongly-typed language) has no way to express the value type of your map in a statically-type-safe way. That is because the element types are only known at runtime.
Some common checked exceptions in Java are IOException, SQLException and ParseException. The Exception class is the superclass of checked exceptions, so we can create a custom checked exception by extending Exception: public IncorrectFileNameException(String errorMessage) {. super (errorMessage); 3.
There are a few ways to handle unchecked cast warnings: 1. Avoid Raw Types Entirely. Ideally, avoid using raw types and favor generic types for type safety: 1 Map<String, LocalDate> safeMap = new HashMap<>(); java. 2. Use @SuppressWarnings Annotation. Use @SuppressWarnings("unchecked") if certain the cast is safe: