- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
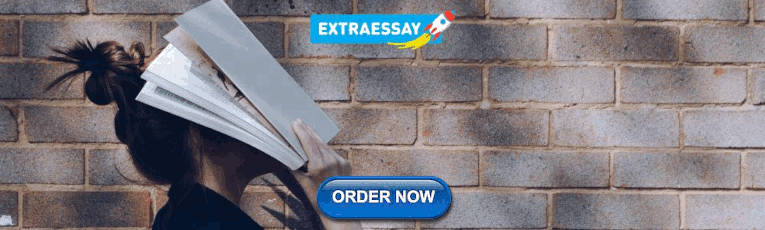
Expressions and operators
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more.
At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that purely evaluate .
The expression x = 7 is an example of the first type. This expression uses the = operator to assign the value seven to the variable x . The expression itself evaluates to 7 .
The expression 3 + 4 is an example of the second type. This expression uses the + operator to add 3 and 4 together and produces a value, 7 . However, if it's not eventually part of a bigger construct (for example, a variable declaration like const z = 3 + 4 ), its result will be immediately discarded — this is usually a programmer mistake because the evaluation doesn't produce any effects.
As the examples above also illustrate, all complex expressions are joined by operators , such as = and + . In this section, we will introduce the following operators:
Assignment operators
Comparison operators, arithmetic operators, bitwise operators, logical operators, bigint operators, string operators, conditional (ternary) operator, comma operator, unary operators, relational operators.
These operators join operands either formed by higher-precedence operators or one of the basic expressions . A complete and detailed list of operators and expressions is also available in the reference .
The precedence of operators determines the order they are applied when evaluating an expression. For example:
Despite * and + coming in different orders, both expressions would result in 7 because * has precedence over + , so the * -joined expression will always be evaluated first. You can override operator precedence by using parentheses (which creates a grouped expression — the basic expression). To see a complete table of operator precedence as well as various caveats, see the Operator Precedence Reference page.
JavaScript has both binary and unary operators, and one special ternary operator, the conditional operator. A binary operator requires two operands, one before the operator and one after the operator:
For example, 3 + 4 or x * y . This form is called an infix binary operator, because the operator is placed between two operands. All binary operators in JavaScript are infix.
A unary operator requires a single operand, either before or after the operator:
For example, x++ or ++x . The operator operand form is called a prefix unary operator, and the operand operator form is called a postfix unary operator. ++ and -- are the only postfix operators in JavaScript — all other operators, like ! , typeof , etc. are prefix.
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = f() is an assignment expression that assigns the value of f() to x .
There are also compound assignment operators that are shorthand for the operations listed in the following table:
Assigning to properties
If an expression evaluates to an object , then the left-hand side of an assignment expression may make assignments to properties of that expression. For example:
For more information about objects, read Working with Objects .
If an expression does not evaluate to an object, then assignments to properties of that expression do not assign:
In strict mode , the code above throws, because one cannot assign properties to primitives.
It is an error to assign values to unmodifiable properties or to properties of an expression without properties ( null or undefined ).
Destructuring
For more complex assignments, the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
Without destructuring, it takes multiple statements to extract values from arrays and objects:
With destructuring, you can extract multiple values into distinct variables using a single statement:
Evaluation and nesting
In general, assignments are used within a variable declaration (i.e., with const , let , or var ) or as standalone statements).
However, like other expressions, assignment expressions like x = f() evaluate into a result value. Although this result value is usually not used, it can then be used by another expression.
Chaining assignments or nesting assignments in other expressions can result in surprising behavior. For this reason, some JavaScript style guides discourage chaining or nesting assignments . Nevertheless, assignment chaining and nesting may occur sometimes, so it is important to be able to understand how they work.
By chaining or nesting an assignment expression, its result can itself be assigned to another variable. It can be logged, it can be put inside an array literal or function call, and so on.
The evaluation result matches the expression to the right of the = sign in the "Meaning" column of the table above. That means that x = f() evaluates into whatever f() 's result is, x += f() evaluates into the resulting sum x + f() , x **= f() evaluates into the resulting power x ** f() , and so on.
In the case of logical assignments, x &&= f() , x ||= f() , and x ??= f() , the return value is that of the logical operation without the assignment, so x && f() , x || f() , and x ?? f() , respectively.
When chaining these expressions without parentheses or other grouping operators like array literals, the assignment expressions are grouped right to left (they are right-associative ), but they are evaluated left to right .
Note that, for all assignment operators other than = itself, the resulting values are always based on the operands' values before the operation.
For example, assume that the following functions f and g and the variables x and y have been declared:
Consider these three examples:
Evaluation example 1
y = x = f() is equivalent to y = (x = f()) , because the assignment operator = is right-associative . However, it evaluates from left to right:
- The y on this assignment's left-hand side evaluates into a reference to the variable named y .
- The x on this assignment's left-hand side evaluates into a reference to the variable named x .
- The function call f() prints "F!" to the console and then evaluates to the number 2 .
- That 2 result from f() is assigned to x .
- The assignment expression x = f() has now finished evaluating; its result is the new value of x , which is 2 .
- That 2 result in turn is also assigned to y .
- The assignment expression y = x = f() has now finished evaluating; its result is the new value of y – which happens to be 2 . x and y are assigned to 2 , and the console has printed "F!".
Evaluation example 2
y = [ f(), x = g() ] also evaluates from left to right:
- The y on this assignment's left-hand evaluates into a reference to the variable named y .
- The function call g() prints "G!" to the console and then evaluates to the number 3 .
- That 3 result from g() is assigned to x .
- The assignment expression x = g() has now finished evaluating; its result is the new value of x , which is 3 . That 3 result becomes the next element in the inner array literal (after the 2 from the f() ).
- The inner array literal [ f(), x = g() ] has now finished evaluating; its result is an array with two values: [ 2, 3 ] .
- That [ 2, 3 ] array is now assigned to y .
- The assignment expression y = [ f(), x = g() ] has now finished evaluating; its result is the new value of y – which happens to be [ 2, 3 ] . x is now assigned to 3 , y is now assigned to [ 2, 3 ] , and the console has printed "F!" then "G!".
Evaluation example 3
x[f()] = g() also evaluates from left to right. (This example assumes that x is already assigned to some object. For more information about objects, read Working with Objects .)
- The x in this property access evaluates into a reference to the variable named x .
- Then the function call f() prints "F!" to the console and then evaluates to the number 2 .
- The x[f()] property access on this assignment has now finished evaluating; its result is a variable property reference: x[2] .
- Then the function call g() prints "G!" to the console and then evaluates to the number 3 .
- That 3 is now assigned to x[2] . (This step will succeed only if x is assigned to an object .)
- The assignment expression x[f()] = g() has now finished evaluating; its result is the new value of x[2] – which happens to be 3 . x[2] is now assigned to 3 , and the console has printed "F!" then "G!".
Avoid assignment chains
Chaining assignments or nesting assignments in other expressions can result in surprising behavior. For this reason, chaining assignments in the same statement is discouraged .
In particular, putting a variable chain in a const , let , or var statement often does not work. Only the outermost/leftmost variable would get declared; other variables within the assignment chain are not declared by the const / let / var statement. For example:
This statement seemingly declares the variables x , y , and z . However, it only actually declares the variable z . y and x are either invalid references to nonexistent variables (in strict mode ) or, worse, would implicitly create global variables for x and y in sloppy mode .
A comparison operator compares its operands and returns a logical value based on whether the comparison is true. The operands can be numerical, string, logical, or object values. Strings are compared based on standard lexicographical ordering, using Unicode values. In most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for the comparison. This behavior generally results in comparing the operands numerically. The sole exceptions to type conversion within comparisons involve the === and !== operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality. The following table describes the comparison operators in terms of this sample code:
Note: => is not a comparison operator but rather is the notation for Arrow functions .
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). These operators work as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity ). For example:
In addition to the standard arithmetic operations ( + , - , * , / ), JavaScript provides the arithmetic operators listed in the following table:
A bitwise operator treats their operands as a set of 32 bits (zeros and ones), rather than as decimal, hexadecimal, or octal numbers. For example, the decimal number nine has a binary representation of 1001. Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
The following table summarizes JavaScript's bitwise operators.
Bitwise logical operators
Conceptually, the bitwise logical operators work as follows:
- The operands are converted to thirty-two-bit integers and expressed by a series of bits (zeros and ones). Numbers with more than 32 bits get their most significant bits discarded. For example, the following integer with more than 32 bits will be converted to a 32-bit integer: Before: 1110 0110 1111 1010 0000 0000 0000 0110 0000 0000 0001 After: 1010 0000 0000 0000 0110 0000 0000 0001
- Each bit in the first operand is paired with the corresponding bit in the second operand: first bit to first bit, second bit to second bit, and so on.
- The operator is applied to each pair of bits, and the result is constructed bitwise.
For example, the binary representation of nine is 1001, and the binary representation of fifteen is 1111. So, when the bitwise operators are applied to these values, the results are as follows:
Note that all 32 bits are inverted using the Bitwise NOT operator, and that values with the most significant (left-most) bit set to 1 represent negative numbers (two's-complement representation). ~x evaluates to the same value that -x - 1 evaluates to.
Bitwise shift operators
The bitwise shift operators take two operands: the first is a quantity to be shifted, and the second specifies the number of bit positions by which the first operand is to be shifted. The direction of the shift operation is controlled by the operator used.
Shift operators convert their operands to thirty-two-bit integers and return a result of either type Number or BigInt : specifically, if the type of the left operand is BigInt , they return BigInt ; otherwise, they return Number .
The shift operators are listed in the following table.
Logical operators are typically used with Boolean (logical) values; when they are, they return a Boolean value. However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value. The logical operators are described in the following table.
Examples of expressions that can be converted to false are those that evaluate to null, 0, NaN, the empty string (""), or undefined.
The following code shows examples of the && (logical AND) operator.
The following code shows examples of the || (logical OR) operator.
The following code shows examples of the ! (logical NOT) operator.
Short-circuit evaluation
As logical expressions are evaluated left to right, they are tested for possible "short-circuit" evaluation using the following rules:
- false && anything is short-circuit evaluated to false.
- true || anything is short-circuit evaluated to true.
The rules of logic guarantee that these evaluations are always correct. Note that the anything part of the above expressions is not evaluated, so any side effects of doing so do not take effect.
Note that for the second case, in modern code you can use the Nullish coalescing operator ( ?? ) that works like || , but it only returns the second expression, when the first one is " nullish ", i.e. null or undefined . It is thus the better alternative to provide defaults, when values like '' or 0 are valid values for the first expression, too.
Most operators that can be used between numbers can be used between BigInt values as well.
One exception is unsigned right shift ( >>> ) , which is not defined for BigInt values. This is because a BigInt does not have a fixed width, so technically it does not have a "highest bit".
BigInts and numbers are not mutually replaceable — you cannot mix them in calculations.
This is because BigInt is neither a subset nor a superset of numbers. BigInts have higher precision than numbers when representing large integers, but cannot represent decimals, so implicit conversion on either side might lose precision. Use explicit conversion to signal whether you wish the operation to be a number operation or a BigInt one.
You can compare BigInts with numbers.
In addition to the comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values together, returning another string that is the union of the two operand strings.
For example,
The shorthand assignment operator += can also be used to concatenate strings.
The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two values based on a condition. The syntax is:
If condition is true, the operator has the value of val1 . Otherwise it has the value of val2 . You can use the conditional operator anywhere you would use a standard operator.
This statement assigns the value "adult" to the variable status if age is eighteen or more. Otherwise, it assigns the value "minor" to status .
The comma operator ( , ) evaluates both of its operands and returns the value of the last operand. This operator is primarily used inside a for loop, to allow multiple variables to be updated each time through the loop. It is regarded bad style to use it elsewhere, when it is not necessary. Often two separate statements can and should be used instead.
For example, if a is a 2-dimensional array with 10 elements on a side, the following code uses the comma operator to update two variables at once. The code prints the values of the diagonal elements in the array:
A unary operation is an operation with only one operand.
The delete operator deletes an object's property. The syntax is:
where object is the name of an object, property is an existing property, and propertyKey is a string or symbol referring to an existing property.
If the delete operator succeeds, it removes the property from the object. Trying to access it afterwards will yield undefined . The delete operator returns true if the operation is possible; it returns false if the operation is not possible.
Deleting array elements
Since arrays are just objects, it's technically possible to delete elements from them. This is, however, regarded as a bad practice — try to avoid it. When you delete an array property, the array length is not affected and other elements are not re-indexed. To achieve that behavior, it is much better to just overwrite the element with the value undefined . To actually manipulate the array, use the various array methods such as splice .
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned. The parentheses are optional.
Suppose you define the following variables:
The typeof operator returns the following results for these variables:
For the keywords true and null , the typeof operator returns the following results:
For a number or string, the typeof operator returns the following results:
For property values, the typeof operator returns the type of value the property contains:
For methods and functions, the typeof operator returns results as follows:
For predefined objects, the typeof operator returns results as follows:
The void operator specifies an expression to be evaluated without returning a value. expression is a JavaScript expression to evaluate. The parentheses surrounding the expression are optional, but it is good style to use them to avoid precedence issues.
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
The in operator returns true if the specified property is in the specified object. The syntax is:
where propNameOrNumber is a string, numeric, or symbol expression representing a property name or array index, and objectName is the name of an object.
The following examples show some uses of the in operator.
The instanceof operator returns true if the specified object is of the specified object type. The syntax is:
where objectName is the name of the object to compare to objectType , and objectType is an object type, such as Date or Array .
Use instanceof when you need to confirm the type of an object at runtime. For example, when catching exceptions, you can branch to different exception-handling code depending on the type of exception thrown.
For example, the following code uses instanceof to determine whether theDay is a Date object. Because theDay is a Date object, the statements in the if statement execute.
Basic expressions
All operators eventually operate on one or more basic expressions. These basic expressions include identifiers and literals , but there are a few other kinds as well. They are briefly introduced below, and their semantics are described in detail in their respective reference sections.
Use the this keyword to refer to the current object. In general, this refers to the calling object in a method. Use this either with the dot or the bracket notation:
Suppose a function called validate validates an object's value property, given the object and the high and low values:
You could call validate in each form element's onChange event handler, using this to pass it to the form element, as in the following example:
Grouping operator
The grouping operator ( ) controls the precedence of evaluation in expressions. For example, you can override multiplication and division first, then addition and subtraction to evaluate addition first.
You can use the new operator to create an instance of a user-defined object type or of one of the built-in object types. Use new as follows:
The super keyword is used to call functions on an object's parent. It is useful with classes to call the parent constructor, for example.
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

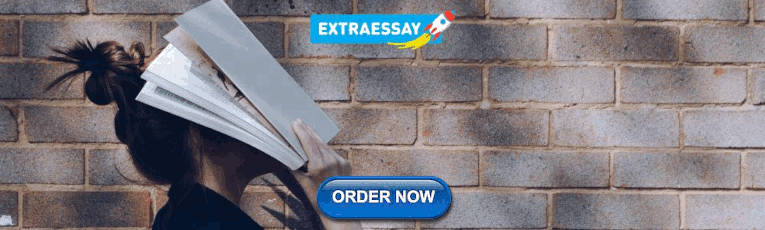
Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
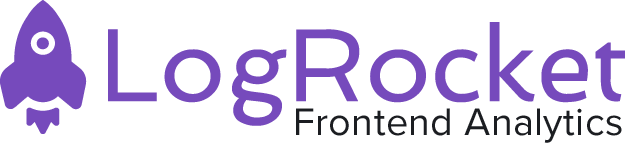
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
18 JavaScript and TypeScript shorthands to know
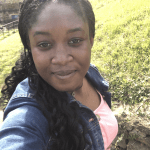
Editor’s note: This guide to the most useful JavaScript and TypeScript shorthands was last updated on 3 January 2023 to address errors in the code and include information about the satisfies operator introduced in TypeScript v4.9.
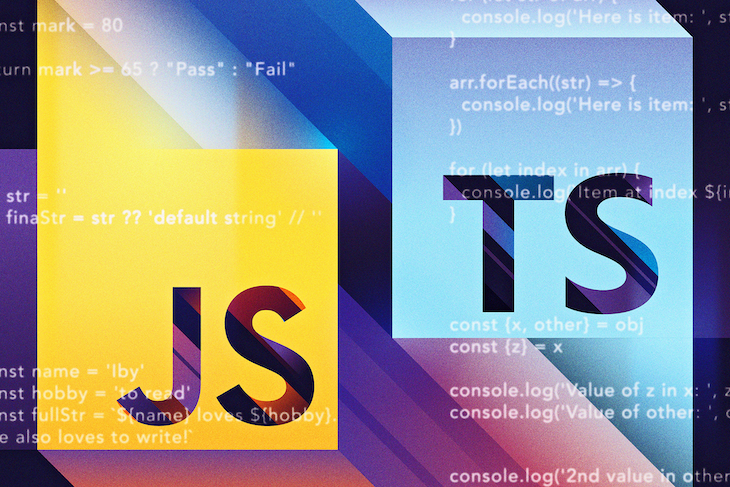
JavaScript and TypeScript share a number of useful shorthand alternatives for common code concepts. Shorthand code alternatives can help reduce lines of code, which is something we typically strive for.
In this article, we will review 18 common JavaScript and TypeScript and shorthands. We will also explore examples of how to use these shorthands.
Read through these useful JavaScript and TypeScript shorthands or navigate to the one you’re looking for in the list below.
Jump ahead:
JavaScript and TypeScript shorthands
Ternary operator, short-circuit evaluation, nullish coalescing operator, template literals, object property assignment shorthand, optional chaining, object destructuring, spread operator, object loop shorthand, array.indexof shorthand using the bitwise operator, casting values to boolean with , arrow/lambda function expression, implicit return using arrow function expressions, double bitwise not operator, exponent power shorthand, typescript constructor shorthand, typescript satisfies operator.
Using shorthand code is not always the right decision when writing clean and scalable code . Concise code can sometimes be more confusing to read and update. So, it is important that your code is legible and conveys meaning and context to other developers.
Our decision to use shorthands must not be detrimental to other desirable code characteristics. Keep this in mind when using the following shorthands for expressions and operators in JavaScript and TypeScript.
All shorthands available in JavaScript are available in the same syntax in TypeScript. The only slight differences are in specifying the type in TypeScript, and the TypeScript constructor shorthand is exclusive to TypeScript.
The ternary operator is one of the most popular shorthands in JavaScript and TypeScript. It replaces the traditional if…else statement. Its syntax is as follows:
The following example demonstrates a traditional if…else statement and its shorthand equivalent using the ternary operator:
The ternary operator is great when you have single-line operations like assigning a value to a variable or returning a value based on two possible conditions. Once there are more than two outcomes to your condition, using if/else blocks are much easier to read.
Another way to replace an if…else statement is with short-circuit evaluation. This shorthand uses the logical OR operator || to assign a default value to a variable when the intended value is falsy.
The following example demonstrates how to use short-circuit evaluation:
This shorthand is best used when you have a single-line operation and your condition depends on the falseness or non-falseness of a value/statement.
The nullish coalescing operator ?? is similar to short-circuit evaluation in that it assigns a variable a default value. However, the nullish coalescing operator only uses the default value when the intended value is also nullish.
In other words, if the intended value is falsy but not nullish, it will not use the default value.
Here are two examples of the nullish coalescing operator:
Example one
Example two, logical nullish assignment operator.
This is similar to the nullish coalescing operator by checking that a value is nullish and has added the ability to assign a value following the null check.
The example below demonstrates how we would check and assign in longhand and shorthand using the logical nullish assignment:
JavaScript has several other assignment shorthands like addition assignment += , multiplication assignment *= , division assignment /= , remainder assignment %= , and several others. You can find a full list of assignment operators here .
Template literals, which was introduced as part of JavaScript’s powerful ES6 features , can be used instead of + to concatenate multiple variables within a string. To use template literals, wrap your strings in `` and variables in ${} within those strings.
The example below demonstrates how to use template literals to perform string interpolation:
You can also use template literals to build multi-line strings without using \n . For example:
Using template literals is helpful for adding strings whose values may change into a larger string, like HTML templates. They are also useful for creating multi-line string because string wrapped in template literals retain all white spacing and indentation.
In JavaScript and TypeScript, you can assign a property to an object in shorthand by mentioning the variable in the object literal. To do this, the variable must be named with the intended key.
See an example of the object property assignment shorthand below:
Dot notation allows us to access the keys or values of an object. With optional chaining , we can go a step further and read keys or values even when we are not sure whether they exist or are set.
When the key does not exist, the value from optional chaining is undefined . This helps us avoid unneeded if/else check conditions when reading values from objects and unnecessary try/catch to handle errors thrown from trying to access object keys that don’t exist.
See an example of optional chaining in action below:
Besides the traditional dot notation, another way to read the values of an object is by destructuring the object’s values into their own variables.
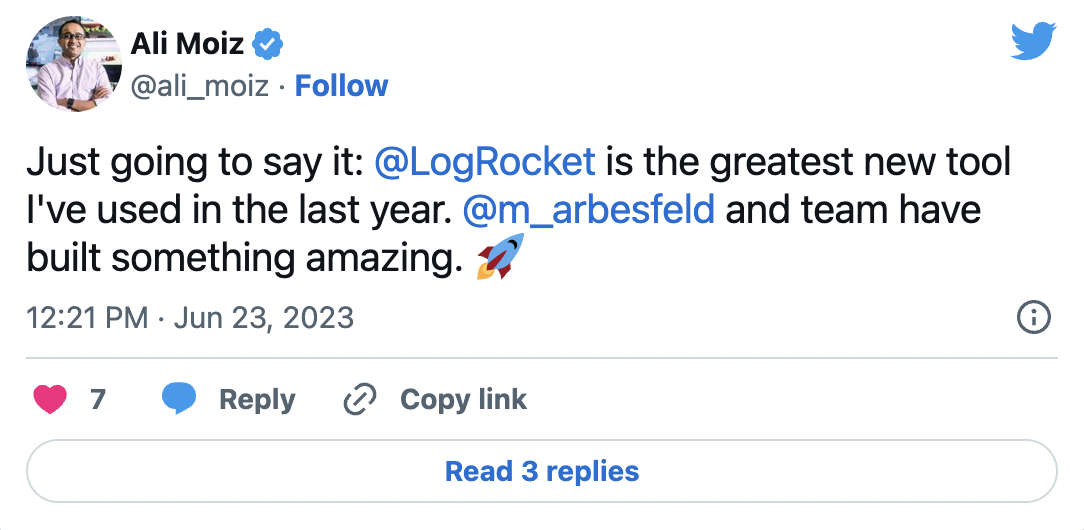
Over 200k developers use LogRocket to create better digital experiences

The following example demonstrates how to read the values of an object using the traditional dot notation compared to the shorthand method using object destructuring:
The spread operator … is used to access the content of arrays and objects. You can use the spread operator to replace array functions , like concat , and object functions, like object.assign .
Review the examples below to see how the spread operator can replace longhand array and object functions:
The traditional JavaScript for loop syntax is as follows:
We can use this loop syntax to iterate through arrays by referencing the array length for the iterator. There are three for loop shorthands that offer different ways to iterate through an array object:
- for…of : To access the array entries
- for…in : To access the indexes of an array and the keys when used on an object literal
- Array.forEach : To perform operations on the array elements and their indexes using a callback function
Please note, Array.forEach callbacks have three possible arguments, which are called in this order:
- The element of the array for the ongoing iteration
- The element’s index
- A full copy of the array
The examples below demonstrate these object loop shorthands in action:
We can look up the existence of an item in an array using the Array.indexOf method. This method returns the index position of the item if it exists in the array and returns -1 if it does not.
In JavaScript, 0 is a falsy value, while numbers less than or greater than 0 are considered truthy. Typically, this means we need to use an if…else statement to determine if the item exists using the returned index.
Using the bitwise operator ~ instead of an if…else statement allows us to get a truthy value for anything greater than or equal to 0 .
The example below demonstrates the Array.indexOf shorthand using the bitwise operator instead of an if…else statement:
In JavaScript, we can cast variables of any type to a Boolean value using the !![variable] shorthand.
See an example of using the !! [variable] shorthand to cast values to Boolean :
Functions in JavaScript can be written using arrow function syntax instead of the traditional expression that explicitly uses the function keyword. Arrow functions are similar to lambda functions in other languages .
Take a look at this example of writing a function in shorthand using an arrow function expression:
In JavaScript, we typically use the return keyword to return a value from a function. When we define our function using arrow function syntax, we can implicitly return a value by excluding braces {} .
For multi-line statements, such as expressions, we can wrap our return expression in parentheses () . The example below demonstrates the shorthand code for implicitly returning a value from a function using an arrow function expression:
In JavaScript, we typically access mathematical functions and constants using the built-in Math object. Some of those functions are Math.floor() , Math.round() , Math.trunc() , and many others.
The Math.trunc() (available in ES6) returns the integer part. For example, number(s) before the decimal of a given number achieves this same result using the Double bitwise NOT operator ~~ .
Review the example below to see how to use the Double bitwise NOT operator as a Math.trunc() shorthand:
It is important to note that the Double bitwise NOT operator ~~ is not an official shorthand for Math.trunc because some edge cases do not return the same result. More details on this are available here .
Another mathematical function with a useful shorthand is the Math.pow() function. The alternative to using the built-in Math object is the ** shorthand.
The example below demonstrates this exponent power shorthand in action:
There is a shorthand for creating a class and assigning values to class properties via the constructor in TypeScript . When using this method, TypeScript will automatically create and set the class properties . This shorthand is exclusive to TypeScript alone and not available in JavaScript class definitions.
Take a look at the example below to see the TypeScript constructor shorthand in action:
The satisfies operator gives some flexibility from the constraints of setting a type with the error handling covering having explicit types.
It is best used when a value has multiple possible types. For example, it can be a string or an array; with this operator, we don’t have to add any checks. Here’s an example:
In the longhand version of our example above, we had to do a typeof check to make sure palette.red was of the type RGB and that we could read its first property with at .
While in our shorthand version, using satisfies , we don’t have the type restriction of palette.red being string , but we can still tell the compiler to make sure palette and its properties have the correct shape.
The Array.property.at() i.e., at() method, accepts an integer and returns the item at that index. Array.at requires ES2022 target, which is available from TypeScript v4.6 onwards. More information is available here .
These are just a few of the most commonly used JavaScript and TypeScript shorthands.
JavaScript and TypeScript longhand and shorthand code typically work the same way under the hood, so choosing shorthand usually just means writing less lines of code. Remember, using shorthand code is not always the best option. What is most important is writing clean and understandable code that other developers can read easily.
What are your favorite JavaScript or TypeScript shorthands? Share them with us in the comments!
LogRocket : Debug JavaScript errors more easily by understanding the context
Debugging code is always a tedious task. But the more you understand your errors, the easier it is to fix them.
LogRocket allows you to understand these errors in new and unique ways. Our frontend monitoring solution tracks user engagement with your JavaScript frontends to give you the ability to see exactly what the user did that led to an error.
LogRocket records console logs, page load times, stack traces, slow network requests/responses with headers + bodies, browser metadata, and custom logs. Understanding the impact of your JavaScript code will never be easier!
Try it for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #typescript
- #vanilla javascript
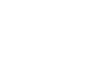
Stop guessing about your digital experience with LogRocket
Recent posts:.
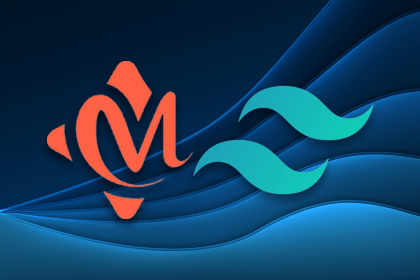
Mojo CSS vs. Tailwind: Choosing the best CSS framework
Compare the established Tailwind CSS framework with the newer Mojo CSS based on benchmarks like plugins, built-in components, and more.
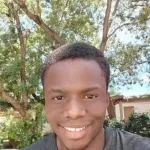
Leader Spotlight: Riding the rocket ship of scale, with Justin Kitagawa
We sit down with Justin Kitagawa to learn more about his leadership style and approach for handling the complexities that come with scaling fast.
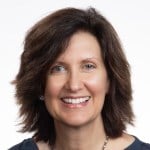
Nx adoption guide: Overview, examples, and alternatives
Let’s explore Nx features, use cases, alternatives, and more to help you assess whether it’s the right tool for your needs.
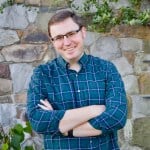
Understanding security in React Native applications
Explore the various security threats facing React Native mobile applications and how to mitigate them.
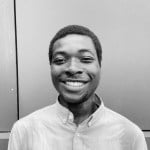
8 Replies to "18 JavaScript and TypeScript shorthands to know"
Thanks you for your article, I learn a lot with it.
But I think that I found a mistake in short circuit evaluation. When you show the traditional version with if…else statement you use logical && operator but I think you wanted use logical || operator.
I think that is just a wrting error but i prefer tell it to you.
Have a good day
Hi Romain thank you for spotting that! I’ll fix it right away
I was avoiding using logical OR to make clear the explanation of short circuit evaluation, so the if statement should be confirming “str” has a valid value. I have switched the assignment statements in the condition so it is correct now.
I think there is an error in the renamed variable of destructured object. Shouldn’t the line const {x: myVar} = object be: const {x: myVar} = obj
This code doesn’t work in Typescript? // for object literals const obj2 = { a: 1, b: 2, c: 3 }
for (let keyLetter in obj2) { console.log(`key: ${keyLetter} value: ${obj2[keyLetter]}`);
Gets error: error: TS7053 [ERROR]: Element implicitly has an ‘any’ type because expression of type ‘string’ can’t be used to index type ‘{ 0: number; 1: number; 2: number; }’. No index signature with a parameter of type ‘string’ was found on type ‘{ 0: number; 1: number; 2: number; }’. console.log(`key: ${keyLetter} value: ${obj2[keyLetter]}`);
Awesome information, thanks for sharing!!! 🚀🚀🚀
Good List of useful operators
~~x is not the same as Math.floor(x) : try it on negative numbers. You’ll find that ~~ is the same as Math.trunc(x) instead.
Leave a Reply Cancel reply

JavaScript — Shorthand Variable Assignment
A three minute introduction into shorthand variable assignment.
Brandon Morelli
This article will take a (very) quick look at shorthand variable assignment in JavaScript.
Assigning Variables to Other Variables
As you’re probably aware, you can assign values to variables separately, like this:
However, if all variables are being assigned equal values, you can shorthand and assign the variables like this:
The assignment operator = in JavaScript has right-to-left associativity. This means that it works from the right of the line, to the left of the line. In this example, here is the order of operation:
- 1 — First, c is set to 1 .
- 2 — Next, b is set equal to c which is already equal to 1 . Therefor, b is set to 1 .
- 3 — Finally, a is set equal to b which is already equal to 1 . Therefor, a is set to 1 .
As you can now see, the shorthand above results in a , b , and c all being set to 1 .
However, this is not a recommended way to assign variables. That’s because in the shorthand variable assignment shown above, we actually never end up declaring variables b or c . Because of this, b and c wont be locally scoped to the current block of code. Both variables b and c will instead be globally scoped and end up polluting the global namespace.
Using Commas When Assigning Variables
Lets look at a new example. Consider the following variable declarations and assignments:
We can shorthand this code using commas:
As you see, we are separating each variable assignment with a comma which allows us to assign different values to each variable.
For ease of reading, most coders who prefer using the comma method will structure their variable assignments like this:
Best of all, in the shorthand variable assignment shown above, we are declaring all three variables: d , e , and f . Because of this, all variables will be locally scoped and we’re able to avoid any scoping problems.
Want to Learn More Shorthands?
Check out my other articles on shorthand coding techniques in JavaScript:
- JavaScript — The Conditional (Ternary) Operator Explained
- JavaScript — Short Circuit Conditionals
- JavaScript — Short Circuit Evaluation
Closing Notes:
Thanks for reading! If you’re ready to finally learn Web Development, check out: The Ultimate Guide to Learning Full Stack Web Development in 6 months .
If you’re working towards becoming a better JavaScript Developer, check out: Ace Your Javascript Interview — Learn Algorithms + Data Structures .
I publish 4 articles on web development each week. Please consider entering your email here if you’d like to be added to my once-weekly email list, or follow me on Twitter .
If this post was helpful, please click the clap 👏 button below a few times to show your support! ⬇⬇
Written by Brandon Morelli
Creator of @codeburstio — Frequently posting web development tutorials & articles. Follow me on Twitter too: @BrandonMorelli
More from Brandon Morelli and codeburst
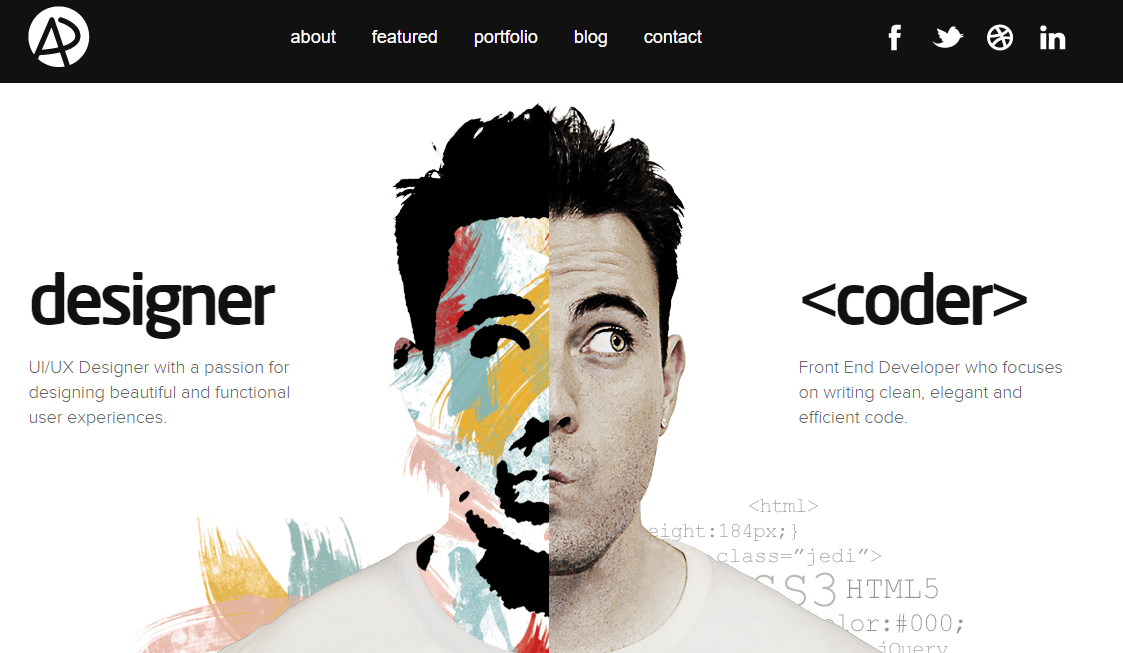
10 Awesome Web Developer Portfolios
Get inspired with these 10 web developer portfolios..
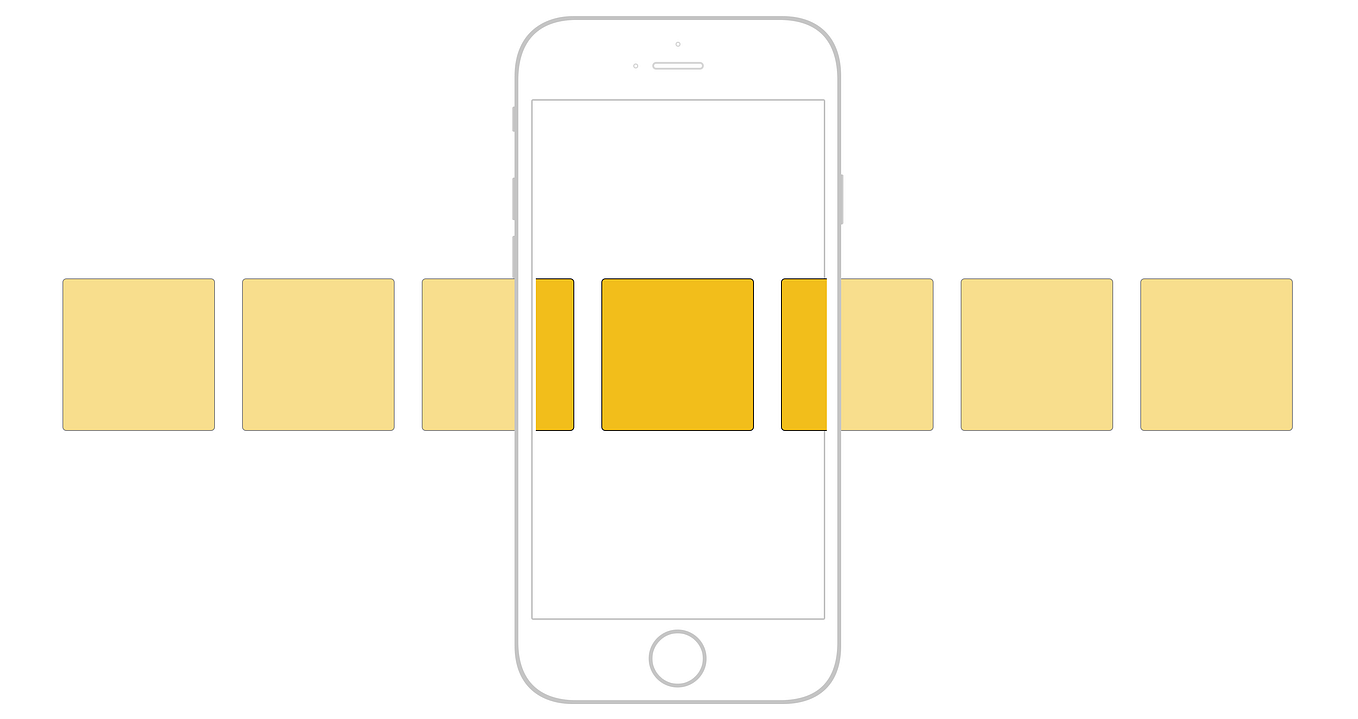
How To Create Horizontal Scrolling Containers
As a front end developer, more and more frequently i am given designs that include a horizontal scrolling component. this has become….
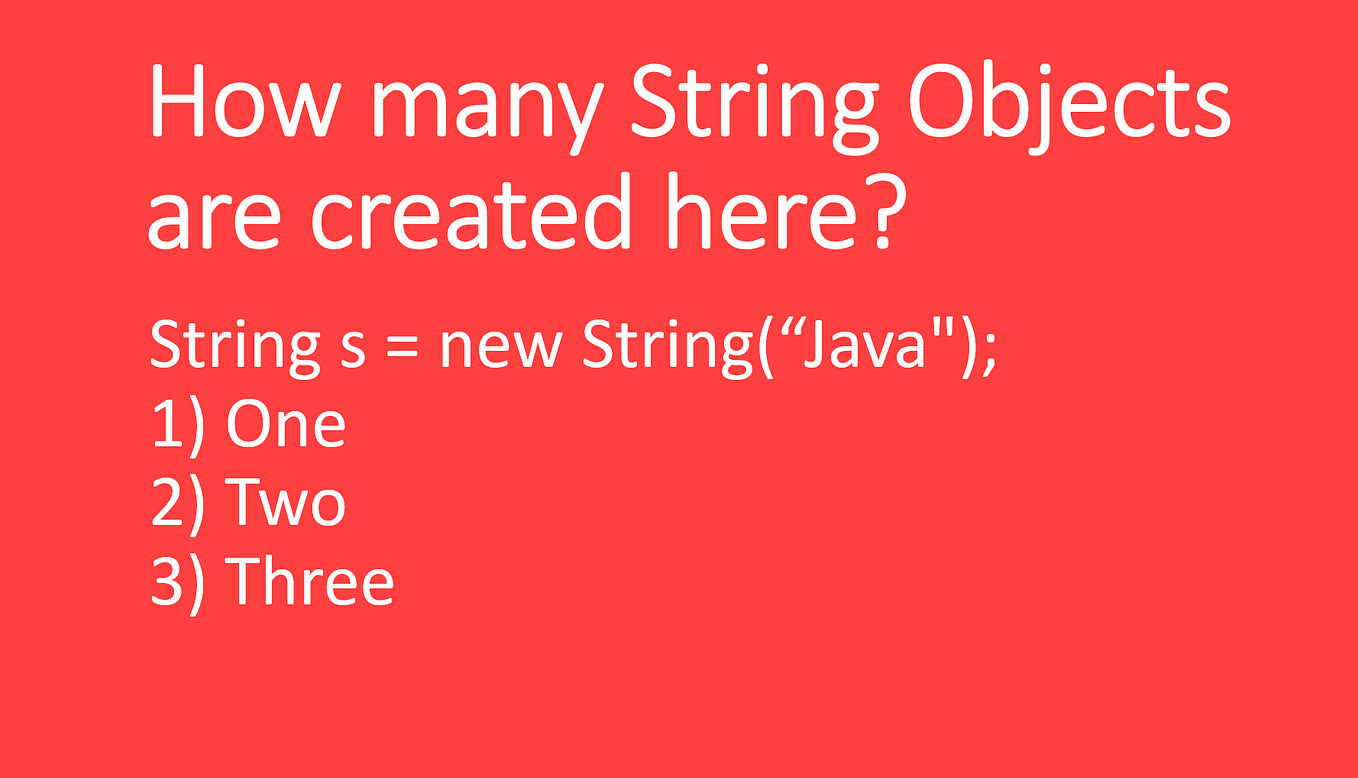
Top 50 Java Interview Questions for Beginners and Junior Developers
A list of frequently asked java questions and answers from programming job interviews of java developers of different experience..
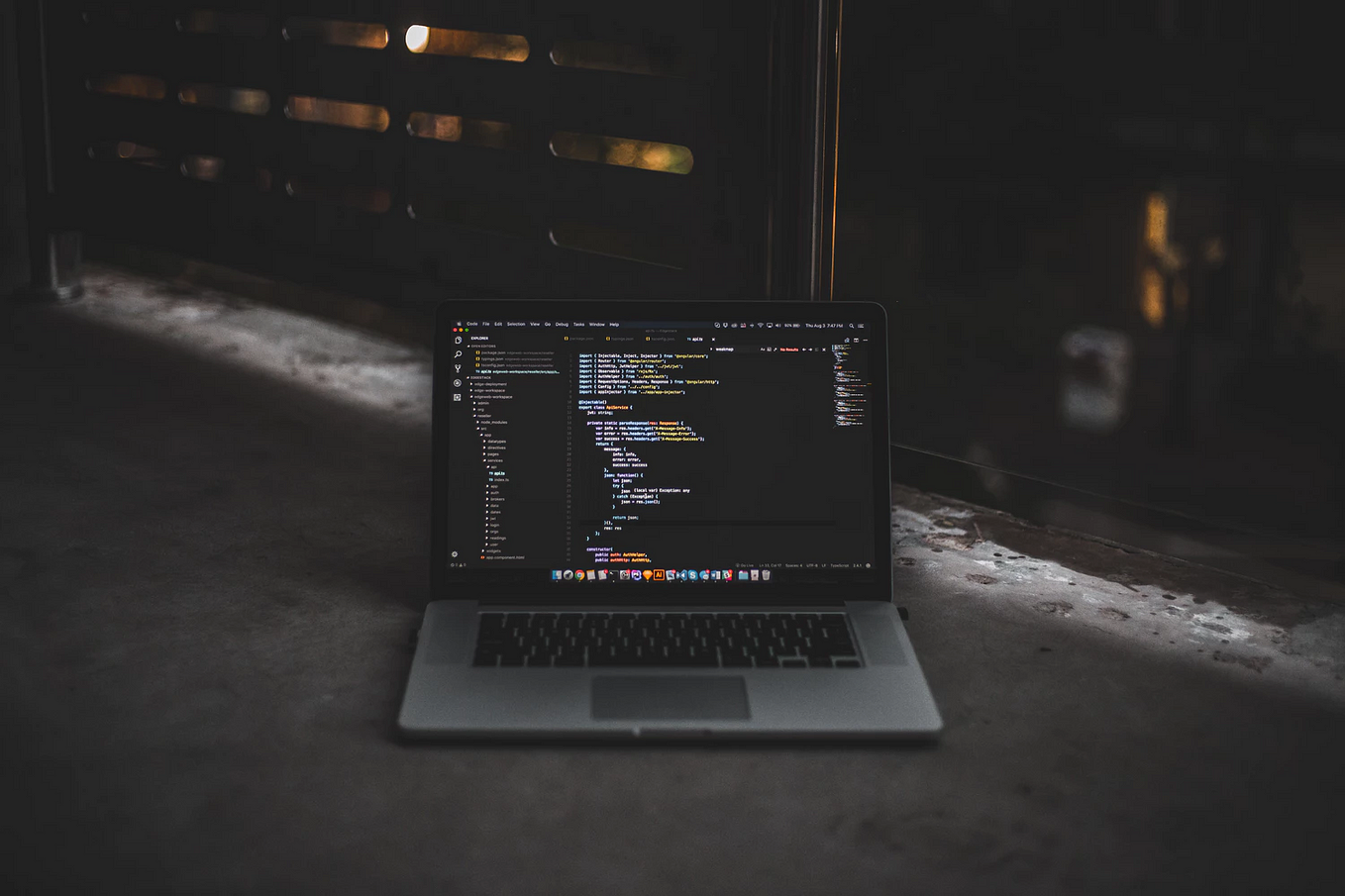
JavaScript ES 2017: Learn Async/Await by Example
Async/await explained through a clear example., recommended from medium.
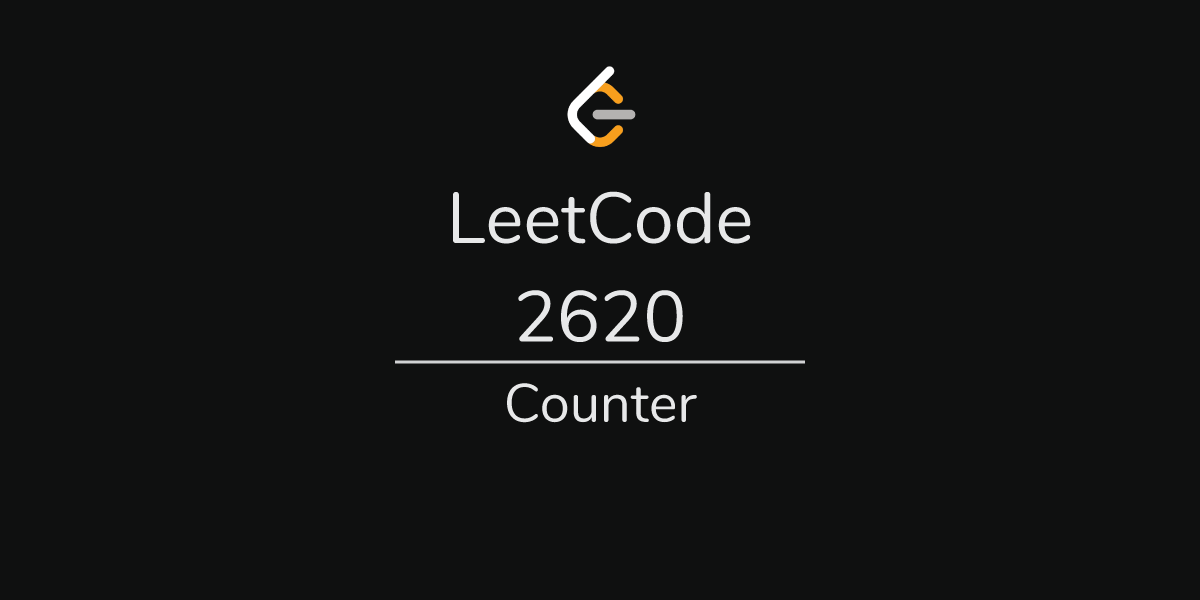
Evan Roberts
LeetCode Problem 2620 Counter — LeetCode: 30 Days of JavaScript
Solving leetcode 30 days of javascript study plan problems.
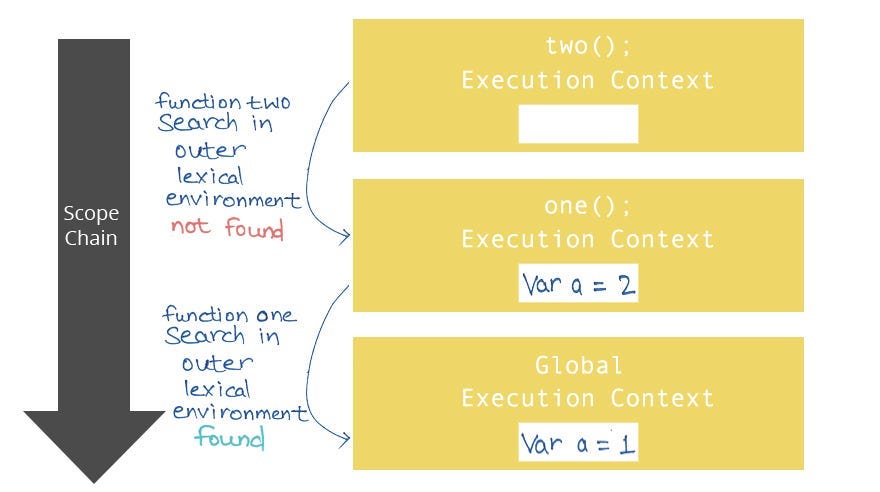
Lexical environment in JavaScript
A lexical environment in javascript is a data structure that stores the variables and functions that are defined in the current scope and….

General Coding Knowledge

Stories to Help You Grow as a Software Developer
Coding & Development

ChatGPT prompts
Ahmad Faisal Frogh
Stackademic
React vs Vanilla JavaScript
Why react rocks for web development.
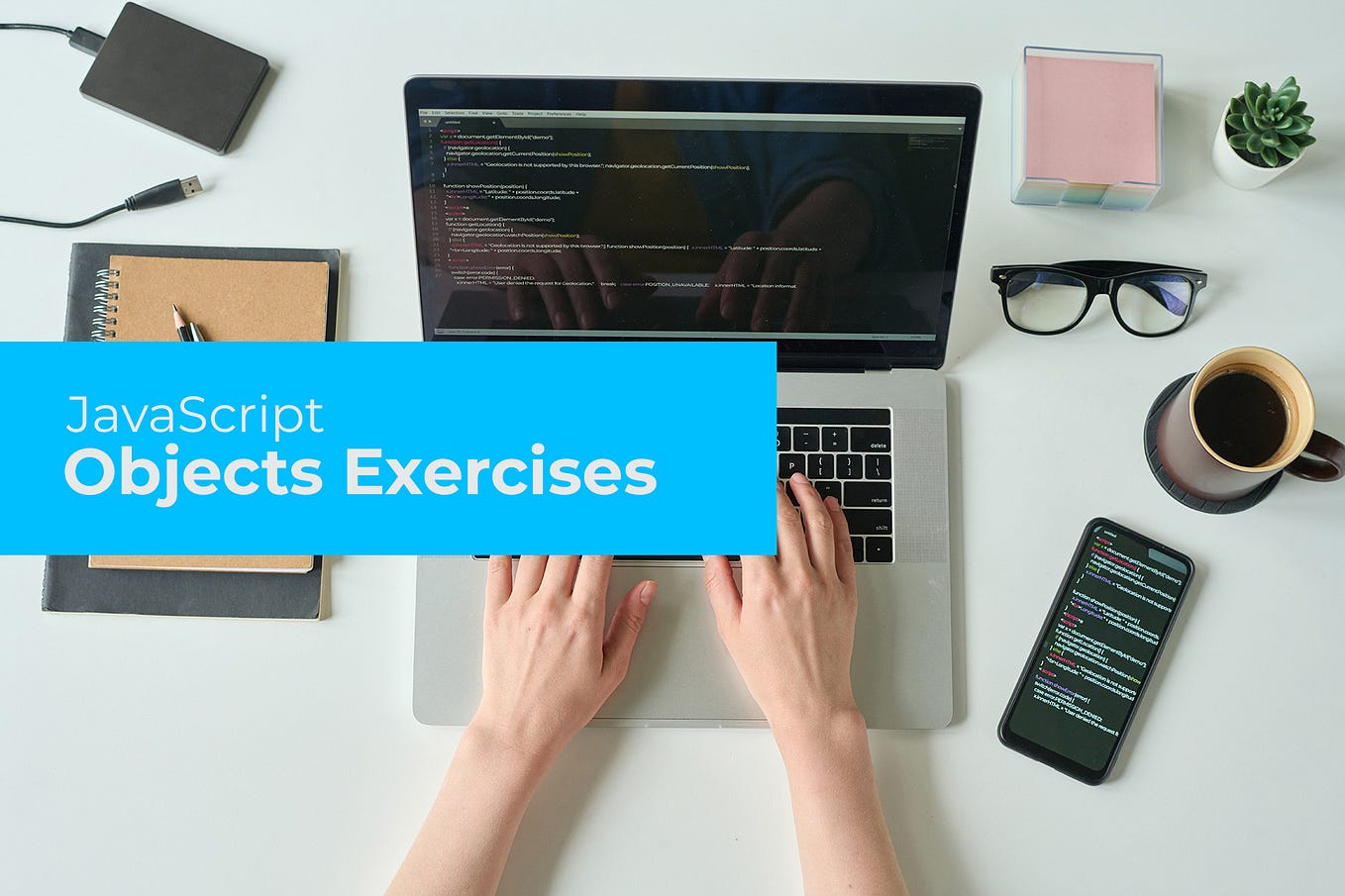
Francesco Saviano
JavaScript Objects: 10 Real-World Exercises
In the dynamic world of web development, javascript stands as a cornerstone, powering interactive and sophisticated web applications. at….
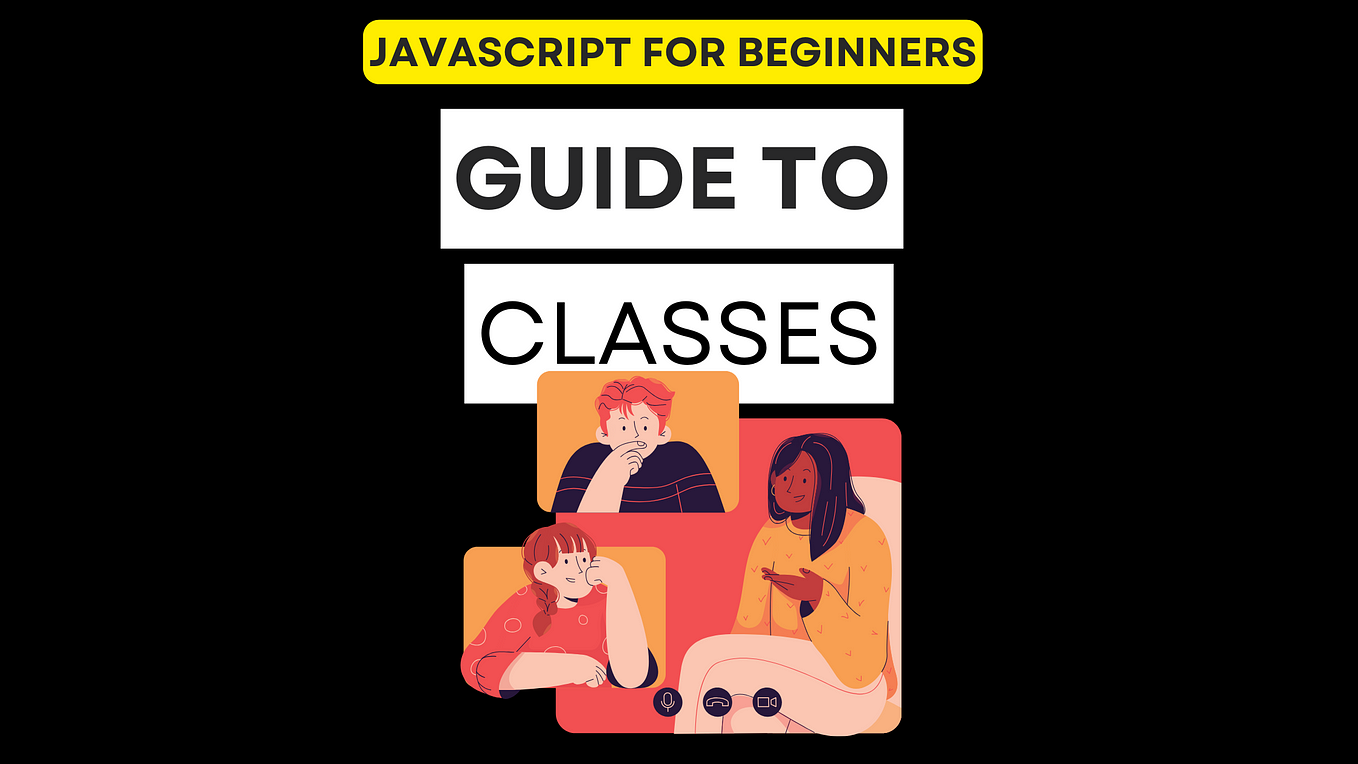
Ekaterine Mitagvaria
JavaScript for Beginners: Classes
Javascript, compared to many other programming languages is a prototype-based language. the creation and behavior of objects are based on….
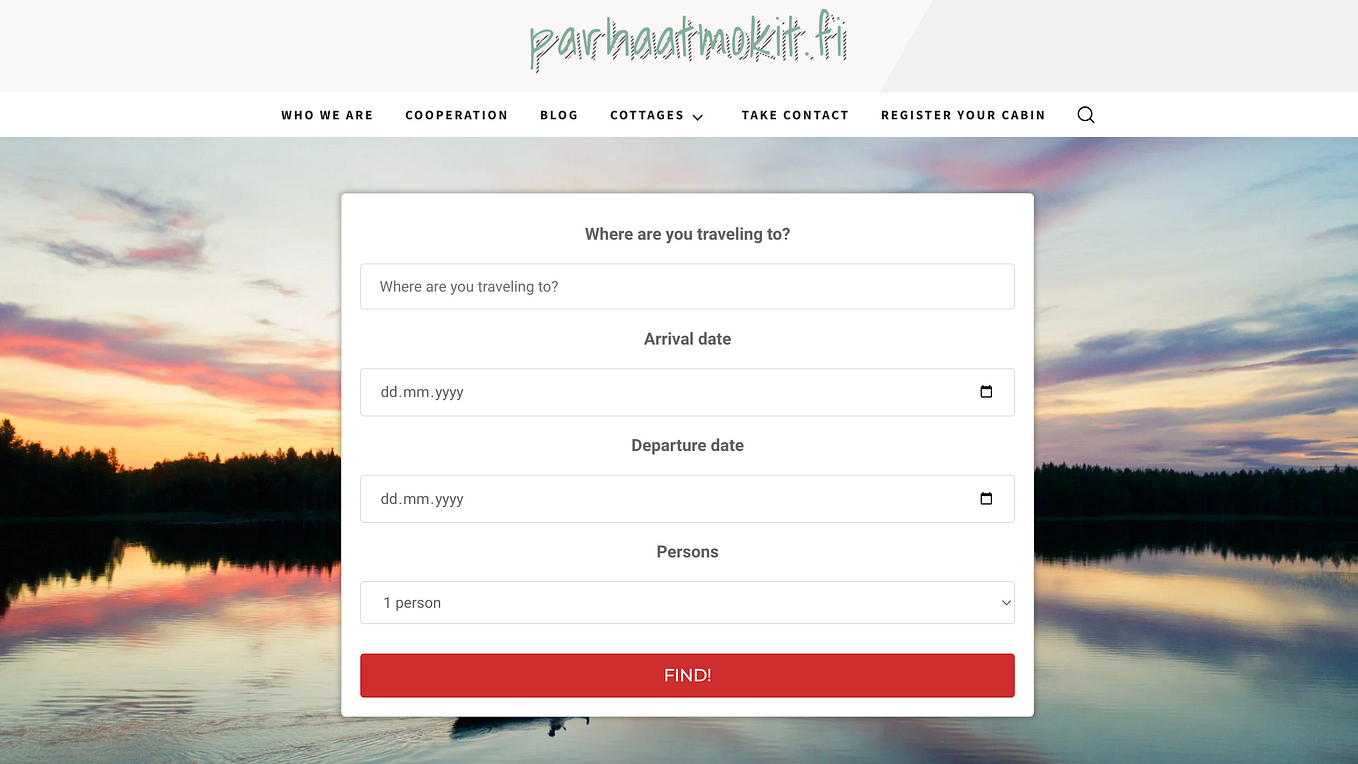
Artturi Jalli
I Built an App in 6 Hours that Makes $1,500/Mo
Copy my strategy.
Text to speech
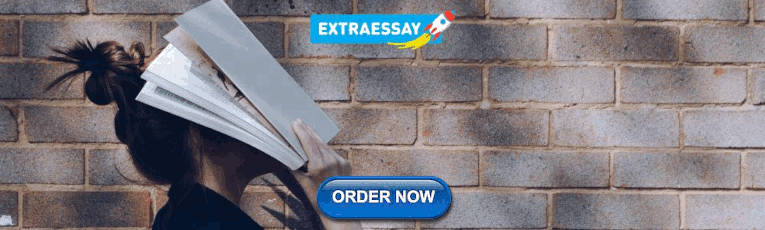
IMAGES
VIDEO
COMMENTS
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
In JavaScript and TypeScript, you can assign a property to an object in shorthand by mentioning the variable in the object literal. To do this, the variable must be named with the intended key. See an example of the object property assignment shorthand below: // Longhand const obj = { x: 1, y: 2, z: 3 }
Now, here’s what the shorthand code to do this looks like: let x = 1, y = 2; x += y; console.log(x); // 3. Instead of using x = x + y, we can write x += y. Both of these code bits do the exact same thing — add x + y, then assign the resulting value to x. Other Shorthands. Best of all, this pattern can be followed for other assignment operators.
However, if all variables are being assigned equal values, you can shorthand and assign the variables like this: var a = b = c = 1; The assignment operator = in JavaScript has right-to-left associativity. This means that it works from the right of the line, to the left of the line. In this example, here is the order of operation: 1 — First, c ...