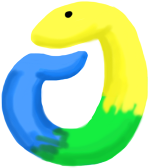
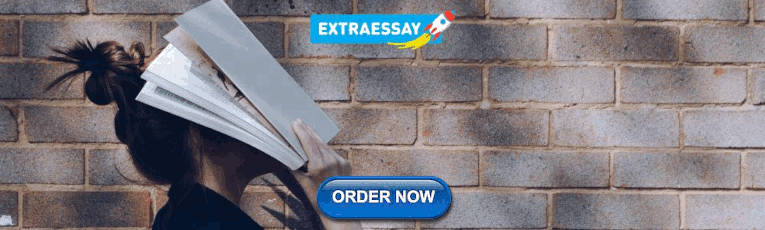
Practice Python
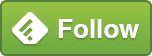
Beginner Python exercises
- Why Practice Python?
- Why Chilis?
- Resources for learners
All Exercises

All Solutions
- 1: Character Input Solutions
- 2: Odd Or Even Solutions
- 3: List Less Than Ten Solutions
- 4: Divisors Solutions
- 5: List Overlap Solutions
- 6: String Lists Solutions
- 7: List Comprehensions Solutions
- 8: Rock Paper Scissors Solutions
- 9: Guessing Game One Solutions
- 10: List Overlap Comprehensions Solutions
- 11: Check Primality Functions Solutions
- 12: List Ends Solutions
- 13: Fibonacci Solutions
- 14: List Remove Duplicates Solutions
- 15: Reverse Word Order Solutions
- 16: Password Generator Solutions
- 17: Decode A Web Page Solutions
- 18: Cows And Bulls Solutions
- 19: Decode A Web Page Two Solutions
- 20: Element Search Solutions
- 21: Write To A File Solutions
- 22: Read From File Solutions
- 23: File Overlap Solutions
- 24: Draw A Game Board Solutions
- 25: Guessing Game Two Solutions
- 26: Check Tic Tac Toe Solutions
- 27: Tic Tac Toe Draw Solutions
- 28: Max Of Three Solutions
- 29: Tic Tac Toe Game Solutions
- 30: Pick Word Solutions
- 31: Guess Letters Solutions
- 32: Hangman Solutions
- 33: Birthday Dictionaries Solutions
- 34: Birthday Json Solutions
- 35: Birthday Months Solutions
- 36: Birthday Plots Solutions
- 37: Functions Refactor Solution
- 38: f Strings Solution
- 39: Character Input Datetime Solution
- 40: Error Checking Solution
10 Python Practice Exercises for Beginners with Solutions

- python basics
- get started with python
- online practice
A great way to improve quickly at programming with Python is to practice with a wide range of exercises and programming challenges. In this article, we give you 10 Python practice exercises to boost your skills.
Practice exercises are a great way to learn Python. Well-designed exercises expose you to new concepts, such as writing different types of loops, working with different data structures like lists, arrays, and tuples, and reading in different file types. Good exercises should be at a level that is approachable for beginners but also hard enough to challenge you, pushing your knowledge and skills to the next level.
If you’re new to Python and looking for a structured way to improve your programming, consider taking the Python Basics Practice course. It includes 17 interactive exercises designed to improve all aspects of your programming and get you into good programming habits early. Read about the course in the March 2023 episode of our series Python Course of the Month .
Take the course Python Practice: Word Games , and you gain experience working with string functions and text files through its 27 interactive exercises. Its release announcement gives you more information and a feel for how it works.
Each course has enough material to keep you busy for about 10 hours. To give you a little taste of what these courses teach you, we have selected 10 Python practice exercises straight from these courses. We’ll give you the exercises and solutions with detailed explanations about how they work.
To get the most out of this article, have a go at solving the problems before reading the solutions. Some of these practice exercises have a few possible solutions, so also try to come up with an alternative solution after you’ve gone through each exercise.
Let’s get started!
Exercise 1: User Input and Conditional Statements
Write a program that asks the user for a number then prints the following sentence that number of times: ‘I am back to check on my skills!’ If the number is greater than 10, print this sentence instead: ‘Python conditions and loops are a piece of cake.’ Assume you can only pass positive integers.
Here, we start by using the built-in function input() , which accepts user input from the keyboard. The first argument is the prompt displayed on the screen; the input is converted into an integer with int() and saved as the variable number. If the variable number is greater than 10, the first message is printed once on the screen. If not, the second message is printed in a loop number times.
Exercise 2: Lowercase and Uppercase Characters
Below is a string, text . It contains a long string of characters. Your task is to iterate over the characters of the string, count uppercase letters and lowercase letters, and print the result:
We start this one by initializing the two counters for uppercase and lowercase characters. Then, we loop through every letter in text and check if it is lowercase. If so, we increment the lowercase counter by one. If not, we check if it is uppercase and if so, we increment the uppercase counter by one. Finally, we print the results in the required format.
Exercise 3: Building Triangles
Create a function named is_triangle_possible() that accepts three positive numbers. It should return True if it is possible to create a triangle from line segments of given lengths and False otherwise. With 3 numbers, it is sometimes, but not always, possible to create a triangle: You cannot create a triangle from a = 13, b = 2, and c = 3, but you can from a = 13, b = 9, and c = 10.
The key to solving this problem is to determine when three lines make a triangle regardless of the type of triangle. It may be helpful to start drawing triangles before you start coding anything.

Notice that the sum of any two sides must be larger than the third side to form a triangle. That means we need a + b > c, c + b > a, and a + c > b. All three conditions must be met to form a triangle; hence we need the and condition in the solution. Once you have this insight, the solution is easy!
Exercise 4: Call a Function From Another Function
Create two functions: print_five_times() and speak() . The function print_five_times() should accept one parameter (called sentence) and print it five times. The function speak(sentence, repeat) should have two parameters: sentence (a string of letters), and repeat (a Boolean with a default value set to False ). If the repeat parameter is set to False , the function should just print a sentence once. If the repeat parameter is set to True, the function should call the print_five_times() function.
This is a good example of calling a function in another function. It is something you’ll do often in your programming career. It is also a nice demonstration of how to use a Boolean flag to control the flow of your program.
If the repeat parameter is True, the print_five_times() function is called, which prints the sentence parameter 5 times in a loop. Otherwise, the sentence parameter is just printed once. Note that in Python, writing if repeat is equivalent to if repeat == True .
Exercise 5: Looping and Conditional Statements
Write a function called find_greater_than() that takes two parameters: a list of numbers and an integer threshold. The function should create a new list containing all numbers in the input list greater than the given threshold. The order of numbers in the result list should be the same as in the input list. For example:
Here, we start by defining an empty list to store our results. Then, we loop through all elements in the input list and test if the element is greater than the threshold. If so, we append the element to the new list.
Notice that we do not explicitly need an else and pass to do nothing when integer is not greater than threshold . You may include this if you like.
Exercise 6: Nested Loops and Conditional Statements
Write a function called find_censored_words() that accepts a list of strings and a list of special characters as its arguments, and prints all censored words from it one by one in separate lines. A word is considered censored if it has at least one character from the special_chars list. Use the word_list variable to test your function. We've prepared the two lists for you:
This is another nice example of looping through a list and testing a condition. We start by looping through every word in word_list . Then, we loop through every character in the current word and check if the current character is in the special_chars list.
This time, however, we have a break statement. This exits the inner loop as soon as we detect one special character since it does not matter if we have one or several special characters in the word.
Exercise 7: Lists and Tuples
Create a function find_short_long_word(words_list) . The function should return a tuple of the shortest word in the list and the longest word in the list (in that order). If there are multiple words that qualify as the shortest word, return the first shortest word in the list. And if there are multiple words that qualify as the longest word, return the last longest word in the list. For example, for the following list:
the function should return
Assume the input list is non-empty.
The key to this problem is to start with a “guess” for the shortest and longest words. We do this by creating variables shortest_word and longest_word and setting both to be the first word in the input list.
We loop through the words in the input list and check if the current word is shorter than our initial “guess.” If so, we update the shortest_word variable. If not, we check to see if it is longer than or equal to our initial “guess” for the longest word, and if so, we update the longest_word variable. Having the >= condition ensures the longest word is the last longest word. Finally, we return the shortest and longest words in a tuple.
Exercise 8: Dictionaries
As you see, we've prepared the test_results variable for you. Your task is to iterate over the values of the dictionary and print all names of people who received less than 45 points.
Here, we have an example of how to iterate through a dictionary. Dictionaries are useful data structures that allow you to create a key (the names of the students) and attach a value to it (their test results). Dictionaries have the dictionary.items() method, which returns an object with each key:value pair in a tuple.
The solution shows how to loop through this object and assign a key and a value to two variables. Then, we test whether the value variable is greater than 45. If so, we print the key variable.
Exercise 9: More Dictionaries
Write a function called consonant_vowels_count(frequencies_dictionary, vowels) that takes a dictionary and a list of vowels as arguments. The keys of the dictionary are letters and the values are their frequencies. The function should print the total number of consonants and the total number of vowels in the following format:
For example, for input:
the output should be:
Working with dictionaries is an important skill. So, here’s another exercise that requires you to iterate through dictionary items.
We start by defining a list of vowels. Next, we need to define two counters, one for vowels and one for consonants, both set to zero. Then, we iterate through the input dictionary items and test whether the key is in the vowels list. If so, we increase the vowels counter by one, if not, we increase the consonants counter by one. Finally, we print out the results in the required format.
Exercise 10: String Encryption
Implement the Caesar cipher . This is a simple encryption technique that substitutes every letter in a word with another letter from some fixed number of positions down the alphabet.
For example, consider the string 'word' . If we shift every letter down one position in the alphabet, we have 'xpse' . Shifting by 2 positions gives the string 'yqtf' . Start by defining a string with every letter in the alphabet:
Name your function cipher(word, shift) , which accepts a string to encrypt, and an integer number of positions in the alphabet by which to shift every letter.
This exercise is taken from the Word Games course. We have our string containing all lowercase letters, from which we create a shifted alphabet using a clever little string-slicing technique. Next, we create an empty string to store our encrypted word. Then, we loop through every letter in the word and find its index, or position, in the alphabet. Using this index, we get the corresponding shifted letter from the shifted alphabet string. This letter is added to the end of the new_word string.
This is just one approach to solving this problem, and it only works for lowercase words. Try inputting a word with an uppercase letter; you’ll get a ValueError . When you take the Word Games course, you slowly work up to a better solution step-by-step. This better solution takes advantage of two built-in functions chr() and ord() to make it simpler and more robust. The course contains three similar games, with each game comprising several practice exercises to build up your knowledge.
Do You Want More Python Practice Exercises?
We have given you a taste of the Python practice exercises available in two of our courses, Python Basics Practice and Python Practice: Word Games . These courses are designed to develop skills important to a successful Python programmer, and the exercises above were taken directly from the courses. Sign up for our platform (it’s free!) to find more exercises like these.
We’ve discussed Different Ways to Practice Python in the past, and doing interactive exercises is just one way. Our other tips include reading books, watching videos, and taking on projects. For tips on good books for Python, check out “ The 5 Best Python Books for Beginners .” It’s important to get the basics down first and make sure your practice exercises are fun, as we discuss in “ What’s the Best Way to Practice Python? ” If you keep up with your practice exercises, you’ll become a Python master in no time!
You may also like
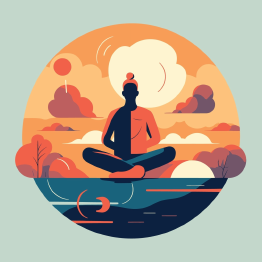
How Do You Write a SELECT Statement in SQL?
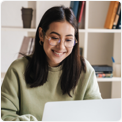
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Exercise with Practice Questions and Solutions
- Python List Exercise
- Python String Exercise
- Python Tuple Exercise
- Python Dictionary Exercise
- Python Set Exercise
Python Matrix Exercises
- Python program to a Sort Matrix by index-value equality count
- Python Program to Reverse Every Kth row in a Matrix
- Python Program to Convert String Matrix Representation to Matrix
- Python - Count the frequency of matrix row length
- Python - Convert Integer Matrix to String Matrix
- Python Program to Convert Tuple Matrix to Tuple List
- Python - Group Elements in Matrix
- Python - Assigning Subsequent Rows to Matrix first row elements
- Adding and Subtracting Matrices in Python
- Python - Convert Matrix to dictionary
- Python - Convert Matrix to Custom Tuple Matrix
- Python - Matrix Row subset
- Python - Group similar elements into Matrix
- Python - Row-wise element Addition in Tuple Matrix
- Create an n x n square matrix, where all the sub-matrix have the sum of opposite corner elements as even
Python Functions Exercises
- Python splitfields() Method
- How to get list of parameters name from a function in Python?
- How to Print Multiple Arguments in Python?
- Python program to find the power of a number using recursion
- Sorting objects of user defined class in Python
- Assign Function to a Variable in Python
- Returning a function from a function - Python
- What are the allowed characters in Python function names?
- Defining a Python function at runtime
- Explicitly define datatype in a Python function
- Functions that accept variable length key value pair as arguments
- How to find the number of arguments in a Python function?
- How to check if a Python variable exists?
- Python - Get Function Signature
- Python program to convert any base to decimal by using int() method
Python Lambda Exercises
- Python - Lambda Function to Check if value is in a List
- Difference between Normal def defined function and Lambda
- Python: Iterating With Python Lambda
- How to use if, else & elif in Python Lambda Functions
- Python - Lambda function to find the smaller value between two elements
- Lambda with if but without else in Python
- Python Lambda with underscore as an argument
- Difference between List comprehension and Lambda in Python
- Nested Lambda Function in Python
- Python lambda
- Python | Sorting string using order defined by another string
- Python | Find fibonacci series upto n using lambda
- Overuse of lambda expressions in Python
- Python program to count Even and Odd numbers in a List
- Intersection of two arrays in Python ( Lambda expression and filter function )
Python Pattern printing Exercises
- Simple Diamond Pattern in Python
- Python - Print Heart Pattern
- Python program to display half diamond pattern of numbers with star border
- Python program to print Pascal's Triangle
- Python program to print the Inverted heart pattern
- Python Program to print hollow half diamond hash pattern
- Program to Print K using Alphabets
- Program to print half Diamond star pattern
- Program to print window pattern
- Python Program to print a number diamond of any given size N in Rangoli Style
- Python program to right rotate n-numbers by 1
- Python Program to print digit pattern
- Print with your own font using Python !!
- Python | Print an Inverted Star Pattern
- Program to print the diamond shape
Python DateTime Exercises
- Python - Iterating through a range of dates
- How to add time onto a DateTime object in Python
- How to add timestamp to excel file in Python
- Convert string to datetime in Python with timezone
- Isoformat to datetime - Python
- Python datetime to integer timestamp
- How to convert a Python datetime.datetime to excel serial date number
- How to create filename containing date or time in Python
- Convert "unknown format" strings to datetime objects in Python
- Extract time from datetime in Python
- Convert Python datetime to epoch
- Python program to convert unix timestamp string to readable date
- Python - Group dates in K ranges
- Python - Divide date range to N equal duration
- Python - Last business day of every month in year
Python OOPS Exercises
- Get index in the list of objects by attribute in Python
- Python program to build flashcard using class in Python
- How to count number of instances of a class in Python?
- Shuffle a deck of card with OOPS in Python
- What is a clean and Pythonic way to have multiple constructors in Python?
- How to Change a Dictionary Into a Class?
- How to create an empty class in Python?
- Student management system in Python
- How to create a list of object in Python class
Python Regex Exercises
- Validate an IP address using Python without using RegEx
- Python program to find the type of IP Address using Regex
- Converting a 10 digit phone number to US format using Regex in Python
- Python program to find Indices of Overlapping Substrings
- Python program to extract Strings between HTML Tags
- Python - Check if String Contain Only Defined Characters using Regex
- How to extract date from Excel file using Pandas?
- Python program to find files having a particular extension using RegEx
- How to check if a string starts with a substring using regex in Python?
- How to Remove repetitive characters from words of the given Pandas DataFrame using Regex?
- Extract punctuation from the specified column of Dataframe using Regex
- Extract IP address from file using Python
- Python program to Count Uppercase, Lowercase, special character and numeric values using Regex
- Categorize Password as Strong or Weak using Regex in Python
- Python - Substituting patterns in text using regex
Python LinkedList Exercises
- Python program to Search an Element in a Circular Linked List
- Implementation of XOR Linked List in Python
- Pretty print Linked List in Python
- Python Library for Linked List
- Python | Stack using Doubly Linked List
- Python | Queue using Doubly Linked List
- Program to reverse a linked list using Stack
- Python program to find middle of a linked list using one traversal
- Python Program to Reverse a linked list
Python Searching Exercises
- Binary Search (bisect) in Python
- Python Program for Linear Search
- Python Program for Anagram Substring Search (Or Search for all permutations)
- Python Program for Binary Search (Recursive and Iterative)
- Python Program for Rabin-Karp Algorithm for Pattern Searching
- Python Program for KMP Algorithm for Pattern Searching
Python Sorting Exercises
- Python Code for time Complexity plot of Heap Sort
- Python Program for Stooge Sort
- Python Program for Recursive Insertion Sort
- Python Program for Cycle Sort
- Bisect Algorithm Functions in Python
- Python Program for BogoSort or Permutation Sort
- Python Program for Odd-Even Sort / Brick Sort
- Python Program for Gnome Sort
- Python Program for Cocktail Sort
- Python Program for Bitonic Sort
- Python Program for Pigeonhole Sort
- Python Program for Comb Sort
- Python Program for Iterative Merge Sort
- Python Program for Binary Insertion Sort
- Python Program for ShellSort
Python DSA Exercises
- Saving a Networkx graph in GEXF format and visualize using Gephi
- Dumping queue into list or array in Python
- Python program to reverse a stack
- Python - Stack and StackSwitcher in GTK+ 3
- Multithreaded Priority Queue in Python
- Python Program to Reverse the Content of a File using Stack
- Priority Queue using Queue and Heapdict module in Python
- Box Blur Algorithm - With Python implementation
- Python program to reverse the content of a file and store it in another file
- Check whether the given string is Palindrome using Stack
- Take input from user and store in .txt file in Python
- Change case of all characters in a .txt file using Python
- Finding Duplicate Files with Python
Python File Handling Exercises
- Python Program to Count Words in Text File
- Python Program to Delete Specific Line from File
- Python Program to Replace Specific Line in File
- Python Program to Print Lines Containing Given String in File
- Python - Loop through files of certain extensions
- Compare two Files line by line in Python
- How to keep old content when Writing to Files in Python?
- How to get size of folder using Python?
- How to read multiple text files from folder in Python?
- Read a CSV into list of lists in Python
- Python - Write dictionary of list to CSV
- Convert nested JSON to CSV in Python
- How to add timestamp to CSV file in Python
Python CSV Exercises
- How to create multiple CSV files from existing CSV file using Pandas ?
- How to read all CSV files in a folder in Pandas?
- How to Sort CSV by multiple columns in Python ?
- Working with large CSV files in Python
- How to convert CSV File to PDF File using Python?
- Visualize data from CSV file in Python
- Python - Read CSV Columns Into List
- Sorting a CSV object by dates in Python
- Python program to extract a single value from JSON response
- Convert class object to JSON in Python
- Convert multiple JSON files to CSV Python
- Convert JSON data Into a Custom Python Object
- Convert CSV to JSON using Python
Python JSON Exercises
- Flattening JSON objects in Python
- Saving Text, JSON, and CSV to a File in Python
- Convert Text file to JSON in Python
- Convert JSON to CSV in Python
- Convert JSON to dictionary in Python
- Python Program to Get the File Name From the File Path
- How to get file creation and modification date or time in Python?
- Menu driven Python program to execute Linux commands
- Menu Driven Python program for opening the required software Application
- Open computer drives like C, D or E using Python
Python OS Module Exercises
- Rename a folder of images using Tkinter
- Kill a Process by name using Python
- Finding the largest file in a directory using Python
- Python - Get list of running processes
- Python - Get file id of windows file
- Python - Get number of characters, words, spaces and lines in a file
- Change current working directory with Python
- How to move Files and Directories in Python
- How to get a new API response in a Tkinter textbox?
- Build GUI Application for Guess Indian State using Tkinter Python
- How to stop copy, paste, and backspace in text widget in tkinter?
- How to temporarily remove a Tkinter widget without using just .place?
- How to open a website in a Tkinter window?
Python Tkinter Exercises
- Create Address Book in Python - Using Tkinter
- Changing the colour of Tkinter Menu Bar
- How to check which Button was clicked in Tkinter ?
- How to add a border color to a button in Tkinter?
- How to Change Tkinter LableFrame Border Color?
- Looping through buttons in Tkinter
- Visualizing Quick Sort using Tkinter in Python
- How to Add padding to a tkinter widget only on one side ?
- Python NumPy - Practice Exercises, Questions, and Solutions
- Pandas Exercises and Programs
- How to get the Daily News using Python
- How to Build Web scraping bot in Python
- Scrape LinkedIn Using Selenium And Beautiful Soup in Python
- Scraping Reddit with Python and BeautifulSoup
- Scraping Indeed Job Data Using Python
Python Web Scraping Exercises
- How to Scrape all PDF files in a Website?
- How to Scrape Multiple Pages of a Website Using Python?
- Quote Guessing Game using Web Scraping in Python
- How to extract youtube data in Python?
- How to Download All Images from a Web Page in Python?
- Test the given page is found or not on the server Using Python
- How to Extract Wikipedia Data in Python?
- How to extract paragraph from a website and save it as a text file?
- Automate Youtube with Python
- Controlling the Web Browser with Python
- How to Build a Simple Auto-Login Bot with Python
- Download Google Image Using Python and Selenium
- How To Automate Google Chrome Using Foxtrot and Python
Python Selenium Exercises
- How to scroll down followers popup in Instagram ?
- How to switch to new window in Selenium for Python?
- Python Selenium - Find element by text
- How to scrape multiple pages using Selenium in Python?
- Python Selenium - Find Button by text
- Web Scraping Tables with Selenium and Python
- Selenium - Search for text on page
- Python Projects - Beginner to Advanced
Python Exercise: Practice makes you perfect in everything. This proverb always proves itself correct. Just like this, if you are a Python learner, then regular practice of Python exercises makes you more confident and sharpens your skills. So, to test your skills, go through these Python exercises with solutions.
Python is a widely used general-purpose high-level language that can be used for many purposes like creating GUI, web Scraping, web development, etc. You might have seen various Python tutorials that explain the concepts in detail but that might not be enough to get hold of this language. The best way to learn is by practising it more and more.
The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains. So if you are at any stage like beginner, intermediate or advanced this Python practice set will help you to boost your programming skills in Python.
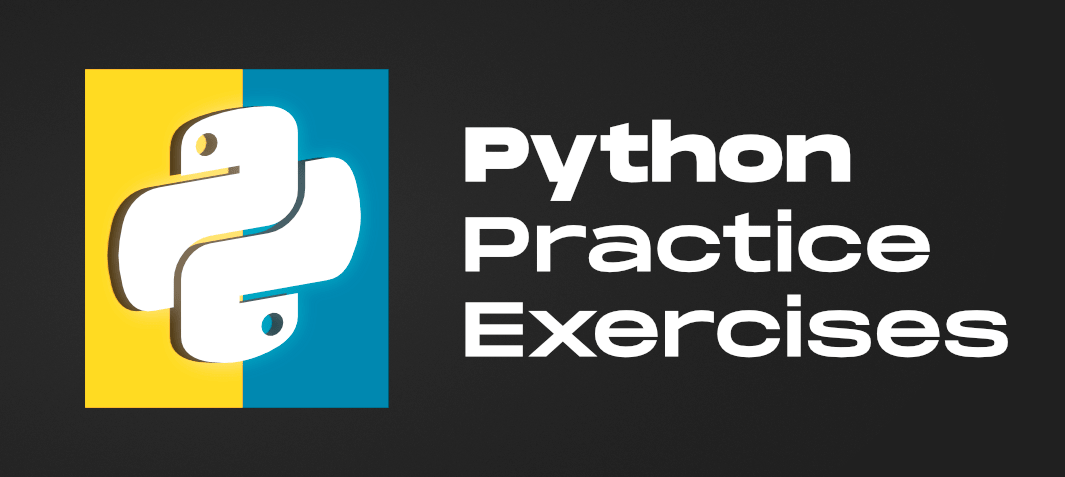
List of Python Programming Exercises
In the below section, we have gathered chapter-wise Python exercises with solutions. So, scroll down to the relevant topics and try to solve the Python program practice set.
Python List Exercises
- Python program to interchange first and last elements in a list
- Python program to swap two elements in a list
- Python | Ways to find length of list
- Maximum of two numbers in Python
- Minimum of two numbers in Python
>> More Programs on List
Python String Exercises
- Python program to check whether the string is Symmetrical or Palindrome
- Reverse words in a given String in Python
- Ways to remove i’th character from string in Python
- Find length of a string in python (4 ways)
- Python program to print even length words in a string
>> More Programs on String
Python Tuple Exercises
- Python program to Find the size of a Tuple
- Python – Maximum and Minimum K elements in Tuple
- Python – Sum of tuple elements
- Python – Row-wise element Addition in Tuple Matrix
- Create a list of tuples from given list having number and its cube in each tuple
>> More Programs on Tuple
Python Dictionary Exercises
- Python | Sort Python Dictionaries by Key or Value
- Handling missing keys in Python dictionaries
- Python dictionary with keys having multiple inputs
- Python program to find the sum of all items in a dictionary
- Python program to find the size of a Dictionary
>> More Programs on Dictionary
Python Set Exercises
- Find the size of a Set in Python
- Iterate over a set in Python
- Python – Maximum and Minimum in a Set
- Python – Remove items from Set
- Python – Check if two lists have atleast one element common
>> More Programs on Sets
- Python – Assigning Subsequent Rows to Matrix first row elements
- Python – Group similar elements into Matrix
>> More Programs on Matrices
>> More Programs on Functions
- Python | Find the Number Occurring Odd Number of Times using Lambda expression and reduce function
>> More Programs on Lambda
- Programs for printing pyramid patterns in Python
>> More Programs on Python Pattern Printing
- Python program to get Current Time
- Get Yesterday’s date using Python
- Python program to print current year, month and day
- Python – Convert day number to date in particular year
- Get Current Time in different Timezone using Python
>> More Programs on DateTime
>> More Programs on Python OOPS
- Python – Check if String Contain Only Defined Characters using Regex
>> More Programs on Python Regex
>> More Programs on Linked Lists
>> More Programs on Python Searching
- Python Program for Bubble Sort
- Python Program for QuickSort
- Python Program for Insertion Sort
- Python Program for Selection Sort
- Python Program for Heap Sort
>> More Programs on Python Sorting
- Program to Calculate the Edge Cover of a Graph
- Python Program for N Queen Problem
>> More Programs on Python DSA
- Read content from one file and write it into another file
- Write a dictionary to a file in Python
- How to check file size in Python?
- Find the most repeated word in a text file
- How to read specific lines from a File in Python?
>> More Programs on Python File Handling
- Update column value of CSV in Python
- How to add a header to a CSV file in Python?
- Get column names from CSV using Python
- Writing data from a Python List to CSV row-wise
>> More Programs on Python CSV
>> More Programs on Python JSON
- Python Script to change name of a file to its timestamp
>> More Programs on OS Module
- Python | Create a GUI Marksheet using Tkinter
- Python | ToDo GUI Application using Tkinter
- Python | GUI Calendar using Tkinter
- File Explorer in Python using Tkinter
- Visiting Card Scanner GUI Application using Python
>> More Programs on Python Tkinter
NumPy Exercises
- How to create an empty and a full NumPy array?
- Create a Numpy array filled with all zeros
- Create a Numpy array filled with all ones
- Replace NumPy array elements that doesn’t satisfy the given condition
- Get the maximum value from given matrix
>> More Programs on NumPy
Pandas Exercises
- Make a Pandas DataFrame with two-dimensional list | Python
- How to iterate over rows in Pandas Dataframe
- Create a pandas column using for loop
- Create a Pandas Series from array
- Pandas | Basic of Time Series Manipulation
>> More Programs on Python Pandas
>> More Programs on Web Scraping
- Download File in Selenium Using Python
- Bulk Posting on Facebook Pages using Selenium
- Google Maps Selenium automation using Python
- Count total number of Links In Webpage Using Selenium In Python
- Extract Data From JustDial using Selenium
>> More Programs on Python Selenium
- Number guessing game in Python
- 2048 Game in Python
- Get Live Weather Desktop Notifications Using Python
- 8-bit game using pygame
- Tic Tac Toe GUI In Python using PyGame
>> More Projects in Python
In closing, we just want to say that the practice or solving Python problems always helps to clear your core concepts and programming logic. Hence, we have designed this Python exercises after deep research so that one can easily enhance their skills and logic abilities.
Please Login to comment...
Similar reads.

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Holy Python
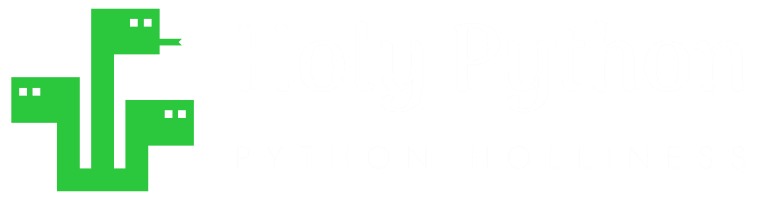
Beginner Python Exercises
Here are some enjoyable Python Exercises for you to solve! We strive to offer a huge selection of Python Exercises so you can internalize the Python Concepts through these exercises.
Among these Python Exercises you can find the most basic Python Concepts about Python Syntax and built-in functions and methods .
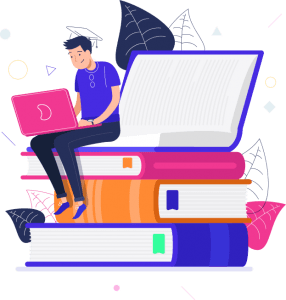
Holy Python is reader-supported. When you buy through links on our site, we may earn an affiliate commission.
Choose the topics you'd like to practice from our extensive exercise list.
Python Beginner Exercises consist of some 125+ exercises that can be solved by beginner coders and newcomers to the Python world.
Majority of these exercises are online and interactive which offers an easier and convenient entry point for beginners.
First batch of the exercises starts with print function as a warm up but quickly, it becomes all about data.
You can start exercising with making variable assignments and move on with the most fundamental data types in Python:
Exercise 1: print() function | (3) : Get your feet wet and have some fun with the universal first step of programming.
Exercise 2: Variables | (2) : Practice assigning data to variables with these Python exercises.
Exercise 3: Data Types | (4) : Integer (int), string (str) and float are the most basic and fundamental building blocks of data in Python.
Exercise 4: Type Conversion | (8) : Practice converting between basic data types of Python when applicable.
Exercise 5: Data Structures | (6) : Next stop is exercises of most commonly used Python Data Structures. Namely;
- Dictionaries and
- Strings are placed under the microscope.
Exercise 6: Lists | (14) : It’s hard to overdo Python list exercises. They are fun and very fundamental so we prepared lots of them. You will also have the opportunity to practice various Python list methods.
Exercise 7: Tuples | (8) : Python Tuples Exercises with basic applications as well as common tuples methods
Exercise 8: Dictionaries | (11) : Practice with Python Dictionaries and some common dictionary methods.
Exercise 9: Strings | (14) : Basic string operations as well as many string methods can be practiced through 10+ Python String Exercises we have prepared for you.
Next batch consist of some builtin Python functions and Python methods. The difference between function and method in Python is that functions are more like standalone blocks of codes that take arguments while methods apply directly on a class of objects.
That’s why we can talk about list methods, string methods, dictionary methods in Python but functions can be used alone as long as appropriate arguments are passed in them.
Exercise 10: len() function | (5) : Python’s len function tells the length of an object. It’s definitely a must know and very useful in endless scenarios. Whether it’s manipulating strings or counting elements in a list, len function is constantly used in computer programming.
Exercise 11: .sort() method | (7) : Practice sort method in Beginner Python Exercises and later on you’ll have the opportunity practice sorted function in Intermediate Python Exercises.
Exercise 12: .pop() method | (3) : A list method pop can be applied to Python list objects. It’s pretty straightforward but actually easy to confuse. These exercises will help you understand pop method better.
Exercise 13: input() function | (6) : Input function is a fun and useful Python function that can be used to acquire input values from the user.
Exercise 14: range() function | (5) : You might want to take a real close look at range function because it can be very useful in numerous scenarios.
These range exercises offer an opportunity to get a good practice and become proficient with the usage of Python’s range function.
Exercise 15: Error Handling | (7) : Error Handling is a must know coding skill and Python has some very explanatory Error Codes that will make a coder’s life easier if he/she knows them!
Exercise 16: Defining Functions | (9) : Practicing user defined Python functions will take your programming skills to the next step. Writing functions are super useful and fun. It makes code reusable too.
Exercise 17: Python Slicing Notation | (8) : Python’s slicing notations are very interesting but can be confusing for beginner coders. These exercises will help you master them.
Exercise 18: Python Operators | (6) : Operators are another fundamental concept. We have prepared multiple exercises so they can be confident in using Python Operators.
If you struggle with any of the exercises, you can always refer to the Beginner Python Lessons .
If you’d like to challenge yourself with the next level of Python exercises check out the Intermediate Python Exercises we have prepared for you.
FREE ONLINE PYTHON COURSES
Choose from over 100+ free online Python courses.
Python Lessons
Beginner lessons.
Simple builtin Python functions and fundamental concepts.
Intermediate Lessons
More builtin Python functions and slightly heavier fundamental coding concepts.
Advanced Lessons
Python concepts that let you apply coding in the real world generally implementing multiple methods.
Python Exercises
Beginner exercises.
Basic Python exercises that are simple and straightforward.
Intermediate Exercises
Slightly more complex Python exercises and more Python functions to practice.
Advanced Exercises
Project-like Python exercises to connect the dots and prepare for real world tasks.
Thank you for checking out our Python programming content. All of the Python lessons and exercises we provide are free of charge. Also, majority of the Python exercises have an online and interactive interface which can be helpful in the early stages of learning computer programming.
However, we do recommend you to have a local Python setup on your computer as soon as possible. Anaconda is a free distribution and it comes as a complete package with lots of libraries and useful software (such as Spyder IDE and Jupyter Notebook) You can check out this: simple Anaconda installation tutorial .

Get started learning Python with DataCamp's free Intro to Python tutorial . Learn Data Science by completing interactive coding challenges and watching videos by expert instructors. Start Now !
Ready to take the test? Head onto LearnX and get your Python Certification!
This site is generously supported by DataCamp . DataCamp offers online interactive Python Tutorials for Data Science. Join 11 millions other learners and get started learning Python for data science today!
Good news! You can save 25% off your Datacamp annual subscription with the code LEARNPYTHON23ALE25 - Click here to redeem your discount!
Welcome to the LearnPython.org interactive Python tutorial.
Whether you are an experienced programmer or not, this website is intended for everyone who wishes to learn the Python programming language.
You are welcome to join our group on Facebook for questions, discussions and updates.
After you complete the tutorials, you can get certified at LearnX and add your certification to your LinkedIn profile.
Just click on the chapter you wish to begin from, and follow the instructions. Good luck!
Learn the Basics
- Hello, World!
- Variables and Types
- Basic Operators
- String Formatting
- Basic String Operations
- Classes and Objects
- Dictionaries
- Modules and Packages
Data Science Tutorials
- Numpy Arrays
- Pandas Basics
Advanced Tutorials
- List Comprehensions
- Lambda functions
- Multiple Function Arguments
- Regular Expressions
- Exception Handling
- Serialization
- Partial functions
- Code Introspection
- Map, Filter, Reduce
Other Python Tutorials
- DataCamp has tons of great interactive Python Tutorials covering data manipulation, data visualization, statistics, machine learning, and more
- Read Python Tutorials and References course from After Hours Programming
Contributing Tutorials
Read more here: Contributing Tutorials
This site is generously supported by DataCamp . DataCamp offers online interactive Python Tutorials for Data Science. Join over a million other learners and get started learning Python for data science today!
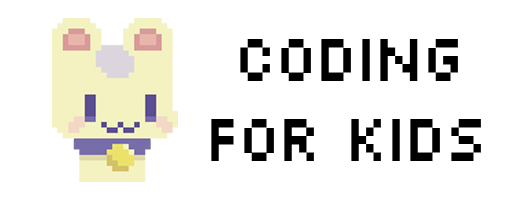
Coding for Kids is an online interactive tutorial that teaches your kids how to code while playing!
Receive a 50% discount code by using the promo code:
Start now and play the first chapter for free, without signing up.
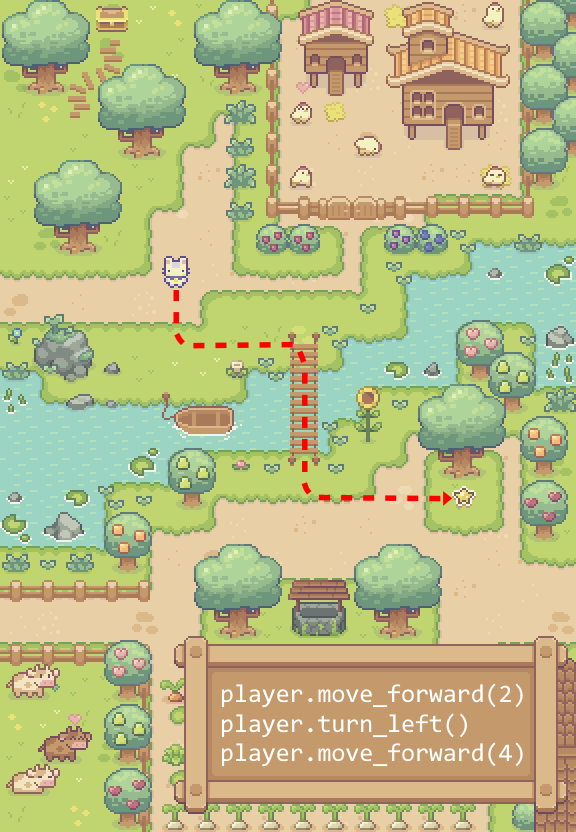
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, learn python programming.
Python is one of the top programming languages in the world, widely used in fields such as data science (AI, machine learning, etc), and web development.
The simple and English-like syntax of Python makes it a go-to language for beginners who want to get into coding quickly.
Because Python is used in multiple fields, there is a high demand for Python developers, with competitive base salaries.
In this guide, we will cover:
Beginner's Guide to Python
Is python for you, best way to learn python, how to run python.
If you are simply looking to learn Python step-by-step, you can follow our free tutorials in the next section.
These tutorials will provide you with a solid foundation in Python and prepare you for your career goals.
How to Get Started With Python?
Your First Python Program
Python Comments
Python Variables, Constants and Literals
Python Type Conversion
Python Basic Input and Output
Python Operators
Python if...else Statement
Python for Loop
Python while Loop
Python break and continue
Python pass Statement
Python Numbers, Type Conversion and Mathematics
Python List
Python Tuple
Python Sets
Python Dictionary
Python Functions
Python Function Arguments
Python Variable Scope
Python Global Keyword
Python Recursion
Python Modules
Python Package
Python Main function
Python Directory and Files Management
Python CSV: Read and Write CSV files
Reading CSV files in Python
Writing CSV files in Python
Python Exceptions
Python Exception Handling
Python Custom Exceptions
Python Objects and Classes
Python Inheritance
Python Multiple Inheritance
Polymorphism in Python
Python Operator Overloading
List comprehension
Python Lambda/Anonymous Function
Python Iterators
Python Generators
Python Namespace and Scope
Python Closures
Python Decorators
Python @property decorator
Python RegEx
Python datetime
Python strftime()
Python strptime()
How to get current date and time in Python?
Python Get Current Time
Python timestamp to datetime and vice-versa
Python time Module
Python sleep()
Precedence and Associativity of Operators in Python
Python Keywords and Identifiers
Python Asserts
Python Json
Python *args and **kwargs
Whether Python is the right choice depends on what you want to accomplish and your career goals.
Python from Learning Perspective
If you are new to programming and prefer simplicity, Python is probably the right choice for you.
Let's see an example.
Here, both programs in C and Python perform the same task. However, the Python code is much easier to understand, even if you have never been a programmer before.
That being said, there are some advantages to learning languages like C as your first language. For example, C is much closer to the hardware and allows you to work with computer memory directly, thus providing you with a deeper understanding of how your code actually works.
On the other hand, Python's clear, English-like syntax allows you to concentrate on problem-solving and building logic without being concerned about unnecessary complexities.
So, it's up to you whether you want to quickly get started with programming or really take your time to understand the nitty-gritty parts of programming.
Python as a Career Choice
Python is a widely used programming language for creating real-world applications. It is extensively used in:
- Data Science
- Artificial Intelligence
- Backend Development
Thus, learning Python offers significant advantages for your career opportunities.
However, there are certain fields where Python doesn't excel. For example, if you are interested in frontend development, game development, or mobile app development, then Python is not the best choice.
In these cases, alternatives such as JavaScript for frontend development, Kotlin, Swift, or Dart for mobile app development, and C++ for game development will be more suitable.
Therefore, your career choices can guide you in selecting which programming language to learn.
There is no right or wrong way to learn Python. It all depends on your learning style and pace.
In this section, we have included the best Python learning resources tailored to your learning preferences, be it text-based, video-based, or interactive courses.
Text-based Tutorial
Best: if you are committed to learning Python but do not want to spend on it
If you want to learn Python for free with a well-organized, step-by-step tutorial, you can use our free Python tutorials .
Our tutorials will guide you through Python one step at a time, using practical examples to strengthen your foundation.
Interactive Course
Best: if you want hands-on learning, get your progress tracked, and maintain a learning streak
Learning to code is tough. It requires dedication and consistency, and you need to write tons of code yourself.
While videos and tutorials provide you with a step-by-step guide, they lack hands-on experience and structure.
Recognizing all these challenges, Programiz offers a premium Learn Python Course that allows you to gain hands-on learning experience by solving challenges, building real-world projects, and tracking your progress.
Online Video
Best: if you are an audio-visual learner and learn by watching others code and following along
If you're more of a visual learner, we have created a comprehensive Python course for beginners that will guide you on your Python journey.
Additionally, there's a popular Python playlist by Corey Schafer available on YouTube to further guide you on your Python journey.
If you're looking for a structured university course at zero cost, visit Python Course - University of Helsinki.
Best: if you are a casual and hobby learner who wants to learn Python on the go.
While it's possible to learn Python from mobile apps, it's not the ideal way because writing code can be challenging. Additionally, it's difficult to build real-world projects with multiple files on mobile devices.
Nevertheless, you can use these apps to try things out.
- Learn Python
Important: You cannot learn to code without developing the habit of writing code yourself. Therefore, whatever method you choose, always write code.
While writing code, you will encounter errors. Don't worry about them, try to understand them and find solutions. Remember, programming is all about solving problems, and errors are part of the process.
1. Run Python in your browser.
We have created an online editor to run Python directly in your browser. You don't have to go through a tedious installation process. It's completely free, and you can start coding directly.
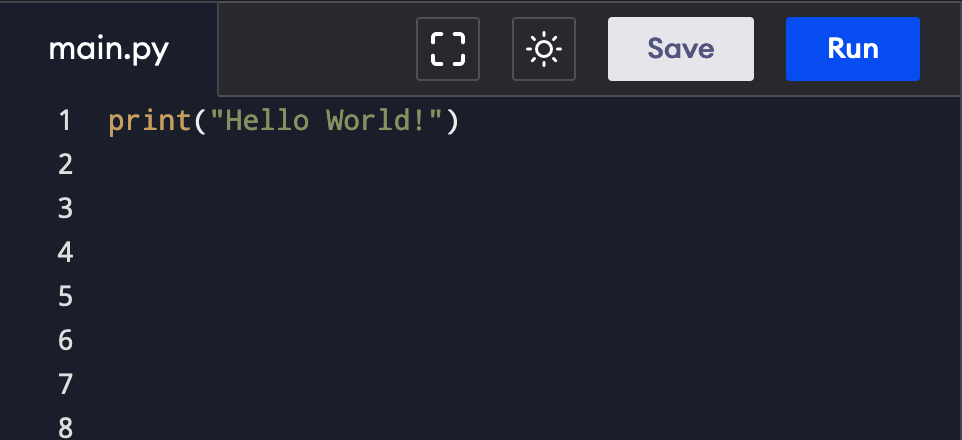
2. Install Python on Your computer.
Once you start writing complex programs and creating projects, you should definitely install Python on your computer. This is especially necessary when you are working with projects that involve multiple files and folders.
To install Python on your device, you can use this guide.
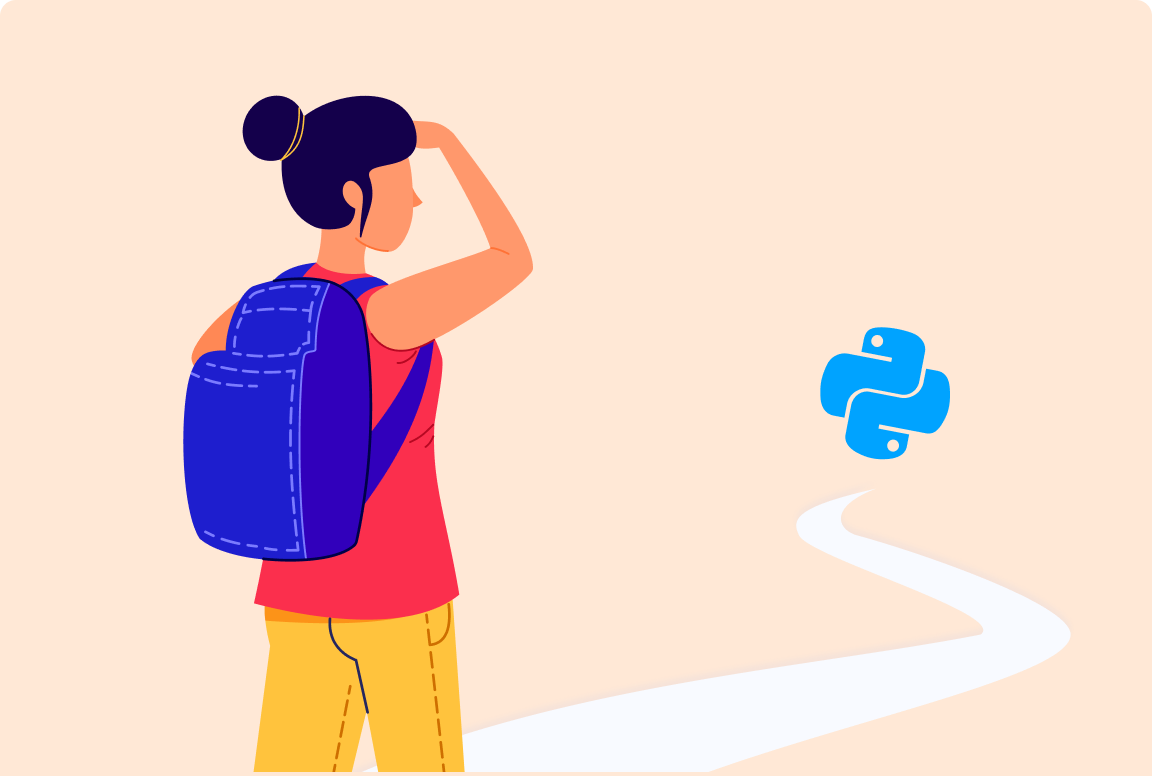
Getting Started with Python
Learn how you can install and use Python on your own computer.
Say "Hello, World!" With Python Easy Max Score: 5 Success Rate: 96.25%
Python if-else easy python (basic) max score: 10 success rate: 89.71%, arithmetic operators easy python (basic) max score: 10 success rate: 97.42%, python: division easy python (basic) max score: 10 success rate: 98.68%, loops easy python (basic) max score: 10 success rate: 98.11%, write a function medium python (basic) max score: 10 success rate: 90.31%, print function easy python (basic) max score: 20 success rate: 97.27%, list comprehensions easy python (basic) max score: 10 success rate: 97.69%, find the runner-up score easy python (basic) max score: 10 success rate: 94.16%, nested lists easy python (basic) max score: 10 success rate: 91.67%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
25 Python Projects for Beginners – Easy Ideas to Get Started Coding Python
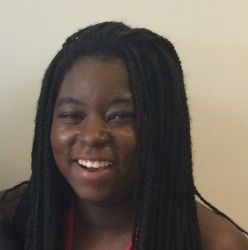
The best way to learn a new programming language is to build projects with it.
I have created a list of 25 beginner friendly project tutorials in Python.
My advice for tutorials would be to watch the video, build the project, break it apart and rebuild it your own way. Experiment with adding new features or using different methods.
That will test if you have really learned the concepts or not.
You can click on any of the projects listed below to jump to that section of the article.
If you are not familiar with the basics of Python, then I would suggest watching this beginner freeCodeCamp Python tutorial .
Python Projects You Can Build
- Guess the Number Game (computer)
- Guess the Number Game (user)
- Rock, paper, scissors
- Countdown Timer
- Password Generator
- QR code encoder / decoder
- Tic-Tac-Toe
- Tic-Tac-Toe AI
- Binary Search
- Minesweeper
- Sudoku Solver
- Photo manipulation in Python
- Markov Chain Text Composer
- Connect Four
- Online Multiplayer Game
- Web Scraping Program
- Bulk file renamer
- Weather Program
Code a Discord Bot with Python - Host for Free in the Cloud
- Space invaders game
Mad libs Python Project
In this Kylie Ying tutorial, you will learn how to get input from the user, work with f-strings, and see your results printed to the console.
This is a great starter project to get comfortable doing string concatenation in Python.
Guess the Number Game Python Project (computer)
In this Kylie Ying tutorial, you will learn how to work with Python's random module , build functions, work with while loops and conditionals, and get user input.
Guess the Number Game Python Project (user)
In this Kylie Ying tutorial, you will build a guessing game where the computer has to guess the correct number. You will work with Python's random module , build functions, work with while loops and conditionals, and get user input.
Rock, paper, scissors Python Project
In this Kylie Ying tutorial , you will work with random.choice() , if statements, and getting user input. This is a great project to help you build on the fundamentals like conditionals and functions.
Hangman Python Project
In this Kylie Ying tutorial, you will learn how to work with dictionaries, lists, and nested if statements. You will also learn how to work with the string and random Python modules.
Countdown Timer Python Project
In this Code With Tomi tutorial , you will learn how to build a countdown timer using the time Python module. This is a great beginner project to get you used to working with while loops in Python.
Password Generator Python Project
In this Code With Tomi tutorial , you will learn how to build a random password generator. You will collect data from the user on the number of passwords and their lengths and output a collection of passwords with random characters.
This project will give you more practice working with for loops and the random Python module.
QR code encoder / decoder Python Project
In this Code With Tomi tutorial , you will learn how to create your own QR codes and encode/decode information from them. This project uses the qrcode library.
This is a great project for beginners to get comfortable working with and installing different Python modules.
Tic-Tac-Toe Python Project
In this Kylie Ying tutorial, you will learn how to build a tic-tac-toe game with various players in the command line. You will learn how to work with Python's time and math modules as well as get continual practice with nested if statements.
Tic-Tac-Toe AI Python Project
In this Kylie Ying tutorial, you will learn how to build a tic-tac-toe game where the computer never loses. This project utilizes the minimax algorithm which is a recursive algorithm used for decision making.
Binary Search Python Project
In this Kylie Ying tutorial, you will learn how to implement the divide and conquer algorithm called binary search. This is a common searching algorithm which comes up in job interviews, which is why it is important to know how to implement it in code.
Minesweeper Python Project
In this Kylie Ying tutorial, you will build the classic minesweeper game in the command line. This project focuses on recursion and classes.
Sudoku Solver Python Project
In this Kylie Ying tutorial, you will learn how to build a sudoku solver which utilizes the backtracking technique. Backtracking is a recursive technique that searches for every possible combination to help solve the problem.
Photo Manipulation in Python Project
In this Kylie Ying tutorial, you will learn how to create an image filter and change the contrast, brightness, and blur of images. Before starting the project, you will need to download the starter files .
Markov Chain Text Composer Python Project
In this Kylie Ying tutorial, you will learn about the Markov chain graph model and how it can be applied the relationship of song lyrics. This project is a great introduction into artificial intelligence in Python.
Pong Python Project
In this Christian Thompson tutorial , you will learn how to recreate the classic pong game in Python. You will be working with the os and turtle Python modules which are great for creating graphics for games.
Snake Python Project
In this Tech with Tim tutorial, you will learn how to recreate the classic snake game in Python. This project uses Object-oriented programming and Pygame which is a popular Python module for creating games.
Connect Four Python Project
In this Keith Galli tutorial, you will learn how to build the classic connect four game. This project utilizes the numpy , math , pygame and sys Python modules.
This project is great if you have already built some smaller beginner Python projects. But if you haven't built any Python projects, then I would highly suggest starting with one of the earlier projects on the list and working your way up to this one.
Tetris Python Project
In this Tech with Tim tutorial, you will learn how to recreate the classic Tetris game. This project utilizes Pygame and is great for beginner developers to take their skills to the next level.
Online Multiplayer Game Python Project
In this Tech with Tim tutorial, you will learn how to build an online multiplayer game where you can play with anyone around the world. This project is a great introduction to working with sockets, networking, and Pygame.
Web Scraping Program Python Project
In this Code With Tomi tutorial , you will learn how to ask for user input for a GitHub user link and output the profile image link through web scraping. Web scraping is a technique that collects data from a web page.
Bulk File Re-namer Python Project
In this Code With Tomi tutorial , you will learn how to build a program that can go into any folder on your computer and rename all of the files based on the conditions set in your Python code.
Weather Program Python Project
In this Code With Tomi tutorial , you will learn how to build a program that collects user data on a specific location and outputs the weather details of that provided location. This is a great project to start learning how to get data from API's.
In this Beau Carnes tutorial , you will learn how to build your own bot that works in Discord which is a platform where people can come together and chat online. This project will teach you how to work with the Discord API and Replit IDE.
After this video was released, Replit changed how you can store your environments variables in your program. Please read through this tutorial on how to properly store environment variables in Replit.
Space Invaders Game Python Project
In this buildwithpython tutorial , you will learn how to build a space invaders game using Pygame. You will learn a lot of basics in game development like game loops, collision detection, key press events, and more.
I am a musician and a programmer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, learn python.
Python is a popular programming language.
Python can be used on a server to create web applications.
Learning by Examples
With our "Try it Yourself" editor, you can edit Python code and view the result.
Click on the "Try it Yourself" button to see how it works.
Python File Handling
In our File Handling section you will learn how to open, read, write, and delete files.
Python Database Handling
In our database section you will learn how to access and work with MySQL and MongoDB databases:
Python MySQL Tutorial
Python MongoDB Tutorial
Python Exercises
Test yourself with exercises.
Insert the missing part of the code below to output "Hello World".
Start the Exercise
Advertisement
Learn by examples! This tutorial supplements all explanations with clarifying examples.
See All Python Examples
Python Quiz
Test your Python skills with a quiz.
My Learning
Track your progress with the free "My Learning" program here at W3Schools.
Log in to your account, and start earning points!
This is an optional feature. You can study at W3Schools without using My Learning.

You will also find complete function and method references:
Reference Overview
Built-in Functions
String Methods
List/Array Methods
Dictionary Methods
Tuple Methods
Set Methods
File Methods
Python Keywords
Python Exceptions
Python Glossary
Random Module
Requests Module
Math Module
CMath Module
Download Python
Download Python from the official Python web site: https://python.org
Python Exam - Get Your Diploma!
Kickstart your career.
Get certified by completing the course

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
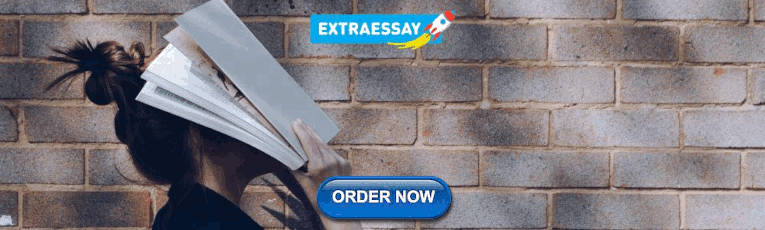
Top Tutorials
Top references, top examples, get certified.
Best Python Homework Help Websites (Reviewed by Experts)

Many teens are fond of technology in all its manifestation. As they grow up, their interest becomes rather professional, and many of them decide to become certified coders to create all those games, software, apps, websites, and so on. This is a very prospective area, which will always be in high demand. Yet, the path to the desired diploma is pretty complicated. Not all assignments can be handled properly. As a result, many learners look for professional help on the Internet.
Smart minds are aware of custom programming platforms that help with all kinds of programming languages. It’s hard to define which one is better than the others because all legal and highly reputed platforms offer pretty much the same guarantees and conveniences of high quality. This is when our comprehensive review will be helpful for students. We have checked the best Python homework help websites. We have opted for Python because of 2 reasons. Firstly, this is one of the most popular and widely used coding languages. You will surely have to master it. Secondly, only one language helps to narrow down your choice. So, read on to find out the possible options that really work.
6 Top Python Assignment Help Websites to Solve All Your Issues
It is good to have a rich choice of coding options. But, the abundance of choices can also be overwhelming. Here are some top recommendations to make the choice easier:
These are the top Python assignment help websites, according to the research of our quality control experts. Let’s look at each of them individually, focusing on a unique benefit offered by each site. This data may help you to define the type of aid you need – high quality, top speed, cheap prices, and so on.
Python Programming Homework Help – How to Choose the Right Site?
When it comes to choosing a custom coding site, you may be puzzled for a long time. There are many great options, and each seems to offer flawless Python programming assignment help. How to define the best platform in the niche? Well, the first step is to create a wish list. It should include the features you expect to get from a pro platform.
Secondly, use the comparison method. You need to shortlist all the options and check what exactly each of them offers. While one offers cheaper prices, another one is faster. So, the choice should be based on your priorities. We have already created a list of the most beneficial sites for you. The last task is to compare them after you read more detailed descriptions.
We’ve shortlisted the most beneficial sites for you. What you’ll be left with is to compare them and pick the one that suits your needs best.
1. CodingHomeworkHelp.org: A Top-Rated Solution for Python Homework
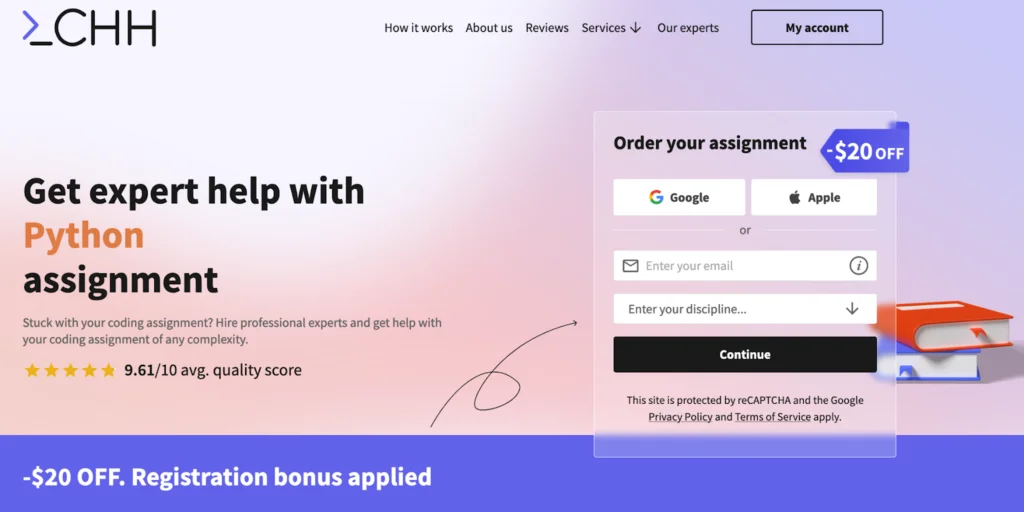
Our first option is called CodingHomeworkHelp , and it has the highest average rating, according to our experts. They’ve given it 9.8 out of 10 possible, which is surely a great achievement. The combination of conditions and guarantees makes it nearly ideal for students needing Python homework help. Let’s take a look at its main features:
- Outstanding quality. This custom coding agency is famous for the quality of its aid, which is always as high as the client desires. It employs only certified and skilled solvers who can meet the demands of the strictest educators and employers (if you already work).
- Timely aid . This platform has delivered almost 97% of all its orders on time. This indicator proves that you may not worry about your time limits. Its specialists are fast enough to meet the shortest timeframes.
- Unique projects . Its experts do all the orders from scratch. It means they never reuse even their own projects. They take into account the slightest details and make every project unique. Your code will surely differ from others, and it will be free of any signs of plagiarism. Don’t worry about this matter.
- Quite cheap prices . You will be pleasantly impressed by the price policy offered by this coding agency. It is quite cheap and fair. Ordinary students will be able to afford its professional aid. Moreover, they can count on great discounts and promos to save up even more of their funds.
- Effective customer support . This site offers a very welcoming and fast team of customer support, which consists of great consultants. They are always at work and provide clear answers to the questions related to the policies of this agency. The answers come in a couple of minutes or so.
2. DoMyAssignments.com: Affordable Python Assignment Assistance

The second site we’d like to recommend is DoMyAssignments , boasting a strong rating of 9.7 out of 10 stars. This impressive score reflects the site’s commitment to excellence, and you can be confident that it can satisfy all your coding needs to the fullest. With a team of real professionals, each selected through a special onboarding process that consists of several stages, only the most gifted specialists make the cut.
How can they assist with your Python assignment? They offer individualized solutions at the cheapest prices among our reviewed sites. You can even modify the order to fit your budget, considering factors like quality, type, size, and urgency.
Besides, the site ensures other vital benefits. Make allowances for them here below:
- Quick assistance : The experts at DoMyAssignments are known for their speed and diligence. An impressive 96% of all their orders were delivered without delays, and 79% were completed long before the deadline . These achievements demonstrate their commitment to timely delivery, even for the most urgent tasks.
- Full privacy : Security is a priority at DoMyAssignments. They ensure the confidentiality of your private data and never share it with third parties. With effective antivirus software and encrypted billing methods, you can trust that you’re 100% safe when using their platform.
- 24/7 support : Need help at any hour? DoMyAssignments runs 24/7, providing immediate access to competent technicians via live chat. Whether you have urgent questions about their policies or need clarification on specific details, you can expect fast and clear answers.
- Individual approach : Personalized service is a standout feature of DoMyAssignments. You can contact your helper at predetermined hours to discuss your project’s progress. This direct communication allows for real-time updates and changes, offering a convenient way to ensure that your project aligns perfectly with your requirements.
You should also know that it practices an individual approach. You are welcome to contact your helper during the predetermined hours. Just discuss with him or her when both of you can be online and check the progress of your project. It’s a fast and convenient way to offer changes on demand and without delays.
3. AssignCode.com: Fast and Reliable Python Homework Help

If speed is your priority, AssignCode is an excellent choice. How fast can you do my Python homework? Well, a lot depends on the demands you have. Nonetheless, most projects are completed there in 4–5 hours only!
Thus, you can place an order even later at night to get it done early in the morning. Just be sure you set manageable conditions. If it’s so, your order will be accepted and completed according to your demands. It will be delivered on time. As for other vital guarantees, you may count on:
- Great quality. This company has a professional staff, which consists of outstanding programmers. They all have confirmed their qualifications and were trained to match the top demands of every high school, college, or university. They can help even already working coders who face some issues at the moment.
- Fair prices. You will surely like the prices set by this coding company. They are quite cheap, and ordinary students will not face problems with ordering professional help on this site. There is a possibility to quickly regulate the prices online. You only need to change the quality, type, volume, or deadline of your assignment. A refund guarantee is given as well.
- All kinds of features . This platform is able to satisfy the slightest demands of the strictest customers. Everything will be done exactly as you want, and can contact your helper directly. He or she will offer all the necessary skills to complete your project perfectly. The platform’s specialists handle all assignment types. Python is only one of the possible areas of their competence.
- A responsive customer support team. In case you don’t understand some policies or limits of this company, turn to its team of support. It consists of polite and knowledgeable operators. They work day and night to provide detailed responses in about 2 minutes or so.
Also read: The 10 Commandments of Coding: Study, Learn and Put into Practice
4. CWAssignments.com: Unique and Customized Python Assistance
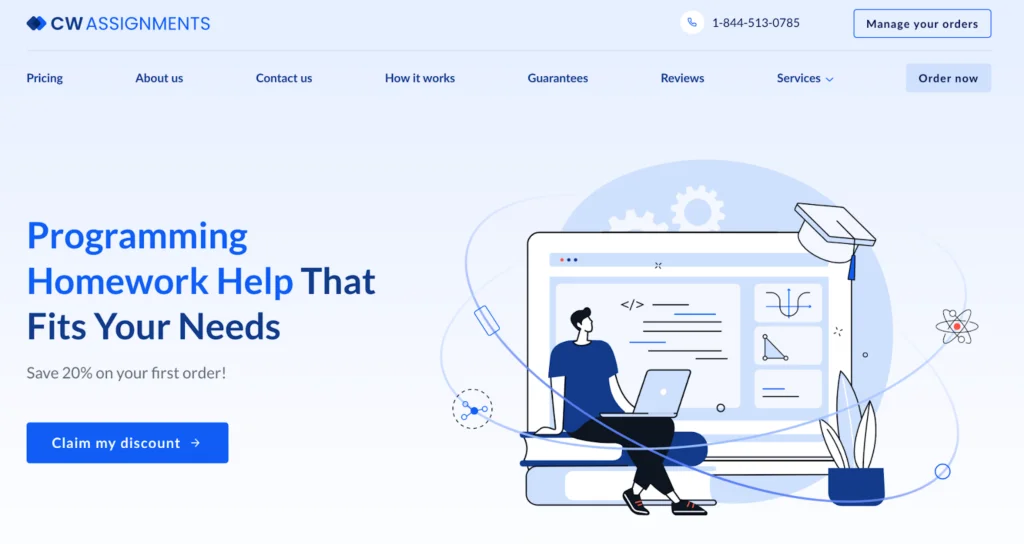
Many students cannot create unique projects in coding, and that is why they may require the unique Python assignment help of CWAssignments . Its rating is 9.4 out of 10, which is a sign of a top-class coding site.
It does all the projects anew and never uses the projects of other coders. Its experts don’t reuse even their old assignments. The new conditions are taken into account and fulfilled uniquely. It also offers other vital benefits. These are as follows:
- Reasonable pricing . You will not spend too much if you request assistance there. The site sets relatively cheap prices and offers full customization of the orders. This puts you in full charge of the total cost. Fill out the compulsory fields and change them to see how you can impact the cost to stop when it suits your budget.
- Top quality. The agency hires only educated and talented coders. They surely understand how to handle any assignment in computer science, engineering, and math. They stick to the official requirements of all educational institutions and can satisfy even the most scrupulous educators. Thus, your chance to get an A+ grade sufficiently increases.
- A personified approach. You may get in touch with your solver whenever his or her aid may be required. Just set a reasonable schedule when both of you can be online to discuss the peculiarities of your order. The specialists will provide updated reports to let you know where your project stands.
- Total online confidentiality . This is a reliable and respectful coding platform that always protects the private data of its clients. It never shares any facts about them with anyone else. It utilizes effective software that protects its databases from all types of online dangers. Thanks to the billing methods offered by the site, you may not worry about your transactions within it. They are encrypted and hidden from other users.
5. HelpHomework.net: Personalized Approach to Python Coding
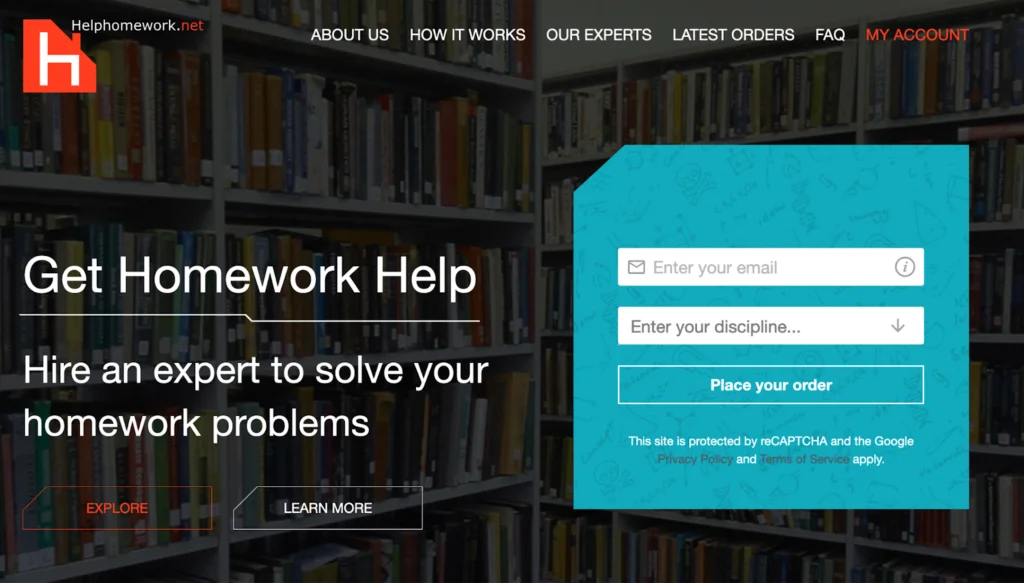
Many learners seek personalized attention for their coding projects. If you need a tailored approach to Python homework help, HelpHomework is the place to go. It offers flexible scheduling for real-time collaboration with your solver.
Simply coordinate a schedule to be online with your solver, using your preferred instant messenger for quick updates. Along with this personalized approach, the platform also provides other key guarantees, including:
- Outstanding Quality: This platform hires only certified coders who pass a rigorous selection process. They’re trained to handle any assignments in computer science, engineering, and math, ensuring precise completion to boost your success.
- Plagiarism-Free Projects: The platform ensures uniqueness in every project. Though coding may seem repetitive, the specialists craft each project from scratch, meeting educators’ expectations for originality.
- 24/7 Support and Supervision: Visit this site anytime, day or night. It operates around the clock, with kind operators ready to provide swift, detailed responses in live chat.
- Reasonable Prices: Offering affordable rates to fit students’ budgets, the company allows you to customize your order’s price by adjusting the project’s quality, size, type, and deadline.
6. CodingAssignments.com: A Wide Range of Python Experts at Your Service
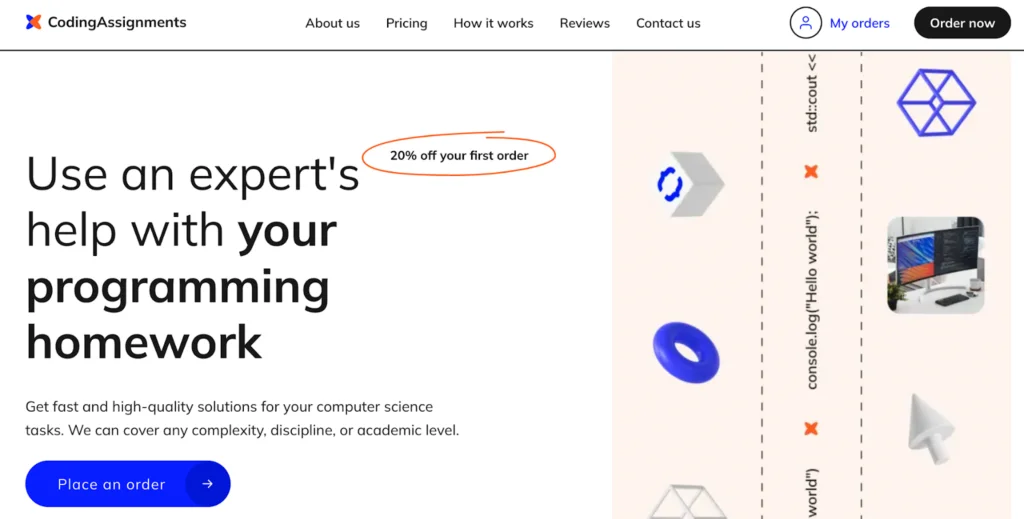
A rich choice of specialists is significant to all. If you want to be sure that you will always find the kind of help with Python assignments or other programming languages, you should opt for CodingAssignments .
This highly reputed coding company boasts over 700 specialists. Thus, you will never be deprived of some privileges. You will find perfect solvers for whatever coding project you must do. The company likewise provides the next benefits:
- On-time deliveries. The experts of the company value the precious time of their customers. They polish all the necessary skills and master the most effective time management methods to meet really short deadlines. Just provide manageable terms. If the assignment is too large and urgent, place it as early as you can. The specialists will check the odds and will surely accept it if it can be completed within the stated period of time.
- Fair pricing . You can count on relatively cheap prices when you deal with this programming site. Thus, common students will be able to afford its aid. Besides, you can count on pleasant promo codes and discounts to save up even more of your funds. Thanks to the refund guarantee, all your investments are secured.
- Full online anonymity. Don’t worry about your online safety when you visit this site. It guards its databases and your private information with reliable antivirus software. The site never reveals any details about its customers to anybody else.
- Hourly supervision . This coding platform operates 24 hours round the clock to let its customers place urgent orders even late at night. Find the chat window and specify the problem you’re facing. There are always operators at work. They provide detailed answers in a couple of minutes or faster.
Also check: Python Course for Beginners Online FREE
FAQs About The Best Python Homework Help Websites
Let’s answer some of the commonly asked questions around our topic of discussion today.
Can I pay someone to do my Python assignment?
Using a legal online coding site requires payment, so choose wisely as different sites set different prices. While 2 sites offer the same level of quality, it would not be wise to choose the one with a more expensive price policy. You’d better study this case before you place the first order.
How can I pay someone to do my Python homework?
To pay for Python homework, register on the site and add a billing method such as PayPal, Visa, or Pioneer. The methods are very convenient and safe. Make sure your debit or credit card has enough money. When you place an order, you will pay the price automatically. The money will be in escrow until the job is done. Check its quality, and if it suits you, release the final payment to your solver.
How can I receive assistance with Python projects?
You can receive professional coding assistance by finding the right coding platforms and hiring the most suitable Python experts. Conduct thorough research to identify the most reliable and suitable sites.
One of the components of your research is surely reading reviews similar to ours. It helps to narrow down the list of potential helping platforms.
Once you’re on the site, check its top performers. Although their prices are higher, you will be safe about the success of your project. Yet, other experts with low ratings can suit you as well. Just check their detailed profiles and read reviews of other clients to be sure they can satisfy all your needs. Hire the required solver, explain what must be done, and pay to get it started.
Where can I get help with Python programming?
You can find Python programming homework help on the Internet. Open the browser and write an accurate keyword search combination.
It may be something like this – the swiftest or cheapest, or best coding site. Check the results, read customers’ reviews, check the reviews of rating agencies (like ours), compare the conditions, and select the most beneficial option for you.
What kind of guarantees can I expect from Python help services?
If you want to find help with Python projects and you will be treated fairly, you need to know the main guarantees every highly reputed programming site is supposed to ensure. These are as follows:
- High quality
- Availability of all skills and assignments
- An individual approach
- Full privacy of your data
- Timely deliveries
- 100% authentic projects
- 24/7 access and support
- Refunds and free revisions
These are the essential guarantees that every legitimate coding site must provide. If some of them lack, it may be better to switch to another option. These guarantees are compulsory, and you should enjoy them all automatically.
Notice: While JavaScript is not essential for this website, your interaction with the content will be limited. Please turn JavaScript on for the full experience.
Notice: Your browser is ancient . Please upgrade to a different browser to experience a better web.
- Chat on IRC
- Python >>>
- About >>>
- Getting Started
Python For Beginners
Welcome! Are you completely new to programming ? If not then we presume you will be looking for information about why and how to get started with Python. Fortunately an experienced programmer in any programming language (whatever it may be) can pick up Python very quickly. It's also easy for beginners to use and learn, so jump in !
Installing Python is generally easy, and nowadays many Linux and UNIX distributions include a recent Python. Even some Windows computers (notably those from HP) now come with Python already installed. If you do need to install Python and aren't confident about the task you can find a few notes on the BeginnersGuide/Download wiki page, but installation is unremarkable on most platforms.
Before getting started, you may want to find out which IDEs and text editors are tailored to make Python editing easy, browse the list of introductory books , or look at code samples that you might find helpful.
There is a list of tutorials suitable for experienced programmers on the BeginnersGuide/Tutorials page. There is also a list of resources in other languages which might be useful if English is not your first language.
The online documentation is your first port of call for definitive information. There is a fairly brief tutorial that gives you basic information about the language and gets you started. You can follow this by looking at the library reference for a full description of Python's many libraries and the language reference for a complete (though somewhat dry) explanation of Python's syntax. If you are looking for common Python recipes and patterns, you can browse the ActiveState Python Cookbook
Looking for Something Specific?
If you want to know whether a particular application, or a library with particular functionality, is available in Python there are a number of possible sources of information. The Python web site provides a Python Package Index (also known as the Cheese Shop , a reference to the Monty Python script of that name). There is also a search page for a number of sources of Python-related information. Failing that, just Google for a phrase including the word ''python'' and you may well get the result you need. If all else fails, ask on the python newsgroup and there's a good chance someone will put you on the right track.
Frequently Asked Questions
If you have a question, it's a good idea to try the FAQ , which answers the most commonly asked questions about Python.
Looking to Help?
If you want to help to develop Python, take a look at the developer area for further information. Please note that you don't have to be an expert programmer to help. The documentation is just as important as the compiler, and still needs plenty of work!
- Comprehensive Learning Paths
- 150+ Hours of Videos
- Complete Access to Jupyter notebooks, Datasets, References.

101 NumPy Exercises for Data Analysis (Python)
- February 26, 2018
- Selva Prabhakaran
The goal of the numpy exercises is to serve as a reference as well as to get you to apply numpy beyond the basics. The questions are of 4 levels of difficulties with L1 being the easiest to L4 being the hardest.
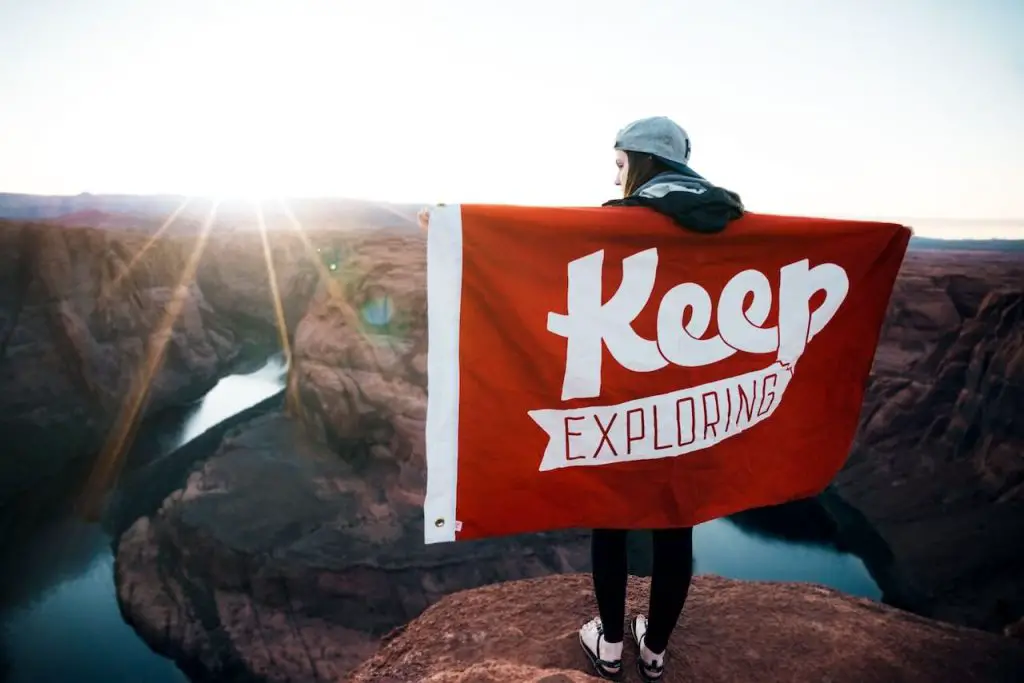
If you want a quick refresher on numpy, the following tutorial is best: Numpy Tutorial Part 1: Introduction Numpy Tutorial Part 2: Advanced numpy tutorials .
Related Post: 101 Practice exercises with pandas .
1. Import numpy as np and see the version
Difficulty Level: L1
Q. Import numpy as np and print the version number.
You must import numpy as np for the rest of the codes in this exercise to work.
To install numpy its recommended to use the installation provided by anaconda.
2. How to create a 1D array?
Q. Create a 1D array of numbers from 0 to 9
Desired output:
3. How to create a boolean array?
Q. Create a 3×3 numpy array of all True’s

4. How to extract items that satisfy a given condition from 1D array?
Q. Extract all odd numbers from arr
5. How to replace items that satisfy a condition with another value in numpy array?
Q. Replace all odd numbers in arr with -1
Desired Output:
6. How to replace items that satisfy a condition without affecting the original array?
Difficulty Level: L2
Q. Replace all odd numbers in arr with -1 without changing arr
7. How to reshape an array?
Q. Convert a 1D array to a 2D array with 2 rows
8. How to stack two arrays vertically?
Q. Stack arrays a and b vertically
9. How to stack two arrays horizontally?
Q. Stack the arrays a and b horizontally.
10. How to generate custom sequences in numpy without hardcoding?
Q. Create the following pattern without hardcoding. Use only numpy functions and the below input array a .
11. How to get the common items between two python numpy arrays?
Q. Get the common items between a and b
12. How to remove from one array those items that exist in another?
Q. From array a remove all items present in array b
13. How to get the positions where elements of two arrays match?
Q. Get the positions where elements of a and b match
14. How to extract all numbers between a given range from a numpy array?
Q. Get all items between 5 and 10 from a .
15. How to make a python function that handles scalars to work on numpy arrays?
Q. Convert the function maxx that works on two scalars, to work on two arrays.
16. How to swap two columns in a 2d numpy array?
Q. Swap columns 1 and 2 in the array arr .
17. How to swap two rows in a 2d numpy array?
Q. Swap rows 1 and 2 in the array arr :
18. How to reverse the rows of a 2D array?
Q. Reverse the rows of a 2D array arr .
19. How to reverse the columns of a 2D array?
Q. Reverse the columns of a 2D array arr .
20. How to create a 2D array containing random floats between 5 and 10?
Q. Create a 2D array of shape 5x3 to contain random decimal numbers between 5 and 10.
21. How to print only 3 decimal places in python numpy array?
Q. Print or show only 3 decimal places of the numpy array rand_arr .
22. How to pretty print a numpy array by suppressing the scientific notation (like 1e10)?
Q. Pretty print rand_arr by suppressing the scientific notation (like 1e10)
23. How to limit the number of items printed in output of numpy array?
Q. Limit the number of items printed in python numpy array a to a maximum of 6 elements.
24. How to print the full numpy array without truncating
Q. Print the full numpy array a without truncating.
25. How to import a dataset with numbers and texts keeping the text intact in python numpy?
Q. Import the iris dataset keeping the text intact.
Since we want to retain the species, a text field, I have set the dtype to object . Had I set dtype=None , a 1d array of tuples would have been returned.
26. How to extract a particular column from 1D array of tuples?
Q. Extract the text column species from the 1D iris imported in previous question.
27. How to convert a 1d array of tuples to a 2d numpy array?
Q. Convert the 1D iris to 2D array iris_2d by omitting the species text field.
28. How to compute the mean, median, standard deviation of a numpy array?
Difficulty: L1
Q. Find the mean, median, standard deviation of iris's sepallength (1st column)
29. How to normalize an array so the values range exactly between 0 and 1?
Difficulty: L2
Q. Create a normalized form of iris 's sepallength whose values range exactly between 0 and 1 so that the minimum has value 0 and maximum has value 1.
30. How to compute the softmax score?
Difficulty Level: L3
Q. Compute the softmax score of sepallength .
31. How to find the percentile scores of a numpy array?
Q. Find the 5th and 95th percentile of iris's sepallength
32. How to insert values at random positions in an array?
Q. Insert np.nan values at 20 random positions in iris_2d dataset
33. How to find the position of missing values in numpy array?
Q. Find the number and position of missing values in iris_2d 's sepallength (1st column)
34. How to filter a numpy array based on two or more conditions?
Q. Filter the rows of iris_2d that has petallength (3rd column) > 1.5 and sepallength (1st column) < 5.0
35. How to drop rows that contain a missing value from a numpy array?
Difficulty Level: L3:
Q. Select the rows of iris_2d that does not have any nan value.
36. How to find the correlation between two columns of a numpy array?
Q. Find the correlation between SepalLength(1st column) and PetalLength(3rd column) in iris_2d
37. How to find if a given array has any null values?
Q. Find out if iris_2d has any missing values.
38. How to replace all missing values with 0 in a numpy array?
Q. Replace all ccurrences of nan with 0 in numpy array
39. How to find the count of unique values in a numpy array?
Q. Find the unique values and the count of unique values in iris's species
40. How to convert a numeric to a categorical (text) array?
Q. Bin the petal length (3rd) column of iris_2d to form a text array, such that if petal length is:
- Less than 3 --> 'small'
- 3-5 --> 'medium'
- '>=5 --> 'large'
41. How to create a new column from existing columns of a numpy array?
Q. Create a new column for volume in iris_2d, where volume is (pi x petallength x sepal_length^2)/3
42. How to do probabilistic sampling in numpy?
Q. Randomly sample iris 's species such that setose is twice the number of versicolor and virginica
Approach 2 is preferred because it creates an index variable that can be used to sample 2d tabular data.
43. How to get the second largest value of an array when grouped by another array?
Q. What is the value of second longest petallength of species setosa
44. How to sort a 2D array by a column
Q. Sort the iris dataset based on sepallength column.
45. How to find the most frequent value in a numpy array?
Q. Find the most frequent value of petal length (3rd column) in iris dataset.
46. How to find the position of the first occurrence of a value greater than a given value?
Q. Find the position of the first occurrence of a value greater than 1.0 in petalwidth 4th column of iris dataset.
47. How to replace all values greater than a given value to a given cutoff?
Q. From the array a , replace all values greater than 30 to 30 and less than 10 to 10.
48. How to get the positions of top n values from a numpy array?
Q. Get the positions of top 5 maximum values in a given array a .
49. How to compute the row wise counts of all possible values in an array?
Difficulty Level: L4
Q. Compute the counts of unique values row-wise.
Output contains 10 columns representing numbers from 1 to 10. The values are the counts of the numbers in the respective rows. For example, Cell(0,2) has the value 2, which means, the number 3 occurs exactly 2 times in the 1st row.
50. How to convert an array of arrays into a flat 1d array?
Difficulty Level: 2
Q. Convert array_of_arrays into a flat linear 1d array.
51. How to generate one-hot encodings for an array in numpy?
Difficulty Level L4
Q. Compute the one-hot encodings (dummy binary variables for each unique value in the array)
52. How to create row numbers grouped by a categorical variable?
Q. Create row numbers grouped by a categorical variable. Use the following sample from iris species as input.
53. How to create groud ids based on a given categorical variable?
Q. Create group ids based on a given categorical variable. Use the following sample from iris species as input.
54. How to rank items in an array using numpy?
Q. Create the ranks for the given numeric array a .
55. How to rank items in a multidimensional array using numpy?
Q. Create a rank array of the same shape as a given numeric array a .
56. How to find the maximum value in each row of a numpy array 2d?
DifficultyLevel: L2
Q. Compute the maximum for each row in the given array.
57. How to compute the min-by-max for each row for a numpy array 2d?
DifficultyLevel: L3
Q. Compute the min-by-max for each row for given 2d numpy array.
58. How to find the duplicate records in a numpy array?
Q. Find the duplicate entries (2nd occurrence onwards) in the given numpy array and mark them as True . First time occurrences should be False .
59. How to find the grouped mean in numpy?
Difficulty Level L3
Q. Find the mean of a numeric column grouped by a categorical column in a 2D numpy array
Desired Solution:
60. How to convert a PIL image to numpy array?
Q. Import the image from the following URL and convert it to a numpy array.
URL = 'https://upload.wikimedia.org/wikipedia/commons/8/8b/Denali_Mt_McKinley.jpg'
61. How to drop all missing values from a numpy array?
Q. Drop all nan values from a 1D numpy array
np.array([1,2,3,np.nan,5,6,7,np.nan])
62. How to compute the euclidean distance between two arrays?
Q. Compute the euclidean distance between two arrays a and b .
63. How to find all the local maxima (or peaks) in a 1d array?
Q. Find all the peaks in a 1D numpy array a . Peaks are points surrounded by smaller values on both sides.
a = np.array([1, 3, 7, 1, 2, 6, 0, 1])
where, 2 and 5 are the positions of peak values 7 and 6.
64. How to subtract a 1d array from a 2d array, where each item of 1d array subtracts from respective row?
Q. Subtract the 1d array b_1d from the 2d array a_2d , such that each item of b_1d subtracts from respective row of a_2d .
65. How to find the index of n'th repetition of an item in an array
Difficulty Level L2
Q. Find the index of 5th repetition of number 1 in x .
66. How to convert numpy's datetime64 object to datetime's datetime object?
Q. Convert numpy's datetime64 object to datetime's datetime object
67. How to compute the moving average of a numpy array?
Q. Compute the moving average of window size 3, for the given 1D array.
68. How to create a numpy array sequence given only the starting point, length and the step?
Q. Create a numpy array of length 10, starting from 5 and has a step of 3 between consecutive numbers
69. How to fill in missing dates in an irregular series of numpy dates?
Q. Given an array of a non-continuous sequence of dates. Make it a continuous sequence of dates, by filling in the missing dates.
70. How to create strides from a given 1D array?
Q. From the given 1d array arr , generate a 2d matrix using strides, with a window length of 4 and strides of 2, like [[0,1,2,3], [2,3,4,5], [4,5,6,7]..]
More Articles
How to convert python code to cython (and speed up 100x), how to convert python to cython inside jupyter notebooks, install opencv python – a comprehensive guide to installing “opencv-python”, install pip mac – how to install pip in macos: a comprehensive guide, scrapy vs. beautiful soup: which is better for web scraping, add python to path – how to add python to the path environment variable in windows, similar articles, complete introduction to linear regression in r, how to implement common statistical significance tests and find the p value, logistic regression – a complete tutorial with examples in r.
Subscribe to Machine Learning Plus for high value data science content
© Machinelearningplus. All rights reserved.

Machine Learning A-Z™: Hands-On Python & R In Data Science
Free sample videos:.

Home » Python Basics
Python Basics
In this section, you’ll learn basic Python. If you’re completely new to Python programming, this Python basics section is perfect for you.
After completing the tutorials, you’ll be confident in Python programming and be able to create simple programs in Python.
Section 1. Fundamentals
- Syntax – introduce you to the basic Python programming syntax.
- Variables – explain to you what variables are and how to create concise and meaningful variables.
- Strings – learn about string data and some basic string operations.
- Numbers – introduce to you the commonly-used number types including integers and floating-point numbers.
- Booleans – explain the Boolean data type, falsy and truthy values in Python.
- Constants – show you how to define constants in Python.
- Comments – learn how to make notes in your code.
- Type conversion – learn how to convert a value of one type to another e.g., converting a string to a number.
Section 2. Operators
- Comparison operators – introduce you to the comparison operators and how to use them to compare two values.
- Logical operators – show you how to use logical operators to combine multiple conditions.
Section 3. Control flow
- if…else statement – learn how to execute a code block based on a condition.
- Ternary operator – introduce you to the Python ternary operator that makes your code more concise.
- for loop with range() – show you how to execute a code block for a fixed number of times by using the for loop with range() function.
- while – show you how to execute a code block as long as a condition is True.
- break – learn how to exit a loop prematurely.
- continue – show you how to skip the current loop iteration and start the next one.
- pass – show you how to use the pass statement as a placeholder.
Section 4. Functions
- Python functions – introduce you to functions in Python, and how to define functions, and reuse them in the program.
- Default parameters – show you how to specify the default values for function parameters.
- Keyword arguments – learn how to use the keyword arguments to make the function call more obvious.
- Recursive functions – learn how to define recursive functions in Python.
- Lambda Expressions – show you how to define anonymous functions in Python using lambda expressions.
- Docstrings – show you how to use docstrings to document a function.
Section 5. Lists
- List – introduce you to the list type and how to manipulate list elements effectively.
- Tuple – introduce you to the tuple which is a list that doesn’t change throughout the program.
- Sort a list in place – show you how to use the sort() method to sort a list in place.
- Sort a List – learn how to use the sorted() function to return a new sorted list from the original list.
- Slice a List – show you how to use the list slicing technique to manipulate lists effectively.
- Unpack a list – show you how to assign list elements to multiple variables using list unpacking.
- Iterate over a List – learn how to use a for loop to iterate over a list.
- Find the index of an element – show you how to find the index of the first occurrence of an element in a list.
- Iterables – explain to you iterables, and the difference between an iterable and an iterator.
- Transform list elements with map() – show you how to use the map() function to transform list elements.
- Filter list elements with filter() – use the filter() function to filter list elements.
- Reduce list elements into a value with reduce() – use the reduce() function to reduce list elements into a single value.
- List comprehensions – show you how to create a new list based on an existing list.
Section 6. Dictionaries
- Dictionary – introduce you to the dictionary type.
- Dictionary comprehension – show you how to use dictionary comprehension to create a new dictionary from an existing one.
Section 7. Sets
- Set – explain to you the Set type and show you how to manipulate set elements effectively.
- Set comprehension – explain to you the set comprehension so that you can create a new set based on an existing set with a more concise and elegant syntax.
- Union of Sets – show you how to union two or more sets using the union() method or set union operator ( | ).
- Intersection of Sets – show you how to intersect two or more sets using the intersection() method or set intersection operator ( & ).
- Difference of sets – learn how to find the difference between sets using the set difference() method or set difference operator ( - )
- Symmetric Difference of sets – guide you on how to find the symmetric difference of sets using the symmetric_difference() method or the symmetric difference operator ( ^ ).
- Subset – check if a set is a subset of another set.
- Superset – check if a set is a superset of another set.
- Disjoint sets – check if two sets are disjoint.
Section 8. Exception handling
- try…except – show you how to handle exceptions more gracefully using the try…except statement.
- try…except…finally – learn how to execute a code block whether an exception occurs or not.
- try…except…else – explain to you how to use the try…except…else statement to control the follow of the program in case of exceptions.
Section 9. More on Python Loops
- for…else – explain to you the for else statement.
- while…else – discuss the while else statement.
- do…while loop emulation – show you how to emulate the do…while loop in Python by using the while loop statement.
Section 10. More on Python functions
- Unpacking tuples – show you how to unpack a tuple that assigns individual elements of a tuple to multiple variables.
- *args Parameters – learn how to pass a variable number of arguments to a function.
- **kwargs Parameters – show you how to pass a variable number of keyword arguments to a function.
- Partial functions – learn how to define partial functions.
- Type hints – show you how to add type hints to the parameters of a function and how to use the static type checker (mypy) to check the type statically.
Section 11. Modules & Packages
- Modules – introduce you to the Python modules and show you how to write your own modules.
- Module search path – explain to you how the Python module search path works when you import a module.
- __name__ variable – show you how to use the __name__ variable to control the execution of a Python file as a script or as a module.
- Packages – learn how to use packages to organize modules in more structured ways.
Section 12. Working with files
- Read from a text file – learn how to read from a text file.
- Write to a text file – show you how to write to a text file.
- Create a new text file – walk you through the steps of creating a new text file.
- Check if a file exists – show you how to check if a file exists.
- Read CSV files – show you how to read data from a CSV file using the csv module.
- Write CSV files – learn how to write data to a CSV file using the csv module.
- Rename a file – guide you on how to rename a file.
- Delete a file – show you how to delete a file.
Section 13. Working Directories
- Working with directories – show you commonly used functions to work with directories.
- List files in a Directory – list files in a directory.
Section 14. Third-party Packages, PIP, and Virtual Environments
- Python Package Index (PyPI) and pip – introduce you to the Python package index and how to install third-party packages using pip.
- Virtual Environments – understand Python virtual environments and more importantly, why you need them.
- Install pipenv on Windows – show you how to install the pipenv tool on Windows.
Section 15. Strings
- F-strings – learn how to use the f-strings to format text strings in a clear syntax.
- Raw strings – use raw strings to handle strings that contain the backslashes.
- Backslash – explain how Python uses the backslashes ( \ ) in string literals.
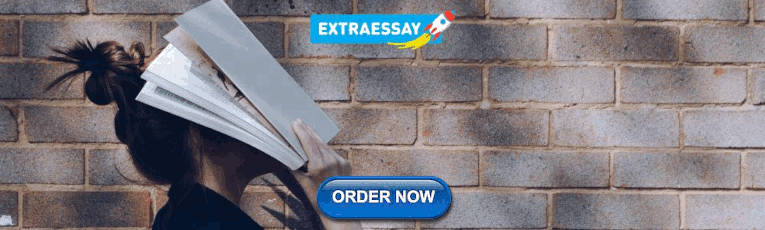
IMAGES
VIDEO
COMMENTS
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
Python Exercises, Practice, Solution: Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java.
Python Practice Problem 1: Average Expenses for Each Semester. John has a list of his monthly expenses from last year: He wants to know his average expenses for each semester. Using a for loop, calculate John's average expenses for the first semester (January to June) and the second semester (July to December).
Beginner Python exercises. Home; Why Practice Python? Why Chilis? Resources for learners; All Exercises. 1: Character Input 2: Odd Or Even 3: List Less Than Ten 4: Divisors 5: List Overlap 6: String Lists 7: List Comprehensions 8: Rock Paper Scissors 9: Guessing Game One 10: List Overlap Comprehensions 11: Check Primality Functions 12: List Ends 13: Fibonacci 14: List Remove Duplicates
In this article, we give you 10 Python practice exercises to boost your skills. Practice exercises are a great way to learn Python. Well-designed exercises expose you to new concepts, such as writing different types of loops, working with different data structures like lists, arrays, and tuples, and reading in different file types.
The best way to learn is by practising it more and more. The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains.
Also, try to solve Python list Exercise. Exercise 7: Return the count of a given substring from a string. Write a program to find how many times substring "Emma" appears in the given string. Given: str_x = "Emma is good developer. Emma is a writer" Code language: Python (python) Expected Output: Emma appeared 2 times
Python Tutorials → In-depth articles and video courses Learning Paths → Guided study plans for accelerated learning Quizzes → Check your learning progress Browse Topics → Focus on a specific area or skill level Community Chat → Learn with other Pythonistas Office Hours → Live Q&A calls with Python experts Podcast → Hear what's new in the world of Python Books →
Mastering Python takes much longer, but you don't need to become a master to get things done! Read more about how long it takes to learn Python. About the author. Charlie Custer. Charlie is a student of data science, and also a content marketer at Dataquest. In his free time, he's learning to mountain bike and making videos about it.
Python Exercises. Learn by doing. Exercises will help you to understand the topic deeply. Exercise for each tutorial topic so you can practice and improve your Python skills. Exercises cover Python basics to data structures and other advanced topics. Each Exercise contains ten questions to solve. Practice each Exercise using Code Editor.
Exercise 1: print () function | (3) : Get your feet wet and have some fun with the universal first step of programming. Exercise 2: Variables | (2) : Practice assigning data to variables with these Python exercises. Exercise 3: Data Types | (4) : Integer (int), string (str) and float are the most basic and fundamental building blocks of data in ...
Welcome to the LearnPython.org interactive Python tutorial. Whether you are an experienced programmer or not, this website is intended for everyone who wishes to learn the Python programming language. You are welcome to join our group on Facebook for questions, discussions and updates. After you complete the tutorials, you can get certified at ...
This course provides an introduction to programming and the Python language. Students are introduced to core programming concepts like data structures, conditionals, loops, variables, and functions. This course includes an overview of the various tools available for writing and running Python, and gets students coding quickly.
Python is easy to learn. Its syntax is easy and code is very readable. Python has a lot of applications. It's used for developing web applications, data science, rapid application development, and so on. Python allows you to write programs in fewer lines of code than most of the programming languages. The popularity of Python is growing rapidly.
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews.
And particularly in Python. 1. BOOKWORM HUB. Website: BookwormHub.com. BookwormHub is a group of qualified professionals in many scientific areas. The list of their specializations comprises core fields including math, chemistry, biology, statistics, and engineering homework help. However, its primary focus is on the provision of programming ...
Hangman Python Project. In this Kylie Ying tutorial, you will learn how to work with dictionaries, lists, and nested if statements. You will also learn how to work with the string and random Python modules. Countdown Timer Python Project. In this Code With Tomi tutorial, you will learn how to build a countdown timer using the time Python module ...
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
best one to "do my Python homework" individually. 9.1. 🏅 CodingAssignments.com. the biggest number of experts to "do my Python assignment". These are the top Python assignment help websites, according to the research of our quality control experts. Let's look at each of them individually, focusing on a unique benefit offered by ...
Learning. Before getting started, you may want to find out which IDEs and text editors are tailored to make Python editing easy, browse the list of introductory books, or look at code samples that you might find helpful.. There is a list of tutorials suitable for experienced programmers on the BeginnersGuide/Tutorials page. There is also a list of resources in other languages which might be ...
101 Practice exercises with pandas. 1. Import numpy as np and see the version. Difficulty Level: L1. Q. Import numpy as np and print the version number. 2. How to create a 1D array? Difficulty Level: L1. Q. Create a 1D array of numbers from 0 to 9.
Python is a beginner-friendly programming language to learn. You can learn Python's syntax and other fundamentals in a few hours and start writing simple programs. But if you're preparing for interviews—for any role in software engineering or data science—and would like to use Python, you need to know way beyond the basics. ...
Section 3. Control flow. if…else statement - learn how to execute a code block based on a condition.; Ternary operator - introduce you to the Python ternary operator that makes your code more concise.; for loop with range() - show you how to execute a code block for a fixed number of times by using the for loop with range() function. while- show you how to execute a code block as ...
Hello everyone! I am new to python and am working on some homework and have a question about a problem I'm working: "Define a function consolidate_change that takes as arguments counts of different US coins (similar to the previous function) and prints out the simplest number of bills and coins needed to make that amount. ...