Create MS PowerPoint Presentations in C#
MS PowerPoint presentations allow you to create slide shows containing text, images, charts, animations, and other elements. Various additional formatting options let you make your presentations more appealing. In this post, you will come to know how to create PowerPoint PPT PPTX in C# . You will learn how to insert text, tables, images, and charts in PowerPoint PPT in programmatically in C# .
- C# PowerPoint API
- Create a PowerPoint Presentation
- Open an Existing PowerPoint Presentation
- Add Slide to a Presentation
- Add Text to Presentation’s Slide
- Create a Table in Presentation
- Create a Chart in Presentation
- Add an Image in Presentation
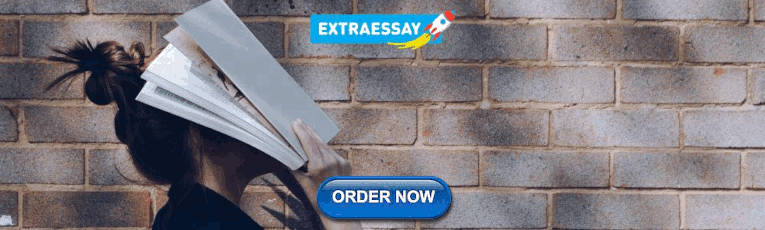
C# API to Create PowerPoint PPT - Free Download #
Aspose.Slides for .NET is a presentation manipulation API that lets you create and manipulate PowerPoint documents from within your .NET applications. The API provides nearly all possible features required to implement basic as well as advanced PowerPoint automation features. You can either download the API or install it via NuGet .
Create a PowerPoint PPT in C# #
Let’s start by creating an empty PowerPoint PPT/PPTX presentation using Aspose.Slides for .NET. The following are the steps to do so.
- Create an instance of the Presentation class.
- Save it as PPTX using Presentation.Save(String, SaveFormat) method.
The following code sample shows how to create a PowerPoint presentation in C#.
Open an Existing PowerPoint Presentation in C# #
You don’t need to put extra efforts in order to open an existing PowerPoint presentation. Simply provide the path of the PPTX file to the constructor of the Presentation class and you are done. The following code sample shows how to open an existing PPTX presentation.
Add a Slide to PPT in C# #
Once you have created the presentation, you can start adding the slides to it. The following are the steps to add a slide in the presentation using Aspose.Slides for .NET.
- Instantiate ISlideCollection class by setting a reference to the Presentations.Slides property.
- Add an empty slide to the presentation using Slide.AddEmptySlide(ILayoutSlide) method exposed by ISlideCollection object
- Save the presentation file using the Presentation.Save(String, SaveFormat) method.
The following code sample shows how to add a slide in a PowerPoint presentation using C#.
Insert Text in a PPT Slide using C# #
Now we can add content to the slides in the PowerPoint presentation. Let’s first add a piece of text to the slide using the following steps.
- Create a new presentation using the Presentation class.
- Obtain the reference of the slide in the presentation.
- Add an IAutoShape with ShapeType as Rectangle at a specified position of the slide.
- Obtain the reference of that newly added IAutoShape object.
- Add a TextFrame to the AutoShape containing the default text.
- Save the presentation as a PPTX file.
The following code sample shows how to add text to slide in a presentation using C#.
Create Table in a PPT Presentation using C# #
Aspose.Slides for .NET provides an easy way to create a table in the presentation document. The following are the steps for it.
- Obtain the reference of a slide by using its index.
- Define arrays of columns with width and rows with height.
- Add a table to the slide using Slide.Shapes.AddTable() method exposed by IShapes object and get the reference to the table in ITable instance.
- Iterate through each cell to apply the formatting.
- Add text to the cells using Table.Rows[][].TextFrame.Text property.
The following code sample shows how to create table in a slide of PowerPoint presentation.
Create Chart in a PowerPoint PPT using C# #
The following are the steps to add a chart in PowerPoint presentation using C#.
- Obtain the reference of a slide by index.
- Add a chart with the desired type using ISlide.Shapes.AddChart(ChartType, Single, Single, Single, Single) method.
- Add a chart title.
- Access the chart data worksheet.
- Clear all the default series and categories.
- Add new series and categories.
- Add new chart data for chart series.
- Set fill color for chart series.
- Add chart series labels.
The following code sample shows how to add chart in a presentation using C#.
Learn more about the presentation charts here .
Add an Image in PowerPoint Presentation #
The following are the steps to add images in the presentation slide.
- Create a new presentation using Presentation class.
- Read SVG image using File.ReadAllText(String path) method.
- Add image to slide using Presentation.Slides[0].Shapes.AddPictureFrame(ShapeType shapeType, float x, float y,float width, float height, IPPImage image) method.
- Save the presentation.
The following code sample shows how to add image to a presentation in C#.
C# .NET PowerPoint API - Get a Free License #
You can use Aspose.Slides for .NET without evaluation limitations by getting a free temporary license .
Conclusion #
In this article, you have learned how to create PowerPoint PPT presentations from scratch using C#. In addition, you have seen how to add slides, text, tables, images, and charts in new or existing PPTX presentations. You can learn more about the API using the documentation .
- Protect PowerPoint PPTX Presentations using C#
- Create MS PowerPoint Presentations in ASP.NET
- Convert PowerPoint PPTX/PPT to PNG Images in C#
- Set Slide Background in PowerPoint Presentations using C#
- Generate Thumbnails for PowerPoint PPTX or PPT using C#
- Apply Animation to Text in PowerPoint using C#
- Split PowerPoint Presentations using C#
Tip: Besides the creation of slides or presentations, Aspose.Slides provides many features that allow you to work with presentations. For example, using its own APIs, Aspose developed a free online viewer for Microsoft PowerPoint Presentations .
The C# Excel Library
- Intuitive C# & VB.NET Excel Document API
- No need to install Microsoft Office or Excel Interop
- Read , edit & create Excel spreadsheet files
- Fully supports .NET 7,6,5, Core, Framework, and Azure
- Read Excel Files without Interop
- Excel Worksheets
- Create Excel Files
- Convert Spreadsheet File Types
- Excel to SQL via System.Data.DataSet
- Excel to SQL and DataGrid via DataTable
- Excel Conditional Formatting
- Protect Excel Files
- Excel Formulas in C#
- Edit Excel Metadata in C#
- Update Excel Database Records
- Style Excel Cell Borders & Fonts
- Sort Excel Ranges in C#
- Repeat Excel Rows & Columns
- Excel Print Setup
- Cell Data Display Format
- Load Excel From SQL Database
- Combine Excel Ranges
- Select Excel Range
- Convert Excel to HTML
- Aggregate Excel Functions
- Create Excel Line Chart
- Freeze Panes in Excel
- Copy Excel Worksheets
IronXL Blog
- Excel Tools
- C# Create PowerPoint From Template
Published March 27, 2024
How to Use Create PowerPoint from a Template in C#
Introduction.
PowerPoint presentations continue to be a standard for efficiently communicating information in commercial, educational, and other domains. PowerPoint is a flexible tool that may improve your communication efforts, whether you're teaching a class, giving project updates to your team, or making a presentation to possible investors. However creating presentations from scratch might take a lot of time, and the efficiency and uniformity of the design may not always be what is wanted. This is where PowerPoint templates come in handy, providing ready-made slides with themes and layouts that can be altered to fit your requirements.
In this post, we'll look at how to use C# to create a PowerPoint from a template. We can expedite the procedure and enable customers to create polished presentations with ease by utilizing the Microsoft PowerPoint Interop library .
How to Use Create PowerPoint from a Template in C#
- Create a new C# project.
- Launch the PowerPoint program in a new instance.
- Insert slides from a template into a newly created presentation.
- Create a new file and save the presentation.
- Close the PowerPoint program and end the presentation.
Understanding of PowerPoint from template
Let's first review the basic ideas behind writing PowerPoint presentations programmatically before getting into the code. With the PowerPoint Interop library from Microsoft, developers may use .NET languages like C# to communicate with PowerPoint applications. Many functions, including the ability to create slides, add text, insert pictures, and apply formatting, are exposed by this package.
Create a New Visual Studio Project
To start a new Visual Studio project, follow the instructions below.
Launch the Visual Studio application. Before using Visual Studio, make sure you have installed it on your computer.
Select File, then New, and lastly Project.

Choose your preferred programming language (C#, for example) from the left side of the "Create a new project" box. Next, from the list of project templates that are available, choose the "Console App" or "Console App (.NET Core)" template. To give your project a name, please fill out the "Name" field.

Decide where the project will be stored. To begin working on a new Console application project, click "Next". Then select the appropriate .NET Framework and click on "Create".

In a freshly established C# project, add a reference to the Microsoft PowerPoint Interop library. It is now easier to develop and interact with presentations thanks to this library, which enables communication between your C# code and the PowerPoint application.
Create PowerPoint from Template using C#
Loading the PowerPoint template that forms the basis of our new presentation is the first step. Predefined slide layouts, themes, and content placeholders are frequently seen in templates. The Microsoft.Office.PowerPoint.Interop library's function allows us to open the template file and retrieve its contents from within our C# source code.
After loading the template, we build a new presentation using its layout. We make use of the PowerPoint presentation file ( output.pptx ). Which helps us to create a PowerPoint presentation object that will act as a canvas for our material. The template's layout and theme will be carried over into this presentation, giving it a unified visual style.

The presentation.Slides.InsertFromFile() method takes four parameters, namely FileName , Index , SlideStart , SlideEnd .
The path to the PowerPoint file containing the slides you wish to include in your presentation is specified as the first parameter ( demo.pptx ). The next parameter is the number of slides the user needs to copy to the new file from the template. The other two parameters are optional. These parameters allow us to pass the start and end slide indexes. If we need to extract slides in between two slides we can use these parameters.
You may use a presentation.Save() method to save the presentation after the slides have been inserted using InsertFromFile() method, close the PowerPoint program using the Close() method, and then exit the presentation with the Quit() method. This way, you can improve the content and flexibility of your PowerPoint presentation by dynamically adding slides from external files by utilizing the InsertFromFile technique.

Introducing a feature-rich C# library for working with Excel files called IronXL . Iron Software's IronXL provides a wide range of tools for dynamically creating, populating, and formatting Excel documents. With its user-friendly API and extensive documentation, IronXL streamlines Excel interactions in C# and provides developers with a seamless experience for Excel-related tasks.
Features of IronXL
- Extensive Excel Support : IronXL is capable of opening and manipulating a large number of Excel files in various Excel formats, such as CSV, XLS, and XLSX file formats. Developers can effectively extract data from an existing Excel file utilizing both old and contemporary Excel files thanks to IronXL's robust parsing features.
- High speed : IronXL prioritizes performance optimization. Excel interactions are reliable and quick because of the use of efficient algorithms and memory management strategies. IronXL provides reduced memory overhead and optimized processing rates, so developers can easily manage huge Excel files.
- Straightforward and user-friendly API : IronXL's user-friendly API makes it suitable for developers of all skill levels. IronXL reduces the learning curve for C# developers and expedites the process of creating and reading an existing file by providing simple ways to read Excel files, access Excel workbooks, and get data from cells.
To know more about IronXL documentation refer here .
Install IronXL
Io install IronXL, use the instructions and command given in the following steps:
In Visual Studio, navigate to Tools -> NuGet Package Manager -> Package Manager interface.
Enter the following code in the package manager's console tab:
The IronXL package is now usable after being downloaded and installed on the current project.
Create Excel Using IronXL
Let's now examine an actual code sample that demonstrates how to write data to an Excel document in C# using IronXL. We'll walk through how to launch a fresh Excel workbook, complete a worksheet, and then save the information to a file:
In this code sample, we first create a new WorkBook object using IronXL's Create() function, specifying the necessary Excel file format. The next step is to make a new worksheet within the workbook and explain some sample data in a two-dimensional array. Next, in order to populate the spreadsheet with the sample data, we utilize nested loops to access and set the values of different cells. In order to free up system resources, we shut down the workbook after using the SaveAs() method to save it to a file named "SampleData.xlsx". Similarly, we are able to generate more than one sheet for the target file.
The Excel file created as an output is shown below. To know more about writing Excel files refer to the code examples page .

In conclusion, utilizing C# to create PowerPoint presentations from templates enables users to improve design consistency, expedite workflow, and produce content that has an effect. Developers may save time and work while guaranteeing professional outcomes by automating the creation process and utilizing the features of the Microsoft PowerPoint Interop library. Learning this approach will help you as a presenter, business professional, or educator improve your presenting skills and capture your audience with eye-catching slides.
For C# developers, IronXL is a powerful alternative to Microsoft Excel, providing full support for Excel, great performance, and seamless interaction with the .NET framework. IronXL's user-friendly API and fine-grained control over Excel documents simplify Excel writing in C#. This facilitates the process for developers to automate Excel-related tasks, export data, and generate dynamic reports. Whether they are creating Excel files for desktop, web, or mobile apps, C# developers can count on IronXL to streamline Excel-related tasks and unleash the full potential of Excel in their C# programs.
IronXL costs $749 when it first launches. In addition, users have the option to pay a membership fee of one year to obtain product assistance and updates. For a fee, IronXL offers protection against unrestrained redistribution. To find out more about the approximate cost, please visit this license page . View more about Iron Software by clicking this website link .
- All Articles
- Using IronXL
- Compare to Other Components
Ready to get started? Version: 2024.4 just released
Get your free.
30-day Trial Key instantly.
Your trial key should be in the email. If it is not, please contact [email protected]
- In Solution Explorer, right-click References, Manage NuGet Packages
- Select Browse and search "IronXL"
- Select the package and install
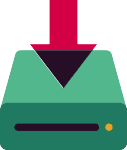
- Download and unzip IronXL to a location such as ~/Libs within your Solution directory
- In Visual Studio Solution Explorer, right click References. Select Browse, "IronXL.dll"
Have a question? Get in touch with our development team.
Want to deploy IronXL to a live project for FREE?
The trial form was submitted successfully .
Get started for FREE
No credit card required
Test in a live environment
Test in production without watermarks. Works wherever you need it to.
Fully-functional product
Get 30 days of fully functional product. Have it up and running in minutes.
24/5 technical support
Full access to our support engineering team during your product trial
Get your free 30-day Trial Key instantly.
Trusted by over 2 million engineers worldwide.
Licenses from $749 . Have a question? Get in touch .
Fully-functional product, get the key instantly
PM > Install-Package IronXL.Excel
IronXL is a part of IRON SUITE
9 .NET API products for your office documents
Your license key has been delivered to the email provided. Contact us
24-Hour Upgrade Offer:
Save 50% on a Professional Upgrade
Go Professional to cover 10 developers and unlimited projects.
Upgrade to Professional
Professional
- 10 developers
- 10 locations
- 10 projects
5 .NET Products for the Price of 2
Total Suite Value:
Upgrade price
After 24 Hrs
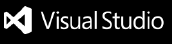
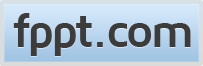
Create PowerPoint .PPT programmatically using C#
Last updated on July 27th, 2023
If you are a developed and want to create your PPT presentations using C# or VB, then you can use the Office Interop libraries to code your own PPT and .pptx files programmatically. This article will show you a quick snippet to make PowerPoint files on the fly.
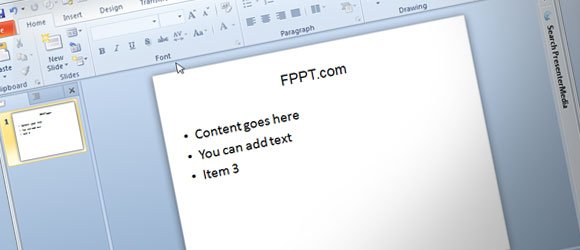
First, you need to prepare your environment and associate the Interop libraries. You can download Interop libraries for free, but you will need to have Office installed.
Now, we will explain what this snippet does.
First, we obtain the Presentation object from the Interop library, which will help us to access PowerPoint and other Microsoft Office programs. Of course to make this work you will need to have Office installed and the Interop libraries referenced in the application.
Then, we will create a Custom Layout using the pptLayoutText that has the presentation title and content. It is the default layout that we can find when we open PowerPoint.
Now we get the slides array and add a new slide object. This will be inserted as the slide #1.
Next, we get the title shape and write the presentation title.
We will do the same with the presentation content. Notice that here we are inserting a bullet list (separated by \n) using C#. This can be helpful to insert a list of items, but if you don’t want to insert a bullet list you can just insert the plain text.
Then, will add a simple comment into the speaker notes to demonstrate that it is also possible to insert notes.
And finally we will need to save the presentation as an output file, unless you want to keep it opened in your window. Notice that to choose if the window should be opened or not, it is defined in the
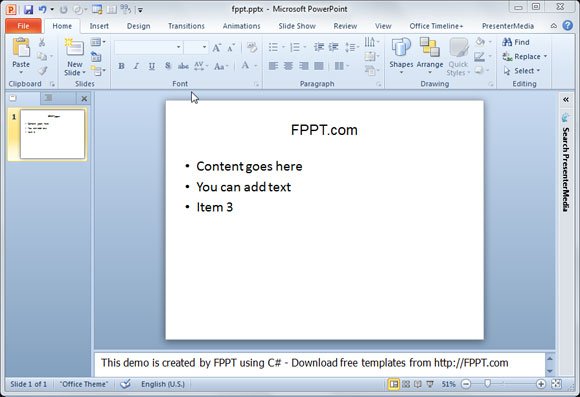
One comment on “ Create PowerPoint .PPT programmatically using C# ”
- Pingback: Create PowerPoint .PPT programmatically using C# | PowerPoint Presentation | PowerPoint presentations and PPT templates | Scoop.it
Comments are closed.
We will send you our curated collections to your email weekly. No spam, promise!
Aspose Knowledge Base
Find advice and answers for most commonly faced scenarios.
Find Answers by API
- Aspose.Total Product Family
- Aspose.Words Product Family
- Aspose.PDF Product Family
- Aspose.Cells Product Family
- Aspose.Email Product Family
- Aspose.Slides Product Family
- Aspose.Imaging Product Family
- Aspose.BarCode Product Family
- Aspose.Diagram Product Family
- Aspose.Tasks Product Family
- Aspose.OCR Product Family
- Aspose.Note Product Family
- Aspose.CAD Product Family
- Aspose.3D Product Family
- Aspose.HTML Product Family
- Aspose.GIS Product Family
- Aspose.ZIP Product Family
- Aspose.Page Product Family
- Aspose.PSD Product Family
- Aspose.OMR Product Family
- Aspose.PUB Product Family
- Aspose.SVG Product Family
- Aspose.Finance Product Family
- Aspose.Drawing Product Family
- Aspose.Font Product Family
- Aspose.TeX Product Family
How to Create PowerPoint Presentation using C#
In this simple tutorial, we will show how to create PowerPoint Presentation using C# along with the detailed steps to set up the environment on your end. While working in C# create PowerPoint presentation using simple steps without any reliance on PowerPoint. Moreover, the provided example can be seamlessly used on all .NET supported platforms.
Steps to Create PowerPoint Presentation using C#
- Download and install Aspose.Slides for .NET package from the NuGet
- Use Aspose.Slides , Aspose.Slides.Export and System.Drawing namespaces in your project
- Create an empty presentation by using the instance of the Presentation class
- Add a slide with a Blank Layout type inside the presentation slides collection
- Add a Rectangle AutoShape inside the newly created slide
- Add a text frame inside added shape and set its textual properties
- Save the presentation as PPTX on the disk using the Save method
The above steps in C# create PPTX file without any dependence on MS PowerPoint. The process starts by creating a presentation using the Presentation class instance, which is then followed by adding a blank slide and an autoshape inside the slide. Subsequently, the text is added and formatted inside the added shape before saving the presentation file as PPTX on the disk.
Code to Generate PowerPoint Presentation in C#
By using the above example in C# Presentation in PPTX format has been saved on the disk. The SaveFormat enumerator also gives the options to save the presentation in PPT, PPS, PPSX, ODP, POT and POTX formats. You can also customize the text using different options exposed by the PortionFormat and ParagraphFormat classes which include setting the options like bullets, margins, indentations, highlighting and text strike-through.
Earlier, we have witnessed how to save slide as SVG in C# in another how-to topic. However, in this topic, we have explored how using C# PowerPoint presentation in different formats can be generated.
Updated on 10 May 2022
- Presentation
How to create a PowerPoint presentation file in C#, VB.NET
PowerPoint presentation is a file format that allows you to create and show a series of slides containing text, shapes, charts, images and SmartArt diagrams with rich set of formatting options to make your presentation more attractive and memorable. Syncfusion Essential Presentation is a .NET PowerPoint library used to create , open , read , edit , and convert PowerPoint presentations. Using this library, you can start creating a PowerPoint document in C# and VB.NET .
Steps to create PowerPoint programmatically:
- Create a new C# console application.

- Install Syncfusion.Presentation.Base NuGet package to the console application project from nuget.org . For more information about adding a NuGet feed in Visual Studio and installing NuGet packages, refer to documentation .

- Include the following namespace in the Program.cs file. using Syncfusion.Presentation;
- Use the following code snippet to create PowerPoint presentation with “Hello World” text. //Create a new PowerPoint presentation IPresentation powerpointDoc = Presentation.Create(); //Add a blank slide to the presentation ISlide slide = powerpointDoc.Slides.Add(SlideLayoutType.Blank); //Add a textbox to the slide IShape shape = slide.AddTextBox(10, 10, 500, 100); //Add a text to the textbox. shape.TextBody.AddParagraph("Hello World!!!"); //Save the PowerPoint presentation powerpointDoc.Save("Sample.pptx"); //Close the PowerPoint presentation powerpointDoc.Close();
You can download the working sample from Create-PowerPoint.Zip .
By executing the program, you will get the PowerPoint document as follows.

Take a moment to peruse the documentation , where you can find other options to format the text with code examples.
Refer here to explore the rich set of Syncfusion Essential Presentation features.
An online sample link to add text to a slide Hello world PowerPoint document
Create a PowerPoint file in ASP.NET MVC
Create a PowerPoint file in ASP.NET
Create a PowerPoint file in Windows Forms
Create a PowerPoint file in WPF
Create a PowerPoint file in UWP
Starting with v16.2.0.x, if you reference Syncfusion assemblies from trial setup or from the NuGet feed, include a license key in your projects. Refer to link to learn about generating and registering Syncfusion license key in your application to use the components without trail message.
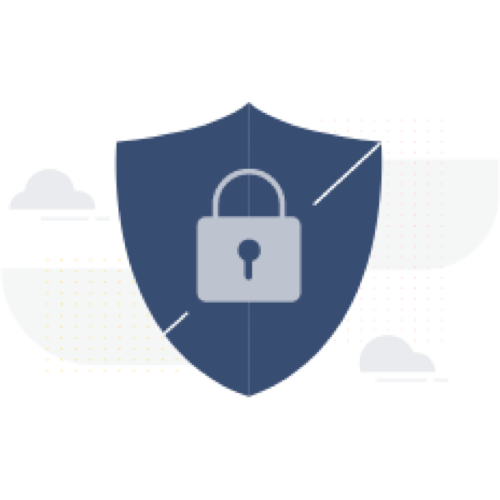
Access denied
- Presentation
- .NET
- FileFormat.Slides for .NET
FileFormat.Slides for .NET
Simplify presentation creation and customization with .net api, create & customize presentations easily with fileformat.slides, an open-source .net api. simplify slides generation & automation with this lightweight library..
FileFormat.Slides for .NET offers a simple, easy and user-friendly experience, making it the ideal choice for those looking to work with Microsoft PowerPoint presentation using an open-source API. This open-source .NET API has been thoughtfully designed to simplify the process of creating and customizing PowerPoint presentation. With this intuitive C# library, you can now easily generate and manipulate presentations with just a few lines of code.
The installation process of this lightweight solution is seamless and offers a stack of features to cater to all your presentation requirements. FileFormat.Slides for .NET leverage the OpenXML SDK, a technology endorsed by Microsoft. FileFormat.Slides for .NET serves as a convenient wrapper that simplifies the utilization of its advanced capabilities.
In consideration of developers, this open-source .NET library makes automating PowerPoint presentation creation and editing a breeze with just a few lines of code. You can enhance its capabilities using the OpenXML SDK library. Handling FileFormat.Slides for .NET is easy, thanks to its user-friendly design. The library comes with intelligent features like adding sheets, text, opening existing presentations in a stream, applying formatting to the entire presentation or specific text shape, adding images to presentations, and much more.
Explore our GitHub repository to contribute, suggest improvements, and enhance this Open Source API: https://github.com/fileformat-slides/FileFormat.Slides-for-.NET
- At a Glance
Platform Independence
- Supported File Formats
At A Glance
An overview of FileFormat.Slides for .NET features.
- Create Presentation
- Open Presentation in Stream
- Insert Slides into Presentations
- Apply Formatting to Presentation
- Add Text to Slides
- Apply Formatting to Text
- Add Image to Slides
FileFormat.Slides for .NET supports popular Microsoft PowerPoint file formats listed below.
FileFormat.Slides for .NET only requires .NET framework, or .NET Core
Getting Started with FileFormat.Slides for .NET
The recommended way to install FileFormat.Slides for .NET is using NuGet . Please use the following command for a smooth installation.
Install FileFormat.Slides for .NET via NuGet
Creating an powerpoint presentation programmatically.
The following code snippet creates an empty PowerPoint presentation programmatically.
Create a PowerPoint presentation via .NET API
Insert text into presentation programmatically.
The following code snippet inserts a text into presentation programmatically.
Insert a Text into Presentation via .NET API
Edit Presentations PPT/PPTX in C#

The most common and widely used presentation file formats are PPT , PPTX , and ODP . The famous Microsoft PowerPoint, OpenOffice Impress and Apple Keynote support these formats and we normally use these formats for making spectacular presentations. As a developer, we can edit presentations in our applications programmatically. In this article, we will discuss how to edit PPT/PPTX presentations in C# using the .NET API for presentation editing.
The following are the topics discussed briefly in this article:
- .NET API - Presentation Slides Editing
- Edit PPT/PPTX Presentations in C#
.NET API for Presentations Editing and Automation #
Now, we will be using the GroupDocs.Editor for .NET in below C# examples. It is the presentation editing API and allows developers to load, edit, and save the edited presentations in other formats like PPT, PPTX, PDF. In addition to the presentation formats, the API supports editing word-processing documents, spreadsheets, HTML, XML, JSON, TXT, TSV, and CSV formats.
Download the DLLs or MSI installer from the downloads section or install the API in your .NET application via NuGet .
Edit PPTX/PPTX Presentations in C# #
Just after setting up the API, you can quickly move towards editing your presentation slides. The following steps will let you edit the presentation of PPT/PPTX and other supported formats.
- Load the presentation.
- Edit using the available options.
- Save the edited presentation.
Load the PPT/PPTX Presentation #
Firstly, load the presentation by providing the presentation file path and the password, if the presentation is protected.
Edit the PPT/PPTX Presentation Slides #
After loading, you can edit the loaded presentation as per requirement. Here I am replacing all the occurrences of word “documents” with the “presentation” in a PPTX presentation using the below C# code.
Save the Edited PowerPoint presentation with Options #
Lastly, while saving the edited presentation content, you can further set various options. These options include; set password, output format settings. I set the above options in the below-mentioned code and save the edited presentation as a password-protected PPTX file.
Complete Code #
For the convenience, here is the complete C# example that is explained above and it edits the PowerPoint presentation and then saves it in PPTX format.
The following is the output presentation in which all the occurrences are replaced using the above code.

Output presentation - ‘documents’ occurrences are replaced by ‘presentation’
Conclusion #
To conclude, we discussed editing presentations slides in C# using presentation editing API for .NET applications. You can use the API with WYSIWYG editors for the visual editing of your presentations. After that, you can move ahead to build your own presentation editor. Similarly, you can also integrate the editing feature within your .NET application.
For more details, options, and examples, you can visit the documentation and the GitHub repository. For further queries, contact the support on the forum .
Related Articles #
- Edit PowerPoint Presentations Online
- Word Documents Editing using C#
- Excel Spreadsheets Editing using C#
- How to Edit XML files in C#
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
🍂 A .NET library for manipulating PowerPoint presentations
ShapeCrawler/ShapeCrawler
Folders and files, repository files navigation.
ShapeCrawler (formerly SlideDotNet) is a .NET library for manipulating PowerPoint presentations. It provides a simplified object model on top of the Open XML SDK , allowing users to process presentations without having Microsoft Office installed.
Quick Start
Create presentation, more samples, prerelease version, have questions.
- Code Contributing
install-package ShapeCrawler
Visit the Wiki page to find more usage samples.
To access prerelease builds from master branch, add https://www.myget.org/F/shape/api/v3/index.json as a package source:

If you have a question:
- Join our Discussions Forum and open a discussion;
- You can always email the author at [email protected]
How to contribute?
Give a star⭐ if you find this useful, please give it a star to show your support.
If you encounter an issue, report the bug on the issue page.
To be able to reproduce a bug, it's often necessary to have the original presentation file attached to the issue description. If this file contains confidential data and cannot be shared publicly, you can securely send it to [email protected] . Of course, if your security policy allow this. We assure you that only the maintainer will access this file, and it will not be shared publicly.
Code contributing
Pull Requests are welcome! Please read the Contribution Guide for more details.
Version 0.50.3 - 2024-03-06
🐞Fixed IShape.AsTable()
Visit CHANGELOG.md to see the full log.
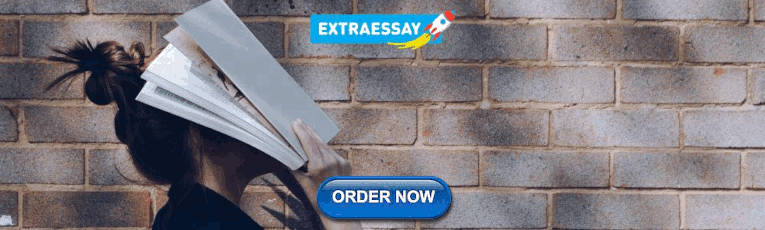
Code of conduct
Releases 53, sponsor this project, contributors 8.
- Batchfile 0.1%
BoldDesk Premium customer service software with affordable pricing: $10 for 3 agents.
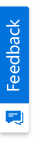
C# POWERPOINT LIBRARY High-performance .NET Core PowerPoint Framework
- Create, and edit PowerPoint files in just a few lines of code.
- Use top features like SmartArt, charts, tables, and animations.
- Combine PowerPoint flies or individual slides.
Trusted by the world’s leading companies

.NET core PowerPoint framework
Syncfusion .NET Core PowerPoint framework allows you to create, read, and edit PowerPoint files in any .NET Core application without Microsoft Office or interop dependency.
PowerPoint Framework
Creation & editing apis.
- Slide Transition
Bullets and Numbering
- Copy and Merge PowerPoints
- Notes pages
Other Supporting Frameworks
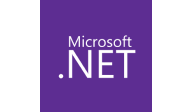
Why choose Syncfusion .NET Core PowerPoint Library?
Blazing fast performance.
It’s optimized for use in a server environment where speed and low memory usage are critical.
No server deployment fees
There are no distribution fees, per-server licensing fees, or royalties, which makes PowerPoint Presentation very cost-effective.
Powerful and comprehensive APIs
All the elements in a typical PowerPoint slide like text, formatting, images, shapes, and tables are accessible through a comprehensive set of APIs.
Fully documented
The ASP.NET Core PowerPoint Framework comes with extensive documentation, a Knowledge Base, and samples.
Stand-alone library
.NET Core PowerPoint Library is a non-UI component that provides a full-fledged PowerPoint presentation instance that allows creating and editing the PowerPoint files without any dependency of Microsoft Office COM libraries and or Microsoft Office.
Cross platforms
Use a single API across all the platforms.
PowerPoint - creation and editing APIs
Create PowerPoint presentations from scratch with text, charts, tables, images, SmartArt diagrams, animations, transitions, and more. Manipulate or edit existing PowerPoint files with just a few lines of code.
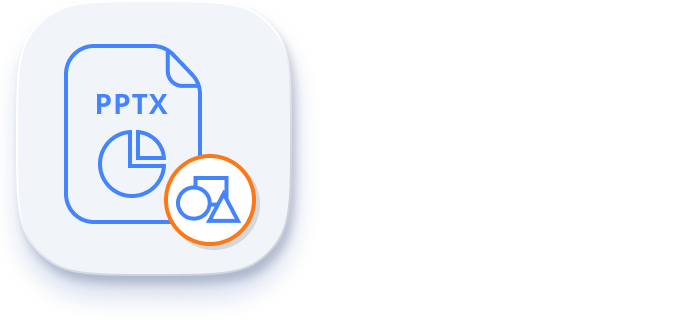
PowerPoint Presentation allows you to add, rearrange, duplicate, format, and delete shapes in a PowerPoint slide.
- PowerPoint shapes documentation
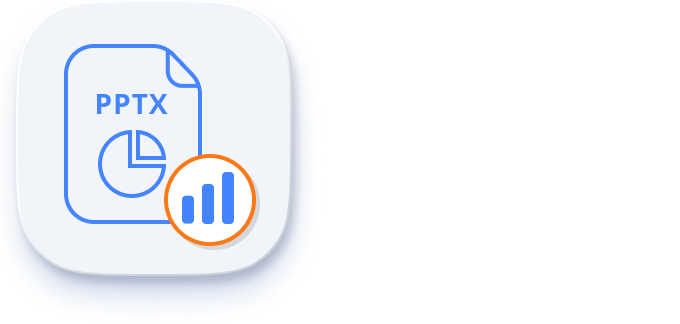
Create and edit more than 80 chart types with elements like chart title, legend, axis title, data labels, line styles, and more.
- PowerPoint charts documentation
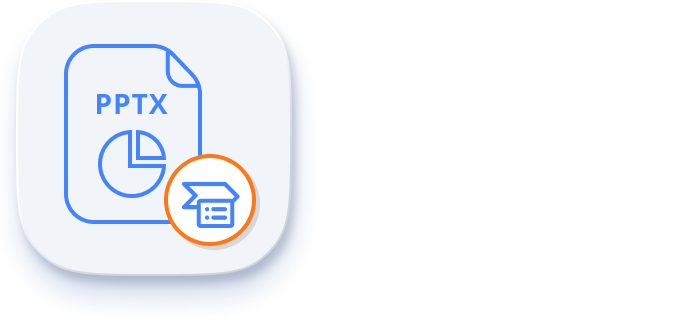
Create, modify, and format 134 SmartArt diagrams to quickly make a visual representation of data with ease.
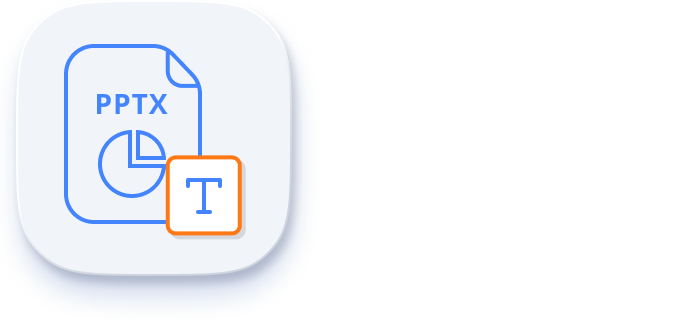
Text formatting
Includes support to add, format, and delete text. Also provides support for all formatting options supported by Microsoft PowerPoint, such as bold, italic, subscript, superscript, text color, font, paragraph, alignment, indentation, and more.
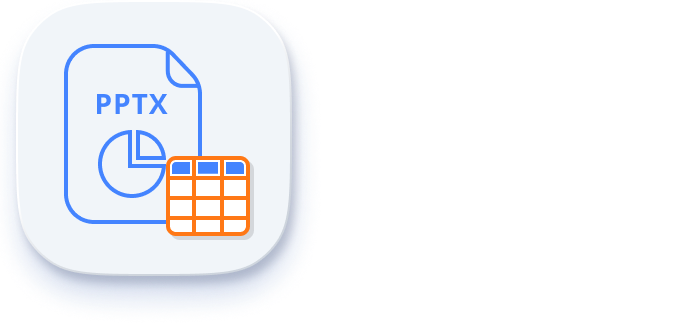
Create, modify, and format tables to keep data organized. Cell and row-level operations like adding, inserting, and deleting rows or columns are also supported. Built-in table styles equivalent to Microsoft PowerPoint table styles are available.
- Learn more about PowerPoint tables
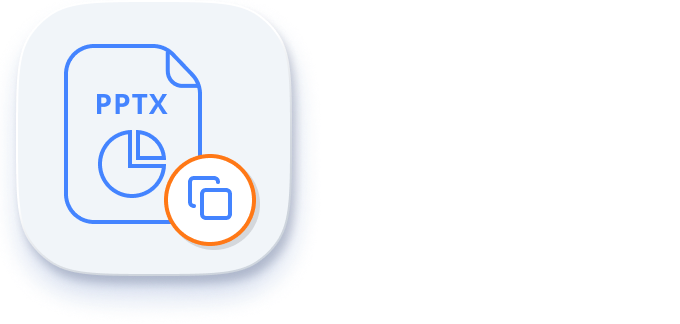
Copy and combine PowerPoints
A slide can be copied and pasted to the same or a different PowerPoint presentation. Copied slides can be merged with source and destination formatting.
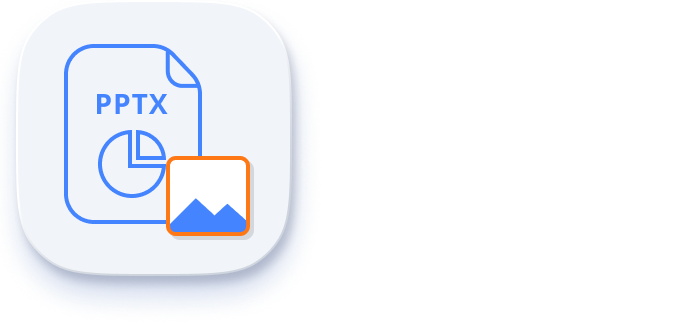
Insert, delete, replace, and format pictures in a PowerPoint slide.
- PowerPoint images documentation
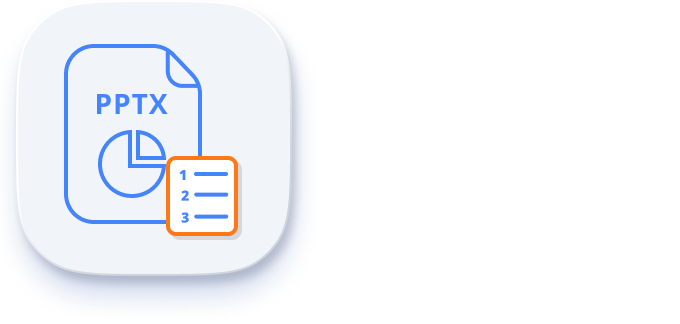
Add and manipulate single-level and multilevel lists like Microsoft PowerPoint.
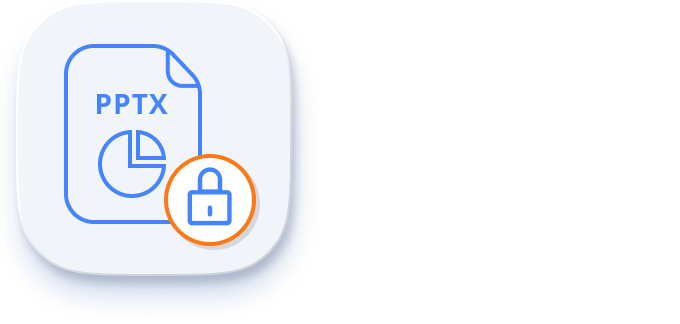
Read and write encrypted PowerPoint presentations. The library allows marking a PowerPoint file as final to prevent editing.
.NET Core PowerPoint Code Example
Easily get started with the .NET Core PowerPoint library using a few simple lines of C# code example as demonstrated below. Also explore our .NET Core PowerPoint Library Example that shows you how to render and configure the .NET Core PowerPoint.
Create and manipulate PowerPoints on many platforms
Unlock the power of PowerPoint creation and manipulation on any platform with our PowerPoint Framework. It empowers you to easily create, read, convert, and manipulate PowerPoint documents programmatically across various platforms (applications), including .NET, Blazor, .NET MAUI, .NET Core, WinUI, Xamarin, and UWP. Explore the platform-wise features of our PowerPoint creation, conversion, and manipulation library.
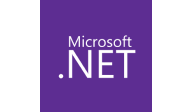
PowerPoint converters
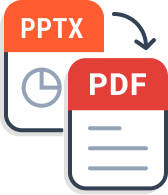
PowerPoint to PDF
PowerPoint Library provides the ability to convert a PowerPoint presentation to PDF. The conversion can be customized with handouts, notes pages, and font substitutions. The size of the converted PDF document can also be optimized.
- Learn more about PowerPoint to PDF conversion
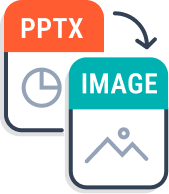
PowerPoint to image
An entire PowerPoint presentation or a specific slide can be converted to an image. JPEG, PNG, BMP, TIFF, EMF, and GIF formats are supported. When converting a PowerPoint slide to image, the PowerPoint framework provides support to use any fallback fonts for missing fonts.
- Learn more about PowerPoint to Image conversion
Not sure how to create your first PowerPoint in .NET Core? Our documentation can help.
Frequently asked questions, ✅ why should i choose syncfusion .net core powerpoint framework.
The Syncfusion .NET Core PowerPoint Framework supports the following:
- Create PowerPoint presentations from scratch.
- Create and edit PowerPoint files with just a few lines of code.
- Top features: SmartArt, Charts, tables , and animations .
- Combine PowerPoint files or individual slides.
- Formatting options equivalent to Microsoft.
- Convert PowerPoint to PDF and PowerPoint to Image .
- One of the best .NET Core PowerPoint libraries on the market, offering a rich set of APIs without Office or interop dependencies.
- Extensive demos and documentation to learn quickly and get started with .NET Core PowerPoint framework.
✅ Can I download and utilize the Syncfusion .NET Core PowerPoint Framework for free?
No, this is a commercial product and requires a paid license. However, a free community license is also available for companies and individuals whose organizations have less than $1 million USD in annual gross revenue, 5 or fewer developers, and 10 or fewer total employees.
✅ How do I get started with Syncfusion .NET Core PowerPoint Frameworks?
A good place to start would be our comprehensive getting started documentation .
✅ What are the platforms Syncfusion PowerPoint Framework supports other than .NET Core?
Apart from .NET Core, the Syncfusion PowerPoint Framework supports platforms for web ( Blazor , ASP.NET MVC , and ASP.NET Web Forms ), mobile ( .NET MAUI , Xamarin , and UWP ), and desktop ( Windows Forms , WPF , WinUI , .NET MAUI , Xamarin , and UWP ).
✅ Where can I find the Syncfusion .NET Core PowerPoint Frameworks demo?
You can find our .NET Core PowerPoint Frameworks demo , which demonstrates how to render and configure the .NET Core PowerPoint.
Our Customers Love Us

Rated by users across the globe
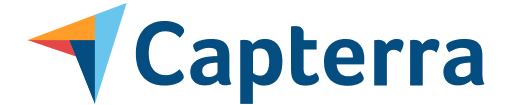
New Library Creates and Manipulates PowerPoint Files
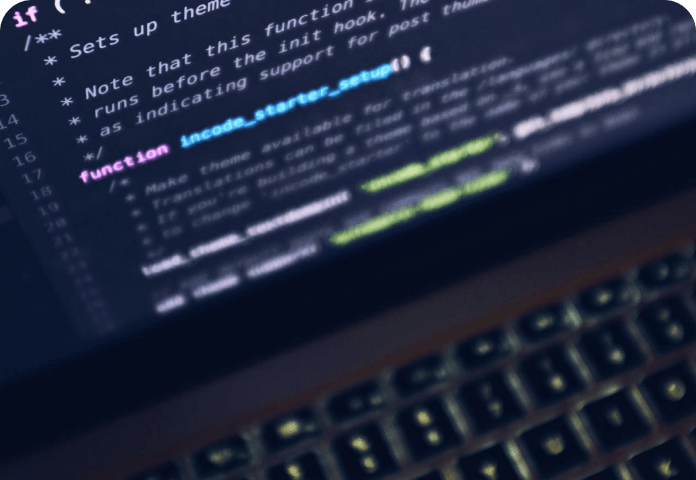
Syncfusion’s file format components helped me create the reports I needed, fast. – J. Pereira, Software Developer
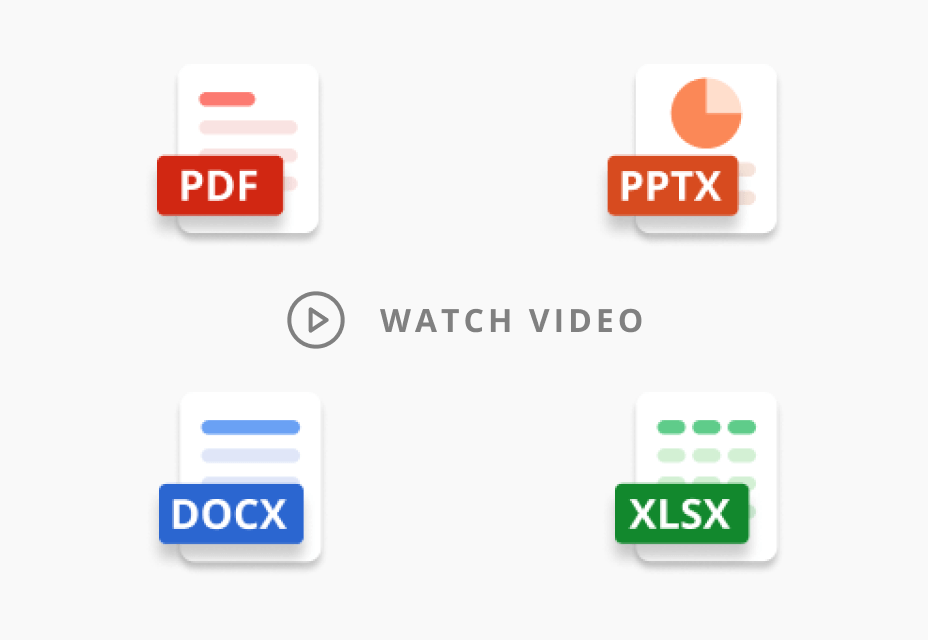
Syncfusion File Format Libraries - Manipulate Excel, Word, PowerPoint, and PDF Files
Want to create, view, and edit ppt files in c# or vb.net, no credit card required., other document processing libraries.
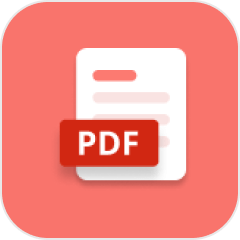
A PDF creation and manipulating framework that allows you to create, read, and manipulate PDF files in any .NET or Flutter application.
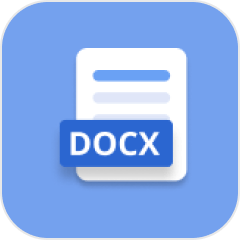
A Word creation and editing framework that allows you to create, read, and edit Word documents in any .NET or Java application.
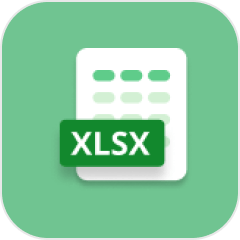
An Excel creation and editing framework that allows you to create, read, and edit Microsoft Excel files in any .NET or Flutter application.
Greatness—it’s one thing to say you have it, but it means more when others recognize it. Syncfusion is proud to hold the following industry awards.
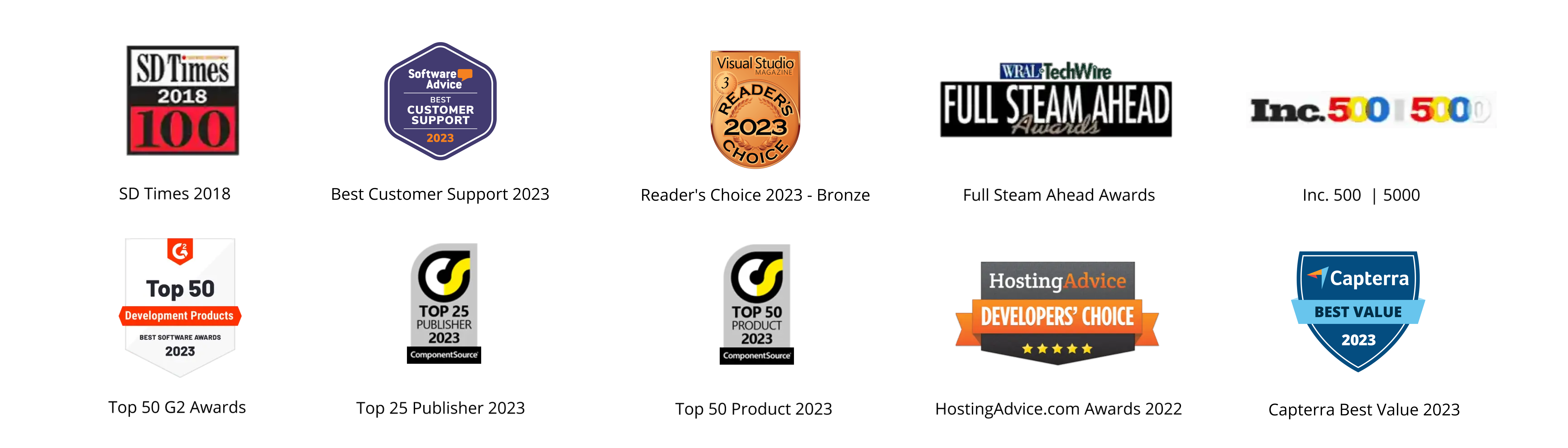
- Developer Platform
- Analytics Platform
- Reporting Platform
- eSignature Software and API
- Help Desk Software
- Knowledge Base Software
- White Papers
- Case Studies
- Technical FAQ
- Code Examples
- Accessibility
- Web Stories
- Community Forum
- Knowledge Base
- Contact Support
- Features & Bugs
- Product Life Cycle
- Documentation
- Release History
- Tutorial Videos
- Blazor Competitive Upgrade
- Angular Competitive Upgrade
- JavaScript Competitive Upgrade
- React Competitive Upgrade
- Vue Competitive Upgrade
- Xamarin Competitive Upgrade
- WinForms Competitive Upgrade
- WPF Competitive Upgrade
- PDF Competitive Upgrade
- Word Competitive Upgrade
- Excel Competitive Upgrade
- PPT Competitive Upgrade
- News & Events
- Fax: +1 919.573.0306
- US: +1 919.481.1974
- UK: +44 20 7084 6215
- Toll Free (USA):
- 1-888-9DOTNET
- [email protected]
- All Products
- Pricing Information
- Free Trials
- Temporary License
- My Orders & Quotes
- Renew an Order
- Upgrade an Order
- Paid Support
- Paid Consulting
- API Reference
- Code Samples
- Free Support
- Free Consulting
- Knowledge Base
- New Releases
- Websites aspose.com aspose.cloud aspose.app aspose.org aspose.dev aspose.ai groupdocs.com groupdocs.cloud groupdocs.app conholdate.com conholdate.app conholdate.cloud
- Acquisition
Create Presentation in .NET
Create powerpoint presentation.
To add a simple plain line to a selected slide of the presentation, please follow the steps below:
- Create an instance of Presentation class.
- Obtain the reference of a slide by using its Index.
- Add an AutoShape of Line type using AddAutoShape method exposed by Shapes object.
- Write the modified presentation as a PPTX file.
In the example given below, we have added a line to the first slide of the presentation.
Create and Save Presentation
Steps: Create and Save Presentation in C#
- Save Presentation to any format supported by SaveFormat
Open and Save Presentation
Steps: Open and Save Presentation in C#
- Create an instance of Presentation class with any format i.e. PPT, PPTX, ODP etc.
Subscribe to Aspose Product Updates
Get monthly newsletters & offers directly delivered to your mailbox.
© Aspose Pty Ltd 2001-2024. All Rights Reserved.
- File Formats
- Presentation
Overview of PowerPoint Presentation
21 Mar 2024 1 minute to read
The PowerPoint framework is a feature rich .NET PowerPoint class library that can be used by developers to create, read, and write Microsoft PowerPoint files by using C#, VB.NET, and managed C++ code. The library can be used in Windows Forms , WPF , ASP.NET Web Forms , ASP.NET MVC , ASP.NET Core , Blazor , UWP , Xamarin , WinUI and .NET MAUI applications.
It is a non-UI component that provides a full-fledged PowerPoint presentation instance that facilitates accessing and manipulating the presentations without any dependency of Microsoft Office COM libraries and Microsoft Office.
Key features
- Support to create PowerPoint presentation from scratch.
- Open , modify , and save existing presentations.
- Ability to convert PowerPoint presentation to PDF .
- Ability to convert PowerPoint slides to images .
- Ability to create and edit charts.
- Ability to convert chart in a slide to image .
- Ability to clone and merge slides in presentation
- Ability to create and edit animations.
- Ability to create and edit transition effects.
- Ability to create and edit comments.
- Ability to encrypt and decrypt PowerPoint presentation.
- Ability to set and remove write protection of PowerPoint presentation.
- Ability to access the Built-in and Custom document properties.
- Ability to create and modify sections in PowerPoint presentation.
Compatible Microsoft PowerPoint Versions
- Microsoft PowerPoint 2007
- Microsoft PowerPoint 2010
- Microsoft PowerPoint 2013
- Microsoft PowerPoint 2016
- Microsoft PowerPoint 2019
- Microsoft 365
NOTE The current version of Essential Presentation supports the .PPTX, .PPTM, .POTX, .POTM file formats only. The current version of Essential Presentation does not support some features in Microsoft PowerPoint such as Word Art, creation and editing of Handouts, equations, create and edit audio and video content, built-in themes, and its variants.
- Create a presentation Article
- Save Article
- Design Article
- Share and collaborate Article
- Give a presentation Article
- Set up your mobile apps Article
- Learn more Article
Create a presentation
Create a presentation in PowerPoint
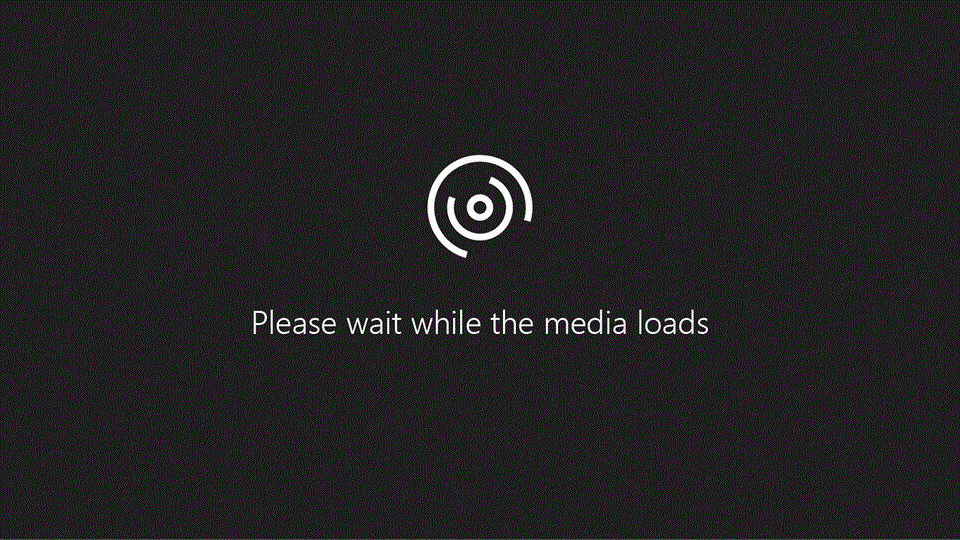
Create presentations from scratch or start with a professionally designed, fully customizable template from Microsoft Create .
Open PowerPoint.
In the left pane, select New .
Select an option:
To create a presentation from scratch, select Blank Presentation .
To use a prepared design, select one of the templates.
To see tips for using PowerPoint, select Take a Tour , and then select Create , .

Add a slide
In the thumbnails on the left pane, select the slide you want your new slide to follow.
In the Home tab, in the Slides section, select New Slide .
In the Slides section, select Layout , and then select the layout you want from the menu.
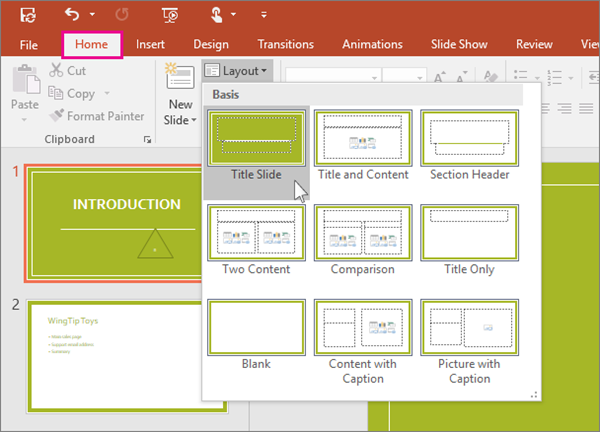
Add and format text
Place the cursor inside a text box, and then type something.
Select the text, and then select one or more options from the Font section of the Home tab, such as Font , Increase Font Size , Decrease Font Size , Bold , Italic , Underline , etc.
To create bulleted or numbered lists, select the text, and then select Bullets or Numbering .
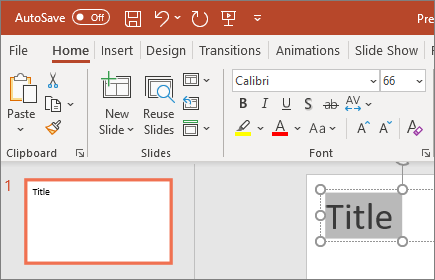
Add a picture, shape, and more
Go to the Insert tab.
To add a picture:
In the Images section, select Pictures .
In the Insert Picture From menu, select the source you want.
Browse for the picture you want, select it, and then select Insert .
To add illustrations:
In the Illustrations section, select Shapes , Icons , 3D Models , SmartArt , or Chart .
In the dialog box that opens when you click one of the illustration types, select the item you want and follow the prompts to insert it.
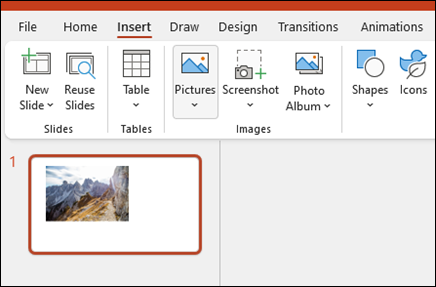
Need more help?
Want more options.
Explore subscription benefits, browse training courses, learn how to secure your device, and more.
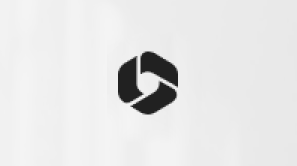
Microsoft 365 subscription benefits
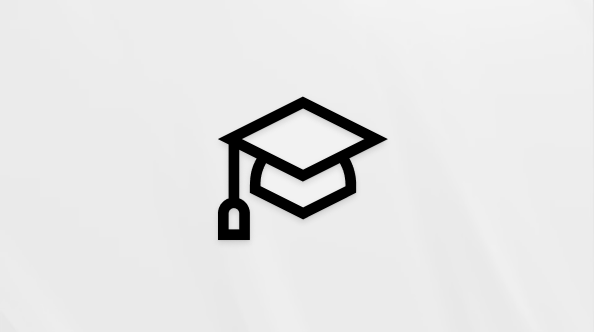
Microsoft 365 training
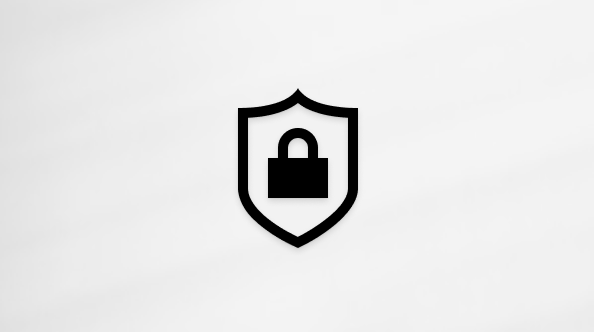
Microsoft security
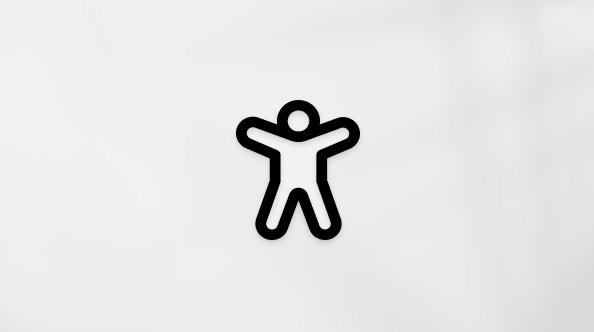
Accessibility center
Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.

Ask the Microsoft Community
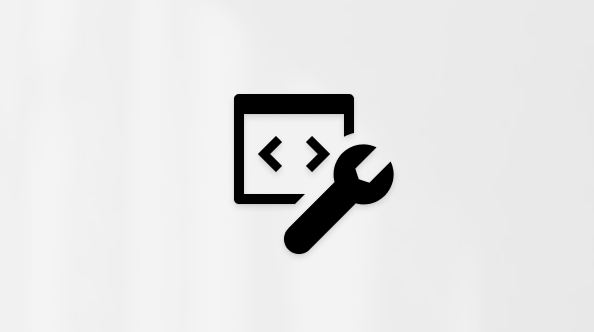
Microsoft Tech Community
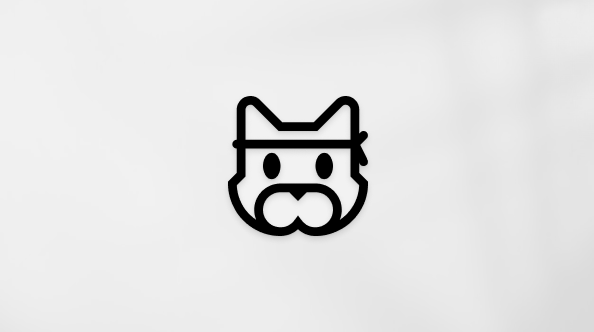
Windows Insiders
Microsoft 365 Insiders
Find solutions to common problems or get help from a support agent.

Online support
Was this information helpful?
Thank you for your feedback.

- SUGGESTED TOPICS
- The Magazine
- Newsletters
- Managing Yourself
- Managing Teams
- Work-life Balance
- The Big Idea
- Data & Visuals
- Reading Lists
- Case Selections
- HBR Learning
- Topic Feeds
- Account Settings
- Email Preferences
How to Make a “Good” Presentation “Great”
- Guy Kawasaki

Remember: Less is more.
A strong presentation is so much more than information pasted onto a series of slides with fancy backgrounds. Whether you’re pitching an idea, reporting market research, or sharing something else, a great presentation can give you a competitive advantage, and be a powerful tool when aiming to persuade, educate, or inspire others. Here are some unique elements that make a presentation stand out.
- Fonts: Sans Serif fonts such as Helvetica or Arial are preferred for their clean lines, which make them easy to digest at various sizes and distances. Limit the number of font styles to two: one for headings and another for body text, to avoid visual confusion or distractions.
- Colors: Colors can evoke emotions and highlight critical points, but their overuse can lead to a cluttered and confusing presentation. A limited palette of two to three main colors, complemented by a simple background, can help you draw attention to key elements without overwhelming the audience.
- Pictures: Pictures can communicate complex ideas quickly and memorably but choosing the right images is key. Images or pictures should be big (perhaps 20-25% of the page), bold, and have a clear purpose that complements the slide’s text.
- Layout: Don’t overcrowd your slides with too much information. When in doubt, adhere to the principle of simplicity, and aim for a clean and uncluttered layout with plenty of white space around text and images. Think phrases and bullets, not sentences.
As an intern or early career professional, chances are that you’ll be tasked with making or giving a presentation in the near future. Whether you’re pitching an idea, reporting market research, or sharing something else, a great presentation can give you a competitive advantage, and be a powerful tool when aiming to persuade, educate, or inspire others.

- Guy Kawasaki is the chief evangelist at Canva and was the former chief evangelist at Apple. Guy is the author of 16 books including Think Remarkable : 9 Paths to Transform Your Life and Make a Difference.
Partner Center
View, manage, and install add-ins for Excel, PowerPoint, and Word
When you install and use an add-in, it adds custom commands and extends the features of your Microsoft 365 programs to help increase your productivity.
Note: This article only applies to add-ins in Excel, PowerPoint, and Word. For guidance on how to view, install, and manage add-ins in Outlook, see Use add-ins in Outlook .
View installed add-ins

You can directly install add-ins from this page or select More Add-ins to explore.
In the Office Add-ins dialog, select the My Add-ins tab.
Select an add-in you want to view the details for and right-click to select Add-in details option.
Install an add-in
Tip: If you selected Home > Add-ins , directly install popular add-ins from the menu that appears, or select More Add-ins to view more options.
Select Add from the add-in you want to install.
Manage installed add-ins
To manage and view information about your installed add-ins, perform the following:
Select File > Get Add-ins . Alternatively, select Home > Add-ins > More add-ins .
In the Office Add-ins dialog, select the My Add-ins tab.
Select Manage My Add-ins . This opens the Office Store page in your preferred browser with a list of your installed add-ins.
Remove an add-in
To remove an add-in you installed, follow these steps.
Select File > Get Add-ins . Alternatively, select Home > Add-ins .
In the Office Add-ins dialog, select My Add-ins tab.
Select an add-in you want to remove and right click to select Remove option.
Note: Add-ins that appear in the Admin Managed section of the Office Add-ins dialog can only be removed by your organization's administrator.
Cancel an add-in subscription
To discontinue your subscription to an add-in, do the following:
Open the Microsoft 365 application and select the Home tab.
Select Add-ins from the ribbon, then select More Add-ins .
Select the My Add-ins tab to view your existing add-ins.
Select Manage My Add-ins .
Under the Payment and Billing section, choose Cancel Subscription .
Select OK , then Continue .
Once you've cancelled your subscription, you should see a message that says "You have cancelled your app subscription" in the comments field of your add-in list.
Manage an add-in's access to your devices
Note: The information in this section only applies to Excel on the web, Outlook on the web, PowerPoint on the web, and Word on the web running in Chromium-based browsers, such as Microsoft Edge and Google Chrome.
When an installed add-in requires access to your devices, such as your camera or microphone, you will be shown a dialog with the option to allow, allow once, or deny permission.
If you select Allow , the add-in will have access to the requested devices. The permission you grant persists until you uninstall the add-in or until you clear the cache of the browser where the add-in is running.
If you select Allow Once , the add-in will have access to the requested devices until it's relaunched in the browser.
If you select Deny , the add-in won't be able to access the requested devices. This persists until you uninstall the add-in or until you clear the cache of the browser where the add-in is running.
If you want to change an add-in's access to your devices after selecting Allow or Deny , you must first uninstall the add-in or clear your browser cache.
Add or load a PowerPoint add-in
Add or remove add-ins in Excel
Get a Microsoft 365 Add-in for Excel
Get a Microsoft 365 Add-in for Outlook
Help for Excel for Windows add-ins

Need more help?
Want more options.
Explore subscription benefits, browse training courses, learn how to secure your device, and more.
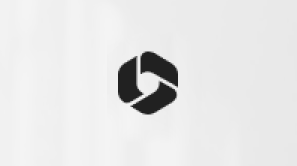
Microsoft 365 subscription benefits
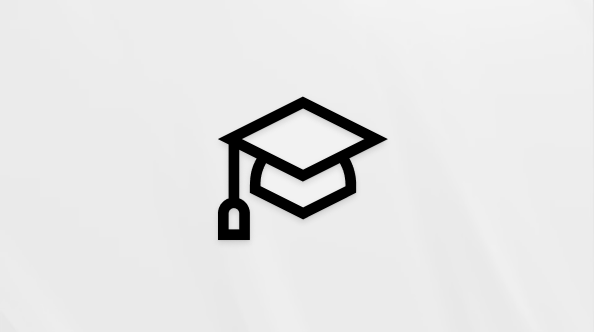
Microsoft 365 training
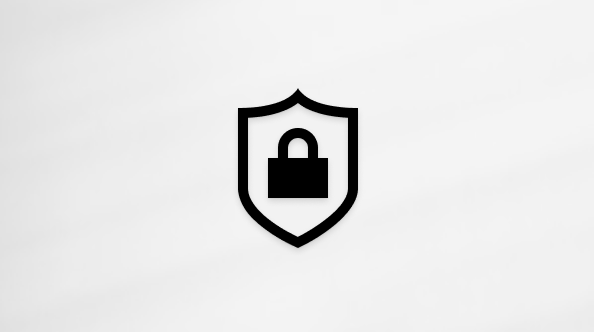
Microsoft security
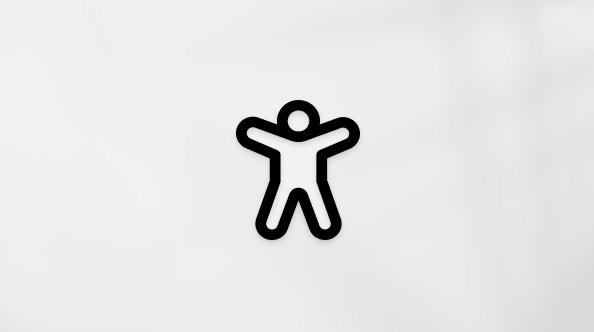
Accessibility center
Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.

Ask the Microsoft Community
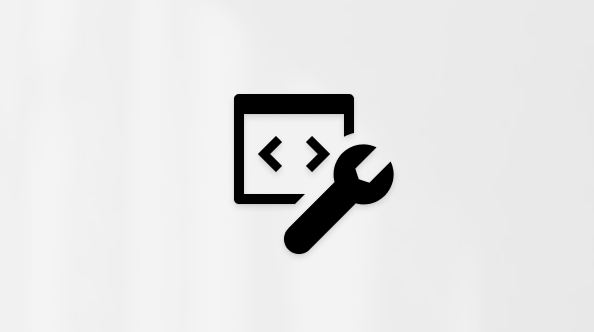
Microsoft Tech Community
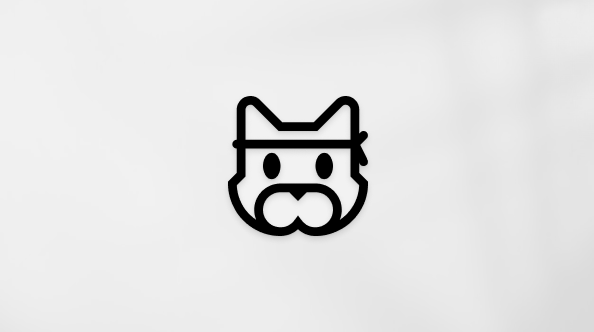
Windows Insiders
Microsoft 365 Insiders
Find solutions to common problems or get help from a support agent.

Online support
Was this information helpful?
Thank you for your feedback.
How to Use Copilot in Powerpoint
Click the icon in the upper-right, and then use it to create a rough draft or revise what you've done
What To Know
- You'll need Microsoft 365 and a Copilot subscription to use Copilot in Powerpoint.
- Select the Copilot icon and then chat to it about what you want it to do.
- It can draft new presentations and slides, or edit what you're already made.
This guide will show you how to use Microsoft Copilot in Powerpoint .
While generative AI can be a convenient and powerful tool, you should only use it to get started, and you should verify everything it produces for factual accuracy. Trying to sell or pass off completely AI-generated work as your own can lead to bad outcomes for both yourself and your audience.
How to Get Access to Copilot in Powerpoint
To use Copilot in Powerpoint, you need a subscription to the Microsoft Copilot Pro plan , which costs around $20 per month per user. You get one month free, so you can use the free trial period to play around with the feature if you like. This membership lets you use Copilot in the free web versions of Microsoft's various office applications, like Word and Powerpoint.
However, to use Copilot in the offline version of those tools, you'll need a subscription to Microsoft 365 as well. That plan lets you use Copilot on- and offline in any of Microsoft's various office applications.
How to Create New Slides With Copilot in Powerpoint
To start creating new slides in Powerpoint using Copilot, all you need to do is ask it, giving it any information you feel it might need to be up to the task.
Open Powerpoint and select the Copilot icon in the top-right. It looks like two orange-edged rings intersecting.
The Copilot chat window will open. Type into the chat bar in the bottom right what you want Copilot to do.
In your request, include any information you feel is relevant to the task. You can optionally paste text or links to online content into the chat window to ask Copilot to include it.
In this example, we had Copilot produce a guide on how to use Copilot in Powerpoint, using a link to Microsoft's explainer page about the technology.
If you're unsure about how to write a prompt for Copilot, you can select the Prompt Book icon next to the Microphone at the bottom of the chat window. This will provide you with a number of pre-written prompts to select from.
To change the look or style of a presentation or slide once Copilot has drafted it, you can make manual changes yourself, or select the Designer icon to use the Designer tool to see alternate versions.
Choose a blank presentation to start from scratch, or a template or theme to give you a starting off point. Copilot can utilize either or both to help generate slides and presentations in line with your needs.
How to Create a New Presentation From a Word File With Copilot
If you have some existing data or text that you want to turn into a presentation, you can use Copilot to do it. Here's how.
Open Powerpoint and launch Copilot by selecting the Copilot icon, as above.
Select the Prompt Book to get a list of Copilot prompts. It's the book icon next to the Microphone at the bottom of the chat window. Then, select Create.
Select View More Prompts .
Choose Create a new presentation based on [file] .
Choose the file you want to use to create the Powerpoint, and Copilot will make a presentation or slide. You can then edit it manually yourself, or use the Designer tab to choose between different variations on the same design.
Read the original article on Lifewire .
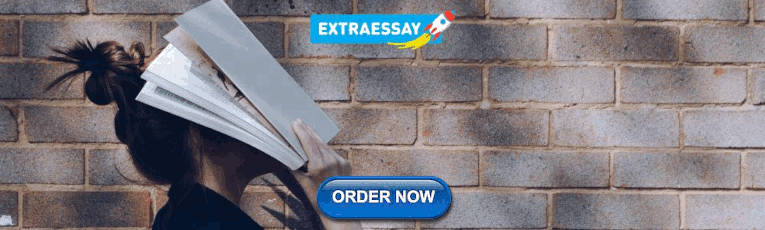
IMAGES
VIDEO
COMMENTS
6. You can also look at Aspose Slides, a component for .NET and Java that makes it easy to generate powerpoint documents. answered Jan 26, 2009 at 8:12. Rad. 8,359 4 46 46. 3. Worth noting that this component works great for small presentations but has lots of memory issues with large or complex slides.
The following are the steps to add a chart in PowerPoint presentation using C#. Create an instance of the Presentation class. Obtain the reference of a slide by index. Add a chart with the desired type using ISlide.Shapes.AddChart (ChartType, Single, Single, Single, Single) method. Add a chart title.
Install Free Spire.Presentation for .NET. Method 1: Download Free Spire.Presentation and unzip the package somewhere on your disk to find the "BIN" folder. Free Spire.Presentation has the DLLs ...
How to Use Create PowerPoint from a Template in C#. Create a new C# project. Launch the PowerPoint program in a new instance. Insert slides from a template into a newly created presentation. Create a new file and save the presentation. Close the PowerPoint program and end the presentation.
Syncfusion's PowerPoint framework is a feature rich .NET PowerPoint library developed with 100% managed C# code that can be used to create, read and write PowerPoint files. The library can be used in ASP.NET Core, Blazor, UWP, Xamarin, WinUI, and .NET MAUI applications, as well as the Unity platform, without any dependency on Adobe Acrobat.
If you are a developed and want to create your PPT presentations using C# or VB, then you can use the Office Interop libraries to code your own PPT and .pptx files programmatically. ... Create PowerPoint .PPT programmatically using C#. Application pptApplication = new Application(); Microsoft.Office.Interop.PowerPoint.Slides slides; Microsoft ...
How to create Power Point Presentation using ASP NET C# I have explained the following:How to Create PPTHow to Add Slide in PPTHow to Update Title in PPTHow ...
Steps to Create PowerPoint Presentation using C#. Download and install Aspose.Slides for .NET package from the NuGet. Use Aspose.Slides, Aspose.Slides.Export and System.Drawing namespaces in your project. Create an empty presentation by using the instance of the Presentation class. Add a slide with a Blank Layout type inside the presentation ...
Steps to create, read and edit PowerPoint slides Programmatically: Step 1: Create a new Windows Forms application. Name it as GettingStarted. Step 2: Install Presentation assemblies with NuGet. In Visual Studio, select Tools > NuGet Package Manager > Package Manager Console and execute the following command for the project. Step 3: Add a new ...
Steps to create PowerPoint programmatically: Create a new C# console application. Install Syncfusion.Presentation.Base NuGet package to the console application project from nuget.org. For more information about adding a NuGet feed in Visual Studio and installing NuGet packages, refer to documentation. Include the following namespace in the ...
The following code snippet inserts a text into presentation programmatically. Insert a Text into Presentation via .NET API. // Create a new PowerPoint presentation at the specified file path. Presentation presentation = Presentation.Create("D:\\AsposeSampleResults\\test2.pptx");
Here I am replacing all the occurrences of word "documents" with the "presentation" in a PPTX presentation using the below C# code. // Edit Presentation using (Editor editor = new Editor( delegate { return fs; }, delegate { return loadOptions; })) {. Options.PresentationEditOptions editOptions = new PresentationEditOptions();
See how to create a PowerPoint presentation in ASP .NET Core using the Syncfusion .NET PowerPoint Library. In this video, I will demonstrate how to create an ASP .NET Core application in Visual Studio and install the Syncfusion PowerPoint .NET Core package. ... The April edition of 5 C# Tips & Tricks. Let's find out some awesome stuff about ...
MIT license. ShapeCrawler (formerly SlideDotNet) is a .NET library for manipulating PowerPoint presentations. It provides a simplified object model on top of the Open XML SDK, allowing users to process presentations without having Microsoft Office installed.
Easily get started with the .NET Core PowerPoint library using a few simple lines of C# code example as demonstrated below. Also explore our .NET Core PowerPoint Library Example that shows you how to render and configure the .NET Core PowerPoint. c#. //Creates PowerPoint Presentation. using (IPresentation pptxDoc = Presentation.Create()) {.
I have tried looking into the creation of a PowerPoint presentation using C# but i was not able to find how i would clone slides from a template and add charts to a presentation slide on the fly using C#. After the presentation has been created save the file as well. Using Aspose.slide for .NET. c#. powerpoint.
Create PowerPoint Presentation. To add a simple plain line to a selected slide of the presentation, please follow the steps below: ... Steps: Open and Save Presentation in C#. Create an instance of Presentation class with any format i.e. PPT, PPTX, ODP etc.
Overview of PowerPoint Presentation. 21 Mar 2024 1 minute to read. The PowerPoint framework is a feature rich .NET PowerPoint class library that can be used by developers to create, read, and write Microsoft PowerPoint files by using C#, VB.NET, and managed C++ code. The library can be used in Windows Forms, WPF, ASP.NET Web Forms, ASP.NET MVC, ASP.NET Core, Blazor, UWP, Xamarin, WinUI and ...
Create a presentation. Open PowerPoint. In the left pane, select New. Select an option: To create a presentation from scratch, select Blank Presentation. To use a prepared design, select one of the templates. To see tips for using PowerPoint, select Take a Tour, and then select Create, . Add a slide.
When in doubt, adhere to the principle of simplicity, and aim for a clean and uncluttered layout with plenty of white space around text and images. Think phrases and bullets, not sentences. As an ...
1. Assuming you have Microsoft Office installed, follow these steps: Open the Project menu > Add Reference > COM tab > Microsoft PowerPoint xx.x Object Library. Add the following using statement at the top of your file: using Microsoft.Office.Interop.PowerPoint; Make sure that the Embed Interop Type property of the reference is set to true:
Open a new Microsoft PowerPoint. Click the Copilot button from the top bar. This will open a Copilot section from the left pane. Now, you can start creating a presentation by asking Copilot to ...
Note: This article only applies to add-ins in Excel, PowerPoint, and Word.For guidance on how to view, install, and manage add-ins in Outlook, see Use add-ins in Outlook.
With a C# add-in, I am trying to add a button to PowerPoint and when I click the button, a new slide is added to the current presentation. I have found this solution - it is really close to what I want to do... but it is intended to open another PowerPoint file and add a slide to it. But what I want to do is to add a slide to the current presentation.
Choose Create a new presentation based on [file]. Choose the file you want to use to create the Powerpoint, and Copilot will make a presentation or slide.
4. Before going to develop a template for PowerPoint, read the presentationML structure from this PDF, Refer the follwing link for creating PPT using OPENXML. How to: Create a presentation document by providing a file name (Open XML SDK) Refer following link for insert image into the PPT Insert image into the PPT File.